在Android应用开发中,自定义标题栏是一种常见的需求,它能提供独特的用户界面风格和功能。本教程将详细讲解如何创建一个自定义标题栏,并在其中添加控件,以及实现这些控件的监听和响应事件。 我们需要理解Android系统的布局层次结构。标题栏(Toolbar)通常位于Activity的顶部,它是ActionBar的现代替代品,提供了更灵活的定制选项。要自定义标题栏,我们首先要创建一个新的布局文件,例如`toolbar_custom.xml`,在该文件中定义我们想要的标题栏布局。 ```xml <!-- toolbar_custom.xml --> <androidx.appcompat.widget.Toolbar xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/custom_toolbar" android:layout_width="match_parent" android:layout_height="?attr/actionBarSize" android:background="?attr/colorPrimary" android:elevation="4dp"> <!-- 在这里添加自定义控件 --> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:gravity="center_vertical"> <ImageView android:id="@+id/icon" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/ic_launcher_foreground" /> <TextView android:id="@+id/title" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="自定义标题" android:textColor="@android:color/white" android:textSize="20sp" /> <Button android:id="@+id/button_right" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="按钮" /> </LinearLayout> </androidx.appcompat.widget.Toolbar> ``` 接下来,我们需要在Activity的布局文件中包含这个自定义的标题栏,并在Activity的代码中设置它为Activity的顶级布局。这样,我们就可以使用`setSupportActionBar()`方法来启用自定义的标题栏。 ```java // 在Activity的onCreate()方法中 setContentView(R.layout.activity_main); Toolbar customToolbar = findViewById(R.id.custom_toolbar); setSupportActionBar(customToolbar); getSupportActionBar().setDisplayHomeAsUpEnabled(false); // 隐藏返回图标 ``` 为了实现标题栏中的控件监听和响应事件,我们需要在Activity中找到这些控件并设置监听器。例如,对于上面XML中的`Button`,我们可以这样做: ```java Button buttonRight = findViewById(R.id.button_right); buttonRight.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Toast.makeText(MainActivity.this, "按钮被点击了", Toast.LENGTH_SHORT).show(); // 在这里处理点击事件 } }); ``` 此外,对于`ImageView`或`TextView`等其他控件,我们也可以使用相同的方法设置监听器。如果需要更复杂的交互,如长按、滑动等,可以使用`OnLongClickListener`、`OnTouchListener`等。 在实际项目中,我们可能还需要处理标题栏的其他行为,比如导航按钮的点击事件、菜单项的选择等。这可以通过重写`onOptionsItemSelected(MenuItem item)`和`onOptionsItemSelected(MenuItem item)`方法来实现。 总结来说,自定义Android应用的标题栏主要包括以下几个步骤: 1. 创建自定义标题栏的布局文件。 2. 在Activity的布局中包含自定义标题栏。 3. 设置自定义标题栏为Activity的顶级布局。 4. 对标题栏中的控件进行事件监听和响应。 通过这种方式,开发者可以自由地设计和控制标题栏的行为,从而提升用户体验。同时,注意保持代码的清晰和模块化,以便于维护和扩展。
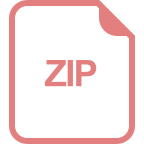
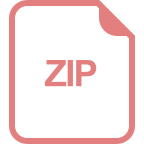
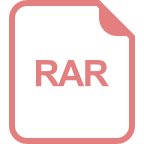
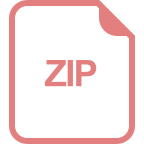
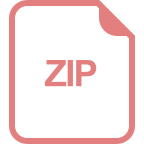
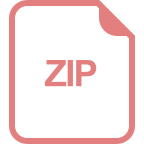
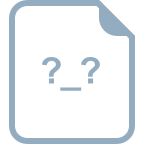
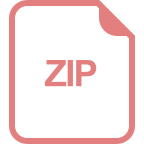
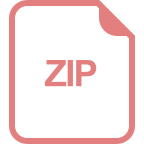
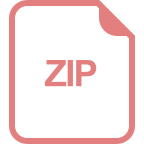
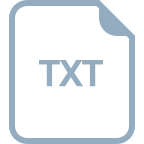
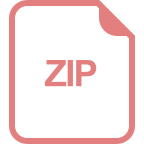
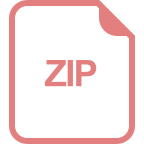
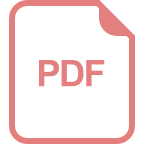
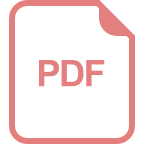
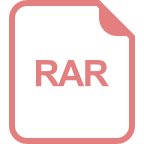
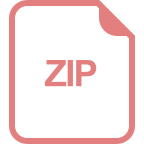
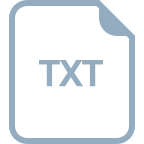
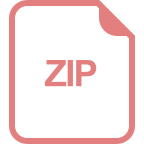
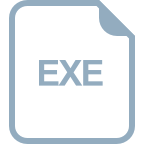
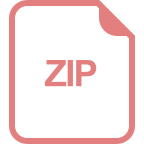

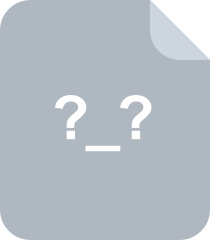
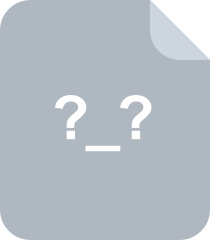
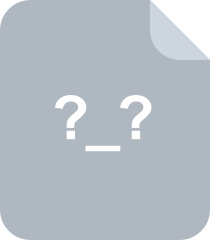
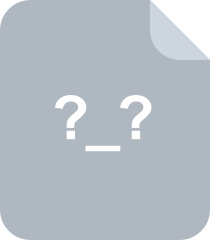
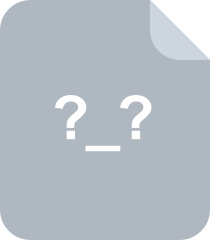
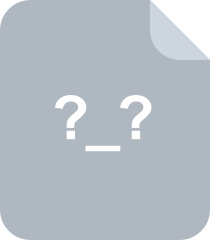
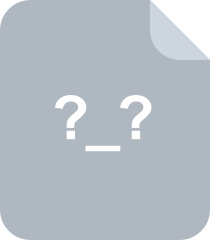
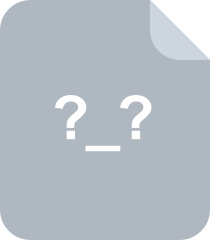
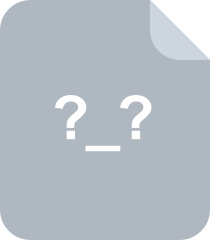
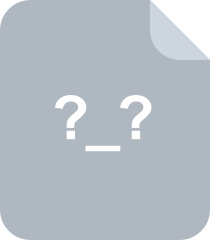
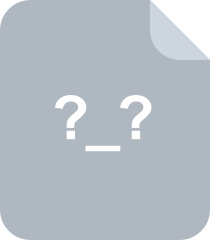
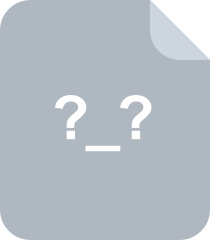
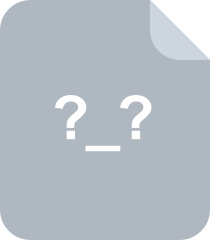
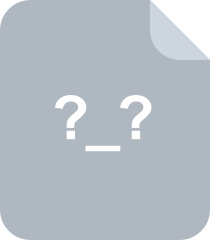
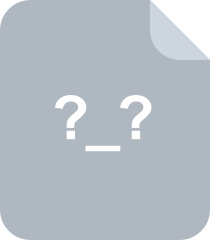
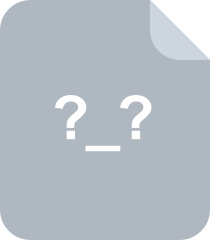
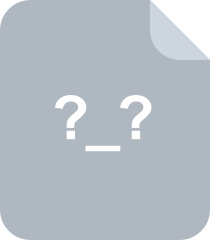
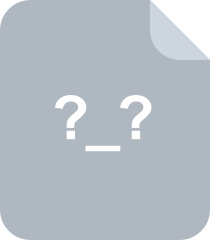
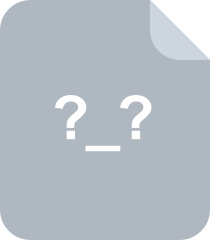
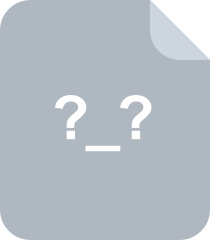
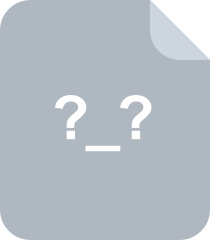
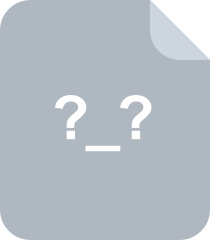
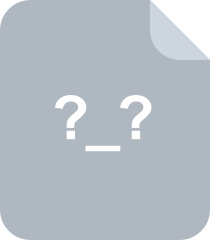
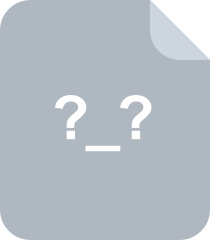
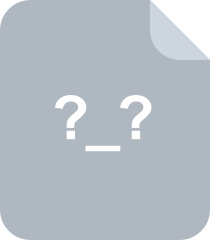
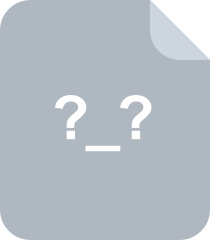
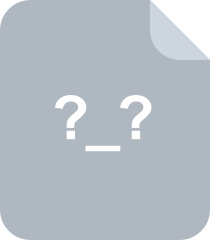
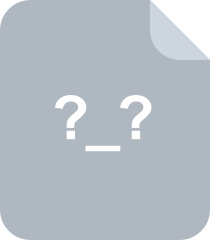
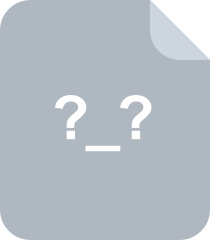
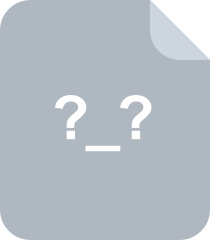
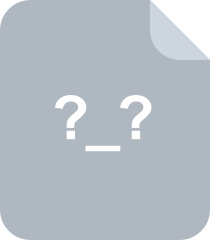
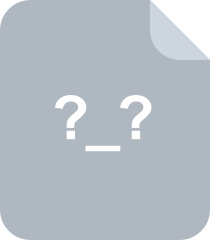
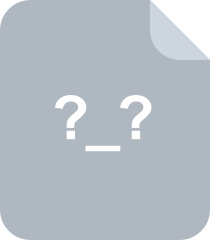
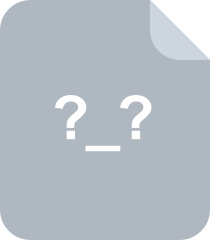
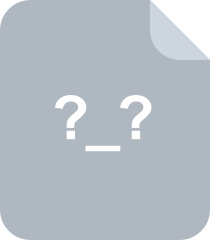
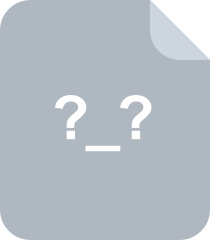
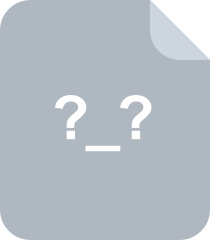
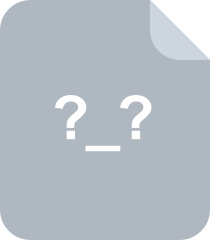
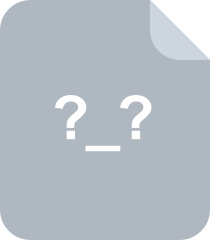
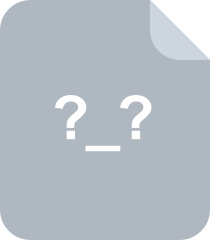
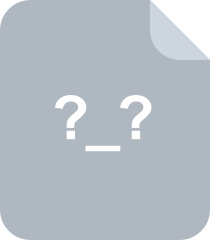
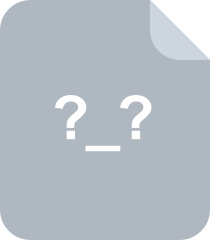
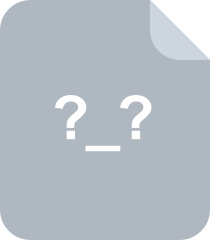
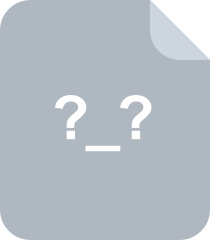
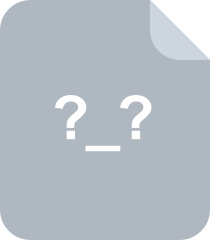
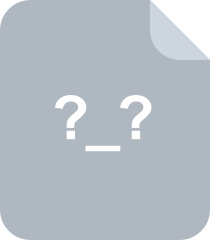
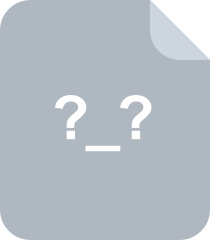
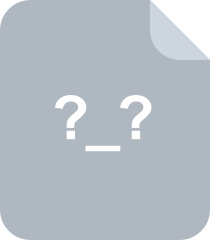
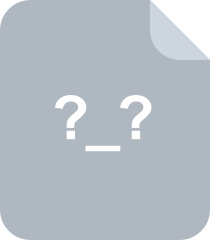
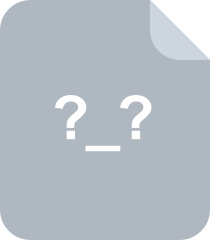
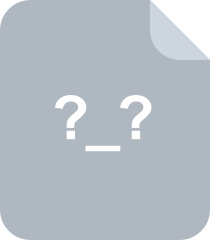
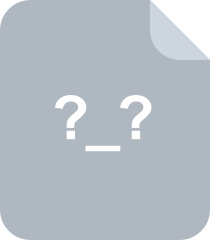
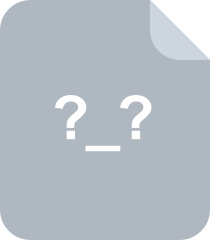
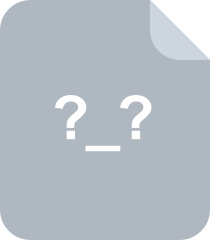
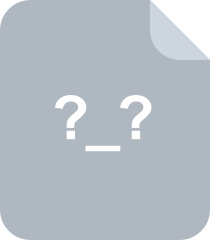
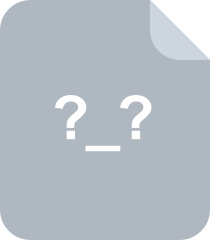
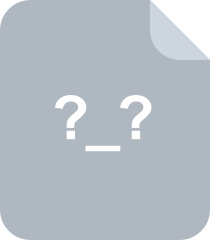
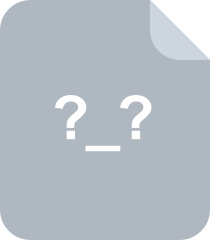
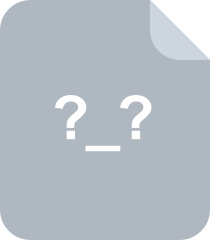
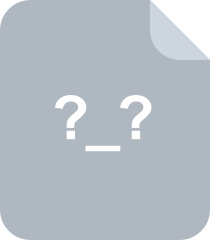
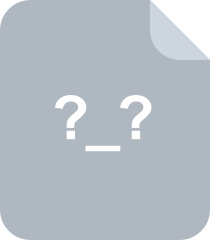
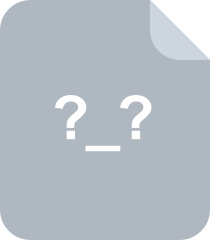
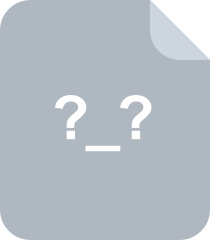
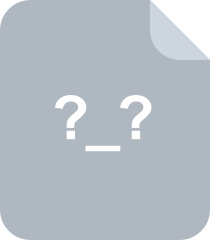
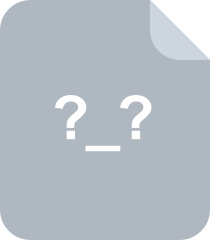
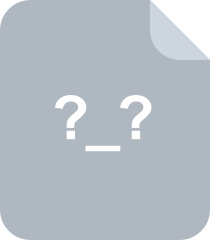
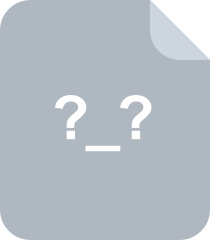
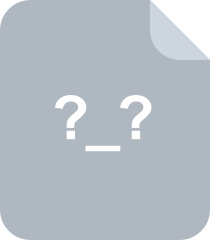
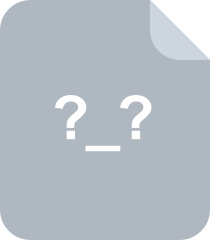
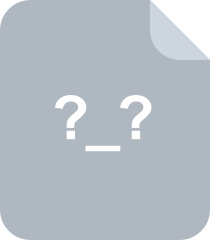
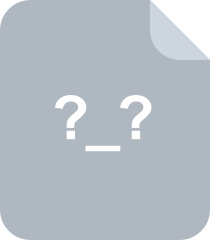
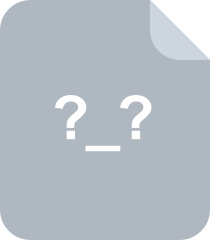
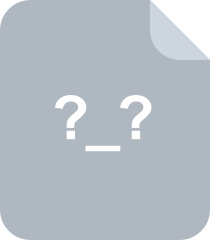
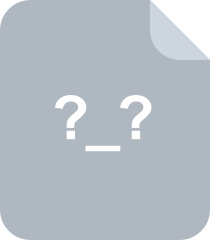
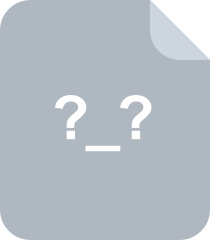
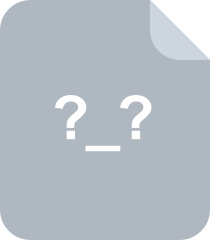
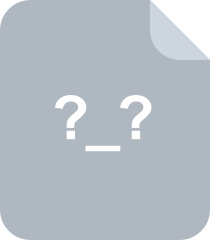
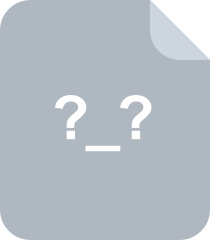
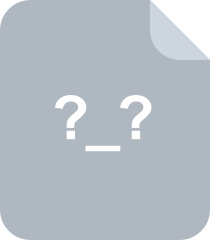
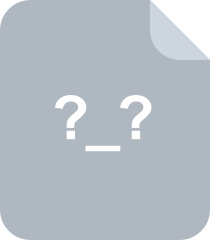
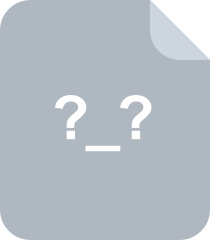
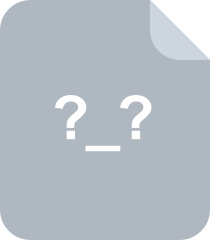
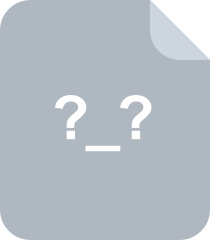
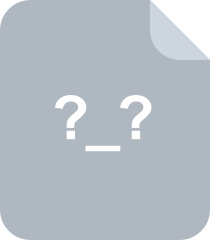
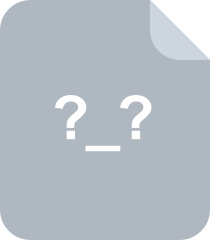
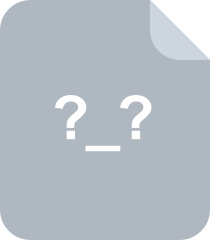
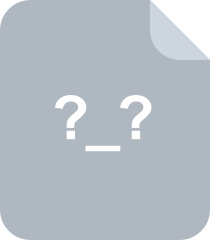
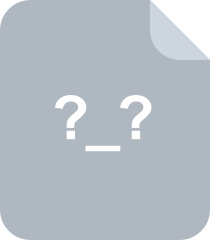
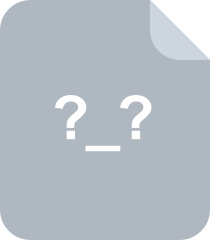
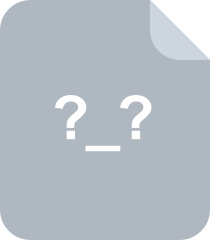
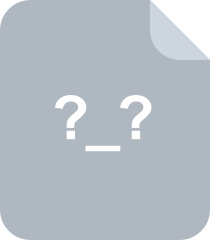
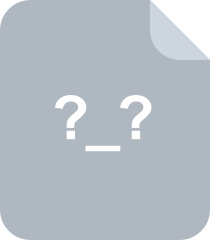
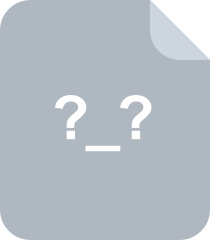
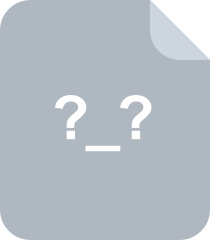
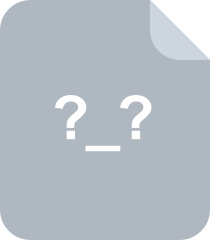
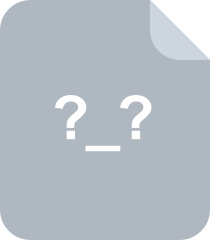
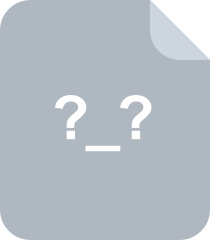
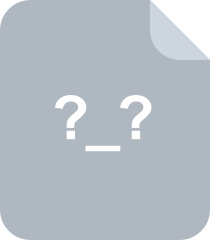
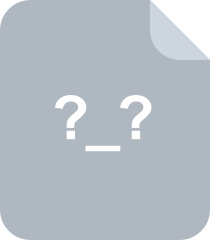
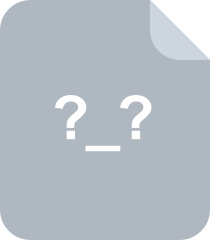
- 1
- 2
- 3
- 4
- 5
- 6
- 19
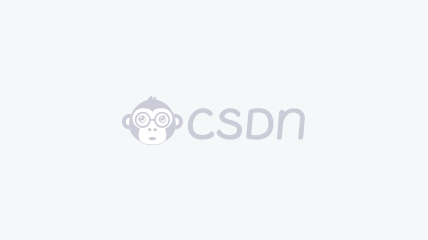

- 粉丝: 10
- 资源: 38
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

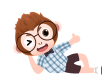
最新资源

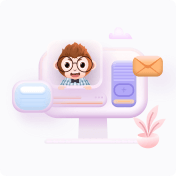
