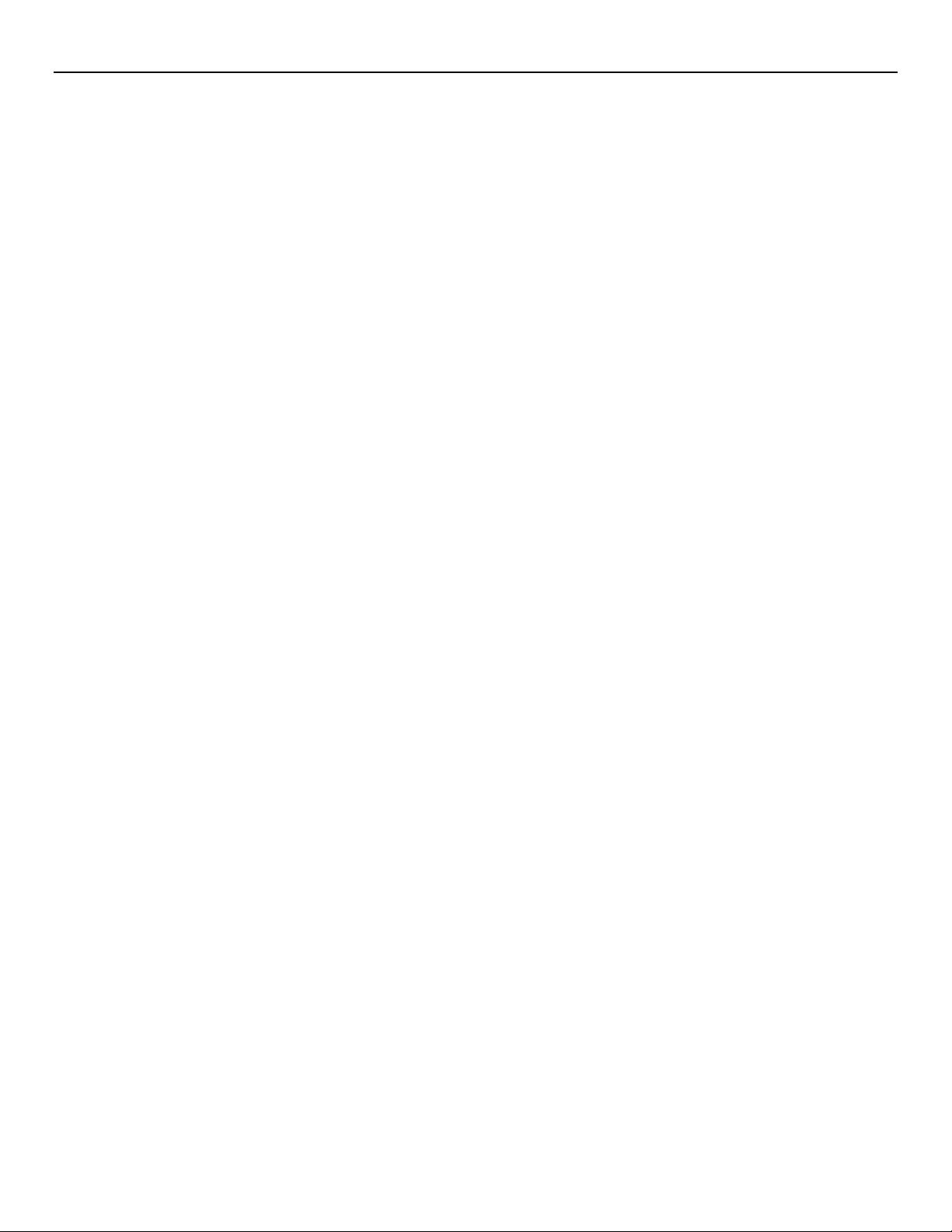
Windows via C/C++, Fifth Edition by Jeffrey Richter and Christophe Nasarre
Windows via C/C++, Fifth Edition .....................................................................................................................................................7
List of Figures ......................................................................................................................................................................... 10
List of Tables .......................................................................................................................................................................... 12
Introduction ........................................................................................................................................................................... 14
Overview ........................................................................................................................................................................ 14
64-Bit Windows ............................................................................................................................................................. 15
What's New in the Fifth Edition ..................................................................................................................................... 16
Code Samples and System Requirements ..................................................................................................................... 17
Support for This Book .................................................................................................................................................... 17
Part I: Required Reading ........................................................................................................................................................ 18
Chapter 1: Error Handling ...................................................................................................................................... 19
Overview ........................................................................................................................................................ 19
Defining Your Own Error Codes ..................................................................................................................... 23
The ErrorShow Sample Application ............................................................................................................... 24
Chapter 2: Working with Characters and Strings ................................................................................................... 26
Overview ........................................................................................................................................................ 26
Character Encodings ...................................................................................................................................... 27
ANSI and Unicode Character and String Data Types ...................................................................................... 29
Unicode and ANSI Functions in Windows ...................................................................................................... 31
Unicode and ANSI Functions in the C Run-Time Library ................................................................................ 33
Secure String Functions in the C Run-Time Library ........................................................................................ 34
Why You Should Use Unicode ........................................................................................................................ 42
How We Recommend Working with Characters and Strings ......................................................................... 42
Translating Strings Between Unicode and ANSI ............................................................................................. 43
Chapter 3: Kernel Objects ...................................................................................................................................... 49
Overview ........................................................................................................................................................ 49
What Is a Kernel Object? ............................................................................................................................... 50
A Process' Kernel Object Handle Table .......................................................................................................... 54
Sharing Kernel Objects Across Process Boundaries ....................................................................................... 59
Part II: Getting Work Done .................................................................................................................................................... 79
Chapter 4: Processes.............................................................................................................................................. 80
Overview ........................................................................................................................................................ 80
Writing Your First Windows Application ........................................................................................................ 81
The CreateProcess Function .......................................................................................................................... 99
Terminating a Process .................................................................................................................................. 114
Child Processes ............................................................................................................................................ 117
When Administrator Runs as a Standard User ............................................................................................ 120
Chapter 5: Jobs .................................................................................................................................................... 134
Overview ...................................................................................................................................................... 134
Placing Restrictions on a Job's Processes..................................................................................................... 138
Placing a Process in a Job ............................................................................................................................. 145
Terminating All Processes in a Job ............................................................................................................... 146
Job Notifications .......................................................................................................................................... 149
The Job Lab Sample Application .................................................................................................................. 152