/*
* Copyright (c) 2001-2017 Stephen Williams (steve@icarus.com)
*
*/
VVP SIMULATION ENGINE
The VVP simulator takes as input source code not unlike assembly
language for a conventional processor. It is intended to be machine
generated code emitted by other tools, including the Icarus Verilog
compiler, so the syntax, though readable, is not necessarily
convenient for humans.
GENERAL FORMAT
The source file is a collection of statements. Each statement may have
a label, an opcode, and operands that depend on the opcode. For some
opcodes, the label is optional (or meaningless) and for others it is
required.
Every statement is terminated by a semicolon. The semicolon is also
the start of a comment line, so you can put comment text after the
semicolon that terminates a statement. Like so:
Label .functor and, 0x5a, x, y ; This is a comment.
The semicolon is required, whether the comment is there or not.
Statements may span multiple lines, as long as there is no text (other
then the first character of a label) in the first column of the
continuation line.
HEADER SYNTAX
Before any other non-commentary code starts, the source may contain
some header statements. These are used for passing parameters or
global details from the compiler to the vvp run-time. In all cases,
the header statement starts with a left-justified keyword.
* :module "name" ;
This header statement names a vpi module that vvp should load before
the rest of the program is compiled. The compiler looks in the
standard VPI_MODULE_PATH for files named "name.vpi", and tries to
dynamic load them.
* :vpi_time_precision [+|-]<value>;
This header statement specifies the time precision of a single tick of
the simulation clock. This is mostly used for display (and VPI)
purposes, because the engine itself does not care about units. The
compiler scales time values ahead of time.
The value is the size of a simulation tick in seconds, and is
expressed as a power of 10. For example, +0 is 1 second, and -9 is 1
nanosecond. If the record is left out, then the precision is taken to
be +0.
LABELS AND SYMBOLS
Labels and symbols consist of the characters:
a-z
A-Z
0-9
.$_<>
Labels and symbols may not start with a digit or a '.', so that they
are easily distinguished from keywords and numbers. A Label is a
symbol that starts a statement. If a label is present in a statement,
it must start in the first text column. This is how the lexical
analyzer distinguishes a label from a symbol. If a symbol is present
in a statement, it is in the operand. Opcodes of statements must be a
keyword.
Symbols are references to labels. It is not necessary for a label to
be declared before its use in a symbol, but it must be declared
eventually. When symbols refer to functors, the symbol represents the
vvp_ipoint_t pointer to the output. (Inputs cannot, and need not, be
references symbolically.)
If the functor is part of a vector, then the symbol is the
vvp_ipoint_t for the first functor. The [] operator can then be used
to reference a functor other than the first in the vector.
There are some special symbols that in certain contexts have special
meanings. As inputs to functors, the symbols "C<0>", "C<1>", "C<x>"
and "C<z>" represent a constant driver of the given value.
NUMBERS:
decimal number tokens are limited to 64bits, and are unsigned. Some
contexts may constrain the number size further.
SCOPE STATEMENTS:
The syntax of a scope statement is:
<label> .scope <type>, <name> <type-name> <file> <lineno> ;
<label> .scope <type>, <name> <type-name> <file> <lineno>, \
<def-file> <def-lineno> <is-cell>, <parent> ;
The <type> is the general type of the scope: module, autofunction,
function, autotask, task, begin, fork, autobegin, autofork, or generate.
The <name> is a string that is the base name of the instance. For
modules, this is the instance name. For tasks and functions, this is
the task or function name.
The <type-name> is the name of the type. For most scope types, this is
the name as the <name>, but for module and class scopes, this is the
name of the definition, and not the instance.
The <file> and <lineno> are the location of the instantiation of this
scope. For a module, it is the location of the instance.
The <def-file> and <def-lineno> is the source file and line number for
the definition of the scope. For modules, this is where the module is
defined instead of where it is instantiated.
The <is-cell> flag is only useful for module instances. It is true
(not zero) if the module is a celltype instead of a regular module.
The short form of the scope statement is only used for root scopes.
PARAMETER STATEMENTS:
Parameters are named constants within a scope. These parameters have a
type and value, and also a label so that they can be referenced as VPI
objects.
The syntax of a parameter is:
<label> .param/str <name>, <value>;
<label> .param/b <name>, <value> [<msb>,<lsb>];
<label> .param/l <name>, <value> [<msb>,<lsb>];
<label> .param/r <name>, <value>;
The <name> is a string that names the parameter. The name is placed in
the current scope as a vpiParameter object. The .param suffix
specifies the parameter type.
.param/str -- The parameter has a string value
.param/l -- The parameter has a logic vector value
.param/b -- The parameter has a boolean vector value
.param/r -- The parameter has a real value
The value, then, is appropriate for the data type. For example:
P_123 .param/str "hello", "Hello, World.";
The boolean and logic values can also be signed or not. If signed, the
value is preceded by a '+' character. (Note that the value is 2s
complement, so the '+' says only that it is signed, not positive.)
FUNCTOR STATEMENTS:
A functor statement is a statement that uses the ``.functor''
opcode. Functors are the basic structural units of a simulation, and
include a type (in the form of a truth table) and up to four inputs. A
label is required for functors.
The general syntax of a functor is:
<label> .functor <type>, symbol_list ;
<label> .functor <type> [<drive0> <drive1>], symbol_list ;
The symbol list is 4 names of labels of other functors. These connect
inputs of the functor of the statement to the output of other
functors. If the input is unconnected, use a C<?> symbol instead. The
type selects the truth lookup table to use for the functor
implementation. Most of the core gate types have built in tables.
The initial values of all the inputs and the output is x. Any other
value is passed around as run-time behavior. If the inputs have C<?>
symbols, then the inputs are initialized to the specified bit value,
and if this causes the output to be something other than x, a
propagation event is created to be executed at the start of run time.
The strengths of inputs are ignored by functors, and the output has
fixed drive0 and drive1 strengths. So strength information is
typically lost as it passes through functors.
Almost all of the structural aspects of a simulation can be
represented by functors, which perform the very basic task of
combining up to four inputs down to one output.
- MUXZ
Q | A B S n/a
--+-------------
A | * * 0
B | * * 1
DFF AND LATCH STATEMENTS:
The Verilog language itself does not have a DFF primitive, but post
synthesis readily creates DFF devices that are best simulated with a
common device. Thus, there is the DFF statement to create DFF devices:
<label> .dff/p <width> <d>, <clk>, <ce>;
<label> .dff/n <width> <d>, <clk>, <ce>;
<label> .dff/p/aclr <width> <d>, <clk>, <ce>, <async-input>;
<label> .dff/n/aclr <width> <d>, <clk>, <ce>, <async-input>;
<label> .dff/p/aset <width> <d>, <clk>, <ce>, <async-input>[, <set-value>];
<label> .dff/n/aset <width> <d>, <clk>, <ce>, <async-input>[, <set-value>];
The /p variants simulate positive-edge triggered flip-flops and the
/n variants simulate negative-edge triggered flip-flops. The generated
fu
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
项目工程资源经过严格测试可直接运行成功且功能正常的情况才上传,可轻松复刻,拿到资料包后可轻松复现出一样的项目,本人系统开发经验充足(全领域),有任何使用问题欢迎随时与我联系,我会及时为您解惑,提供帮助。 【资源内容】:包含完整源码+工程文件+说明(如有)等。答辩评审平均分达到96分,放心下载使用!可轻松复现,设计报告也可借鉴此项目,该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的。 【提供帮助】:有任何使用问题欢迎随时与我联系,我会及时解答解惑,提供帮助 【附带帮助】:若还需要相关开发工具、学习资料等,我会提供帮助,提供资料,鼓励学习进步 【项目价值】:可用在相关项目设计中,皆可应用在项目、毕业设计、课程设计、期末/期中/大作业、工程实训、大创等学科竞赛比赛、初期项目立项、学习/练手等方面,可借鉴此优质项目实现复刻,设计报告也可借鉴此项目,也可基于此项目来扩展开发出更多功能 下载后请首先打开README文件(如有),项目工程可直接复现复刻,如果基础还行,也可在此程序基础上进行修改,以实现其它功能。供开源学习/技术交流/学习参考,勿用于商业用途。质量优质,放心下载使用。
资源推荐
资源详情
资源评论




























收起资源包目录

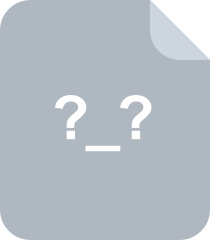
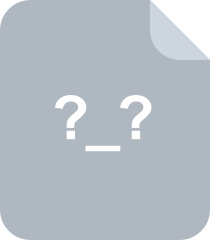
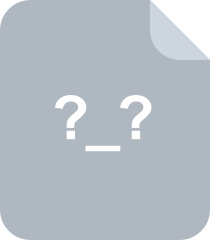
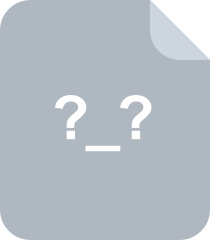
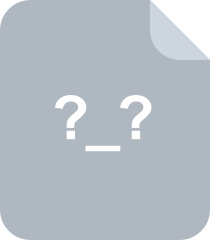
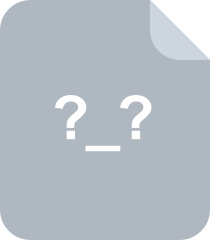
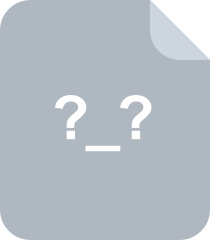
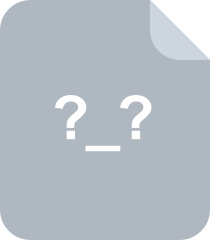
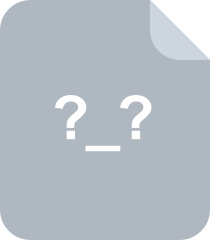
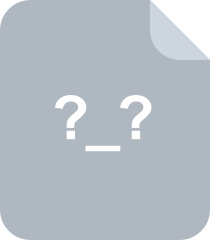
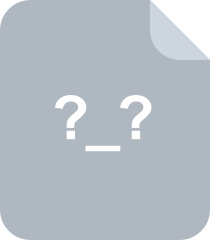
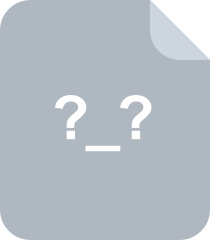
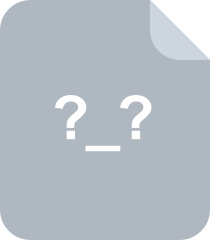
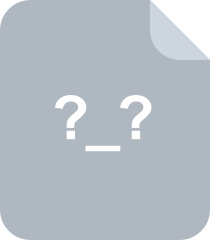
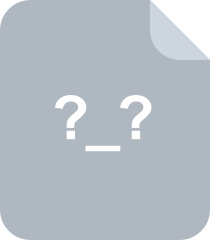
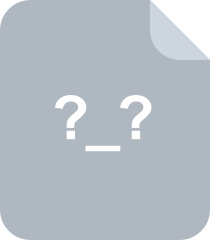
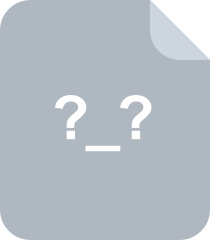
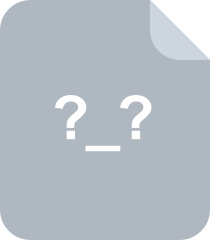
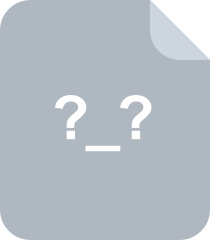
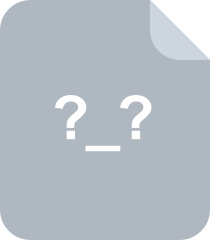
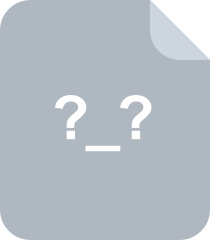
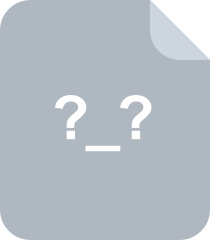
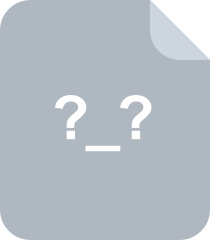
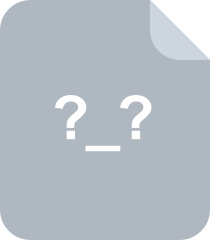
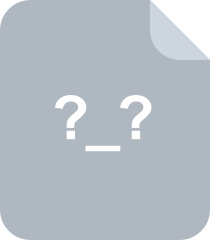
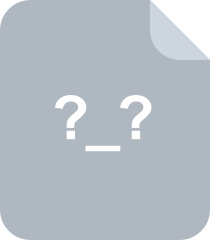
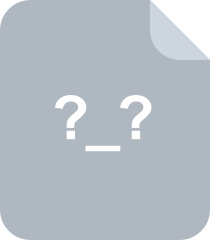
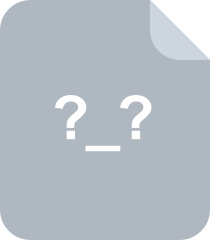
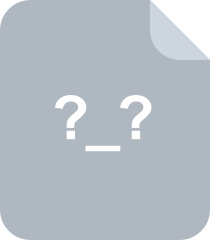
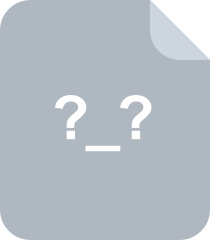
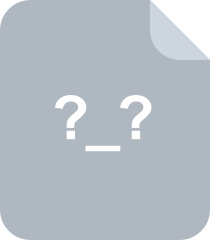
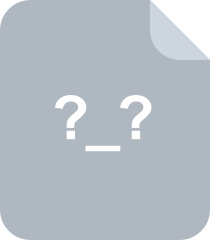
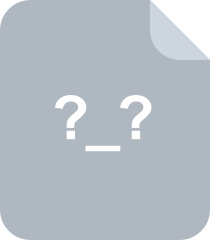
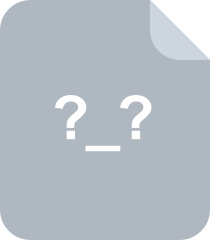
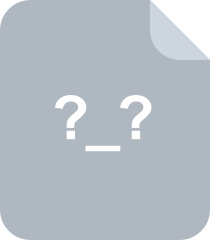
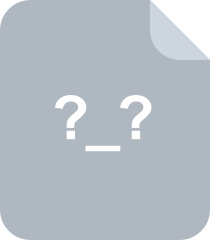
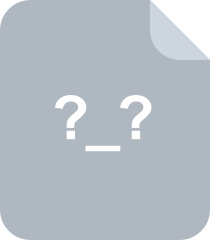
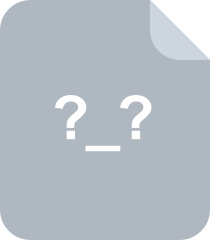
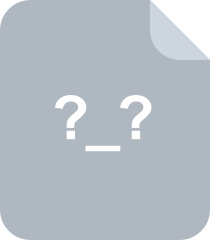
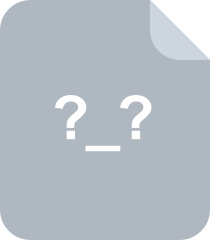
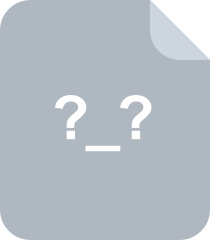
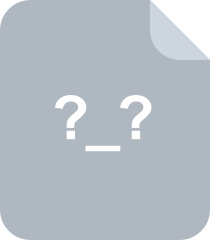
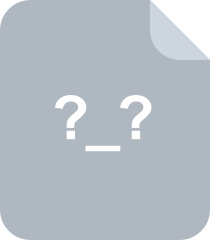
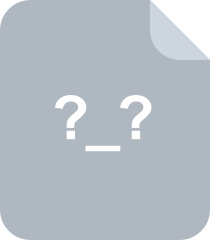
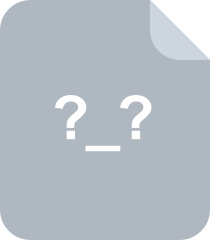
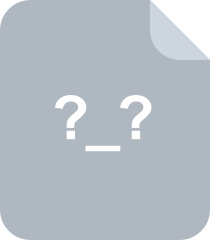
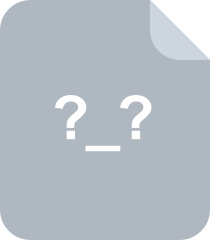
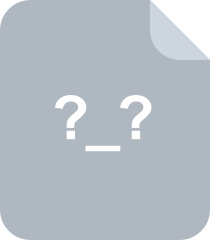
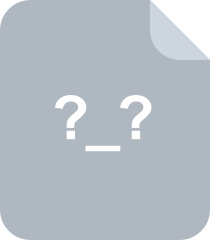
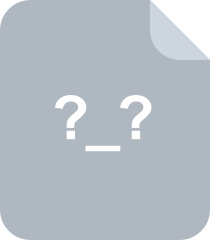
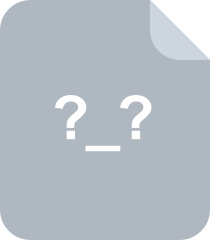
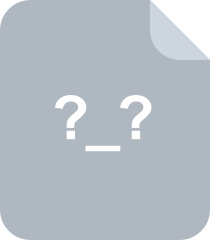
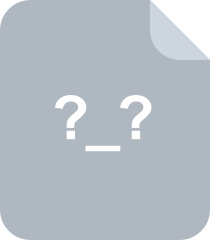
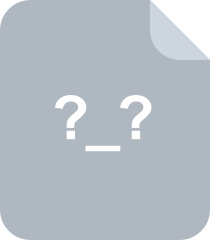
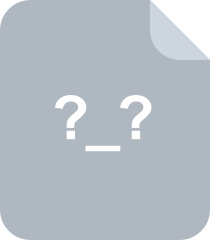
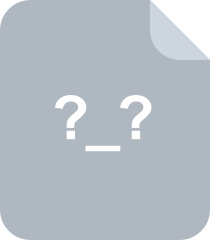
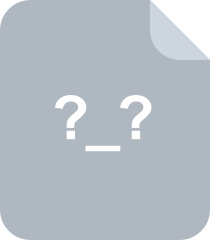
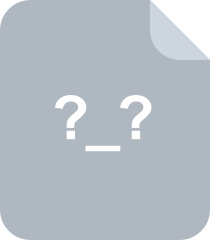
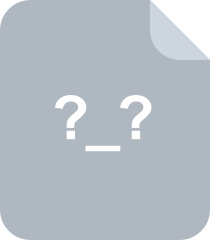
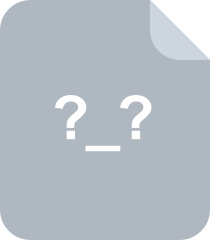
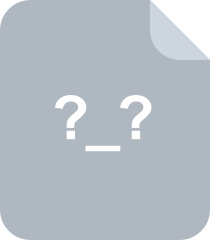
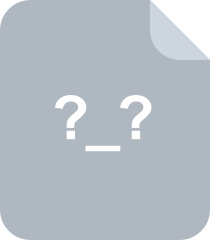
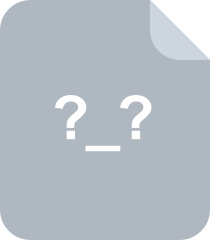
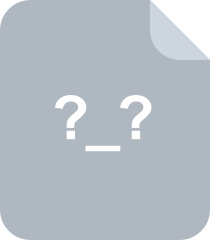
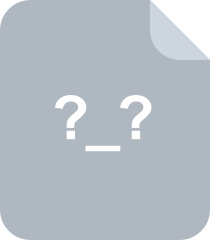
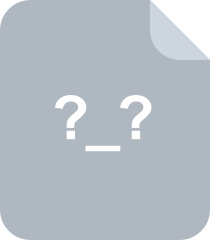
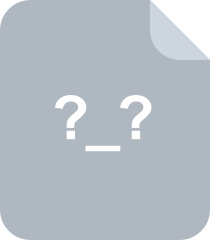
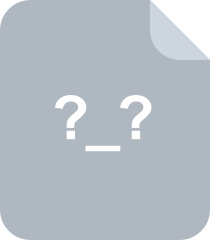
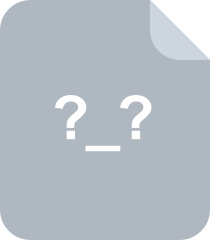
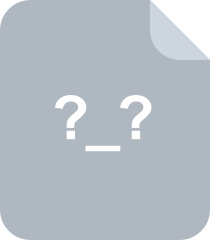
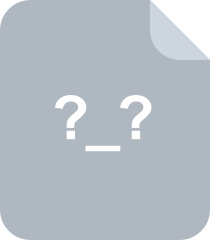
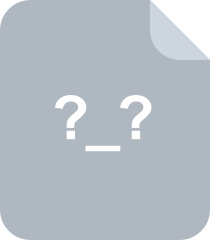
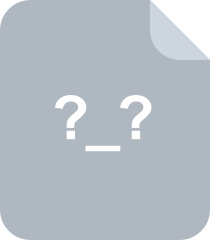
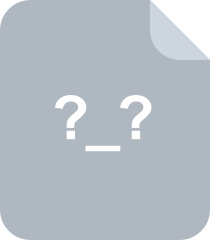
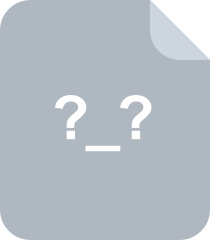
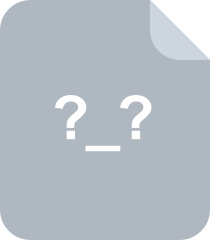
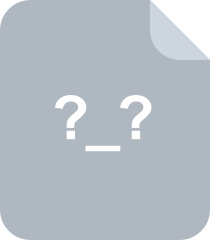
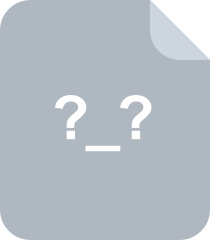
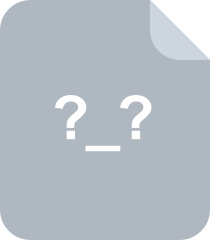
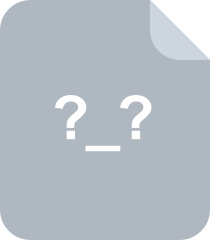
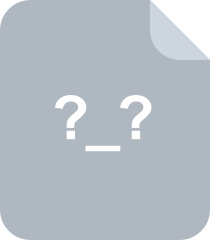
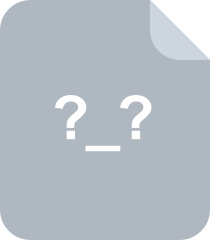
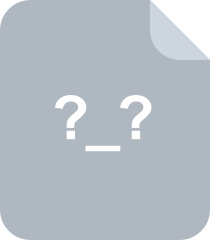
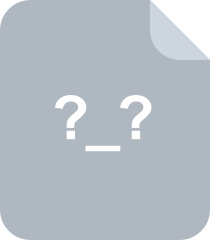
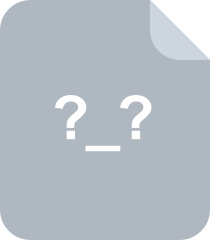
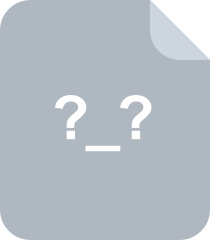
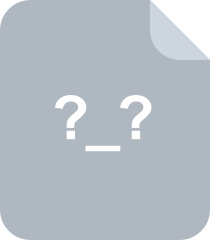
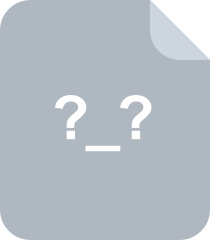
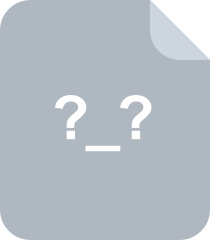
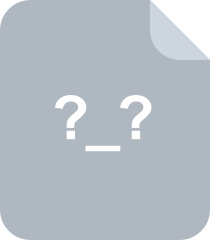
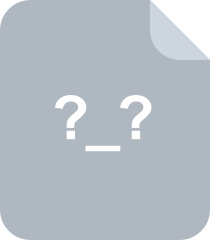
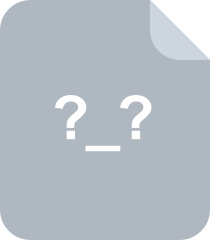
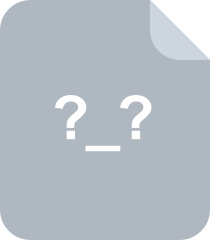
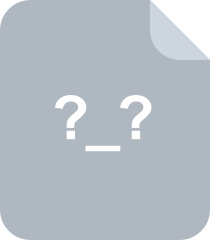
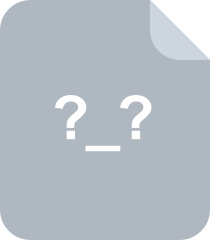
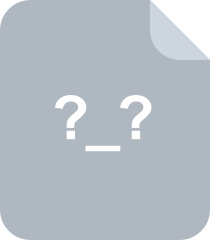
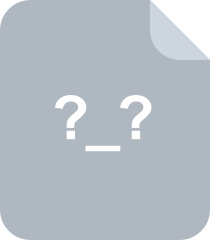
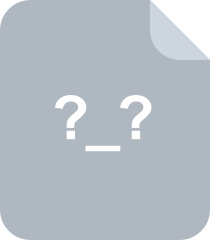
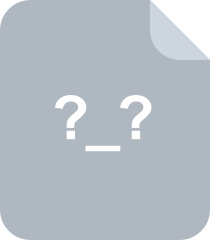
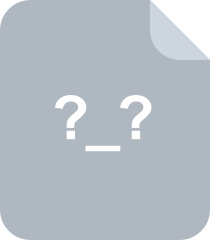
共 855 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9
资源评论
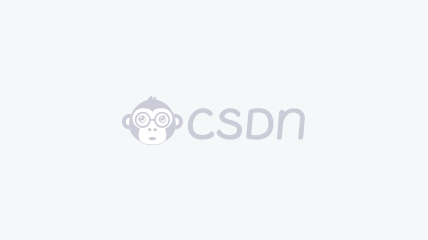

热爱技术。
- 粉丝: 3090
- 资源: 7985
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

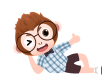
最新资源
- java项目,毕业设计(包含源代码)-基于vue的地方美食分享网站
- DeepSeek+15天指导手册-从入门到精通-热乎版.pdf DeepSeek+15天指导手册-从入门到精通-热乎版.pdf DeepSeek+15天指导手册-从入门到精通-热乎版.pdf
- dify-main文件
- Nginx源码分析与导读 ppt
- java项目,毕业设计(包含源代码)-基于web的智慧养老平台
- 清华大学第二弹:DeepSeek赋能职场.pdf 从提示语技巧到多场景应用的人工智能解决方案
- DeepSeek+15天指导手册-AI应用从入门到精通涵盖基础知识及多场景实战
- TSP问题求解:蚁群算法与遗传算法优化 + 2-opt局部搜索(含MATLAB代码及节点数据)
- java项目,毕业设计(包含源代码)-一起来约苗系统
- 淮北市乡镇边界,shp格式
- 邻家小厨网上订餐系统asp.net源码
- lua程序设计 入门到精通 资料
- 铜陵市乡镇边界,shp格式
- “健康早知道”微信小程序.zip
- 基于微信小程序的新生报到系统.zip
- “最多跑一次”微信小程序.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


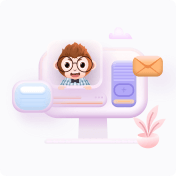
安全验证
文档复制为VIP权益,开通VIP直接复制
