<?php
error_reporting(E_ALL);
//
// CONFIGURABLE OPTIONS
//
$_config = array
(
'url_var_name' => 'q',
'flags_var_name' => 'hl',
'get_form_name' => '____pgfa',
'basic_auth_var_name' => '____pbavn',
'max_file_size' => -1,
'allow_hotlinking' => 0,
'upon_hotlink' => 1,
'compress_output' => 0
);
$_flags = array
(
'include_form' => 1,
'remove_scripts' => 1,
'accept_cookies' => 1,
'show_images' => 1,
'show_referer' => 1,
'rotate13' => 0,
'base64_encode' => 1,
'strip_meta' => 1,
'strip_title' => 0,
'session_cookies' => 1
);
$_frozen_flags = array
(
'include_form' => 0,
'remove_scripts' => 0,
'accept_cookies' => 0,
'show_images' => 0,
'show_referer' => 0,
'rotate13' => 0,
'base64_encode' => 0,
'strip_meta' => 0,
'strip_title' => 0,
'session_cookies' => 0
);
$_labels = array
(
'include_form' => array('TOP代理', '在您要浏览的页面,包括一个小的代理TOP'),
'remove_scripts' => array('屏蔽JS', '屏蔽类似于 Javascript的脚本 (i.e. Javascript)'),
'accept_cookies' => array('接受Cookies', '接受 HTTP cookies'),
'show_images' => array('显示图像', '允许显示图像'),
'show_referer' => array('显示Referer', '显示浏览的网站'),
'rotate13' => array('Rotate13', '使用 rotate13 加密引擎加密URL'),
'base64_encode' => array('Base64', '使用 base64 加密引擎加密URL'),
'strip_meta' => array('屏蔽标签', '屏蔽要浏览页面的Meta标签'),
'strip_title' => array('屏蔽标题', '屏蔽要浏览页面的标题'),
'session_cookies' => array('存储Cookies', '存储浏览的 cookies')
);
$_hosts = array
(
'#^127\.|192\.168\.|10\.|172\.(1[6-9]|2[0-9]|3[01])\.|localhost#i'
);
$_hotlink_domains = array();
$_insert = array();
//
// END CONFIGURABLE OPTIONS. The ride for you ends here. Close the file.
//
$_iflags = '';
$_system = array
(
'ssl' => extension_loaded('openssl') && version_compare(PHP_VERSION, '4.3.0', '>='),
'uploads' => ini_get('file_uploads'),
'gzip' => extension_loaded('zlib') && !ini_get('zlib.output_compression'),
'stripslashes' => get_magic_quotes_gpc()
);
$_proxify = array('text/html' => 1, 'application/xml+xhtml' => 1, 'application/xhtml+xml' => 1, 'text/css' => 1);
$_version = 'Powered by oroa.cn V 0.1';
$_http_host = isset($_SERVER['HTTP_HOST']) ? $_SERVER['HTTP_HOST'] : (isset($_SERVER['SERVER_NAME']) ? $_SERVER['SERVER_NAME'] : 'localhost');
$_script_url = 'http' . ((isset($_ENV['HTTPS']) && $_ENV['HTTPS'] == 'on') || $_SERVER['SERVER_PORT'] == 443 ? 's' : '') . '://' . $_http_host . ($_SERVER['SERVER_PORT'] != 80 && $_SERVER['SERVER_PORT'] != 443 ? ':' . $_SERVER['SERVER_PORT'] : '') . $_SERVER['PHP_SELF'];
$_script_base = substr($_script_url, 0, strrpos($_script_url, '/')+1);
$_url = '';
$_url_parts = array();
$_base = array();
$_socket = null;
$_request_method = $_SERVER['REQUEST_METHOD'];
$_request_headers = '';
$_cookie = '';
$_post_body = '';
$_response_headers = array();
$_response_keys = array();
$_http_version = '';
$_response_code = 0;
$_content_type = 'text/html';
$_content_length = false;
$_content_disp = '';
$_set_cookie = array();
$_retry = false;
$_quit = false;
$_basic_auth_header = '';
$_basic_auth_realm = '';
$_auth_creds = array();
$_response_body = '';
//
// FUNCTION DECLARATIONS
//
function show_report($data)
{
include $data['which'] . '.inc.php';
exit(0);
}
function add_cookie($name, $value, $expires = 0)
{
return rawurlencode(rawurlencode($name)) . '=' . rawurlencode(rawurlencode($value)) . (empty($expires) ? '' : '; expires=' . gmdate('D, d-M-Y H:i:s \G\M\T', $expires)) . '; path=/; domain=.' . $GLOBALS['_http_host'];
}
function set_post_vars($array, $parent_key = null)
{
$temp = array();
foreach ($array as $key => $value)
{
$key = isset($parent_key) ? sprintf('%s[%s]', $parent_key, urlencode($key)) : urlencode($key);
if (is_array($value))
{
$temp = array_merge($temp, set_post_vars($value, $key));
}
else
{
$temp[$key] = urlencode($value);
}
}
return $temp;
}
function set_post_files($array, $parent_key = null)
{
$temp = array();
foreach ($array as $key => $value)
{
$key = isset($parent_key) ? sprintf('%s[%s]', $parent_key, urlencode($key)) : urlencode($key);
if (is_array($value))
{
$temp = array_merge_recursive($temp, set_post_files($value, $key));
}
else if (preg_match('#^([^\[\]]+)\[(name|type|tmp_name)\]#', $key, $m))
{
$temp[str_replace($m[0], $m[1], $key)][$m[2]] = $value;
}
}
return $temp;
}
function url_parse($url, & $container)
{
$temp = @parse_url($url);
if (!empty($temp))
{
$temp['port_ext'] = '';
$temp['base'] = $temp['scheme'] . '://' . $temp['host'];
if (isset($temp['port']))
{
$temp['base'] .= $temp['port_ext'] = ':' . $temp['port'];
}
else
{
$temp['port'] = $temp['scheme'] === 'https' ? 443 : 80;
}
$temp['path'] = isset($temp['path']) ? $temp['path'] : '/';
$path = array();
$temp['path'] = explode('/', $temp['path']);
foreach ($temp['path'] as $dir)
{
if ($dir === '..')
{
array_pop($path);
}
else if ($dir !== '.')
{
for ($dir = rawurldecode($dir), $new_dir = '', $i = 0, $count_i = strlen($dir); $i < $count_i; $new_dir .= strspn($dir{$i}, 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789$-_.+!*\'(),?:@&;=') ? $dir{$i} : rawurlencode($dir{$i}), ++$i);
$path[] = $new_dir;
}
}
$temp['path'] = str_replace('/%7E', '/~', '/' . ltrim(implode('/', $path), '/'));
$temp['file'] = substr($temp['path'], strrpos($temp['path'], '/')+1);
$temp['dir'] = substr($temp['path'], 0, strrpos($temp['path'], '/'));
$temp['base'] .= $temp['dir'];
$temp['prev_dir'] = substr_count($temp['path'], '/') > 1 ? substr($temp['base'], 0, strrpos($temp['base'], '/')+

大黄鸭duck.
- 粉丝: 6762
- 资源: 1万+
最新资源
- 网络分析中最短路径的计算Matlab代码.rar
- 系数加权小波相干分析CW-WTC是WTC的优化,用于在最大显著性和设置周期范围内提取两个序列之间的时变响应周期和时滞特征matlab代码.rar
- 无损失二次系统的有界性分析 matlab代码.rar
- 药物通过表面侵蚀释放。PDE系统采用线性方法求解 matlab代码.rar
- 一个简单的MATLAB函数,用于绘制两到四个具有可选标签的集合的venn图.rar
- 系统性地同时优化一系列目标函数的过程,也被称为矢量优化Matlab代码.rar
- 一种计算矩形通道层流有效滑移长度的工具,matlab代码.rar
- 一级欠驱动机械系统的互连和阻尼分配无源控制(VITOL动力学)matlab代码.rar
- 应用于跟踪卫星星座轨道和规划优化轨道机动 matlab代码.rar
- 用于估计部分信息分解(PID)项的Matlab工具箱.rar
- 用于估计互信息率(MIR)及其分解度量的Matlab工具箱 matlab代码.rar
- 用于根据自行车模型计算车辆的偏航力矩图和相图 matlab代码.rar
- 用于抗丢失数据轴承故障诊断的快速频率稀疏学习方法”的MATLAB代码 matlab代码.rar
- 用于模拟所提出的移动人类自组网模型,以模拟基于空气传播的传染病传播matlab代码.rar
- 用于可视化pharlap光线追踪结果的Matlab方法.rar
- 用于生物制造的病毒转导和繁殖的模拟 (2) matlab代码.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


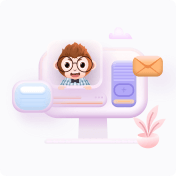