# Viewer.js
[](https://travis-ci.org/fengyuanchen/viewerjs) [](https://www.npmjs.com/package/viewerjs) [](https://www.npmjs.com/package/viewerjs)
> JavaScript image viewer.
- [Website](https://fengyuanchen.github.io/viewerjs)
- [jquery-viewer](https://github.com/fengyuanchen/jquery-viewer) - A jQuery plugin wrapper for Viewer.js.
## Table of contents
- [Features](#features)
- [Main](#main)
- [Getting started](#getting-started)
- [Keyboard support](#keyboard-support)
- [Options](#options)
- [Methods](#methods)
- [Events](#events)
- [No conflict](#no-conflict)
- [Browser support](#browser-support)
- [Contributing](#contributing)
- [Versioning](#versioning)
- [License](#license)
## Features
- Supports 42 [options](#options)
- Supports 23 [methods](#methods)
- Supports 9 [events](#events)
- Supports modal and inline modes
- Supports touch
- Supports move
- Supports zoom
- Supports rotation
- Supports scale (flip)
- Supports keyboard
- Cross-browser support
## Main
```text
dist/
├── viewer.css
├── viewer.min.css (compressed)
├── viewer.js (UMD)
├── viewer.min.js (UMD, compressed)
├── viewer.common.js (CommonJS, default)
└── viewer.esm.js (ES Module)
```
## Getting started
### Installation
```shell
npm install viewerjs
```
In browser:
```html
<link href="/path/to/viewer.css" rel="stylesheet">
<script src="/path/to/viewer.js"></script>
```
The [cdnjs](https://github.com/cdnjs/cdnjs) provides CDN support for Viewer.js's CSS and JavaScript. You can find the links [here](https://cdnjs.com/libraries/viewerjs).
### Usage
#### Syntax
```js
new Viewer(element[, options])
```
- **element**
- Type: `HTMLElement`
- The target image or container of images for viewing.
- **options** (optional)
- Type: `Object`
- The options for viewing. Check out the available [options](#options).
#### Example
```html
<!-- a block container is required -->
<div>
<img id="image" src="picture.jpg" alt="Picture">
</div>
<div>
<ul id="images">
<li><img src="picture-1.jpg" alt="Picture 1"></li>
<li><img src="picture-2.jpg" alt="Picture 2"></li>
<li><img src="picture-3.jpg" alt="Picture 3"></li>
</ul>
</div>
```
```js
// You should import the CSS file.
// import 'viewerjs/dist/viewer.css';
import Viewer from 'viewerjs';
// View an image
const viewer = new Viewer(document.getElementById('image'), {
inline: true,
viewed() {
viewer.zoomTo(1);
},
});
// Then, show the image by click it, or call `viewer.show()`.
// View a list of images
const gallery = new Viewer(document.getElementById('images'));
// Then, show one image by click it, or call `gallery.show()`.
```
## Keyboard support
> Only available in modal mode.
- `Esc`: Exit full screen or close the viewer or exit modal mode or stop play.
- `Space`: Stop play.
- `←`: View the previous image.
- `→`: View the next image.
- `↑`: Zoom in the image.
- `↓`: Zoom out the image.
- `Ctrl + 0`: Zoom out to initial size.
- `Ctrl + 1`: Zoom in to natural size.
[⬆ back to top](#table-of-contents)
## Options
You may set viewer options with `new Viewer(image, options)`.
If you want to change the global default options, You may use `Viewer.setDefaults(options)`.
### backdrop
- Type: `Boolean` or `String`
- Default: `true`
Enable a modal backdrop, specify `static` for a backdrop which doesn't close the modal on click.
### button
- Type: `Boolean`
- Default: `true`
Show the button on the top-right of the viewer.
### navbar
- Type: `Boolean` or `Number`
- Default: `true`
- Options:
- `0` or `false`: hide the navbar
- `1` or `true`: show the navbar
- `2`: show the navbar only when the screen width is greater than 768 pixels
- `3`: show the navbar only when the screen width is greater than 992 pixels
- `4`: show the navbar only when the screen width is greater than 1200 pixels
Specify the visibility of the navbar.
### title
- Type: `Boolean` or `Number` or `Function` or `Array`
- Default: `true`
- Options:
- `0` or `false`: hide the title
- `1` or `true` or `Function` or `Array`: show the title
- `2`: show the title only when the screen width is greater than 768 pixels
- `3`: show the title only when the screen width is greater than 992 pixels
- `4`: show the title only when the screen width is greater than 1200 pixels
- `Function`: customize the title content
- `[Number, Function]`: the first element indicate the visibility, the second element customize the title content
Specify the visibility and the content of the title.
> The name comes from the `alt` attribute of an image element or the image name parsed from URL.
For example, `title: 4` equals to:
```js
new Viewer(image, {
title: [4, (image, imageData) => `${image.alt} (${imageData.naturalWidth} × ${imageData.naturalHeight})`]
});
```
### toolbar
- Type: `Boolean` or `Number` or `Object`
- Default: `true`
- Options:
- `0` or `false`: hide the toolbar.
- `1` or `true`: show the toolbar.
- `2`: show the toolbar only when the screen width is greater than 768 pixels.
- `3`: show the toolbar only when the screen width is greater than 992 pixels.
- `4`: show the toolbar only when the screen width is greater than 1200 pixels.
- `{ key: Boolean | Number }`: show or hide the toolbar.
- `{ key: String }`: customize the size of the button.
- `{ key: Function }`: customize the click handler of the button.
- `{ key: { show: Boolean | Number, size: String, click: Function }`: customize each property of the button.
- Available keys: "zoomIn", "zoomOut", "oneToOne", "reset", "prev", "play", "next", "rotateLeft", "rotateRight", "flipHorizontal" and "flipVertical".
- Available sizes: "small", "medium" (default) and "large".
Specify the visibility and layout of the toolbar its buttons.
For example, `toolbar: 4` equals to:
```js
new Viewer(image, {
toolbar: {
zoomIn: 4,
zoomOut: 4,
oneToOne: 4,
reset: 4,
prev: 4,
play: {
show: 4,
size: 'large',
},
next: 4,
rotateLeft: 4,
rotateRight: 4,
flipHorizontal: 4,
flipVertical: 4,
},
});
```
### className
- Type: `String`
- Default: `''`
Custom class name(s) to add to the viewer's root element.
### container
- Type: `Element` or `String`
- Default: `'body'`
- An element or a valid selector for [Document.querySelector](https://developer.mozilla.org/en-US/docs/Web/API/Document/querySelector)
The container to put the viewer in modal mode.
> Only available when the `inline` option is set to `false`.
### filter
- Type: `Function`
- Default: `null`
Filter the images for viewing (should return `true` if the image is viewable).
For example:
```js
new Viewer(image, {
filter(image) {
return image.complete;
},
});
```
### fullscreen
- Type: `Boolean`
- Default: `true`
Enable to request full screen when play.
> Requires the browser supports [Full Screen API](https://caniuse.com/fullscreen).
### initialViewIndex
- Type: `Number`
- Default: `0`
Define the initial index of image for viewing.
> Also used as the default parameter value of the `view` method.
### inline
- Type: `Boolean`
- Default: `false`
Enable inline mode.
### interval
- Type: `Number`
- Default: `5000`
The amount of time to delay between automatically cycling an image when playing.
### keyboard
- Type: `Boolean`
- Default: `true`
Enable keyboard support.
### loading
- Type: `Boolean`
- Default: `true`
Indicate if show a loading spinner when load image or not.
### loop
- Type: `Boolean`
- Default: `true`
Indicate if enable loop viewing or not.
> If the current image is the last one, then the next one to view is the first one, and vice versa.
### minWidth
- Type: `Number`
- Default: 200
Define the minimum width of the viewer.
> Only available in inline
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
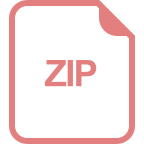
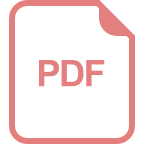
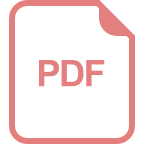
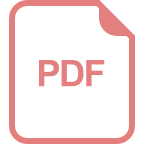
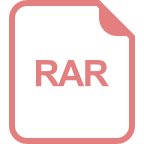
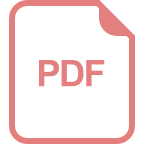
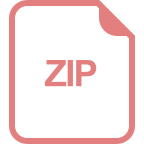
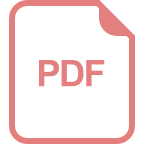
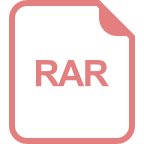
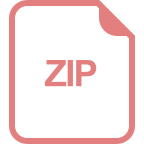
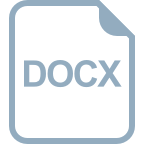
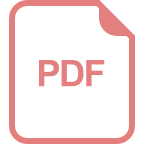
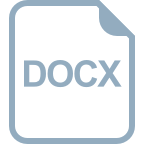
收起资源包目录

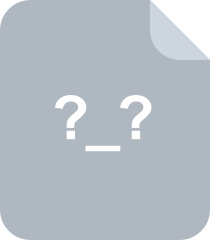
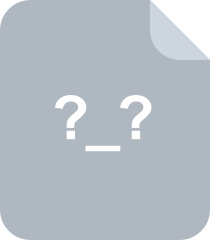
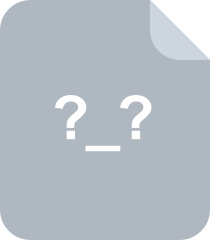
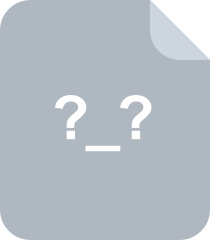
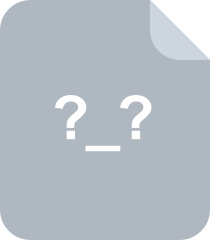
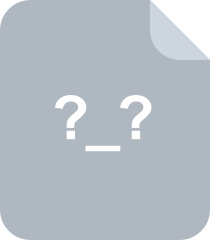
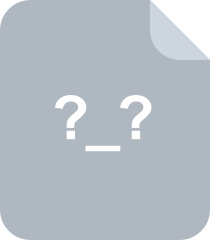
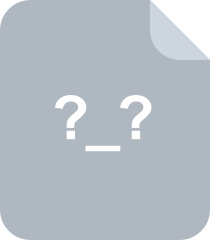
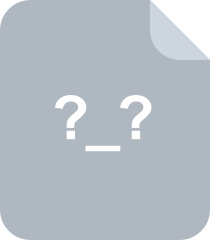
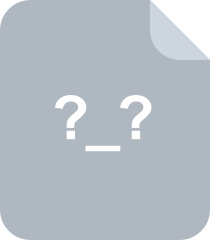
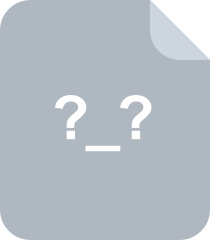
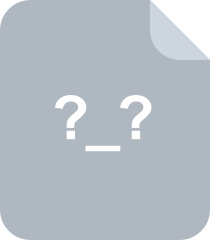
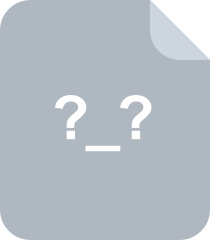
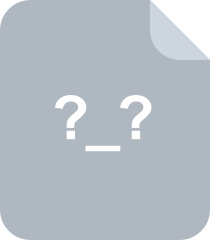
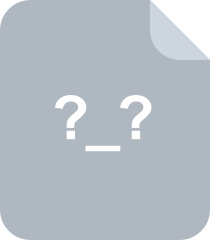
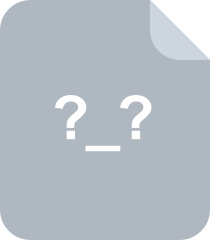
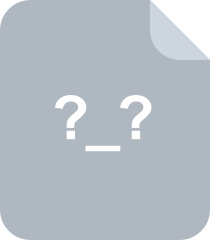
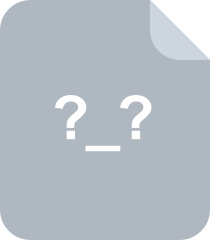
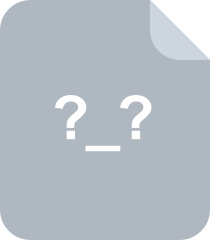
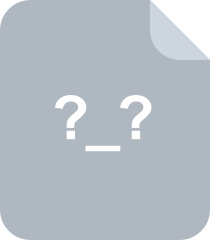
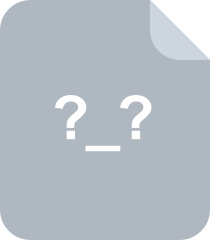
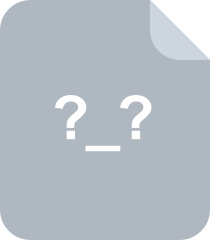
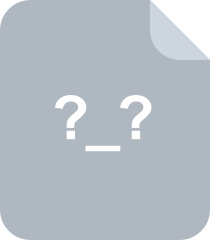
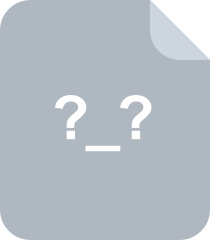
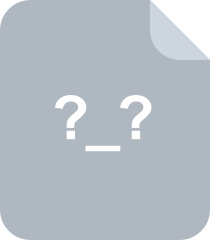
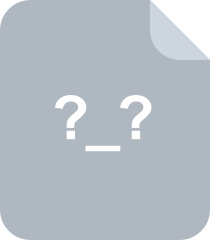
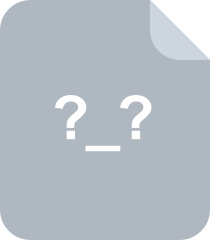
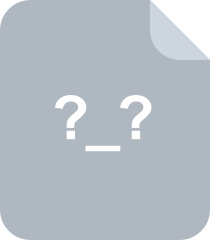
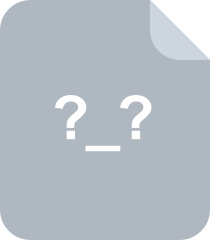
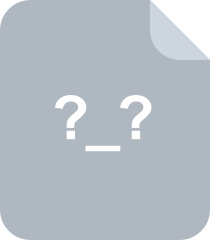
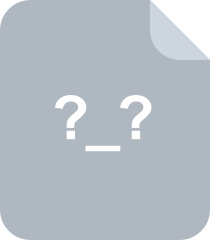
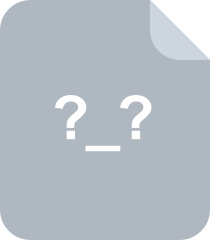
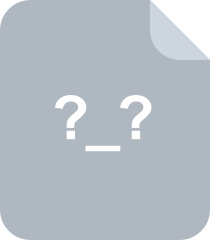
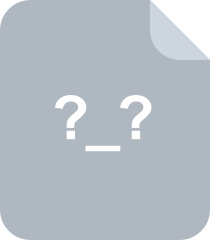
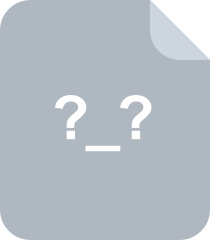
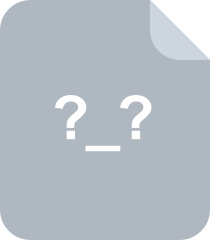
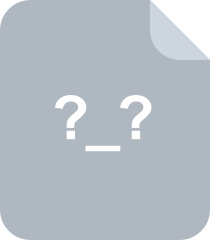
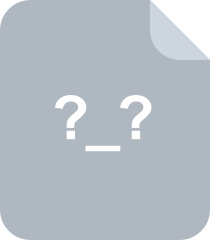
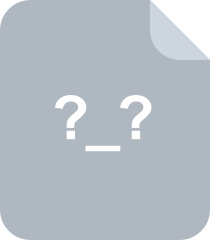
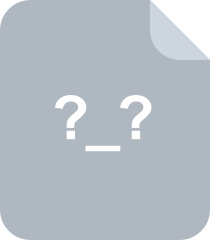
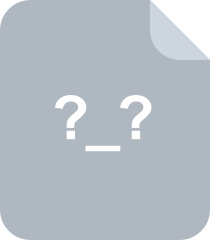
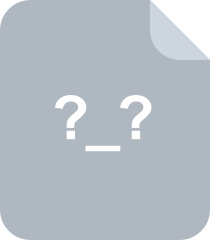
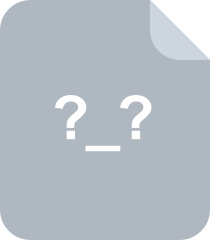
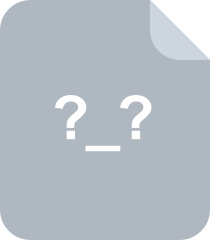
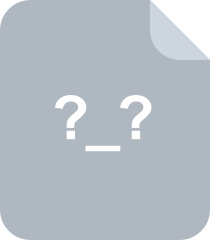
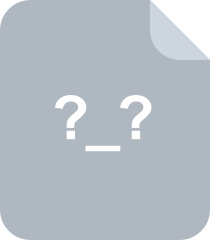
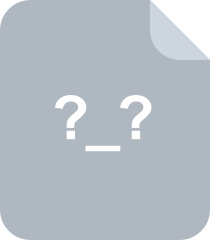
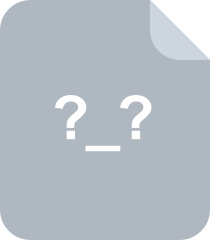
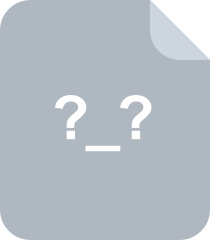
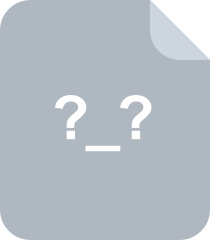
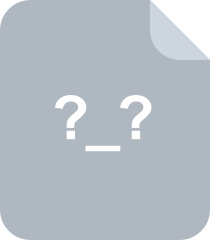
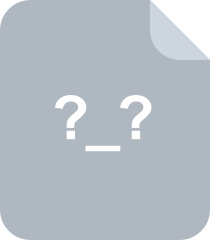
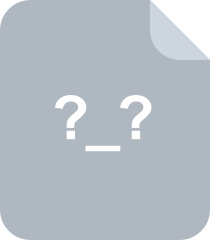
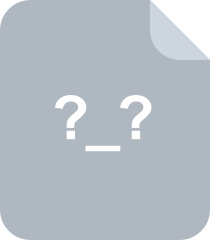
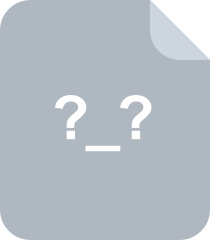
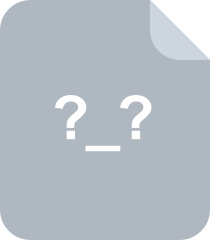
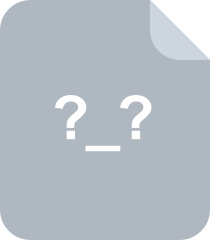
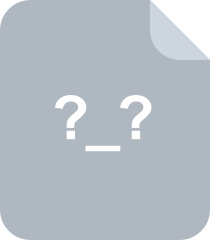
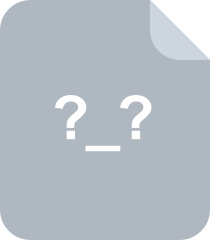
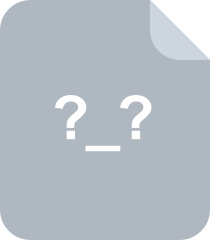
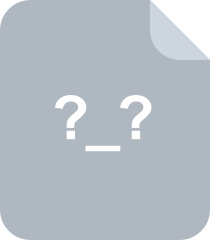
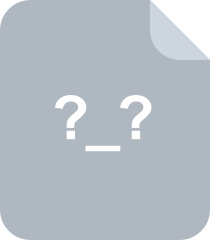
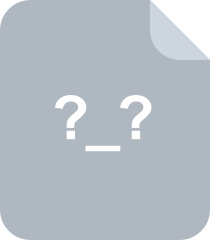
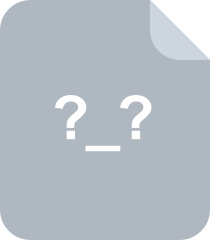
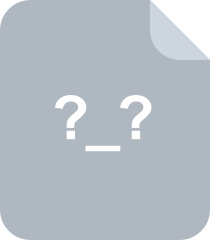
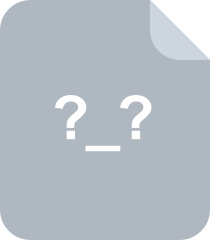
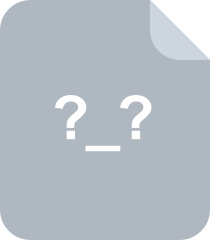
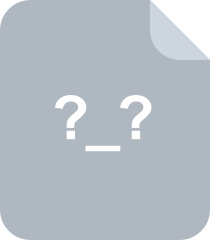
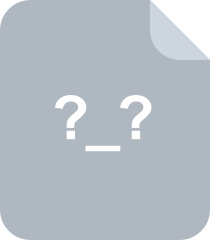
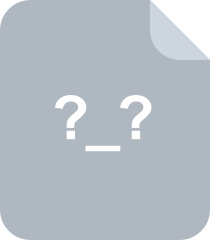
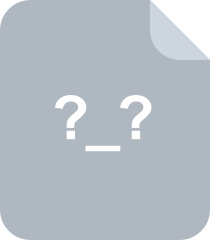
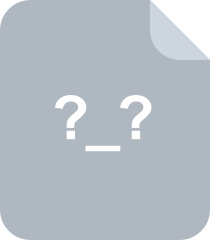
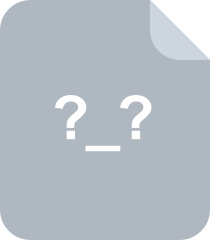
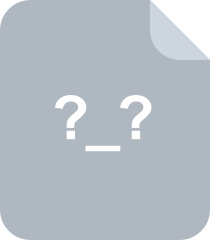
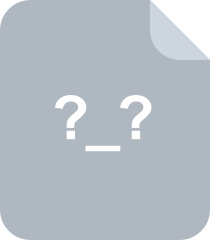
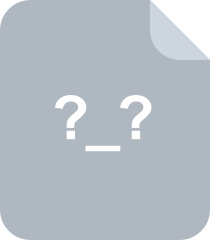
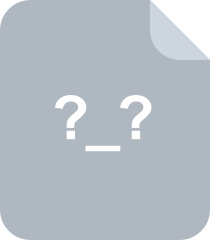
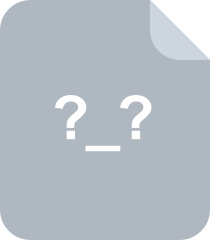
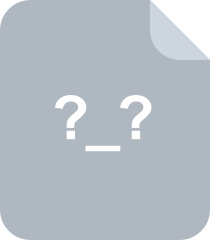
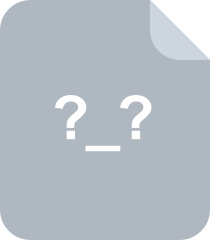
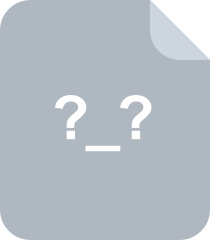
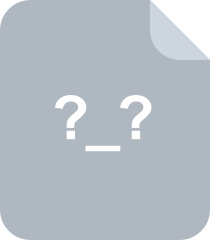
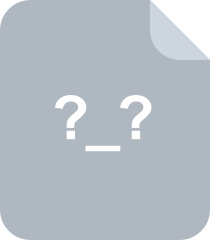
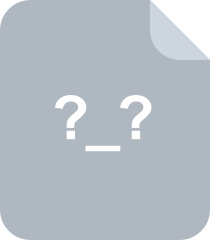
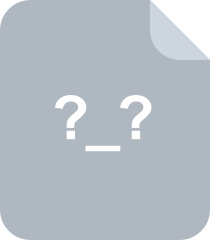
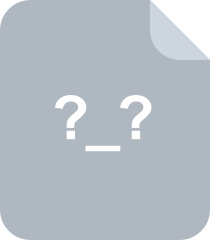
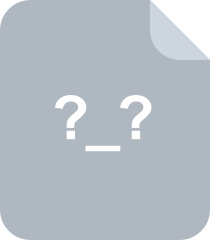
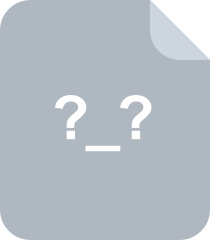
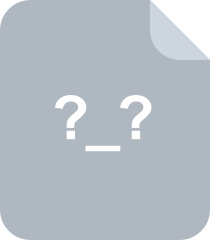
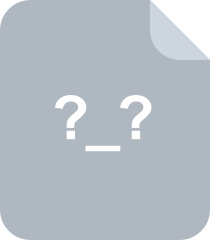
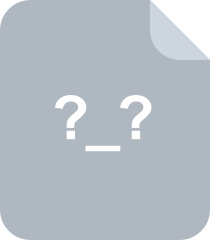
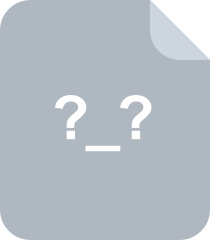
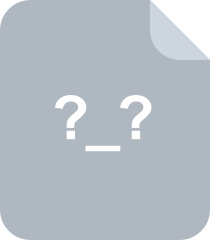
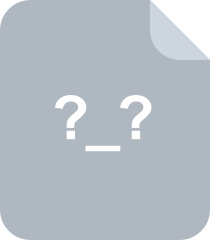
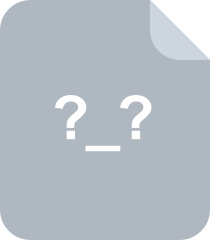
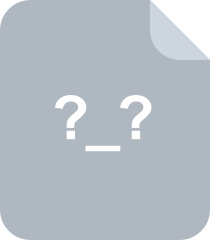
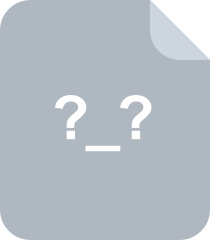
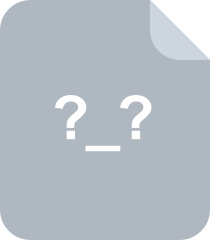
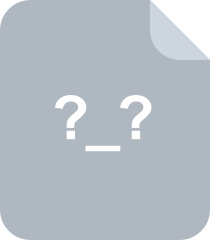
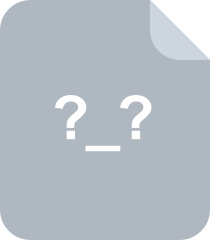
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
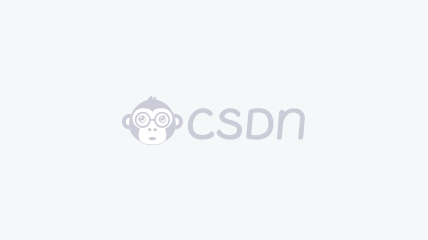

苹果酱0567
- 粉丝: 1220
- 资源: 403
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

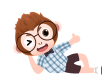
最新资源
- 数据库中实现数据完整性约束的深入探讨
- 采用Qt开发Wireshark的pcapng数据根据数据长度和字头的重新发送软件(源码)
- 卡尔曼滤波效果; mix.wav是混音的音频 k.wav是滤波后的音频
- 使用Python Turtle和Matplotlib绘制爱心图案
- CVE-2024-51567 CyberPanel upgrademysqlstatus 远程命令执行批量检测poc
- 字符串文本批量替换修改工具软件 -使用教程视频
- 中国古籍北斗经.pdf
- 《太上玄霊北斗本命延生真経》,万历四十三年刻本.pdf
- 数据库领域 MySQL 主从复制的原理与配置详解
- CVE-2024-51567 CyberPanel upgrademysqlstatus 远程命令执行poc
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


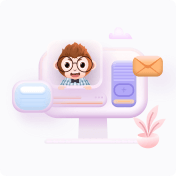
安全验证
文档复制为VIP权益,开通VIP直接复制
