# UITableView for Android



## Usage
### UITableView
#### Defining your layout
<br.com.dina.ui.widget.UITableView
android:id="@+id/tableView"
style="@style/UITableView" />
#### Working on your activity
public class Example1Activity extends Activity {
UITableView tableView;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
tableView = (UITableView) findViewById(R.id.tableView);
createList();
Log.d("Example1Activity", "total items: " + tableView.getCount());
tableView.commit();
}
private void createList() {
CustomClickListener listener = new CustomClickListener();
tableView.setClickListener(listener);
tableView.addItem("Example 1", "Summary text 1");
tableView.addItem("Example 2", "Summary text 2");
tableView.addItem("Example 3", "Summary text 3");
tableView.addItem("Example 4", "Summary text 4");
}
private class CustomClickListener implements ClickListener {
@Override
public void onClick(int index) {
Toast.makeText(Example1Activity.this, "item clicked: " + index, Toast.LENGTH_SHORT).show();
}
}
}
### UITableViewActivity
In order to use the a default list you can extend the [UITableViewActivity](https://github.com/thiagolocatelli/android-uitableview/blob/master/android-uitableview/src/br/com/dina/ui/activity/UITableViewActivity.java), a simple example can be found in the source code below:
public class ExampleActivity extends UITableViewActivity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
CustomClickListener listener = new CustomClickListener();
getUITableView().setClickListener(listener);
}
private class CustomClickListener implements ClickListener {
@Override
public void onClick(int index) {
Toast.makeText(ExampleActivity.this, "item clicked: " + index, Toast.LENGTH_SHORT).show();
}
}
@Override
protected void populateList() {
getUITableView().addItem("Example 1", "Summary text 1");
getUITableView().addItem("Example 2", "Summary text 2");
getUITableView().addItem("Example 3", "Summary text 3");
getUITableView().addItem("Example 4", "Summary text 4");
getUITableView().addItem("Example 5", "Summary text 5");
}
}
In this example you don't even need to care about the xml since the [UITableViewActivity](https://github.com/thiagolocatelli/android-uitableview/blob/master/android-uitableview/src/br/com/dina/ui/activity/UITableViewActivity.java) is using a default layout template the only displays the list in the screen. It is pretty mych the same list you are seeing in the screenshot provided at the beginning of this explanation.
### UIButton
<LinearLayout
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<br.com.dina.ui.widget.UIButton
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:padding="10dip"
ui:title="some title one"/>
<br.com.dina.ui.widget.UIButton
android:layout_width="fill_parent"
android:layout_height="fill_parent"
ui:title="some title two"
ui:subtitle="some subtitle two"
android:padding="10dip" />
<br.com.dina.ui.widget.UIButton
android:layout_width="fill_parent"
android:layout_height="fill_parent"
ui:title="some title three"
ui:subtitle="with image"
ui:image="@drawable/search_image"
android:padding="10dip"/>
</LinearLayout>
### Customization
UITableView is an Android Library Project and all its resources will be merged into the referring project. So, in order tu customize the colors of the UITableView and its elements, you need to create the same resources on your own project and this resources will be before the default values provided by the library project.
If you don't like the default colors that is defined in the [colors.xml](https://github.com/thiagolocatelli/android-uitableview/blob/master/android-uitableview/res/values/colors.xml) file simply override the default values in the main projects colors.xml file. These are the keys you need to work on to have your customized UITableView working.
<?xml version="1.0" encoding="utf-8"?>
<resources>
<!-- LIST BORDER COLOR -->
<color name="rounded_container_border">#ffb7babb</color>
<!-- ITEM BACKGROUND COLOR - STATE - DEFAULT -->
<color name="base_start_color_default">#FFFFFF</color>
<color name="base_end_color_default">#FFFFFF</color>
<!-- ITEM BACKGROUND COLOR - STATE - PRESSED -->
<color name="base_start_color_pressed">#ff3590c4</color>
<color name="base_end_color_pressed">#ff2570ba</color>
<!-- ITEM TEXT COLORS - STATES - PRESSED AND DEFAULT -->
<color name="text_color_default">#000000</color>
<color name="text_color_pressed">#ffffff</color>
</resources>
#### Example


The theme above was created using the following set of colors:
<resources>
<color name="rounded_container_border">#50b7babb</color>
<color name="base_start_color_default">#B0FFFFFF</color>
<color name="base_end_color_default">#B0FFFFFF</color>
<color name="base_start_color_pressed">#B03590c4</color>
<color name="base_end_color_pressed">#B02570ba</color>
<color name="text_color_default">#000000</color>
<color name="text_color_pressed">#ffffff</color>
</resources>
## Android applications using it
## Contributions
Functionallity improvements and performance enhancements are always welcome. Feel free to fork and apply your changes.
### TODO list
* Hability to let the user define the custom layout for the item
* Hability to create Items that expand/collapse a set of items
## Other Android Libraries
Use these libraries also to get a better UI for your android application
* [Android ActionBar](https://github.com/johannilsson/android-actionbar) by [Johan Nilsson](https://github.com/johannilsson)
* [Android Pull to Refresh](https://github.com/johannilsson/android-pulltorefresh) by [Johan Nilsson](https://github.com/johannilsson)
* [SwipeView](https://github.com/fry15/uk.co.jasonfry.android.tools) by [Jason Fry](https://github.com/fry15)
* [Facebook Integration](https://github.com/lorensiuswlt/AndroidFacebook) by [Lorensius](https://github.com/lorensiuswlt)
* [Twitter Integration](https://github.com/lorensiuswlt/AndroidTwitter) by [Lorensius](https://github.com/lorensiuswlt)
* [Quick Actions](https://github.com/lorensiuswlt/NewQuickAction) by [Lorensius](https://github.com/lorensiuswlt)
## License
Copyright (c) 2011 [Thiago Locatelli] - "thiago:locatelli$gmail:com".replace(':','.').replace('$','@')
Licensed under the [Apache License, Version 2.0](http://www.apache.org/licenses/LICENSE-2.0.html)
没有合适的资源?快使用搜索试试~ 我知道了~
安卓源码 UITableView ios风格控件.zip
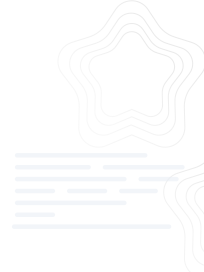
共72个文件
xml:27个
png:23个
java:14个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 96 浏览量
2023-03-04
16:54:52
上传
评论
收藏 59KB ZIP 举报
温馨提示
免责声明:资料部分来源于合法的互联网渠道收集和整理,部分自己学习积累成果,供大家学习参考与交流。收取的费用仅用于收集和整理资料耗费时间的酬劳。 本人尊重原创作者或出版方,资料版权归原作者或出版方所有,本人不对所涉及的版权问题或内容负法律责任。如有侵权,请举报或通知本人删除。
资源推荐
资源详情
资源评论
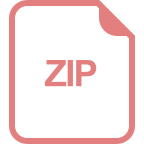
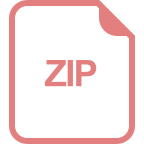
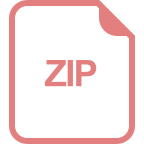
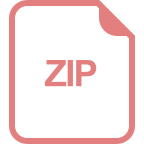
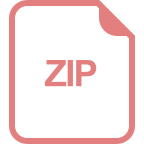
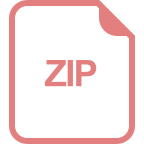
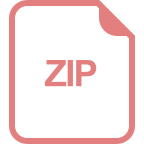
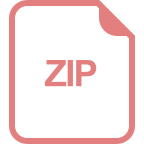
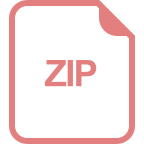
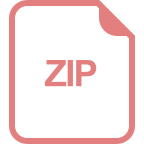
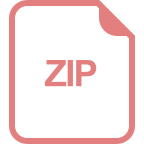
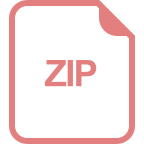
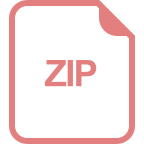
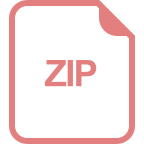
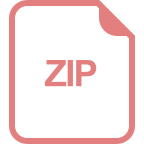
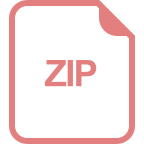
收起资源包目录

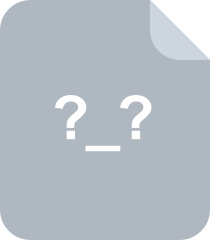


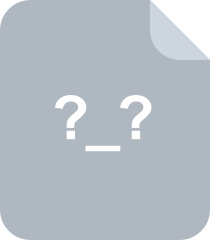






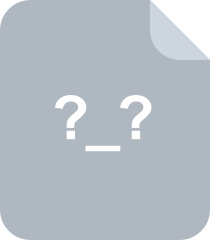
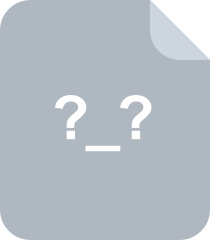
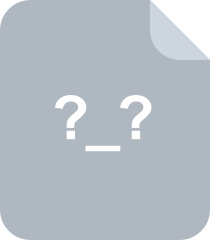
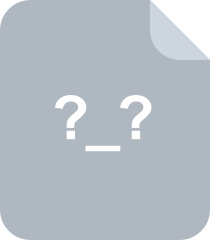
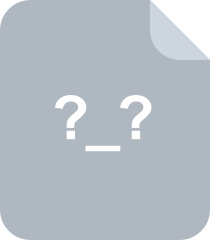
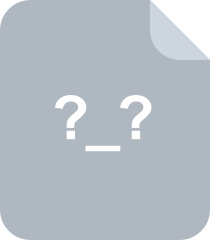
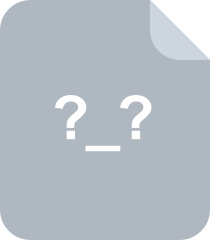
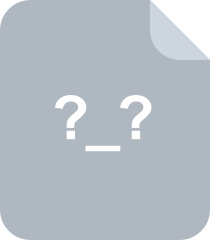


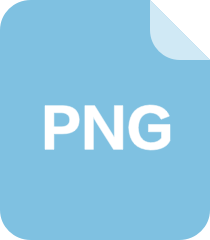
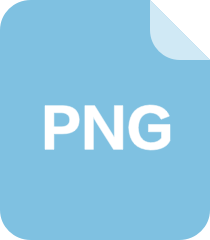
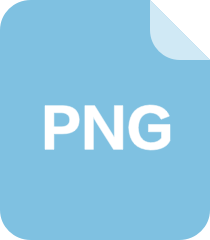

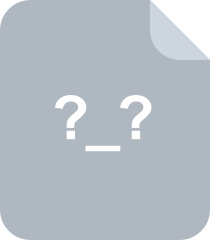
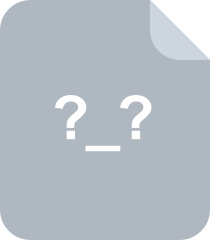

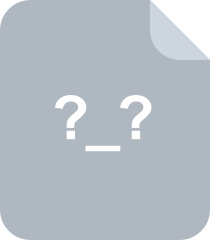
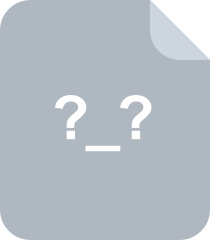
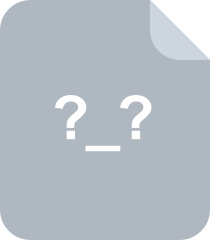
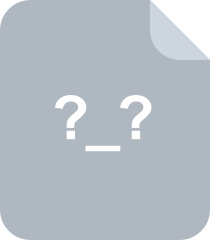
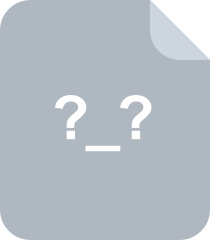

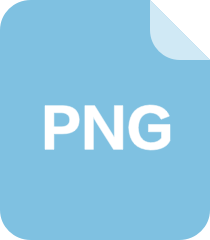
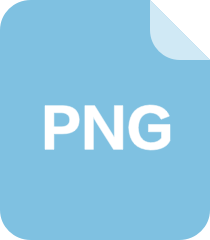
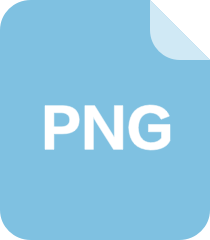
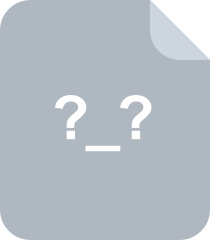
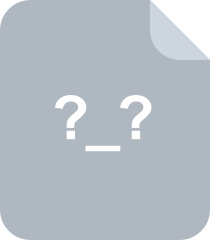

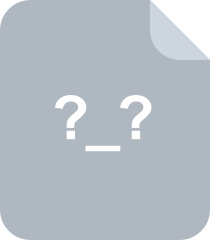






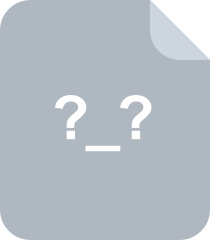

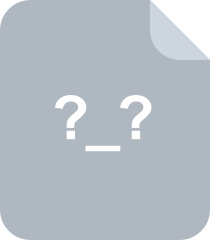
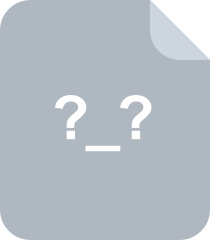
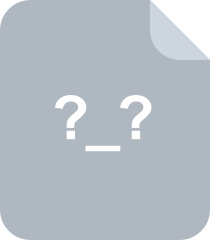

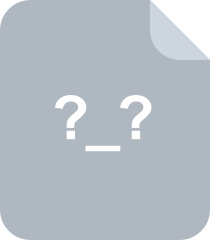
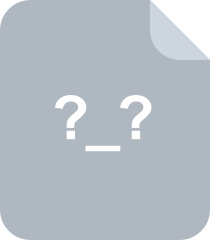


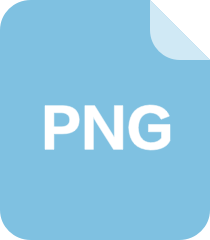
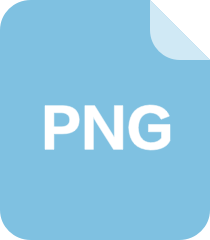
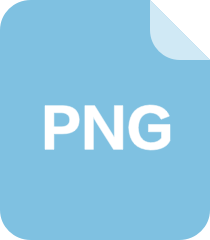
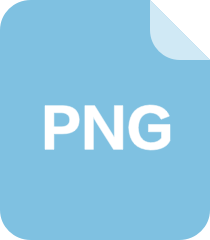

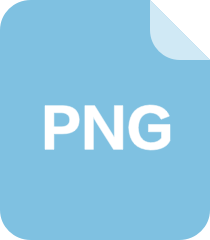
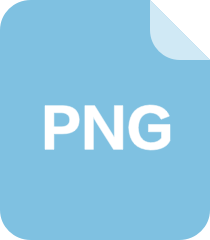
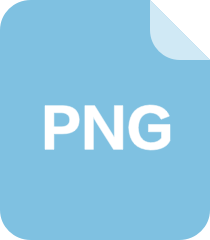
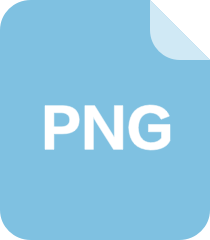

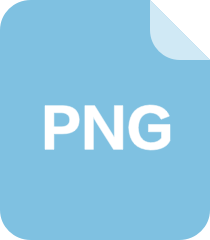
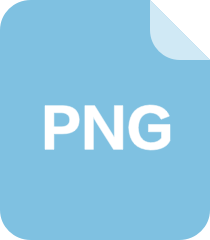
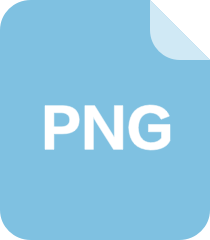
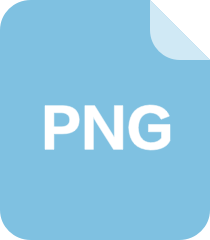

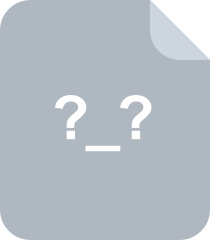

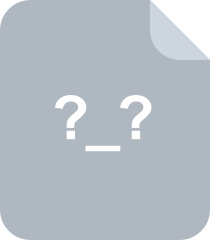
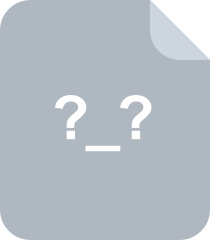
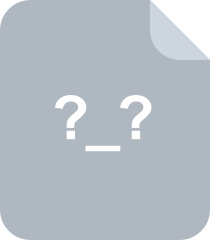
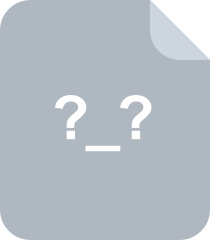

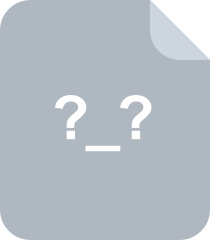
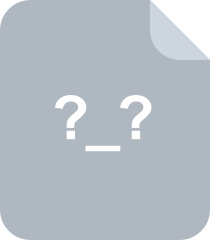
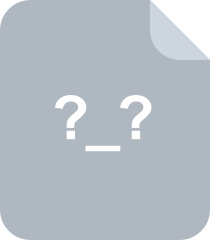
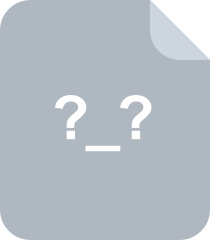
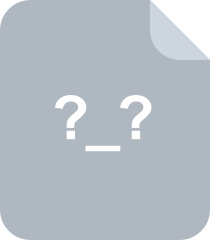
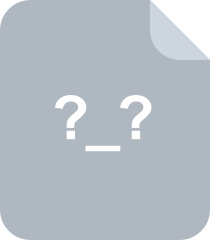

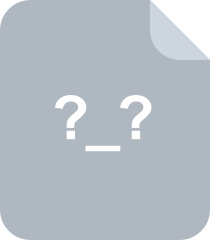
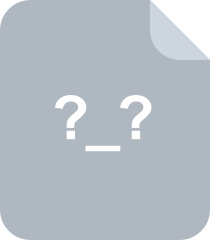
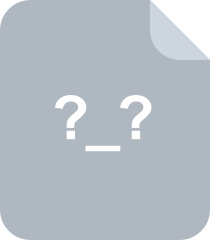
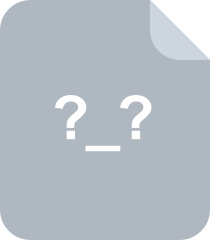
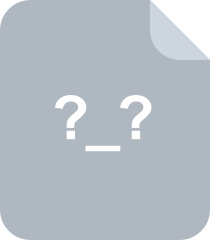
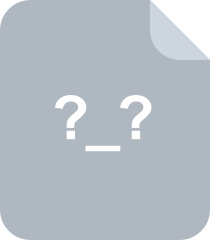
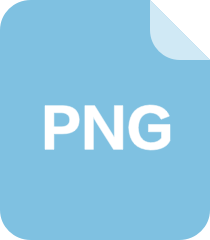
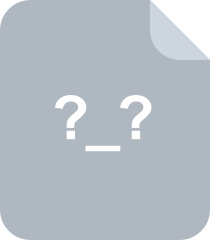

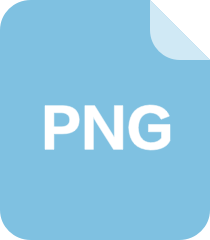
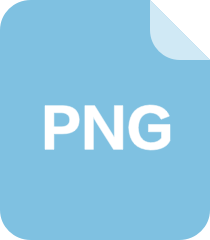
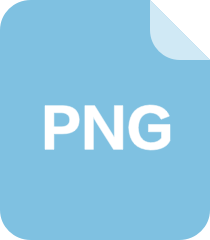
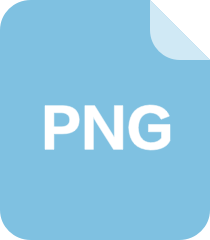
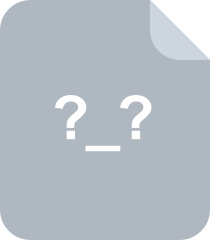
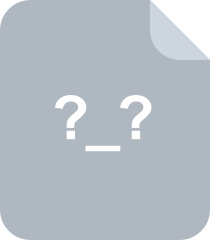
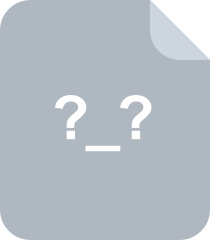
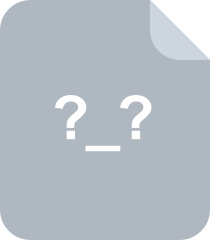
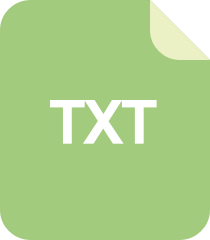
共 72 条
- 1
资源评论
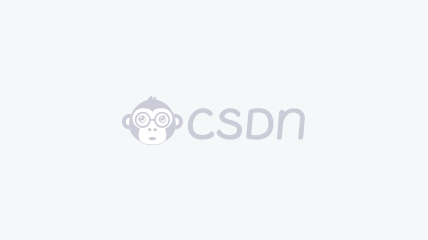

金枝玉叶9
- 粉丝: 195
- 资源: 7637
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

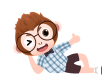
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


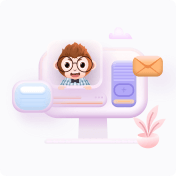
安全验证
文档复制为VIP权益,开通VIP直接复制
