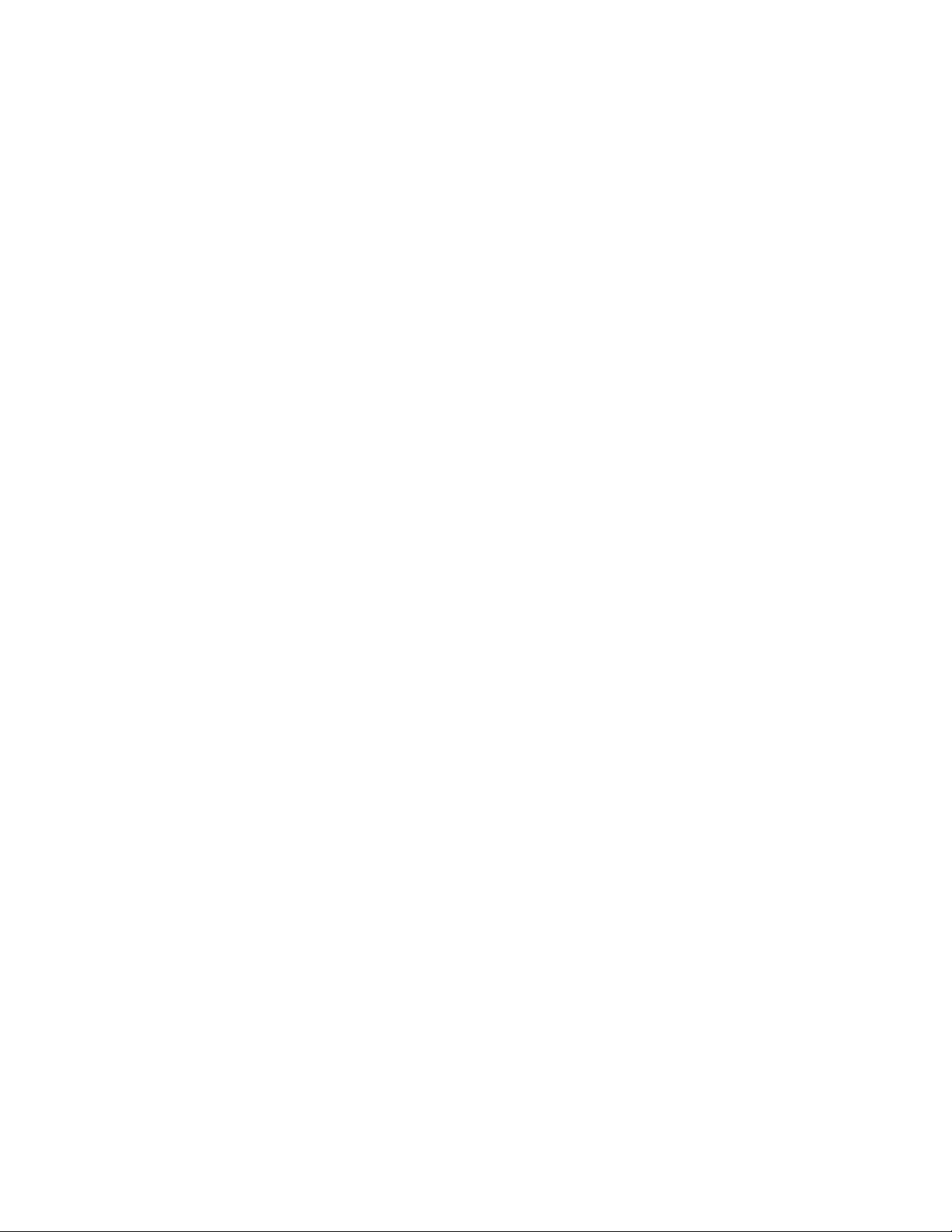
CONTENTS
2.1 The Two Purposes of Routing . . . . . . . . . . . . . . . . . . . . 49
2.2 The routes.rb File . . . . . . . . . . . . . . . . . . . . . . . . . . . . 50
2.3 Route Globbing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 63
2.4 Named Routes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 65
2.5 Scoping Routing Rules . . . . . . . . . . . . . . . . . . . . . . . . . 71
2.6 Listing Routes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 75
2.7 Conclusion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 76
3. REST, Resources, and Rails ........................ 77
3.1 REST in a Rather Small Nutshell . . . . . . . . . . . . . . . . . . 78
3.2 Resources and Representations . . . . . . . . . . . . . . . . . . . 80
3.3 REST in Rails . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 81
3.4 Routing and CRUD . . . . . . . . . . . . . . . . . . . . . . . . . . . 82
3.5 The Standard RESTful Controller Actions . . . . . . . . . . . . . 86
3.6 Singular Resource Routes . . . . . . . . . . . . . . . . . . . . . . . 91
3.7 Nested Resources . . . . . . . . . . . . . . . . . . . . . . . . . . . 92
3.8 Routing Concerns . . . . . . . . . . . . . . . . . . . . . . . . . . . . 98
3.9 RESTful Route Customizations . . . . . . . . . . . . . . . . . . . . 99
3.10 Controller-Only Resources . . . . . . . . . . . . . . . . . . . . . . 104
3.11 Different Representations of Resources . . . . . . . . . . . . . . 107
3.12 The RESTful Rails Action Set . . . . . . . . . . . . . . . . . . . . . 110
3.13 Conclusion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 116
4. Working with Controllers .........................117
4.1 Rack . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 118
4.2 Action Dispatch: Where It All Begins . . . . . . . . . . . . . . . . 122
4.3 Render unto View… . . . . . . . . . . . . . . . . . . . . . . . . . . 126
4.4 Additional Layout Options . . . . . . . . . . . . . . . . . . . . . . 140
4.5 Redirecting . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 141
4.6 Controller/View Communication . . . . . . . . . . . . . . . . . . . 146
4.7 Action Callbacks . . . . . . . . . . . . . . . . . . . . . . . . . . . . 147
4.8 Streaming . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 153
4.9 Variants . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 161
4.10 Conclusion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 163
5. Working with Active Record .......................164
5.1 The Basics . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 165
5.2 Macro-Style Methods . . . . . . . . . . . . . . . . . . . . . . . . . 167
5.3 Defining Attributes . . . . . . . . . . . . . . . . . . . . . . . . . . . 170
5.4 CRUD: Creating, Reading, Updating, Deleting . . . . . . . . . . 173
5.5 Database Locking . . . . . . . . . . . . . . . . . . . . . . . . . . . . 188
5.6 Querying . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 192
5.7 Ignoring Columns . . . . . . . . . . . . . . . . . . . . . . . . . . . . 214