package com.system.po;
import java.util.ArrayList;
import java.util.List;
public class CourseExample {
protected String orderByClause;
protected boolean distinct;
protected List<Criteria> oredCriteria;
public CourseExample() {
oredCriteria = new ArrayList<Criteria>();
}
public void setOrderByClause(String orderByClause) {
this.orderByClause = orderByClause;
}
public String getOrderByClause() {
return orderByClause;
}
public void setDistinct(boolean distinct) {
this.distinct = distinct;
}
public boolean isDistinct() {
return distinct;
}
public List<Criteria> getOredCriteria() {
return oredCriteria;
}
public void or(Criteria criteria) {
oredCriteria.add(criteria);
}
public Criteria or() {
Criteria criteria = createCriteriaInternal();
oredCriteria.add(criteria);
return criteria;
}
public Criteria createCriteria() {
Criteria criteria = createCriteriaInternal();
if (oredCriteria.size() == 0) {
oredCriteria.add(criteria);
}
return criteria;
}
protected Criteria createCriteriaInternal() {
Criteria criteria = new Criteria();
return criteria;
}
public void clear() {
oredCriteria.clear();
orderByClause = null;
distinct = false;
}
protected abstract static class GeneratedCriteria {
protected List<Criterion> criteria;
protected GeneratedCriteria() {
super();
criteria = new ArrayList<Criterion>();
}
public boolean isValid() {
return criteria.size() > 0;
}
public List<Criterion> getAllCriteria() {
return criteria;
}
public List<Criterion> getCriteria() {
return criteria;
}
protected void addCriterion(String condition) {
if (condition == null) {
throw new RuntimeException("Value for condition cannot be null");
}
criteria.add(new Criterion(condition));
}
protected void addCriterion(String condition, Object value, String property) {
if (value == null) {
throw new RuntimeException("Value for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value));
}
protected void addCriterion(String condition, Object value1, Object value2, String property) {
if (value1 == null || value2 == null) {
throw new RuntimeException("Between values for " + property + " cannot be null");
}
criteria.add(new Criterion(condition, value1, value2));
}
public Criteria andCourseidIsNull() {
addCriterion("courseID is null");
return (Criteria) this;
}
public Criteria andCourseidIsNotNull() {
addCriterion("courseID is not null");
return (Criteria) this;
}
public Criteria andCourseidEqualTo(Integer value) {
addCriterion("courseID =", value, "courseid");
return (Criteria) this;
}
public Criteria andCourseidNotEqualTo(Integer value) {
addCriterion("courseID <>", value, "courseid");
return (Criteria) this;
}
public Criteria andCourseidGreaterThan(Integer value) {
addCriterion("courseID >", value, "courseid");
return (Criteria) this;
}
public Criteria andCourseidGreaterThanOrEqualTo(Integer value) {
addCriterion("courseID >=", value, "courseid");
return (Criteria) this;
}
public Criteria andCourseidLessThan(Integer value) {
addCriterion("courseID <", value, "courseid");
return (Criteria) this;
}
public Criteria andCourseidLessThanOrEqualTo(Integer value) {
addCriterion("courseID <=", value, "courseid");
return (Criteria) this;
}
public Criteria andCourseidIn(List<Integer> values) {
addCriterion("courseID in", values, "courseid");
return (Criteria) this;
}
public Criteria andCourseidNotIn(List<Integer> values) {
addCriterion("courseID not in", values, "courseid");
return (Criteria) this;
}
public Criteria andCourseidBetween(Integer value1, Integer value2) {
addCriterion("courseID between", value1, value2, "courseid");
return (Criteria) this;
}
public Criteria andCourseidNotBetween(Integer value1, Integer value2) {
addCriterion("courseID not between", value1, value2, "courseid");
return (Criteria) this;
}
public Criteria andCoursenameIsNull() {
addCriterion("courseName is null");
return (Criteria) this;
}
public Criteria andCoursenameIsNotNull() {
addCriterion("courseName is not null");
return (Criteria) this;
}
public Criteria andCoursenameEqualTo(String value) {
addCriterion("courseName =", value, "coursename");
return (Criteria) this;
}
public Criteria andCoursenameNotEqualTo(String value) {
addCriterion("courseName <>", value, "coursename");
return (Criteria) this;
}
public Criteria andCoursenameGreaterThan(String value) {
addCriterion("courseName >", value, "coursename");
return (Criteria) this;
}
public Criteria andCoursenameGreaterThanOrEqualTo(String value) {
addCriterion("courseName >=", value, "coursename");
return (Criteria) this;
}
public Criteria andCoursenameLessThan(String value) {
addCriterion("courseName <", value, "coursename");
return (Criteria) this;
}
public Criteria andCoursenameLessThanOrEqualTo(String value) {
addCriterion("courseName <=", value, "coursename");
return (Criteria) this;
}
public Criteria andCoursenameLike(String value) {
addCriterion("courseName like", value, "coursename");
return (Criteria) this;
}
public Criteria andCoursenameNotLike(String value) {
addCriterion("courseName not like", value, "coursename");
return (Criteria) this;
}
public Criteria andCoursenameIn(List<String> values) {
addCriterion("courseName in", values, "coursename");
return (Criteria) this;
}
public Criteria andCoursenameNotIn(List<String> values) {
addCriterion("courseName not in", values, "coursename");
return (Criteria) this;
}
public Criteria andCoursenameBetween(String value1, String value2) {
addCriterion("courseName between", value1, value2, "coursename");
return (Criteria) this;
}
public Criteria andCoursenameNotBetween(String value1, String value2) {
addCriterion("courseName not between", value1, value2, "coursename");
return (Criteria) this;
}
public Criteria andTeacheridIsNull() {
addCriterion("teacherID is null");
return (Criteria) this;
}
public Criteria andTeacheridIsNotNull() {
addCriterion("teacherID is not null");
return (Criteria) this;
}
public Criteria andTeacheridEqualTo(Integer value) {
addCriterion("teacherID =", value, "teacherid");
return (Criteria) this;
}
public Criteria andTeacheridNotEqualTo(Integer value) {
addCriterion("teacherID <>", value, "teacherid");
return (Criteria) this;
}
public Criteria andTeacheridGre
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
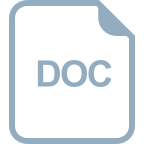
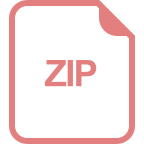
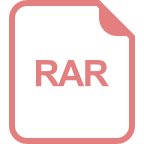
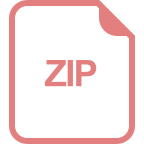
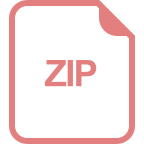
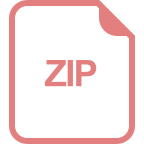
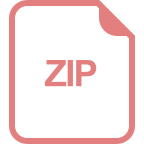
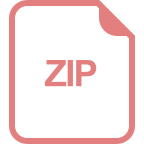
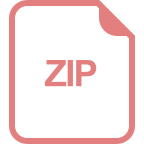
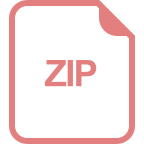
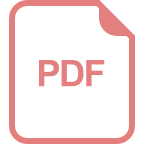
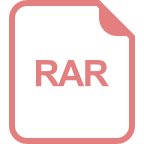
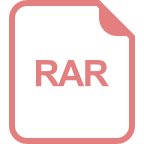
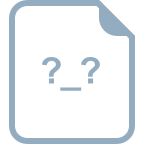
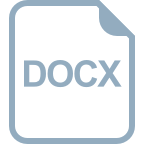
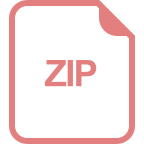
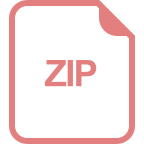
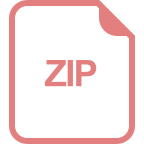
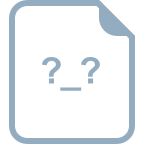
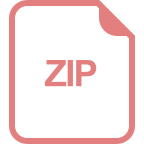
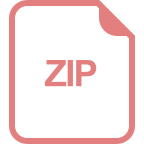
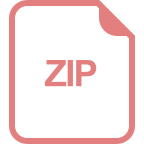
收起资源包目录

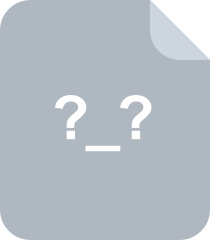
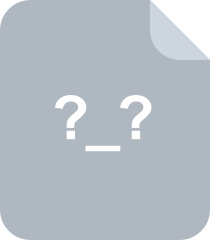
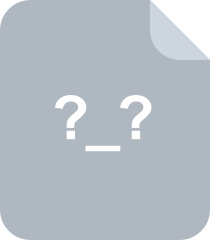
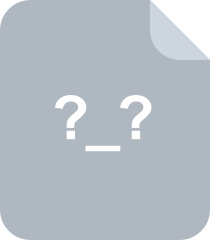
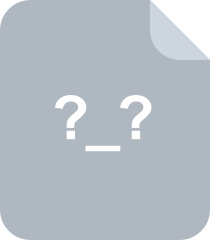
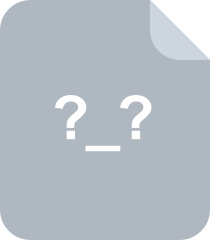
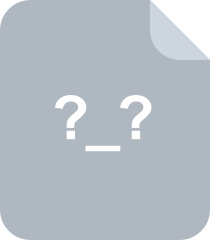
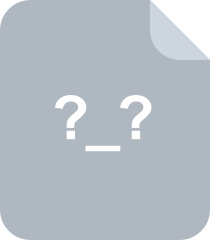
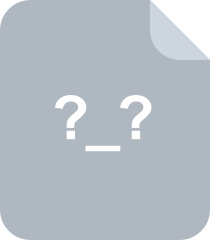
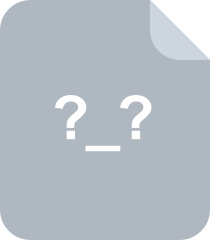
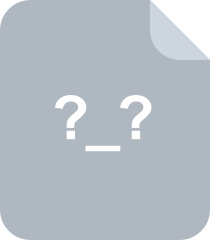
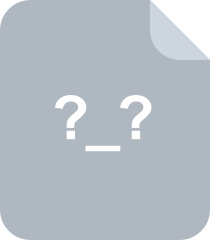
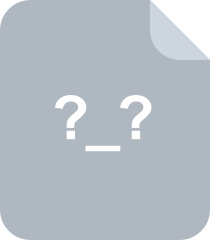
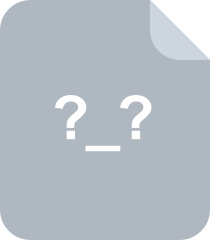
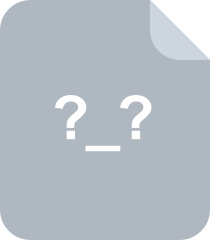
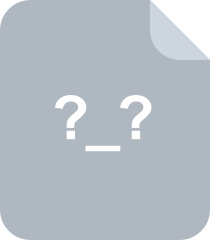
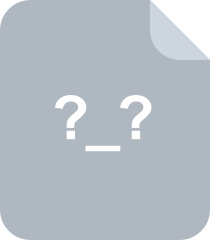
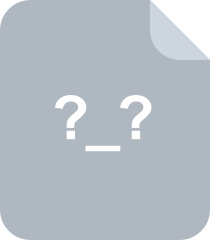
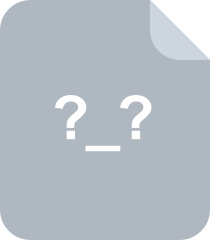
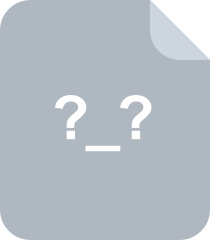
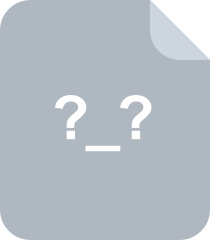
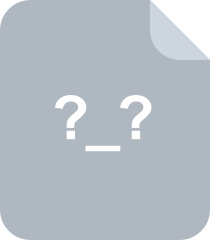
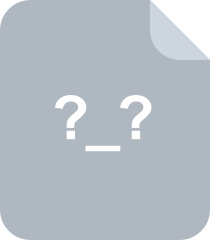
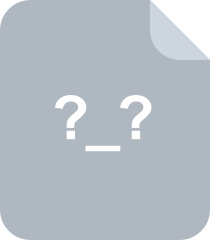
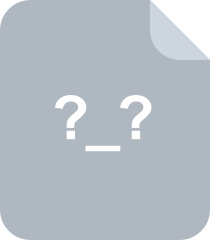
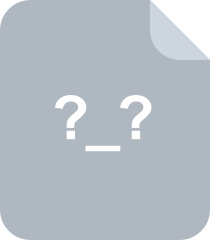
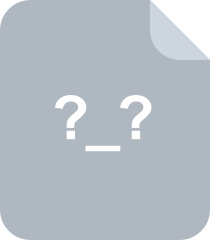
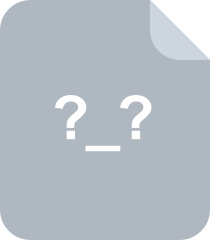
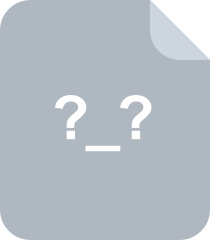
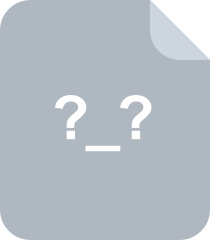
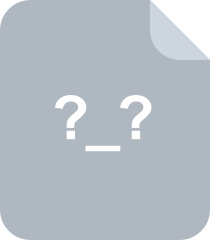
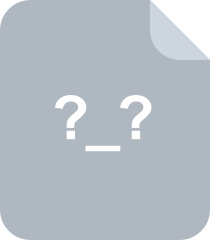
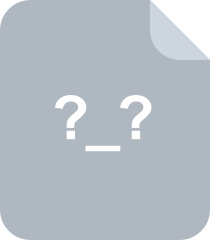
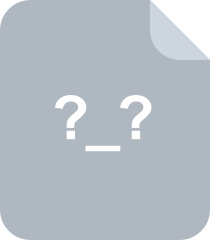
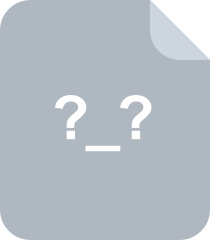
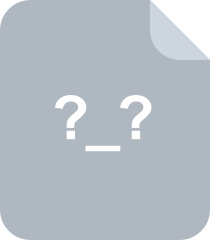
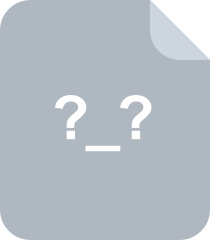
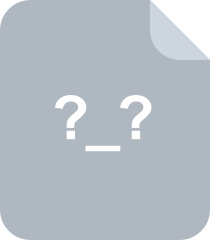
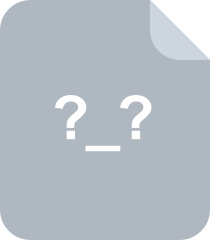
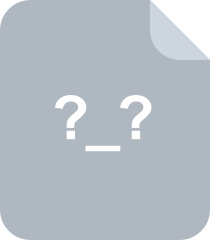
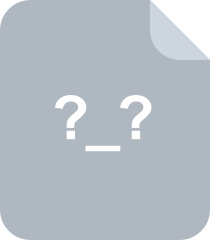
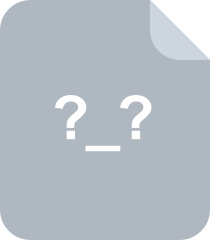
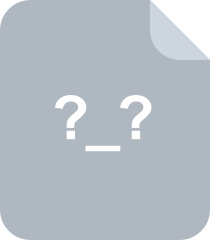
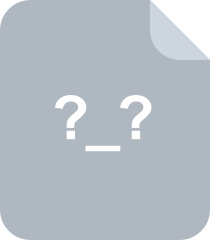
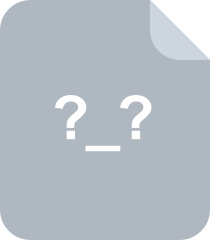
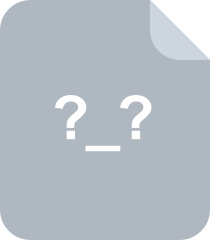
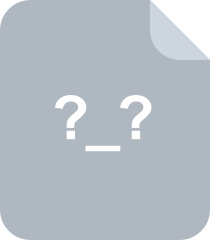
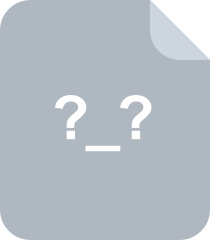
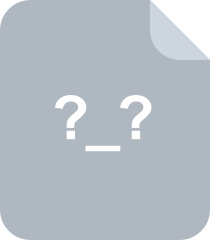
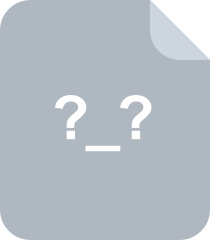
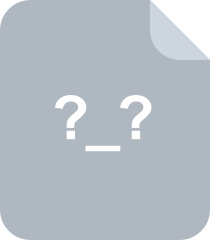
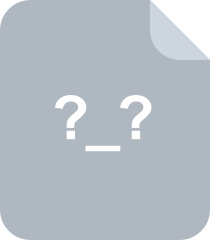
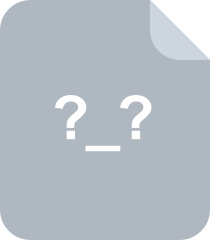
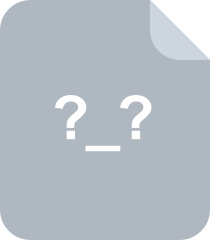
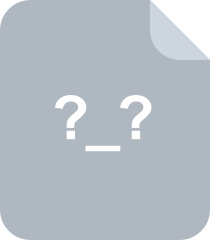
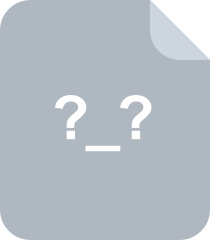
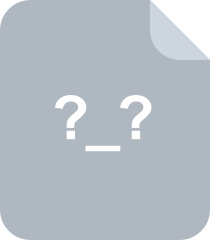
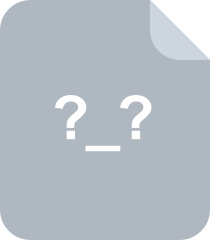
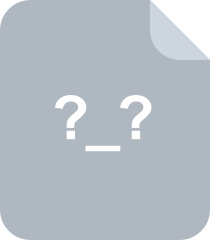
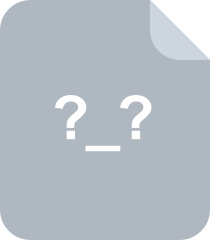
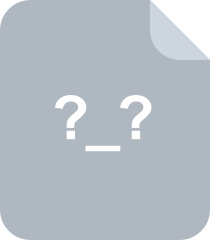
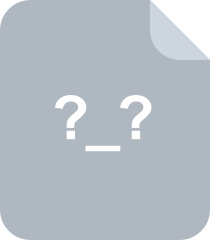
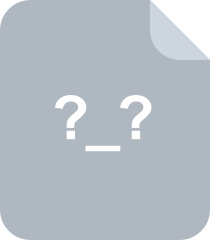
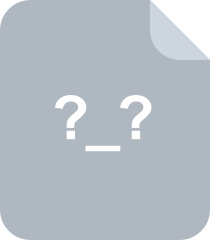
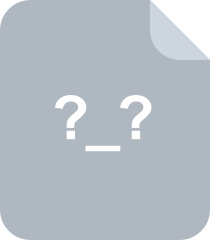
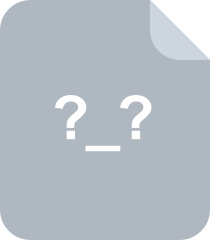
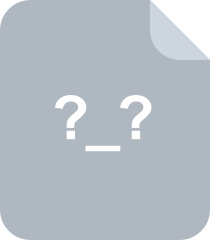
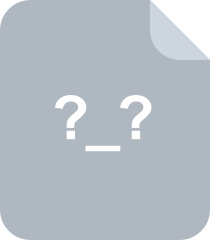
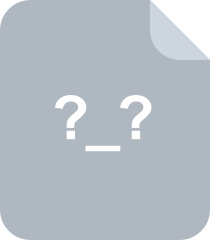
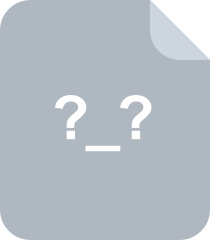
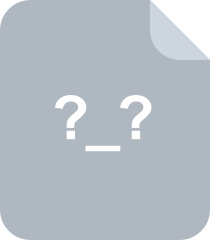
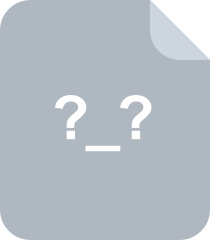
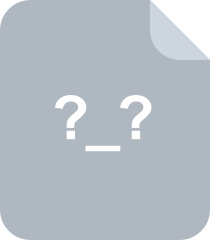
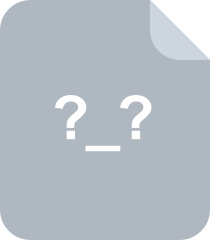
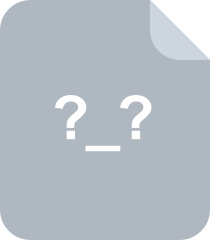
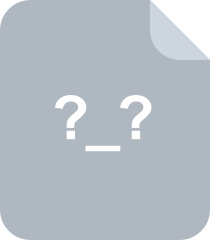
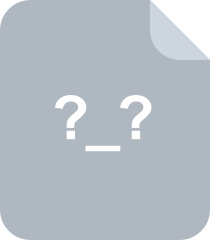
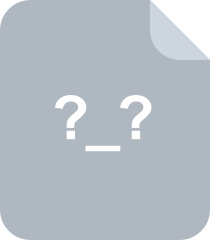
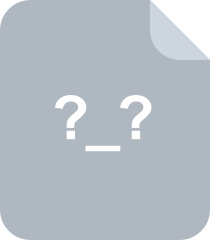
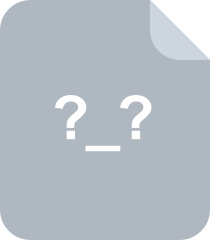
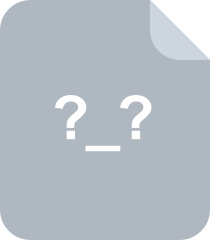
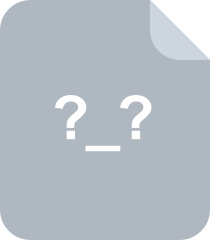
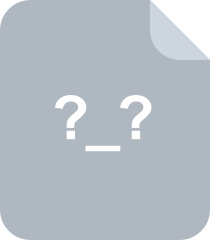
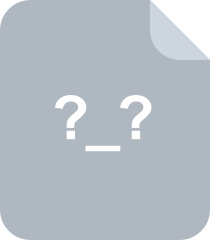
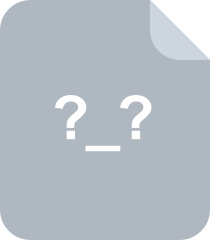
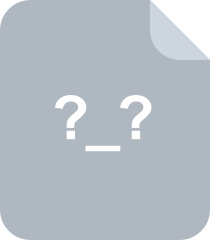
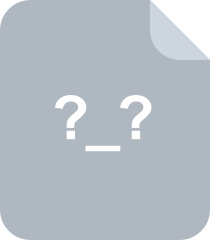
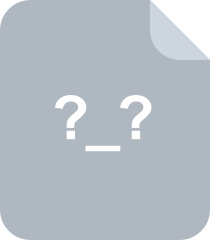
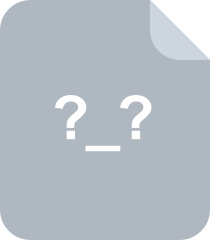
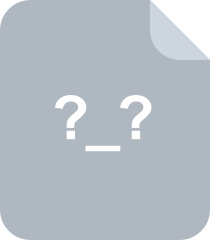
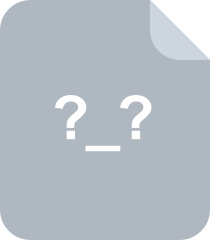
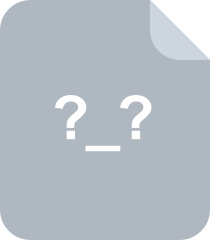
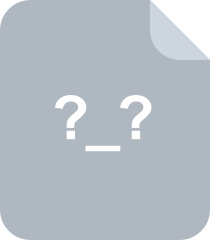
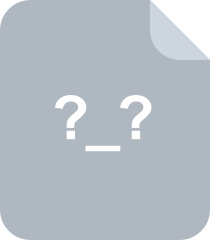
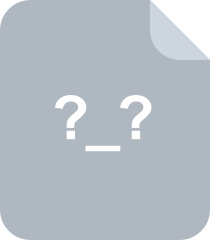
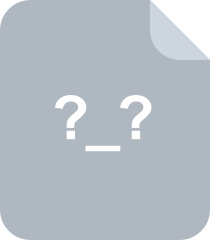
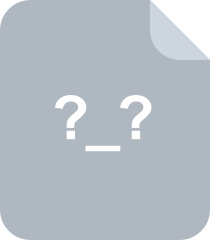
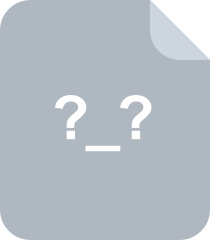
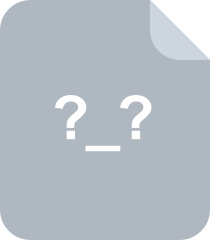
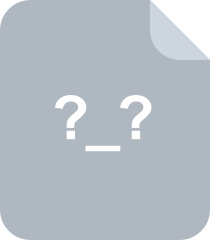
共 425 条
- 1
- 2
- 3
- 4
- 5
资源评论
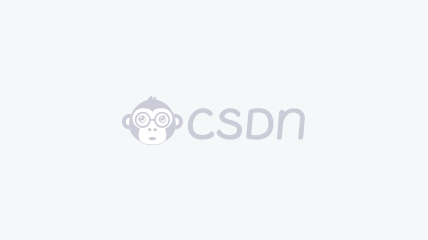

取址执行
- 粉丝: 228
- 资源: 71
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

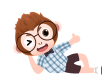
最新资源
- 建筑工程消防验收现场评定表.docx
- 交叉检查记录表(急救分中心).doc
- 交叉检查记录表(社区服务中心和乡镇卫生院).doc
- 居家适老化改造补贴实施细则产品功能表.docx
- 井田勘探探矿权出让收益评估报告( 模板).doc
- 髋关节功能丧失程度评定表.docx
- 买断式回购应急确认对话报价申请单.docx
- 每月质量安全调度会议纪要.docx
- 每月电梯安全调度会议纪要.docx
- 每周电梯质量安全排查报告.docx
- 每周电梯安全排查报告.docx
- 每月质量安全调度会议纪要表.docx
- 排水管网情况表.docx
- 聘请服务审批表(表格模板).docx
- 培训班次计划表.doc
- 密闭式输液表格、注意事项.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


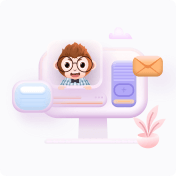
安全验证
文档复制为VIP权益,开通VIP直接复制
