/*
* JWebcamPlayer.java
*
* Created on March 21, 2005, 1:31 AM
*/
/**
*
* @author Alvaro Salmador (nx5) (naplam33 at msn.com)
*/
import java.applet.*;
import java.applet.AppletContext.*;
import java.awt.*;
import java.awt.event.*;
import java.awt.image.*;
import java.awt.Image;
import java.net.URL;
import javax.swing.*;
//import javax.swing.table.*;
import javax.imageio.*;
//import java.util.*;
import java.io.*;
import java.net.*;
public class JWebcamPlayer extends javax.swing.JApplet implements MouseListener, MouseMotionListener
{
public static final boolean DEBUGGING = false;
public JWebcamPlayer()
{
}
public static String checkAppletLoaded()
{
return "ok";
}
public static int unsignedByteToInt( byte b )
{
return (int) b & 0xff;
}
public void init()
{
m_strColor = getParameter("Color");
if (m_strColor==null || m_strColor=="") m_strColor = "#FFFFFF";
m_strServer = getCodeBase().getHost();//getParameter("Server");
if (m_strServer==null || m_strServer=="") m_strServer = "127.0.0.1";
m_strPort = getParameter("Port");
if (m_strPort==null || m_strPort=="") m_strPort = "7070";
overlay = toBufferedImage(getImage(getDocumentBase(), "control.jpg" ));
do_overlay = false;
addMouseListener(this);
addMouseMotionListener(this);
}
public void createGUI()
{
setBackground(Color.decode(m_strColor));
try {
//UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName()); //native
UIManager.setLookAndFeel(UIManager.getCrossPlatformLookAndFeelClassName()); //java
} catch(Exception e) { }
Container content = getContentPane();
content.setBackground(Color.decode(m_strColor));
GridBagLayout gbl = new GridBagLayout();
content.setLayout(gbl);
GridBagConstraints c = new GridBagConstraints();
c.fill = GridBagConstraints.BOTH;
c.weighty = 1.0;
c.weightx = 1.0;
c.gridwidth = 1;
c.anchor = GridBagConstraints.NORTHWEST;
c.insets = new Insets(0, 0,0,0);
c.gridheight = 1;
c.gridx = 0;
c.gridy = 0;
m_label = new JLabel();
m_label.setHorizontalAlignment(SwingConstants.LEFT);
m_label.setVerticalAlignment(SwingConstants.TOP);
m_label.setText("JWebcamPlayer Applet");
content.add(m_label, c);
}
public void start()
{
m_stop = false;
try {
javax.swing.SwingUtilities.invokeAndWait(new Runnable()
{
public void run() {
createGUI();
}
} );
} catch (Exception e) {
}
m_worker = new SwingWorker() {
public Object construct() {
Socket connection;
InputStream in;
OutputStream out;
try {
int port = 0;
try {
port = Integer.parseInt(m_strPort);
}
catch(Exception e) {
port = 0;
}
if (port==0) port = 7070;
connection = new Socket(m_strServer, port);
in = connection.getInputStream();
out = connection.getOutputStream();
}
catch (IOException e) {
System.out.println(
"Attempt to create connection failed with error: " + e);
m_label.setIcon(null);
m_label.setText("JWebcamPlayer - Connection failed");
m_stop = true;
return null;
}
byte [] buffer = new byte [512*1024];
m_label.setText("");
while(!m_stop)
{
// bucle, constantemente lee imagen del server y la muestra
byte [] b = {'O','K',0,0, 0,0,0,0,0,0,0,0,0};
if(bright_up) {
b[7] = 1;
bright_up = false;
}
if(bright_down) {
b[7] = 2;
bright_down = false;
}
if(contrast_up) {
b[8] = 1;
contrast_up = false;
}
if(contrast_down) {
b[8] = 2;
contrast_down = false;
}
int n = 0;
int siz = 0;
try {
out.write(b);
if (DEBUGGING)
System.out.print("-sending my header ("+Integer.toString(b.length)+") bytes");
n = in.read(buffer, 0, HDRLEN);
/*while(n<HDRLEN && n>0) {
int r = in.read(buffer, n, HDRLEN-n);
if (r<=0) break;
}*/
if (DEBUGGING)
System.out.print("-read header ("+Integer.toString(n)+") bytes");
}
catch(Exception e) {
if (DEBUGGING)
e.printStackTrace();
m_stop = true;
}
if (n<HDRLEN)
{
if (n<=0)
m_stop = true;
continue;
}
else
{
// can someone point out a more straightforward way to convert 4 bytes
// to a java integer? this is just awful...
int ssz = SZOFS;
siz += unsignedByteToInt(buffer[ssz+3]) << 24;
siz += unsignedByteToInt(buffer[ssz+2]) << 16;
siz += unsignedByteToInt(buffer[ssz+1]) << 8;
siz += unsignedByteToInt(buffer[ssz]) ;
if (DEBUGGING)
System.out.println("** reading jpeg, size="+Integer.toString(siz));
n = HDRLEN;
if (buffer[0]!='S' || buffer[1]!='P' || buffer[2]!='C' || buffer[3]!='A')
{
if (DEBUGGING)
System.out.println("*Header missing 'SPCA'");
continue;
}
else if (siz<=0 || siz>(512*1024))
{
siz = 0;
try {
in.read(buffer, n, buffer.length);
}
catch(Exception e) {
if (DEBUGGING)
e.printStackTrace();
}
if (DEBUGGING)
System.out.println("*Illegal image size");
continue;
}
else
{
do
{
int r = 0;
try {
//r = in.read(buffer, n, HDLREN + siz - n);
r = in.read(buffer, n, buffer.length - n);
}
catch(Exception e)
{
e.printStackTrace();
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
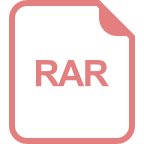
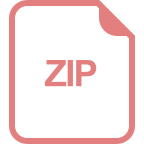
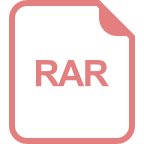
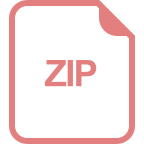
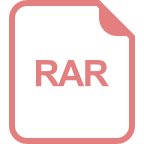
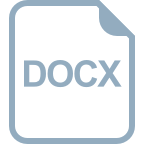
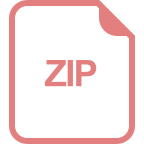
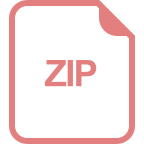
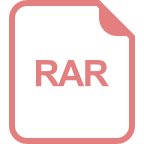
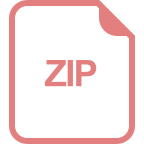
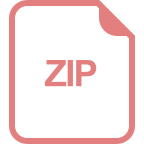
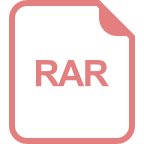
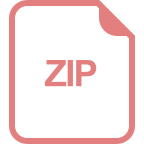
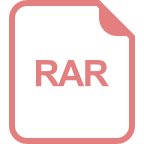
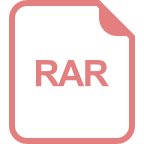
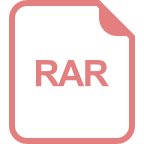
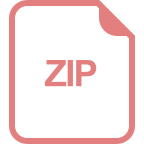
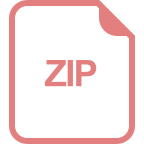
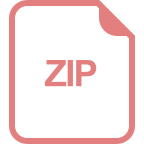
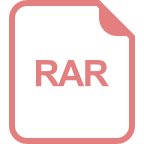
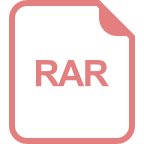
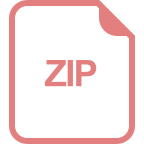
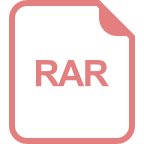
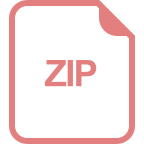
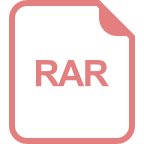
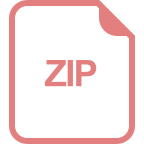
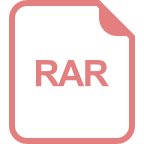
收起资源包目录



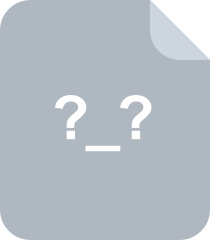
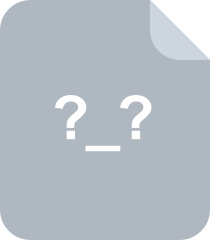
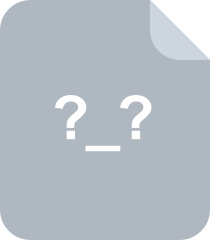
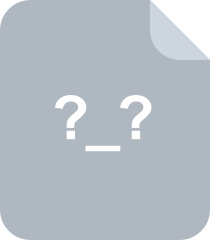
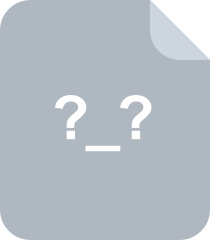
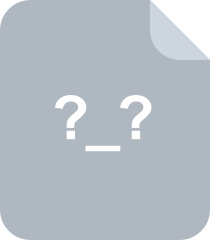
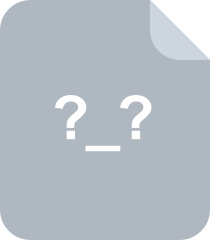
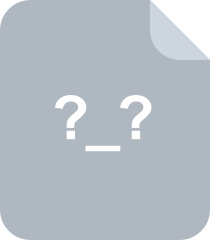
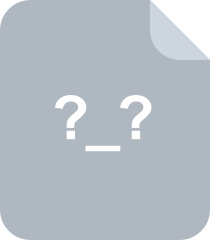
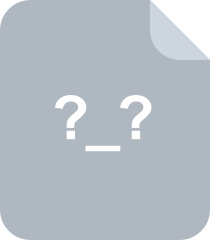
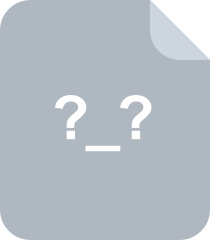
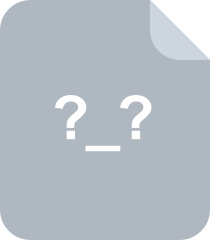
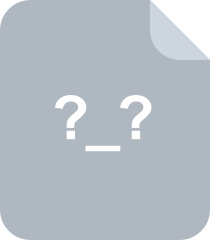
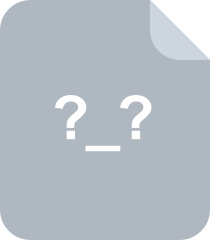
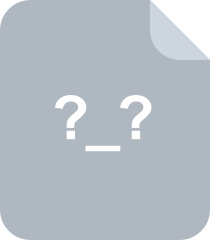
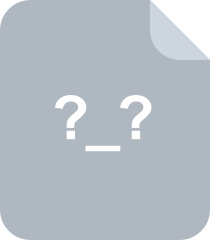
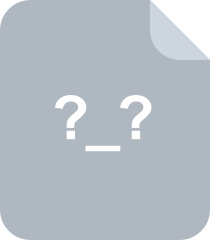
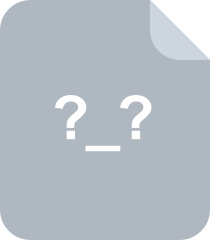
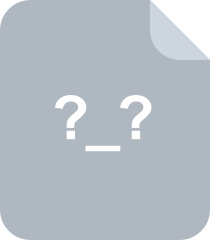
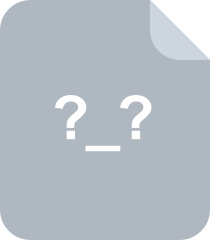
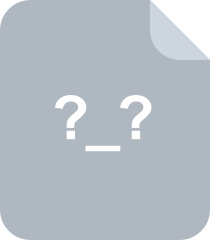

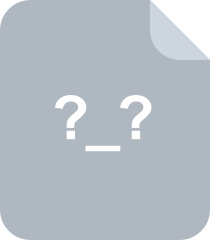
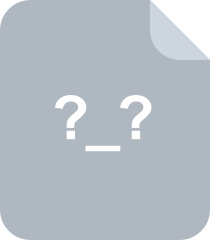
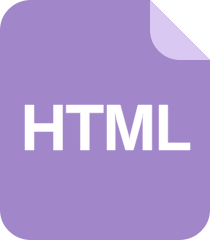
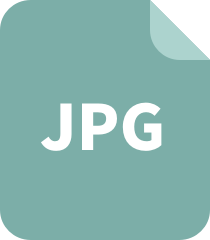
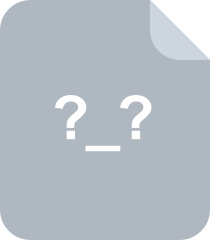
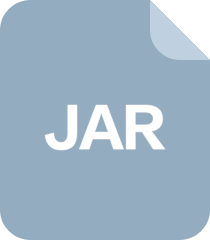
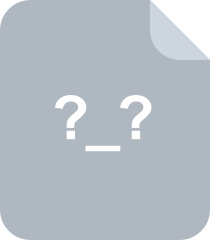
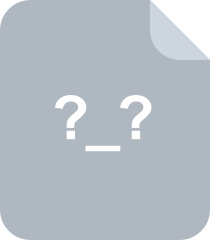
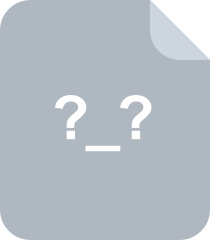
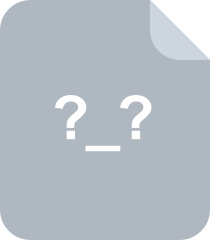
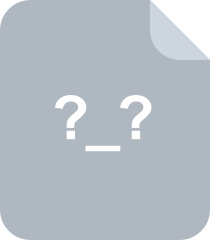
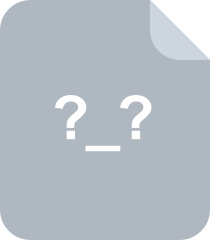
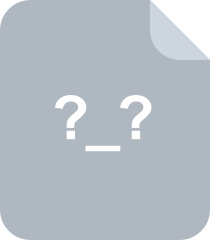
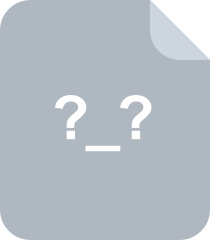
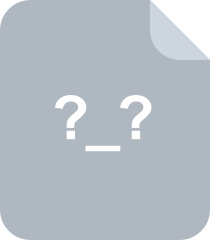
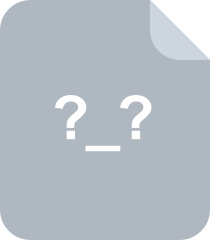
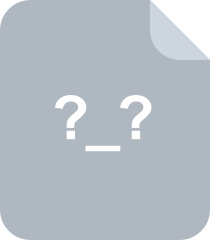
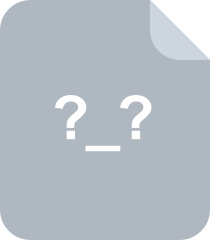
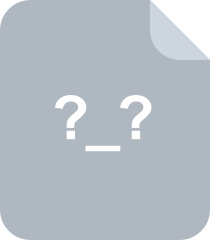
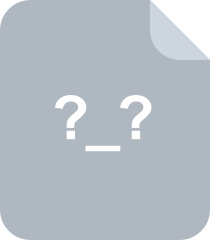
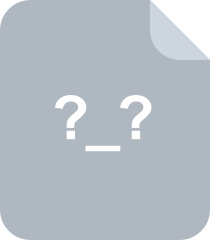
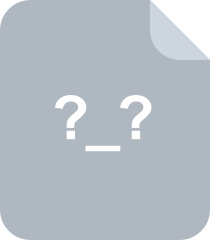
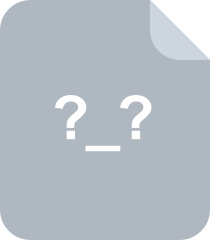
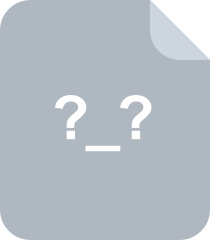
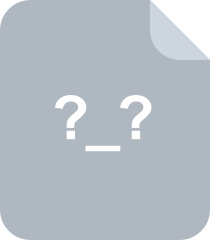
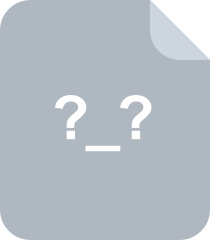
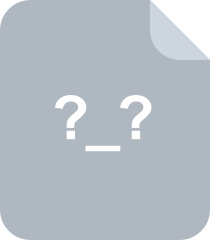
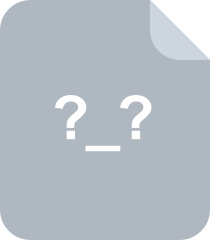
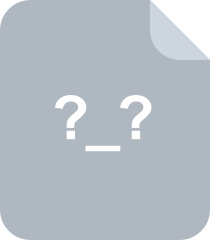
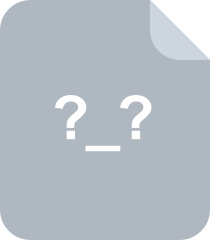
共 51 条
- 1

tinygg
- 粉丝: 6
- 资源: 34
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

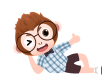
最新资源
- python《K近邻算法实现简单的手写数字识别》+项目源码+文档说明
- C#MVC4权限管理系统开发框架源码数据库 SQL2008源码类型 WebForm
- Windows系统tcping文件,测试tcp端口能否通信
- 技术资料分享ATK-HC05蓝牙串口模块使用说明-AN1301很好的技术资料.zip
- 课程设计《C++实现无UI界面的、涉及MySQL连接的快递管理系统》+项目源码+文档说明
- STM32控制蜂鸣器播放音乐
- DLL文件快速修复工具
- xwalk-core-library-23.53.589.4
- RKNN3588-YOLOv8的PT的requirements.txt
- C#ASP.NET手机端H5会议室预约系统源码 手机版会议室预约源码数据库 SQL2008源码类型 WebForm
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


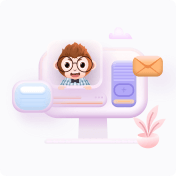
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页