**Ajax调用WCF服务详解** 在Web开发中,Asynchronous JavaScript and XML(Ajax)技术被广泛用于实现页面的无刷新更新,提高了用户体验。而Windows Communication Foundation(WCF)是微软提供的一种强大的服务导向架构,用于构建分布式系统。本文将详细讲解如何使用Ajax以GET和POST方式调用WCF服务,包括带参数和不带参数的情况。 我们需要理解Ajax的基本原理。Ajax通过JavaScript创建XMLHttpRequest对象,然后利用这个对象与服务器进行异步通信。对于GET和POST两种HTTP方法,它们的主要区别在于: 1. **GET方式**:在URL中附加参数,数据可见且有限制(一般不超过2KB),适合获取少量数据。 2. **POST方式**:数据放在请求体中,对数据量无限制,适合传递大量或敏感数据。 在调用WCF服务时,我们需要创建一个WCF服务接口和实现类。例如,定义一个简单的服务接口`IService1`: ```csharp [ServiceContract] public interface IService1 { [OperationContract] [WebInvoke(Method = "GET", UriTemplate = "GetData?value={value}")] string GetData(string value); [OperationContract] [WebInvoke(Method = "POST", RequestFormat = WebMessageFormat.Json, UriTemplate = "PostData")] string PostData(MyData data); } ``` 其中,`GetData`方法使用GET方式,接收一个字符串参数;`PostData`方法使用POST方式,接收一个自定义类型`MyData`的数据。 接下来,我们讨论如何使用Ajax进行调用: ### GET方式调用 对于GET方式,我们可以使用jQuery的`$.ajax`或者`$.get`方法。假设WCF服务地址为`http://localhost/Service1.svc`,调用如下: ```javascript $.ajax({ type: "GET", url: "http://localhost/Service1.svc/GetData", data: { value: "test" }, success: function (response) { console.log(response); }, error: function (xhr, status, error) { console.error("Error:", error); } }); ``` ### POST方式调用 对于POST方式,我们通常会发送JSON格式的数据。同样使用jQuery的`$.ajax`方法: ```javascript var data = { value: "test", anotherField: "anotherValue" }; $.ajax({ type: "POST", url: "http://localhost/Service1.svc/PostData", contentType: "application/json; charset=utf-8", data: JSON.stringify(data), dataType: "json", success: function (response) { console.log(response); }, error: function (xhr, status, error) { console.error("Error:", error); } }); ``` 这里的`contentType`指定了数据格式,`dataType`指定了期望的响应格式。`JSON.stringify()`用于将JavaScript对象转换为JSON字符串。 以上就是使用Ajax以GET和POST方式调用WCF服务的基本步骤。实际应用中,还需要考虑跨域问题、错误处理、数据验证等复杂情况。在`WcfTest.zip`和`yfm.zip`文件中可能包含了示例代码和更详细的教程,可以进一步学习和实践。在开发过程中,结合Visual Studio、Fiddler等工具,可以帮助更好地理解和调试WCF服务的调用过程。
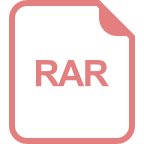
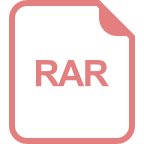
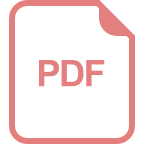
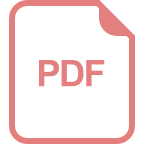
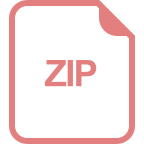
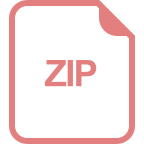
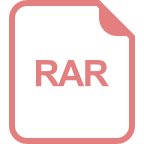
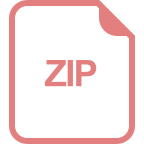
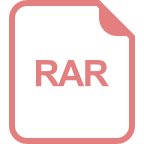
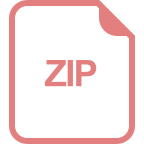
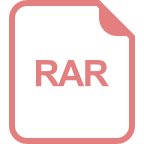
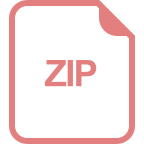
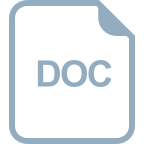

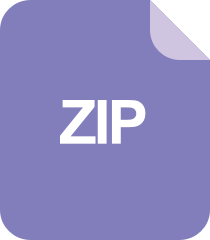
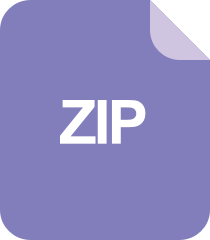
- 1
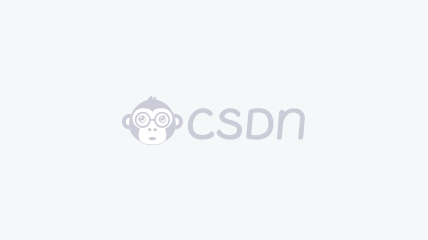
- 尧曦2019-02-19wcf引用坏了 只能http post也不好用
- 计算机码农2018-08-31psot提交获取不到数据

- 粉丝: 31
- 资源: 22
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

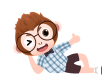
最新资源
- 章节1:Python入门视频
- 无需样板的 Python 类.zip
- ESP32 : 32-bit MCU & 2.4 GHz Wi-Fi & BT/BLE SoCs
- 博物馆文博资源库-JAVA-基于springBoot博物馆文博资源库系统设计与实现
- 旅游网站-JAVA-springboot+vue的桂林旅游网站系统设计与实现
- 小说网站-JAVA-基于springBoot“西贝”小说网站的设计与实现
- 游戏分享网站-JAVA-基于springBoot“腾达”游戏分享网站的设计与实现
- 学习交流-JAVA-基于springBoot“非学勿扰”学习交流平台设计与实现
- EDAfloorplanning
- 所有课程均提供 Python 复习部分.zip

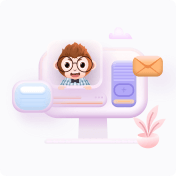
