<img src="https://github.com/AtsushiSakai/PythonRobotics/raw/master/icon.png?raw=true" align="right" width="300" alt="header pic"/>
# PythonRobotics



[](https://ci.appveyor.com/project/AtsushiSakai/pythonrobotics)
[](https://codecov.io/gh/AtsushiSakai/PythonRobotics)
Python codes for robotics algorithm.
# Table of Contents
* [What is this?](#what-is-this)
* [Requirements](#requirements)
* [Documentation](#documentation)
* [How to use](#how-to-use)
* [Localization](#localization)
* [Extended Kalman Filter localization](#extended-kalman-filter-localization)
* [Particle filter localization](#particle-filter-localization)
* [Histogram filter localization](#histogram-filter-localization)
* [Mapping](#mapping)
* [Gaussian grid map](#gaussian-grid-map)
* [Ray casting grid map](#ray-casting-grid-map)
* [Lidar to grid map](#lidar-to-grid-map)
* [k-means object clustering](#k-means-object-clustering)
* [Rectangle fitting](#rectangle-fitting)
* [SLAM](#slam)
* [Iterative Closest Point (ICP) Matching](#iterative-closest-point-icp-matching)
* [FastSLAM 1.0](#fastslam-10)
* [Path Planning](#path-planning)
* [Dynamic Window Approach](#dynamic-window-approach)
* [Grid based search](#grid-based-search)
* [Dijkstra algorithm](#dijkstra-algorithm)
* [A* algorithm](#a-algorithm)
* [D* algorithm](#d-algorithm)
* [D* Lite algorithm](#d-lite-algorithm)
* [Potential Field algorithm](#potential-field-algorithm)
* [Grid based coverage path planning](#grid-based-coverage-path-planning)
* [State Lattice Planning](#state-lattice-planning)
* [Biased polar sampling](#biased-polar-sampling)
* [Lane sampling](#lane-sampling)
* [Probabilistic Road-Map (PRM) planning](#probabilistic-road-map-prm-planning)
* [Rapidly-Exploring Random Trees (RRT)](#rapidly-exploring-random-trees-rrt)
* [RRT*](#rrt)
* [RRT* with reeds-shepp path](#rrt-with-reeds-shepp-path)
* [LQR-RRT*](#lqr-rrt)
* [Quintic polynomials planning](#quintic-polynomials-planning)
* [Reeds Shepp planning](#reeds-shepp-planning)
* [LQR based path planning](#lqr-based-path-planning)
* [Optimal Trajectory in a Frenet Frame](#optimal-trajectory-in-a-frenet-frame)
* [Path Tracking](#path-tracking)
* [move to a pose control](#move-to-a-pose-control)
* [Stanley control](#stanley-control)
* [Rear wheel feedback control](#rear-wheel-feedback-control)
* [Linear–quadratic regulator (LQR) speed and steering control](#linearquadratic-regulator-lqr-speed-and-steering-control)
* [Model predictive speed and steering control](#model-predictive-speed-and-steering-control)
* [Nonlinear Model predictive control with C-GMRES](#nonlinear-model-predictive-control-with-c-gmres)
* [Arm Navigation](#arm-navigation)
* [N joint arm to point control](#n-joint-arm-to-point-control)
* [Arm navigation with obstacle avoidance](#arm-navigation-with-obstacle-avoidance)
* [Aerial Navigation](#aerial-navigation)
* [drone 3d trajectory following](#drone-3d-trajectory-following)
* [rocket powered landing](#rocket-powered-landing)
* [Bipedal](#bipedal)
* [bipedal planner with inverted pendulum](#bipedal-planner-with-inverted-pendulum)
* [License](#license)
* [Use-case](#use-case)
* [Contribution](#contribution)
* [Citing](#citing)
* [Support](#support)
* [Sponsors](#sponsors)
* [JetBrains](#JetBrains)
* [1Password](#1password)
* [Authors](#authors)
# What is this?
This is a Python code collection of robotics algorithms.
Features:
1. Easy to read for understanding each algorithm's basic idea.
2. Widely used and practical algorithms are selected.
3. Minimum dependency.
See this paper for more details:
- [\[1808\.10703\] PythonRobotics: a Python code collection of robotics algorithms](https://arxiv.org/abs/1808.10703) ([BibTeX](https://github.com/AtsushiSakai/PythonRoboticsPaper/blob/master/python_robotics.bib))
# Requirements
For running each sample code:
- [Python 3.12.x](https://www.python.org/)
- [NumPy](https://numpy.org/)
- [SciPy](https://scipy.org/)
- [Matplotlib](https://matplotlib.org/)
- [cvxpy](https://www.cvxpy.org/)
For development:
- [pytest](https://pytest.org/) (for unit tests)
- [pytest-xdist](https://pypi.org/project/pytest-xdist/) (for parallel unit tests)
- [mypy](http://mypy-lang.org/) (for type check)
- [sphinx](https://www.sphinx-doc.org/) (for document generation)
- [pycodestyle](https://pypi.org/project/pycodestyle/) (for code style check)
# Documentation
This README only shows some examples of this project.
If you are interested in other examples or mathematical backgrounds of each algorithm,
You can check the full documentation online: [Welcome to PythonRobotics’s documentation\! — PythonRobotics documentation](https://atsushisakai.github.io/PythonRobotics/index.html)
All animation gifs are stored here: [AtsushiSakai/PythonRoboticsGifs: Animation gifs of PythonRobotics](https://github.com/AtsushiSakai/PythonRoboticsGifs)
# How to use
1. Clone this repo.
```terminal
git clone https://github.com/AtsushiSakai/PythonRobotics.git
```
2. Install the required libraries.
- using conda :
```terminal
conda env create -f requirements/environment.yml
```
- using pip :
```terminal
pip install -r requirements/requirements.txt
```
3. Execute python script in each directory.
4. Add star to this repo if you like it :smiley:.
# Localization
## Extended Kalman Filter localization
<img src="https://github.com/AtsushiSakai/PythonRoboticsGifs/raw/master/Localization/extended_kalman_filter/animation.gif" width="640" alt="EKF pic">
Ref:
- [documentation](https://atsushisakai.github.io/PythonRobotics/modules/localization/extended_kalman_filter_localization_files/extended_kalman_filter_localization.html)
## Particle filter localization

This is a sensor fusion localization with Particle Filter(PF).
The blue line is true trajectory, the black line is dead reckoning trajectory,
and the red line is an estimated trajectory with PF.
It is assumed that the robot can measure a distance from landmarks (RFID).
These measurements are used for PF localization.
Ref:
- [PROBABILISTIC ROBOTICS](http://www.probabilistic-robotics.org/)
## Histogram filter localization

This is a 2D localization example with Histogram filter.
The red cross is true position, black points are RFID positions.
The blue grid shows a position probability of histogram filter.
In this simulation, x,y are unknown, yaw is known.
The filter integrates speed input and range observations from RFID for localization.
Initial position is not needed.
Ref:
- [PROBABILISTIC ROBOTICS](http://www.probabilistic-robotics.org/)
# Mapping
## Gaussian grid map
This is a 2D Gaussian grid mapping example.

## Ray casting grid map
This is a 2D ray casting grid mapping example.

yava_free
- 粉丝: 5319
- 资源: 2084
最新资源
- 音圈电机控制,双闭环pid控制
- 北方苍鹰优化算法(NGO)求解混合储能容量配置经济成本 2022年新算法哦 1、微电网混合储能容量配置优化 适合(光伏、风电)模型,(有参考文献) 2、目标经济性、可靠性负荷失电率, 3、matl
- comsol线偏振转多重干涉
- 固高GTS8轴或4轴控制卡,视觉点胶涂覆,伺服运动控制
- comsol声学超材料 吸隔声仿真计算模型可以个人定制任意声学模型
- 大厂FPGA AXI verilog源代码,4个master+10个slave配置,企业级应用源码,适合需要学习ic设计验证及soc开发的工程师 提供databook资料和verilog完整源代码
- comsol光子晶体波导法诺共振
- comsol模型 堤坝边坡稳定性 利用流固耦合接口
- C#开发上位机控制系统 主控界面,可以PLC通讯,可以接入485通讯 可多样形成工艺编辑界面,避免Excel表格的繁琐 曲线显示美观,纵横坐标可以放缩,游标可以显示具体数值
- a星+动态窗口法的融合路径规划算法 可自行更改地图,定义起始点目标点位置、未知障碍物位置 matlab实现
- comsol相控阵三维聚焦探头 7*7阵元三维相控阵聚焦探头,焦点大概在20mm位置处,介质为水,频率设的0.5MHz,焦距可调 效果看动画吧 图1 2 3分别为剖面下的声场收缩 聚焦 扩散时刻图
- comsol不同温度下相变材料二氧化钒VO2设置 包含可见光近红外太赫兹波段
- 光伏并网 单相 三相 逆变 lcl 仿真 光伏并网 研究电能质量 有源滤波器 谐波检测 功率因数 光伏对配电网继电保护影响 5kw光伏并网逆变器的设计 本硕电气工程
- stm32低压无感BLDC方波控制方案 1.启动传统三段式,强拖的步数少,启动快,任意电机基本可以顺利启动切闭环; 2.配有英非凌电感法,脉冲注入算法; 3.开环,速度环,限流环; 4.欠压,过压
- SMT轨迹导入程序,C#导入CAD的DXF文件,生成G代码,
- Matlab光伏加蓄电池发电系统simulink仿真模型
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


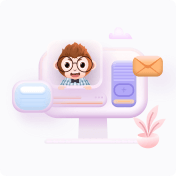