/*
* Copyright (C) 2008 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.android.toolKit.union;
import java.io.Writer;
import java.util.ArrayList;
import javax.microedition.khronos.egl.EGL10;
import javax.microedition.khronos.egl.EGL11;
import javax.microedition.khronos.egl.EGLConfig;
import javax.microedition.khronos.egl.EGLContext;
import javax.microedition.khronos.egl.EGLDisplay;
import javax.microedition.khronos.egl.EGLSurface;
import javax.microedition.khronos.opengles.GL;
import javax.microedition.khronos.opengles.GL10;
import android.content.Context;
import android.content.pm.ConfigurationInfo;
import android.graphics.PixelFormat;
import android.opengl.GLDebugHelper;
//import android.os.SystemProperties;
import android.util.AttributeSet;
import android.util.Log;
import android.view.SurfaceHolder;
import android.view.SurfaceView;
/**
* An implementation of SurfaceView that uses the dedicated surface for
* displaying OpenGL rendering.
* <p>
* A GLSurfaceView provides the following features:
* <p>
* <ul>
* <li>Manages a surface, which is a special piece of memory that can be
* composited into the Android view system.
* <li>Manages an EGL display, which enables OpenGL to render into a surface.
* <li>Accepts a user-provided Renderer object that does the actual rendering.
* <li>Renders on a dedicated thread to decouple rendering performance from the
* UI thread.
* <li>Supports both on-demand and continuous rendering.
* <li>Optionally wraps, traces, and/or error-checks the renderer's OpenGL calls.
* </ul>
*
* <h3>Using GLSurfaceView</h3>
* <p>
* Typically you use GLSurfaceView by subclassing it and overriding one or more of the
* View system input event methods. If your application does not need to override event
* methods then GLSurfaceView can be used as-is. For the most part
* GLSurfaceView behavior is customized by calling "set" methods rather than by subclassing.
* For example, unlike a regular View, drawing is delegated to a separate Renderer object which
* is registered with the GLSurfaceView
* using the {@link #setRenderer(Renderer)} call.
* <p>
* <h3>Initializing GLSurfaceView</h3>
* All you have to do to initialize a GLSurfaceView is call {@link #setRenderer(Renderer)}.
* However, if desired, you can modify the default behavior of GLSurfaceView by calling one or
* more of these methods before calling setRenderer:
* <ul>
* <li>{@link #setDebugFlags(int)}
* <li>{@link #setEGLConfigChooser(boolean)}
* <li>{@link #setEGLConfigChooser(EGLConfigChooser)}
* <li>{@link #setEGLConfigChooser(int, int, int, int, int, int)}
* <li>{@link #setGLWrapper(GLWrapper)}
* </ul>
* <p>
* <h4>Specifying the android.view.Surface</h4>
* By default GLSurfaceView will create a PixelFormat.RGB_565 format surface. If a translucent
* surface is required, call getHolder().setFormat(PixelFormat.TRANSLUCENT).
* The exact format of a TRANSLUCENT surface is device dependent, but it will be
* a 32-bit-per-pixel surface with 8 bits per component.
* <p>
* <h4>Choosing an EGL Configuration</h4>
* A given Android device may support multiple EGLConfig rendering configurations.
* The available configurations may differ in how may channels of data are present, as
* well as how many bits are allocated to each channel. Therefore, the first thing
* GLSurfaceView has to do when starting to render is choose what EGLConfig to use.
* <p>
* By default GLSurfaceView chooses a EGLConfig that has an RGB_656 pixel format,
* with at least a 16-bit depth buffer and no stencil.
* <p>
* If you would prefer a different EGLConfig
* you can override the default behavior by calling one of the
* setEGLConfigChooser methods.
* <p>
* <h4>Debug Behavior</h4>
* You can optionally modify the behavior of GLSurfaceView by calling
* one or more of the debugging methods {@link #setDebugFlags(int)},
* and {@link #setGLWrapper}. These methods may be called before and/or after setRenderer, but
* typically they are called before setRenderer so that they take effect immediately.
* <p>
* <h4>Setting a Renderer</h4>
* Finally, you must call {@link #setRenderer} to register a {@link Renderer}.
* The renderer is
* responsible for doing the actual OpenGL rendering.
* <p>
* <h3>Rendering Mode</h3>
* Once the renderer is set, you can control whether the renderer draws
* continuously or on-demand by calling
* {@link #setRenderMode}. The default is continuous rendering.
* <p>
* <h3>Activity Life-cycle</h3>
* A GLSurfaceView must be notified when the activity is paused and resumed. GLSurfaceView clients
* are required to call {@link #onPause()} when the activity pauses and
* {@link #onResume()} when the activity resumes. These calls allow GLSurfaceView to
* pause and resume the rendering thread, and also allow GLSurfaceView to release and recreate
* the OpenGL display.
* <p>
* <h3>Handling events</h3>
* <p>
* To handle an event you will typically subclass GLSurfaceView and override the
* appropriate method, just as you would with any other View. However, when handling
* the event, you may need to communicate with the Renderer object
* that's running in the rendering thread. You can do this using any
* standard Java cross-thread communication mechanism. In addition,
* one relatively easy way to communicate with your renderer is
* to call
* {@link #queueEvent(Runnable)}. For example:
* <pre class="prettyprint">
* class MyGLSurfaceView extends GLSurfaceView {
*
* private MyRenderer mMyRenderer;
*
* public void start() {
* mMyRenderer = ...;
* setRenderer(mMyRenderer);
* }
*
* public boolean onKeyDown(int keyCode, KeyEvent event) {
* if (keyCode == KeyEvent.KEYCODE_DPAD_CENTER) {
* queueEvent(new Runnable() {
* // This method will be called on the rendering
* // thread:
* public void run() {
* mMyRenderer.handleDpadCenter();
* }});
* return true;
* }
* return super.onKeyDown(keyCode, event);
* }
* }
* </pre>
*
*/
public class Code_GLSurfaceView extends SurfaceView implements SurfaceHolder.Callback {
private final static boolean LOG_THREADS = false;
private final static boolean LOG_PAUSE_RESUME = false;
private final static boolean LOG_SURFACE = false;
private final static boolean LOG_RENDERER = false;
private final static boolean LOG_RENDERER_DRAW_FRAME = false;
private final static boolean LOG_EGL = false;
// Work-around for bug 2263168
private final static boolean DRAW_TWICE_AFTER_SIZE_CHANGED = true;
/**
* The renderer only renders
* when the surface is created, or when {@link #requestRender} is called.
*
* @see #getRenderMode()
* @see #setRenderMode(int)
* @see #requestRender()
*/
public final static int RENDERMODE_WHEN_DIRTY = 0;
/**
* The renderer is called
* continuously to re-render the scene.
*
* @see #getRenderMode()
* @see #setRenderMode(int)
*/
public final static int RENDERMODE_CONTINUOUSLY = 1;
/**
* Check glError() after every GL call and throw an exception if glError indicates
* that an error has occurred. This can be used to help track down which OpenGL ES call
* is causing an error.
*
* @see #getDebugFlags
* @see #setDebugFlags
*/
没有合适的资源?快使用搜索试试~ 我知道了~
Launcher 桌面 安卓桌面应用EyeRoom(程序源码).zip
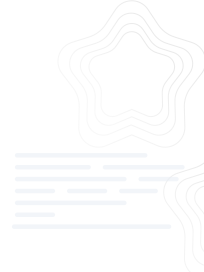
共1169个文件
s3dm:340个
png:297个
java:196个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 193 浏览量
2023-03-16
11:03:43
上传
评论
收藏 24.82MB ZIP 举报
温馨提示
免责声明:资料部分来源于合法的互联网渠道收集和整理,部分自己学习积累成果,供大家学习参考与交流。收取的费用仅用于收集和整理资料耗费时间的酬劳。 本人尊重原创作者或出版方,资料版权归原作者或出版方所有,本人不对所涉及的版权问题或内容负法律责任。如有侵权,请举报或通知本人删除。
资源推荐
资源详情
资源评论
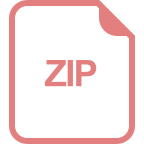
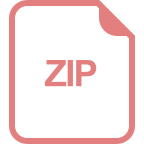
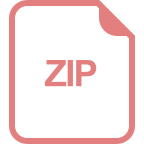
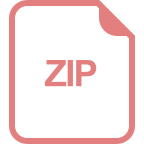
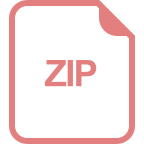
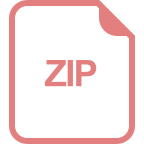
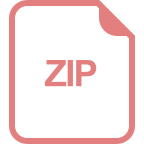
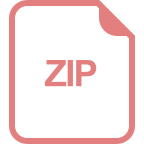
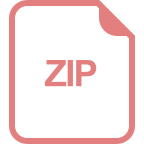
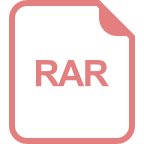
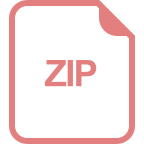
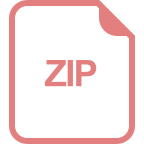
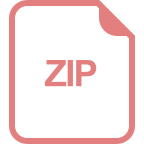
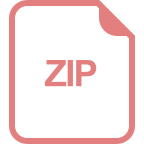
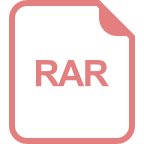
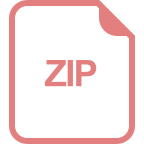
收起资源包目录

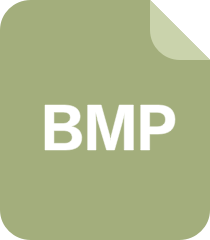
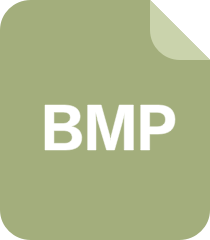
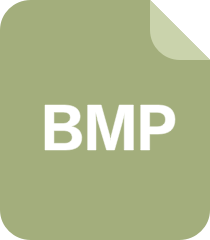
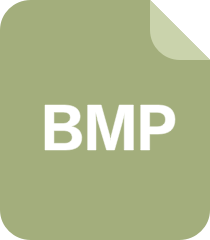
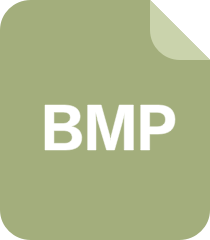
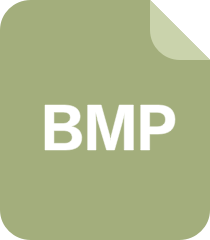
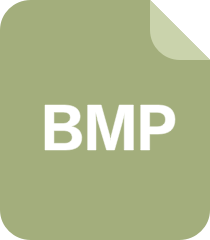
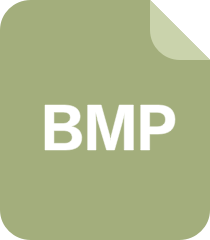
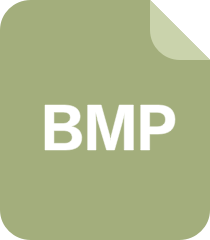
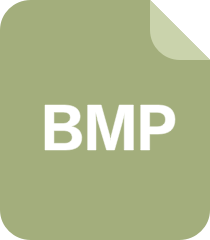
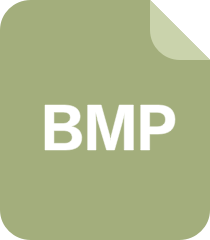
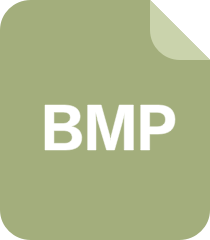
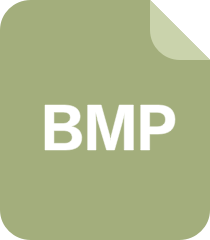
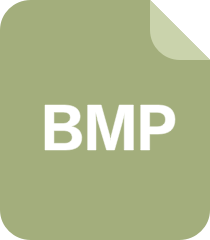
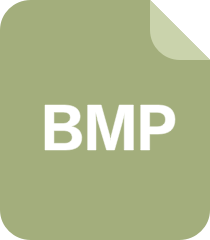
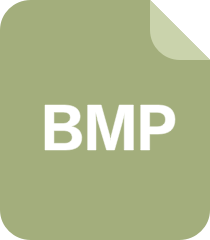
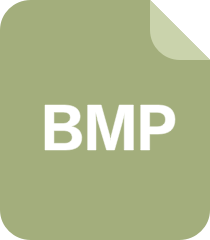
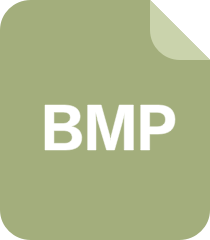
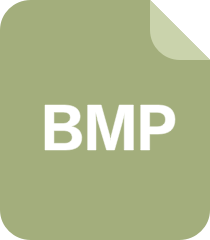
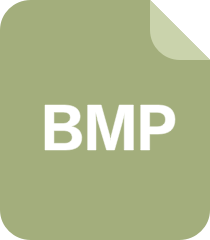
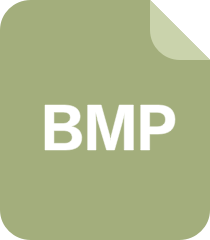
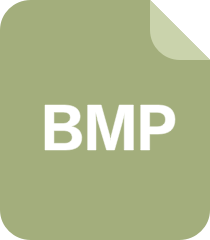
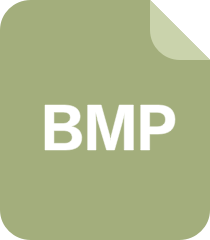
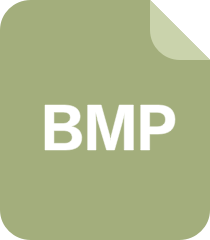
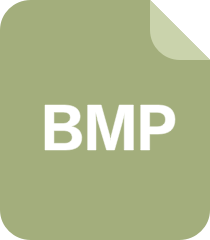
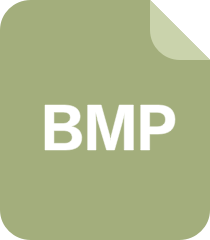
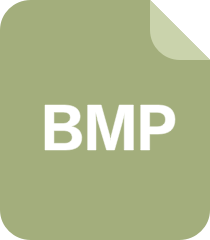
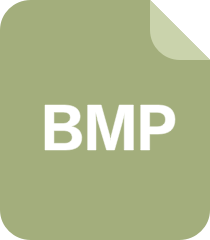
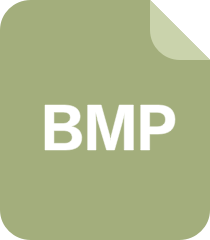
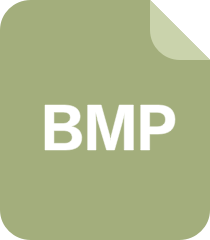
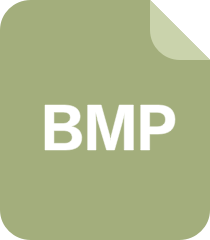
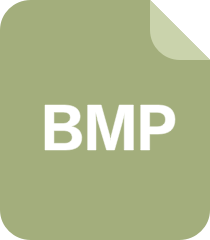
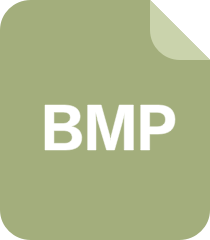
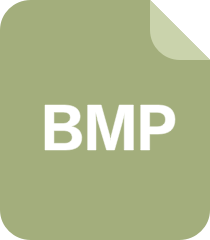
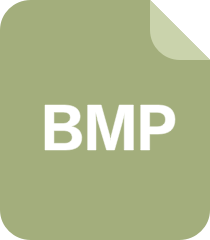
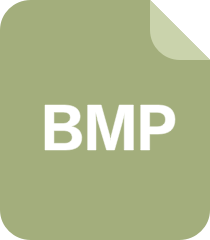
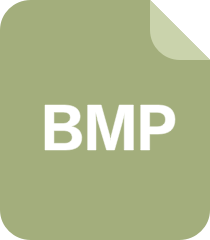
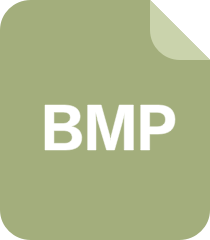
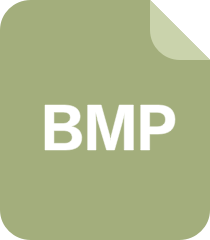
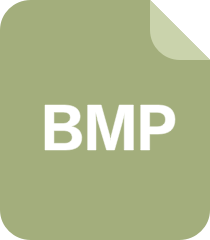
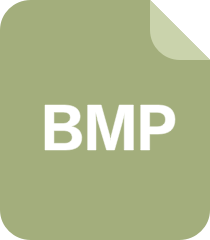
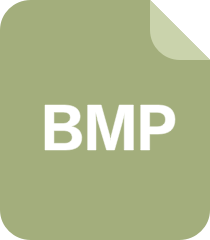
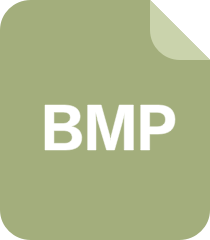
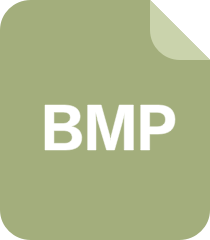
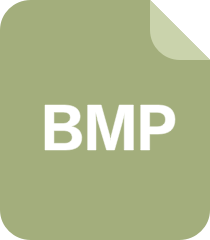
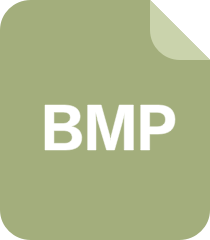
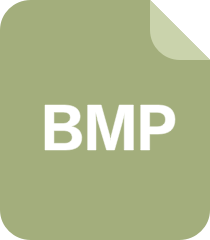
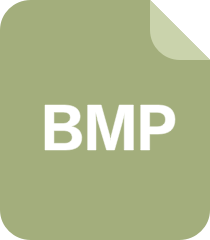
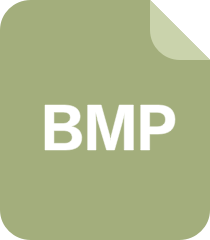
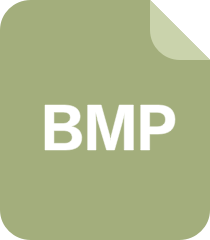
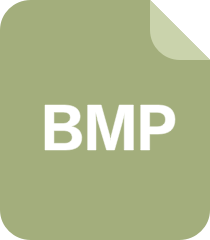
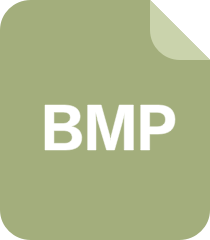
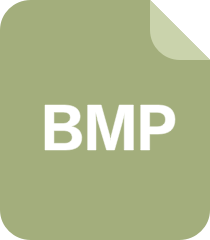
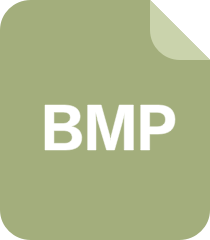
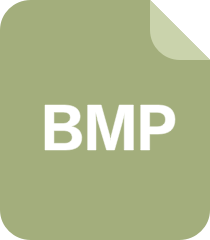
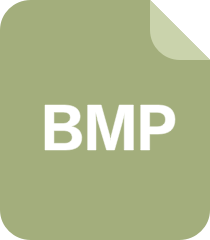
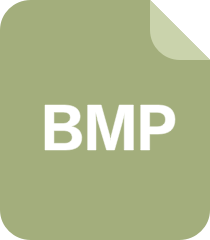
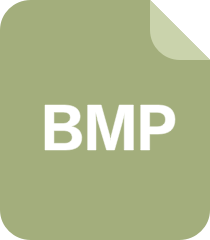
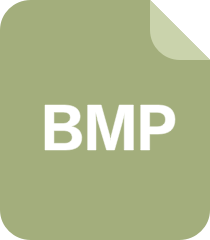
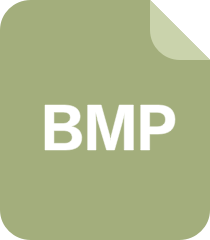
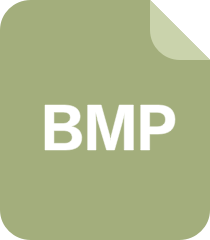
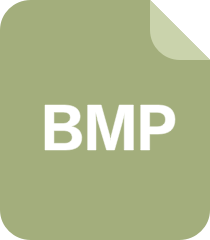
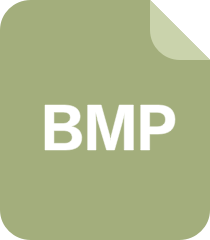
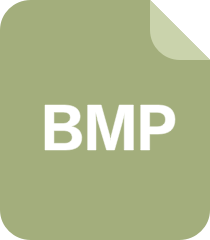
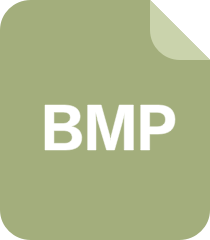
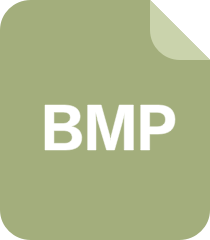
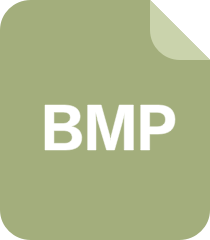
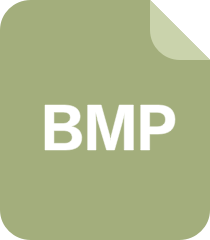
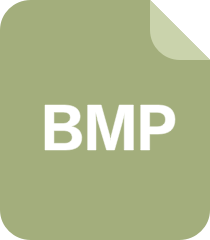
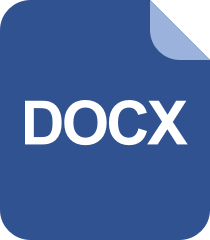
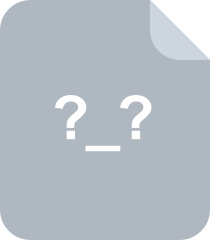
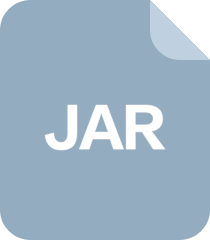
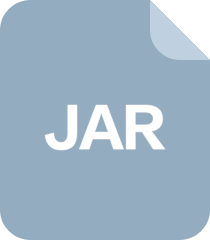
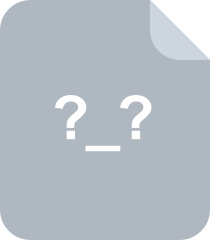
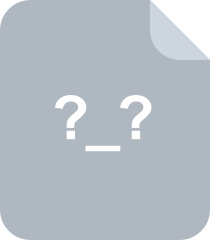
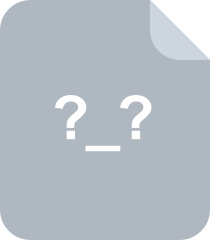
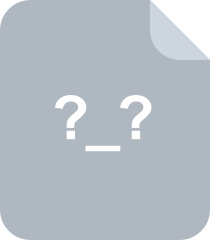
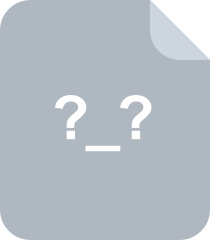
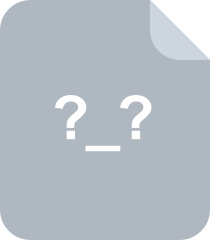
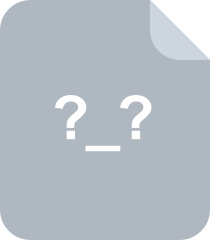
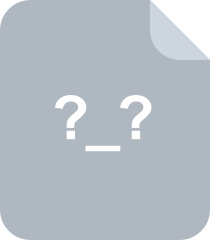
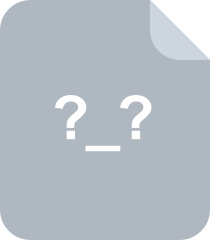
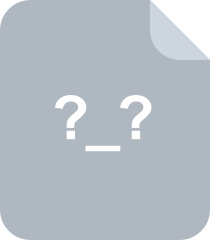
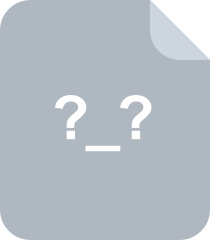
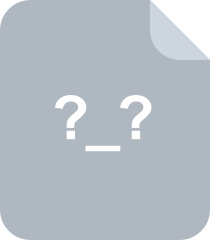
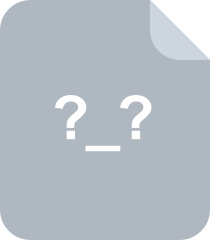
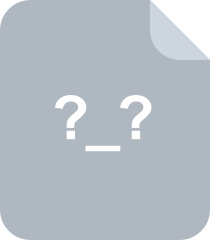
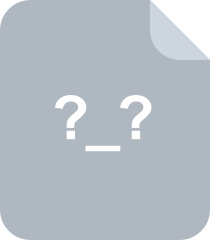
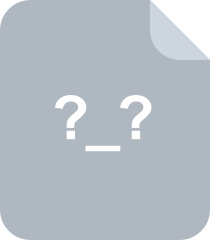
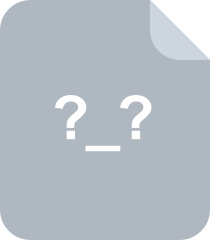
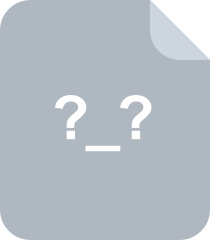
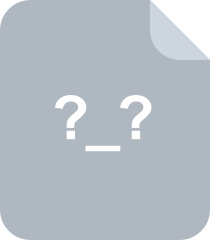
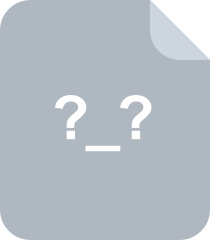
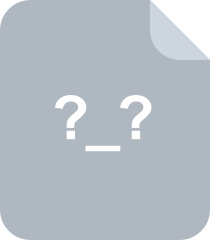
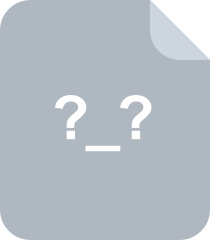
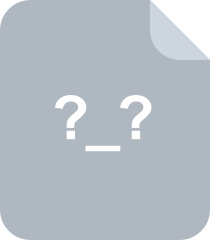
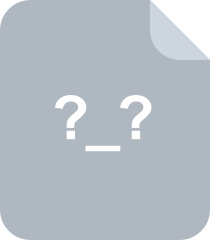
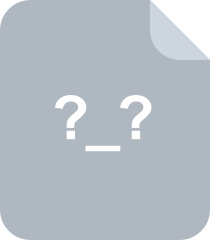
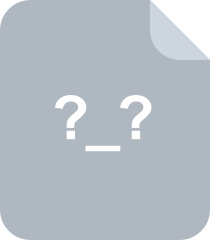
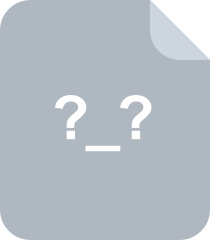
共 1169 条
- 1
- 2
- 3
- 4
- 5
- 6
- 12
资源评论
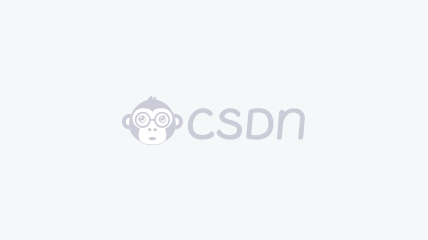

金枝玉叶9
- 粉丝: 195
- 资源: 7637
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

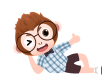
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


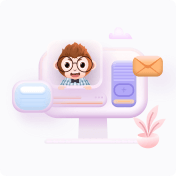
安全验证
文档复制为VIP权益,开通VIP直接复制
