### 如何使用Visual C# 加密和解密文件 在现代软件开发中,数据安全变得越来越重要。为了保护敏感信息不被未授权访问,开发者经常需要实现数据加密功能。本篇文章将详细介绍如何利用Visual C# 来加密和解密文件。 #### 知识点概述 1. **System.IO**: 提供了文件读写的基本操作。 2. **System.Security**: 包含了一系列与安全相关的类。 3. **System.Security.Cryptography**: 提供了多种加密和解密算法的实现。 4. **CryptoStream 类**: 专门用于处理流形式的数据加密和解密。 5. **DES (Data Encryption Standard)**: 一种对称加密算法,用于加密和解密数据。 #### 使用的命名空间 ```csharp using System; using System.IO; using System.Security; using System.Security.Cryptography; using System.Runtime.InteropServices; using System.Text; ``` #### 代码解析 ##### 1. 密钥管理和清理 ```csharp // 清除内存中的密钥以提高安全性 [DllImport("KERNEL32.DLL", EntryPoint = "RtlZeroMemory")] public static extern bool ZeroMemory(IntPtr Destination, int Length); // 生成64位密钥 static string GenerateKey() { // 创建 DES 对象 DESCryptoServiceProvider desCrypto = (DESCryptoServiceProvider)DESCryptoServiceProvider.Create(); // 使用自动生成的密钥 return ASCIIEncoding.ASCII.GetString(desCrypto.Key); } ``` #### 加密文件 ```csharp static void EncryptFile(string sInputFilename, string sOutputFilename, string sKey) { // 打开输入文件 FileStream fsInput = new FileStream(sInputFilename, FileMode.Open, FileAccess.Read); // 创建输出文件 FileStream fsEncrypted = new FileStream(sOutputFilename, FileMode.Create, FileAccess.Write); // 创建 DES 对象并设置密钥 DESCryptoServiceProvider DES = new DESCryptoServiceProvider(); DES.Key = ASCIIEncoding.ASCII.GetBytes(sKey); DES.IV = ASCIIEncoding.ASCII.GetBytes(sKey); // 创建加密器 ICryptoTransform desEncrypt = DES.CreateEncryptor(); // 创建加密流 CryptoStream cryptoStream = new CryptoStream(fsEncrypted, desEncrypt, CryptoStreamMode.Write); // 读取原始文件内容 byte[] byteArrayInput = new byte[fsInput.Length]; fsInput.Read(byteArrayInput, 0, byteArrayInput.Length); // 加密文件内容 cryptoStream.Write(byteArrayInput, 0, byteArrayInput.Length); // 关闭流 cryptoStream.Close(); fsInput.Close(); fsEncrypted.Close(); } ``` #### 解密文件 ```csharp static void DecryptFile(string sInputFilename, string sOutputFilename, string sKey) { // 创建 DES 对象并设置密钥 DESCryptoServiceProvider DES = new DESCryptoServiceProvider(); DES.Key = ASCIIEncoding.ASCII.GetBytes(sKey); DES.IV = ASCIIEncoding.ASCII.GetBytes(sKey); // 创建文件流以读取加密文件 FileStream fsRead = new FileStream(sInputFilename, FileMode.Open, FileAccess.Read); // 创建 DES 解密器 ICryptoTransform desDecrypt = DES.CreateDecryptor(); // 创建解密流 CryptoStream cryptoStream = new CryptoStream(fsRead, desDecrypt, CryptoStreamMode.Read); // 创建临时缓冲区 byte[] tempBuffer = new byte[fsRead.Length]; // 读取加密数据 int bytesRead = cryptoStream.Read(tempBuffer, 0, (int)fsRead.Length); // 创建输出文件 FileStream fsWrite = new FileStream(sOutputFilename, FileMode.Create, FileAccess.Write); // 写入解密后的数据 fsWrite.Write(tempBuffer, 0, bytesRead); // 关闭流 cryptoStream.Close(); fsRead.Close(); fsWrite.Close(); } ``` #### 结论 通过以上示例代码,我们可以看到如何使用 Visual C# 和 .NET Framework 的 `System.Security.Cryptography` 命名空间中的类来实现文件的加密和解密功能。这些技术对于保护用户数据至关重要,并且在实际应用中非常有用。了解并掌握这些基础知识有助于开发者构建更加安全可靠的应用程序。
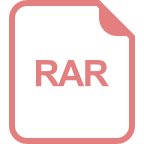
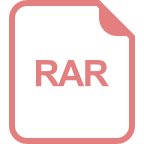
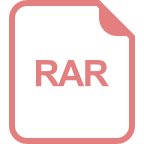
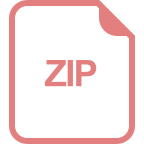
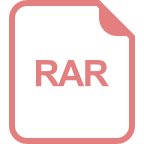
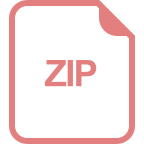
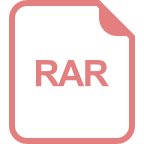
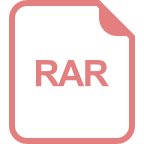
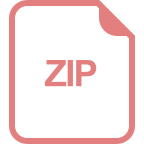
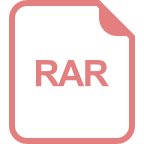
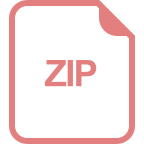
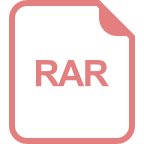
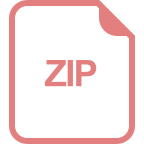
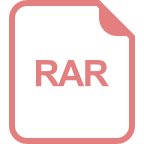
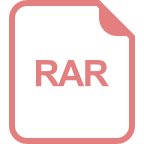
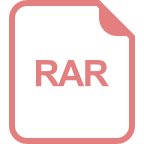
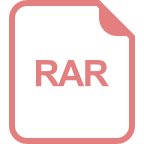
using System.IO;
using System.Security;
using System.Security.Cryptography;
using System.Runtime.InteropServices;
using System.Text;
namespace CSEncryptDecrypt
{
class Class1
{
// Call this function to remove the key from memory after use for security
[System.Runtime.InteropServices.DllImport("KERNEL32.DLL", EntryPoint="RtlZeroMemory")]
public static extern bool ZeroMemory(IntPtr Destination, int Length);
// Function to Generate a 64 bits Key.
static string GenerateKey()
{
// Create an instance of Symetric Algorithm. Key and IV is generated automatically.
DESCryptoServiceProvider desCrypto =(DESCryptoServiceProvider)DESCryptoServiceProvider.Create();
// Use the Automatically generated key for Encryption.
return ASCIIEncoding.ASCII.GetString(desCrypto.Key);
}
static void EncryptFile(string sInputFilename,
string sOutputFilename,
string sKey)
{
FileStream fsInput = new FileStream(sInputFilename,
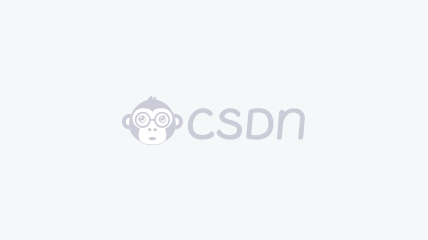

- 粉丝: 1
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

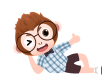
最新资源
- SIMULINK 基于反推控制速度控制器的永磁同步电机控制系统研究
- springboot037基于SpringBoot的墙绘产品展示交易平台的设计与实现.zip
- springboot239华府便利店信息管理系统_0303173844.zip
- springboot239华府便利店信息管理系统.zip
- springboot038基于SpringBoot的网上租赁系统设计与实现.zip
- 关于AUTOSAR组织结构的介绍ppt
- 三菱FX3U与台达变频器通讯 器件:三菱FX3U PLC+F X3U 485BD板,台达VFD变频器,昆仑通态触摸屏 功能:采用485方式,modbus RTU协议,对台达变频器频率设定,正反转,点动
- springboot040社区医院信息平台.zip
- springboot039基于Web足球青训俱乐部管理后台系统开发.zip
- springboot240基于Spring boot的名城小区物业管理系统.zip
- springboot041师生健康信息管理系统.zip
- springboot242基于SpringBoot的失物招领平台的设计与实现.zip
- 基于三菱PLC和组态王供暖控制系统热器控制 带解释的梯形图程序,接线图原理图图纸,io分配,组态画面
- springboot241基于SpringBoot+Vue的电商应用系统的设计与实现.zip
- C++、基于OpenCV和MFC框架的口罩缺陷检测.zip
- springboot042IT技术交流和分享平台的设计与实现.zip

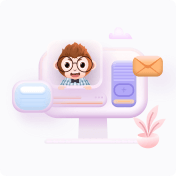
