#if USE_UNI_LUA
using LuaAPI = UniLua.Lua;
using RealStatePtr = UniLua.ILuaState;
using LuaCSFunction = UniLua.CSharpFunctionDelegate;
#else
using LuaAPI = XLua.LuaDLL.Lua;
using RealStatePtr = System.IntPtr;
using LuaCSFunction = XLua.LuaDLL.lua_CSFunction;
#endif
using XLua;
using System.Collections.Generic;
<%ForEachCsList(namespaces, function(namespace)%>using <%=namespace%>;<%end)%>
<%
require "TemplateCommon"
local OpNameMap = {
op_Addition = "__AddMeta",
op_Subtraction = "__SubMeta",
op_Multiply = "__MulMeta",
op_Division = "__DivMeta",
op_Equality = "__EqMeta",
op_UnaryNegation = "__UnmMeta",
op_LessThan = "__LTMeta",
op_LessThanOrEqual = "__LEMeta",
op_Modulus = "__ModMeta",
op_BitwiseAnd = "__BandMeta",
op_BitwiseOr = "__BorMeta",
op_ExclusiveOr = "__BxorMeta",
op_OnesComplement = "__BnotMeta",
op_LeftShift = "__ShlMeta",
op_RightShift = "__ShrMeta",
}
local OpCallNameMap = {
op_Addition = "+",
op_Subtraction = "-",
op_Multiply = "*",
op_Division = "/",
op_Equality = "==",
op_UnaryNegation = "-",
op_LessThan = "<",
op_LessThanOrEqual = "<=",
op_Modulus = "%",
op_BitwiseAnd = "&",
op_BitwiseOr = "|",
op_ExclusiveOr = "^",
op_OnesComplement = "~",
op_LeftShift = "<<",
op_RightShift = ">>",
}
local obj_method_count = 0
local obj_getter_count = 0
local obj_setter_count = 0
local meta_func_count = operators.Count
local cls_field_count = 1
local cls_getter_count = 0
local cls_setter_count = 0
ForEachCsList(methods, function(method)
if method.IsStatic then
cls_field_count = cls_field_count + 1
else
obj_method_count = obj_method_count + 1
end
end)
ForEachCsList(events, function(event)
if event.IsStatic then
cls_field_count = cls_field_count + 1
else
obj_method_count = obj_method_count + 1
end
end)
ForEachCsList(getters, function(getter)
if getter.IsStatic then
if getter.ReadOnly then
cls_field_count = cls_field_count + 1
else
cls_getter_count = cls_getter_count + 1
end
else
obj_getter_count = obj_getter_count + 1
end
end)
ForEachCsList(setters, function(setter)
if setter.IsStatic then
cls_setter_count = cls_setter_count + 1
else
obj_setter_count = obj_setter_count + 1
end
end)
ForEachCsList(lazymembers, function(lazymember)
if lazymember.IsStatic == 'true' then
if 'CLS_IDX' == lazymember.Index then
cls_field_count = cls_field_count + 1
elseif 'CLS_GETTER_IDX' == lazymember.Index then
cls_getter_count = cls_getter_count + 1
elseif 'CLS_SETTER_IDX' == lazymember.Index then
cls_setter_count = cls_setter_count + 1
end
else
if 'METHOD_IDX' == lazymember.Index then
obj_method_count = obj_method_count + 1
elseif 'GETTER_IDX' == lazymember.Index then
obj_getter_count = obj_getter_count + 1
elseif 'SETTER_IDX' == lazymember.Index then
obj_setter_count = obj_setter_count + 1
end
end
end)
local generic_arg_list, type_constraints = GenericArgumentList(type)
%>
namespace XLua.CSObjectWrap
{
using Utils = XLua.Utils;
public class <%=CSVariableName(type)%>Wrap<%=generic_arg_list%> <%=type_constraints%>
{
public static void __Register(RealStatePtr L)
{
ObjectTranslator translator = ObjectTranslatorPool.Instance.Find(L);
System.Type type = typeof(<%=CsFullTypeName(type)%>);
Utils.BeginObjectRegister(type, L, translator, <%=meta_func_count%>, <%=obj_method_count%>, <%=obj_getter_count%>, <%=obj_setter_count%>);
<%ForEachCsList(operators, function(operator)%>Utils.RegisterFunc(L, Utils.OBJ_META_IDX, "<%=(OpNameMap[operator.Name]):gsub('Meta', ''):lower()%>", <%=OpNameMap[operator.Name]%>);
<%end)%>
<%ForEachCsList(methods, function(method) if not method.IsStatic then %>Utils.RegisterFunc(L, Utils.METHOD_IDX, "<%=method.Name%>", _m_<%=method.Name%>);
<% end end)%>
<%ForEachCsList(events, function(event) if not event.IsStatic then %>Utils.RegisterFunc(L, Utils.METHOD_IDX, "<%=event.Name%>", _e_<%=event.Name%>);
<% end end)%>
<%ForEachCsList(getters, function(getter) if not getter.IsStatic then %>Utils.RegisterFunc(L, Utils.GETTER_IDX, "<%=getter.Name%>", _g_get_<%=getter.Name%>);
<%end end)%>
<%ForEachCsList(setters, function(setter) if not setter.IsStatic then %>Utils.RegisterFunc(L, Utils.SETTER_IDX, "<%=setter.Name%>", _s_set_<%=setter.Name%>);
<%end end)%>
<%ForEachCsList(lazymembers, function(lazymember) if lazymember.IsStatic == 'false' then %>Utils.RegisterLazyFunc(L, Utils.<%=lazymember.Index%>, "<%=lazymember.Name%>", type, <%=lazymember.MemberType%>, <%=lazymember.IsStatic%>);
<%end end)%>
Utils.EndObjectRegister(type, L, translator, <% if type.IsArray or ((indexers.Count or 0) > 0) then %>__CSIndexer<%else%>null<%end%>, <%if type.IsArray or ((newindexers.Count or 0) > 0) then%>__NewIndexer<%else%>null<%end%>,
null, null, null);
Utils.BeginClassRegister(type, L, __CreateInstance, <%=cls_field_count%>, <%=cls_getter_count%>, <%=cls_setter_count%>);
<%ForEachCsList(methods, function(method) if method.IsStatic then %>Utils.RegisterFunc(L, Utils.CLS_IDX, "<%=method.Overloads[0].Name%>", _m_<%=method.Name%>);
<% end end)%>
<%ForEachCsList(events, function(event) if event.IsStatic then %>Utils.RegisterFunc(L, Utils.CLS_IDX, "<%=event.Name%>", _e_<%=event.Name%>);
<% end end)%>
<%ForEachCsList(getters, function(getter) if getter.IsStatic and getter.ReadOnly then %>Utils.RegisterObject(L, translator, Utils.CLS_IDX, "<%=getter.Name%>", <%=CsFullTypeName(type).."."..getter.Name%>);
<%end end)%>
<%ForEachCsList(getters, function(getter) if getter.IsStatic and (not getter.ReadOnly) then %>Utils.RegisterFunc(L, Utils.CLS_GETTER_IDX, "<%=getter.Name%>", _g_get_<%=getter.Name%>);
<%end end)%>
<%ForEachCsList(setters, function(setter) if setter.IsStatic then %>Utils.RegisterFunc(L, Utils.CLS_SETTER_IDX, "<%=setter.Name%>", _s_set_<%=setter.Name%>);
<%end end)%>
<%ForEachCsList(lazymembers, function(lazymember) if lazymember.IsStatic == 'true' then %>Utils.RegisterLazyFunc(L, Utils.<%=lazymember.Index%>, "<%=lazymember.Name%>", type, <%=lazymember.MemberType%>, <%=lazymember.IsStatic%>);
<%end end)%>
Utils.EndClassRegister(type, L, translator);
}
[MonoPInvokeCallbackAttribute(typeof(LuaCSFunction))]
static int __CreateInstance(RealStatePtr L)
{
<%
if constructors.Count == 0 and (not type.IsValueType) then
%>return LuaAPI.luaL_error(L, "<%=CsFullTypeName(type)%> does not have a constructor!");<%
else %>
try {
ObjectTranslator translator = ObjectTranslatorPool.Instance.Find(L);
<%
local hasZeroParamsCtor = false
ForEachCsList(constructors, function(constructor, ci)
local parameters = constructor:GetParameters()
if parameters.Length == 0 then
hasZeroParamsCtor = true
end
local def_count = constructor_def_vals[ci]
local param_count = parameters.Length
local in_num = CalcCsList(parameters, function(p) return not (p.IsOut and p.ParameterType.IsByRef) end)
local out_num = CalcCsList(parameters, function(p) return p.IsOut or p.ParameterType.IsByRef end)
local real_param_count = param_count - def_count
local has_v_params = param_count > 0 and IsParams(parameters[param_count - 1])
local in_pos = 0
%>if(LuaAPI.lua_gettop(L) <%=has_v_params and ">=" or "=="%> <%=in_num + 1 - def_count - (has_v_params and 1 or 0)%><%ForEachCsList(parameters, function(parameter, pi)
if pi >= real_param_count then return end
local parameterType = parameter.ParameterType
if has_v_params and pi == param_count - 1 then parameterType = parameterType:GetElementType() end
if not (param
没有合适的资源?快使用搜索试试~ 我知道了~
Unity项目XLUA 的一个基础,基于这个基础,继续制作GamePlay.zip
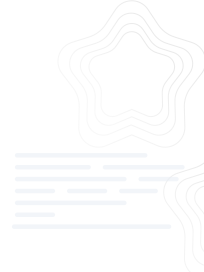
共595个文件
meta:324个
cs:127个
asset:36个

0 下载量 174 浏览量
2024-08-22
16:47:19
上传
评论
收藏 9.17MB ZIP 举报
温馨提示
项目工程资源经过严格测试可直接运行成功且功能正常的情况才上传,可轻松copy复刻,拿到资料包后可轻松复现出一样的项目,本人系统开发经验充足(全栈开发),有任何使用问题欢迎随时与我联系,我会及时为您解惑,提供帮助 【资源内容】:项目具体内容可查看/点击本页面下方的*资源详情*,包含完整源码+工程文件+说明(若有)等。【若无VIP,此资源可私信获取】 【本人专注IT领域】:有任何使用问题欢迎随时与我联系,我会及时解答,第一时间为您提供帮助 【附带帮助】:若还需要相关开发工具、学习资料等,我会提供帮助,提供资料,鼓励学习进步 【适合场景】:相关项目设计中,皆可应用在项目开发、毕业设计、课程设计、期末/期中/大作业、工程实训、大创等学科竞赛比赛、初期项目立项、学习/练手等方面中 可借鉴此优质项目实现复刻,也可基于此项目来扩展开发出更多功能 #注 1. 本资源仅用于开源学习和技术交流。不可商用等,一切后果由使用者承担 2. 部分字体及插图等来自网络,若是侵权请联系删除,本人不对所涉及的版权问题或内容负法律责任。收取的费用仅用于整理和收集资料耗费时间的酬劳 3. 积分资源不提供使用问题指导/解答
资源推荐
资源详情
资源评论
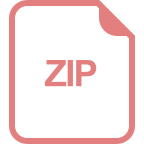
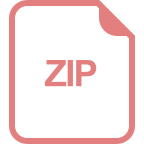
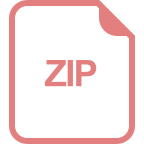
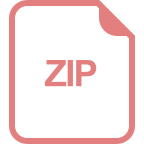
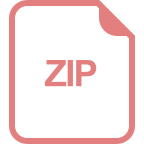
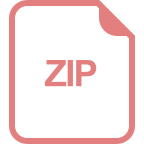
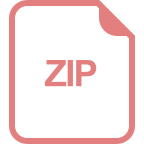
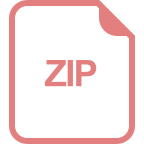
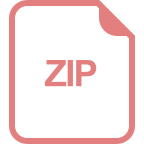
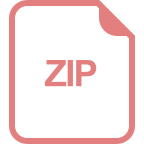
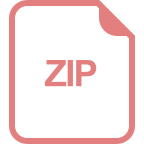
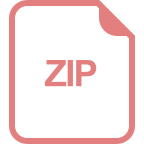
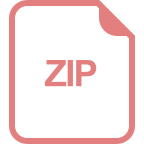
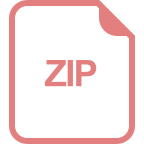
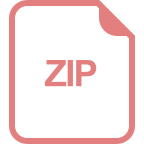
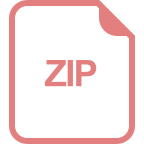
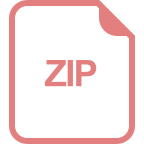
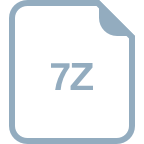
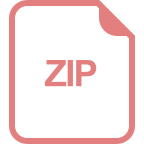
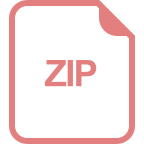
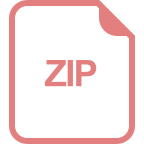
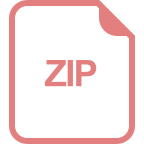
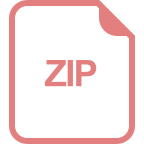
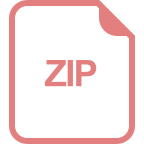
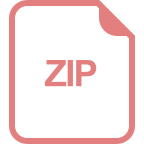
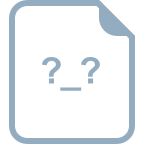
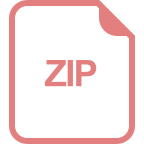
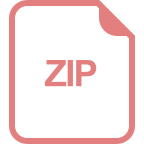
收起资源包目录

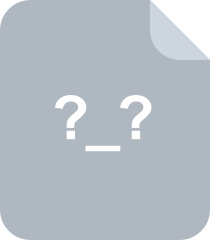
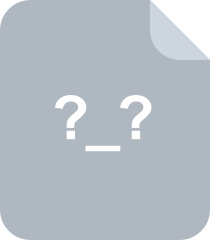
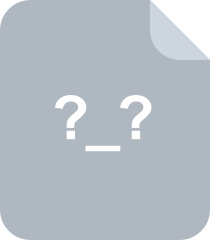
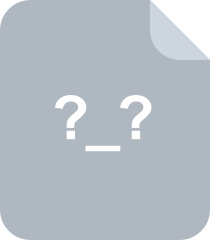
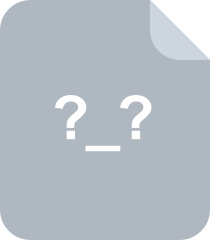
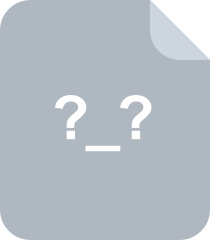
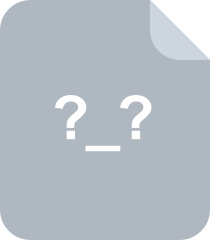
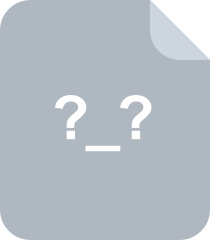
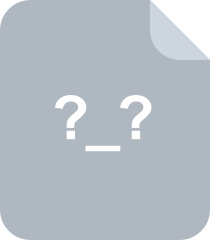
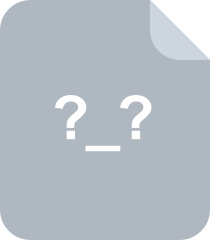
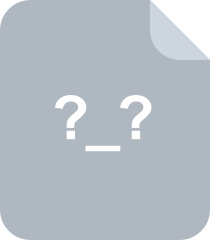
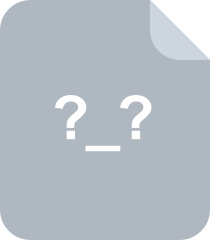
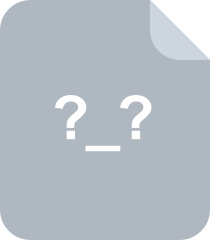
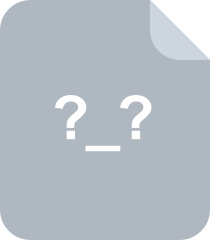
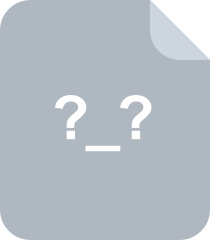
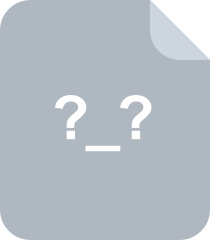
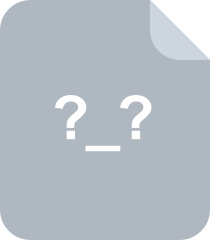
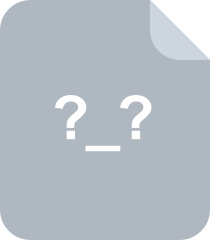
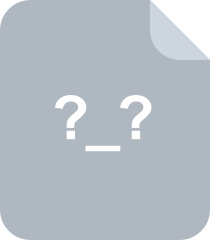
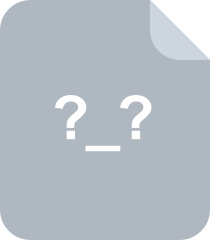
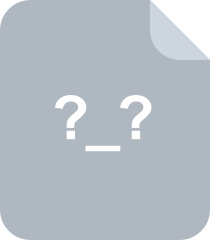
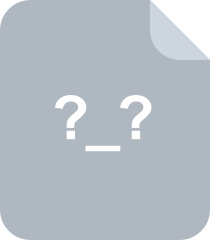
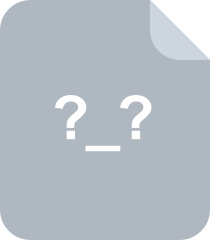
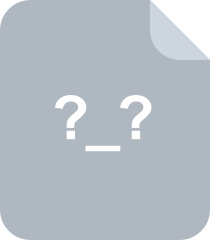
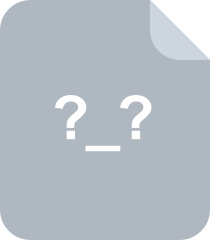
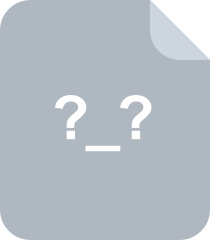
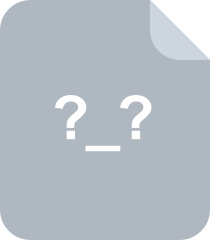
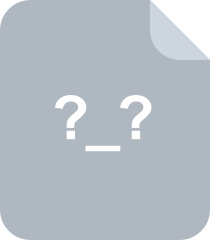
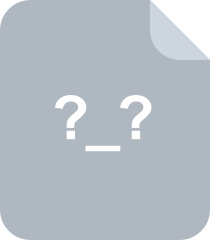
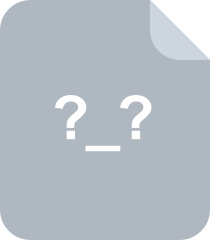
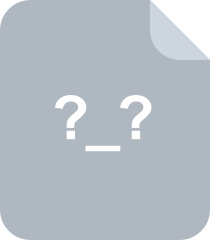
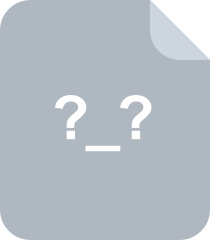
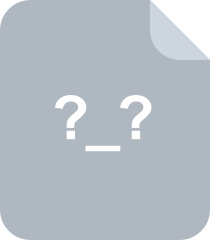
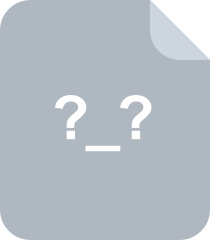
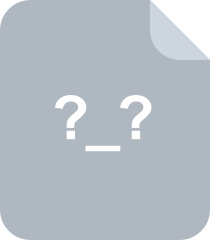
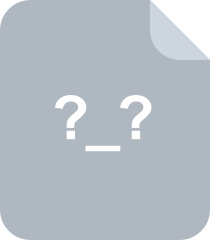
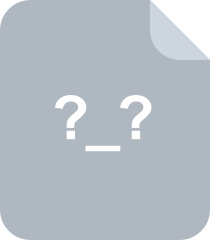
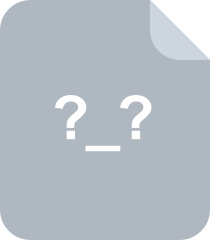
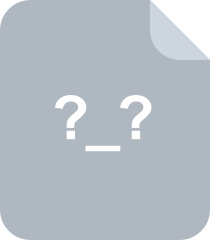
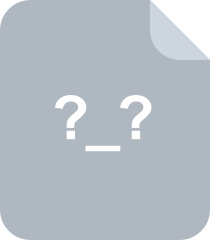
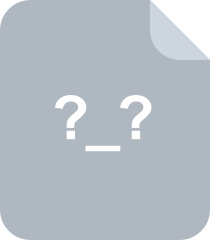
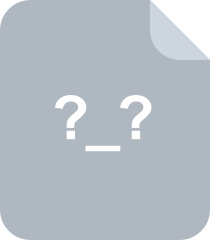
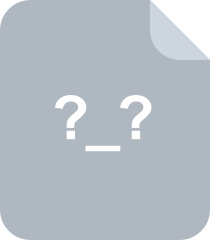
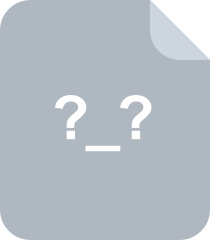
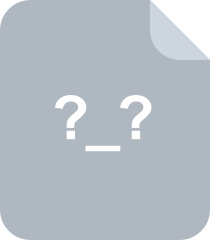
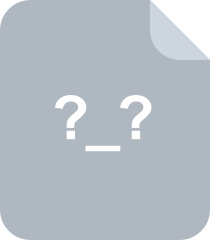
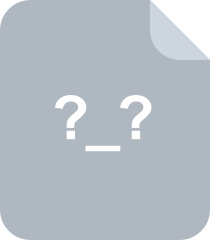
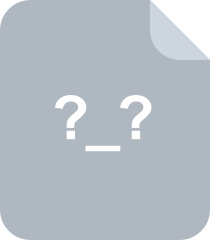
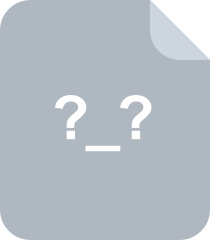
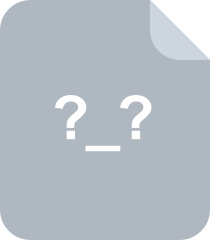
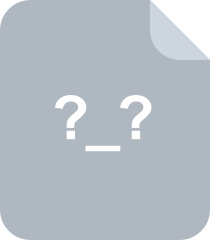
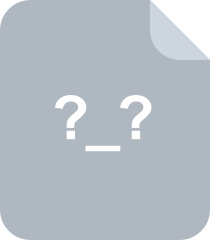
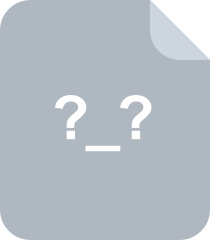
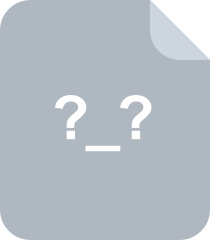
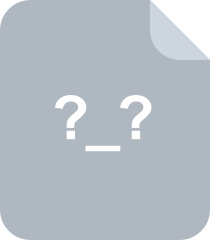
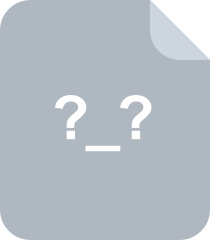
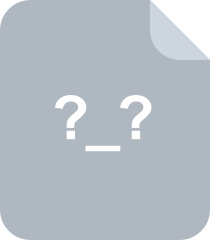
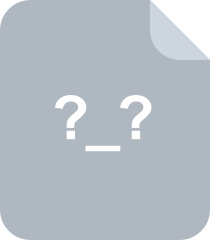
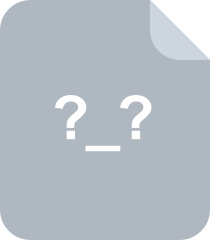
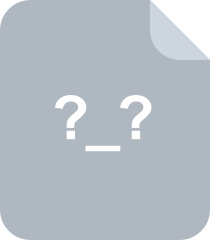
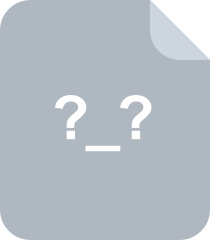
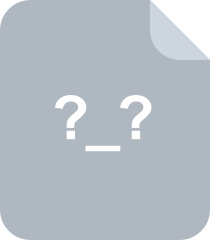
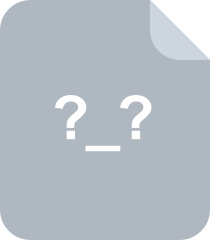
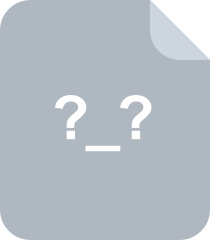
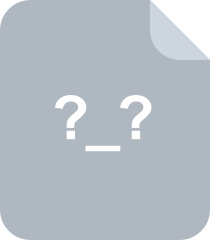
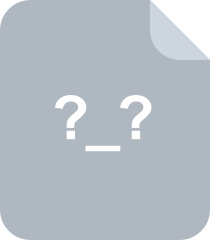
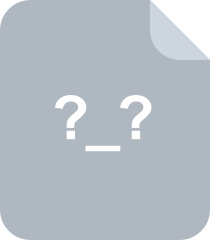
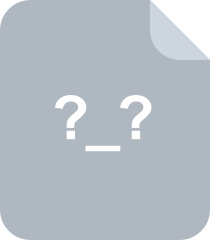
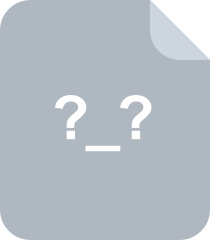
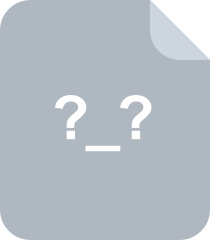
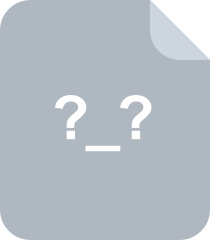
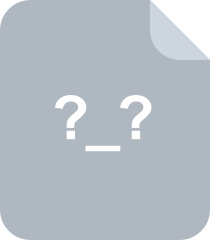
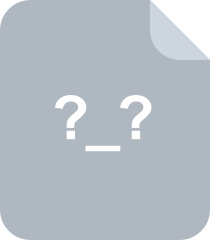
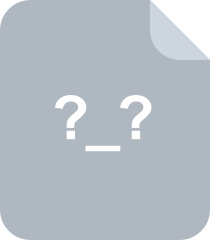
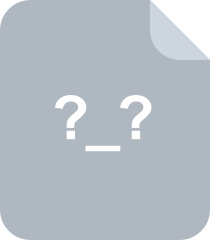
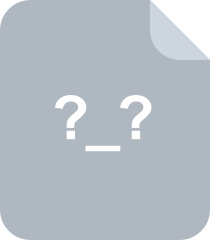
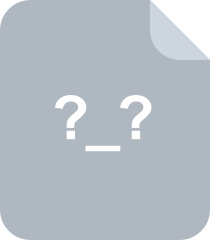
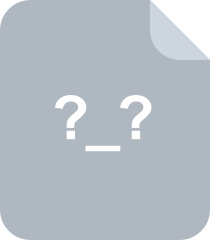
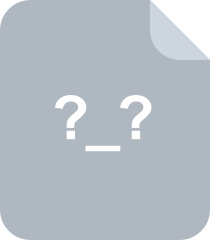
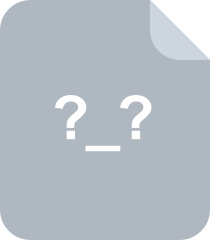
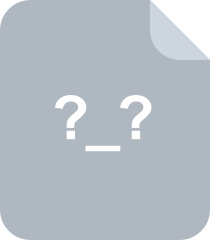
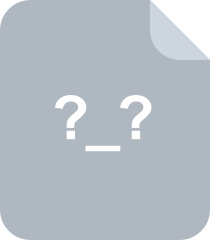
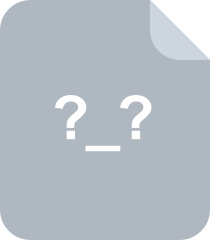
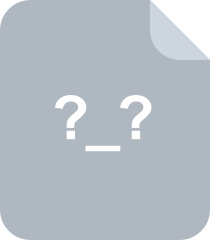
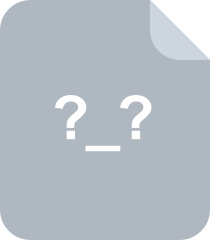
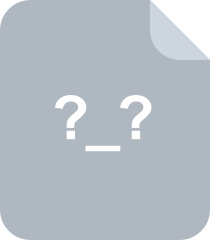
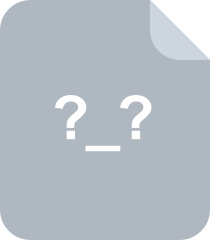
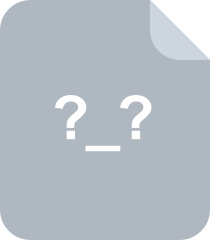
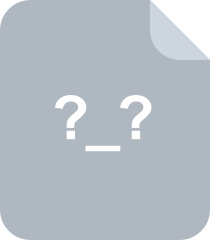
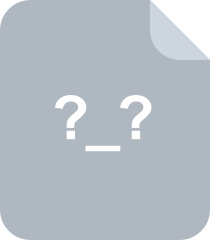
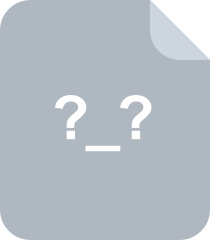
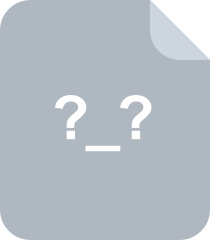
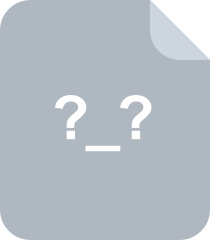
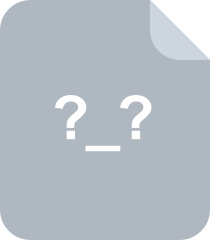
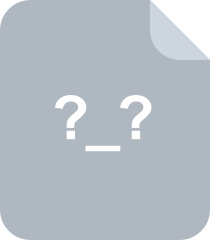
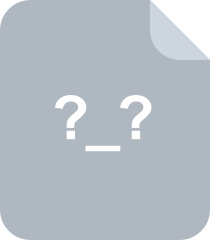
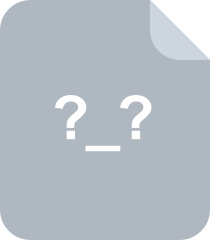
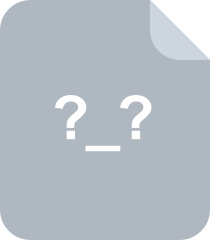
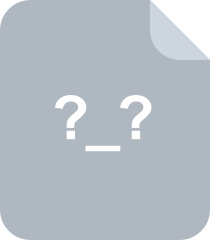
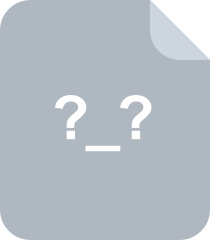
共 595 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
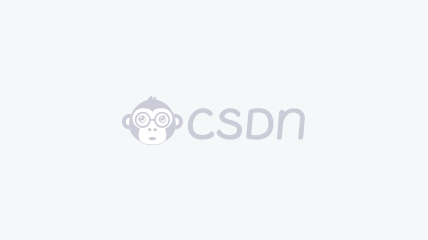

热爱技术。
- 粉丝: 2394
- 资源: 7862
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

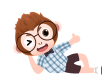
最新资源
- android文心一言的一个demo
- 1而长期无人称其为率请问去
- 而是根深蒂固很多水果和时代光华士大夫
- 2023-04-06-项目笔记 - 第三百零九阶段 - 4.4.2.307全局变量的作用域-307 -2025.11.06
- android studio原生开发的一个联接打印机的程序,实际应用的,打便携蓝牙打印机打印地磅单子的程序
- fmDrive-win-v1.2.3.exe
- 2023-04-06-项目笔记 - 第三百零九阶段 - 4.4.2.307全局变量的作用域-307 -2025.11.06
- 基于ssm框架+Spring boot+Mybatis整合实现增删改查(适合初学者入门必备也可以做脚手架开发)
- python实现DES算法
- 基于php+html实现的成绩管理系统【源码+数据库】
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


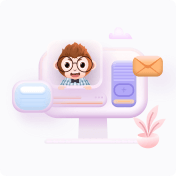
安全验证
文档复制为VIP权益,开通VIP直接复制
