### C 语言库函数使用大全 #### 1. 函数名: abort - **功能**:此函数用于异常终止一个进程。 - **用法**:`void abort(void);` - **参数**:此函数无参数。 - **返回值**:无返回值。一旦调用此函数,进程将被立即终止,不会执行后续代码。 - **示例**: ```c #include <stdio.h> #include <stdlib.h> int main(void) { printf("Calling abort()\n"); abort(); return 0; /* This is never reached */ } ``` - **说明**:通常在遇到不可恢复的错误时使用此函数来结束程序。 #### 2. 函数名: abs - **功能**:此函数用于计算整数的绝对值。 - **用法**:`int abs(int i);` - **参数**:接受一个整型参数 `i`。 - **返回值**:返回 `i` 的绝对值。 - **示例**: ```c #include <stdio.h> #include <math.h> int main(void) { int number = -1234; printf("Number: %d Absolute value: %d\n", number, abs(number)); return 0; } ``` - **说明**:适用于整数类型的绝对值计算。 #### 3. 函数名: absread, abswrite - **功能**:这两个函数用于绝对磁盘扇区的读写操作。 - **用法**: - `int absread(int drive, int nsects, int sectno, void *buffer);` - `int abswrite(int drive, int nsects, int sectno, void *buffer);` - **参数**: - `drive`: 驱动器编号。 - `nsects`: 扇区数量。 - `sectno`: 起始扇区号。 - `buffer`: 数据缓冲区指针。 - **返回值**:如果成功则返回0,失败返回非零值。 - **示例**: ```c #include <stdio.h> #include <conio.h> #include <process.h> #include <dos.h> int main(void) { int i, strt, ch_out, sector; char buf[512]; printf("Insert a diskette into drive A and press any key\n"); getch(); sector = 0; if (absread(0, 1, sector, &buf) != 0) { perror("Disk problem"); exit(1); } printf("Read OK\n"); strt = 3; for (i = 0; i < 80; i++) { ch_out = buf[strt + i]; putchar(ch_out); } printf("\n"); return 0; } ``` - **说明**:这些函数用于直接读写磁盘扇区,主要用于低级磁盘操作。 #### 4. 函数名: access - **功能**:此函数用于检查指定文件是否存在以及是否具有指定的访问权限。 - **用法**:`int access(const char *filename, int amode);` - **参数**: - `filename`: 文件路径。 - `amode`: 检查模式(例如 F_OK 检查文件存在性,R_OK 检查读权限等)。 - **返回值**:若文件存在且符合访问条件,则返回0;否则返回-1。 - **示例**: ```c #include <stdio.h> #include <io.h> int file_exists(char *filename); int main(void) { printf("Does NOTEXIST.FIL exist: %s\n", file_exists("NOTEXISTS.FIL") ? "YES" : "NO"); return 0; } int file_exists(char *filename) { return access(filename, 0) == 0; } ``` - **说明**:此函数常用于判断文件或目录是否存在,并检查其访问权限。 #### 5. 函数名: acos - **功能**:此函数用于计算给定数值的反余弦值。 - **用法**:`double acos(double x);` - **参数**:接受一个 `double` 类型的参数 `x`,范围为 [-1, 1]。 - **返回值**:返回 `x` 的反余弦值(弧度制)。 - **示例**: ```c #include <stdio.h> #include <math.h> int main(void) { double result; double x = 0.5; result = acos(x); printf("The arccosine of %lf is %lf\n", x, result); return 0; } ``` - **说明**:用于数学运算中的角度转换,输入值必须在 [-1, 1] 区间内。 #### 6. 函数名: allocmem - **功能**:此函数用于分配 DOS 存储段。 - **用法**:`int allocmem(unsigned size, unsigned *seg);` - **参数**: - `size`: 分配内存大小(以段为单位,每个段16字节)。 - `seg`: 指向一个变量,用于存储分配内存的段地址。 - **返回值**:如果成功,则返回 0;如果失败,则返回一个非零值。 - **示例**: ```c #include <dos.h> #include <alloc.h> #include <stdio.h> int main(void) { unsigned int size, segp; int stat; size = 64; /* (64x16)=1024 bytes */ stat = allocmem(size, &segp); if (stat == -1) printf("Allocated memory at segment: %x\n", segp); else printf("Failed: maximum number of paragraphs available is %u\n", stat); return 0; } ``` - **说明**:此函数用于早期的操作系统中进行内存分配,现在已较少使用。 #### 7. 函数名: arc - **功能**:此函数用于绘制一条弧线。 - **用法**:`void far arc(int x, int y, int stangle, int endangle, int radius);` - **参数**: - `x`: 圆心的 x 坐标。 - `y`: 圆心的 y 坐标。 - `stangle`: 开始角度(度)。 - `endangle`: 结束角度(度)。 - `radius`: 半径长度。 - **返回值**:无返回值。 - **示例**:(由于示例代码未完整给出,此处不提供具体示例) - **说明**:用于图形编程中绘制圆弧,常与图形库结合使用。 以上是 C 语言中一些常用库函数的介绍及示例。这些函数涵盖了从文件处理到图形绘制等多个方面,对于 C 语言的学习者来说,掌握这些函数的使用方法是非常重要的。通过实际编写和运行示例代码,可以更好地理解这些函数的功能及其应用场景。
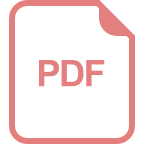
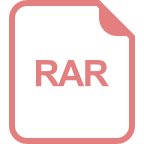
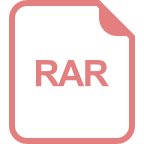
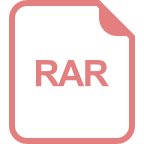
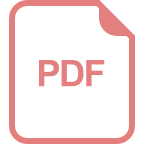
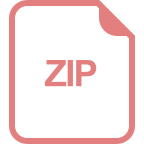
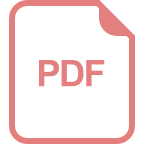
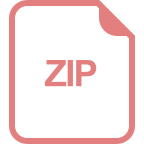
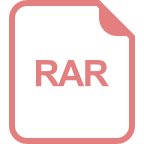
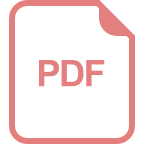
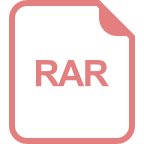
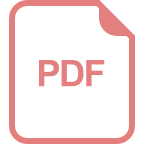
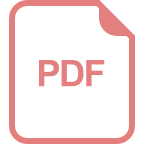
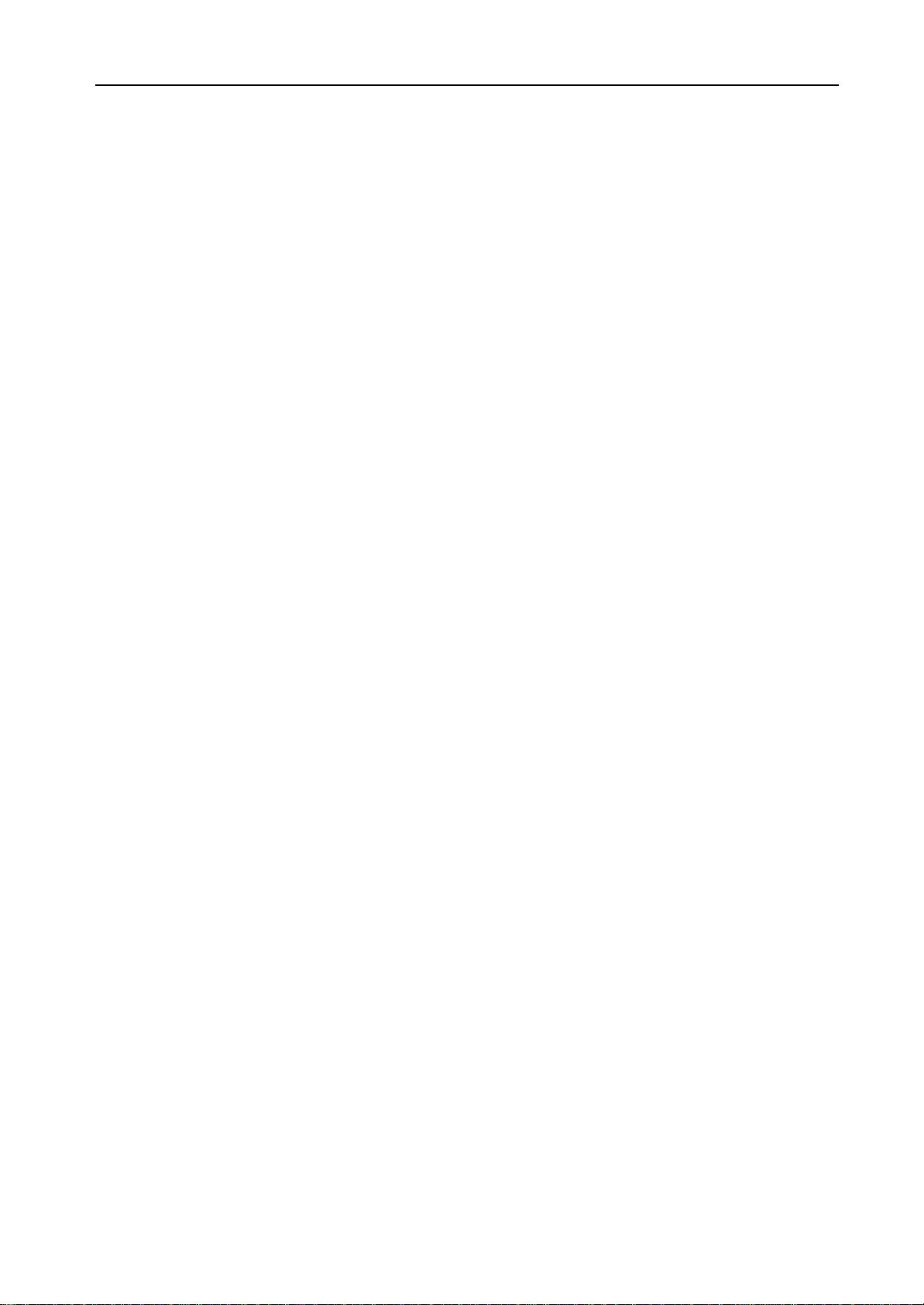
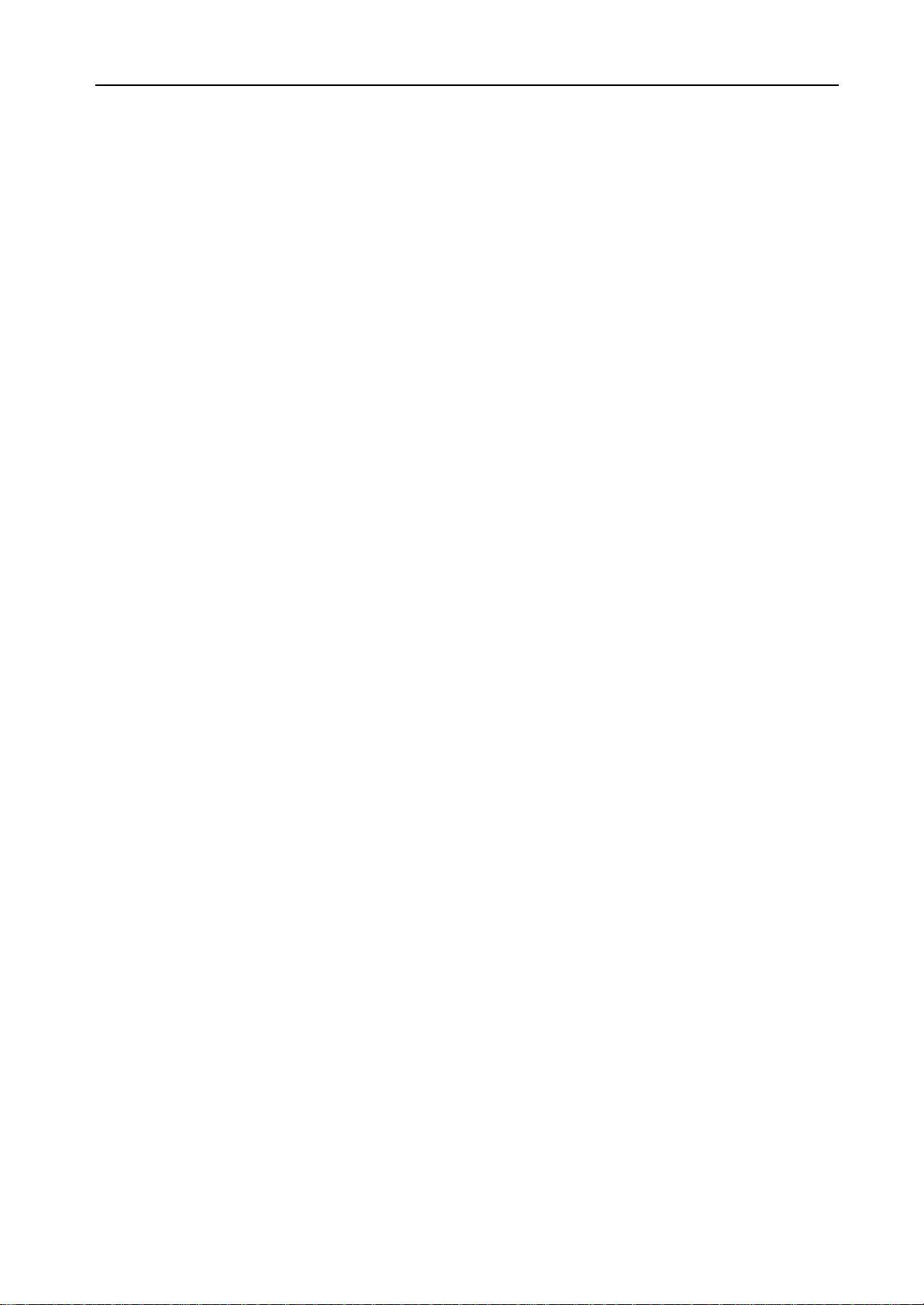
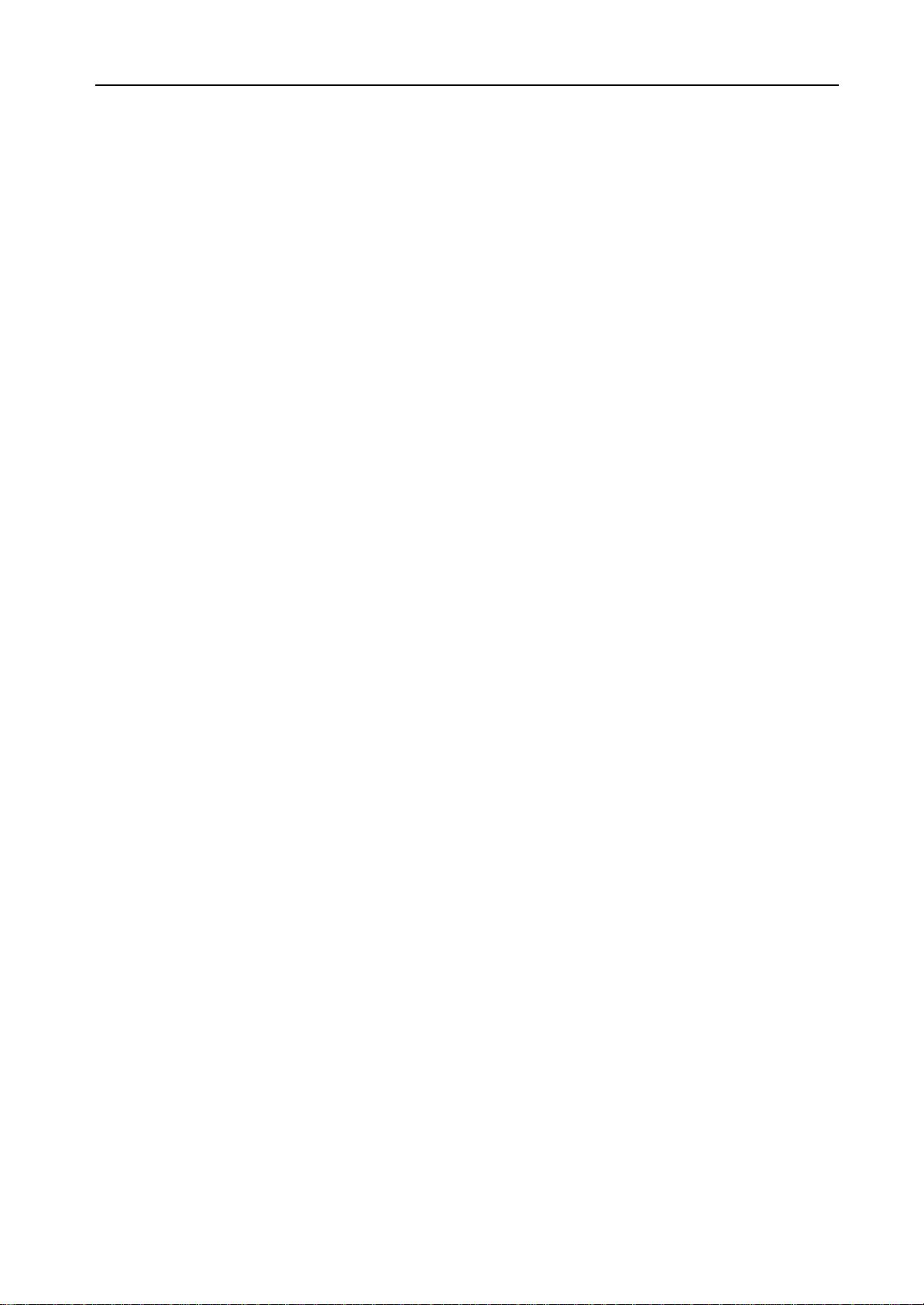
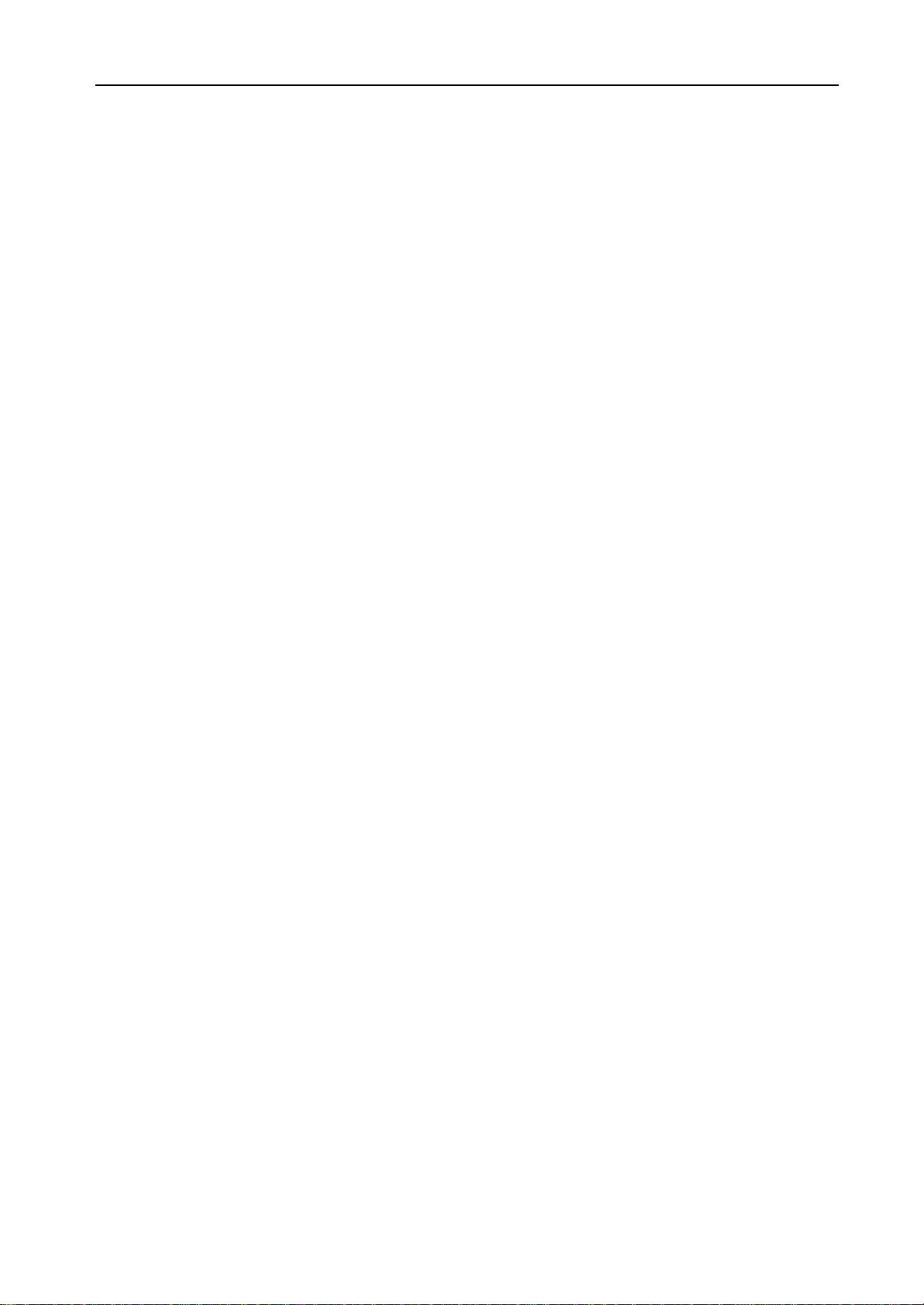
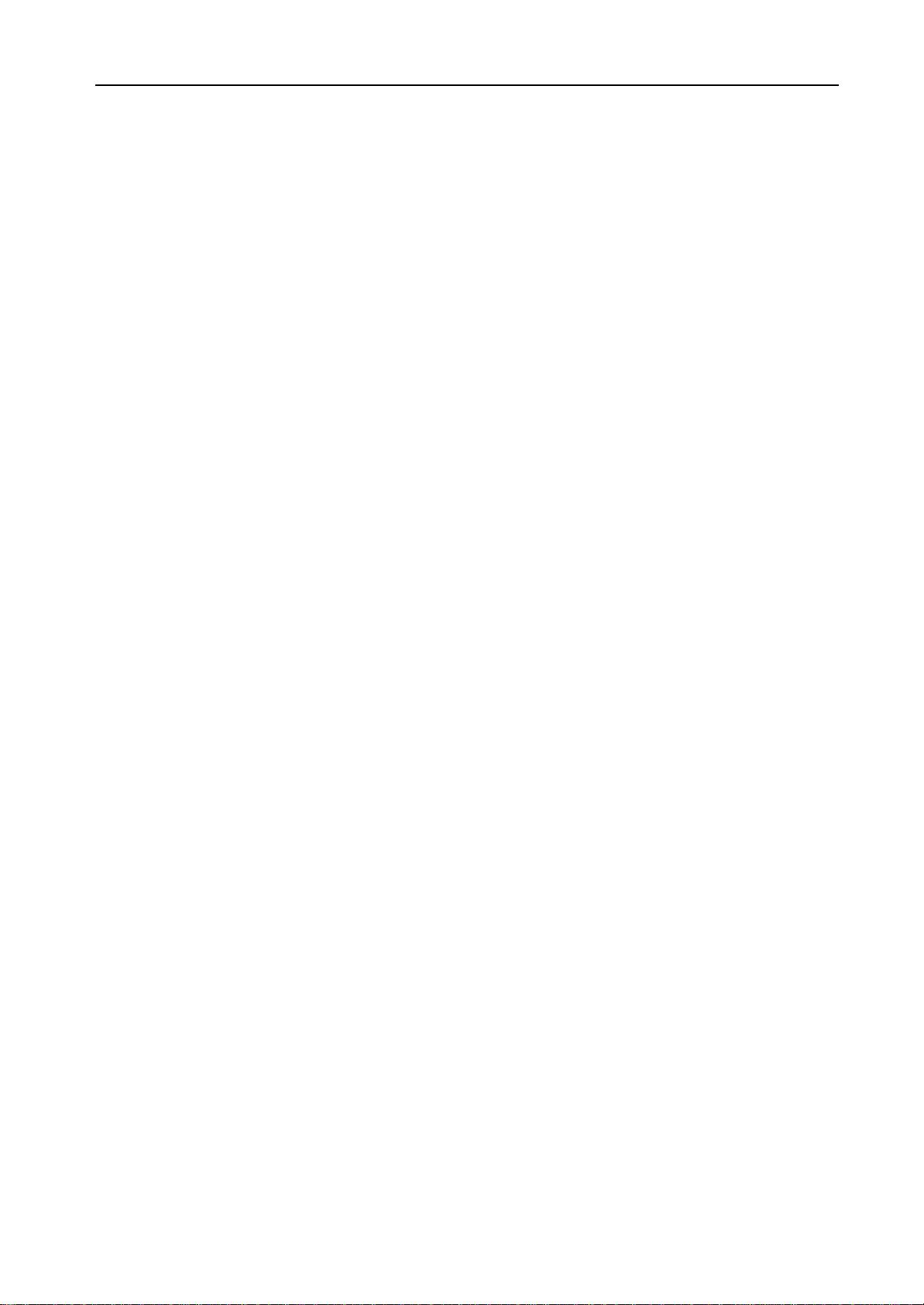
剩余256页未读,继续阅读
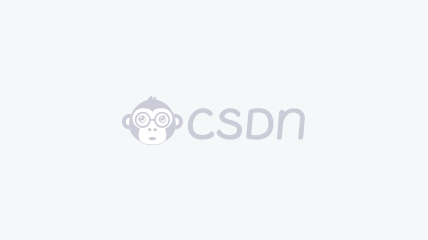

- 粉丝: 0
- 资源: 3
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

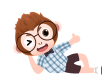
最新资源
- Matlab_Matlab线性算子工具箱.zip
- Matlab_Matlab文件用于各种类型的波束形成.zip
- Matlab_Matlab循环统计工具箱.zip
- Matlab_Matlab中的BP神经网络.zip
- Matlab_Matlab研究工具,读取、写入和处理地震数据.zip
- Matlab_Matlab中的曝光融合.zip
- Matlab_Matlab中的图像视频隐写.zip
- Matlab_Matlab中的图形信号处理.zip
- Matlab_MCMC工具箱的Matlab.zip
- Matlab_Matlab中的遗传算法.zip
- Matlab_MIDI工具箱11 2016是一个分析MIDI文件的Matlab函数集合.zip
- Matlab_MPC的简短例子,特别是随机MPC的SMPC与机会约束的Matlab.zip
- Matlab_NCTOOLBOX一个Matlab工具箱,用于处理常见的数据模型数据集.zip
- Matlab_MTEX是一个免费的Matlab定量纹理分析工具箱主页.zip
- Matlab_PILCO策略搜索框架Matlab版.zip
- Matlab_NIPS 2015论文的Matlab代码和补充材料用于序列建模的深度时序s型信念网络.zip

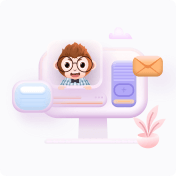
