C# winForm 创建水晶风格的按钮
需积分: 12 145 浏览量
2011-03-14
17:09:29
上传
评论
收藏 6KB TXT 举报

是非在浴室里
- 粉丝: 0
- 资源: 3
最新资源
- 珍藏多年的基于matlab实现潮流计算程序源代码集合,包含多个潮流计算程序.rar
- 使用FPGA实现串-并型乘法器
- 基于matlab实现针对基于双曲线定位的DV-Hop算法中误差误差出一种基于加权双曲线定位的DV-Hop改进算法.rar
- 基于matlab实现由遗传算法开发的整数规划,车辆调度问题.rar
- 电视家7.0(对电视配置要求高).apk
- 免费计算机毕业设计-基于JavaEE的医院病历管理系统设计与实现(包含论文+源码)
- 手机端 我的世界融合植物大战僵尸版.apk
- 植物大战僵尸 · 戴夫的老年生活 手机版.apk
- Runcraft · 我的世界跑酷游戏 手机端.apk
- pta题库答案c语言.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


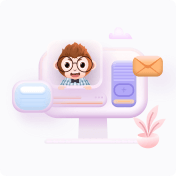