<?php
/**
*
* FusionCharts Exporter is a PHP script that handles
* FusionCharts (since v3.1) Server Side Export feature.
* This in conjuncture with other resource PHP scripts would
* process FusionCharts Export Data POSTED to it from FusionCharts
* and convert the data to image or PDF and subsequently save to the
* server or response back as http response to client side as download.
*
* This script might be called as the FusionCharts Exporter - main module
*
* @author FusionCharts
* @description FusionCharts Exporter (Server-Side - PHP)
* @version 2.0 [ 12 February 2009 ]
*
*/
/**
* ChangeLog / Version History:
* ----------------------------
*
* 2.0 [ 12 February 2009 ]
* - Integrated with new Export feature of FusionCharts 3.1
* - can save to server side directory
* - can provide download or open in popupr window
* - can report back to chart
* - can save as PDF/JPG/PNG/GIF
*
* 1.0 [ 16 August 2007 ]
* - can process chart data to jpg image and response back to client side as download.
*
*/
/**
* Copyright (c) 2009 InfoSoft Global Private Limited. All Rights Reserved.
*
*/
/**
* GENERAL NOTES
* -------------
*
* Chart would POST export data (which consists of encoded image data stream,
* width, height, background color and various other export parameters like
* exportFormat, exportFileName, exportAction, exportTargetWindow) to this script.
*
* The script would process this data using appropriate resource files and build
* export binary (PDF/image)
*
* It either saves the binary as file to a server side directory or push it as
* Download to client side.
*
*
* ISSUES
* ------
* Q. What if someone wishes to open in the same page where the chart existed as postback
* replacing the old page?
*
* A. Not directly supported using any chart attribute or parameter but can do by
* removing/commenting the line containing 'header( content-disposition ...'
*
*/
/**
* @requires FCExporter_{format}.php as export resouce for the specific format
* e.g. FCExporter_PDF.php (containing PDF Releated Export resource functions)
* FCExporter_IMG.php (containing JPEG/PNG/GIF Releated Export resource functions)
*
* Details
* -------
* Only one export resource would be included at one time.
*
* The resource files would have these things as common:
*
* a) a constant - MIMETYPES that would have a string
* containing semicolon separated key value pairs.
* Each key can be a format name specified in the
* HANDLERASSOCIATIONS constant. The associated value
* would be the mimetype for the specified format.
*
* e.g. define("MIMETYPES","jpg=image/jpeg;jpeg=image/jpeg;png=image/png;gif=image/gif");
*
*
* b) a constant - EXTENSIONS that again contain a string of
* semicolon separated key value pair. Each key would again be the
* format name and the extension would be the file extension.
*
* e.g. define("EXTENSIONS","jpg=jpg;jpeg=jpg;png=png;gif=gif");
*
*
* c) a function - exportProcessor ( $stream , $meta )
* It would take the FusionCharts exncoded image string as $stream &
* an associative array $meta containging width, height and bgColor keys.
*
* The function would return an object of mixed type which would contain
* the processed binary/relevant export object.
*
*
* d) a function - exportOutput ( $exportObj, $exportSettings, $quality=1 )
* It would take the processed export object and other export setting as parameter.
* Moreover, it would take an optional parameter - $quality (in scale of 0 to 1).
* By Default, the $quality is passed as 1 (best quality)
*
* The function would return the file path on success or return false on failure.
*
* [ The other code in the resource file can be anything that support this architecture ]
*
*/
// =============================================================================
// == Constants and Variables ==
// =============================================================================
// USERS are to EDIT the values of the following constants.
/* ----------------------- EXPORT PATH & URI -------------------------------- */
/**
* IMPORTANT: You need to change the location of folder where
* the exported chart images/PDFs will be saved on your
* server. Please specify the path to a folder with
* write permissions in the constant SAVE_PATH below.
*
* Please provide the path as per PHP path conventions. You can use relative or
* absolute path.
* Examples: './' , '/users/me/images/' , './myimages'
*
* For Windows servers you can ALSO use \\ as path separator too. e.g. c:\\php\\mysite\\
*/
define ( "SAVE_PATH", "./" );
/**
* IMPORTANT: This constant HTTP_URI stores the HTTP reference to
* the folder where exported charts will be saved.
* Please enter the HTTP representation of that folder
* in this constant e.g., http://www.yourdomain.com/images/
*/
define ( "HTTP_URI", "http://www.yourdomain.com/images/" );
// ==============================================================================
// Users are recommended NOT to perform any editing beyond this point. ==
// ==============================================================================
/* ------------------------- EXPORT RESOURCES -------------------------------- */
// This constant defines the name of the export handler script file
// The name is appended with a suffix (from constant HANDLERASSOCIATIONS)
define ( "EXPORTHANDLER", "FCExporter_" );
// This constant lists all the currently supported export formats
// and related export handler file suffix.
// e.g. for JPEG the suffix is
define ( "HANDLERASSOCIATIONS", "PDF=PDF;JPEG=IMG;JPG=IMG;PNG=IMG;GIF=IMG" );
// Path where the export handler files are located
// Please note that the resource path should be relative to
// FCExporter.php file's directory
// By default the path is "./Resources/"
define ( "RESOURCEPATH","Resources/" );
/* ---------------------------- Export Settings ------------------------------- */
/**
* OVERWRITEFILE sets whether the export handler would overwrite an existing file
* the newly created exported file. If it is set to false the export handler would
* not overwrite. In this case if INTELLIGENTFILENAMING is set to true the handler
* would add a suffix to the new file name. The suffix is a randomly generated UUID.
* Additionally, you add a timestamp or random number as additional suffix.
*
*/
define ( "OVERWRITEFILE", false );
define ( "INTELLIGENTFILENAMING", true );
define ( "FILESUFFIXFORMAT", "TIMESTAMP" ) ;// value can be either 'TIMESTAMP' or 'RANDOM'
// List the default exportParameter values taken if not provided by chart
$defaultParameterValues = array(
"exportfilename" => "FusionCharts",
"exportaction" => "download",
"exporttargetwindow" => "_self",
"exportformat" => "PDF"
);
// Stores server notices if any as string [ to be send back to chart after save ]
$notices = "" ;
// =============================================================================
// == processing ==
// =============================================================================
/**
* Retrieve export data from POST Request sent by chart
* Parse the Request stream into export data readable by this script
*
* Store export data into an array containing keys 'stream' (contains encoded
* image data) ; 'meta' ( contains an array with 'width', 'height' and 'bgColor' key
没有合适的资源?快使用搜索试试~ 我知道了~
FusionMaps_Enterprise.rar
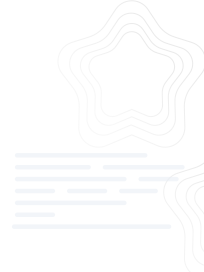
共1165个文件
html:804个
jpg:97个
gif:70个


温馨提示
FusionMaps_Enterprise.rarFusionMaps_Enterprise.rarFusionMaps_Enterprise.rar
资源推荐
资源详情
资源评论
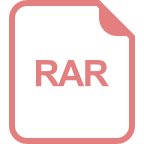
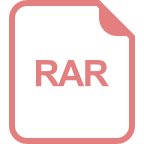
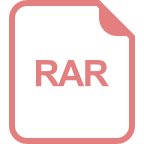
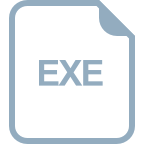
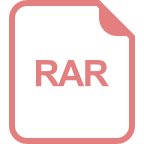
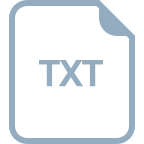
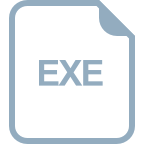
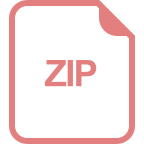
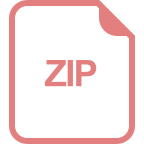
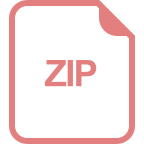
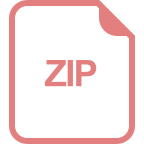
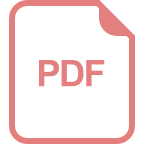
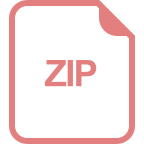
收起资源包目录

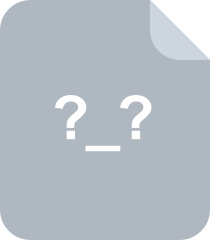
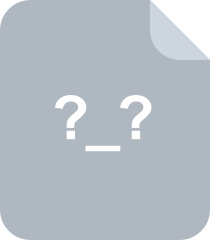
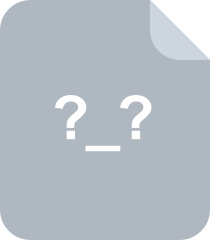
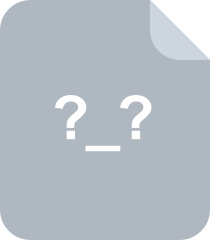
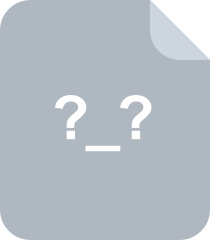
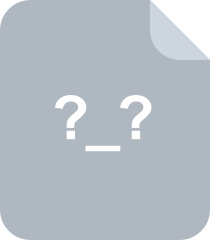
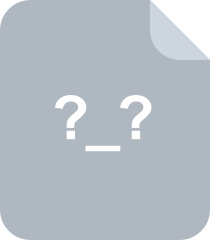
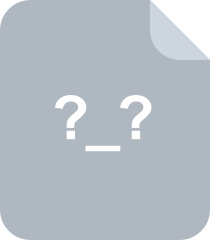
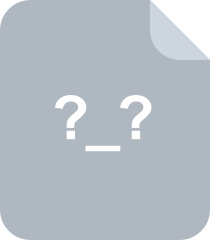
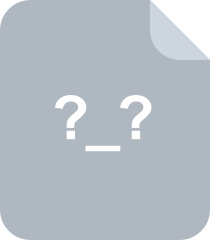
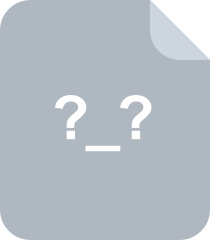
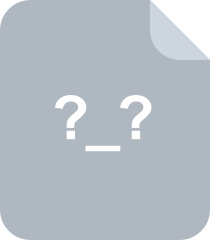
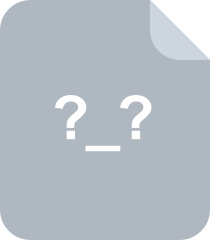
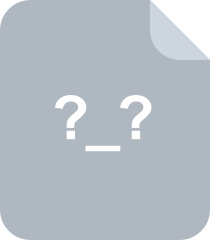
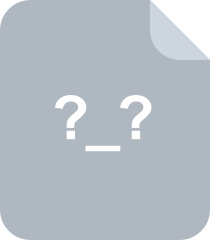
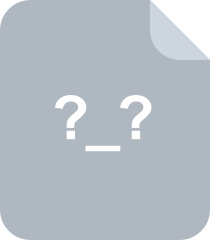
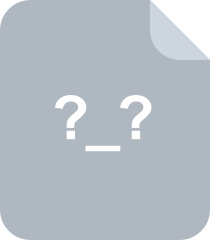
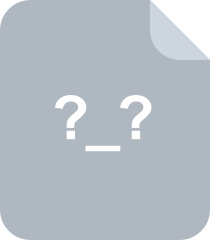
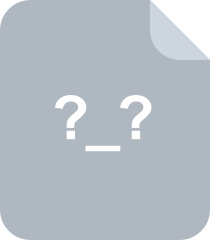
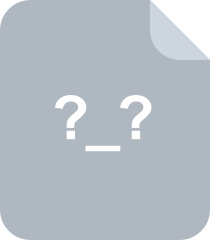
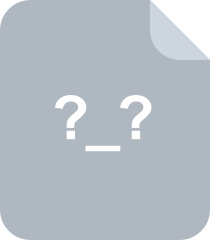
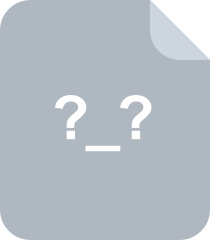
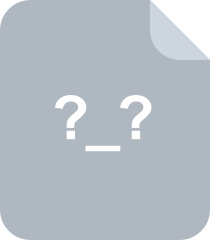
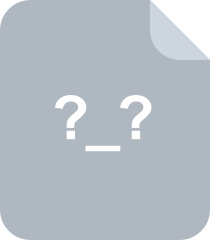
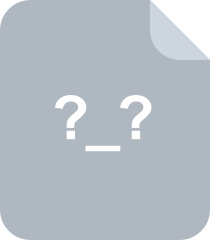
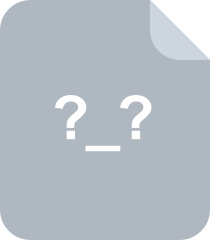
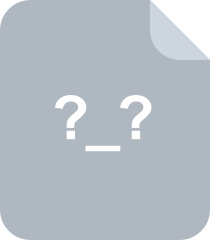
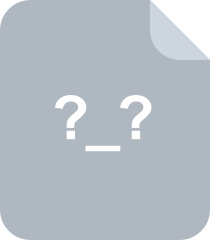
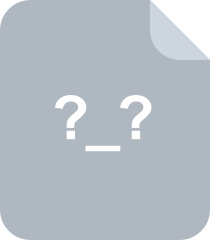
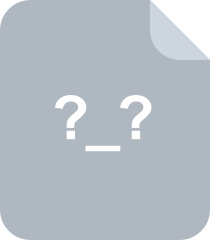
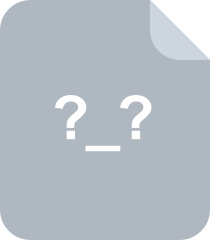
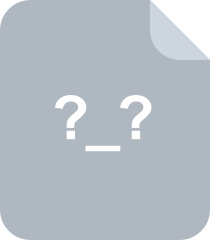
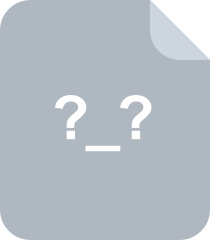
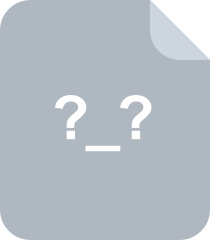
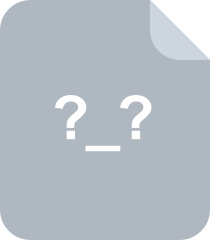
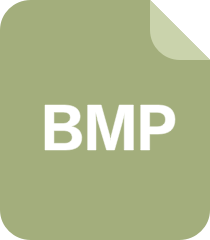
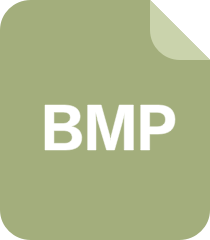
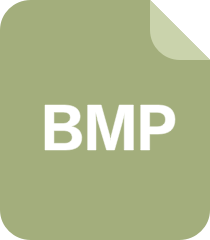
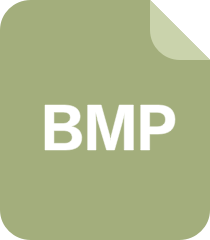
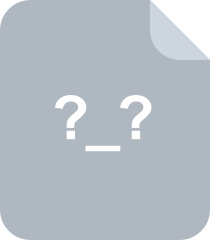
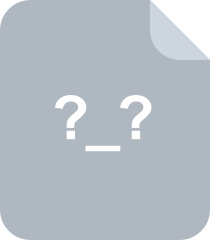
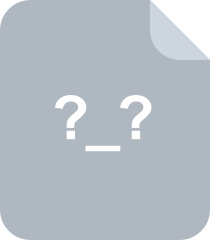
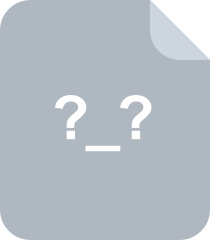
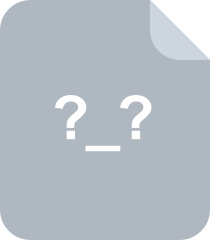
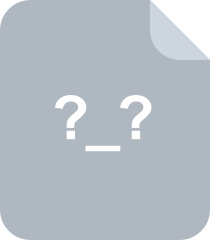
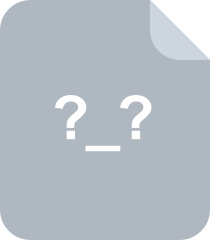
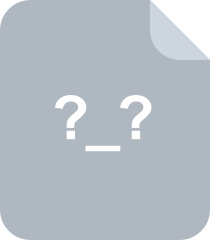
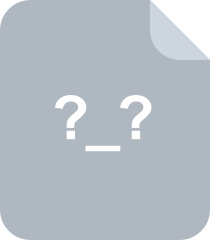
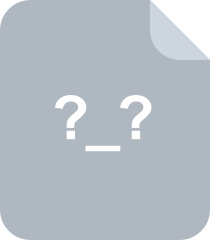
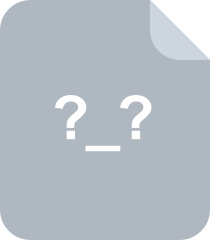
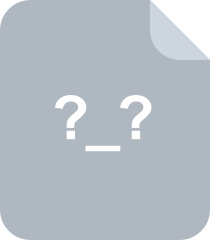
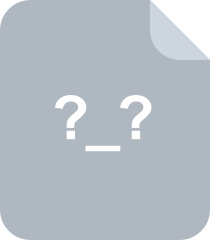
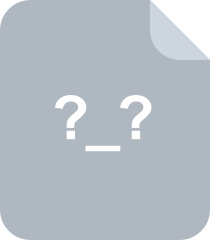
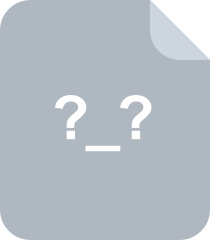
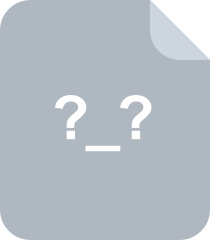
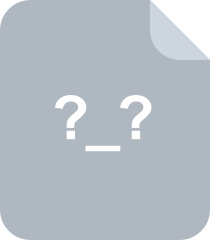
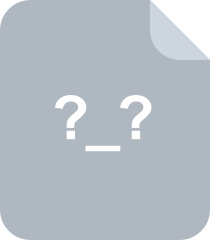
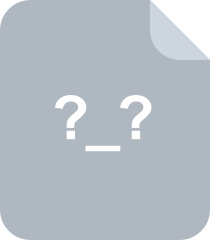
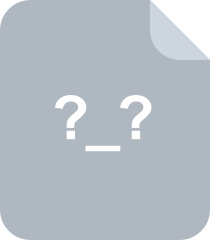
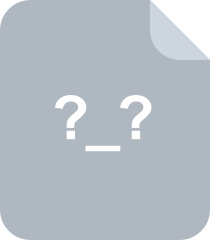
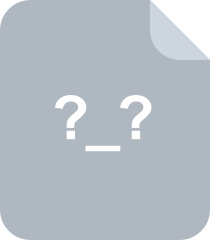
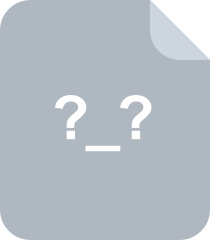
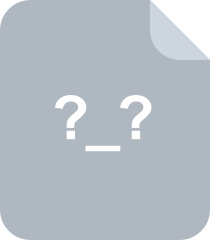
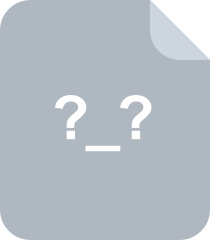
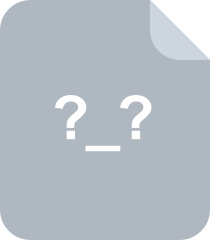
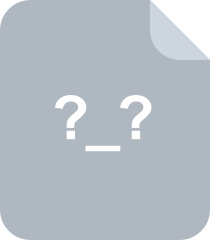
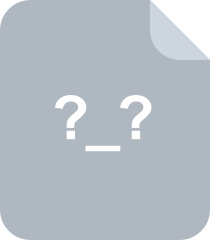
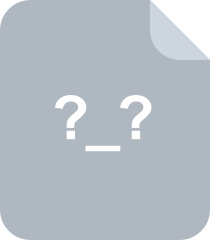
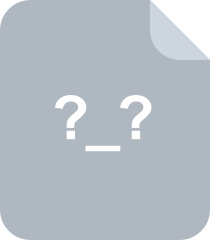
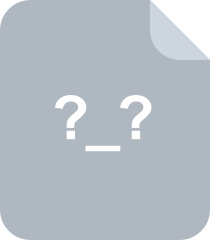
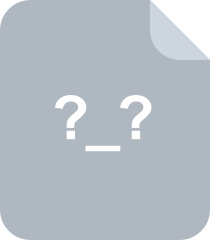
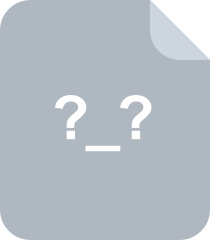
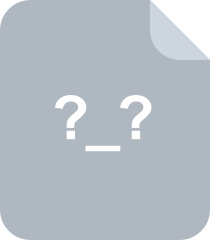
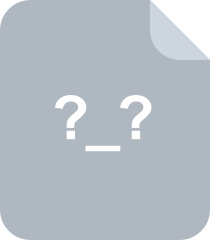
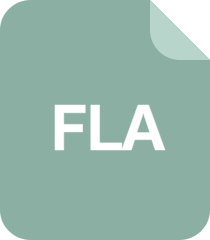
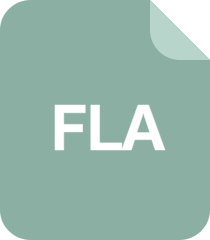
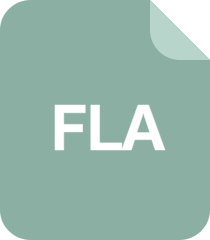
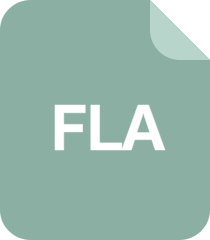
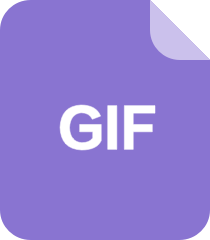
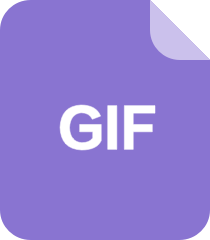
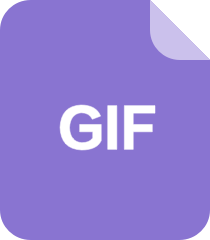
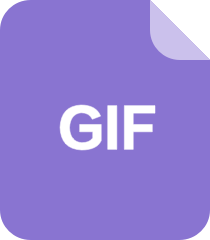
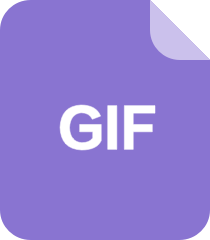
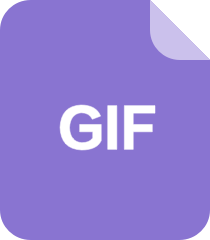
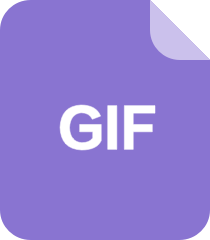
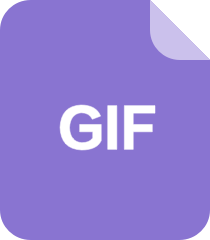
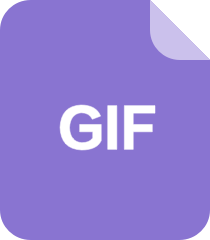
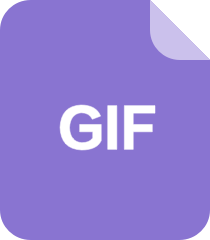
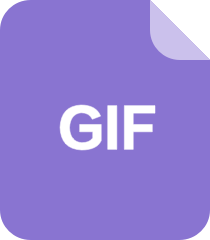
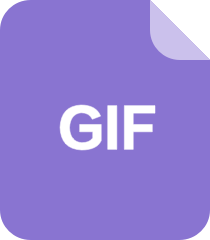
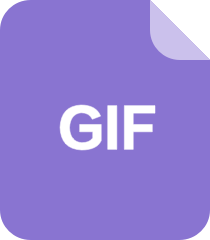
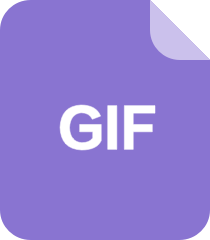
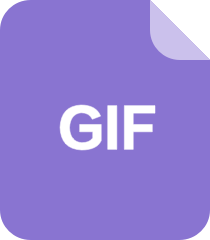
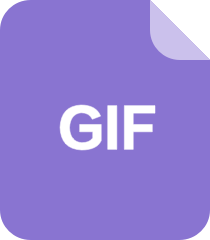
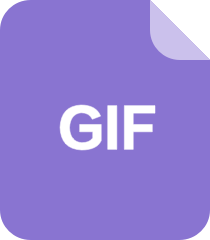
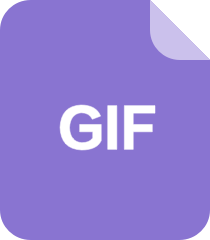
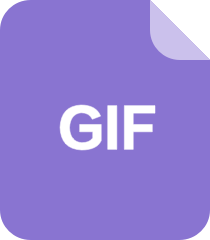
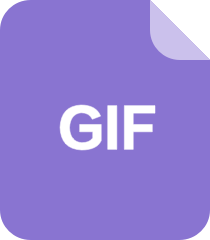
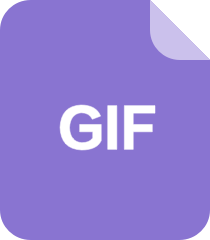
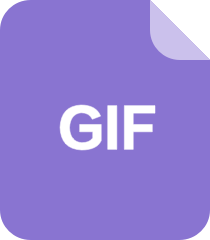
共 1165 条
- 1
- 2
- 3
- 4
- 5
- 6
- 12
资源评论
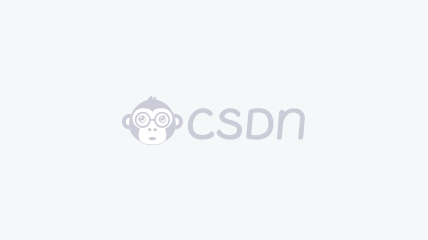
- weihaibao2012-03-30好资源,感谢楼主,可以自定义地图。
- 我是java菜鸟2014-04-02楼主很厚道,没有要积分!
- aaa31317272014-05-24Gallery中的例子怎么无法查看???但是其文件代码可以供我这样的初学者参考。

sdfyc2007
- 粉丝: 2
- 资源: 23
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

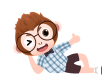
安全验证
文档复制为VIP权益,开通VIP直接复制
