This project was bootstrapped with [Create React App](https://github.com/facebookincubator/create-react-app).
Below you will find some information on how to perform common tasks.<br>
You can find the most recent version of this guide [here](https://github.com/facebookincubator/create-react-app/blob/master/packages/react-scripts/template/README.md).
## Table of Contents
- [Updating to New Releases](#updating-to-new-releases)
- [Sending Feedback](#sending-feedback)
- [Folder Structure](#folder-structure)
- [Available Scripts](#available-scripts)
- [npm start](#npm-start)
- [npm test](#npm-test)
- [npm run build](#npm-run-build)
- [npm run eject](#npm-run-eject)
- [Supported Browsers](#supported-browsers)
- [Supported Language Features and Polyfills](#supported-language-features-and-polyfills)
- [Syntax Highlighting in the Editor](#syntax-highlighting-in-the-editor)
- [Displaying Lint Output in the Editor](#displaying-lint-output-in-the-editor)
- [Debugging in the Editor](#debugging-in-the-editor)
- [Formatting Code Automatically](#formatting-code-automatically)
- [Changing the Page `<title>`](#changing-the-page-title)
- [Installing a Dependency](#installing-a-dependency)
- [Importing a Component](#importing-a-component)
- [Code Splitting](#code-splitting)
- [Adding a Stylesheet](#adding-a-stylesheet)
- [Post-Processing CSS](#post-processing-css)
- [Adding a CSS Preprocessor (Sass, Less etc.)](#adding-a-css-preprocessor-sass-less-etc)
- [Adding Images, Fonts, and Files](#adding-images-fonts-and-files)
- [Using the `public` Folder](#using-the-public-folder)
- [Changing the HTML](#changing-the-html)
- [Adding Assets Outside of the Module System](#adding-assets-outside-of-the-module-system)
- [When to Use the `public` Folder](#when-to-use-the-public-folder)
- [Using Global Variables](#using-global-variables)
- [Adding Bootstrap](#adding-bootstrap)
- [Using a Custom Theme](#using-a-custom-theme)
- [Adding Flow](#adding-flow)
- [Adding a Router](#adding-a-router)
- [Adding Custom Environment Variables](#adding-custom-environment-variables)
- [Referencing Environment Variables in the HTML](#referencing-environment-variables-in-the-html)
- [Adding Temporary Environment Variables In Your Shell](#adding-temporary-environment-variables-in-your-shell)
- [Adding Development Environment Variables In `.env`](#adding-development-environment-variables-in-env)
- [Can I Use Decorators?](#can-i-use-decorators)
- [Fetching Data with AJAX Requests](#fetching-data-with-ajax-requests)
- [Integrating with an API Backend](#integrating-with-an-api-backend)
- [Node](#node)
- [Ruby on Rails](#ruby-on-rails)
- [Proxying API Requests in Development](#proxying-api-requests-in-development)
- ["Invalid Host Header" Errors After Configuring Proxy](#invalid-host-header-errors-after-configuring-proxy)
- [Configuring the Proxy Manually](#configuring-the-proxy-manually)
- [Configuring a WebSocket Proxy](#configuring-a-websocket-proxy)
- [Using HTTPS in Development](#using-https-in-development)
- [Generating Dynamic `<meta>` Tags on the Server](#generating-dynamic-meta-tags-on-the-server)
- [Pre-Rendering into Static HTML Files](#pre-rendering-into-static-html-files)
- [Injecting Data from the Server into the Page](#injecting-data-from-the-server-into-the-page)
- [Running Tests](#running-tests)
- [Filename Conventions](#filename-conventions)
- [Command Line Interface](#command-line-interface)
- [Version Control Integration](#version-control-integration)
- [Writing Tests](#writing-tests)
- [Testing Components](#testing-components)
- [Using Third Party Assertion Libraries](#using-third-party-assertion-libraries)
- [Initializing Test Environment](#initializing-test-environment)
- [Focusing and Excluding Tests](#focusing-and-excluding-tests)
- [Coverage Reporting](#coverage-reporting)
- [Continuous Integration](#continuous-integration)
- [Disabling jsdom](#disabling-jsdom)
- [Snapshot Testing](#snapshot-testing)
- [Editor Integration](#editor-integration)
- [Debugging Tests](#debugging-tests)
- [Debugging Tests in Chrome](#debugging-tests-in-chrome)
- [Debugging Tests in Visual Studio Code](#debugging-tests-in-visual-studio-code)
- [Developing Components in Isolation](#developing-components-in-isolation)
- [Getting Started with Storybook](#getting-started-with-storybook)
- [Getting Started with Styleguidist](#getting-started-with-styleguidist)
- [Publishing Components to npm](#publishing-components-to-npm)
- [Making a Progressive Web App](#making-a-progressive-web-app)
- [Opting Out of Caching](#opting-out-of-caching)
- [Offline-First Considerations](#offline-first-considerations)
- [Progressive Web App Metadata](#progressive-web-app-metadata)
- [Analyzing the Bundle Size](#analyzing-the-bundle-size)
- [Deployment](#deployment)
- [Static Server](#static-server)
- [Other Solutions](#other-solutions)
- [Serving Apps with Client-Side Routing](#serving-apps-with-client-side-routing)
- [Building for Relative Paths](#building-for-relative-paths)
- [Azure](#azure)
- [Firebase](#firebase)
- [GitHub Pages](#github-pages)
- [Heroku](#heroku)
- [Netlify](#netlify)
- [Now](#now)
- [S3 and CloudFront](#s3-and-cloudfront)
- [Surge](#surge)
- [Advanced Configuration](#advanced-configuration)
- [Troubleshooting](#troubleshooting)
- [`npm start` doesn’t detect changes](#npm-start-doesnt-detect-changes)
- [`npm test` hangs on macOS Sierra](#npm-test-hangs-on-macos-sierra)
- [`npm run build` exits too early](#npm-run-build-exits-too-early)
- [`npm run build` fails on Heroku](#npm-run-build-fails-on-heroku)
- [`npm run build` fails to minify](#npm-run-build-fails-to-minify)
- [Moment.js locales are missing](#momentjs-locales-are-missing)
- [Alternatives to Ejecting](#alternatives-to-ejecting)
- [Something Missing?](#something-missing)
## Updating to New Releases
Create React App is divided into two packages:
* `create-react-app` is a global command-line utility that you use to create new projects.
* `react-scripts` is a development dependency in the generated projects (including this one).
You almost never need to update `create-react-app` itself: it delegates all the setup to `react-scripts`.
When you run `create-react-app`, it always creates the project with the latest version of `react-scripts` so you’ll get all the new features and improvements in newly created apps automatically.
To update an existing project to a new version of `react-scripts`, [open the changelog](https://github.com/facebookincubator/create-react-app/blob/master/CHANGELOG.md), find the version you’re currently on (check `package.json` in this folder if you’re not sure), and apply the migration instructions for the newer versions.
In most cases bumping the `react-scripts` version in `package.json` and running `npm install` in this folder should be enough, but it’s good to consult the [changelog](https://github.com/facebookincubator/create-react-app/blob/master/CHANGELOG.md) for potential breaking changes.
We commit to keeping the breaking changes minimal so you can upgrade `react-scripts` painlessly.
## Sending Feedback
We are always open to [your feedback](https://github.com/facebookincubator/create-react-app/issues).
## Folder Structure
After creation, your project should look like this:
```
my-app/
README.md
node_modules/
package.json
public/
index.html
favicon.ico
src/
App.css
App.js
App.test.js
index.css
index.js
logo.svg
```
For the project to build, **these files must exist with exact filenames**:
* `public/index.html` is the page template;
* `src/index.js` is the JavaScript entry point.
You can delete or rename the other files.
You may create subdirectories inside `src`. For faster rebuilds, only files inside `src` are processed by Webpack.<br>
You need to **put any JS and CSS files inside `src`**, otherwise Webpack won’t see them.
Only files inside `pub
没有合适的资源?快使用搜索试试~ 我知道了~
Web前端工程化与组件化开发实践.zip
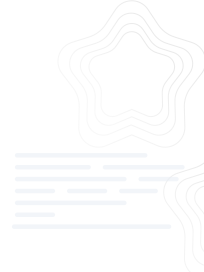
共389个文件
md:107个
png:89个
js:62个

0 下载量 30 浏览量
2024-08-21
12:13:41
上传
评论
收藏 8.02MB ZIP 举报
温馨提示
项目工程资源经过严格测试可直接运行成功且功能正常的情况才上传,可轻松copy复刻,拿到资料包后可轻松复现出一样的项目,本人系统开发经验充足(全栈开发),有任何使用问题欢迎随时与我联系,我会及时为您解惑,提供帮助 【资源内容】:项目具体内容可查看/点击本页面下方的*资源详情*,包含完整源码+工程文件+说明(若有)等。【若无VIP,此资源可私信获取】 【本人专注IT领域】:有任何使用问题欢迎随时与我联系,我会及时解答,第一时间为您提供帮助 【附带帮助】:若还需要相关开发工具、学习资料等,我会提供帮助,提供资料,鼓励学习进步 【适合场景】:相关项目设计中,皆可应用在项目开发、毕业设计、课程设计、期末/期中/大作业、工程实训、大创等学科竞赛比赛、初期项目立项、学习/练手等方面中 可借鉴此优质项目实现复刻,也可基于此项目来扩展开发出更多功能 #注 1. 本资源仅用于开源学习和技术交流。不可商用等,一切后果由使用者承担 2. 部分字体及插图等来自网络,若是侵权请联系删除,本人不对所涉及的版权问题或内容负法律责任。收取的费用仅用于整理和收集资料耗费时间的酬劳 3. 积分资源不提供使用问题指导/解答
资源推荐
资源详情
资源评论
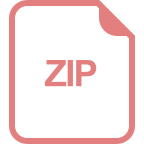
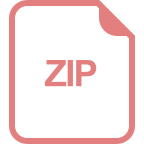
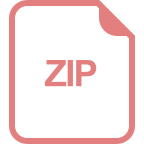
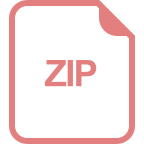
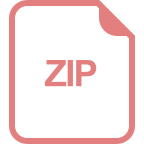
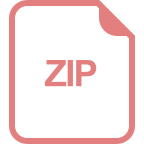
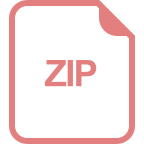
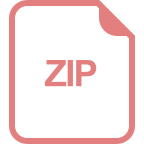
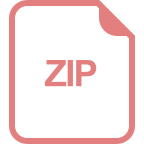
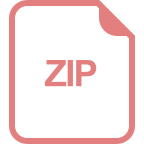
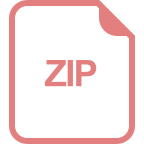
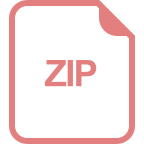
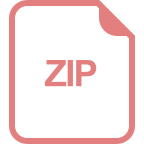
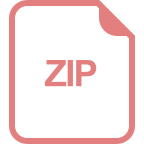
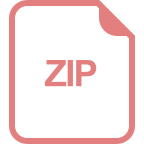
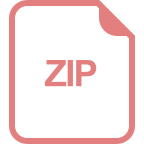
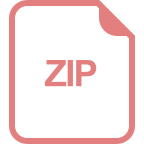
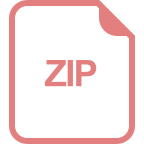
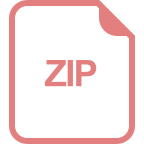
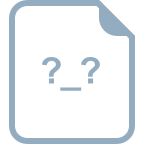
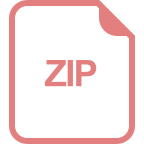
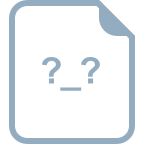
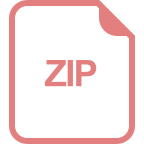
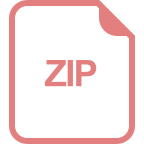
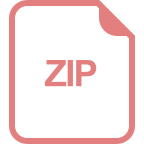
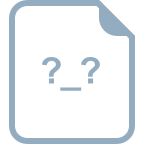
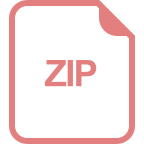
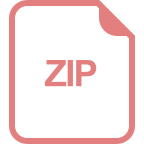
收起资源包目录

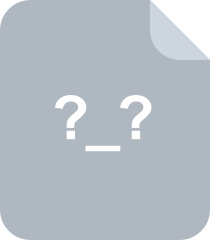
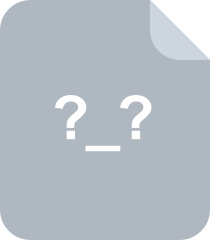
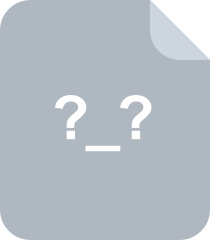
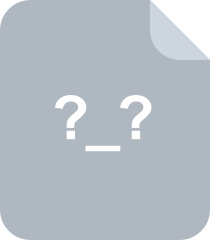
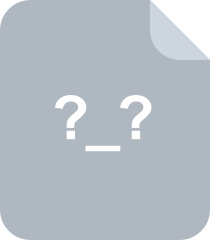
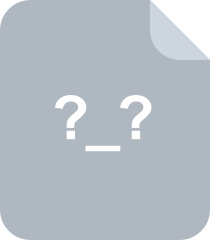
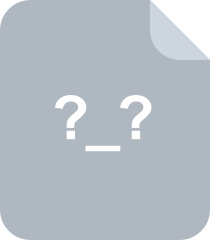
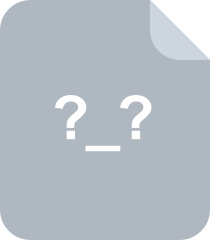
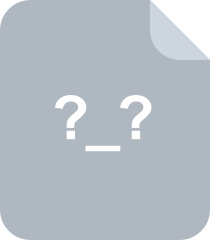
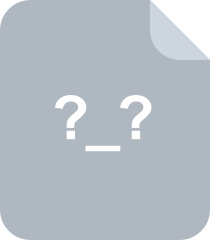
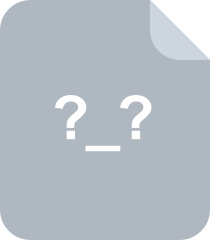
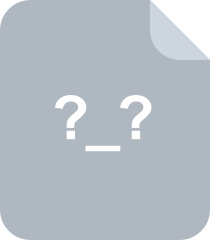
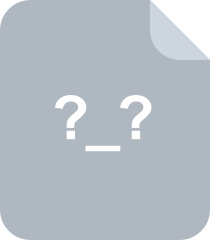
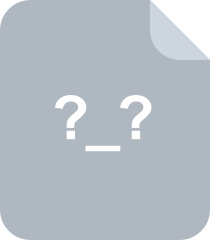
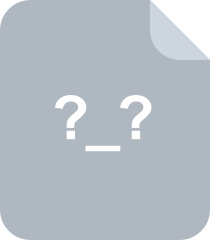
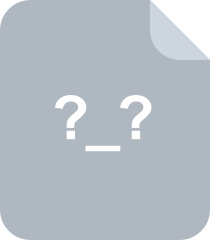
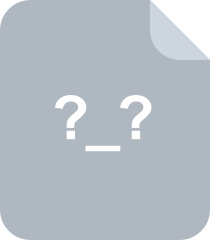
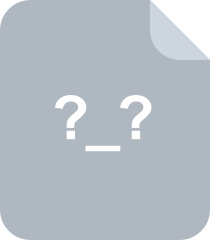
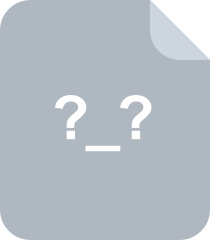
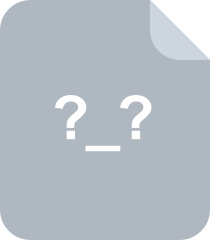
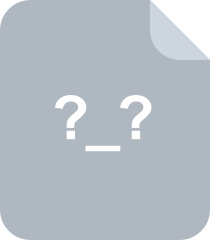
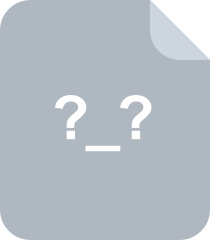
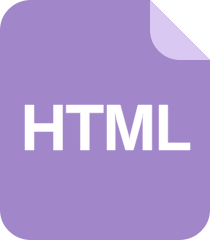
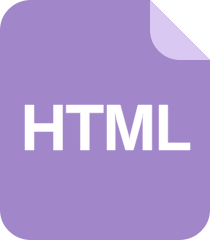
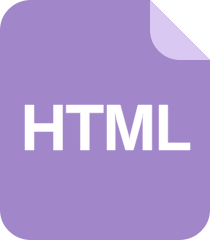
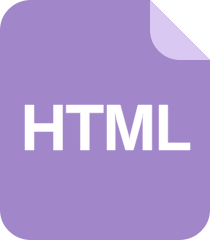
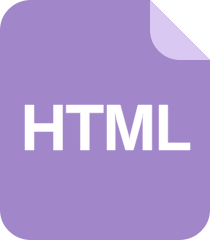
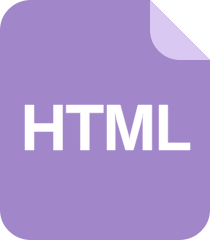
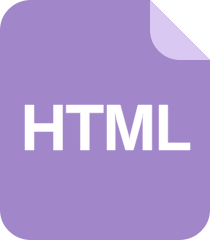
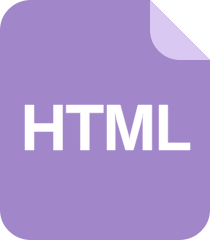
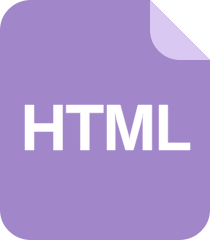
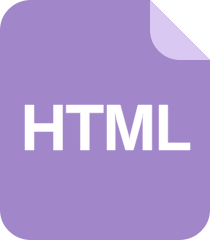
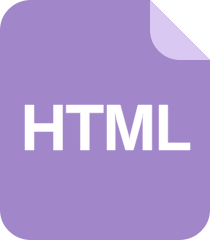
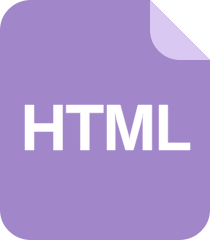
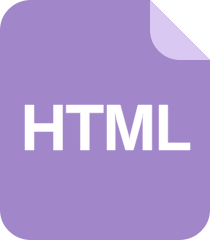
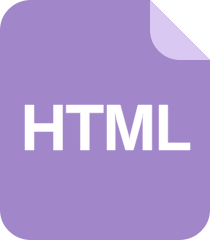
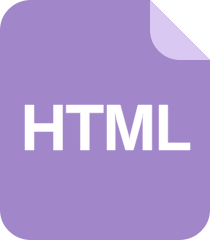
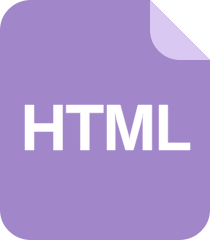
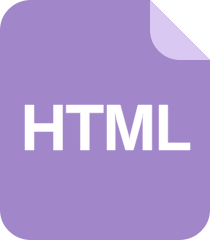
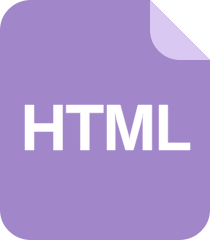
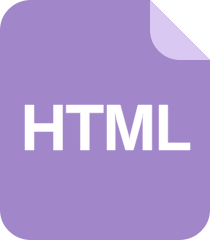
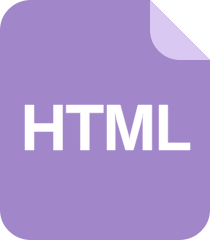
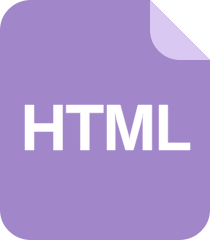
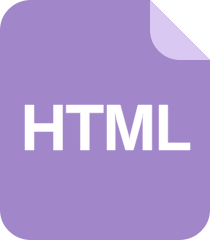
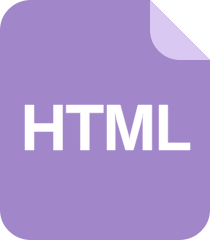
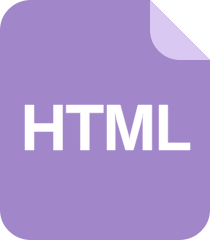
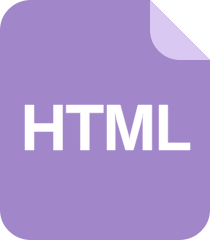
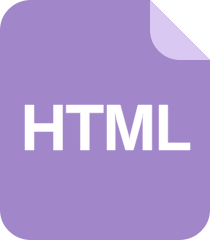
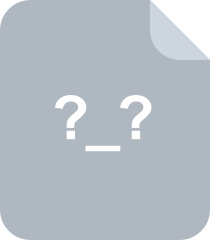
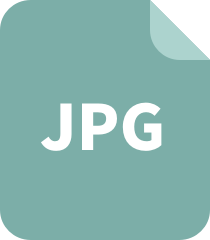
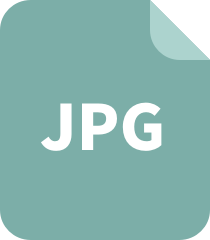
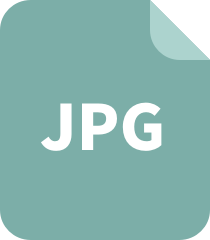
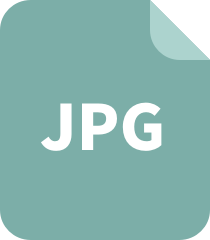
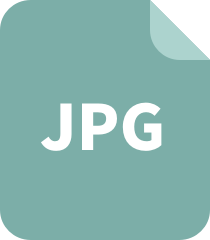
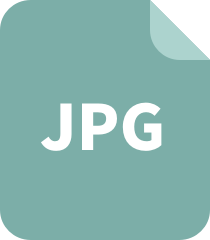
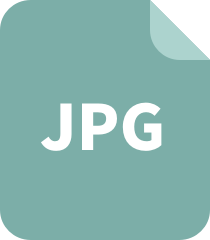
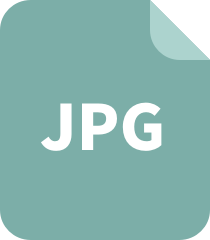
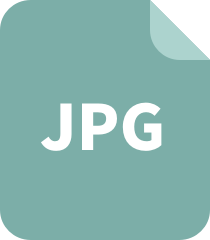
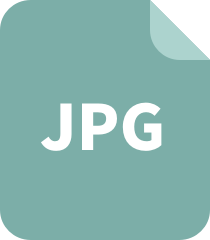
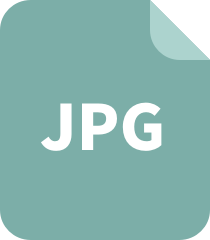
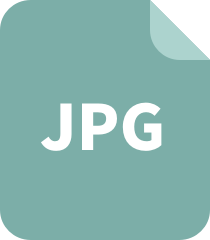
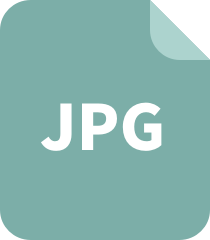
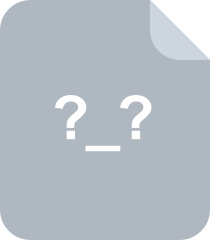
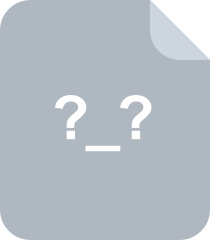
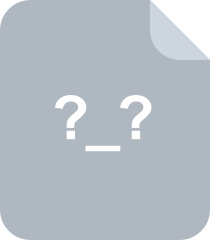
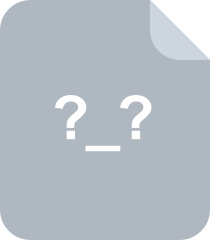
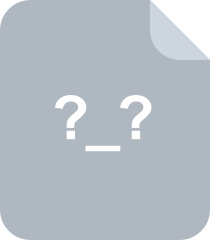
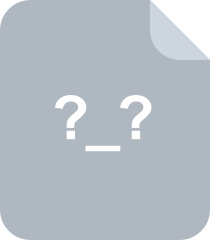
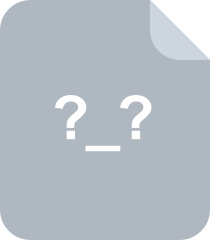
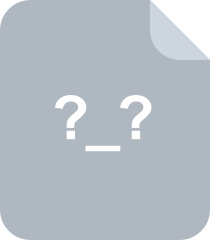
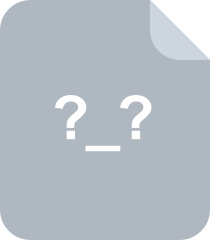
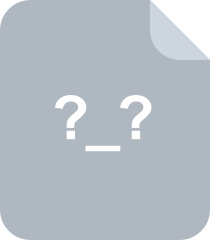
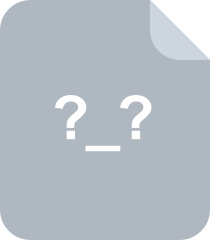
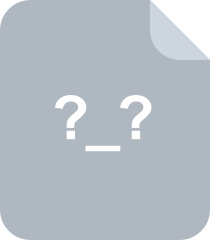
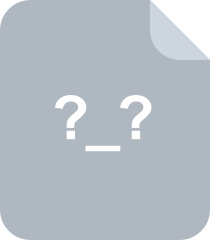
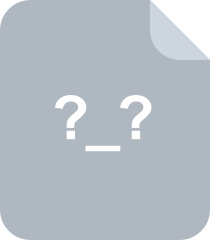
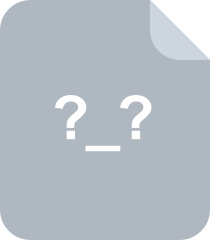
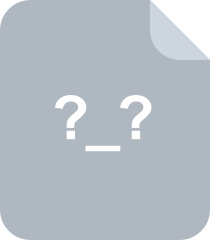
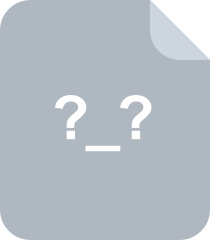
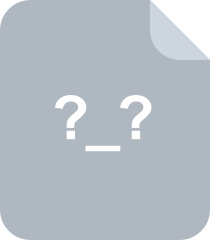
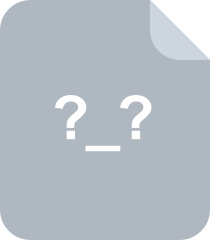
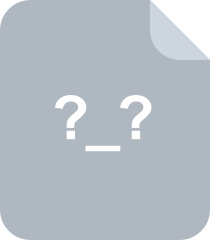
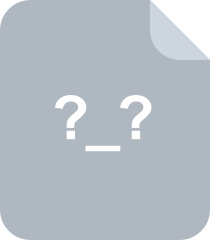
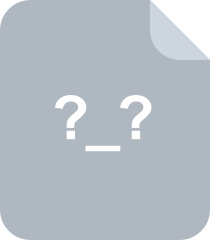
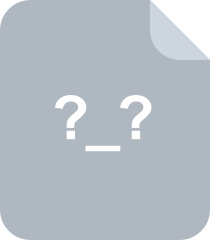
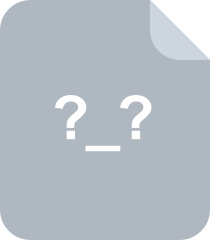
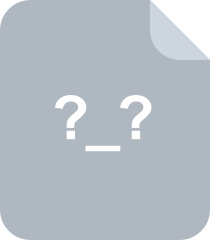
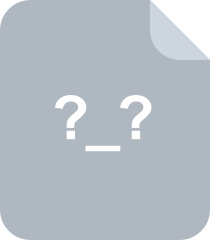
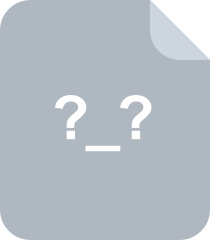
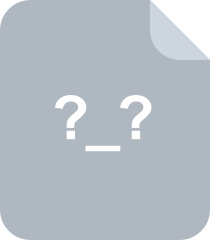
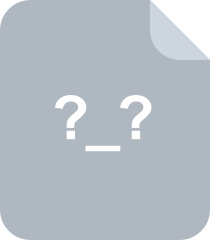
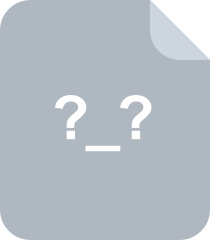
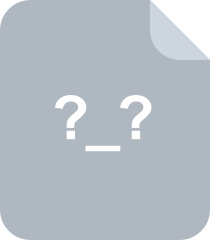
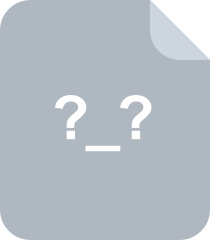
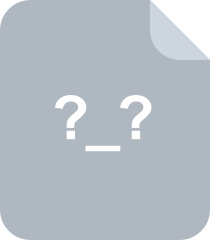
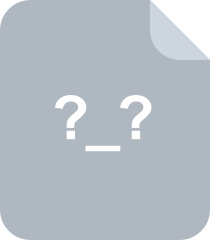
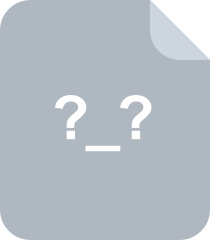
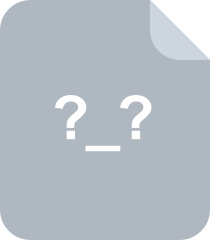
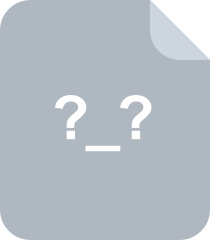
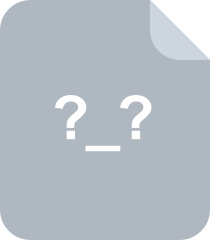
共 389 条
- 1
- 2
- 3
- 4
资源评论
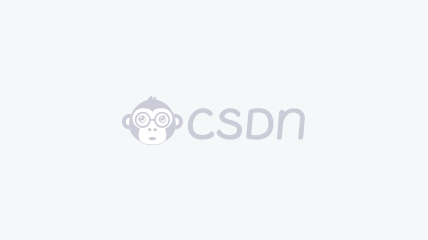

热爱技术。
- 粉丝: 2965
- 资源: 7864
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

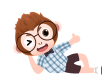
最新资源
- Alook浏览器安卓版(8倍速手机浏览器)v9.5.0.mp4
- Avast清理 Avast Cleanup v24.22.0高级版.mp4
- Apt Full(AI生产力工具v2.7.1.0绿色无需联网.mp4
- 01-03-若依表结构.xmind
- AZ屏幕录制工具Screen Recorder v6.3.6高级版.mp4
- 【本体】Internet Download Manager v6.42.26.zip
- Bing Wallpaper微软壁纸v2.0.1.4 中文多语版.mp4
- bsod蓝屏伪装者v1.0单文件版.mp4
- B站0粉代码强开自动回复-附源代码.mp4
- Canvas制作的粒子十秒倒计时HTML源码.mp4
- B站视频下载器 v2024.12.11小工具软件.mp4
- Complete Internet Repair 网络修复工具v11.1.3.mp4
- Converter视频音频转换器v2.2.5.2解锁VIP版.mp4
- CPU-Z处理器相关系统信息识别v2.13.0单文件.mp4
- pdf-reader.zip
- DNS快捷工具箱轻量高性能DNS服务器工具.mp4
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


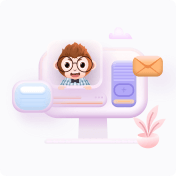
安全验证
文档复制为VIP权益,开通VIP直接复制
