中文转utf8 16

### 知识点总结 #### 一、UTF-8 编码原理与16进制转换 **UTF-8(8位 Unicode 转换格式)**是一种变长字符编码,用于表示 Unicode 字符集。它能高效地表示 ASCII 字符,并支持世界上几乎所有的字符。在 UTF-8 中,一个字符可以占用 1 至 4 个字节。对于中文字符,通常使用 3 个字节来表示。 #### 二、Java 中的字符编码转换 Java 提供了丰富的 API 来处理不同的字符编码。在这个示例中,主要关注的是如何将 GB2312(或 GBK)编码的字符串转换为 UTF-8 编码。 #### 三、代码分析 ##### 1. 主类 `utf8` ```java public class utf8 { public static void main(String[] args) { String str = "This is a test for *!@#$"; try { File f = new File("D:/test.txt"); FileOutputStream fio = new FileOutputStream(f); String s = gbToUtf8(str); fio.write(s.getBytes("UTF-8")); fio.close(); } catch (Exception e) { e.printStackTrace(); } } //... } ``` - **功能描述**:主方法创建了一个文本文件 `test.txt`,并将字符串 `"This is a test for *!@#$"` 从 GB2312 编码转换为 UTF-8 编码后写入该文件。 - **关键点**: - 使用 `File` 和 `FileOutputStream` 类进行文件操作。 - `gbToUtf8` 方法用于编码转换。 ##### 2. GB2312 到 UTF-8 的转换方法 `gbToUtf8` ```java public static String gbToUtf8(String str) throws UnsupportedEncodingException { StringBuffer sb = new StringBuffer(); for (int i = 0; i < str.length(); i++) { String s = str.substring(i, i + 1); if (s.charAt(0) > 0x80) { byte[] bytes = s.getBytes("Unicode"); String binaryStr = ""; for (int j = 2; j < bytes.length; j += 2) { // 处理 Unicode 编码 //... // 最终将 Unicode 转换为 UTF-8 //... } sb.append(ss); } else { sb.append(s); } } return sb.toString(); } ``` - **功能描述**:此方法实现了从 GB2312 编码到 UTF-8 编码的转换。它首先检查每个字符是否是 ASCII 范围内的字符(即是否小于 0x80),如果是,则直接添加到结果中;如果不是,则将其从 GB2312 编码转换为 Unicode 编码,再从 Unicode 编码转换为 UTF-8 编码。 - **关键点**: - 使用 `getBytes("Unicode")` 获取字符的 Unicode 编码。 - 将 Unicode 编码转换为 UTF-8 编码的过程较为复杂,涉及到二进制位操作。 ##### 3. 辅助方法 `getHexString` 和 `getBinaryString` 这两个辅助方法用于获取字节的十六进制表示和二进制表示: ```java private static String getHexString(byte b) { String hexStr = Integer.toHexString(b); int m = hexStr.length(); if (m < 2) { hexStr = "0" + hexStr; } else { hexStr = hexStr.substring(m - 2); } return hexStr; } private static String getBinaryString(int i) { String binaryStr = Integer.toBinaryString(i); int length = binaryStr.length(); for (int l = 0; l < 8 - length; l++) { binaryStr = "0" + binaryStr; } return binaryStr; } ``` - **功能描述**:`getHexString` 方法将一个字节转换为两位十六进制数字的字符串。`getBinaryString` 方法将一个整数转换为八位的二进制字符串。 - **关键点**: - `getHexString` 确保返回的字符串始终为两位。 - `getBinaryString` 确保返回的字符串始终为八位。 ### 总结 通过上述分析,我们可以看到 Java 提供了多种方式来处理不同编码的字符串。本示例中的 `gbToUtf8` 方法提供了一种将 GB2312 编码转换为 UTF-8 编码的方法,尽管其实现较为复杂,但能够很好地实现编码转换的需求。对于实际应用来说,通常可以直接使用 Java 内置的方法如 `new String(bytes, "UTF-8")` 或 `string.getBytes("GB2312")` 进行编码转换,这些方法更加简洁且易于理解。
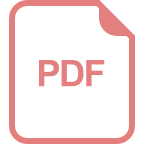
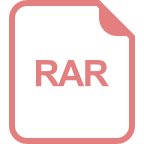
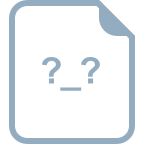
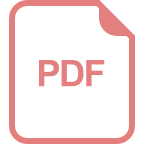
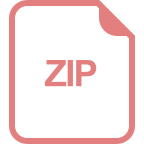
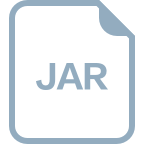
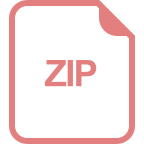
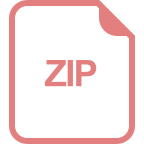
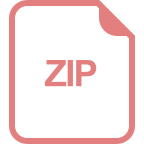
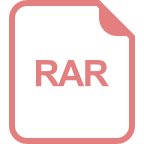
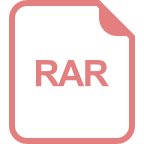
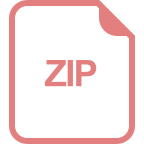
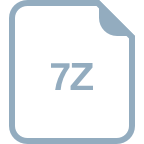
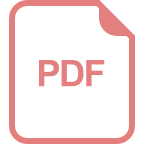
import java.io.File;
import java.io.FileOutputStream;
import java.io.UnsupportedEncodingException;
public class utf8 {
public static void main(String[] args) {
String str = "This is a test for *ÖÐÍø!@#$¡££¬£¿";
try {
File f = new File("D:/test.txt");
FileOutputStream fio = new FileOutputStream(f);
String s = gbToUtf8(str);
fio.write(s.getBytes("UTF-8"));
fio.close(); }
catch (Exception e) {
e.printStackTrace();
}
}
public static String gbToUtf8(String str) throws UnsupportedEncodingException {
StringBuffer sb = new StringBuffer();
for (int i = 0; i < str.length(); i++) {
String s = str.substring(i, i + 1);
if (s.charAt(0) > 0x80) {
byte[] bytes = s.getBytes("Unicode");
String binaryStr = "";
for (int j = 2; j < bytes.length; j += 2) {
// the first byte
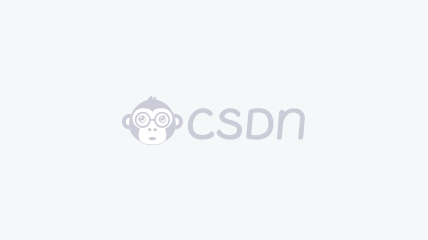
- shujieqiu2013-02-18非常好用的小软件,能够对文本进行编码转换,特别是UTF16
- reder19882013-05-29不好用,没有起到作用
- heisetaiyang2013-03-11简单,能用
- gongweijun0072013-08-06很实用 对我帮助很大
- 想娜娜的虫2013-04-11不是很好用 白花了

- 粉丝: 0
- 资源: 30
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

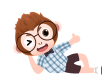
