### 遗传算法C++实现详解 #### 一、遗传算法概述 遗传算法(Genetic Algorithm, GA)是一种借鉴生物进化过程中的自然选择和自然遗传机制的随机搜索方法,用于解决优化问题和搜索问题。它模拟了生物进化过程中染色体通过交叉变异等操作逐步进化的过程。 #### 二、遗传算法的基本流程 1. **初始化种群**:生成初始解集。 2. **适应度评估**:计算每个个体的适应度值。 3. **选择**:根据适应度值选择个体进行繁殖。 4. **交叉**:通过交叉操作产生新的后代。 5. **变异**:对新后代进行变异操作增加种群多样性。 6. **终止条件判断**:如果满足终止条件则结束算法;否则返回第二步继续迭代。 #### 三、C++实现遗传算法的关键步骤 本节将结合题目给出的部分代码详细解析遗传算法在C++中的具体实现过程。 ##### 1. 数据结构定义 - `struct City`: 定义城市结构体,包含城市的坐标信息。 - `float X`: 城市的X坐标。 - `float Y`: 城市的Y坐标。 - 其他数据类型定义: - `#define NumCity 30`: 城市数量。 - `#define ChrLen NumCity`: 染色体长度等于城市数量。 - `#define PopSize 2*NumCity`: 种群大小。 - `#define ProbilityVariation 0.2`: 变异概率。 - `#define ProbilityCross 0.9`: 交叉概率。 - `#define NumIteration 1000`: 最大迭代次数。 ##### 2. 函数实现 - **读取数据**: `void readData(char* FILENAME, City* city);` 从文件中读取城市坐标数据。 - **写入最优路径**: `void writeData(int OptChr[ChrLen], City city[NumCity], float RunningTime);` 将最优路径及运行时间写入文件。 - **生成随机数**: `void genRandom(int* a);` 生成不重复的随机整数。 - **初始化种群**: `void init(int Pop[PopSize][ChrLen]);` 初始化种群。 - **计算距离**: `float calcDistance(City city1, City city2);` 计算两个城市之间的欧氏距离。 - **计算总距离**: `float calcTotalDistance(int Chr[ChrLen], City city[NumCity]);` 计算一条路径上的总距离。 - **计算适应度**: `void calcFitness(int Pop[PopSize][ChrLen], City city[NumCity], float Fitness[PopSize]);` 计算种群中每个个体的适应度。 - **计算评估值**: `void calcEval(float Fitness[PopSize], float Eval[PopSize]);` 计算评估值,为后续的选择操作做准备。 - **选择**: `void selcet(int Pop[][ChrLen], float Eval[PopSize], int NewPop[][ChrLen]);` 根据评估值选择下一代个体。 - **交叉**: `void crossoverPop(int OldPop[][ChrLen], int NewPop[][ChrLen]);` 对种群执行交叉操作。 - **变异**: `void varyPop(int OldPop[][ChrLen], int NewPop[][ChrLen]);` 对种群执行变异操作。 - **复制染色体**: `void copyChr(int OldChr[ChrLen], int NewChr[ChrLen]);` 复制染色体。 - **复制种群**: `void copyPop(int OldPop[PopSize][ChrLen], int NewPop[PopSize][ChrLen]);` 复制整个种群。 - **打印结果**: `void printResult(int Pop[PopSize][ChrLen], City city[NumCity], int OptChr[ChrLen]);` 打印最终的最优路径和相关统计信息。 ##### 3. 主函数逻辑 - **初始化**: 读取数据并初始化种群。 - **迭代过程**: - 计算适应度。 - 选择操作。 - 交叉与变异。 - 更新最优解。 - **终止条件**: 达到最大迭代次数后结束程序。 #### 四、遗传算法的特点及应用 遗传算法具有全局搜索能力和较好的鲁棒性,适用于解决组合优化问题,如旅行商问题(TSP)、调度问题等。此外,遗传算法还可应用于机器学习中的参数优化、神经网络训练等多个领域。 #### 五、总结 本篇详细介绍了遗传算法的基础概念及其在C++中的具体实现过程。通过对代码的深入分析,读者可以更好地理解遗传算法的工作原理,并能够将其应用于实际问题中。遗传算法作为一种强大的搜索工具,在解决复杂优化问题时展现出其独特的优势,是现代优化技术中不可或缺的一部分。
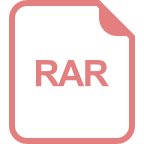
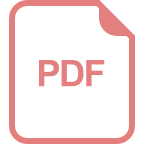
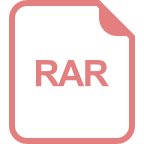
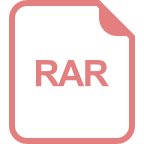
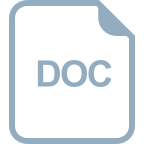
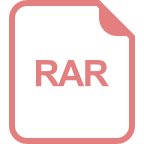
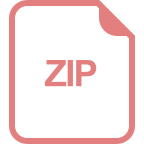
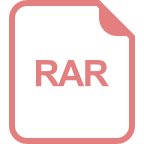
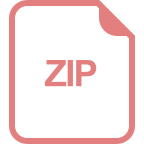
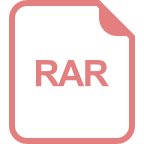
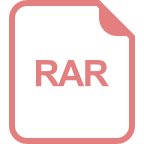
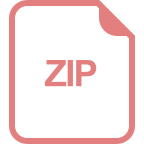
#include <stdio.h>
#include <stdlib.h>
#include <iostream>
#include <fstream>
#include <string>
#include <iomanip>
#include <math.h>
#include <time.h>
using namespace std;
#define NumCity 30
#define ChrLen NumCity //chromosome length
#define PopSize 2*NumCity
#define ProbilityVariation 0.2
#define ProbilityCross 0.9
#define NumIteration 1000
struct City
{
float X;
float Y;
};
void readData(char *FILENAME, City * city);//read city data from "data.txt"
void writeData(int OptChr[ChrLen], City city[NumCity], float RunningTime);
void genRandom(int * a);//generate random which are int type and not replicated
void init(int Pop[PopSize][ChrLen]);
float calcTotalDistance(int Chr[ChrLen], City city[NumCity]);
void calcFitness(int Pop[PopSize][ChrLen], City city[NumCity], float Fitness[PopSize]);
void calcEval(float Fitness[PopSize], float Eval[PopSize]);
void selcet(int Pop[][ChrLen], float Eval[PopSize], int NewPop[][ChrLen]);
void crossoverChr(int father1[ChrLen], int father2[ChrLen], int son1[ChrLen], int son2[ChrLen]);
void crossoverPop(int OldPop[][ChrLen], int NewPop[][ChrLen]);
void varyPop(int OldPop[][ChrLen], int NewPop[][ChrLen]);
void varyChr(int Old[ChrLen], int New[ChrLen]);
void copyChr(int OldChr[ChrLen], int NewChr[ChrLen]);
void copyPop(int OldPop[PopSize][ChrLen], int NewPop[PopSize][ChrLen]);
void printResult(int Pop[PopSize][ChrLen], City city[NumCity], int OptChr[ChrLen]);
void main()
{
char* FILENAME="data.txt";
City city[NumCity];
int Pop[PopSize][ChrLen];
int NewPop[PopSize][ChrLen], CrossPop[PopSize][ChrLen], MutPop[PopSize][ChrLen];
int OptChr[ChrLen];
float Eval[PopSize],Fitness[PopSize];
int CountIteration, i, j;
clock_t StartTime, FinishTime;
float RunningTime;
readData(FILENAME, city);
StartTime = clock();
剩余14页未读,继续阅读
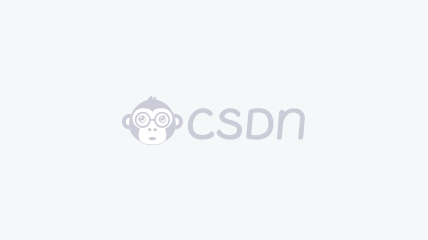


- 粉丝: 1
- 资源: 3
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

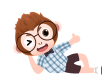
最新资源
- Wkhtmltopdf python 包装器将 html 转换为 pdf.zip
- Windows 事件日志文件 (.evtx) 的纯 Python 解析器.zip
- jQuery实现一个加购物车飞入动画
- bootstrap企业网站前端模板下载
- 矩阵作业-包含Eigen安装相关内容
- CSS3几何透明层文本悬停变色特效代码.zip
- CSS3实现的九宫格图片鼠标悬停去除遮罩层特效源码.zip
- MQTT协议的原理、特点、工作流程及应用场景
- Ruby语言教程从介绍入门到精通详教程跟代码.zip
- PM2.5-Prediction-Based-on-Random-Forest-Algorithm-master.zip

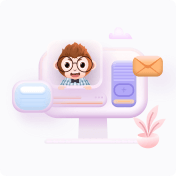
