package com.javapandeng.utils;
import java.sql.Timestamp;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.ParsePosition;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
/**
* 日期格式化类
*/
public class DateUtil extends java.util.Date {
private static final long serialVersionUID = 1L;
private static final DateFormat datetimeFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
private static final DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
private static final String strFormat1 = "yyyy-MM-dd HH:mm";
/**
M 年中的月份 Month July; Jul; 07
w 年中的周数 Number 27
W 月份中的周数 Number 2
D 年中的天数 Number 189
d 月份中的天数 Number 10
F 月份中的星期 Number 2
E 星期中的天数 Text Tuesday; Tue
a Am/pm 标记 Text PM
H 一天中的小时数(0-23) Number 0
k 一天中的小时数(1-24) Number 24
K am/pm 中的小时数(0-11) Number 0
h am/pm 中的小时数(1-12) Number 12
m 小时中的分钟数 Number 30
s 分钟中的秒数 Number 55
S 毫秒数 Number 978
z 时区 General time zone Pacific Standard Time; PST; GMT-08:00
Z 时区 RFC 822 time zone -0800
*/
/**
* 构造函数
*/
public DateUtil() {
super(getSystemDate().getTime().getTime());
}
/**
* 当前时间
*
* @return 时间Timestamp
*/
public java.sql.Timestamp parseTime() {
return new java.sql.Timestamp(this.getTime());
}
/**
* 将Date类型转换为字符串 yyyy-MM-dd HH:mm:ss
*
* @param Date
* @return String
*/
public static String format(Date date) {
return format(date, null);
}
/**
* 将Date类型转换为字符串
*
* @param Date
* @param pattern 字符串格式
* @return String
*/
public static String format(Date date, String pattern) {
if (date == null) {
return "";
} else if (pattern == null || pattern.equals("") || pattern.equals("null")) {
return datetimeFormat.format(date);
} else {
return new SimpleDateFormat(pattern).format(date);
}
}
/**
* 将Date类型转换为字符串 yyyy-MM-dd
*
* @param Date
* @return String
*/
public static String formatDate(Date date) {
if (date == null) {
return "";
}
return dateFormat.format(date);
}
/**
* 将字符串转换为Date类型
*
* @param sDate yyyy-MM-dd HH:mm:ss
* @return
*/
public static Date convert(String sDate) {
try {
if (sDate != null) {
if (sDate.length() == 10) {
return dateFormat.parse(sDate);
} else if (sDate.length() == 19) {
return datetimeFormat.parse(sDate);
}
}
} catch (ParseException pe) {
}
return convert(sDate, null);
}
/**
* 将字符串转换为Date类型
*
* @param sDate
* @param pattern 格式
* @return
*/
public static Date convert(String sDate, String pattern) {
Date date = null;
try {
if (sDate == null || sDate.equals("") || sDate.equals("null")) {
return null;
} else if (pattern == null || pattern.equals("") || pattern.equals("null")) {
return datetimeFormat.parse(sDate);
} else {
return new SimpleDateFormat(pattern).parse(sDate);
}
} catch (ParseException pe) {
}
return date;
}
/**
* String转换为Date
*
* @param sDate 日期"yyyy-MM-dd"
* @return 日期Date
*/
public static Date convertDate(String dateStr) {
return convert(dateStr, "yyyy-MM-dd");
}
/**
* String转换为Timestamp
*
* @param sDate 日期 "yyyy-MM-dd" / "yyyy-MM-dd HH:mm:ss"
* @return 日期Timestamp
*/
public static Timestamp convertTimestamp(String sDate) {
if (sDate.length() == 10) {
sDate = sDate + " 00:00:00";
}
if (sDate.length() == 16) {
sDate = sDate + ":00";
}
return Timestamp.valueOf(sDate);
}
/**
* Date转换为Timestamp
*/
public static Timestamp convert(Date date) {
return new Timestamp(date.getTime());
}
/**
* 取当前日期(yyyy-mm-dd)
*
* @return 时间Timestamp
*/
public static String getTodayDate() {
return formatDate(new Date());
}
/**
* 取当前日期(yyyy-mm-dd hh:mm:ss)
*
* @return 时间Timestamp
*/
public static String getTodayDateTime() {
return format(new Date());
}
/**
* 取得n分钟后的时间
*
* @param date 日期
* @param afterMins
* @return 时间Timestamp
*/
public static Date getAfterMinute(Date date, long afterMins) {
if (date == null)
date = new Date();
long longTime = date.getTime() + afterMins * 60 * 1000;
return new Date(longTime);
}
/**
* add by yinshengming start 2016-4-7
* 取得n秒后的时间
*
* @param date 日期
* @param afterMins
* @return 时间Timestamp
*/
public static Date getAfterSecond(Date date, long afterMins) {
if (date == null)
date = new Date();
long longTime = date.getTime() + afterMins * 1000;
return new Date(longTime);
}
// public static void main(String[] arg) {
// System.err.println(format((new Date())));
// System.err.println(format(getAfterMinute(new Date(), 3)));
// }
/**
* 取得指定日期几天后的日期
*
* @param date 日期
* @param afterDays 天数
* @return 日期
*/
public static Date getAfterDay(Date date, int afterDays) {
if (date == null)
date = new Date();
GregorianCalendar cal = new GregorianCalendar();
cal.setTime(date);
cal.add(java.util.Calendar.DATE, afterDays);
return cal.getTime();
}
/**
* 取得指定日期几天后的日期
*
* @param sDate 日期 yyyy-MM-dd
* @param afterDays 天数
* @return 日期
*/
public static String getAfterDay(String sDate, int afterDays) {
Date date = convertDate(sDate);
date = getAfterDay(date, afterDays);
return formatDate(date);
}
/**
* 取得指定日期几天前的日期
*
* @param date 日期
* @param beforeDays 天数(大于0)
* @return 日期
*/
public static Date getBeforeDay(Date date, int beforeDays) {
if (date == null)
date = new Date();
GregorianCalendar cal = new GregorianCalendar();
cal.setTime(date);
cal.add(java.util.Calendar.DATE, 0 - Math.abs(beforeDays));
return cal.getTime();
}
/**
* 取得指定日期几天前的日期
*
* @param sDate 日期 yyyy-MM-dd
* @param beforeDays 天数(大于0)
* @return 日期
*/
public static String getBeforeDay(String sDate, int beforeDays) {
Date date = convertDate(sDate);
date = getBeforeDay(date, beforeDays);
return formatDate(date);
}
/**
* 获得几个月后的日期
*
* @param date 日期
* @param afterMonth 月数
* @return 日期Date
没有合适的资源?快使用搜索试试~ 我知道了~
Java实现SSM的商城管理系统源码和数据库.zip
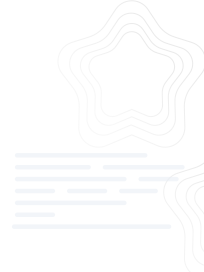
共2005个文件
jpg:457个
png:414个
css:302个

0 下载量 67 浏览量
2024-08-06
17:11:45
上传
评论
收藏 124.82MB ZIP 举报
温馨提示
javaJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJava实现SSM的商城管理系统源码和数据库.zipJa
资源推荐
资源详情
资源评论
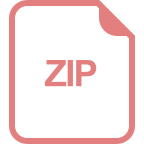
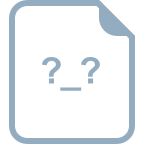
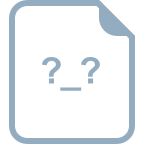
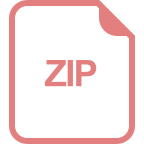
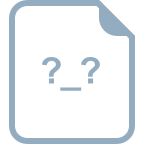
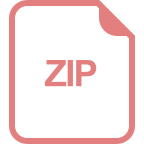
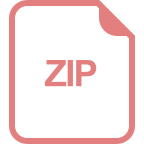
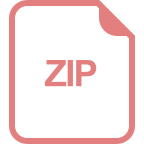
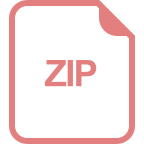
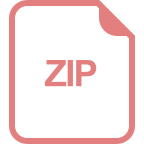
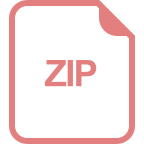
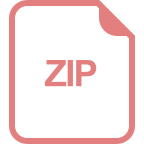
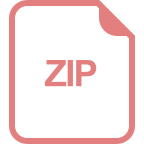
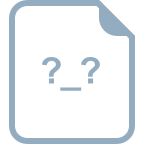
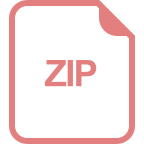
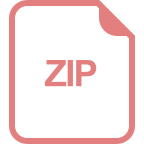
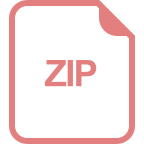
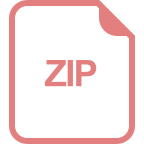
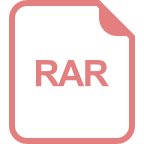
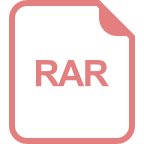
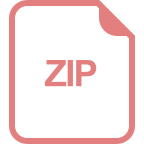
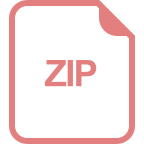
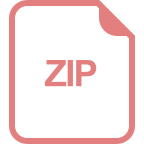
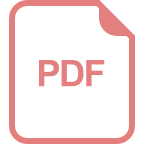
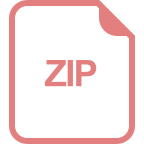
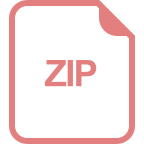
收起资源包目录

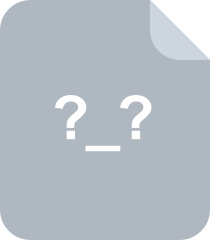
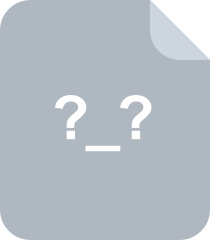
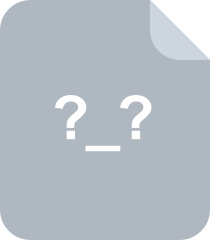
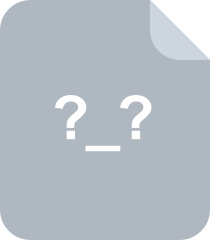
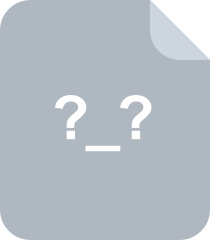
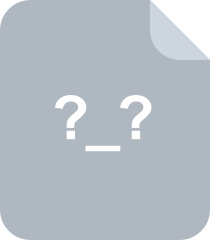
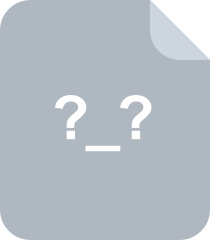
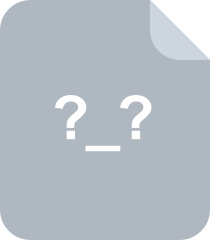
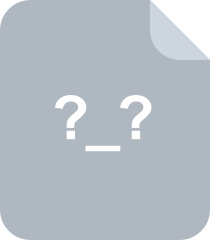
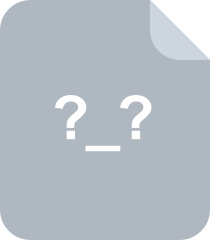
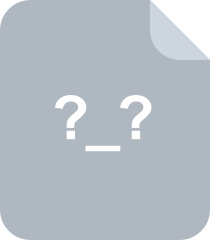
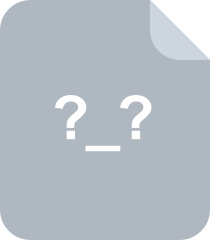
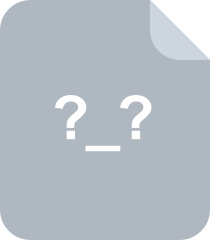
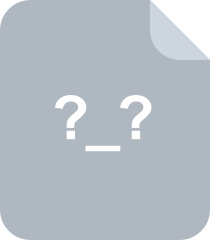
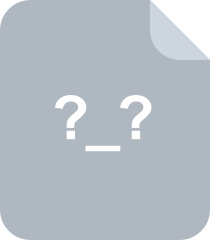
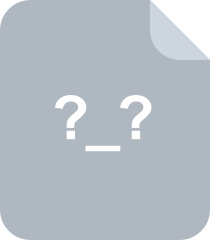
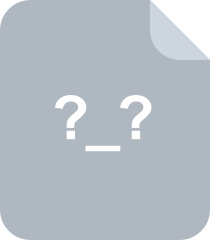
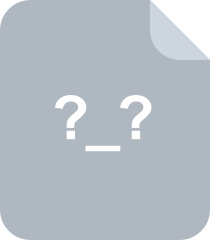
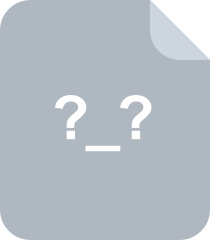
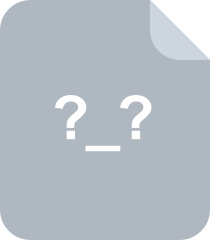
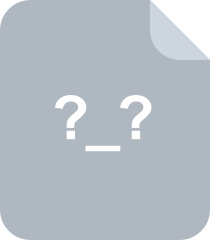
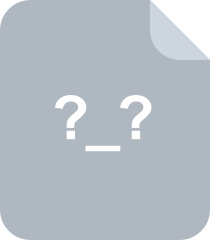
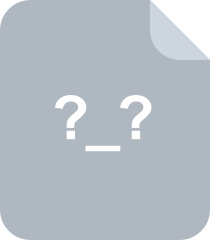
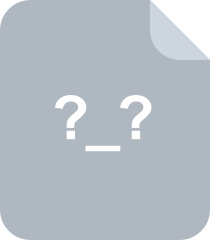
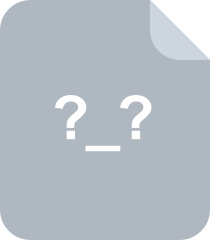
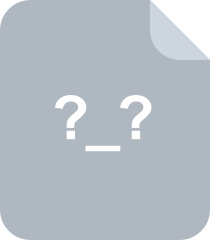
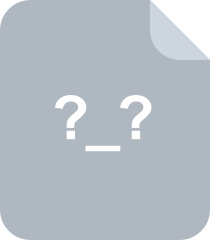
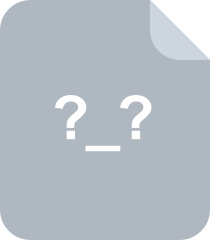
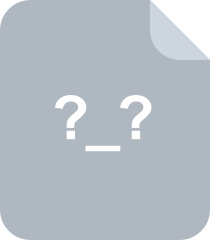
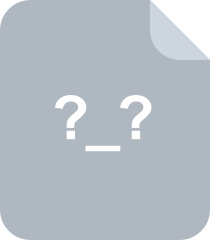
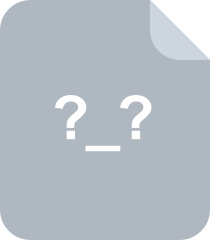
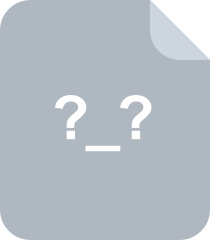
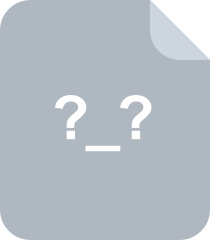
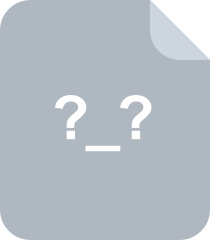
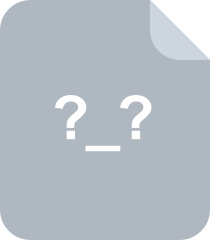
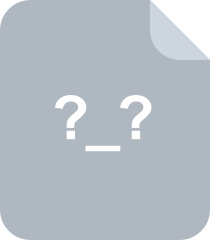
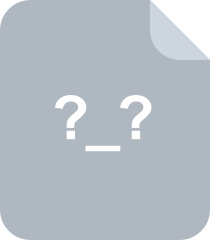
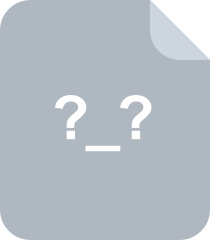
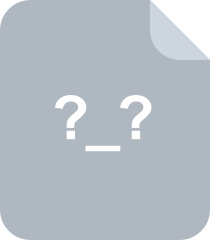
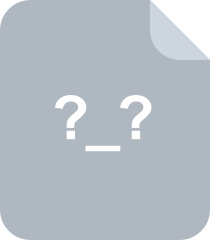
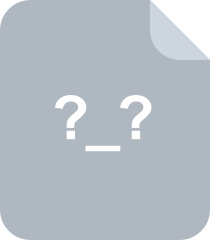
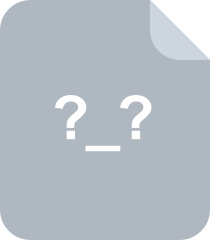
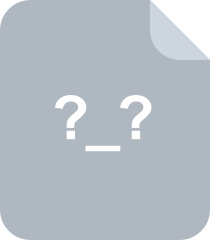
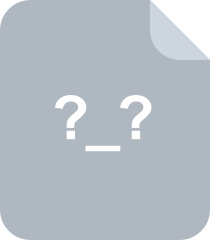
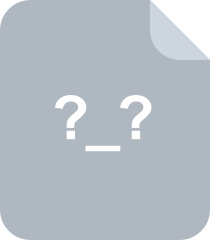
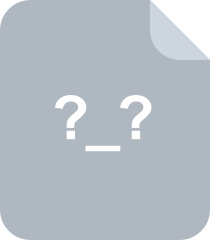
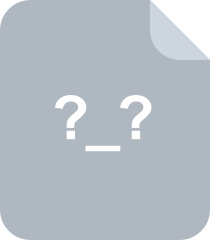
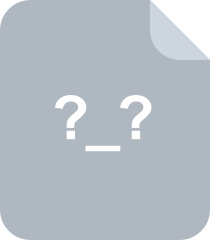
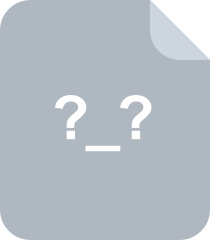
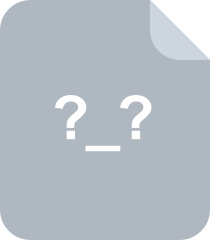
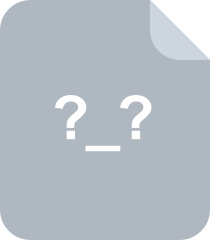
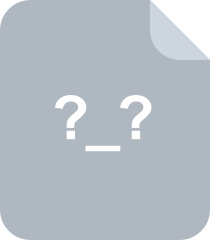
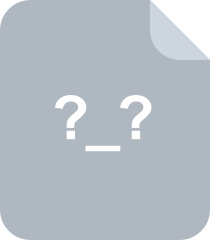
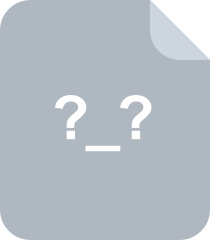
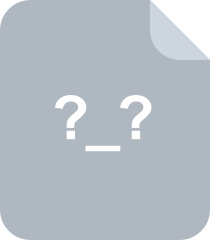
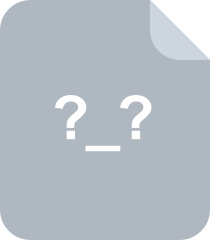
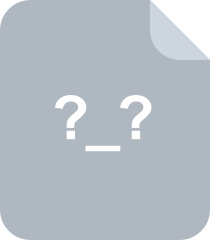
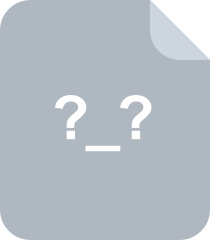
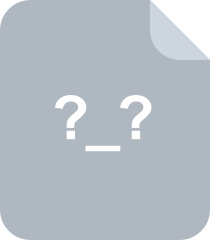
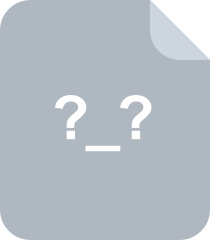
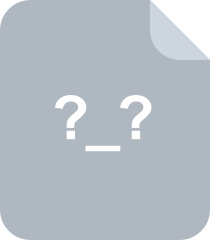
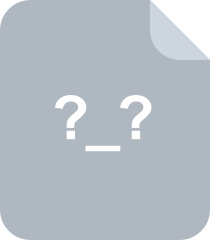
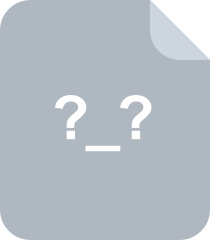
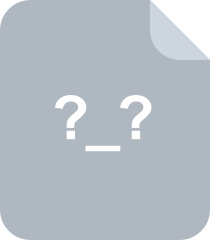
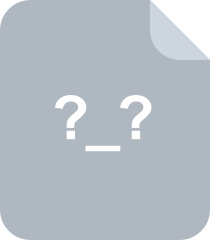
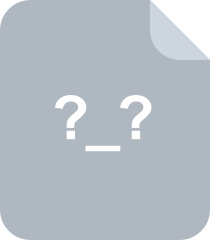
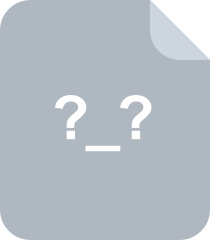
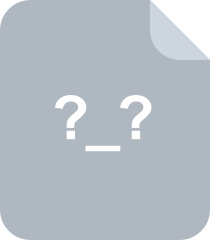
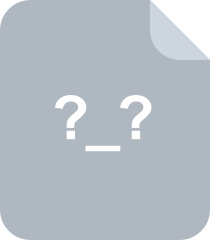
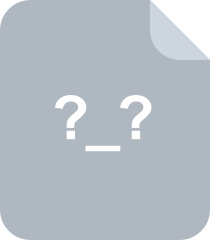
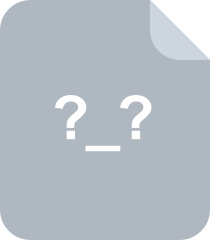
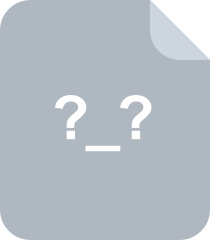
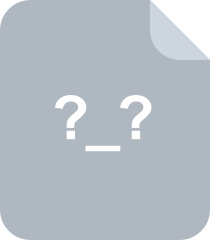
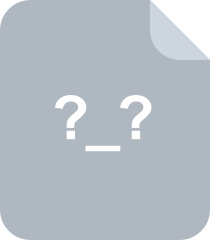
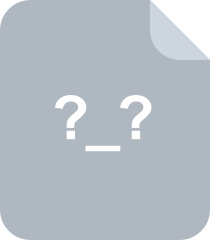
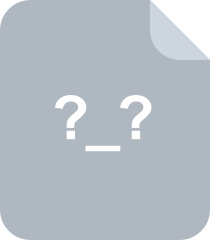
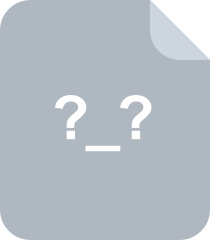
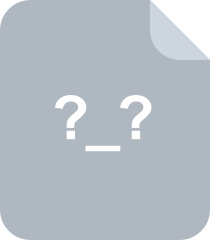
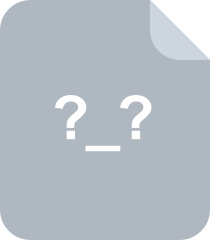
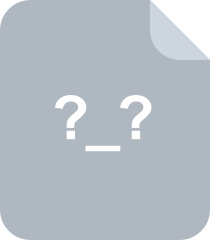
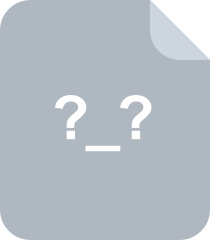
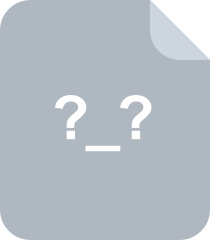
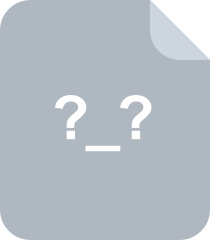
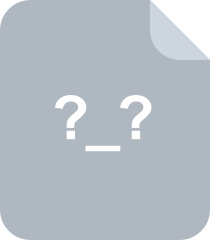
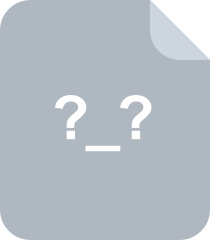
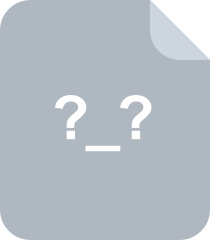
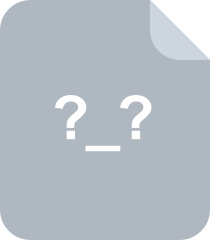
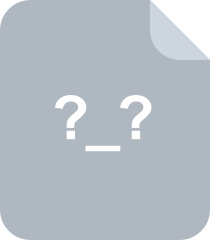
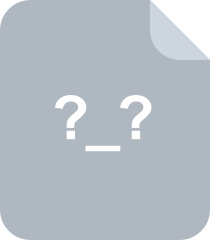
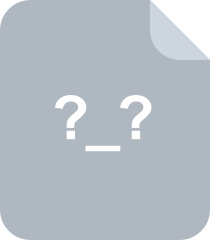
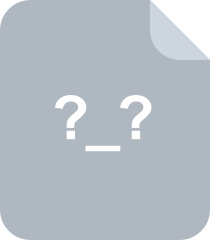
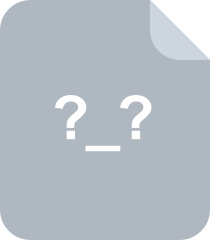
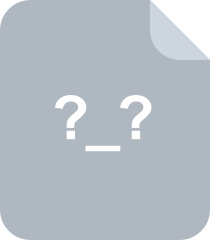
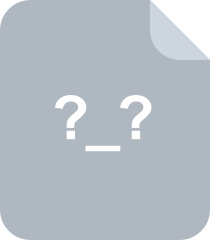
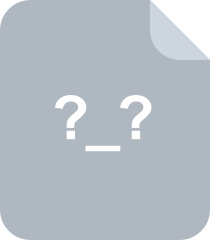
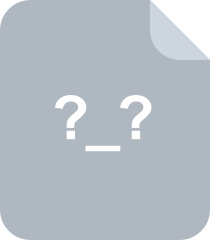
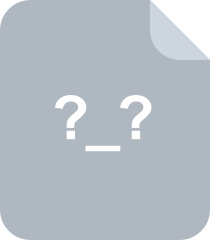
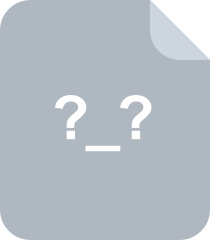
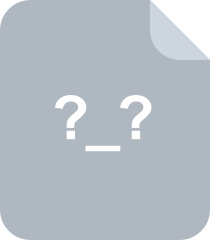
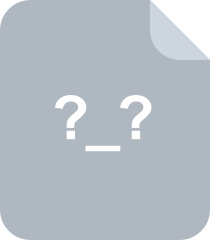
共 2005 条
- 1
- 2
- 3
- 4
- 5
- 6
- 21
资源评论
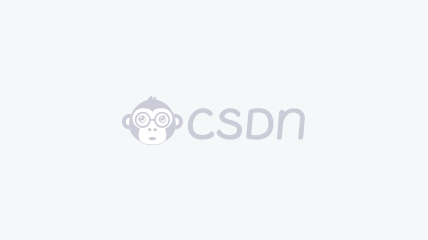

yava_free
- 粉丝: 2375
- 资源: 183
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

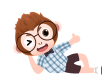
最新资源
- jdk - 22.0.2 - windows graalVM
- jdk - 22.0.2 - windows
- 496785224932493FLUENT_VOF&熔化_2D (不含仿真数据).zip
- jdk - 22.0.2 - macos
- 在Windows系统中管理Mac磁盘的实用工具-在Windows系统中创建并使用Mac磁盘,读取Mac磁盘中的文件
- PFC理论基础与Matlab仿真模型学习笔记(1)-PFC电路概述
- 吞食天地2马腾传.nes
- 西部数据发布的一款西数硬盘检测修复工具-支持WD-L/WD-ROYL板,能进行硬盘软复位,可识别硬盘查看或清除-供大家学习参考
- wwwwwwwwwwwwwwwwwww
- 利用恒源云在云端租用GPU服务器训练YOLOv8模型(包括Linux系统命令讲解)_恒源云跑模型-CSDN博客.html
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


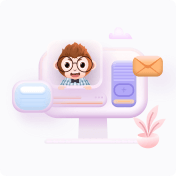
安全验证
文档复制为VIP权益,开通VIP直接复制
