// Copyright 2007-2011 Baptiste Lepilleur and The JsonCpp Authors
// Copyright (C) 2016 InfoTeCS JSC. All rights reserved.
// Distributed under MIT license, or public domain if desired and
// recognized in your jurisdiction.
// See file LICENSE for detail or copy at http://jsoncpp.sourceforge.net/LICENSE
#if !defined(JSON_IS_AMALGAMATION)
#include "json/json_tool.h"
#include "json/assertions.h"
#include "json/reader.h"
#include "json/value.h"
#endif // if !defined(JSON_IS_AMALGAMATION)
#include <algorithm>
#include <cassert>
#include <cmath>
#include <cstring>
#include <iostream>
#include <istream>
#include <limits>
#include <memory>
#include <set>
#include <sstream>
#include <utility>
#include <cstdio>
#if __cplusplus >= 201103L
#if !defined(sscanf)
#define sscanf std::sscanf
#endif
#endif //__cplusplus
#if defined(_MSC_VER)
#if !defined(_CRT_SECURE_CPP_OVERLOAD_STANDARD_NAMES)
#define _CRT_SECURE_CPP_OVERLOAD_STANDARD_NAMES 1
#endif //_CRT_SECURE_CPP_OVERLOAD_STANDARD_NAMES
#endif //_MSC_VER
#if defined(_MSC_VER)
// Disable warning about strdup being deprecated.
#pragma warning(disable : 4996)
#endif
// Define JSONCPP_DEPRECATED_STACK_LIMIT as an appropriate integer at compile
// time to change the stack limit
#if !defined(JSONCPP_DEPRECATED_STACK_LIMIT)
#define JSONCPP_DEPRECATED_STACK_LIMIT 1000
#endif
static size_t const stackLimit_g =
JSONCPP_DEPRECATED_STACK_LIMIT; // see readValue()
namespace Json {
#if __cplusplus >= 201103L || (defined(_CPPLIB_VER) && _CPPLIB_VER >= 520)
using CharReaderPtr = std::unique_ptr<CharReader>;
#else
using CharReaderPtr = std::auto_ptr<CharReader>;
#endif
// Implementation of class Features
// ////////////////////////////////
Features::Features() = default;
Features Features::all() { return {}; }
Features Features::strictMode() {
Features features;
features.allowComments_ = false;
features.strictRoot_ = true;
features.allowDroppedNullPlaceholders_ = false;
features.allowNumericKeys_ = false;
return features;
}
// Implementation of class Reader
// ////////////////////////////////
bool Reader::containsNewLine(Reader::Location begin, Reader::Location end) {
return std::any_of(begin, end, [](char b) { return b == '\n' || b == '\r'; });
}
// Class Reader
// //////////////////////////////////////////////////////////////////
Reader::Reader() : features_(Features::all()) {}
Reader::Reader(const Features& features) : features_(features) {}
bool Reader::parse(const std::string& document, Value& root,
bool collectComments) {
document_.assign(document.begin(), document.end());
const char* begin = document_.c_str();
const char* end = begin + document_.length();
return parse(begin, end, root, collectComments);
}
bool Reader::parse(std::istream& is, Value& root, bool collectComments) {
// std::istream_iterator<char> begin(is);
// std::istream_iterator<char> end;
// Those would allow streamed input from a file, if parse() were a
// template function.
// Since String is reference-counted, this at least does not
// create an extra copy.
String doc(std::istreambuf_iterator<char>(is), {});
return parse(doc.data(), doc.data() + doc.size(), root, collectComments);
}
bool Reader::parse(const char* beginDoc, const char* endDoc, Value& root,
bool collectComments) {
if (!features_.allowComments_) {
collectComments = false;
}
begin_ = beginDoc;
end_ = endDoc;
collectComments_ = collectComments;
current_ = begin_;
lastValueEnd_ = nullptr;
lastValue_ = nullptr;
commentsBefore_.clear();
errors_.clear();
while (!nodes_.empty())
nodes_.pop();
nodes_.push(&root);
bool successful = readValue();
Token token;
skipCommentTokens(token);
if (collectComments_ && !commentsBefore_.empty())
root.setComment(commentsBefore_, commentAfter);
if (features_.strictRoot_) {
if (!root.isArray() && !root.isObject()) {
// Set error location to start of doc, ideally should be first token found
// in doc
token.type_ = tokenError;
token.start_ = beginDoc;
token.end_ = endDoc;
addError(
"A valid JSON document must be either an array or an object value.",
token);
return false;
}
}
return successful;
}
bool Reader::readValue() {
// readValue() may call itself only if it calls readObject() or ReadArray().
// These methods execute nodes_.push() just before and nodes_.pop)() just
// after calling readValue(). parse() executes one nodes_.push(), so > instead
// of >=.
if (nodes_.size() > stackLimit_g)
throwRuntimeError("Exceeded stackLimit in readValue().");
Token token;
skipCommentTokens(token);
bool successful = true;
if (collectComments_ && !commentsBefore_.empty()) {
currentValue().setComment(commentsBefore_, commentBefore);
commentsBefore_.clear();
}
switch (token.type_) {
case tokenObjectBegin:
successful = readObject(token);
currentValue().setOffsetLimit(current_ - begin_);
break;
case tokenArrayBegin:
successful = readArray(token);
currentValue().setOffsetLimit(current_ - begin_);
break;
case tokenNumber:
successful = decodeNumber(token);
break;
case tokenString:
successful = decodeString(token);
break;
case tokenTrue: {
Value v(true);
currentValue().swapPayload(v);
currentValue().setOffsetStart(token.start_ - begin_);
currentValue().setOffsetLimit(token.end_ - begin_);
} break;
case tokenFalse: {
Value v(false);
currentValue().swapPayload(v);
currentValue().setOffsetStart(token.start_ - begin_);
currentValue().setOffsetLimit(token.end_ - begin_);
} break;
case tokenNull: {
Value v;
currentValue().swapPayload(v);
currentValue().setOffsetStart(token.start_ - begin_);
currentValue().setOffsetLimit(token.end_ - begin_);
} break;
case tokenArraySeparator:
case tokenObjectEnd:
case tokenArrayEnd:
if (features_.allowDroppedNullPlaceholders_) {
// "Un-read" the current token and mark the current value as a null
// token.
current_--;
Value v;
currentValue().swapPayload(v);
currentValue().setOffsetStart(current_ - begin_ - 1);
currentValue().setOffsetLimit(current_ - begin_);
break;
} // Else, fall through...
default:
currentValue().setOffsetStart(token.start_ - begin_);
currentValue().setOffsetLimit(token.end_ - begin_);
return addError("Syntax error: value, object or array expected.", token);
}
if (collectComments_) {
lastValueEnd_ = current_;
lastValue_ = ¤tValue();
}
return successful;
}
void Reader::skipCommentTokens(Token& token) {
if (features_.allowComments_) {
do {
readToken(token);
} while (token.type_ == tokenComment);
} else {
readToken(token);
}
}
bool Reader::readToken(Token& token) {
skipSpaces();
token.start_ = current_;
Char c = getNextChar();
bool ok = true;
switch (c) {
case '{':
token.type_ = tokenObjectBegin;
break;
case '}':
token.type_ = tokenObjectEnd;
break;
case '[':
token.type_ = tokenArrayBegin;
break;
case ']':
token.type_ = tokenArrayEnd;
break;
case '"':
token.type_ = tokenString;
ok = readString();
break;
case '/':
token.type_ = tokenComment;
ok = readComment();
break;
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9':
case '-':
token.type_ = tokenNumber;
readNumber();
break;
case 't':
token.type_ = tokenTrue;
ok = match("rue", 3);
break;
case 'f':
token.type_ = tokenFalse;
ok = match("alse", 4);
break;
case 'n':
token.type_ = tokenNull;
ok = match("ull", 3);
break;
case ',':
token.type_ = tokenArraySeparator;
break;
case ':':
token.type_ = tokenMemberSeparator;
break;
case 0:
token.type_ = tokenEndOfStream;
break;
default:
MinGW64是一个针对Windows平台的GCC(GNU Compiler Collection)移植版,提供了64位的编译环境。在本文中,我们将深入探讨如何使用MinGW64编译JsonCpp库,一个广泛使用的C++库,用于处理JSON(JavaScript Object Notation)数据格式。JsonCpp不仅支持读写JSON数据,还能在C++程序中方便地表示和操作这些数据。 JsonCpp库分为动态链接库(.dll)和静态链接库(.lib)两种形式。动态链接库在运行时需要对应的.dll文件,而静态链接库则将JsonCpp的功能直接集成到你的可执行文件中。这两种方式各有优缺点:动态链接库可以减少应用程序的大小,但需要确保系统路径中有相应的.dll文件;静态链接库则避免了依赖问题,但可能导致最终程序体积增大。 在MinGW64环境下编译JsonCpp,首先需要安装MinGW64,并配置好环境变量,确保g++编译器可以在命令行中使用。接着,你需要获取JsonCpp的源码和头文件,这些通常包含在.zip或.tar.gz格式的压缩包中。在这个特定的案例中,压缩包已经包含了所需的文件,包括Makefile,这是一个用于自动化编译过程的脚本。 要编译JsonCpp的动态链接库,你可以打开命令行窗口,导航到解压后的JsonCpp源码目录,然后执行`make`命令。Makefile通常会定义一系列规则,指示编译器如何处理源代码,生成目标文件,最终链接成动态库。例如,它可能包含以下步骤: 1. 预处理(预处理器将宏展开,处理条件编译指令等)。 2. 编译(编译器将预处理后的.c或.cpp文件转化为汇编代码)。 3. 汇编(汇编器将汇编代码转化为机器代码)。 4. 链接(链接器将多个目标文件合并为单一的可执行文件或库,同时处理符号引用)。 在生成动态链接库的过程中,Makefile会指定`-shared`选项,告诉g++创建一个动态库。此外,还需要指定库的输出名称和依赖的库(如`-lstdc++`),以及可能的其他编译器选项。 编译静态链接库的过程类似,但在Makefile中需要指定`-static`选项。这将告诉g++生成一个静态库文件,其扩展名为`.a`。使用静态库时,JsonCpp的代码会直接集成到你的程序中,不需要运行时加载额外的库文件。 在编译完成后,你会在指定的输出目录下找到编译好的动态库(如`libjsoncpp.dll`和`libjsoncpp.dll.a`)和静态库(如`libjsoncpp.a`)。动态库通常用于开发阶段,因为它们允许快速调试和更新,而静态库则适用于部署到不希望依赖外部库的环境中。 使用MinGW64编译JsonCpp库涉及设置编译环境、准备源码、编写或使用已有的Makefile,以及执行编译命令。通过动态或静态链接,你可以根据项目需求灵活选择最适合的库类型。理解这个过程有助于你在其他C++项目中更有效地管理依赖库。























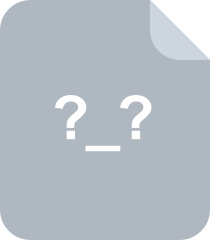
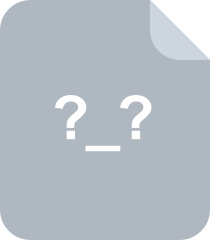
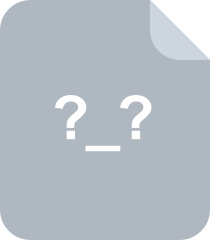
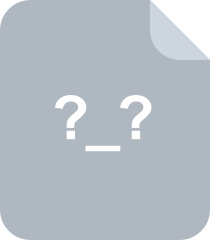
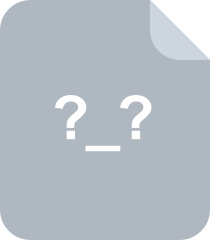

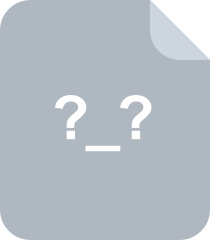
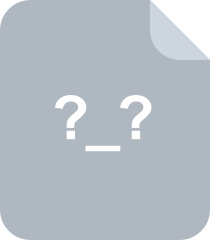
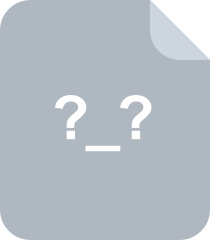
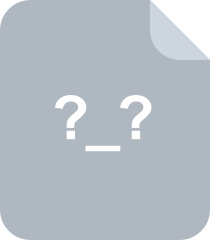
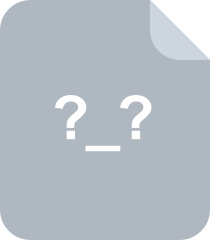
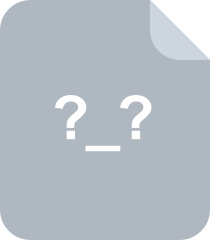
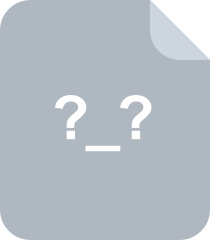
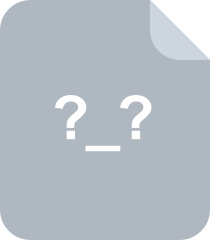
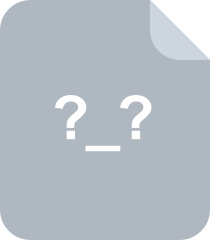
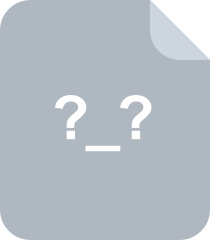
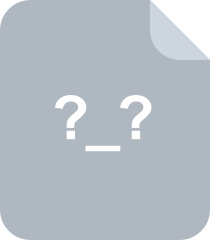
- 1
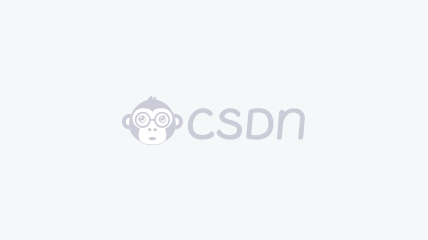
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整

- 粉丝: 6
- 资源: 10
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

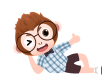
最新资源
- WAVGATvcu控制器应用层软件策略详解:基于AUTOSAR架构的量产车型整车控制器策略开发介绍,WAVGATvcu控制器应用层软件策略详解:基于AUTOSAR架构的量产车型整车控制器策略开发介绍
- 基于Matlab实现报童问题仿真(源码+详细注释).rar
- javaweb校园二手交易系统v3-lw.zip
- 卷积神经网络CNN的多特征输入单输出二分类与多分类模型matlab程序注释详解及可视化效果展示,卷积神经网络CNN的多特征输入单输出二分类与多分类模型matlab实现,附详细注释及可视化效果展示,利用
- JAVAWEB校园订餐系统项目源码.zip
- javaweb小区停车位管理系统-lw.zip
- 免疫优化算法在选址应用中的探索:最佳物流中心选址方案与适应度函数可视化,免疫优化算法在选址决策中的应用:迭代寻优与物流中心选址方案输出,免疫优化算法选址 1、免疫算法是一种具有生成+检测 (gener
- WPF 上使用Open AI API
- 适用于S32K144的UDS规范Bootloader,独立Flash驱动文件,适用于量产项目快速部署,适用于S32K144的UDS规范Bootloader,独立Flash驱动文件,适用于量产项目快速部
- SONYEQ6标定数据放慢速度克利夫兰撒大了
- 25T1_HW1-Questions.pdf
- Matlab在综合能源系统优化调度中的应用研究:涵盖两阶段鲁棒、复杂鲁棒优化及主从博弈等多领域策略考量,Matlab在综合能源系统优化调度中的应用研究:涵盖两阶段鲁棒、复杂鲁棒优化、主从博弈及阶梯型碳
- 电力系统拓扑识别:线变、户变及相位关系的仿真数据生成与算法研究,电力系统拓扑识别:线变、户变及相位关系的仿真数据批量处理与算法研究,电力系统拓扑识别相关的内容:线变关系,户变关系和相位关系识别 既编
- 几分钟Java程序员快速上手Kotlin
- 新亚空调自控程序 冻干车间空调自控系统 HMI屏幕 博途V16项目
- CNN-GRU多变量回归预测模型(Matlab实现)基于CNN与GRU的联合应用进行多维数据拟合与预测,CNN-GRU多变量回归预测模型(Matlab实现)用于多维数据拟合与预测,CNN-GRU多变量

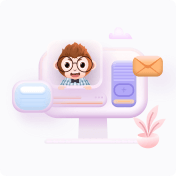
