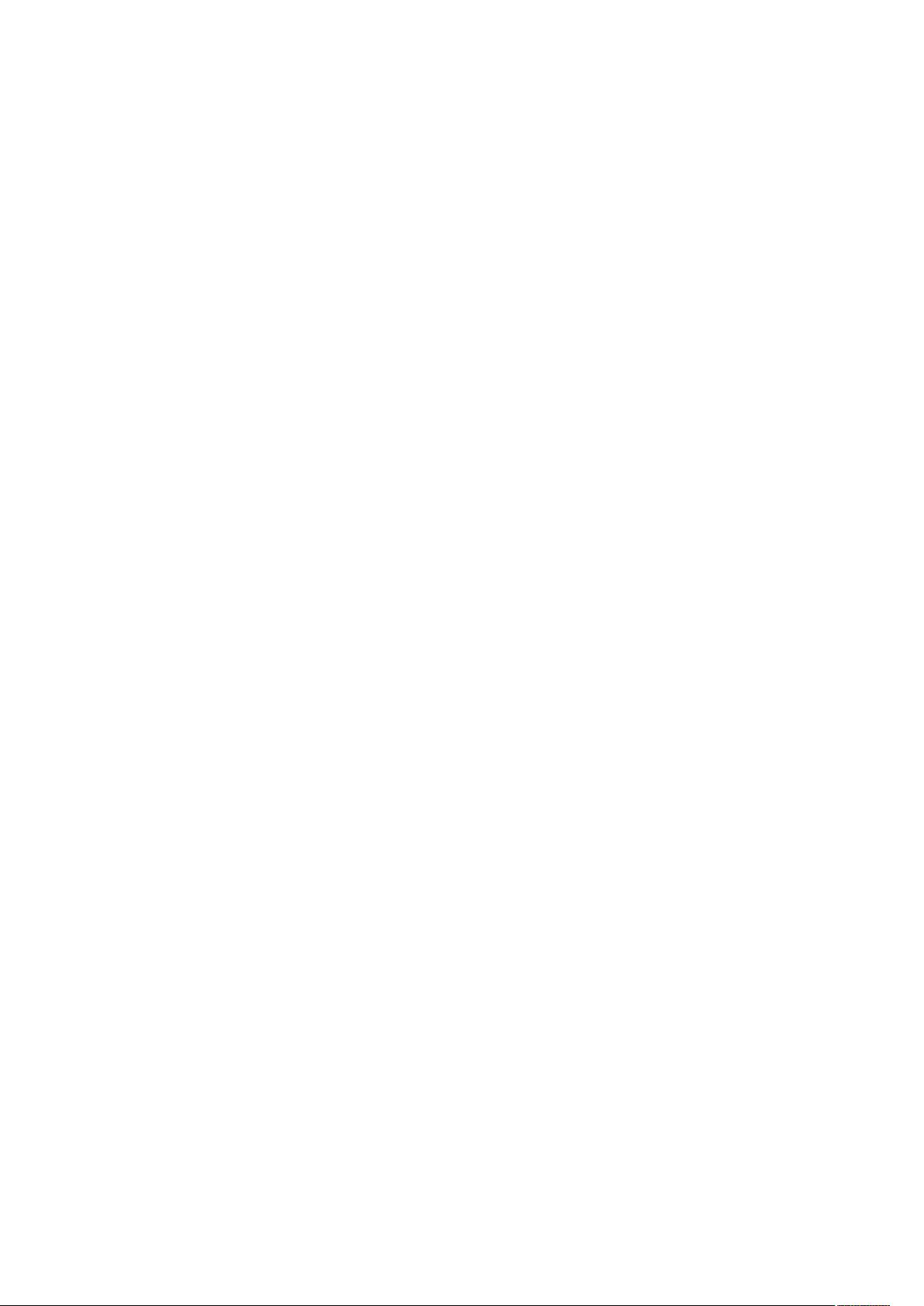
当然,我可以为您详细解释 Java 中快速排序算法的实现,并提供一个代码示例。快速排序
是一种高效的排序算法,基于分治策略。其基本步骤如下:
1. 选择一个基准元素(pivot),通常选择数组的第一个元素或者最后一个元素,但也有其他
选取策略如“三数取中”等。
2. 重新排列数组,使得所有小于基准元素的元素移动到基准的左侧,大于基准的元素移动
到基准的右侧,这个过程称为分区操作。
3. 对基准左右两侧的两个子数组递归地执行以上两步。
以下是一个简单的 Java 代码实现快速排序:
```java
public class QuickSort {
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
// Partitioning index
int pivotIndex = partition(arr, low, high);
// Recursively sort elements before and after the pivot
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
private static int partition(int[] arr, int low, int high) {
// Choose the rightmost element as pivot
int pivot = arr[high];
int i = (low - 1); // Index of smaller element
for (int j = low; j < high; j++) {
// If current element is smaller than or equal to pivot
if (arr[j] <= pivot) {
i++;
// Swap arr[i] and arr[j]
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
// Swap arr[i+1] and arr[high] (or pivot)
int temp = arr[i + 1];
arr[i + 1] = arr[high];