#include "../header/main.h"
#include <time.h>
#include<stdlib.h>
#include<stdio.h>
#include<fstream>
#include <json/json.h>
#include <map>
static class MY_SQLC msql;
static class MY_SQLC msql1;
static class MY_SQLC msql2;
static char cmd[10240] = {0};
//char host[]="192.168.81.38";
char* var;
char* keystoneAddr;
char* novaAddr;
char* ceilometerAddr;
char* tenantName;
char* cloudUsername;
char* cloudPassword;
char* mysqlHost;
char* mysqlUsername;
char* mysqlPwd;
char* database;
string token;
string tenant_id;
string json_dir;
int rc =0;
char* key = new char[20];
typedef map<string,string> STR_STR_MAP;
STR_STR_MAP user_map;
STR_STR_MAP image_map;
STR_STR_MAP flavor_vcpu_map;
STR_STR_MAP flavor_ram_map;
STR_STR_MAP flavor_disk_map;
STR_STR_MAP tenant_instance_quota;
STR_STR_MAP tenant_core_quota;
STR_STR_MAP tenant_ram_quota;
char* quota_scan(char* project_id,char* key)
{
string str_tenant_id = string(project_id);
char* temp = NULL;
if(string(key)=="instances")
{
string instances_quota = tenant_instance_quota[str_tenant_id];
temp = new char[instances_quota.length()+1];
strcpy(temp,instances_quota.c_str());
return temp;
}
else if(string(key)=="cores")
{
string cores_quota = tenant_core_quota[str_tenant_id];
temp = new char[cores_quota.length()+1];
strcpy(temp,cores_quota.c_str());
return temp;
}
else if(string(key)=="ram")
{
string ram_quota = tenant_ram_quota[str_tenant_id];
temp = new char[ram_quota.length()+1];
strcpy(temp,ram_quota.c_str());
return temp;
}
}
/*
扫描用户,并进一步计算用户的积分
*/
int user_scan()
{
//memset(cmd,0,sizeof(cmd));
//snprintf(cmd,sizeof(cmd),"%s","select keystone.user.name as username , keystone.user.id as user_id ,default_project_id as project_id , keystone.project.name as project_name from keystone.user inner join keystone.project where keystone.user.default_project_id = keystone.project.id;");
//msql.free_res();
//msql.query_select(cmd);
//int record_count = msql.getrecordcount();
char* username = NULL;
char* user_id = NULL;
char* project_id = NULL;
double SumScoreRecharge = 0;
double SumScoreUsed = 0;
double vcpu_hour = 0;
int instances_all =0;
int instances_used =0;
int instances_active =0;
int instances_shutdown =0;
int instances_error =0;
int instances_paused =0;
int instances_building =0;
int instances_deleted =0;
int cores_all =0;
int cores_used =0;
int ram_all = 0;
int ram_used = 0;
//char filename[100];memset(filename,0,sizeof(filename);) = "/root/user.json";
char filename[100];memset(filename,0,sizeof(filename));
sprintf(filename,"%s/user.json",json_dir.c_str());
Json::Reader reader;// 解析json用Json::Reader
Json::Value root; // Json::Value是一种很重要的类型,可以代表任意类型。如int, string, object, array
std::ifstream is;
is.open (filename, std::ios::binary );
if (reader.parse(is, root, 0))
{
int user_size = root["users"].size(); // 得到"files"的数组个数
for(int i = 0; i < user_size; ++i) // 遍历数组
{
/****使用ldap时****/
/**
std::string name = root["users"][i]["username"].asString();
username = new char[name.length()+1];
strcpy(username,name.c_str());
**/
/****不使用ldap时****/
/****Watch out differences between openstack icehouse and kilo!****/
std::string name = root["users"][i]["username"].asString();
std::string id = root["users"][i]["id"].asString();
std::string pro_id = root["users"][i]["tenantId"].asString();
username = new char[name.length()+1];
strcpy(username,name.c_str());
user_id = new char[id.length()+1];
strcpy(user_id,id.c_str());
project_id = new char[pro_id.length()+1];
strcpy(project_id,pro_id.c_str());
if((name=="nova")||(name=="glance")||(name=="quantum")||(name=="cinder")||(name=="neutron")||(name=="ceilometer")||(name=="glance"))
continue;
memset(cmd,0,sizeof(cmd));
//snprintf(cmd,sizeof(cmd),"select sum(ScoreUsed) as SumScoreUsed , sum(ScoreRecharge) as SumScoreRecharge from %s.user_score where user_name = '%s';",username);
snprintf(cmd,sizeof(cmd),"select %s.userinfo.SumScoreUsed as SumScoreUsed ,%s.userinfo.SumScoreRecharge as SumScoreRecharge from %s.userinfo where user_name = '%s';",database,database,database,username);
msql1.free_res();
msql1.query_select(cmd);
if(!msql1.FetchRow()||!msql1.getrecordcount())
{
SumScoreUsed = 0;
SumScoreRecharge =0;
}
else{
strcpy(key,"SumScoreUsed");
SumScoreUsed = atof(msql1.GetField(key));
strcpy(key,"SumScoreRecharge");
SumScoreRecharge = atof(msql1.GetField(key));
}
/****使用ldap时****/
/**
//这里project_id存的是username
strcpy(key,"instances";
instances_all = atoi(quota_scan(username,key));
strcpy(key,"cores";
cores_all = atoi(quota_scan(username,key));
strcpy(key,"ram";
ram_all = atoi(quota_scan(username,key));
**/
/****不使用ldap时****/
strcpy(key,"instances");
instances_all = atoi(quota_scan(username,key));
strcpy(key,"cores");
cores_all = atoi(quota_scan(username,key));
strcpy(key,"ram");
ram_all = atoi(quota_scan(username,key));
memset(cmd,0,sizeof(cmd));
/****使用ldap时****/
//snprintf(cmd,sizeof(cmd),"select count(display_name) as used_count from instances where user_id = '%s' and (vm_state = 'active' or vm_state = 'stopped');",username);
/****不使用ldap时****/
snprintf(cmd,sizeof(cmd),"select count(vm_name) as used_count from %s.vms where user_id = '%s' and (vm_state = 'active' or vm_state = 'stopped');",database,user_id);
msql1.free_res();
msql1.query_select(cmd);
strcpy(key,"used_count");
if(!msql1.FetchRow())
{
instances_used = 0;
}
else
{
instances_used = atoi(msql1.GetField(key));
}
memset(cmd,0,sizeof(cmd));
/****使用ldap时****/
//snprintf(cmd,sizeof(cmd),"select count(display_name) as active_count from instances where user_id = '%s' and vm_state = 'active';",username);
/****不使用ldap时****/
snprintf(cmd,sizeof(cmd),"select count(vm_name) as active_count from %s.vms where user_id = '%s' and vm_state = 'active';",database,user_id);
msql1.free_res();
msql1.query_select(cmd);
strcpy(key,"active_count");
if(!msql1.FetchRow())
{
instances_active = 0;
}
else
{
instances_active = atoi(msql1.GetField(key));
}
memset(cmd,0,sizeof(cmd));
/****使用ldap时****/
//snprintf(cmd,sizeof(cmd),"select count(display_name) as shutdown_count from instances where user_id = '%s' and vm_state = 'stopped';",username);
/****不使用ldap时****/
snprintf(cmd,sizeof(cmd),"select count(vm_name) as shutdown_count from %s.vms where user_id = '%s' and vm_state = 'stopped';",database,user_id);
msql1.free_res();
msql1.query_select(cmd);
strcpy(key,"shutdown_count");
if(!msql1.FetchRow())
{
instances_shutdown = 0;
}
else
{
instances_shutdown = atoi(msql1.GetField(key));
}
memset(cmd,0,sizeof(cmd));
/****使用ldap时****/
//snprintf(cmd,sizeof(cmd),"select count(display_name) as shutdown_count from instances where user_id = '%s' and vm_state = 'error';",username);
/****不使用ldap时****/
snprintf(cmd,sizeof(cmd),"select count(vm_name) as error_count from %s.vms where user_id = '%s' and vm_state = 'error';",database,user_id);
msql1.free_res();
msql1.query_select(cmd);
strcpy(key,"error_count");
if(!msql1.FetchRow())
{
instances_error = 0;
}
else
{
instances_error = atoi(msql1.GetField(key));
}
memset(cmd,0,sizeof(cmd
没有合适的资源?快使用搜索试试~ 我知道了~
一个对高能所云计算平台的积分计费程序,不包括前台网页php的部分.zip
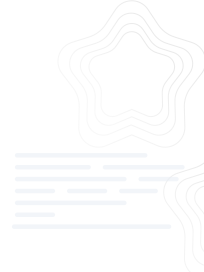
共2000个文件
json:1975个
cpp:18个
sh:4个

需积分: 1 0 下载量 128 浏览量
2024-08-10
10:57:19
上传
评论
收藏 5.54MB ZIP 举报
温馨提示
项目工程资源经过严格测试可直接运行成功且功能正常的情况才上传,可轻松copy复刻,拿到资料包后可轻松复现出一样的项目,本人系统开发经验充足,有任何使用问题欢迎随时与我联系,我会及时为你解惑,提供帮助 【资源内容】:项目具体内容可查看下方的资源详情,包含完整源码+工程文件+说明(若有)等 【附带帮助】:若还需要相关开发工具、学习资料等,我会提供帮助,提供资料,鼓励学习进步 【本人专注计算机领域】:有任何使用问题欢迎随时与我联系,我会及时解答,第一时间为你提供帮助,CSDN博客端可私信,为你解惑,欢迎交流 【适合场景】:相关项目设计中,皆可应用在项目开发、毕业设计、课程设计、期末/期中/大作业、工程实训、大创等学科竞赛比赛、初期项目立项、学习/练手等方面中 可借鉴此优质项目实现复刻,也可以基于此项目进行扩展来开发出更多功能 #注 1. 本资源仅用于开源学习和技术交流。不可商用等,一切后果由使用者承担 2. 部分字体及插图等来自网络,若是侵权请联系删除,本人不对所涉及的版权问题或内容负法律责任。收取的费用仅用于收集和整理资料耗费时问的酬劳
资源推荐
资源详情
资源评论
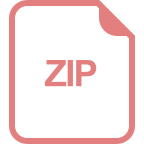
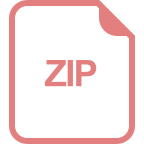
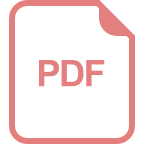
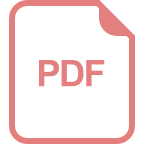
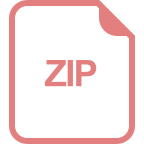
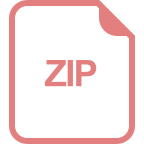
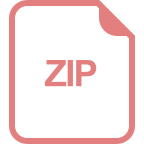
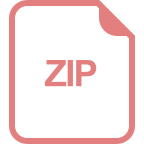
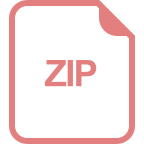
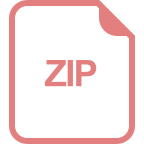
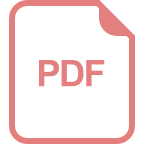
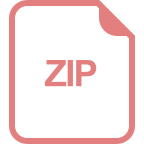
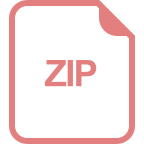
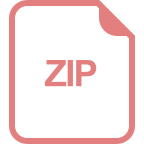
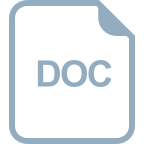
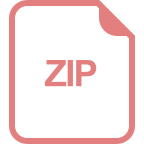
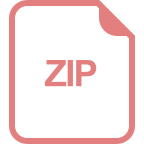
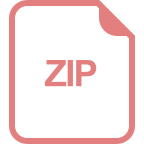
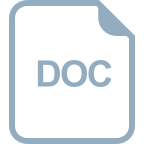
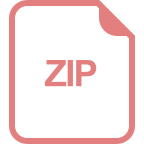
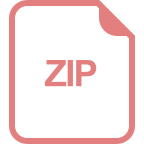
收起资源包目录

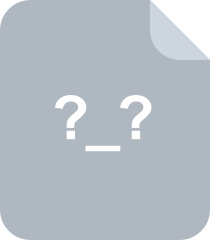
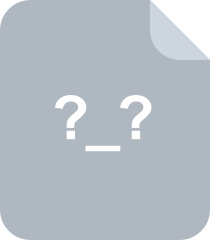
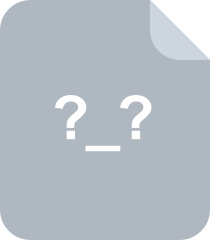
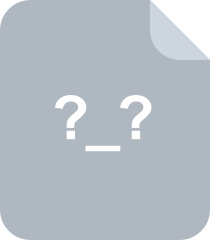
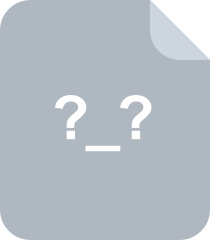
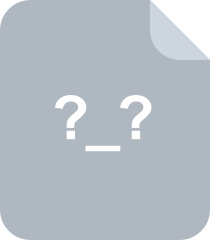
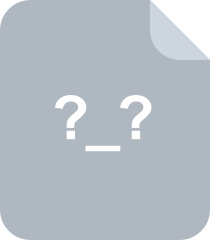
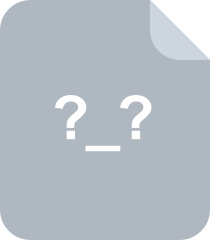
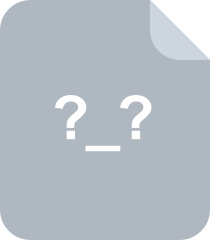
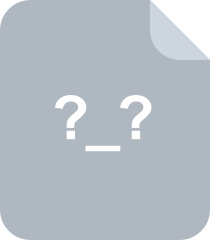
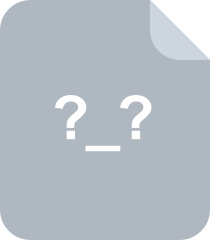
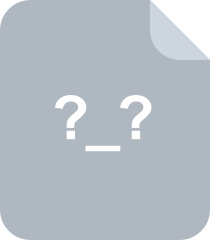
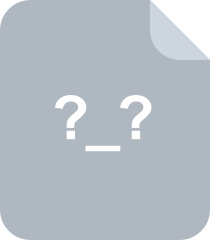
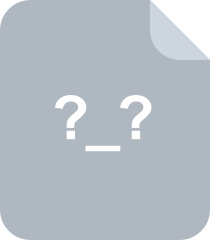
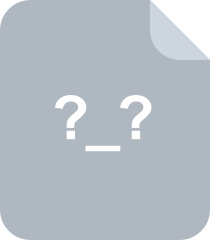
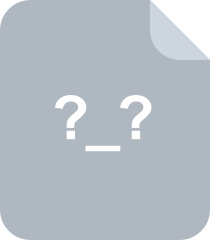
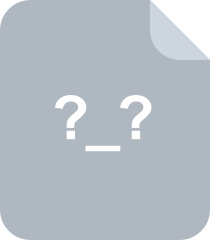
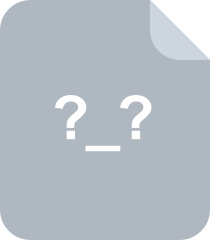
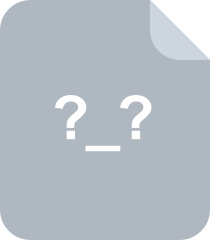
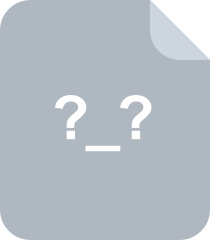
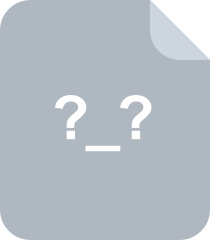
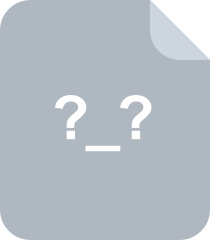
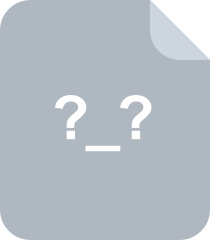
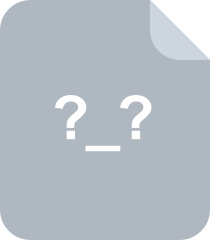
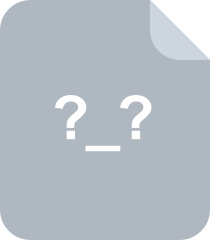
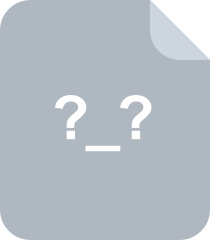
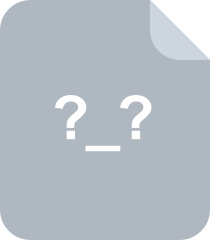
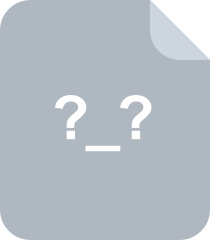
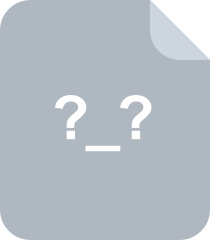
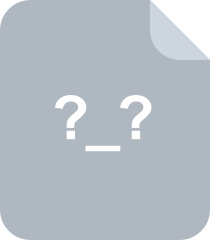
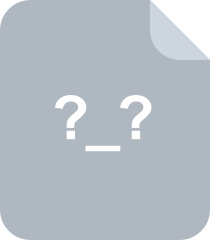
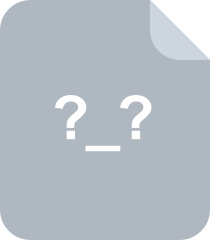
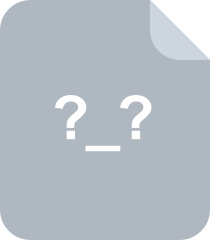
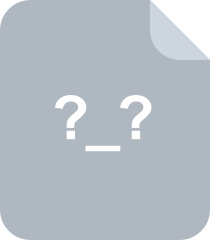
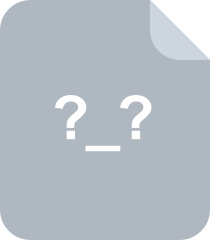
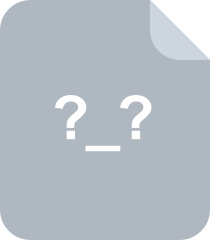
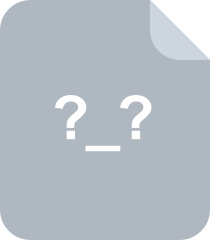
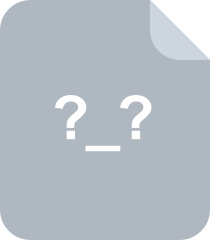
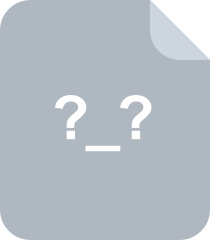
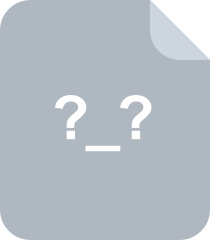
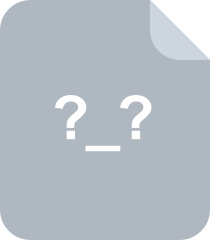
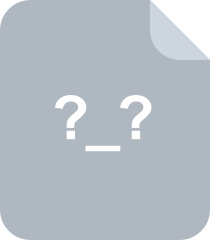
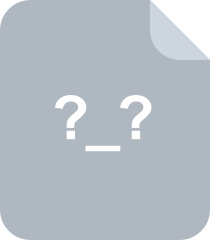
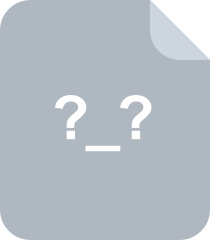
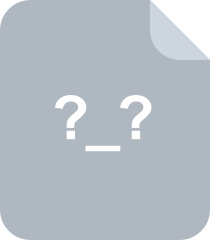
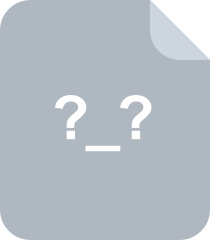
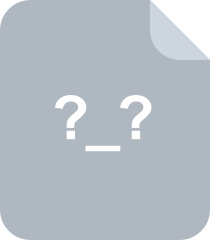
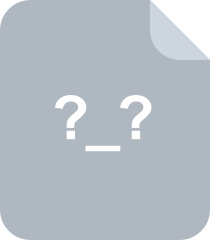
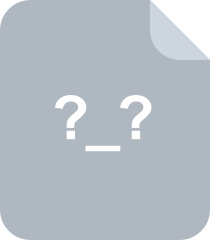
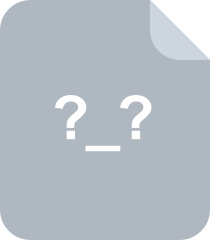
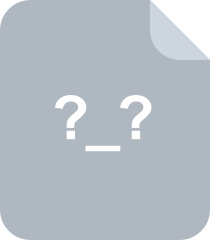
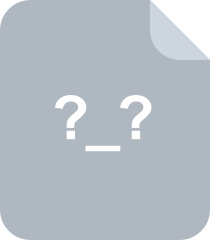
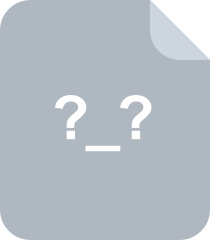
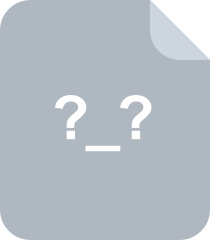
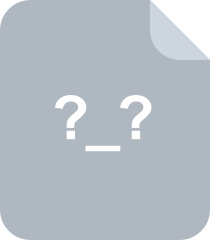
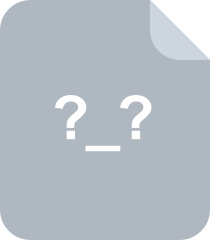
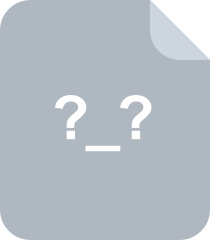
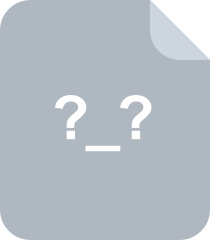
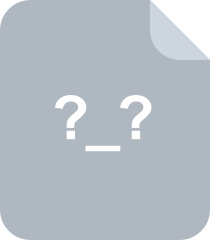
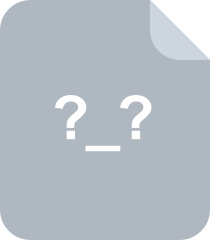
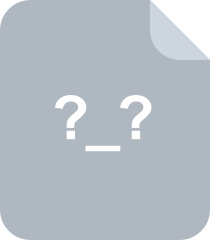
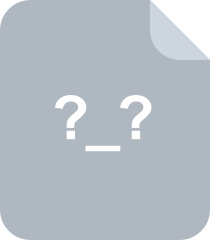
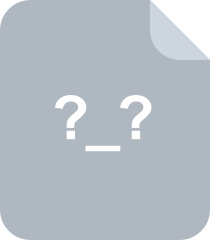
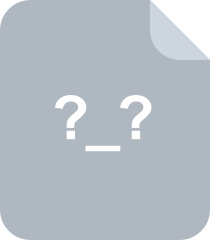
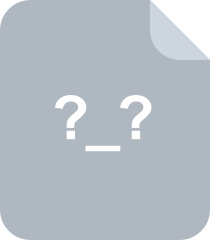
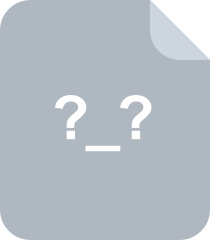
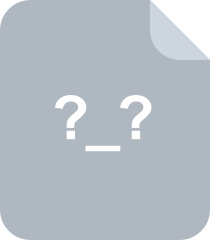
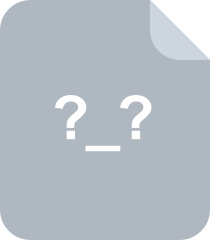
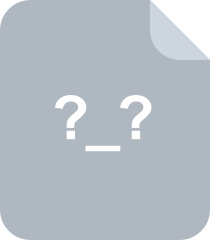
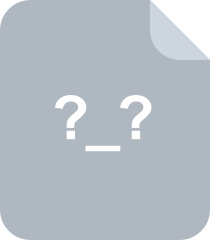
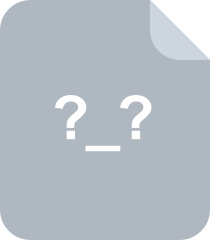
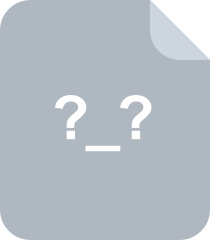
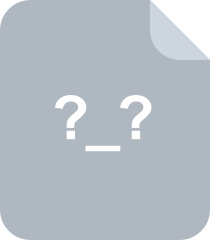
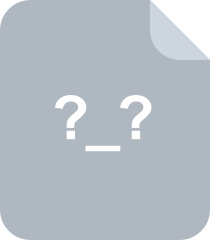
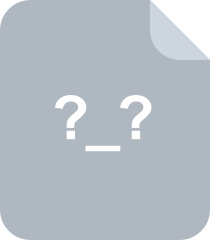
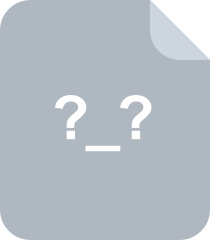
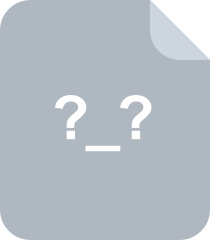
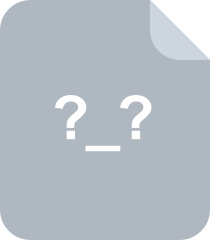
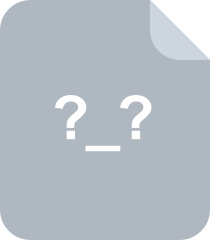
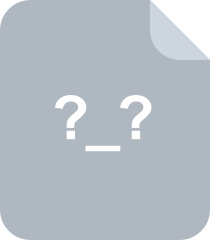
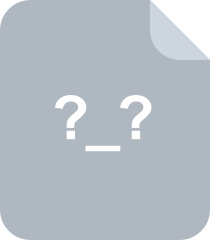
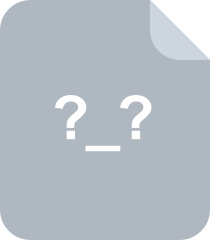
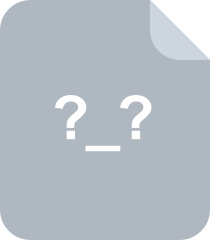
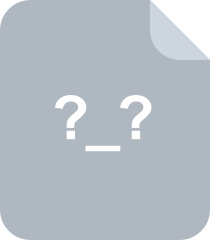
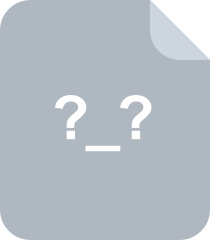
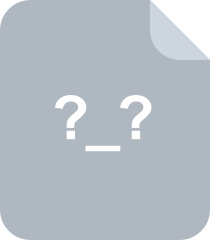
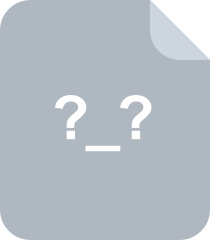
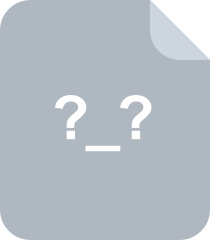
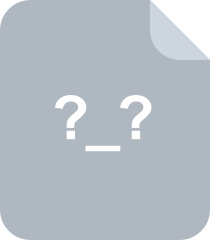
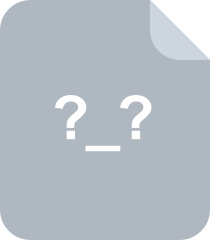
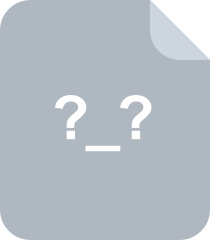
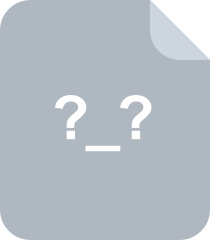
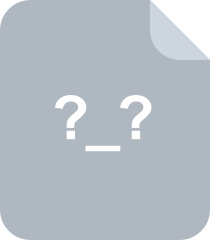
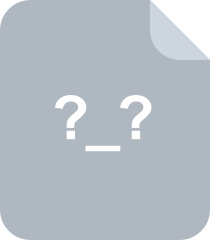
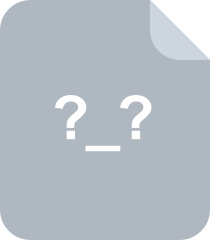
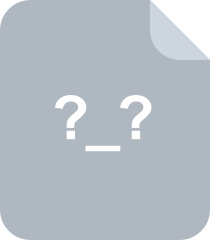
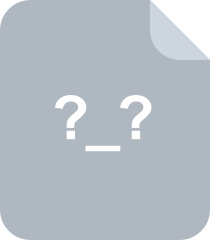
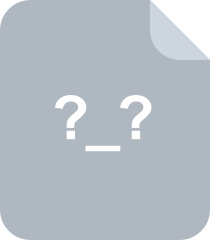
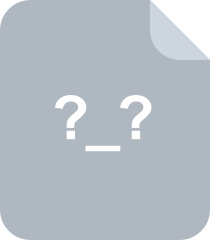
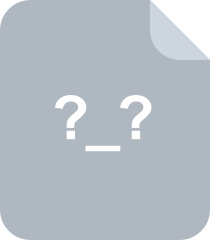
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
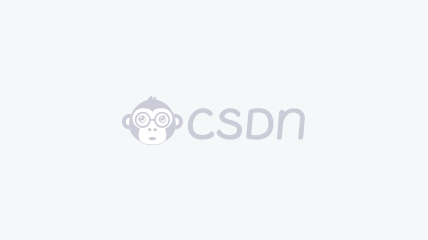

热爱技术。
- 粉丝: 2501
- 资源: 7862
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

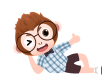
最新资源
- matplotlib图例指南.pdf
- C#ASP.NET精美企业网站后台管理系统源码数据库 SQL2008源码类型 WebForm
- 基于大数据的二手房可视化分析与预测系统
- 基于大语言模型和 RAG 的知识库问答系统 开箱即用、模型中立、灵活编排,支持快速嵌入到第三方业务系统
- 线性回归Tensorflow实现
- lvgl源码-8.2版本
- java浏览器(使用VSCode)
- PHPThinkphp+Vue2.0前后端分离框架通用后台源码数据库 MySQL源码类型 WebForm
- 构建一个大模型训练、微调、评估、推理、部署的全流程开发套件,基于MindSpore内置的并行技术和组件化设计
- 低噪声放大器LNA天线一体化设计-ADS CST工程
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


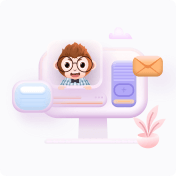
安全验证
文档复制为VIP权益,开通VIP直接复制
