package com.tiangang.service;
import com.alibaba.fastjson.JSONObject;
import kong.unirest.Unirest;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpMethod;
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.HashMap;
import java.util.Map;
/**
* 作者:天罡gg
* 作者博客主页:https://blog.csdn.net/scm_2008
* 博文地址:https://blog.csdn.net/scm_2008/article/details/128764936
* 有任何问题,欢迎大家私信联系或微信联系.
*/
@Service
public class WechatService {
@Autowired
private RestTemplate restTemplate;
private String wechatQrcodeUrl = "https://api.weixin.qq.com/wxa/getwxacodeunlimit?access_token=";
private String wechatAccessTokenUrl = "https://api.weixin.qq.com/cgi-bin/token?appid=%s&secret=%s&grant_type=client_credential";
private String appid = "替换成你的appid";
private String secret = "替换成你的secret";
private String accessTokenCache;
// 自行配置:正式版为 "release",体验版为 "trial",开发版为 "develop"。默认是正式版。
private String envVersion = "trial";
public String generateWechatRrCode(Integer mode) {
// 当前登录账号
String accountId = "123456";
if (accessTokenCache == null) {
accessTokenCache = getAccessToken();
}
String url = wechatQrcodeUrl + accessTokenCache;
Map<String, Object> body = new HashMap<>();
// 场景码,请根据业务场景与【前端】约定
body.put("scene", String.format("a=%s", accountId));
body.put("env_version", envVersion);
// 透明
body.put("is_hyaline", true);
byte[] bytes = null;
switch (mode) {
case 1:
bytes = getWechatQrcodeByHttpURL(url, body);
break;
case 2:
bytes = getWechatQrcodeByHttpClient(url, body);
break;
case 3:
bytes = getWechatQrcodeByOkhttp3(url, body);
break;
case 4:
bytes = getWechatQrcodeByUnirest(url, body);
break;
case 5:
bytes = getWechatQrcodeByRestTemplate(url, body);
break;
}
if (bytes == null) {
return "null";
}
String error = new String(bytes);
if (error.contains("errcode")) {
return error;
}
// 保存到本地
saveQrCodeToLocal(bytes,mode);
return "ok";
}
// 获取access_token
public String getAccessToken() {
String url = String.format(wechatAccessTokenUrl, appid, secret);
Map<String, String> responseBody = restTemplate.exchange(url, HttpMethod.GET, null, new ParameterizedTypeReference<Map<String, String>>() {}).getBody();
if (responseBody == null || responseBody.get("errcode") != null) {
// 获取失败
System.out.println("getAccessToken失败:" + (responseBody == null ? "null" : responseBody.toString()));
return null;
}
return responseBody.get("access_token");
}
// 一、原生HttpURLConnection方式
public byte[] getWechatQrcodeByHttpURL(String url, Map<String, Object> body) {
HttpURLConnection httpURLConnection = null;
try {
httpURLConnection = (HttpURLConnection) new URL(url).openConnection();
httpURLConnection.setRequestMethod("POST");
// 发送POST请求必须设置如下两行
httpURLConnection.setDoOutput(true);
httpURLConnection.setDoInput(true);
// 获取URLConnection对象对应的输出流
PrintWriter printWriter = new PrintWriter(httpURLConnection.getOutputStream());
// 发送请求参数
printWriter.write(JSONObject.toJSONString(body));
// flush输出流的缓冲
printWriter.flush();
//开始获取数据
try (InputStream inputStream = httpURLConnection.getInputStream();
ByteArrayOutputStream out = new ByteArrayOutputStream()) {
byte[] buffer = new byte[1024];
int len = -1;
while ((len = inputStream.read(buffer)) != -1) {
out.write(buffer, 0, len);
}
return out.toByteArray();
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (httpURLConnection != null) {
httpURLConnection.disconnect();
}
}
return null;
}
// 二、apache.httpclient方式
public byte[] getWechatQrcodeByHttpClient(String url, Map<String, Object> body) {
CloseableHttpClient httpClient = HttpClientBuilder.create().build();
HttpPost httpPost = new HttpPost(url);
try {
StringEntity entity = new StringEntity(JSONObject.toJSONString(body));
entity.setContentType("image/png");
httpPost.setEntity(entity);
HttpResponse response = httpClient.execute(httpPost);
try (InputStream inputStream = response.getEntity().getContent();
ByteArrayOutputStream out = new ByteArrayOutputStream()) {
byte[] buffer = new byte[1024];
int len = -1;
while ((len = inputStream.read(buffer)) != -1) {
out.write(buffer, 0, len);
}
return out.toByteArray();
}
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
// 三、okhttp3方式
public byte[] getWechatQrcodeByOkhttp3(String url, Map<String, Object> body) {
OkHttpClient client = new OkHttpClient().newBuilder().build();
okhttp3.MediaType mediaType = okhttp3.MediaType.parse("application/json");
RequestBody requestBody = RequestBody.create(mediaType, JSONObject.toJSONString(body));
Request request = new Request.Builder().url(url).method("POST", requestBody).build();
try {
Response response = client.newCall(request).execute();
if (response.isSuccessful()) {
return response.body().bytes();
}
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
// 四、Unirest方式
public byte[] getWechatQrcodeByUnirest(String url, Map<String, Object> body) {
return Unirest.post(url).body(JSONObject.toJSONString(body)).asBytes().getBody();
}
// 五、restTemplate方式
public byte[] getWechatQrcodeByRestTemplate(String url, Map<String, Object> body) {
return restTemplate.exchange(url, HttpMethod.POST, new HttpEntity<>(body, null), byte[].class).getBody();
}
// 保存到本地
private void saveQrCodeToLocal(byte[] bytes, Integer mode) {
try {
InputStream inputStream = new
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
先介绍一下项目场景,主要是通过微信小程序二维码裂变分享,每个账号有专属邀请二维码,分享出去,有新人扫码入驻,就可以得到现金奖励或红包奖励。当然,产品设计会更丰富,不止有裂变模式,还有渠道推广模式,还有各种奖励规则,但核心实现都是生成二维码。对于如何生成微信小程序二维码,本篇一共列举了5种实现方式,文末可打包下载开箱即用的全套源码,我更期待老铁的文末 投票,很想看看哪种才是你的菜~ 项目主要用的是微信官方提供的服务端能力,考虑到涉及secret和token等安全问题,所以从架构上设计的调用链路为:前端->后端API->微信API。裂变的场景决定要选择获取不限制的小程序码接口,永久有效,数量暂无限制,接口英文名:getUnlimitedQRCode。 官方地址:https://developers.weixin.qq.com/miniprogram/dev/OpenApiDoc/qrcode-link/qr-code/getUnlimitedQRCode.html
资源推荐
资源详情
资源评论
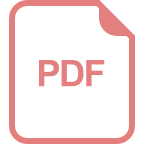
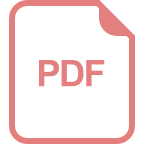
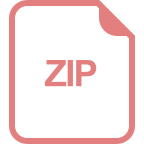
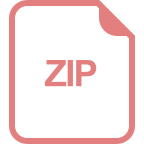
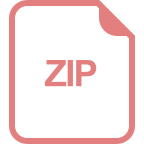
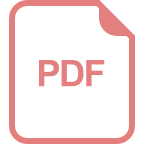
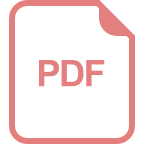
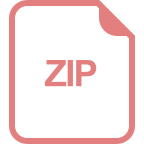
收起资源包目录


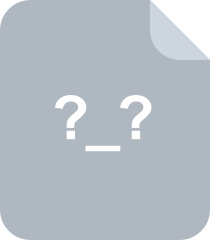





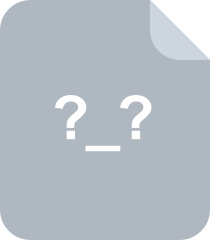




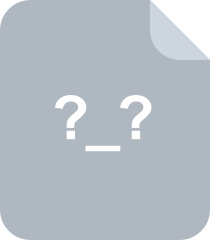

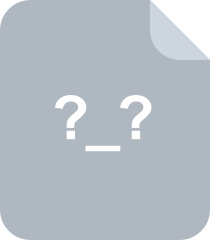
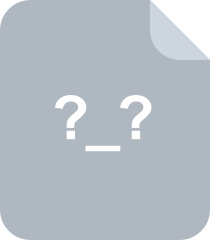

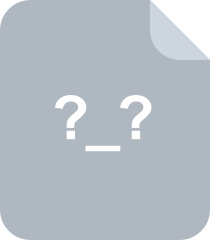

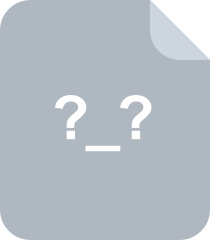
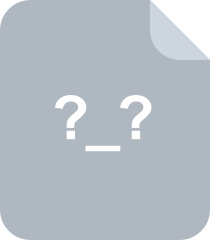

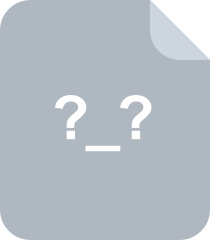
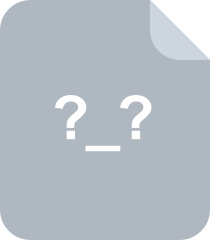
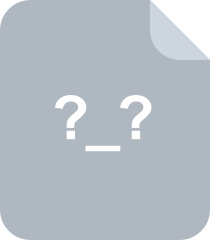
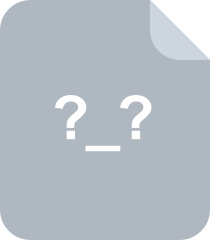
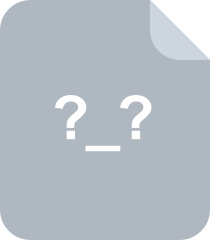
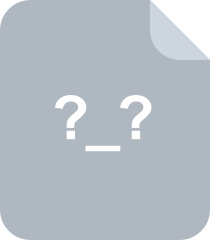
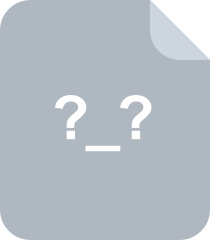
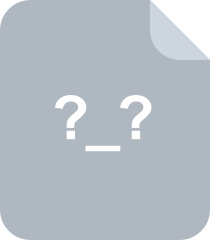
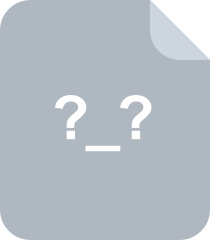
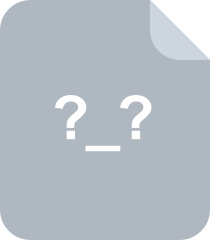
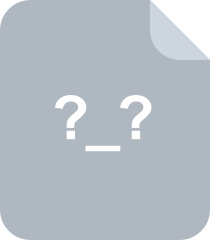
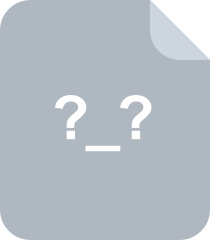
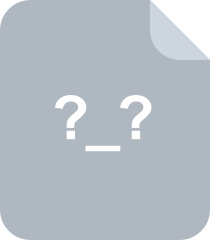
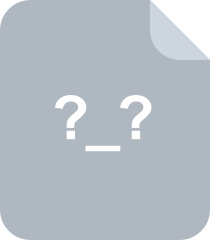
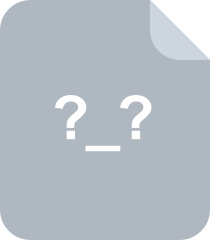
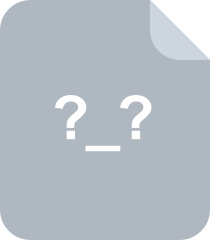
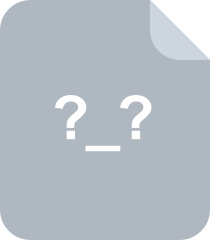
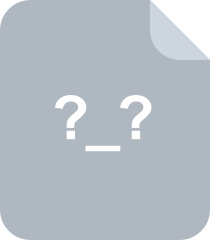
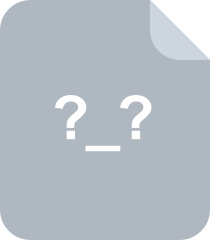
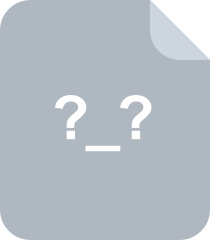
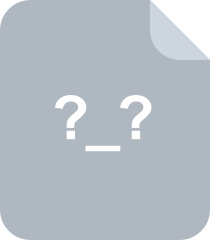
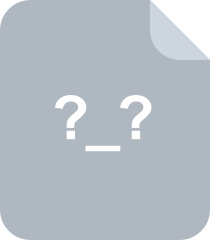
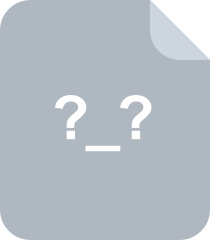
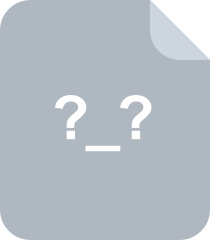
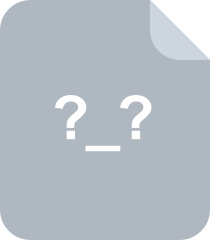
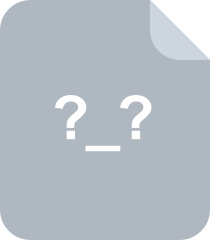
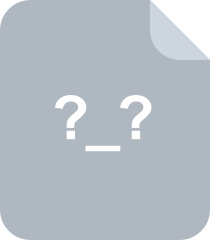
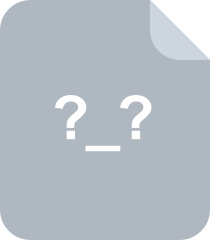
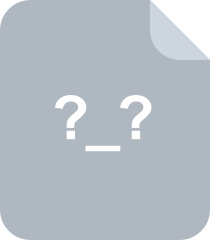
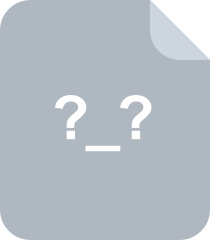
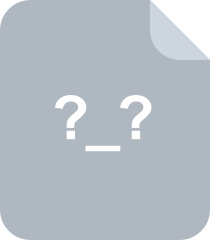
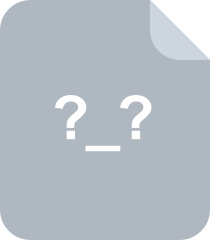
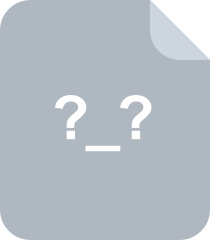
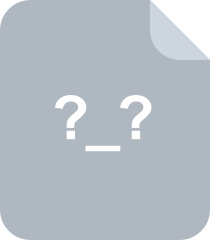
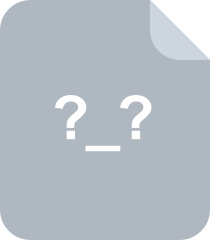
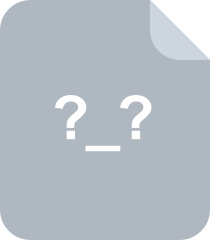
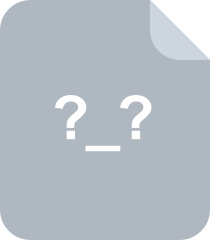
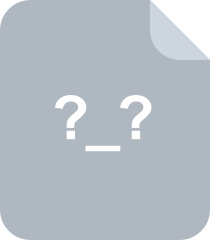
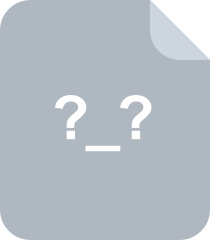
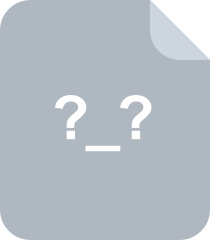
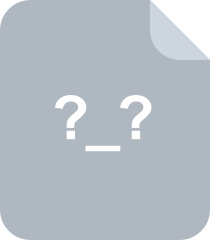
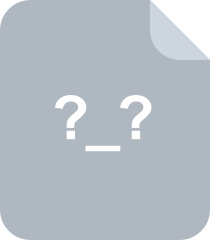
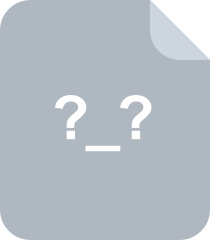
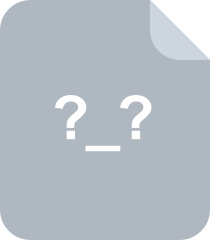
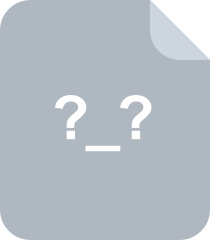
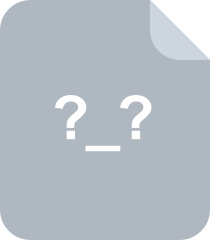
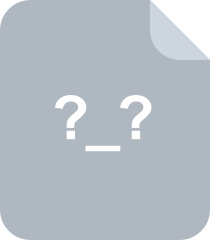
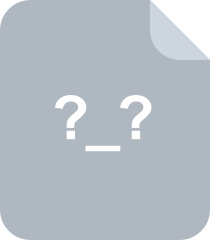
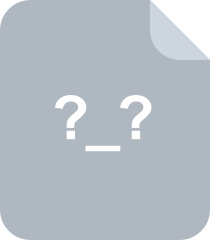


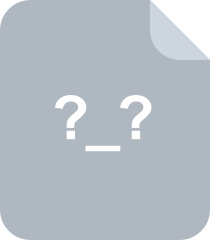


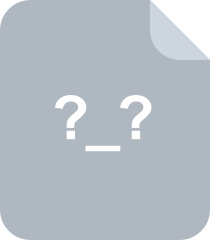

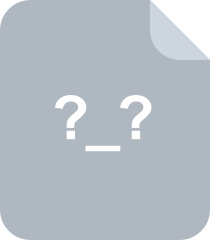

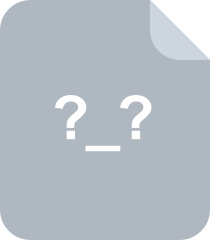
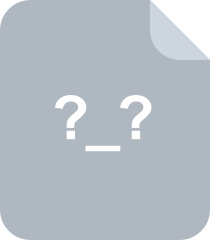

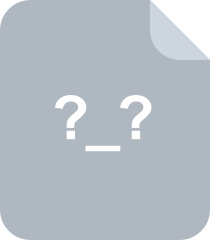
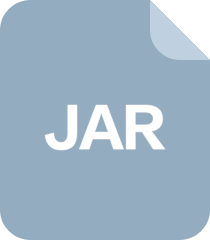





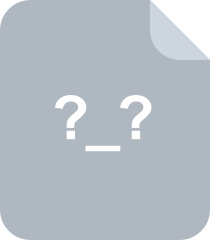
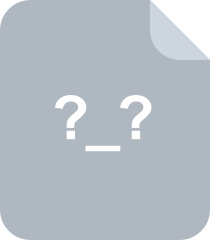


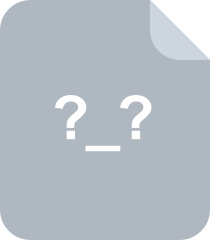
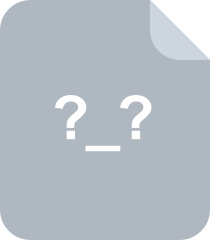

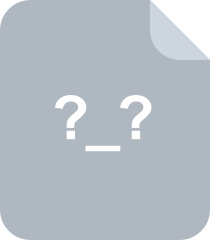




共 69 条
- 1
资源评论
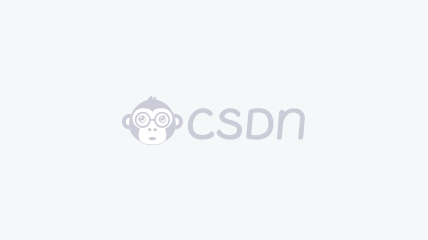

天罡gg
- 粉丝: 6w+
- 资源: 20
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

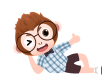
最新资源
- 操作系统实验ucore lab3
- DG储能选址定容模型matlab 程序采用改进粒子群算法,考虑时序性得到分布式和储能的选址定容模型,程序运行可靠 这段程序是一个改进的粒子群算法,主要用于解决电力系统中的优化问题 下面我将对程序进行详
- final_work_job1(1).sql
- 区块链与联邦学习结合:FedChain项目详细复现指南
- 西门子S7 和 S7 Plus 协议开发示例
- 模块化多电平变流器 MMC 的VSG控制 同步发电机控制 MATLAB–Simulink仿真模型 5电平三相MMC,采用VSG控制 受端接可编辑三相交流源,直流侧接无穷大电源提供调频能量 设置频率
- 微电网(两台)主从控制孤岛-并网平滑切的分析 分析了: 1.孤岛下VF控制 2.并网下PQ控制 3.孤岛下主从控制 4.孤岛到并网的平滑切控制 5.除模型外还对分布式发电与主动配电网一些常见问题做了
- 第四组二手产品.zip
- 基于小程序的智慧物业平台源代码(java+小程序+mysql+LW).zip
- MVIMG_20241222_194113.jpg
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


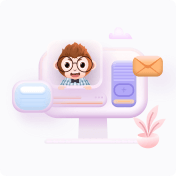
安全验证
文档复制为VIP权益,开通VIP直接复制
