// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT license.
#pragma once
#include "seal/util/common.h"
#include "seal/util/defines.h"
#include "seal/util/pointer.h"
#include <algorithm>
#include <cstddef>
#include <cstdint>
#include <iostream>
#include <iterator>
#include <stdexcept>
#include <tuple>
#include <type_traits>
#include <utility>
#include <vector>
namespace seal
{
class Ciphertext;
namespace util
{
class NTTTables;
/**
@par PolyIter, RNSIter, and CoeffIter
In this file we define a set of custom iterator classes ("SEAL iterators") that are used throughout Microsoft
SEAL for easier iteration over ciphertext polynomials, their RNS components, and the coefficients in the RNS
components. All SEAL iterators satisfy the C++ LegacyRandomAccessIterator requirements. SEAL iterators are ideal
to use with the SEAL_ITERATE macro, which expands to std::for_each_n in C++17 and to seal::util::seal_for_each_n
in C++14. All SEAL iterators derive from SEALIterBase.
The most important SEAL iterator classes behave as illustrated by the following diagram:
+-------------------+
| Pointer & Size | Construct +-----------------+
| or Ciphertext |------------>| (Const)PolyIter | Iterates over RNS polynomials in a ciphertext
+-------------------+ +--------+--------+ (coeff_modulus_size-many RNS components)
|
|
| Dereference
|
|
v
+----------------+ Construct +----------------+
| Pointer & Size |------------->| (Const)RNSIter | Iterates over RNS components in an RNS polynomial
+----------------+ +-------+--------+ (poly_modulus_degree-many coefficients)
|
|
| Dereference
|
|
v
+----------------+ Construct +------------------+
| Pointer & Size |------------>| (Const)CoeffIter | Iterates over coefficients (std::uint64_t) in a single
+----------------+ +---------+--------+ RNS polynomial component
|
|
| Dereference
|
|
v
+-------------------------+
| (const) std::uint64_t & |
+-------------------------+
@par PtrIter and StrideIter
PtrIter<T *> and StrideIter<T *> are both templated SEAL iterators that wrap raw pointers. The difference
between these two types is that advancing PtrIter<T *> always advances the wrapped pointer by one, whereas the
step size (stride) can be set to be anything for a StrideIter<T *>. CoeffIter is a typedef of
PtrIter<std::uint64_t *> and and RNSIter is almost the same as StrideIter<std::uint64_t *>, but still a
different type.
+----------+ Construct +-------------------+
| MyType * |------------->| PtrIter<MyType *> | Simple wrapper for raw pointers
+----------+ +----+----------+---+
| |
| |
Dereference | | PtrIter<MyType *>::ptr()
| | or implicit conversion
| |
v v
+----------+ +----------+
| MyType & | | MyType * |
+----------+ +----------+
+----------+ Construct +----------------------+
| MyType * |------------->| StrideIter<MyType *> | Simple wrapper for raw pointers with custom stride size
+----------+ +-----+----------+-----+
| |
| |
Dereference | | StrideIter<MyType *>::ptr()
| | or implicit conversion
| |
v v
+----------+ +----------+
| MyType & | | MyType * |
+----------+ +----------+
@par IterTuple
An extremely useful template class is the (variadic) IterTuple<...> that allows multiple SEAL iterators to be
zipped together. An IterTuple is itself a SEAL iterator and nested IterTuple types are used commonly in the
library. Dereferencing an IterTuple always yields an std::tuple, with each IterTuple element dereferenced.
Since an IterTuple can be constructed from an std::tuple holding the respective single-parameter constructor
arguments for each iterator, the dereferenced std::tuple can often be directly passed on to functions expecting
an IterTuple.
The individual components of an IterTuple can be accessed with the seal::util::get<i>(...) functions. The
behavior of IterTuple is summarized in the following diagram:
+-----------------------------------------+
| IterTuple<PolyIter, RNSIter, CoeffIter> |
+--------------------+--------------------+
|
|
| Dereference
|
|
v
+--------------------------------------------------+
| std::tuple<RNSIter, CoeffIter, std::uint64_t &>> |
+------+-------------------+-------------------+---+
| | |
| | |
| std::get<0> | std::get<1> | std::get<2>
| | |
| | |
v v v
+-------------+ +---------------+ +-----------------+
| RNSIter | | CoeffIter | | std::uint64_t & |
+-------------+ +---------------+ +-----------------+
Sometimes we have to use multiple nested iterator tuples. In this case accessing the nested iterators can be
tedious with nested get<...> calls. Consider the following, where encrypted1 and encrypted2 are Ciphertexts
and destination is either a Ciphertext or a PolyIter:
IterTuple<PolyIter, PolyIter> I(encrypted1, encrypted2);
IterTuple<decltype(I), PolyIter> J(I, destination);
auto encrypted1_iter = get<0>(get<0>(J));
auto encrypted2_iter = get<1>(get<0>(J));
An easier way is to use another form of get<...> that accepts multiple indices and accesses the structure in a
nested manner. For example, in the above we could also write:
auto encrypted1_iter = get<0, 0>(J));
auto encr

azh-1415926
- 粉丝: 17
- 资源: 27
最新资源
- 汇川H5U搭配IT7070系列PLC模块化程序:功能齐全,人性化设计,提高生产调试效率与设备操作体验,汇川H5U搭配汇川IT7070系列案例程序,可做为模板程序使用 PL程序可以直接与触摸屏进行离线仿
- "非隔离双向DC-DC变换器(Buck-Boost转换器)的仿真研究:电压外环与电流内环双闭环控制下的充电与放电特性分析 - 基于Matlab Simulink模型",非隔离双向DC DC变器 buc
- 汇川AM系列程序与全自动N95口罩机:高级编程、精准控制与系统整合,汇川AM401系列程序 汇川AM403程序,搭配汇川总线伺服,汇川IT7070系列触摸屏 全自动N95口罩机 大型程序近20000步
- 基于MATLAB Simulink R2015b的太阳能光伏MPPT控制蓄电池充电仿真模型,采用扰动观测法实现高效充电控制,附详细仿真说明文档,59C.Solar-Charge-Controller:
- MATLAB研究:基于石川算法求解齿轮时变啮合刚度与齿面接触变形量分析,齿轮动力学图谱解析及故障诊断学习资料,MATLAB:考虑齿面接触变形量,基于石川算法求解齿轮时变啮合刚度,齿轮动力学时域图、相图
- MATLAB Simulink模拟:基于下垂控制的光储直流微电网离网运行控制的Vf与交流负载控制策略,MATLAB Simukink基于下垂控制的光储直流微电网离网运行控制 关键字:离网;直流下垂;交
- 永磁同步旋转电机发电并网控制仿真模型详解:包含PMSG、整流桥、逆变桥及双闭环PI控制策略讲解,永磁同步旋转电机发电并网控制仿真模型(可讲解) 联系本链接包括以下部分: 1. 仿真中含永磁同步发电机(
- 《深入探讨3、5自由度座椅悬架系统:模型构建、仿真研究与文献综述》,5自由度座椅悬架: 详情请csdn搜索博客:3、5自由度座椅悬架,以及5自由度座椅人体悬架仿真研究 模型保证正确架构清晰有对应参考文
- 局部遮阴条件下光伏MPPT的粒子群优化算法仿真模型研究,局部遮阴下光伏MPPT-粒子群算法,仿真模型 ,核心关键词:局部遮阴下光伏MPPT; 粒子群算法; 仿真模型;,局部遮阴光伏MPPT优化:粒子
- 光伏MPPT仿真:电导增量法模型详解与Video explanation(含自建光伏电池替换功能),光伏MPPT仿真-电导增量法,仿真模型,可替自建光伏电池,有Video explanation(原创
- 【线性二次型最优控制目标函数下的被动与主动悬架模型研究】,【被动 LQR主动悬架模型】 采用LQR控制的主动悬架模型,选取车身加速度、悬架动挠度等参数构造线性二次型最优控制目标函数 输
- 微电网三相交流下垂控制:传统阻感型输出有功、无功与频率波形的深度解析,微电网,下垂控制(三相交流) 传统阻感型下垂控制输出有功 无功 频率波形 ,核心关键词:微电网; 下垂控制(三相交流); 传
- "创新LD孤岛微电网二次控制策略:下垂控制结合动态事件触发实现有功功率均分与异步通信一致性处理",创新,LD,孤岛微电网二次控制,下垂控制,动态事件触发,实现了二次控制,达成了有功功率均分,处理异步通
- MATLAB模拟:分布式电源(如光伏、风机)接入对节点电压与系统网损影响的多维度分析比较研究 ,MATLAB程序-分布式电源(光伏风机等DG)接入对节点电压(或系统网损)的影响,对比了不同容量DG、不
- 粉床数值模拟:SLM增材制造选区激光熔化技术与软件详解,涵盖模型建立、模拟流程与热通量分析,slm 增材制造选区激光熔化SLM的粉床数值模拟 备注:资料一直在更新,不断完善,尽可能把所有的内容讲详细
- 基于Matlab的语音识别技术:利用GMM和MFCC识别说话内容与说话人,训练集与测试集详解,Matlab语音识别,识别说话内容、识别说话人等,使用GMM和MFCC,有训练集和测试集,带说明等
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


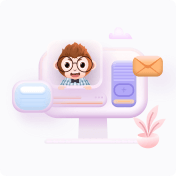