# Roundabout
**Note: Roundabout is no longer under active development. I've moved the documentation over here to support the exists plugin, but there are known issues and such that won't be fixed. Use at your own risk.**
Roundabout is a jQuery plugin that easily converts unordered lists & other nested HTML structures into entertaining, interactive, turntable-like areas.
It’s ready-to-go straight out of the box, but if you want to get crazy, Roundabout is highly-customizable with an extensive API that allows for some pretty amazing results.
Roundabout requires jQuery (at least version 1.2).
## Add-Ons
Roundabout is equipped to play nicely with a couple of other plugins if they’re made available.
- [Roundabout Shapes](http://fredhq.com/projects/roundabout-shapes) by Fred LeBlanc
Roundabout can move in more ways than just a turntable. With Shapes, you have many other ways you can move your content around the page.
- [jQuery Easing](http://gsgd.co.uk/sandbox/jquery/easing/) by George McGinley Smith
jQuery comes with two easing styles built in, but this plugin adds many, many more. Include this script and use any of its defined easing functions in your Roundabout animations.
- [Event.drag](http://threedubmedia.com/code/event/drag) & [Event.drop](http://threedubmedia.com/code/event/drop) by ThreeDubMedia
In addition to rotating on click, Roundabout can also move by clicking and dragging on the Roundabout element itself. Include these scripts and turn on enableDrag.
### But That’s Not All!
The list above is only a list of the plugins that have support baked in, but Roundabout will play nicely with many other plugins. (It’s up to you to integrate those yourself.)
## Using Roundabout
Include the `jquery.roundabout.js` JavaScript file on your page after you include jQuery itself. Also, either link to the included CSS file, or copy the CSS styles from that file and paste them into your site's CSS file.
To activate Roundabout in its simplest form, you can do this:
```javascript
$('ul').roundabout();
```
Of course, this will change *all* of your `ul` elements into Roundabouts, which probably isn't what you want, but you can easily change the selector to only target the elements you wish to convert
### Setting Options
You can set options on Roundabout to change how it behaves. Do this by passing in an object of options into the main Roundabout call upon initialization, like so:
```javascript
$('ul').roundabout({
btnNext: ".next"
});
```
### Incorporating Roundabout Shapes
If you're using the sister plugin Roundabout Shapes, be sure to include the `jquery.roundabout-shapes.js` file after you include the main Roundabout JavaScript file. Next, you'll select the shape as on of the options that you pass into Roundabout upon initialization:
```javascript
$('ul').roundabout({
btnNext: ".next",
shape: "figure8"
});
```
### Calling Roundabout Methods
Roundabout comes with a number of methods you can call to better control how it works. Calling those methods are done by re-calling `roundabout` on the element that Roundabout is already working on and passing in the name of the method to use as the first parameter. If the method requires other parameters, pass those in as subsequent parameters.
For example, instead of using the `btnNext` option, you can manually implement this yourself like this:
```javascript
$('.btnNext', function(e) {
e.stopPropagation();
e.preventDefault();
// this is the action
$('ul#myRoundabout').roundabout('animateToNextChild');
return false;
});
```
### Enabling Drag & Drop
Lately it seems that this doesn't work as well as it once did. Not sure why, but you can still enable it. To do this, you'll need to grab v2 of the `jquery.event.drag` and `jquery.event.drop` plugins by ThreeDubMedia. Include them on your page after Roundabout is included.
Next, enable your Roundabout to use drag and drop like so:
```javascript
$('ul').roundabout({
enableDrag: true
});
```
### Using Autoplay
Autoplay lets you have Roundabout automatically animate on an interval. This functionality is included with the Roundabout core, so no additional scripts are needed to get this working.
To enable autoplay, there are three options you can set:
```javascript
$('ul').roundabout({
autoplay: true,
autoplayDuration: 5000,
autoplayPauseOnHover: true
});
```
The first option, `autoplay` will turn on autoplay. The second, `autoplayDuration` is the length of time in milliseconds between animation triggers. The final option, `autoplayPauseOnHover` will force autoplay never to figure while the user has their cursor over the Roundabout itself.
## Support
Version 2 (the current version) works reasonably well, although if you look in the issues you'll see a number of things that don't work for some people. As mentioned above, this plugin is no longer under active development. Feel free to continue submitting issues for others to see, but no further official action can be guaranteed at all.
## API
### Settable Options
Roundabout comes with many settable configuration options that let you customize how it operates.
<table>
<thead>
<tr>
<th scope="col">Option</th>
<th scope="col">Description</th>
<th scope="col">Data Type</th>
<th scope="col" class="default-value">Default</th>
</tr>
</thead>
<tbody>
<tr id="bearing">
<td class="option"><code>bearing</code></td>
<td>
The starting direction in which Roundabout should
face relative to the <code>focusBearing</code>.
</td>
<td class="data-type">float</td>
<td class="default-value"><code>0.0</code></td>
</tr>
<tr id="tilt">
<td class="option"><code>tilt</code></td>
<td>
Slightly alters the calculations of moving elements.
In the default <code>shape</code>,
it adjusts the apparent <code>tilt</code>. Other shapes
will differ.
</td>
<td class="data-type">float</td>
<td class="default-value"><code>0.0</code></td>
</tr>
<tr id="minZ">
<td class="option"><code>minZ</code></td>
<td>
The lowest z-index that will be assigned to a moving
element. This occurs when the moving element is
opposite of (that is, 180° away from) the
<code>focusBearing</code>.
</td>
<td class="data-type">integer</td>
<td class="default-value"><code>100</code></td>
</tr>
<tr id="maxZ">
<td class="option"><code>maxZ</code></td>
<td>
The greatest z-index that will be assigned to a
moving element. This occurs when the moving element
is at the same bearing as the
<code>focusBearing</code>.
</td>
<td class="data-type">integer</td>
<td class="default-value"><code>280</code></td>
</tr>
<tr id="minOpacity">
<td class="option"><code>minOpacity</code></td>
<td>
The lowest opacity that will be assigned to a moving
element. This occurs when the moving element is
opposite of (that is, 180° away
from) the <code>focusBearing</code>.
</td>
<td class="data-type">float</td>
<td class="default-value"><code>0.4</code></td>
</tr>
<tr id="maxOpacity">
<td class="option"><code>maxOpacity</code></td>
<td>
The greatest opacity that will be assigned to a
moving element. This occurs when the moving element
is at the same bearing as the
<code>focusBearing</code>.
</td>
<td class="data-type">float</td>
<td class="default-value"><code>1.0</code></td>
</tr>
<tr id="minScale">
<td class="option"><code>minScale</code></td>
<td>
The lowest size (relative to its starting size) that
will be assigned to a moving element. This occurs
when the moving element is opposite of (that is, 180°
away from) the
<code>focusBearing</code>.
</td>
<td class="data-type">float</td>
<td class="default-value"><code>0.4</code></td>
</tr>
<tr id="maxScale">
<td class="option"><code>maxScale</code></td>
<td>
The greatest size (relative to
没有合适的资源?快使用搜索试试~ 我知道了~
工程项目管理系统,基于 ThinkPHP 3.2.3,工程项目管理、评分和考勤管理.zip
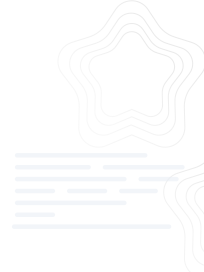
共2000个文件
html:801个
php:315个
css:296个

0 下载量 44 浏览量
2024-08-25
12:45:14
上传
评论
收藏 10.82MB ZIP 举报
温馨提示
项目工程资源经过严格测试可直接运行成功且功能正常的情况才上传,可轻松copy复刻,拿到资料包后可轻松复现出一样的项目,本人系统开发经验充足(全栈开发),有任何使用问题欢迎随时与我联系,我会及时为您解惑,提供帮助 【资源内容】:项目具体内容可查看/点击本页面下方的*资源详情*,包含完整源码+工程文件+说明(若有)等。【若无VIP,此资源可私信获取】 【本人专注IT领域】:有任何使用问题欢迎随时与我联系,我会及时解答,第一时间为您提供帮助 【附带帮助】:若还需要相关开发工具、学习资料等,我会提供帮助,提供资料,鼓励学习进步 【适合场景】:相关项目设计中,皆可应用在项目开发、毕业设计、课程设计、期末/期中/大作业、工程实训、大创等学科竞赛比赛、初期项目立项、学习/练手等方面中 可借鉴此优质项目实现复刻,也可基于此项目来扩展开发出更多功能 #注 1. 本资源仅用于开源学习和技术交流。不可商用等,一切后果由使用者承担 2. 部分字体及插图等来自网络,若是侵权请联系删除,本人不对所涉及的版权问题或内容负法律责任。收取的费用仅用于整理和收集资料耗费时间的酬劳 3. 积分资源不提供使用问题指导/解答
资源推荐
资源详情
资源评论
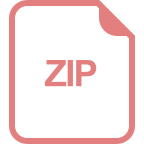
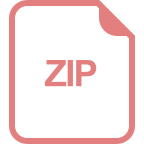
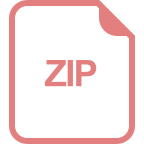
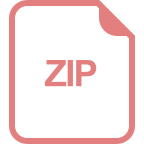
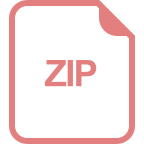
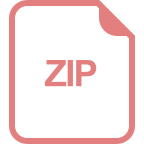
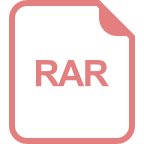
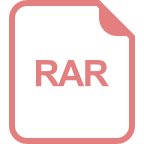
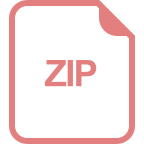
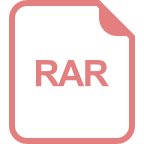
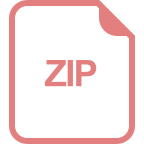
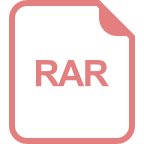
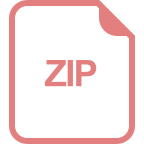
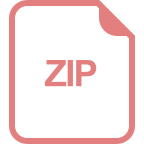
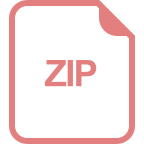
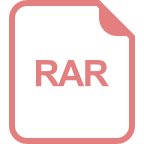
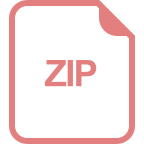
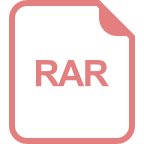
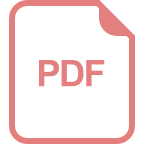
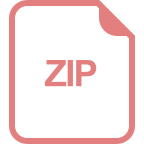
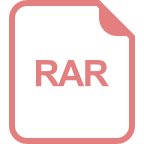
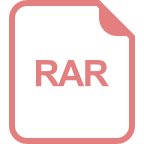
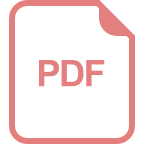
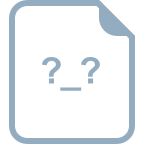
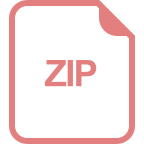
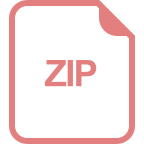
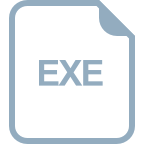
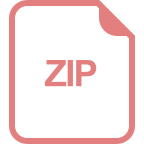
收起资源包目录

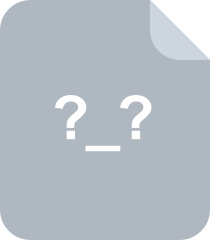
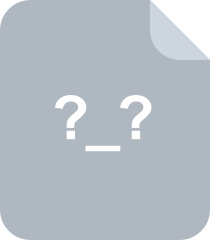
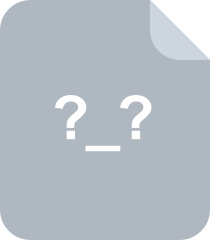
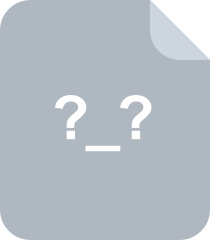
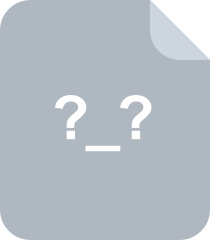
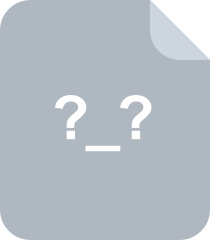
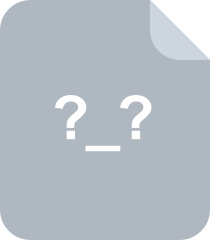
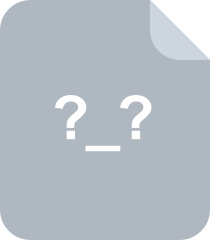
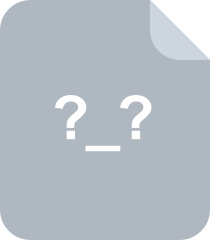
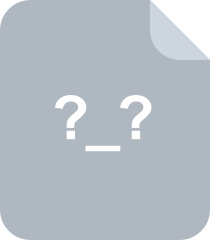
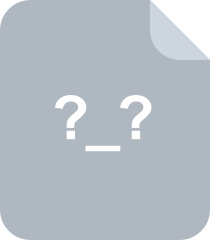
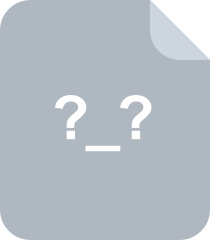
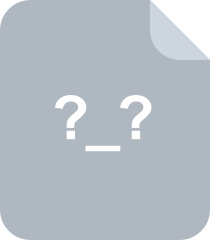
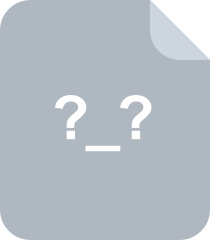
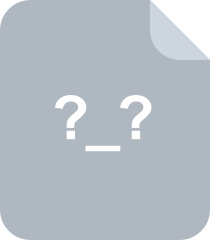
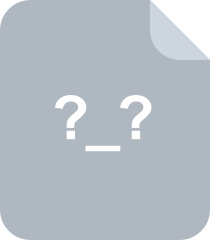
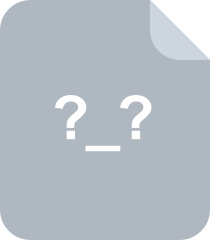
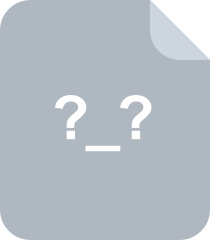
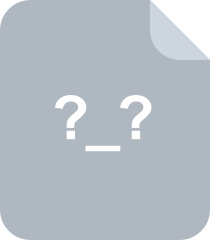
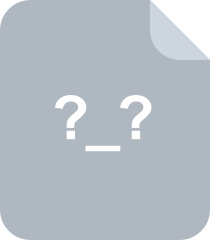
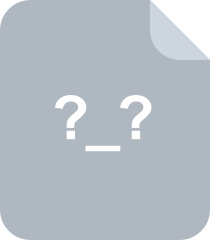
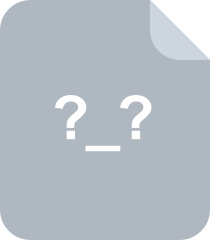
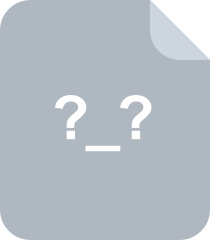
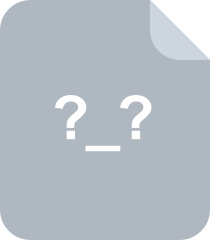
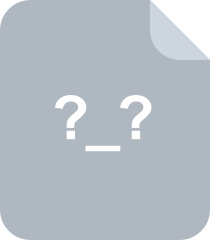
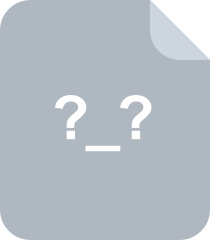
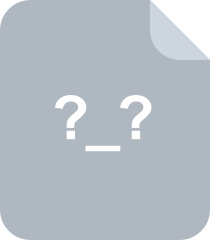
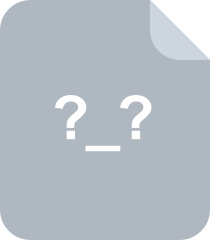
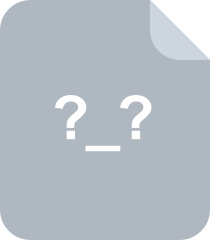
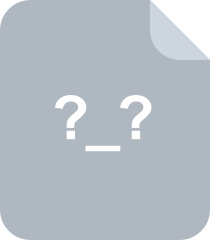
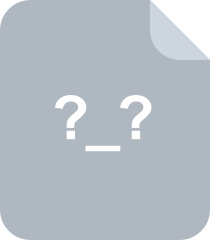
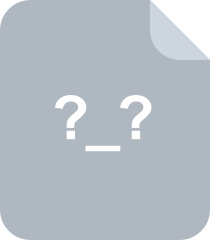
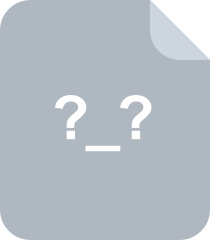
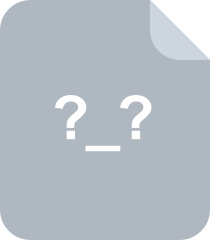
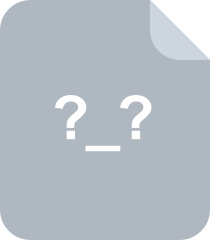
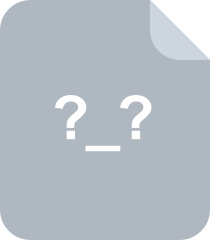
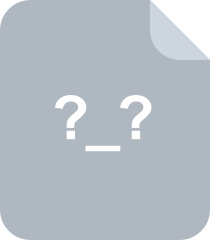
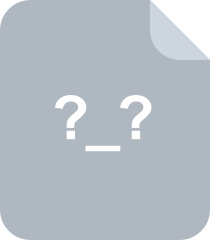
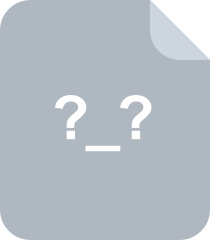
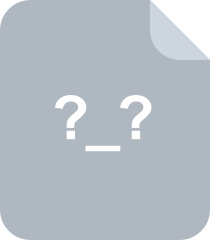
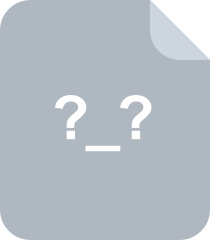
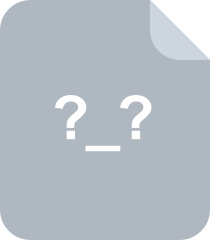
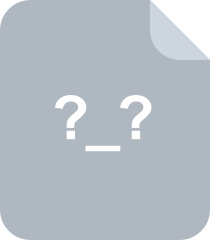
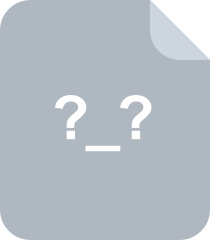
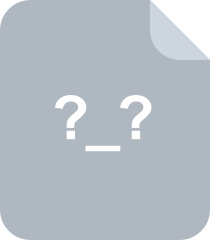
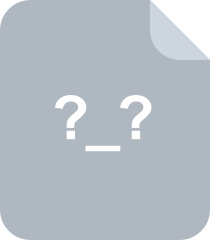
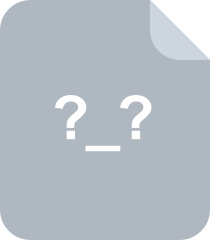
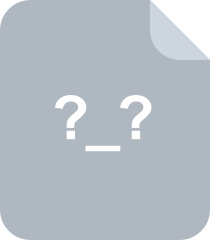
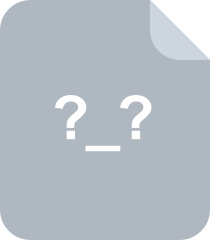
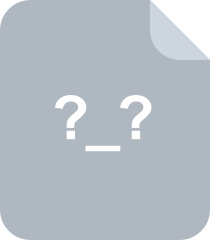
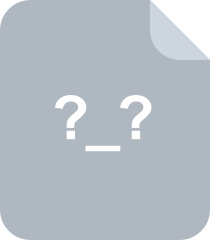
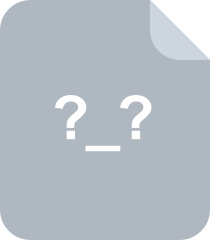
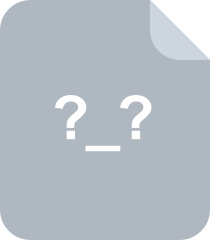
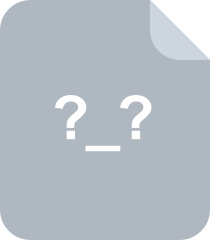
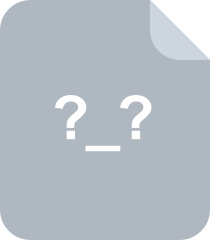
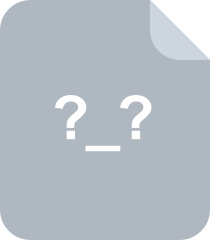
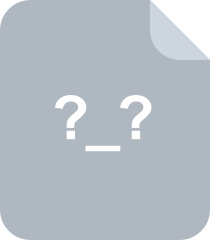
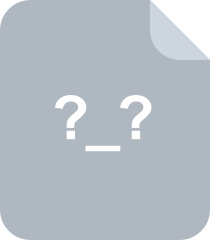
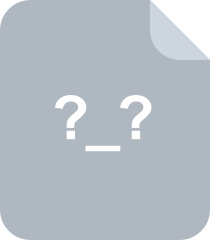
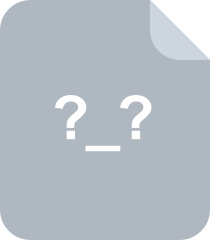
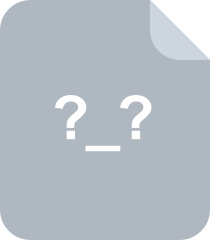
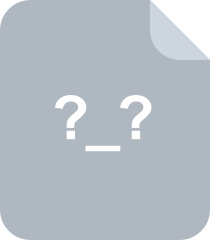
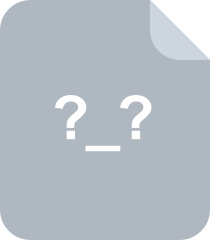
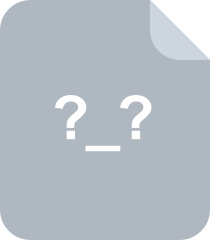
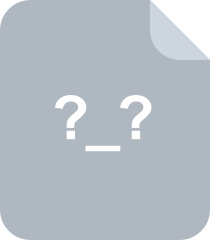
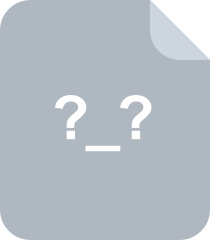
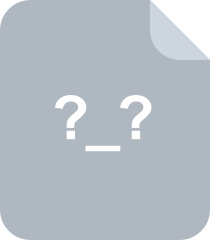
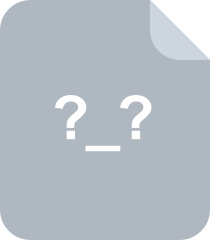
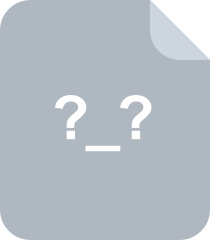
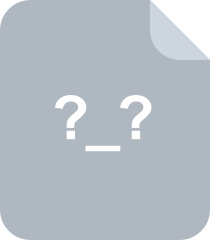
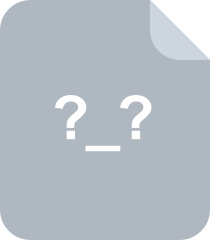
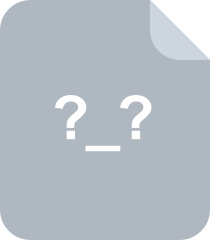
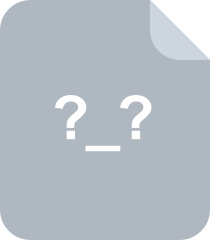
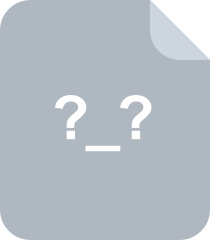
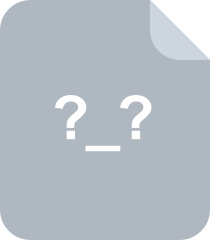
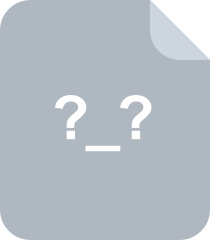
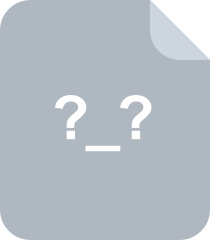
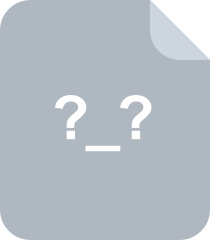
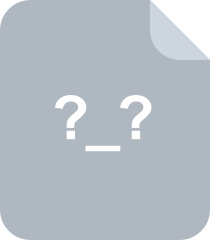
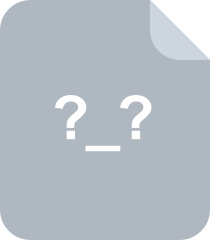
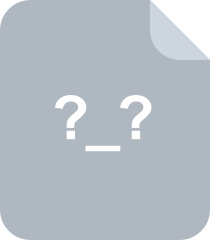
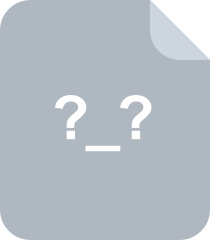
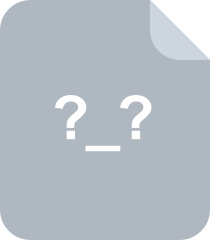
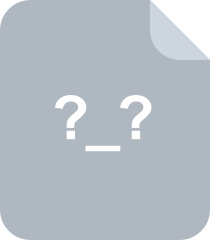
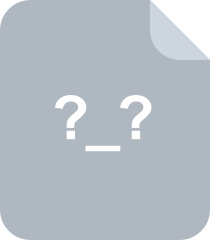
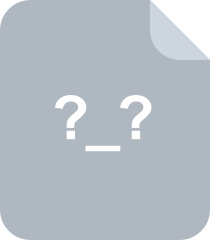
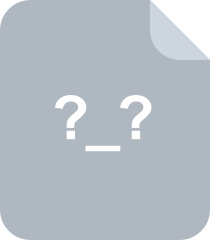
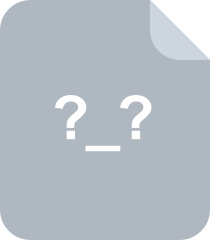
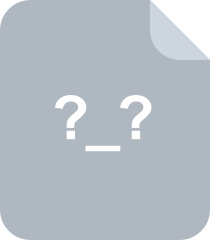
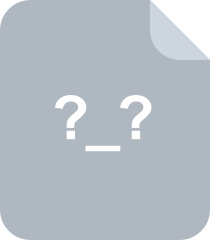
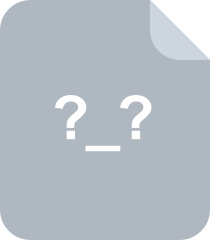
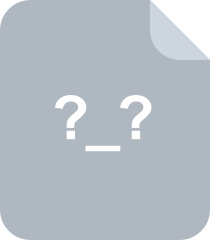
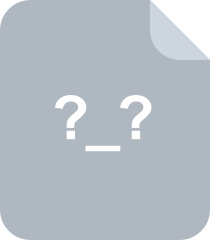
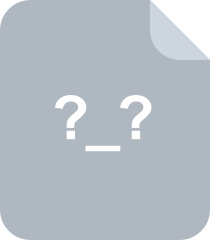
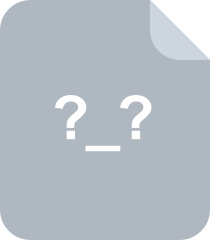
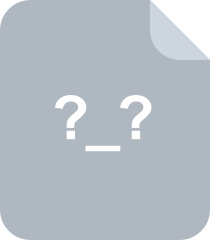
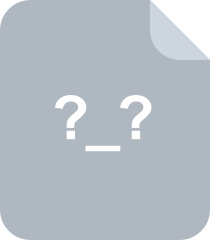
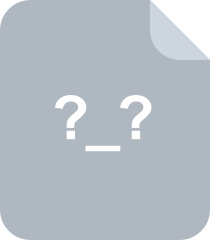
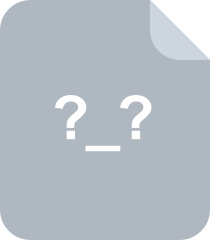
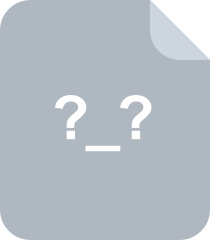
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
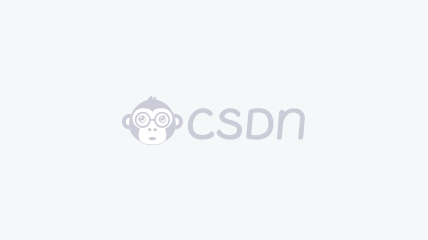

热爱技术。
- 粉丝: 2810
- 资源: 7860
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

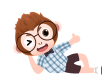
最新资源
- 光储并网VSG系统Matlab simulink仿真模型,附参考文献 系统前级直流部分包括光伏阵列、变器、储能系统和双向dcdc变器,后级交流子系统包括逆变器LC滤波器,交流负载 光储并网VSG系
- file_241223_024438_84523.pdf
- 质子交膜燃料电池PEMFC Matlab simulink滑模控制模型,过氧比控制,温度控制,阴,阳极气压控制
- IMG20241223015444.jpg
- 模块化多电平变器(MMC),本模型为三相MMC整流器 控制策略:双闭环控制、桥臂电压均衡控制、模块电压均衡控制、环流抑制控制策略、载波移相调制,可供参考学习使用,默认发2020b版本及以上
- Delphi 12 控件之FlashAV FFMPEG VCL Player For Delphi v7.0 for D10-D11 Full Source.7z
- Delphi 12 控件之DevExpressVCLProducts-24.2.3.exe.zip
- Mysql配置文件优化内容 my.cnf
- 中国地级市CO2排放数据(2000-2023年).zip
- smart200光栅报警程序
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


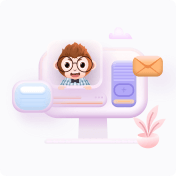
安全验证
文档复制为VIP权益,开通VIP直接复制
