/***************************************
名称:2008奥运会倒计时
***************************************/
#include<reg51.h>
#include<absacc.h>
#include<intrins.h>
#include "delay.h"
#define clock_segment XBYTE[0xBFFF] //时钟段码地址
#define clock_sel XBYTE[0x7FFF] //时钟位码地址
#define year_segment XBYTE[0xEFFF] //日历段码地址
#define year_sel XBYTE[0xDFFF] //日历位码地址
#define retime_segment XBYTE[0xFBFF]//倒计时段码地址
#define retime_sel XBYTE[0xF7FF] //倒计时位码地址
unsigned char time_num=0;//定时一秒次数
unsigned char shi_num=24;//小时位计数
unsigned char miao_ge=0; //秒个位
unsigned char miao_shi=0;//秒十位
unsigned char fen_ge=0;//分个位
unsigned char fen_shi=4;//分十位
unsigned char shi_ge=8;//时个位
unsigned char shi_shi=0;//时十位
unsigned char year_thousand=2;
unsigned char year_hundred=0;
unsigned char year_decade=0;
unsigned char year_unit=7;
unsigned char month_decade=0;
unsigned char month_unit=6;
unsigned char day_decade=2;
unsigned char day_unit=6;
unsigned char retime_unit=0;
unsigned char retime_decade=1;
unsigned char retime_hundred=4;
unsigned char retime_thousand=0;
unsigned char year_thoustore; //保存年千位数据
unsigned char year_hundstore; //保存年百位数据
unsigned char year_decastore; //保存年十位数据
unsigned char year_unitstore; //保存年个位数据
unsigned char month_unitstore;//保存月个位数据
unsigned char month_decastore;//保存月十位数据
unsigned char day_unitstore; //保存日个位数据
unsigned char day_decastore; //保存日十位数据
unsigned char year_unitstore2;//保存年各位数据
unsigned char year_decastore2;//年位组合子程序用来保护数据用
unsigned char year_hundstore2;
unsigned char year_thoustore2;
unsigned char retime_ustore; //保存倒计时各位数据
unsigned char retime_dstore;
unsigned char retime_hstore;
unsigned char retime_tstore;
unsigned char day_numj=31; //奇数月31天
unsigned char day_numo=30; //偶数月30天
unsigned char day_numooo=30;
unsigned char day_numoo;
unsigned char month_num=12;
unsigned int year_jointed; //年各位组合在一起,以便进行闰年判断
unsigned int year_help1;
unsigned int year_help2;
unsigned char xiaoshi_ge;//闪烁时存小时个位
unsigned char xiaoshi_shi;//闪烁时存小时十位
unsigned char fenzhong_ge;//闪烁时存分钟个位
unsigned char fenzhong_shi;//闪烁时存分钟十位
unsigned char cort=0;//判断第几次按下确认键
sbit key_sure=P1^0;//调时或确认键
sbit key_dec=P1^2;//调时减键
bit first_sure=0; //第一次按下确认键
bit second_sure=0;//第二次按下确认键
bit third_sure=0; //第三次按下确认键
bit fourth_sure=0;//第四次按下确认键
bit fifth_sure=0; //第五次按下确认键
bit sixth_sure=0; //第六次按下确认键
bit seventh_sure=0;//第七次按下确认键
unsigned code table[]={0x3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d,0x07,0x7f,0x6f,0x00};//段码表
/************函数声明*************/
void shannum_jian(void);
void inc_key(void);
void dec_key(void);
void dis_dingshi(void);
void hour_shanshuo(void);
void min_shanshuo(void);
void kbscan(void);
void display(void);
void year_joint(void);
unsigned char judgement_leap(void);
void retime_flash(void);
/********************************
显示子程序
*********************************/
void display(void)
{
clock_sel=0xdf;
clock_segment=table[miao_ge]; //送秒个位段位码
delay(3);
clock_sel=0xef;
clock_segment=table[miao_shi]; //送秒十位段位码
delay(3);
clock_sel=0xf7;
clock_segment=table[fen_ge]|0x80; //送分个位段位码
delay(3);
clock_sel=0xfb;
clock_segment=table[fen_shi]; //送分十位段位码
delay(3);
clock_sel=0xfd;
clock_segment=table[shi_ge]|0x80; //送时个位段位码
delay(3);
clock_sel=0xfe;
clock_segment=table[shi_shi]; //送时十位段位码
delay(3);
year_sel=0x7f;
year_segment=table[day_unit]; //送日个位段位码
delay(3);
year_sel=0xbf;
year_segment=table[day_decade]; //送日十位段位码
delay(3);
year_sel=0xdf;
year_segment=table[month_unit]|0x80; //送月个位段位码
delay(3);
year_sel=0xef;
year_segment=table[month_decade]; //送月十位段位码
delay(3);
year_sel=0xf7;
year_segment=table[year_unit]|0x80; //送年个位段位码
delay(3);
year_sel=0xfb;
year_segment=table[year_decade]; //送年十位段位码
delay(3);
year_sel=0xfd;
year_segment=table[year_hundred]; //送年百位段位码
delay(3);
year_sel=0xfe;
year_segment=table[year_thousand]; //送年千位段位码
delay(3);
retime_sel=0xf7;
retime_segment=table[retime_unit]; //送倒计时个位段位码
delay(3);
retime_sel=0xfb;
retime_segment=table[retime_decade]; //送倒计时十位段位码
delay(3);
retime_sel=0xfd;
retime_segment=table[retime_hundred];//送倒计时百位段位码
delay(3);
retime_sel=0xfe;
retime_segment=table[retime_thousand];//送倒计时千位段位码
delay(3);
}
/**********************************
判断闰年子程序
***********************************/
unsigned char judgement_leap(void)
{
year_joint(); //调用年各位组合子程序
if((year_jointed%4==0&&year_jointed%100!=0)||(year_jointed%100==0&&year_jointed%400==0))return(29); //是闰年二月29天
else return(28); //是平年二月28天
}
/**********************************
按键扫描子程序
***********************************/
void kbscan(void)
{
unsigned char sccode;
P1=0xff;
if((P1&0xff)!=0xff)
{
//delay(5);
display();
display();
display();
if((P1&0xff)!=0xff)
{
sccode=P1;
//while((P1&0xff)!=0xff) //按键后消陡
//{
// ;
// }
switch(sccode)
{
case 0xfe:shannum_jian();break;
case 0xfd:inc_key(); break;
case 0xfb:dec_key(); break;
default: break;
}
}
}
}
/********************************
确认键按键次数子程序
********************************/
void shannum_jian(void)
{
cort++;
if(cort==1) //第一次按下确认键
{
first_sure=1;
second_sure=0;
third_sure=0;
fourth_sure=0;
fifth_sure=0;
sixth_sure=0;
seventh_sure=0;
}
else if(cort==2) //第二次按下确认键
{
first_sure=0;
second_sure=1;
third_sure=0;
fourth_sure=0;
fifth_sure=0;
sixth_sure=0;
seventh_sure=0;
}
else if(cort==3) //第三次按下确认键
{
first_sure=0;
second_sure=0;
third_sure=1;
fourth_sure=0;
fifth_sure=0;
sixth_sure=0;
seventh_sure=0;
}
else if(cort==4) //第四次按下确认键
{
first_sure=0;
second_sure=0;
third_sure=0;
fourth_sure=1;
fifth_sure=0;
sixth_sure=0;
seventh_sure=0;
}
else if(cort==5) //第五次按下确认键
{
first_sure=0;
second_sure=0;
third_sure=0;
fourth_sure=0;
fifth_sure=1;
sixth_sure=0;
seventh_sure=0;
}
else if(cort==6) //第六次按下确认键
{
first_sure=0;
second_sure=0;
third_sure=0;
fourth_sure=0;
fifth_sure=0;
sixth_sure=1;
seventh_sure=0;
}
else if(cort==7) //第七次按下确认键
{
cort=0;
first_sure=0;
second_sure=0;
third_sure=0;
fourth_sure=0;
fifth_sure=0;
sixth_sure=0;
seventh_sure=1;
}
}
/*******************************
减一键子程序
*******************************/
void dec_key(void)
{
if(first_sure) //第一次按下确认键,小时位减
{
if(shi_num!=24)
{
++shi_num;

荣华富贵8
- 粉丝: 218
- 资源: 7653
最新资源
- 毕设和企业适用springboot企业数据智能分析平台类及智能农场管理系统源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及智能农业解决方案源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及数字图书馆平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及网络营销平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及个性化广告平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及产品溯源系统源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及资源调度平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及团队协作平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及无人驾驶系统源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及业务流程自动化平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及销售管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及客户关系管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及共享经济平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及客户服务平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及跨平台销售系统源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及平台生态系统源码+论文+视频.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


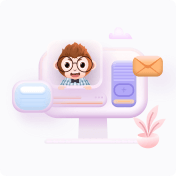