/* -*- c-basic-offset: 8 -*-
rdesktop: A Remote Desktop Protocol client.
User interface services - X Window System
Copyright (C) Matthew Chapman <matthewc.unsw.edu.au> 1999-2008
Copyright 2007-2008 Pierre Ossman <ossman@cendio.se> for Cendio AB
Copyright 2002-2011 Peter Astrand <astrand@cendio.se> for Cendio AB
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
#include <X11/Xlib.h>
#include <X11/Xutil.h>
#include <X11/Xproto.h>
#include <unistd.h>
#include <sys/time.h>
#include <time.h>
#include <errno.h>
#include <strings.h>
#include "rdesktop.h"
#include "xproto.h"
#ifdef HAVE_XRANDR
#include <X11/extensions/Xrandr.h>
#endif
extern int g_sizeopt;
extern int g_width;
extern int g_height;
extern int g_xpos;
extern int g_ypos;
extern int g_pos;
extern RD_BOOL g_sendmotion;
extern RD_BOOL g_fullscreen;
extern RD_BOOL g_grab_keyboard;
extern RD_BOOL g_hide_decorations;
extern RD_BOOL g_pending_resize;
extern char g_title[];
/* Color depth of the RDP session.
As of RDP 5.1, it may be 8, 15, 16 or 24. */
extern int g_server_depth;
extern int g_win_button_size;
Display *g_display;
Time g_last_gesturetime;
static int g_x_socket;
static Screen *g_screen;
Window g_wnd;
/* SeamlessRDP support */
typedef struct _seamless_group
{
Window wnd;
unsigned long id;
unsigned int refcnt;
} seamless_group;
typedef struct _seamless_window
{
Window wnd;
unsigned long id;
unsigned long behind;
seamless_group *group;
int xoffset, yoffset;
int width, height;
int state; /* normal/minimized/maximized. */
unsigned int desktop;
struct timeval *position_timer;
RD_BOOL outstanding_position;
unsigned int outpos_serial;
int outpos_xoffset, outpos_yoffset;
int outpos_width, outpos_height;
unsigned int icon_size;
unsigned int icon_offset;
char icon_buffer[32 * 32 * 4];
struct _seamless_window *next;
} seamless_window;
static seamless_window *g_seamless_windows = NULL;
static unsigned long g_seamless_focused = 0;
static RD_BOOL g_seamless_started = False; /* Server end is up and running */
static RD_BOOL g_seamless_active = False; /* We are currently in seamless mode */
static RD_BOOL g_seamless_hidden = False; /* Desktop is hidden on server */
static RD_BOOL g_seamless_broken_restack = False; /* WM does not properly restack */
extern RD_BOOL g_seamless_rdp;
extern uint32 g_embed_wnd;
RD_BOOL g_enable_compose = False;
RD_BOOL g_Unobscured; /* used for screenblt */
static GC g_gc = NULL;
static GC g_create_bitmap_gc = NULL;
static GC g_create_glyph_gc = NULL;
static XRectangle g_clip_rectangle;
static Visual *g_visual;
/* Color depth of the X11 visual of our window (e.g. 24 for True Color R8G8B visual).
This may be 32 for R8G8B8 visuals, and then the rest of the bits are undefined
as far as we're concerned. */
static int g_depth;
/* Bits-per-Pixel of the pixmaps we'll be using to draw on our window.
This may be larger than g_depth, in which case some of the bits would
be kept solely for alignment (e.g. 32bpp pixmaps on a 24bpp visual). */
static int g_bpp;
static XIM g_IM;
static XIC g_IC;
static XModifierKeymap *g_mod_map;
/* Maps logical (xmodmap -pp) pointing device buttons (0-based) back
to physical (1-based) indices. */
static unsigned char g_pointer_log_to_phys_map[32];
static Cursor g_current_cursor;
static RD_HCURSOR g_null_cursor = NULL;
static Atom g_protocol_atom, g_kill_atom;
extern Atom g_net_wm_state_atom;
extern Atom g_net_wm_desktop_atom;
static RD_BOOL g_focused;
static RD_BOOL g_mouse_in_wnd;
/* Indicates that:
1) visual has 15, 16 or 24 depth and the same color channel masks
as its RDP equivalent (implies X server is LE),
2) host is LE
This will trigger an optimization whose real value is questionable.
*/
static RD_BOOL g_compatible_arch;
/* Indicates whether RDP's bitmaps and our XImages have the same
binary format. If so, we can avoid an expensive translation.
Note that this can be true when g_compatible_arch is false,
e.g.:
RDP(LE) <-> host(BE) <-> X-Server(LE)
('host' is the machine running rdesktop; the host simply memcpy's
so its endianess doesn't matter)
*/
static RD_BOOL g_no_translate_image = False;
/* endianness */
static RD_BOOL g_host_be;
static RD_BOOL g_xserver_be;
static int g_red_shift_r, g_blue_shift_r, g_green_shift_r;
static int g_red_shift_l, g_blue_shift_l, g_green_shift_l;
/* software backing store */
extern RD_BOOL g_ownbackstore;
static Pixmap g_backstore = 0;
/* Moving in single app mode */
static RD_BOOL g_moving_wnd;
static int g_move_x_offset = 0;
static int g_move_y_offset = 0;
static RD_BOOL g_using_full_workarea = False;
/* MWM decorations */
#define MWM_HINTS_DECORATIONS (1L << 1)
#define PROP_MOTIF_WM_HINTS_ELEMENTS 5
typedef struct
{
unsigned long flags;
unsigned long functions;
unsigned long decorations;
long inputMode;
unsigned long status;
}
PropMotifWmHints;
typedef struct
{
uint32 red;
uint32 green;
uint32 blue;
}
PixelColour;
#define ON_ALL_SEAMLESS_WINDOWS(func, args) \
do { \
seamless_window *sw; \
XRectangle rect; \
if (!g_seamless_windows) break; \
for (sw = g_seamless_windows; sw; sw = sw->next) { \
rect.x = g_clip_rectangle.x - sw->xoffset; \
rect.y = g_clip_rectangle.y - sw->yoffset; \
rect.width = g_clip_rectangle.width; \
rect.height = g_clip_rectangle.height; \
XSetClipRectangles(g_display, g_gc, 0, 0, &rect, 1, YXBanded); \
func args; \
} \
XSetClipRectangles(g_display, g_gc, 0, 0, &g_clip_rectangle, 1, YXBanded); \
} while (0)
static void
seamless_XFillPolygon(Drawable d, XPoint * points, int npoints, int xoffset, int yoffset)
{
points[0].x -= xoffset;
points[0].y -= yoffset;
XFillPolygon(g_display, d, g_gc, points, npoints, Complex, CoordModePrevious);
points[0].x += xoffset;
points[0].y += yoffset;
}
static void
seamless_XDrawLines(Drawable d, XPoint * points, int npoints, int xoffset, int yoffset)
{
points[0].x -= xoffset;
points[0].y -= yoffset;
XDrawLines(g_display, d, g_gc, points, npoints, CoordModePrevious);
points[0].x += xoffset;
points[0].y += yoffset;
}
#define FILL_RECTANGLE(x,y,cx,cy)\
{ \
XFillRectangle(g_display, g_wnd, g_gc, x, y, cx, cy); \
ON_ALL_SEAMLESS_WINDOWS(XFillRectangle, (g_display, sw->wnd, g_gc, x-sw->xoffset, y-sw->yoffset, cx, cy)); \
if (g_ownbackstore) \
XFillRectangle(g_display, g_backstore, g_gc, x, y, cx, cy); \
}
#define FILL_RECTANGLE_BACKSTORE(x,y,cx,cy)\
{ \
XFillRectangle(g_display, g_ownbackstore ? g_backstore : g_wnd, g_gc, x, y, cx, cy); \
}
#define FILL_POLYGON(p,np)\
{ \
XFillPolygon(g_display, g_wnd, g_gc, p, np, Complex, CoordModePrevious); \
if (g_ownbackstore) \
XFillPolygon(g_display, g_backstore, g_gc, p, np, Complex, CoordModePrevious); \
ON_ALL_SEAMLESS_WINDOWS(seamless_XFillPolygon, (sw->wnd, p, np, sw->xoffset, sw->yoffset)); \
}
#define DRAW_ELLIPSE(x,y,cx,cy,m)\
{ \
switch (m) \
{ \
case 0: /* Outline */ \
XDrawArc(g_display, g_wnd, g_gc, x, y, cx, cy, 0, 360*64); \
ON_ALL_SEAMLESS_WINDOWS(XDrawArc, (g_display, sw->wnd, g_gc, x-sw->xoffset, y-sw->yoffset, cx, cy, 0, 360*64)); \
if (g_ownbackstore) \
XDrawArc(g_display, g_backstore, g_gc, x, y, cx, cy, 0, 360*64); \
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
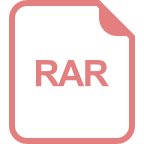
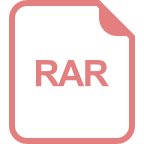
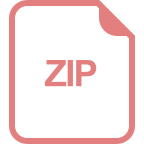
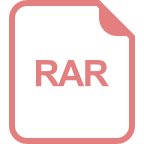
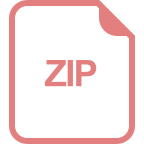
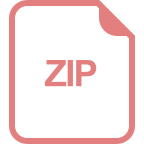
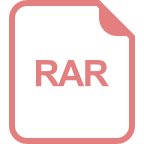
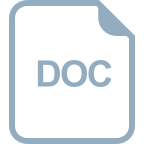
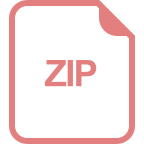
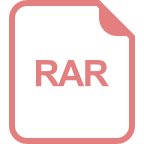
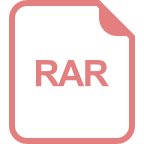
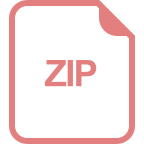
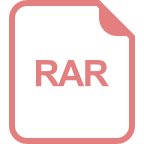
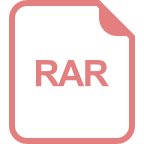
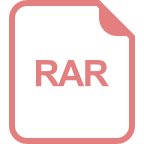
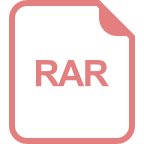
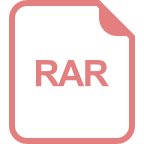
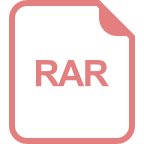
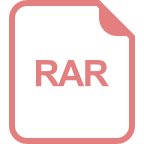
收起资源包目录

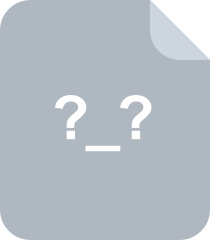
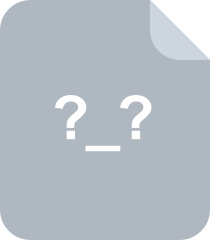
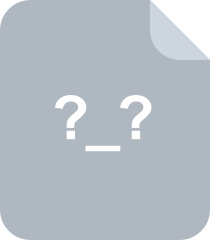
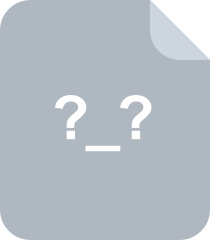
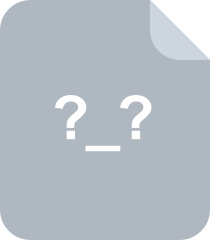
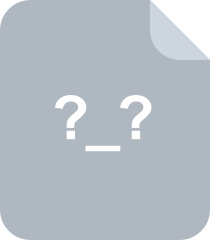
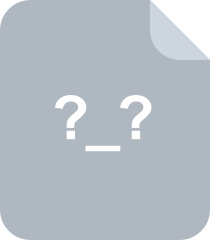
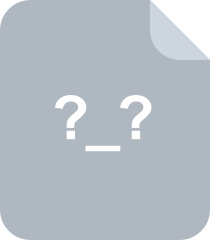
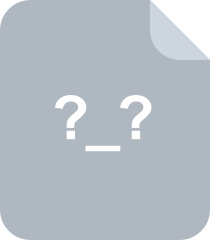
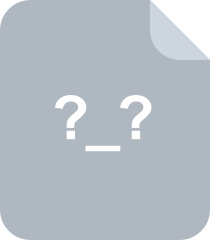
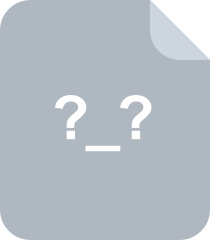
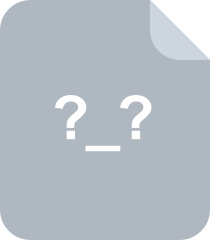
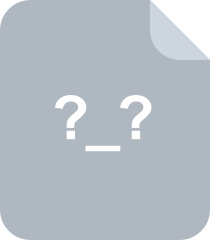
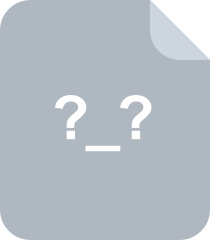
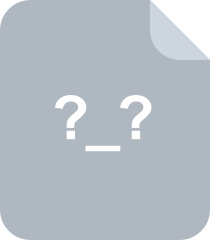
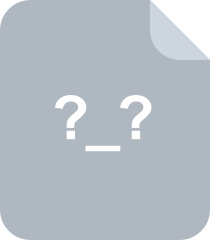
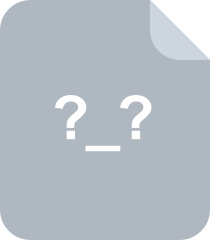
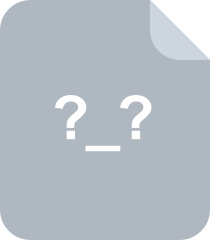
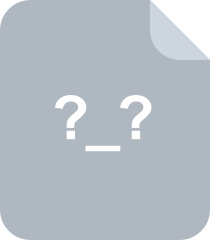
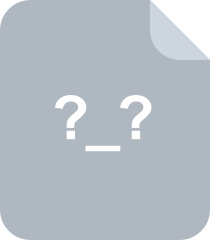
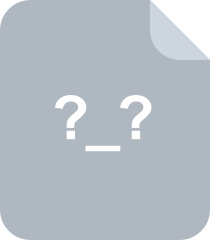
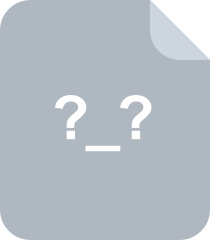
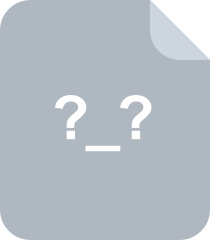
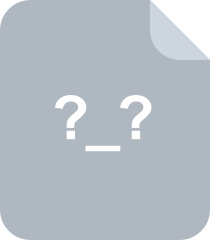
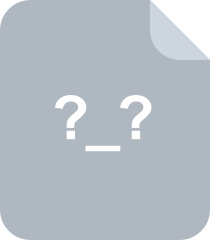
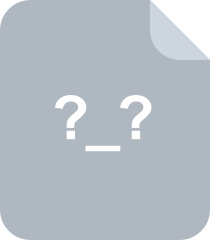
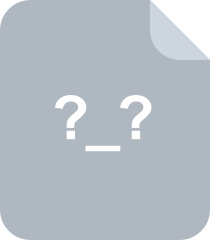
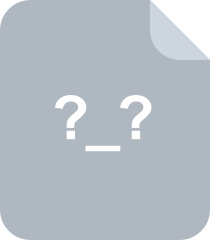
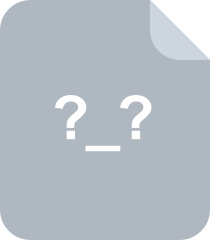
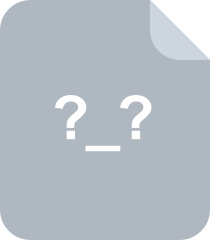
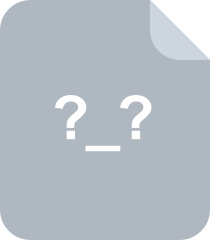
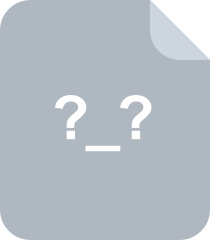
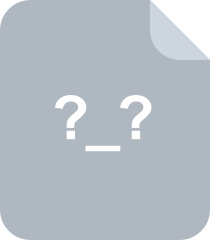
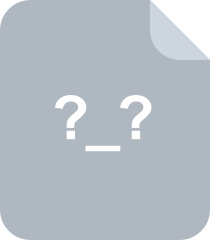
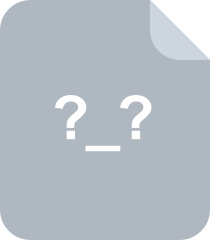
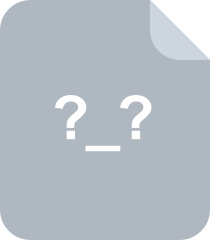
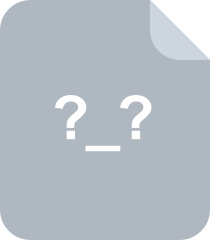
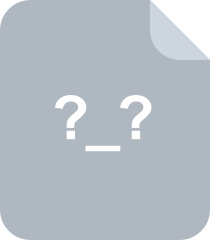
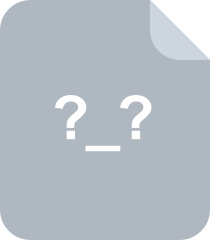
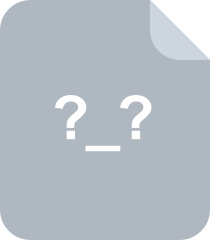
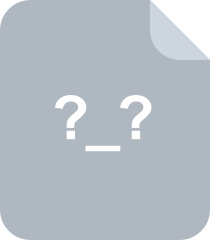
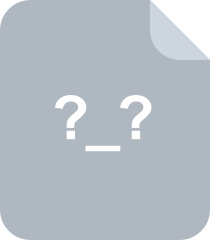
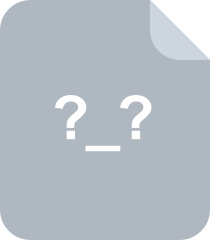
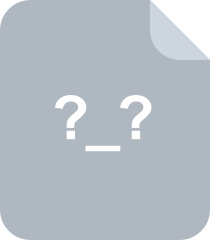
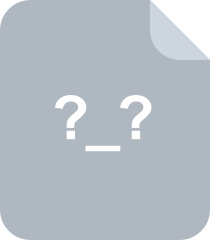
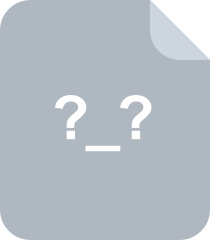
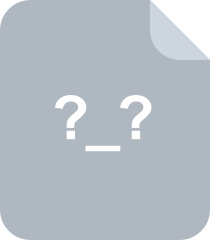
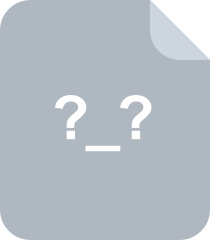
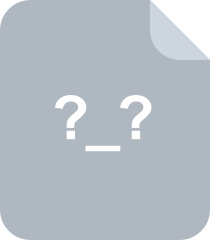
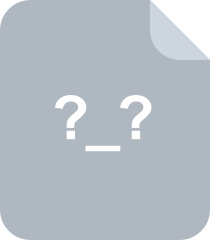
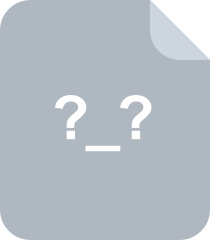
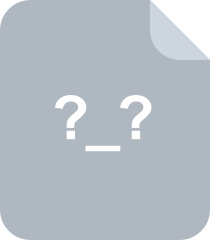
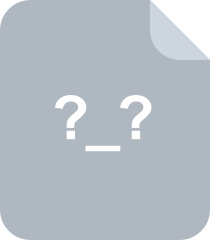
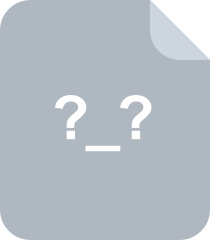
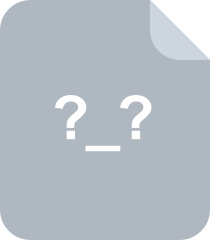
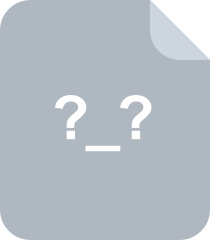
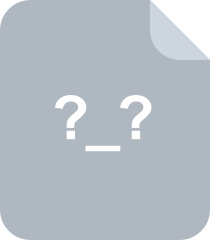
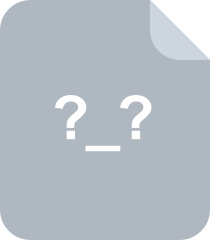
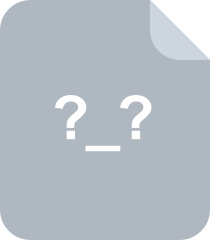
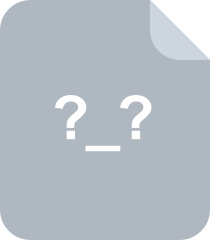
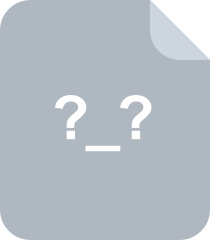
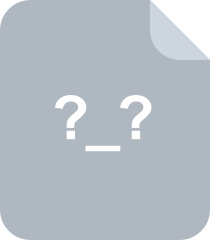
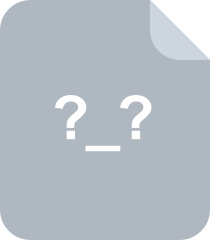
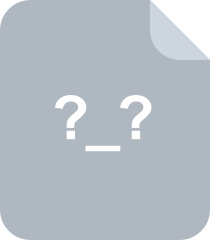
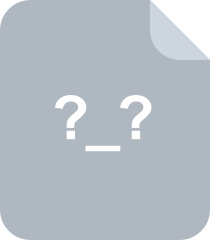
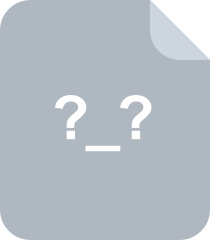
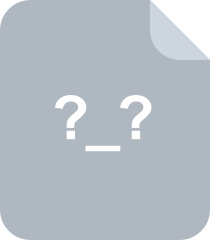
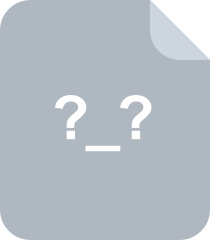
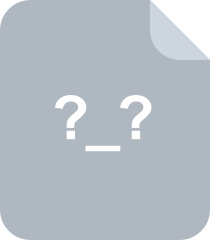
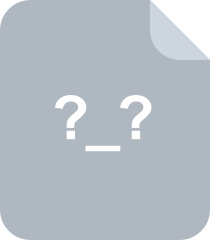
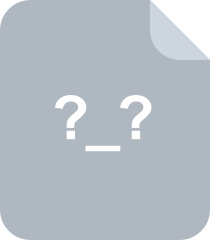
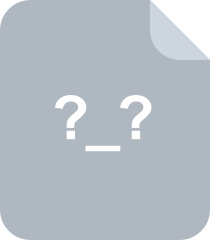
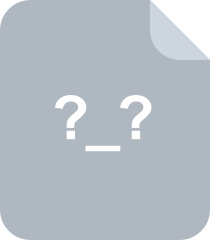
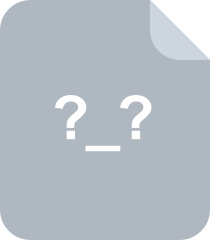
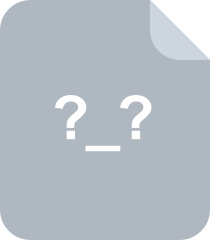
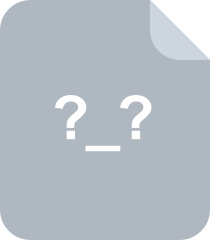
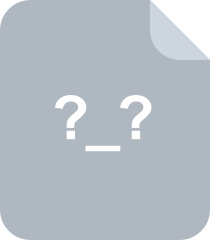
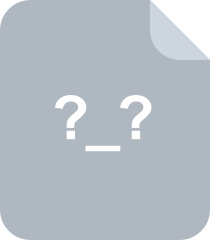
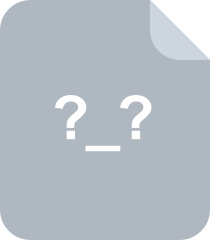
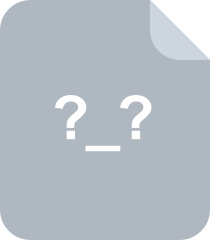
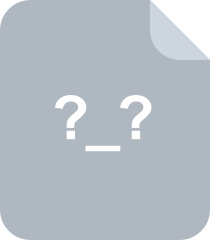
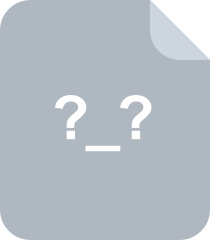
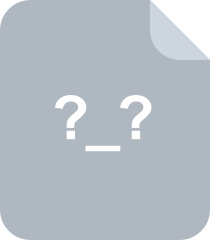
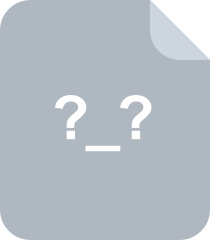
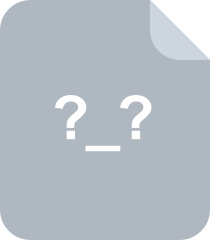
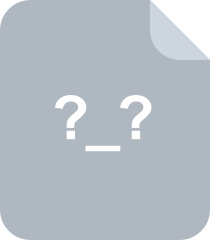
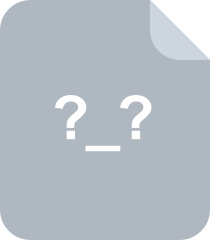
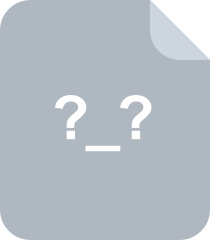
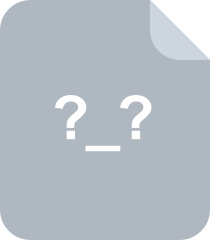
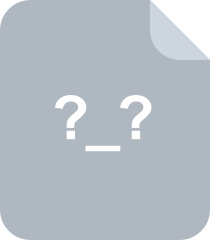
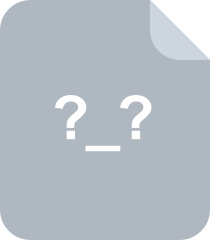
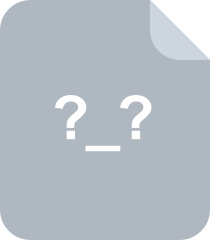
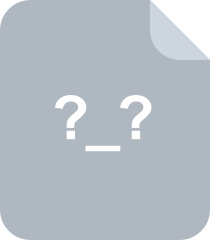
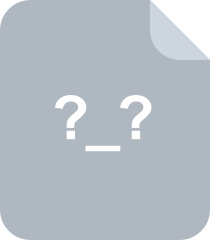
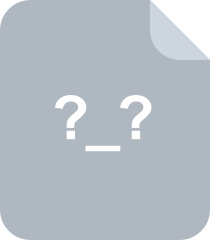
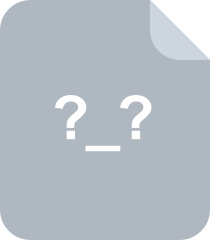
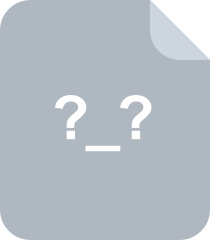
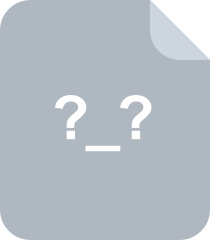
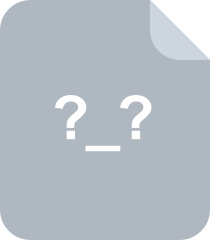
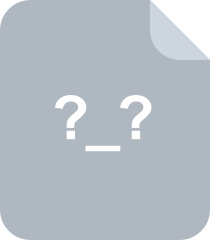
共 112 条
- 1
- 2

宝庆陈
- 粉丝: 7
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

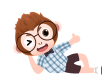
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


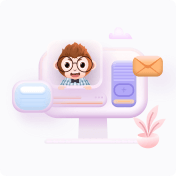
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页