#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <math.h>
#include <time.h>
#include <Windows.h>
#include "unistd.h"
#define Max_Size 20 //最大允许的地图大小
#define TimeInterval 25 //预定的等待时间间隔,可更改
#define ChangeColor_Purple printf("\033[35m")//将颜色换成紫色
#define ChangeColor_Green_ printf("\033[32m")//将颜色换成绿色
#define ChangeColor_Red___ printf("\033[31m")//将颜色换成红色
#define ChangeColor_Yellow printf("\033[33m")//将颜色换成黄色
#define ChangeColor_Whilt_ printf("\033[0m") //将颜色换成白色
_Bool Mode = 0;//用户选择的模拟模式,A为0,B为1
int X_In = 0, Y_In = 0;//入口的坐标
int X_Out = 0, Y_Out = 0;//出口的坐标
///注意,目前仅限于模拟出入口在地图边界且唯一的情况,且仅限于一只无智力随机游走的蚂蚁
///注意,程序并不会自动判断地图是否含有通路
void Start();
void InputTheMap(char M[Max_Size][Max_Size], int width, int height, int lineNeedClean);
void CleanTheScreen();
void Imitate(char M[Max_Size][Max_Size], int width, int height);
void Output(char M[Max_Size][Max_Size], int width, int height);
int main()
{
Start();
if (Mode)//选择了自行输入
{
int LineUsed = 0;
WrongInputSize:{//如果输入的大小超出所允许的范围,则跳回这里
int Width = 0, Height = 0;
char Map[Max_Size][Max_Size] = { " " };
//以下内容提示用户输入所需地图的长宽
ChangeColor_Purple; printf(">");
ChangeColor_Green_; printf("Please input the size of the map:\n");
ChangeColor_Purple; printf("*");
ChangeColor_Green_; printf("Width=");
ChangeColor_Whilt_; scanf("%d", &Width);//读取宽度
ChangeColor_Purple; printf("*");
ChangeColor_Green_; printf("Height=");
ChangeColor_Whilt_; scanf("%d", &Height);//读取高度
LineUsed += 3;
if (Width > 2 && Width <= Max_Size && Height > 2 && Height <= Max_Size)
{
InputTheMap(Map, Width, Height, LineUsed);//输入完整地图
//接下来开始进行模拟
}
else {
ChangeColor_Purple; printf(">");
ChangeColor_Red___; printf("You should input between 3 to %d.Please try again.\n", Max_Size);
LineUsed++;
ChangeColor_Whilt_; goto WrongInputSize;//提示用户输入的数据不符合实际要求
}
}
}
else {//选择使用内置的10*10地图
char Map[Max_Size][Max_Size] = { {'#','*','#','#','#','#','#','#','#','#'},
{'#',' ',' ',' ','#',' ',' ',' ',' ','#'},
{'#',' ','#',' ','#',' ','#','#',' ','#'},
{'#',' ','#',' ',' ',' ','#',' ',' ','#'},
{'#',' ',' ',' ',' ',' ','#',' ','#','#'},
{'#','#',' ','#',' ',' ',' ',' ','#','#'},
{'#','#',' ','#','#',' ','#',' ',' ','#'},
{'#',' ',' ',' ','#',' ','#','#',' ','#'},
{'#','#',' ',' ',' ',' ',' ','#',' ','#'},
{'#','#','#','#','#','#','#','#','!','#'}};//自带地图
X_In = 1;
Y_In = 0;
X_Out = 8;
Y_Out = 9;
CleanTheScreen();//清空屏幕
Imitate(Map, 10, 10);
}
return 0;
}
void Start()
{
char ChooseTheMode = ' ';
ChangeColor_Purple; printf(">");
ChangeColor_Green_; printf("Please input the mode you want(A/B):\n");
ChangeColor_Purple; printf("A.");
ChangeColor_Green_; printf("Using built-in maps\n");
ChangeColor_Purple; printf("B.");
ChangeColor_Green_; printf("Enter the map and its scale on your own\n");
ChangeColor_Whilt_; printf("\033[%d;%dH", 1, 38);//移动光标
do { ChooseTheMode = getchar(); } while (ChooseTheMode != 'A'&&ChooseTheMode != 'B');//持续读取直到读取到A或B
Mode = (_Bool)((int)ChooseTheMode - 65);//利用ASCII码把A和B转为0和1
printf("\033[H");
for (int i = 1; i <= 3; i++)
{
for (int j = 1; j <= 50; j++)
printf(" ");
printf("\n");
}
printf("\033[H");//以上代码清空屏幕
return;
}
void InputTheMap(char M[Max_Size][Max_Size], int width, int height, int lineNeedClean)
{///M是储存地图的二维数组,width与height分别表示地图实际需要的大小,lineNeedClean为需要清理的行数
TheMapInputWrong://如果用户反馈地图并非所需要的,则跳回重新开始
ChangeColor_Whilt_; printf("\033[H");
for (int i = 1; i <= lineNeedClean; i++)
{
for (int j = 1; j <= 50; j++)
printf(" ");
printf("\n");
}
printf("\033[H");
//清空屏幕
TheMapExamedWrong://被检测出有问题就跳到这里
//接下来提示用户应该如何输入地图
ChangeColor_Purple; printf(">");
ChangeColor_Green_; printf("Please read the follows carefully!\n");
ChangeColor_Purple; printf("'*'");
ChangeColor_Green_; printf(" :the enterence\n");
ChangeColor_Purple; printf("'!'");
ChangeColor_Green_; printf(" :the export\n");
ChangeColor_Purple; printf("'#'");
ChangeColor_Green_; printf(" :the wall\n");
ChangeColor_Purple; printf("' '");
ChangeColor_Green_; printf(" :the road\n");
ChangeColor_Purple; printf(">");
ChangeColor_Green_; printf("The size you input is (Width:%d,Height:%d).\n",width,height);
//提示到此为止
//接下来提示用户输入地图并对其进行读取
ChangeColor_Purple; printf("\n>");
ChangeColor_Green_; printf("You should enter as follows' format:\n");
ChangeColor_Yellow;
for (int i = 1; i <= height; i++)
{
for (int j = 1; j <= width; j++)
printf("*");
printf("\n");
}
ChangeColor_Purple; printf(">");
ChangeColor_Green_; printf("Now please input your map:\n");
ChangeColor_Whilt_;
for (int i = 0; i < width*height; i++)
{
scanf("%c", &M[i / width][i%width]);
if (M[i / width][i%width] == '\n') i--;
}//到此读取完了地图
//再次清除屏幕
ChangeColor_Whilt_; printf("\033[H");
for (int i = 1; i <= 75; i++)
{
for (int j = 1; j <= 50; j++)
printf(" ");
printf("\n");
}
printf("\033[H");
//输出确认是否为用户需要的地图
for (int i = 0; i < height; i++)
{
for (int j = 0; j < width; j++)
printf("%c", M[i][j]);
printf("\n");
}
ChangeColor_Purple; printf(">");
ChangeColor_Green_; printf("Is this the map you need?(Y/N)");
ChangeColor_Whilt_;
char TureOrFalse = ' ';
do { TureOrFalse = getchar(); } while (TureOrFalse != 'Y'&&TureOrFalse != 'N');
if (TureOrFalse == 'N')
{//再次清除屏幕
CleanTheScreen();
goto TheMapInputWrong;
}
//到这里用户已经确认完了地图
//进行地图的“初步审核”(是否符合只有一个入口和出口等)
_Bool TheMapIsAllowed = 1;//如果不符合要求就变为0且让用户重新输入
int Num_Enterence = 0, Num_Export = 0, Num_Space = 0;//记录最外层已标记的出口、入口以及空格、墙体的个数
for (int i=0;i<height;i+=height-1)//只循环第一行和最后一行
for (int j = 0; j < width; j++)
{
if (M[i][j] == '*') Num_Enterence++;
if (M[i][j] == '!') Num_Export++;
if (M[i][j] == ' ') Num_Space++;
}
for (int i=1;i<height-1;i++)//循环中间的height-2行了
for (int j = 0; j < width; j += width - 1)//只循环最两边的列
{
if (M[i][j] == '*') Num_Enterence++;
if (M[i][j] == '!') Num_Export++;
if (M[i][j] == ' ') Num_Space++;
}
if (Num_Space != 0 || Num_Enterence != 1 || Num_Export != 1)
{
TheMapIsAllowed = 0;
goto TheMapWrong;//跳过后续判断操作
}
//到此已经完成了最外层的判断
//接下来看所有符号是否符合要求
int Num_Wall = 0;
Num_Enterence = Num_Export = Num_Space = Num_Wall;//先全部归零
for (int i=0;i<height;i++)
for (int j = 0; j < width; j++)
{
if (M[i][j] == '*')
{
Num_Enterence++;
X_In = j;
Y_In = i;//记录入口的位置
}
if (M[i][j] == '!')
{
Num_Export++;
X_Out = j;
Y_Out = i;//记录出口的位置
}
if (M[i][j] == ' ') Num_Space+


青が絶える(青绝悲)
- 粉丝: 621
- 资源: 16
最新资源
- ESP8266/8285 Plane 固件
- tongue sam , 很好用的分割图形资源
- LED闪烁功能代码(基于STM32 HAL库)
- NobelSpider-爬虫
- Hooker Js-javascript
- TSP-旅行商问题TSP-旅行商问题
- CSV文件处理脚本,名为CSV-Handler.py,它提供了CSV文件的读写、数据清洗和转换等功能,适用于各种数据交换场景
- 3333333333333
- 【Unity风格化卡通渲染插件】Flat Kit: Toon Shading and Water
- 3D目标检测跟踪-基于kitti+waymo数据集的自动驾驶场景的3D目标检测+跟踪渲染可视化.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


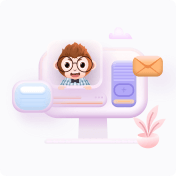