/* TA-LIB Copyright (c) 1999-2007, Mario Fortier
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or
* without modification, are permitted provided that the following
* conditions are met:
*
* - Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* - Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in
* the documentation and/or other materials provided with the
* distribution.
*
* - Neither name of author nor the names of its contributors
* may be used to endorse or promote products derived from this
* software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
* FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
* REGENTS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS
* OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE
* OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE,
* EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
#ifndef TA_FUNC_H
#define TA_FUNC_H
#ifndef TA_COMMON_H
#include "ta_common.h"
#endif
/* This header contains the prototype of all the Technical Analysis
* function provided by TA-LIB.
*/
/* TA-LIB Developer Note: Do not modify this file, it is automaticaly
* generated by gen_code.
*/
#ifdef __cplusplus
extern "C" {
#endif
#ifndef TA_DEFS_H
#include "ta_defs.h"
#endif
/*
* TA_ACOS - Vector Trigonometric ACos
*
* Input = double
* Output = double
*
*/
TA_RetCode TA_ACOS( int startIdx,
int endIdx,
const double inReal[],
int *outBegIdx,
int *outNBElement,
double outReal[] );
TA_RetCode TA_S_ACOS( int startIdx,
int endIdx,
const float inReal[],
int *outBegIdx,
int *outNBElement,
double outReal[] );
int TA_ACOS_Lookback( void );
/*
* TA_AD - Chaikin A/D Line
*
* Input = High, Low, Close, Volume
* Output = double
*
*/
TA_RetCode TA_AD( int startIdx,
int endIdx,
const double inHigh[],
const double inLow[],
const double inClose[],
const double inVolume[],
int *outBegIdx,
int *outNBElement,
double outReal[] );
TA_RetCode TA_S_AD( int startIdx,
int endIdx,
const float inHigh[],
const float inLow[],
const float inClose[],
const float inVolume[],
int *outBegIdx,
int *outNBElement,
double outReal[] );
int TA_AD_Lookback( void );
/*
* TA_ADD - Vector Arithmetic Add
*
* Input = double, double
* Output = double
*
*/
TA_RetCode TA_ADD( int startIdx,
int endIdx,
const double inReal0[],
const double inReal1[],
int *outBegIdx,
int *outNBElement,
double outReal[] );
TA_RetCode TA_S_ADD( int startIdx,
int endIdx,
const float inReal0[],
const float inReal1[],
int *outBegIdx,
int *outNBElement,
double outReal[] );
int TA_ADD_Lookback( void );
/*
* TA_ADOSC - Chaikin A/D Oscillator
*
* Input = High, Low, Close, Volume
* Output = double
*
* Optional Parameters
* -------------------
* optInFastPeriod:(From 2 to 100000)
* Number of period for the fast MA
*
* optInSlowPeriod:(From 2 to 100000)
* Number of period for the slow MA
*
*
*/
TA_RetCode TA_ADOSC( int startIdx,
int endIdx,
const double inHigh[],
const double inLow[],
const double inClose[],
const double inVolume[],
int optInFastPeriod, /* From 2 to 100000 */
int optInSlowPeriod, /* From 2 to 100000 */
int *outBegIdx,
int *outNBElement,
double outReal[] );
TA_RetCode TA_S_ADOSC( int startIdx,
int endIdx,
const float inHigh[],
const float inLow[],
const float inClose[],
const float inVolume[],
int optInFastPeriod, /* From 2 to 100000 */
int optInSlowPeriod, /* From 2 to 100000 */
int *outBegIdx,
int *outNBElement,
double outReal[] );
int TA_ADOSC_Lookback( int optInFastPeriod, /* From 2 to 100000 */
int optInSlowPeriod ); /* From 2 to 100000 */
/*
* TA_ADX - Average Directional Movement Index
*
* Input = High, Low, Close
* Output = double
*
* Optional Parameters
* -------------------
* optInTimePeriod:(From 2 to 100000)
* Number of period
*
*
*/
TA_RetCode TA_ADX( int startIdx,
int endIdx,
const double inHigh[],
const double inLow[],
const double inClose[],
int optInTimePeriod, /* From 2 to 100000 */
int *outBegIdx,
int *outNBElement,
double outReal[] );
TA_RetCode TA_S_ADX( int startIdx,
int endIdx,
const float inHigh[],
const float inLow[],
const float inClose[],
int optInTimePeriod, /* From 2 to 100000 */
int *outBegIdx,
int *outNBElement,
double outReal[] );
int TA_ADX_Lookback( int optInTimePeriod ); /* From 2 to 100000 */
/*
* TA_ADXR - Average Directional Movement Index Rating
*
* Input = High, Low, Close
* Output = double
*
* Optional Parameters
* -------------------
* optInTimePeriod:(From 2 to 100000)
* Number of period
*
*
*/
TA_RetCode TA_ADXR( int startIdx,
int endIdx,
const double inHigh[],
const double inLow[],
const double inClose[],
int optInTimePeriod, /* From 2 to 100000 */
int *outBegIdx,
int *outNBElement,
double outReal[] );
TA_RetCode TA_S_ADXR( int startIdx,
int endIdx,
const float inHigh[],
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
解压c库库路径(如我系统为: C:\ta-lib\c\ 下) 具体setup.py代码如下: if sys.platform == "win32": platform_supported = True lib_talib_name = 'ta_libc_cdr' include_dirs = [r"c:\ta-lib\c\include"] lib_talib_dirs = [r"c:\ta-lib\c\lib"] 3. 执行 pip3 install TA-Lib --user
资源详情
资源评论
资源推荐
收起资源包目录

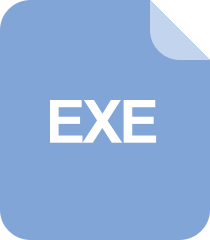

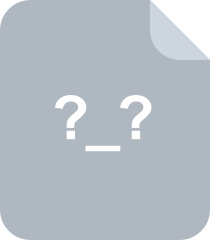
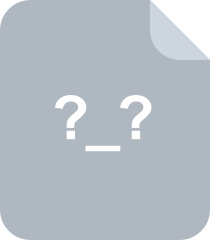
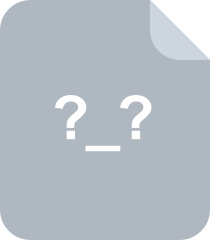
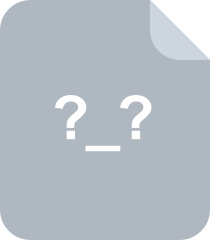
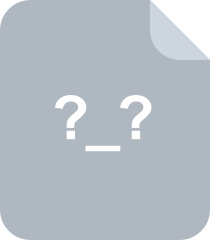
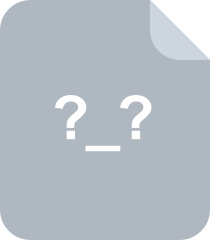

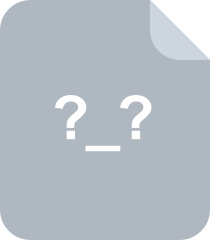
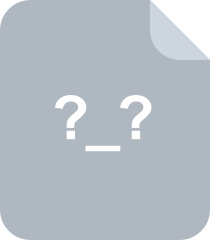
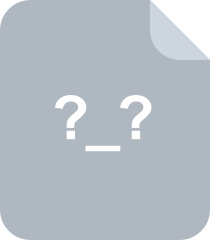
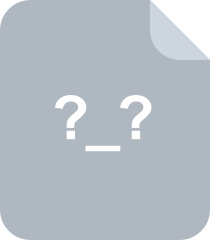
共 11 条
- 1
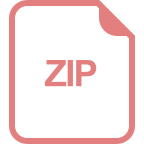
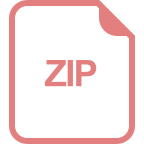
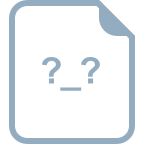
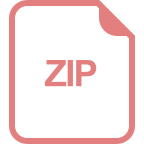
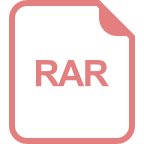
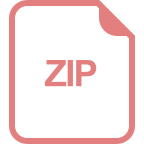
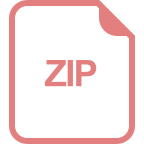
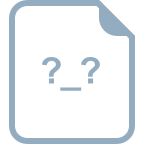
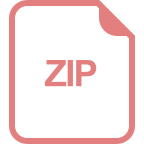
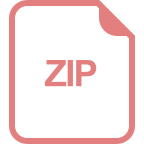
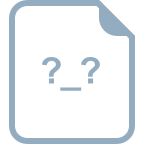
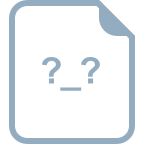
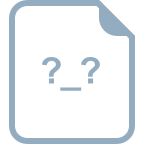
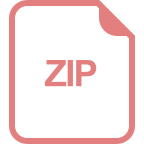
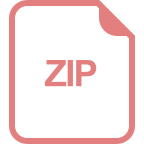
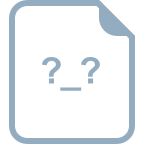
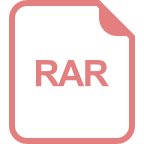
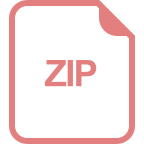
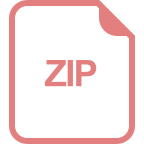
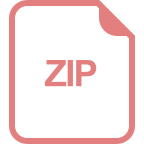
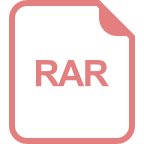
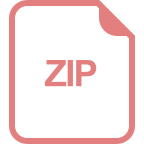

shangmw
- 粉丝: 21
- 资源: 15
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

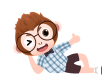
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


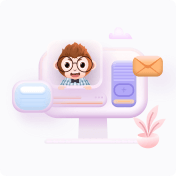
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0