/*
* Copyright (C) 2007-2009 Geometer Plus <contact@geometerplus.com>
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 2 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301, USA.
*/
#include <windows.h>
#include <commctrl.h>
#include "../../../../core/src/util/ZLKeyUtil.h"
#include "W32Control.h"
#include "W32ControlCollection.h"
#include "../../../../core/src/win32/util/W32WCHARUtil.h"
static const WORD CLASS_BUTTON = 0x0080;
static const WORD CLASS_EDIT = 0x0081;
static const WORD CLASS_STATIC = 0x0082;
static const WORD CLASS_LISTBOX = 0x0083;
static const WORD CLASS_SCROLLBAR = 0x0084;
static const WORD CLASS_COMBOBOX = 0x0085;
static const WCHAR CLASSNAME_SPINNER[] = UPDOWN_CLASSW;
W32Control::W32Control(DWORD style) : myStyle(style | WS_CHILD), myX(1), myY(1), mySize(Size(1, 1)), myEnabled(true), myOwner(0), myWindow(0) {
}
void W32Control::setEnabled(bool enabled) {
if (myEnabled != enabled) {
myEnabled = enabled;
if (myWindow != 0) {
EnableWindow(myWindow, myEnabled);
}
}
}
bool W32Control::isEnabled() const {
return myEnabled;
}
void W32Control::setVisible(bool visible) {
if (visible != isVisible()) {
if (visible) {
myStyle |= WS_VISIBLE;
} else {
myStyle &= ~WS_VISIBLE;
}
if (myWindow != 0) {
ShowWindow(myWindow, visible ? SW_SHOW : SW_HIDE);
}
if (myOwner != 0) {
myOwner->invalidate();
}
}
}
bool W32Control::isVisible() const {
return (myStyle & WS_VISIBLE) == WS_VISIBLE;
}
void W32Control::init(HWND parent, W32ControlCollection *collection) {
myOwner = collection;
myWindow = GetDlgItem(parent, collection->addControl(this));
if (!myEnabled) {
EnableWindow(myWindow, false);
}
}
W32StandardControl::W32StandardControl(DWORD style) : W32Control(style) {
}
int W32StandardControl::controlNumber() const {
return 1;
}
int W32StandardControl::allocationSize() const {
return 14;
}
void W32StandardControl::allocate(WORD *&p, short &id) const {
*p++ = LOWORD(myStyle);
*p++ = HIWORD(myStyle);
*p++ = 0;
*p++ = 0;
*p++ = myX;
*p++ = myY;
/*
if ((mySize.Width == 0) || (mySize.Height == 0)) {
mySize = minimumSize();
}
*/
*p++ = mySize.Width;
*p++ = mySize.Height;
*p++ = id++;
*p++ = 0xFFFF;
*p++ = classId();
*p++ = 0;
*p++ = 0;
*p++ = 0;
}
void W32Control::setPosition(int x, int y, Size size) {
bool doMove = (myX != x) || (myY != y);
bool doResize = mySize != size;
myX = x;
myY = y;
mySize = size;
if ((doResize || doMove) && (myWindow != 0)) {
RECT r;
r.left = x;
r.top = y;
r.right = x + size.Width;
r.bottom = y + size.Height;
MapDialogRect(GetParent(myWindow), &r);
DWORD flags = SWP_NOZORDER | SWP_NOOWNERZORDER;
if (!doResize) {
flags |= SWP_NOSIZE;
}
if (!doMove) {
flags |= SWP_NOMOVE;
}
SetWindowPos(myWindow, 0, r.left, r.top, r.right - r.left, r.bottom - r.top, flags);
}
}
void W32Control::commandCallback(DWORD) {
}
void W32Control::notificationCallback(LPARAM) {
}
void W32Control::drawItemCallback(DRAWITEMSTRUCT&) {
}
const std::string W32PushButton::RELEASED_EVENT = "PushButton: released";
W32PushButton::W32PushButton(const std::string &text, ButtonType type) : W32StandardControl(BS_PUSHBUTTON | WS_TABSTOP), myText(text), myType(type) {
//DWORD style = (it == myButtons.begin()) ? BS_DEFPUSHBUTTON : BS_PUSHBUTTON;
}
W32Widget::Size W32PushButton::minimumSize() const {
return Size(4 * (ZLUnicodeUtil::utf8Length(myText) + 3), 15);
}
void W32PushButton::allocate(WORD *&p, short &id) const {
if (myType == NORMAL_BUTTON) {
W32StandardControl::allocate(p, id);
} else {
short specialId = (myType == OK_BUTTON) ? IDOK : IDCANCEL;
W32StandardControl::allocate(p, specialId);
}
}
WORD W32PushButton::classId() const {
return CLASS_BUTTON;
}
void W32PushButton::init(HWND parent, W32ControlCollection *collection) {
myOwner = collection;
short id = -1;
if (myType == OK_BUTTON) {
id = IDOK;
} else if (myType == CANCEL_BUTTON) {
id = IDCANCEL;
}
myWindow = GetDlgItem(parent, collection->addControl(this, id));
if (!myEnabled) {
EnableWindow(myWindow, false);
}
::setWindowText(myWindow, myText);
}
void W32PushButton::commandCallback(DWORD) {
fireEvent(RELEASED_EVENT);
}
W32Label::W32Label(const std::string &text, Alignment alignment) : W32StandardControl(alignment), myText(text) {
myStringCounter = 1;
myMaxLength = 0;
int index = 0;
int newIndex;
while ((newIndex = myText.find('\n', index)) != -1) {
++myStringCounter;
myMaxLength = std::max(
myMaxLength,
(unsigned short)ZLUnicodeUtil::utf8Length(myText.data() + index, newIndex - index)
);
index = newIndex + 1;
}
myMaxLength = std::max(
myMaxLength,
(unsigned short)ZLUnicodeUtil::utf8Length(myText.data() + index, myText.length() - index)
);
}
W32Widget::Size W32Label::minimumSize() const {
return Size(4 * myMaxLength, 12 * myStringCounter);
}
void W32Label::setPosition(int x, int y, Size size) {
::setWindowText(myWindow, "");
W32StandardControl::setPosition(x, y + 2, Size(size.Width, size.Height - 2));
::setWindowText(myWindow, myText);
}
WORD W32Label::classId() const {
return CLASS_STATIC;
}
void W32Label::init(HWND parent, W32ControlCollection *collection) {
W32StandardControl::init(parent, collection);
::setWindowText(myWindow, myText);
}
W32StandardIcon::W32StandardIcon(IconId iconId) : W32StandardControl(SS_CENTER | SS_ICON), myIconId(iconId) {
}
W32Widget::Size W32StandardIcon::minimumSize() const {
// TODO: why multiplier 2?
return Size(SM_CXICON * 2, SM_CYICON * 2);
}
WORD W32StandardIcon::classId() const {
return CLASS_STATIC;
}
void W32StandardIcon::init(HWND parent, W32ControlCollection *collection) {
W32StandardControl::init(parent, collection);
WCHAR *icon;
switch (myIconId) {
case ID_INFORMATION:
icon = IDI_INFORMATION;
break;
case ID_QUESTION:
icon = IDI_QUESTION;
break;
case ID_ERROR:
icon = IDI_ERROR;
break;
default:
icon = IDI_APPLICATION;
break;
}
SendMessage(myWindow, STM_SETICON, (WPARAM)LoadIcon(0, icon), 0);
}
const std::string W32CheckBox::STATE_CHANGED_EVENT = "CheckBox: state changed";
W32CheckBox::W32CheckBox(const std::string &text) : W32StandardControl(BS_AUTOCHECKBOX | WS_TABSTOP), myText(text), myChecked(false) {
}
W32Widget::Size W32CheckBox::minimumSize() const {
return Size(4 * (ZLUnicodeUtil::utf8Length(myText) + 1), 12);
}
WORD W32CheckBox::classId() const {
return CLASS_BUTTON;
}
void W32CheckBox::init(HWND parent, W32ControlCollection *collection) {
W32StandardControl::init(parent, collection);
::setWindowText(myWindow, myText);
SendMessage(myWindow, BM_SETCHECK, myChecked ? BST_CHECKED : BST_UNCHECKED, 0);
}
void W32CheckBox::setEditable(bool editable) {
if (editable) {
myStyle &= ~WS_DISABLED;
} else {
myStyle |= WS_DISABLED;
}
if (myWindow != 0) {
// TODO: check
SetWindowLong(myWindow, GWL_STYLE, myStyle);
}
}
void W32CheckBox::setChecked(bool checked) {
if (checked != myChecked) {
myChecked = checked;
if (myWindow != 0) {
SendMessage(myWindow, BM_SETCHECK, myChecked ? BST_CHECKED : BST_UNCHECKED, 0);
}
fireEvent(STATE_CHANGED_EVENT);
}
}
bool W32CheckBox::isChecked() const {
return myChecked;
}
void W32CheckBox::commandCallback(DWORD) {
if (myChecked != (SendMessage(myWindow, BM_GETCHECK, 0, 0) == BST_CHECKED)) {
myChecked = !myChecked;
fireEvent(STATE_CHANGED_EVENT);
}
}
const std::string
FBReader-0.11.2

FBReader 是一个流行的开源电子书阅读器,专为多种操作系统设计,包括Linux、Windows 32位以及其他平台。它的核心特点在于提供了一个高效且用户友好的界面,使得电子书阅读体验更加舒适。这款软件的源代码是完全开放的,允许开发者进行自定义和扩展,以满足不同用户的需求和偏好。 在FBReader 0.11.2 版本中,我们可以看到它支持多种UI库,包括Qt3、Qt4、GTK以及Windows 32位的原生库。这些UI库的选择使FBReader能够无缝地融入各种桌面环境,无论是基于GNOME、KDE还是Windows桌面系统,都能提供一致且美观的界面。 Qt3和Qt4是Qt开发框架的不同版本,由The Qt Company提供,广泛用于跨平台应用开发。Qt提供了丰富的图形用户界面组件,使得开发人员可以轻松创建功能强大的应用程序。Qt3是较早的版本,而Qt4则引入了许多新特性和性能优化。 GTK(GIMP Toolkit)是另一个流行的开源GUI库,主要用于Linux和其他Unix-like系统的应用程序开发。GTK以其模块化和可定制性著称,允许开发者根据自己的需求调整界面样式和行为。 在Windows 32位环境下,FBReader使用了原生的Windows API,这使得它在该平台上运行时能获得最佳的性能和兼容性,同时保持与系统其他应用的界面一致性。 压缩包中的"fbreader-0.11.2"可能包含了FBReader的源代码、编译脚本、构建系统配置文件、资源文件以及文档。通过分析这些文件,开发者可以深入理解FBReader的内部工作原理,学习如何利用其提供的API和插件系统来扩展功能,或者为新的平台和设备适配FBReader。 此外,FBReader支持多种电子书格式,如EPUB、PDF、FB2等,这些格式的兼容性使其成为电子书爱好者和开发者的重要工具。它的高度可定制性还允许用户根据自己的喜好调整字体、布局、颜色方案等,以实现个性化的阅读体验。 总而言之,FBReader 0.11.2 是一款强大的、跨平台的开源电子书阅读器,通过其丰富的UI库支持和广泛的平台兼容性,为用户和开发者提供了灵活的选择。其源代码的开放性为社区贡献和功能扩展创造了无限可能,是电子书爱好者和软件开发者值得探索的项目。









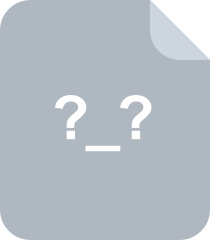
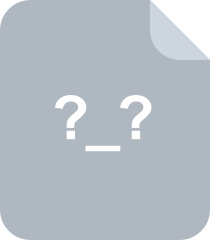
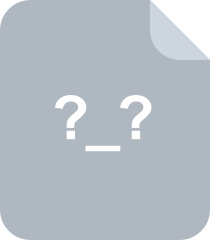
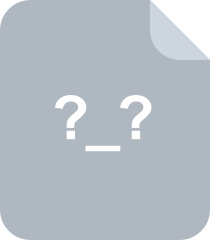
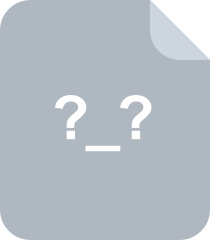
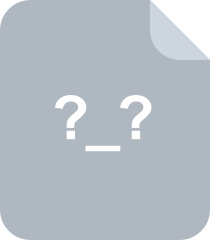
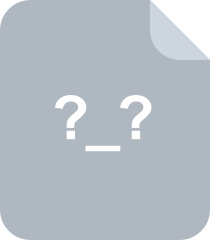
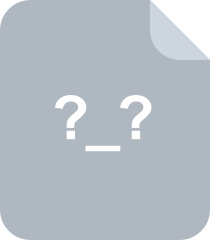
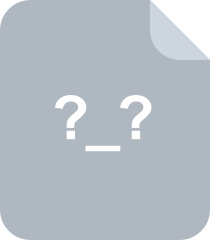
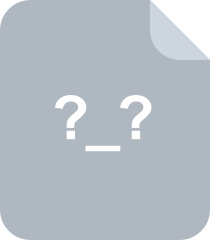
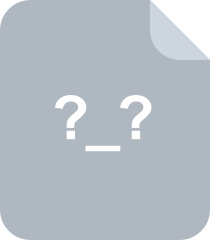
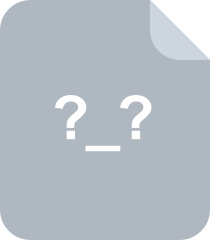
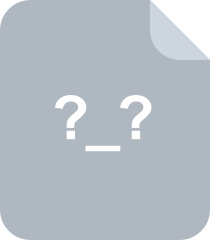
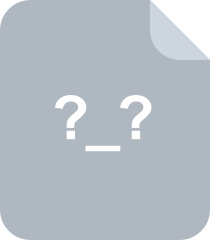
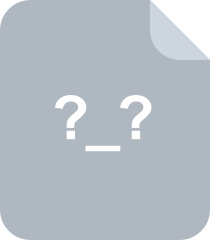
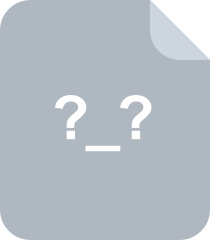
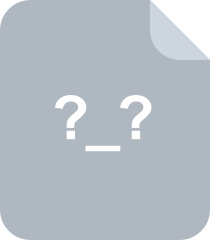
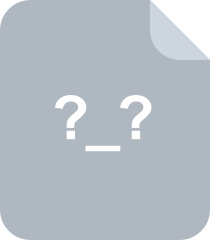
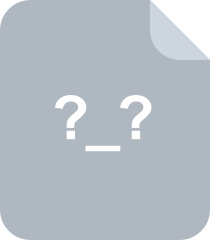
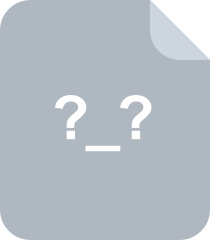
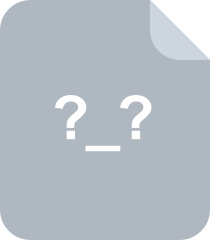
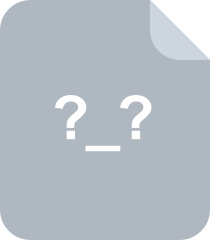
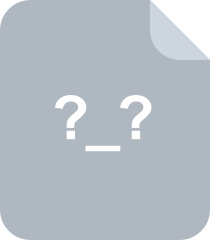
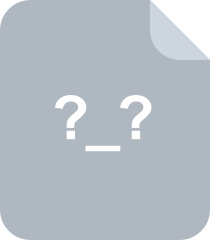
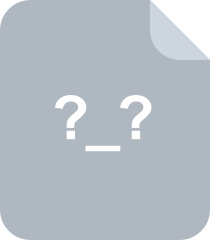
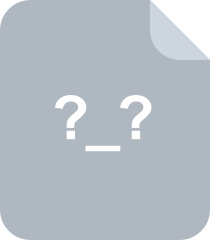
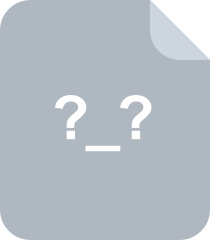
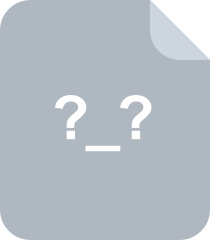
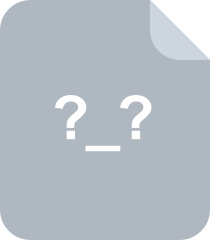
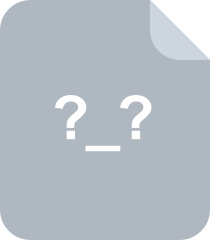
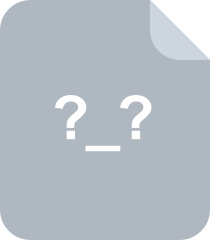
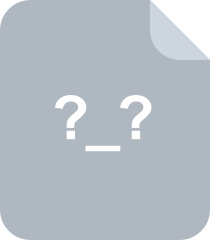
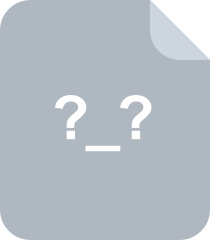
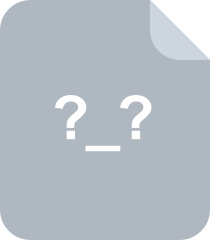
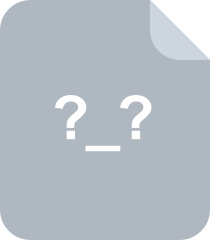
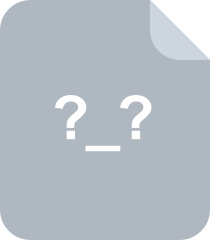
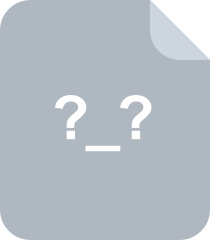
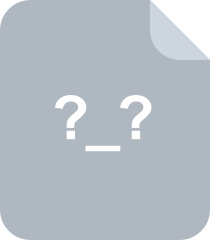
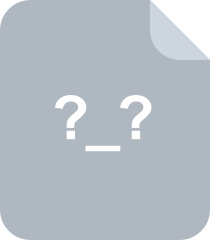
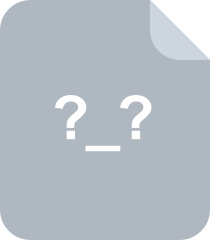
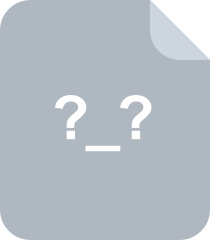
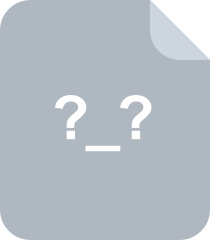
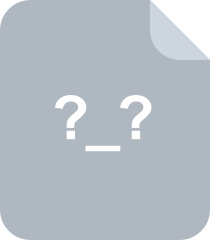
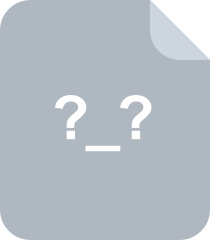
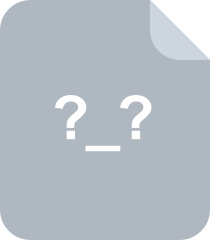
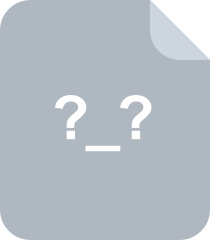
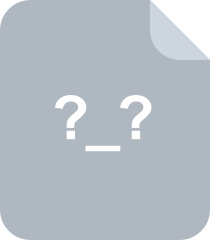
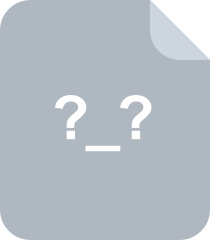
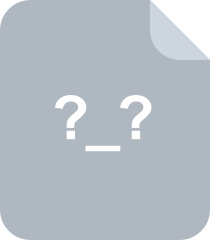
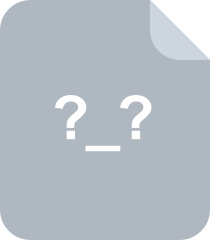
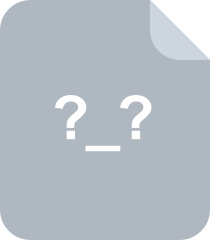
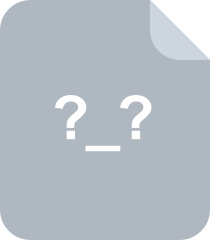
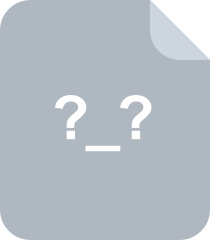
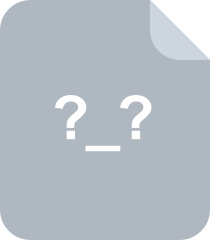
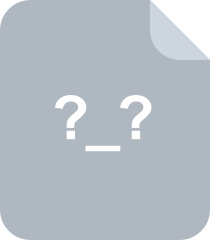
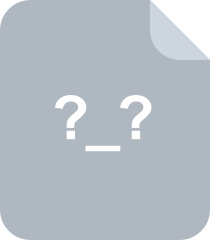
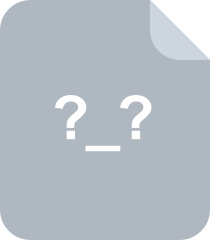
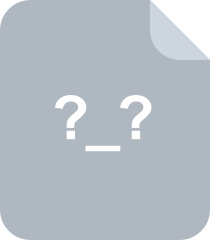
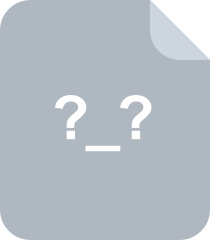
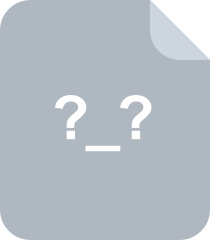
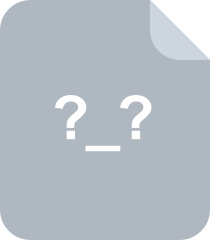
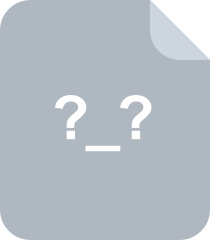
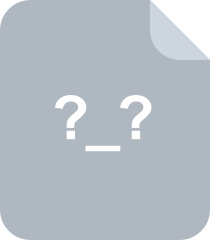
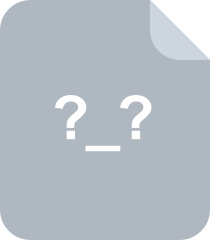
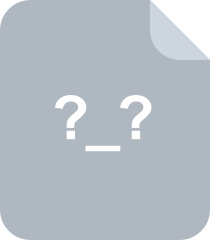
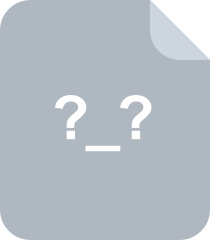
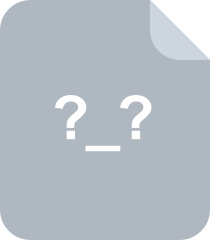
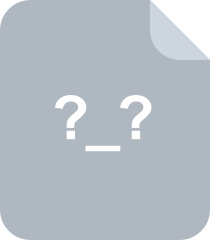
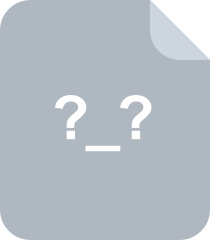
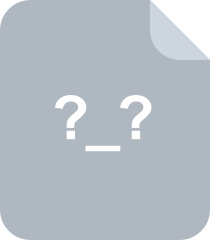
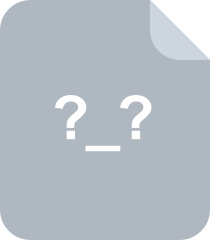
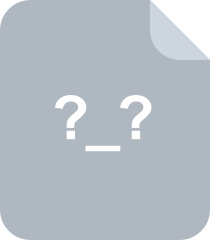
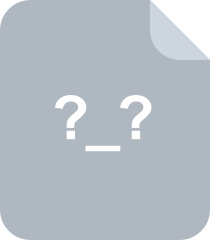
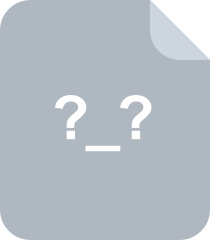
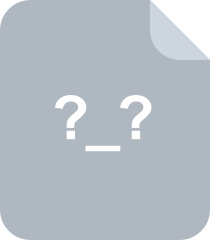
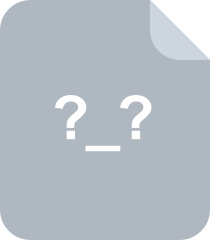
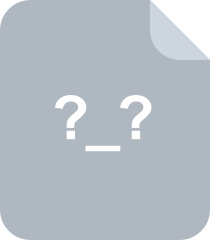
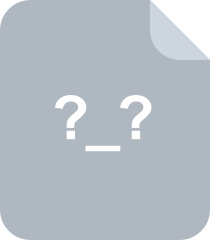
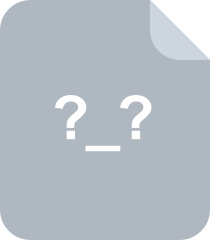
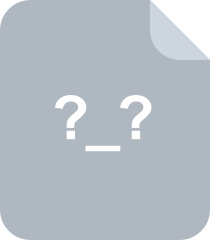
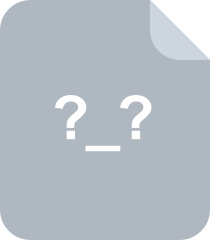
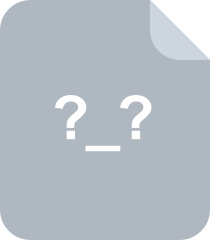
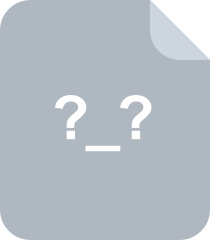
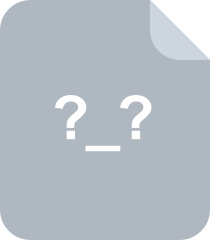
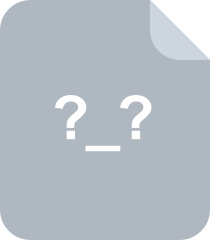
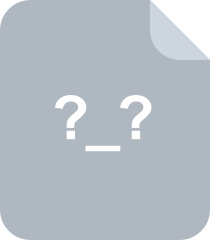
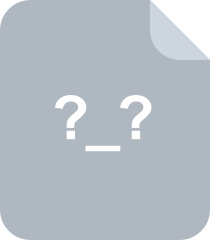
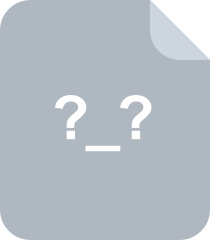
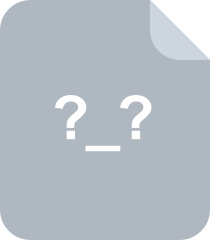
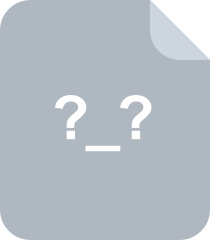
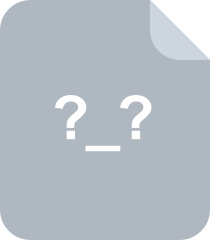
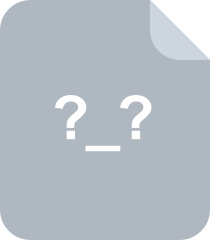
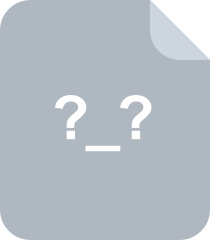
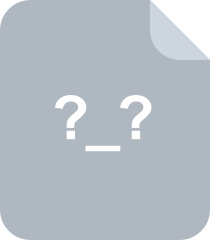
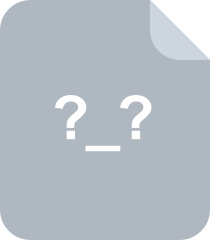
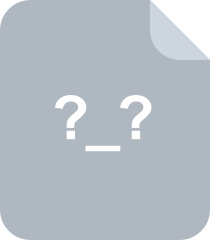
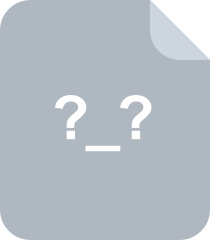
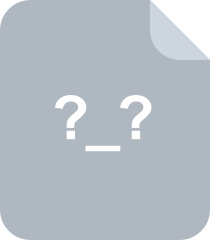
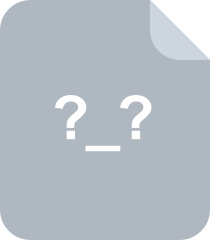
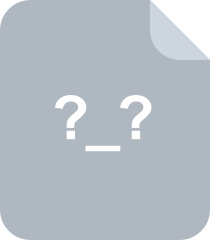
- 1
- 2
- 3
- 4
- 5
- 6
- 20
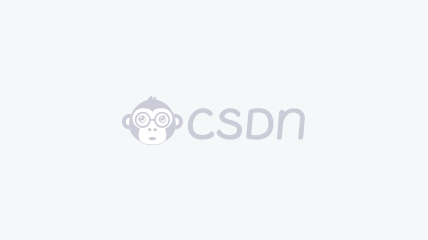
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整
- murphy2013-05-17正好在找0.11版本,谢谢

- 粉丝: 3
- 资源: 4
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

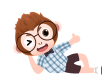
最新资源
- Matlab实现TSOA-CNN-GRU-Mutilhead-Attention凌日优化算法优化卷积门控循环单元融合多头注意力机制多特征分类预测(含模型描述及示例代码)
- Matlab基于LSTM长短期记忆神经网络的锂电池寿命预测(含模型描述及示例代码)
- Matlab实现LSSVM-ABKDE的最小二乘支持向量机结合自适应带宽核密度估计多变量回归区间预测(含模型描述及示例代码)
- 博图7瑞萨RZN2L的联调调试记录分享
- java复习资料复习资料
- Matlab实现KOA-CNN-LSTM-Mutilhead-Attention开普勒算法优化卷积长短期记忆神经网络融合多头注意力机制多变量多步时间序列预测(含模型描述及示例代码)
- Matlab实现EVO-CNN-BiLSTM-Mutilhead-Attention能量谷优化算法优化卷积双向长短期记忆神经网络融合多头注意力机制多变量多步时间序列预测(含模型描述及示例代码)
- 前端开发面试解析-针对前端开发的面试准备、常见面试问题及回答解析进行详细阐述,帮助求职者更好地应对面试
- 基于JavaScript的标题文本轮播系统
- 智慧云桌面系统是一套酒店IPTV管理系统,这是一套没有数据库的源代码,仅供学习参考
- 计算机组成原理:五大核心部件详解及其应用场景
- Matlab实现CNN-LSTM-KDE的卷积长短期神经网络结合核密度估计多变量时序区间预测(含模型描述及示例代码)
- Matlab实现TSOA-CNN-BiGRU-Mutilhead-Attention凌日优化算法优化卷积双向门控循环单元融合多头注意力机制多特征分类预测(含模型描述及示例代码)
- 软件测试项目面试题:核心概念、流程与技巧解析
- Matlab实现TSOA-CNN-LSTM-Mutilhead-Attention凌日优化算法优化卷积长短期记忆神经网络融合多头注意力机制多特征分类预测(含模型描述及示例代码)
- Matlab实现TSOA-CNN-BiLSTM-Mutilhead-Attention凌日优化算法优化卷积双向长短期记忆神经网络融合多头注意力机制多特征分类预测(含模型描述及示例代码)

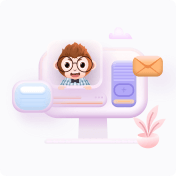
