package jtapp.updateapksamples;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.json.JSONArray;
import org.json.JSONObject;
import android.app.Activity;
import android.app.AlertDialog;
import android.app.Dialog;
import android.app.ProgressDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.os.Environment;
import android.os.Handler;
import android.util.Log;
public class UpdateActivity extends Activity {
private static final String TAG = "Update";
public ProgressDialog pBar;
private Handler handler = new Handler();
private int newVerCode = 0;
private String newVerName = "";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
if (getServerVerCode()) {
int vercode = Config.getVerCode(this);
if (newVerCode > vercode) {
doNewVersionUpdate();
} else {
notNewVersionShow();
}
}
}
private boolean getServerVerCode() {
try {
String verjson = NetworkTool.getContent(Config.UPDATE_SERVER
+ Config.UPDATE_VERJSON);
JSONArray array = new JSONArray(verjson);
if (array.length() > 0) {
JSONObject obj = array.getJSONObject(0);
try {
newVerCode = Integer.parseInt(obj.getString("verCode"));
newVerName = obj.getString("verName");
} catch (Exception e) {
newVerCode = -1;
newVerName = "";
return false;
}
}
} catch (Exception e) {
Log.e(TAG, e.getMessage());
return false;
}
return true;
}
private void notNewVersionShow() {
int verCode = Config.getVerCode(this);
String verName = Config.getVerName(this);
StringBuffer sb = new StringBuffer();
sb.append("当前版本:");
sb.append(verName);
sb.append(" Code:");
sb.append(verCode);
sb.append(",\n已是最新版,无需更新!");
Dialog dialog = new AlertDialog.Builder(UpdateActivity.this)
.setTitle("软件更新").setMessage(sb.toString())// 设置内容
.setPositiveButton("确定",// 设置确定按钮
new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog,
int which) {
finish();
}
}).create();// 创建
// 显示对话框
dialog.show();
}
private void doNewVersionUpdate() {
int verCode = Config.getVerCode(this);
String verName = Config.getVerName(this);
StringBuffer sb = new StringBuffer();
sb.append("当前版本:");
sb.append(verName);
sb.append(" Code:");
sb.append(verCode);
sb.append(", 发现新版本:");
sb.append(newVerName);
sb.append(" Code:");
sb.append(newVerCode);
sb.append(", 是否更新?");
Dialog dialog = new AlertDialog.Builder(UpdateActivity.this)
.setTitle("软件更新")
.setMessage(sb.toString())
// 设置内容
.setPositiveButton("更新",// 设置确定按钮
new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog,
int which) {
pBar = new ProgressDialog(UpdateActivity.this);
pBar.setTitle("正在下载");
pBar.setMessage("请稍候...");
pBar.setProgressStyle(ProgressDialog.STYLE_SPINNER);
downFile(Config.UPDATE_SERVER
+ Config.UPDATE_APKNAME);
}
})
.setNegativeButton("暂不更新",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int whichButton) {
// 点击"取消"按钮之后退出程序
finish();
}
}).create();// 创建
// 显示对话框
dialog.show();
}
void downFile(final String url) {
pBar.show();
new Thread() {
public void run() {
HttpClient client = new DefaultHttpClient();
HttpGet get = new HttpGet(url);
HttpResponse response;
try {
response = client.execute(get);
HttpEntity entity = response.getEntity();
long length = entity.getContentLength();
InputStream is = entity.getContent();
FileOutputStream fileOutputStream = null;
if (is != null) {
File file = new File(
Environment.getExternalStorageDirectory(),
Config.UPDATE_SAVENAME);
fileOutputStream = new FileOutputStream(file);
byte[] buf = new byte[1024];
int ch = -1;
int count = 0;
while ((ch = is.read(buf)) != -1) {
fileOutputStream.write(buf, 0, ch);
count += ch;
if (length > 0) {
}
}
}
fileOutputStream.flush();
if (fileOutputStream != null) {
fileOutputStream.close();
}
down();
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}.start();
}
void down() {
handler.post(new Runnable() {
public void run() {
pBar.cancel();
update();
}
});
}
void update() {
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setDataAndType(Uri.fromFile(new File(Environment
.getExternalStorageDirectory(), Config.UPDATE_SAVENAME)),
"application/vnd.android.package-archive");
startActivity(intent);
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
小程序源码 网络浏览 应用程序自动更新源码.rar
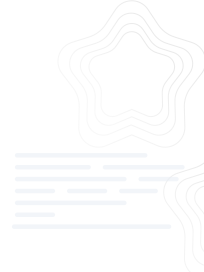
共114个文件
svn-base:31个
all-wcprops:29个
entries:29个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 6 浏览量
2023-03-29
19:55:40
上传
评论
收藏 129KB RAR 举报
温馨提示
免责声明:资料部分来源于合法的互联网渠道收集和整理,部分自己学习积累成果,供大家学习参考与交流。收取的费用仅用于收集和整理资料耗费时间的酬劳。 本人尊重原创作者或出版方,资料版权归原作者或出版方所有,本人不对所涉及的版权问题或内容负法律责任。如有侵权,请举报或通知本人删除。
资源推荐
资源详情
资源评论
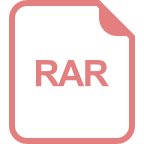
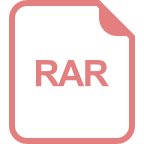
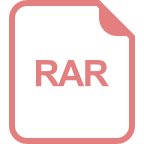
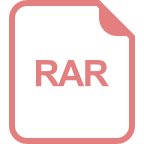
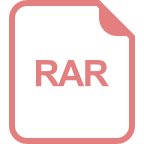
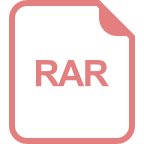
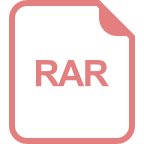
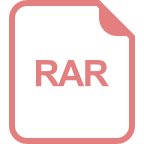
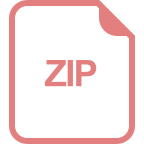
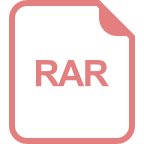
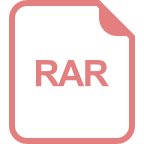
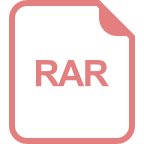
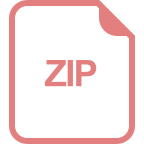
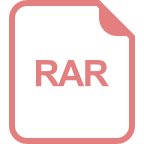
收起资源包目录

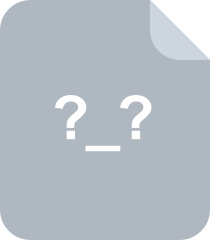
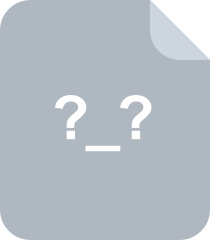
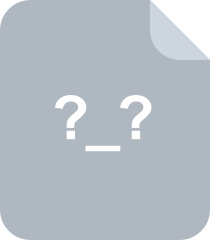
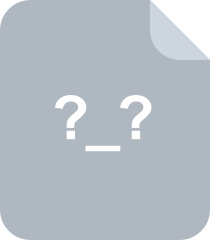
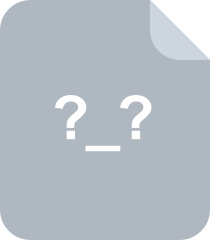
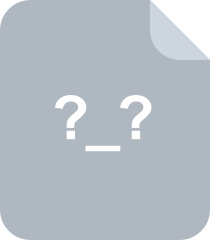
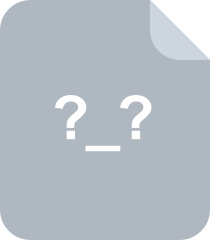
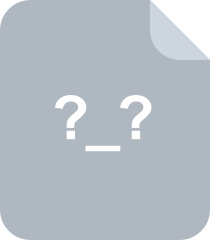
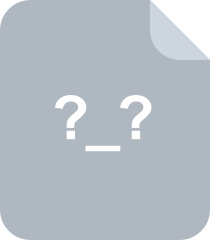
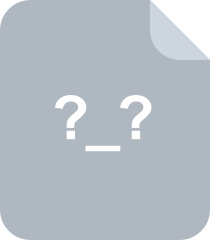
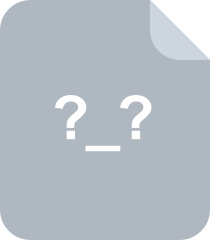
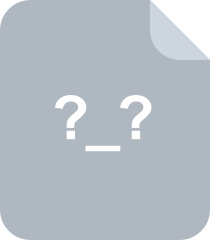
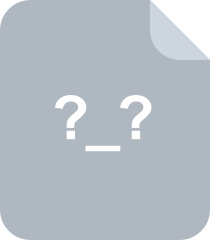
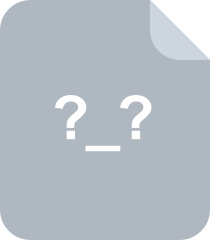
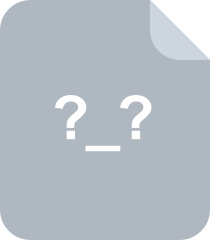
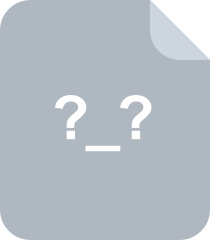
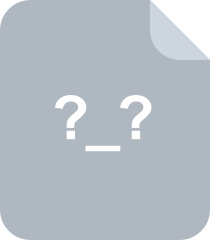
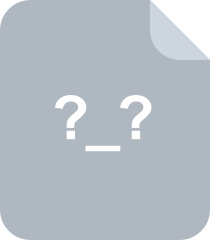
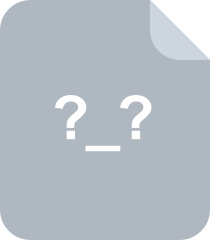
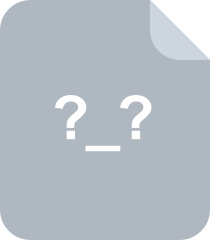
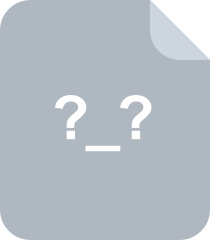
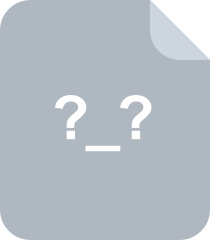
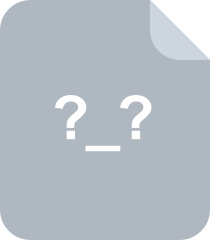
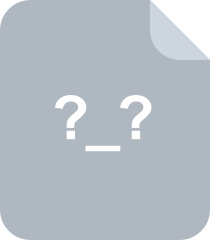
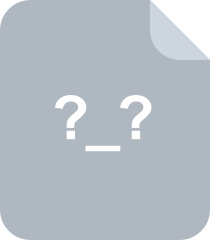
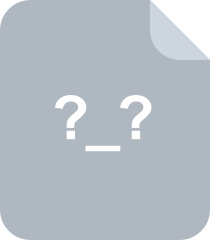
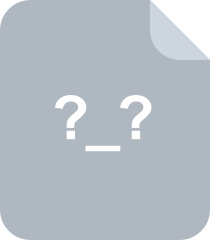
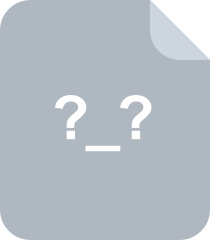
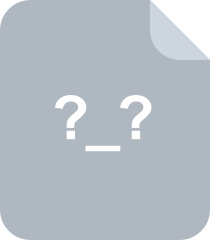
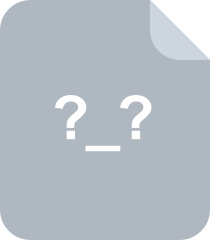
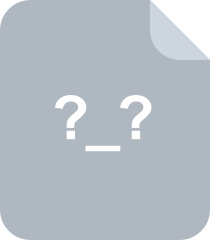
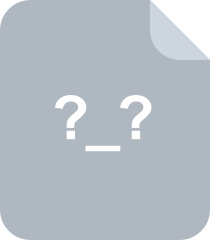
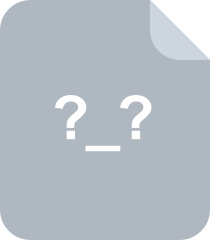
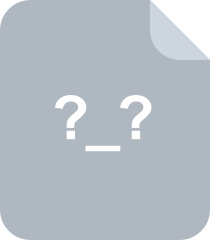
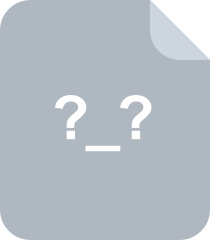
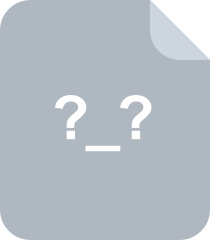
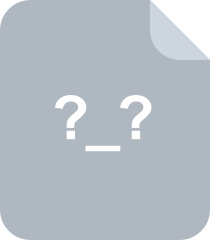
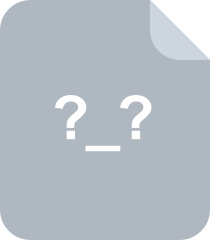
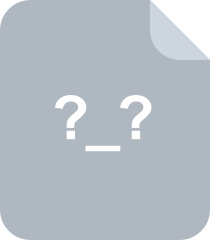
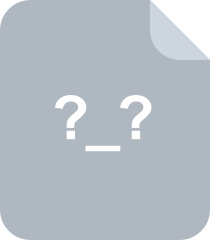
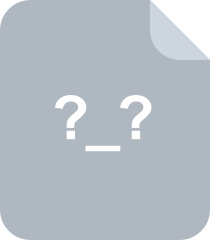
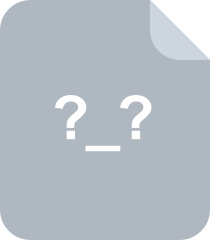
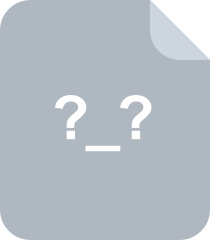
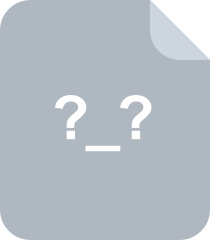
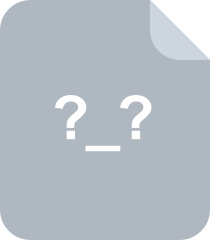
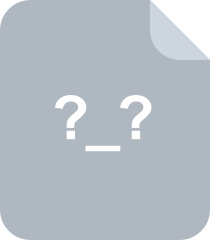
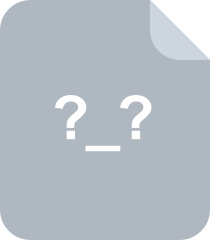
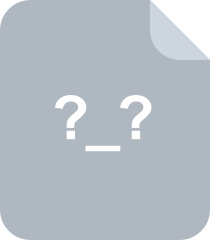
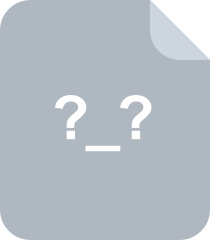
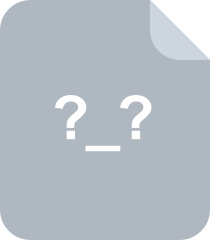
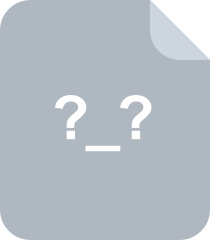
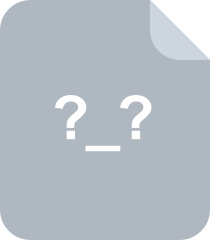
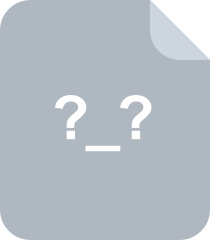
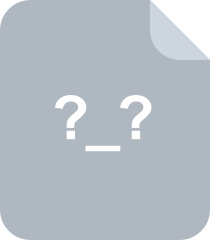
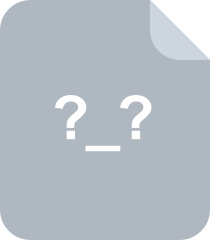
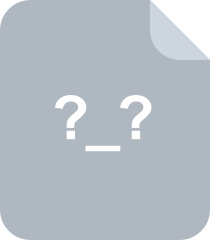
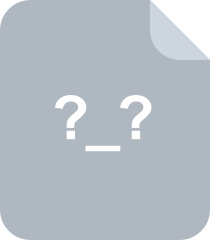
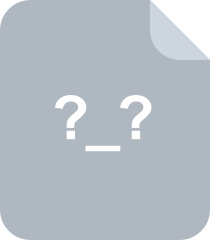
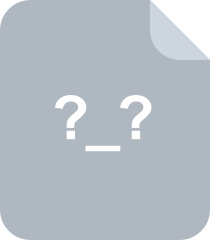
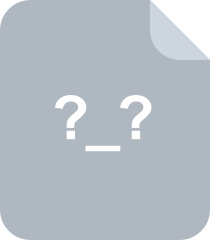
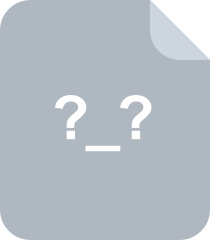
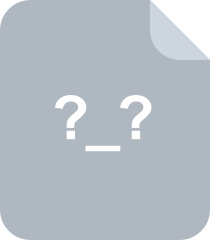
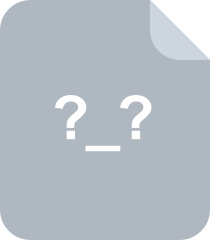
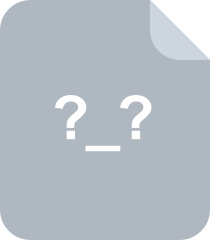
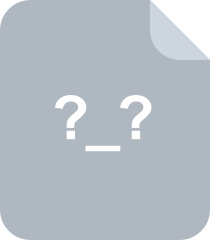
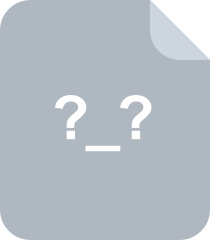
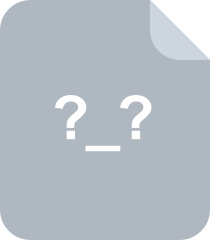
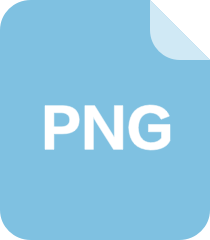
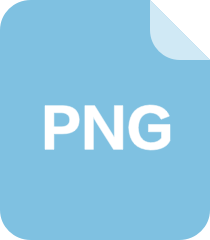
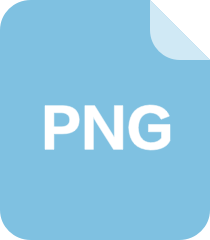
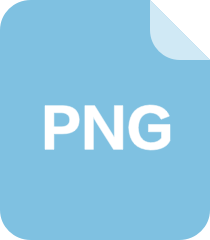
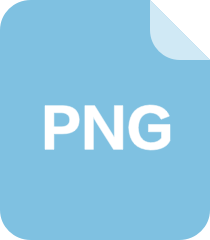
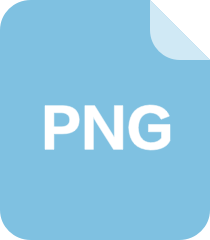
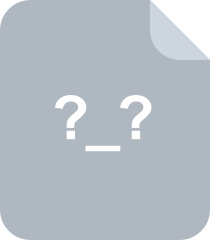
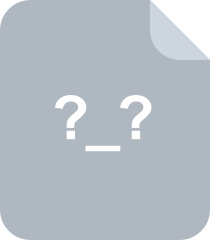
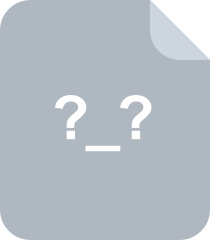
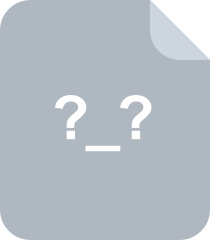
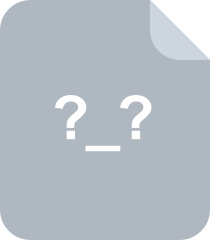
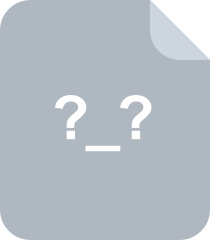
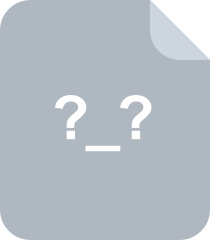
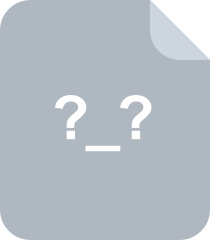
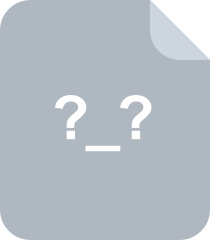
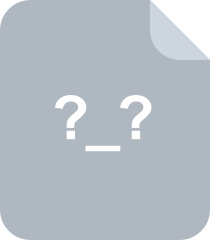
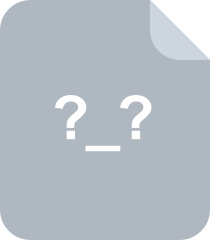
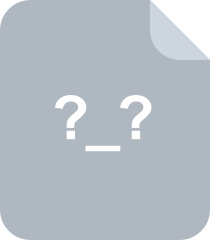
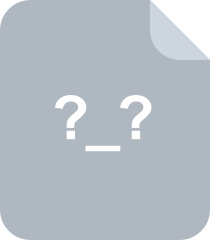
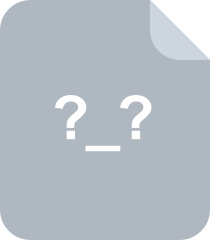
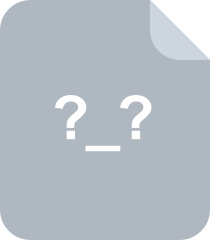
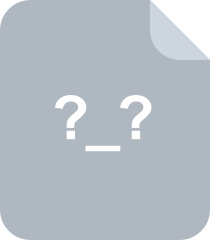
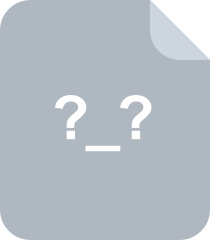
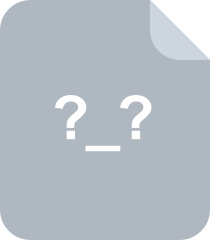
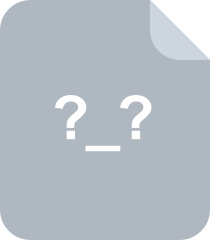
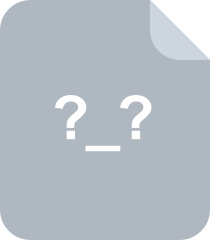
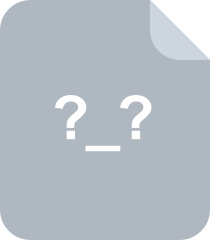
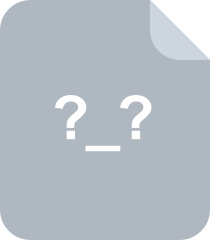
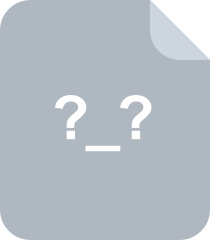
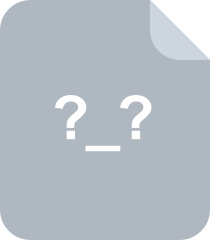
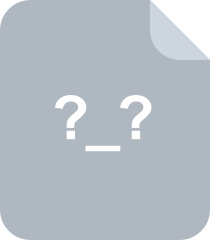
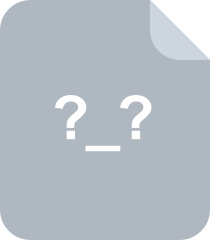
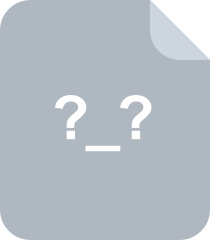
共 114 条
- 1
- 2
资源评论
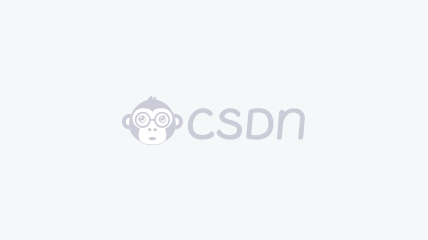

荣华富贵8
- 粉丝: 151
- 资源: 7649
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

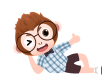
安全验证
文档复制为VIP权益,开通VIP直接复制
