/**
******************************************************************************
* @file stm8s_tim1.c
* @author MCD Application Team
* @version V2.2.0
* @date 30-September-2014
* @brief This file contains all the functions for the TIM1 peripheral.
******************************************************************************
* @attention
*
* <h2><center>© COPYRIGHT 2014 STMicroelectronics</center></h2>
*
* Licensed under MCD-ST Liberty SW License Agreement V2, (the "License");
* You may not use this file except in compliance with the License.
* You may obtain a copy of the License at:
*
* http://www.st.com/software_license_agreement_liberty_v2
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
******************************************************************************
*/
/* Includes ------------------------------------------------------------------*/
#include "stm8s_tim1.h"
/** @addtogroup STM8S_StdPeriph_Driver
* @{
*/
/* Private typedef -----------------------------------------------------------*/
/* Private define ------------------------------------------------------------*/
/* Private macro -------------------------------------------------------------*/
/* Private variables ---------------------------------------------------------*/
/* Private function prototypes -----------------------------------------------*/
static void TI1_Config(uint8_t TIM1_ICPolarity, uint8_t TIM1_ICSelection,
uint8_t TIM1_ICFilter);
static void TI2_Config(uint8_t TIM1_ICPolarity, uint8_t TIM1_ICSelection,
uint8_t TIM1_ICFilter);
static void TI3_Config(uint8_t TIM1_ICPolarity, uint8_t TIM1_ICSelection,
uint8_t TIM1_ICFilter);
static void TI4_Config(uint8_t TIM1_ICPolarity, uint8_t TIM1_ICSelection,
uint8_t TIM1_ICFilter);
/**
* @addtogroup TIM1_Public_Functions
* @{
*/
/**
* @brief Deinitializes the TIM1 peripheral registers to their default reset values.
* @param None
* @retval None
*/
void TIM1_DeInit(void)
{
TIM1->CR1 = TIM1_CR1_RESET_VALUE;
TIM1->CR2 = TIM1_CR2_RESET_VALUE;
TIM1->SMCR = TIM1_SMCR_RESET_VALUE;
TIM1->ETR = TIM1_ETR_RESET_VALUE;
TIM1->IER = TIM1_IER_RESET_VALUE;
TIM1->SR2 = TIM1_SR2_RESET_VALUE;
/* Disable channels */
TIM1->CCER1 = TIM1_CCER1_RESET_VALUE;
TIM1->CCER2 = TIM1_CCER2_RESET_VALUE;
/* Configure channels as inputs: it is necessary if lock level is equal to 2 or 3 */
TIM1->CCMR1 = 0x01;
TIM1->CCMR2 = 0x01;
TIM1->CCMR3 = 0x01;
TIM1->CCMR4 = 0x01;
/* Then reset channel registers: it also works if lock level is equal to 2 or 3 */
TIM1->CCER1 = TIM1_CCER1_RESET_VALUE;
TIM1->CCER2 = TIM1_CCER2_RESET_VALUE;
TIM1->CCMR1 = TIM1_CCMR1_RESET_VALUE;
TIM1->CCMR2 = TIM1_CCMR2_RESET_VALUE;
TIM1->CCMR3 = TIM1_CCMR3_RESET_VALUE;
TIM1->CCMR4 = TIM1_CCMR4_RESET_VALUE;
TIM1->CNTRH = TIM1_CNTRH_RESET_VALUE;
TIM1->CNTRL = TIM1_CNTRL_RESET_VALUE;
TIM1->PSCRH = TIM1_PSCRH_RESET_VALUE;
TIM1->PSCRL = TIM1_PSCRL_RESET_VALUE;
TIM1->ARRH = TIM1_ARRH_RESET_VALUE;
TIM1->ARRL = TIM1_ARRL_RESET_VALUE;
TIM1->CCR1H = TIM1_CCR1H_RESET_VALUE;
TIM1->CCR1L = TIM1_CCR1L_RESET_VALUE;
TIM1->CCR2H = TIM1_CCR2H_RESET_VALUE;
TIM1->CCR2L = TIM1_CCR2L_RESET_VALUE;
TIM1->CCR3H = TIM1_CCR3H_RESET_VALUE;
TIM1->CCR3L = TIM1_CCR3L_RESET_VALUE;
TIM1->CCR4H = TIM1_CCR4H_RESET_VALUE;
TIM1->CCR4L = TIM1_CCR4L_RESET_VALUE;
TIM1->OISR = TIM1_OISR_RESET_VALUE;
TIM1->EGR = 0x01; /* TIM1_EGR_UG */
TIM1->DTR = TIM1_DTR_RESET_VALUE;
TIM1->BKR = TIM1_BKR_RESET_VALUE;
TIM1->RCR = TIM1_RCR_RESET_VALUE;
TIM1->SR1 = TIM1_SR1_RESET_VALUE;
}
/**
* @brief Initializes the TIM1 Time Base Unit according to the specified parameters.
* @param TIM1_Prescaler specifies the Prescaler value.
* @param TIM1_CounterMode specifies the counter mode from @ref TIM1_CounterMode_TypeDef .
* @param TIM1_Period specifies the Period value.
* @param TIM1_RepetitionCounter specifies the Repetition counter value
* @retval None
*/
void TIM1_TimeBaseInit(uint16_t TIM1_Prescaler,
TIM1_CounterMode_TypeDef TIM1_CounterMode,
uint16_t TIM1_Period,
uint8_t TIM1_RepetitionCounter)
{
/* Check parameters */
assert_param(IS_TIM1_COUNTER_MODE_OK(TIM1_CounterMode));
/* Set the Autoreload value */
TIM1->ARRH = (uint8_t)(TIM1_Period >> 8);
TIM1->ARRL = (uint8_t)(TIM1_Period);
/* Set the Prescaler value */
TIM1->PSCRH = (uint8_t)(TIM1_Prescaler >> 8);
TIM1->PSCRL = (uint8_t)(TIM1_Prescaler);
/* Select the Counter Mode */
TIM1->CR1 = (uint8_t)((uint8_t)(TIM1->CR1 & (uint8_t)(~(TIM1_CR1_CMS | TIM1_CR1_DIR)))
| (uint8_t)(TIM1_CounterMode));
/* Set the Repetition Counter value */
TIM1->RCR = TIM1_RepetitionCounter;
}
/**
* @brief Initializes the TIM1 Channel1 according to the specified parameters.
* @param TIM1_OCMode specifies the Output Compare mode from
* @ref TIM1_OCMode_TypeDef.
* @param TIM1_OutputState specifies the Output State from
* @ref TIM1_OutputState_TypeDef.
* @param TIM1_OutputNState specifies the Complementary Output State
* from @ref TIM1_OutputNState_TypeDef.
* @param TIM1_Pulse specifies the Pulse width value.
* @param TIM1_OCPolarity specifies the Output Compare Polarity from
* @ref TIM1_OCPolarity_TypeDef.
* @param TIM1_OCNPolarity specifies the Complementary Output Compare Polarity
* from @ref TIM1_OCNPolarity_TypeDef.
* @param TIM1_OCIdleState specifies the Output Compare Idle State from
* @ref TIM1_OCIdleState_TypeDef.
* @param TIM1_OCNIdleState specifies the Complementary Output Compare Idle
* State from @ref TIM1_OCIdleState_TypeDef.
* @retval None
*/
void TIM1_OC1Init(TIM1_OCMode_TypeDef TIM1_OCMode,
TIM1_OutputState_TypeDef TIM1_OutputState,
TIM1_OutputNState_TypeDef TIM1_OutputNState,
uint16_t TIM1_Pulse,
TIM1_OCPolarity_TypeDef TIM1_OCPolarity,
TIM1_OCNPolarity_TypeDef TIM1_OCNPolarity,
TIM1_OCIdleState_TypeDef TIM1_OCIdleState,
TIM1_OCNIdleState_TypeDef TIM1_OCNIdleState)
{
/* Check the parameters */
assert_param(IS_TIM1_OC_MODE_OK(TIM1_OCMode));
assert_param(IS_TIM1_OUTPUT_STATE_OK(TIM1_OutputState));
assert_param(IS_TIM1_OUTPUTN_STATE_OK(TIM1_OutputNState));
assert_param(IS_TIM1_OC_POLARITY_OK(TIM1_OCPolarity));
assert_param(IS_TIM1_OCN_POLARITY_OK(TIM1_OCNPolarity));
assert_param(IS_TIM1_OCIDLE_STATE_OK(TIM1_OCIdleState));
assert_param(IS_TIM1_OCNIDLE_STATE_OK(TIM1_OCNIdleState));
/* Disable the Channel 1: Reset the CCE Bit, Set the Output State ,
the Output N State, the Output Polarity & the Output N Polarity*/
TIM1->CCER1 &= (uint8_t)(~( TIM1_CCER1_CC1E | TIM1_CCER1_CC1NE
| TIM1_CCER1_CC1P | TIM1_CCER1_CC1NP));
/* Set the Output State & Set the Output N State & Set the Output Polarity &
Set the Output N Polarity */
TIM1->CCER1 |= (uint8_t)((uint8_t)((uint8_t)(TIM1_OutputState & TIM1_CCER1_CC1E)
| (uint8_t)(TIM1_OutputNState & TIM1_CCER1_CC1NE))
| (uint8_t)( (uint8_t)(TIM1_OCPolarity & TIM1_CCER1_CC1P)
| (uint8_t)(TIM1_OCNPolarity & TIM1_CCER1_CC1NP)))
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
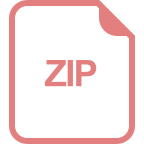
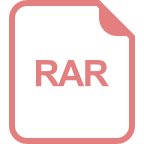
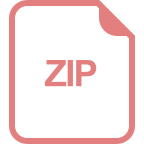
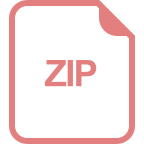
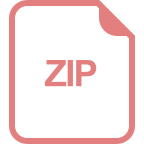
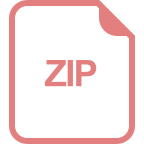
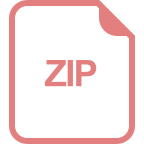
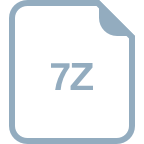
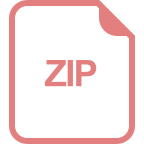
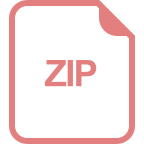
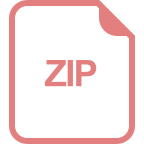
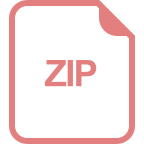
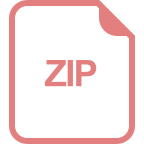
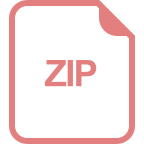
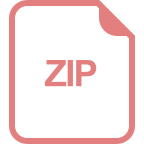
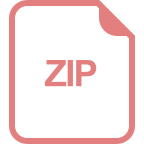
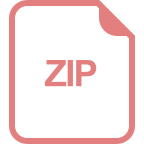
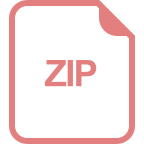
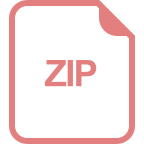
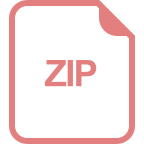
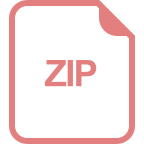
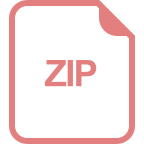
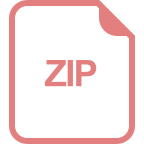
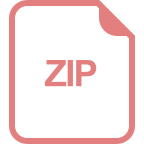
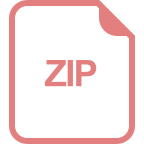
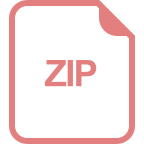
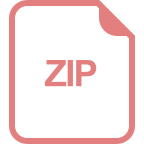
收起资源包目录

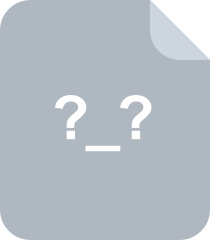
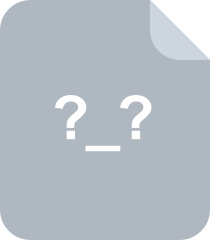
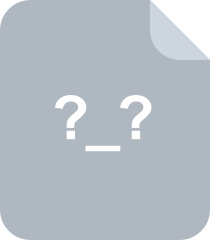
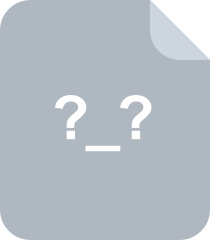
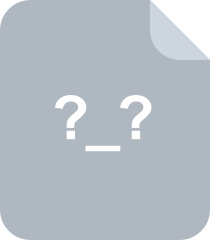
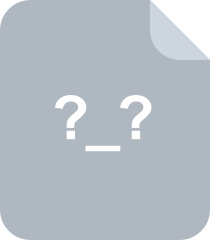
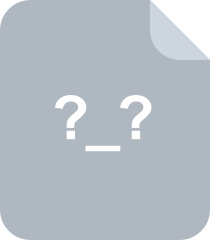
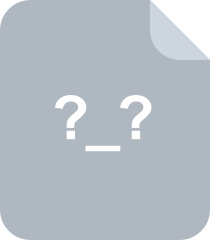
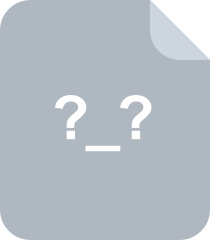
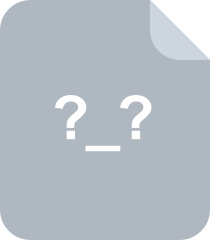
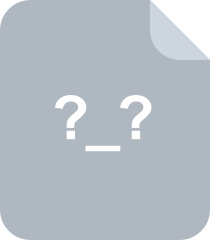
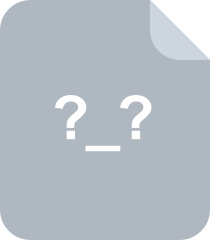
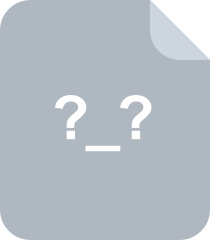
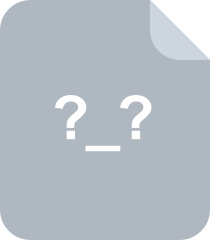
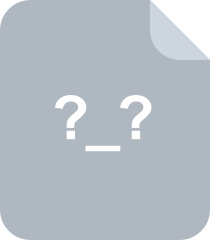
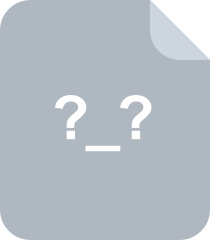
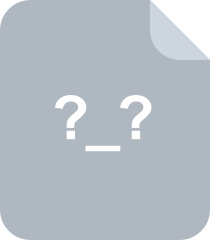
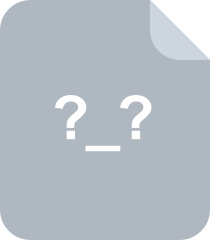
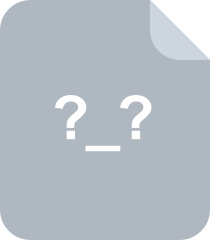
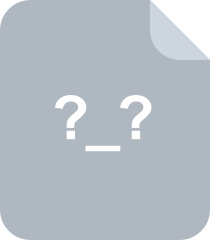
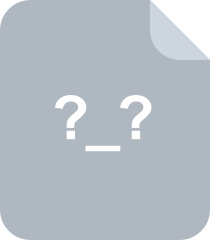
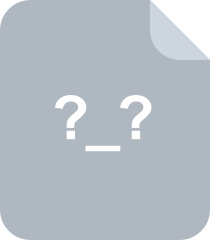
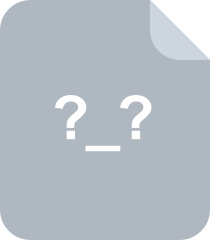
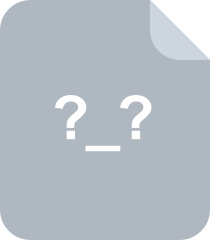
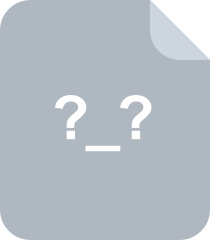
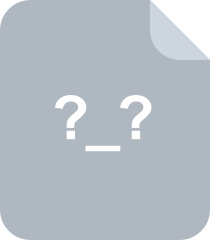
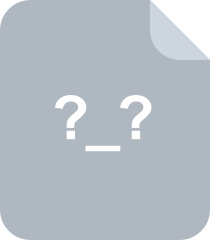
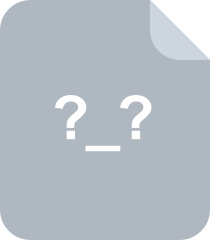
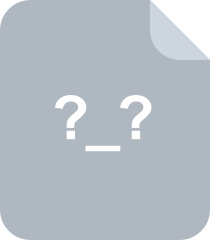
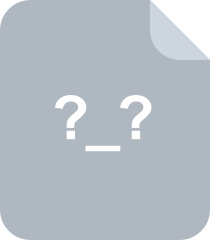
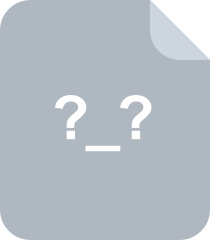
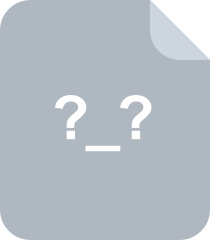
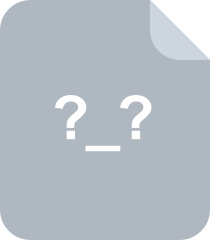
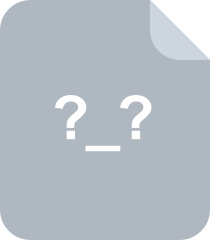
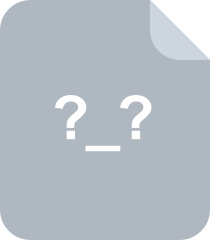
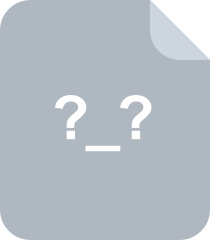
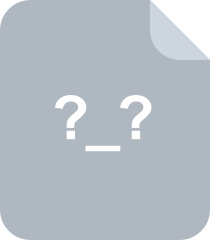
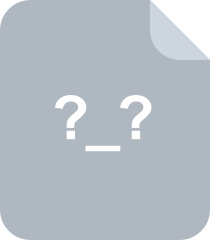
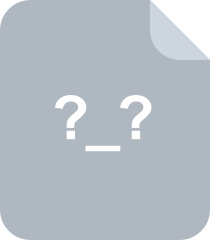
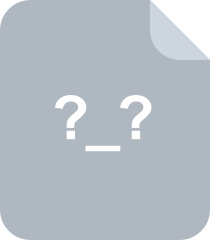
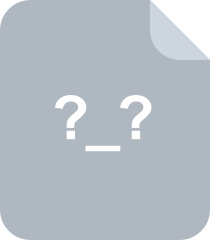
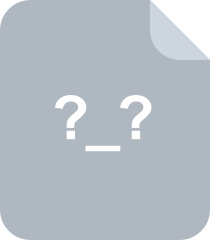
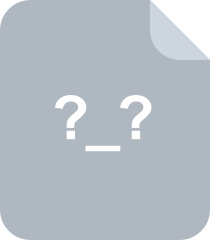
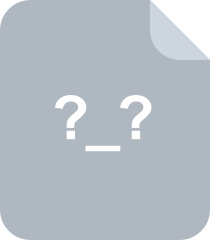
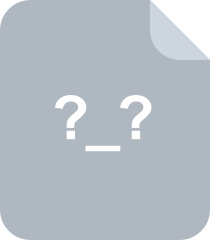
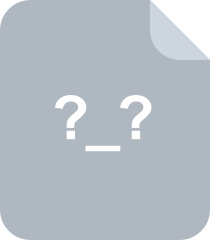
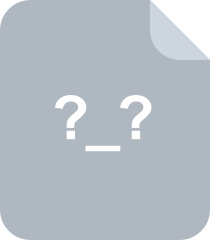
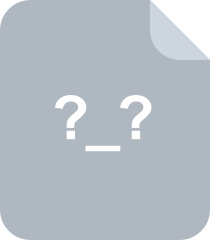
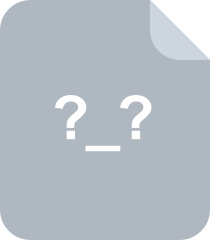
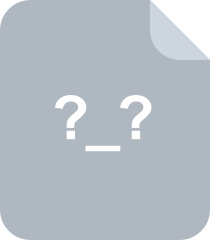
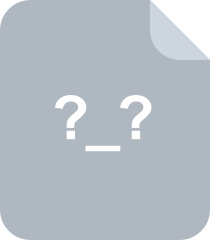
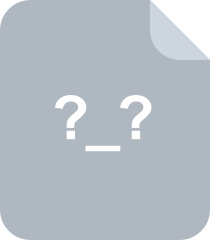
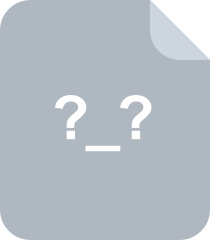
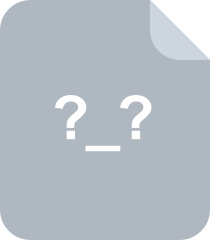
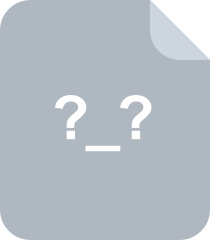
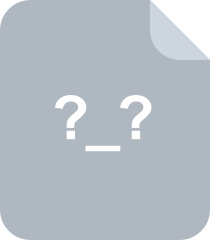
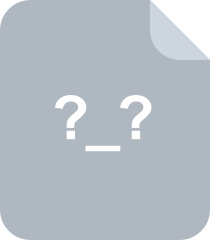
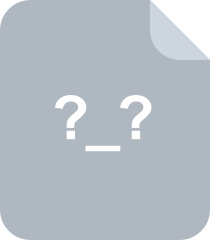
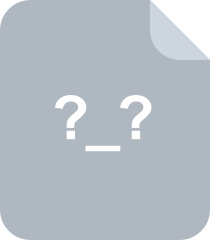
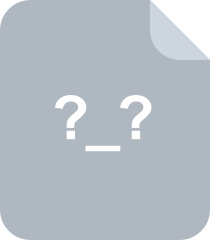
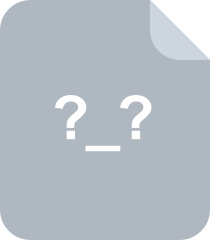
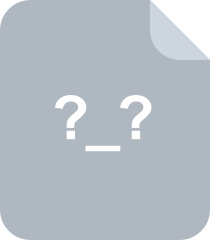
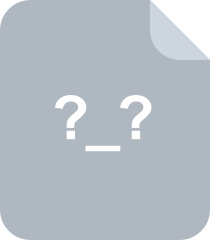
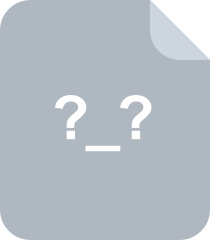
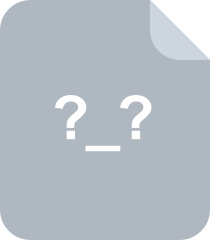
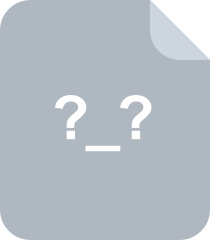
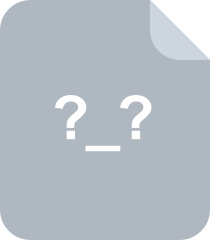
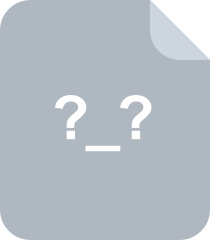
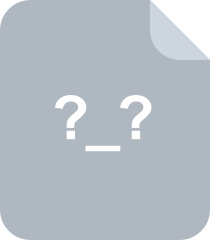
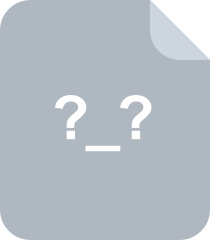
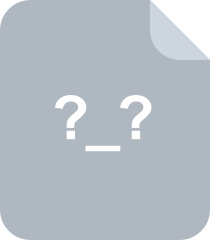
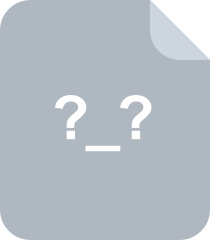
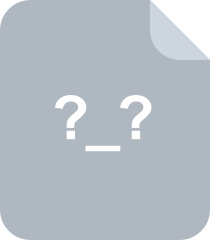
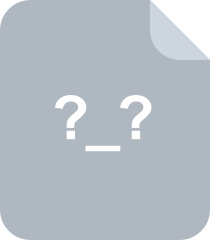
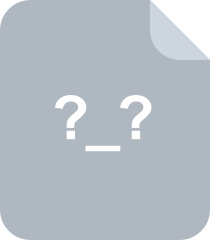
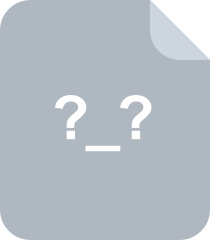
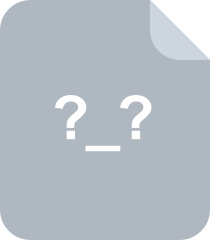
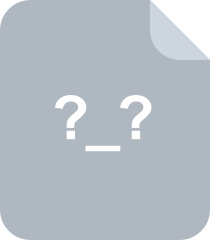
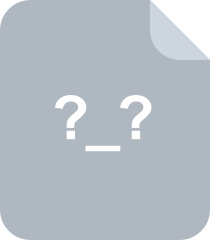
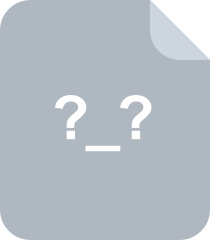
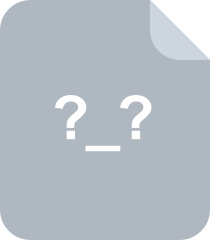
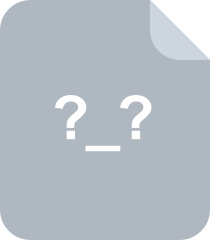
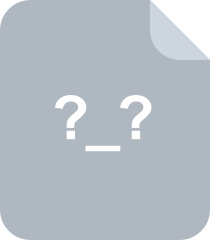
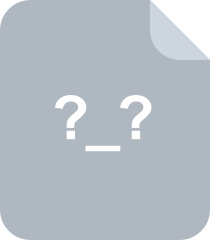
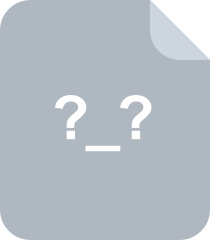
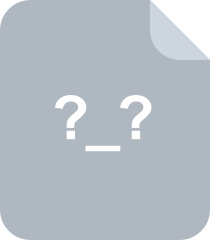
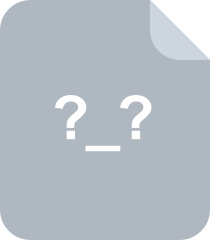
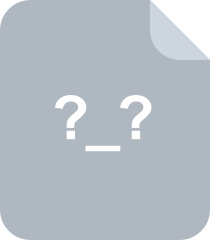
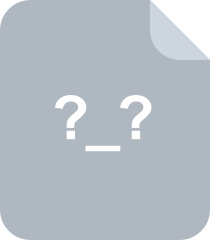
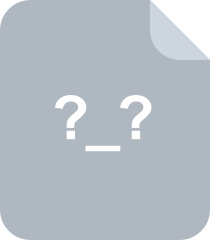
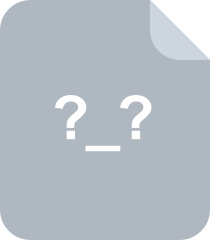
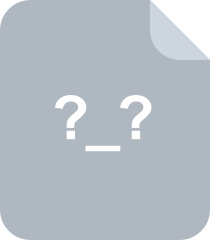
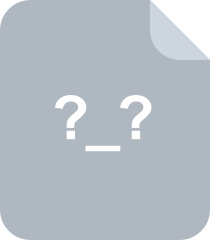
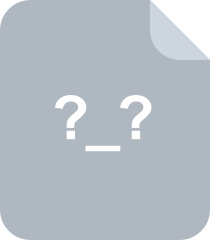
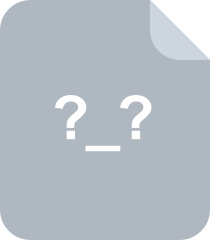
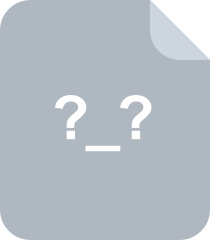
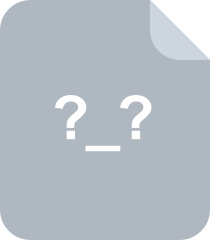
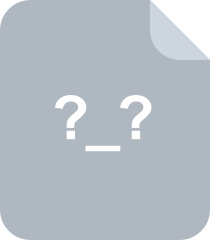
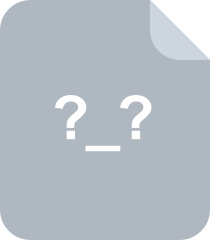
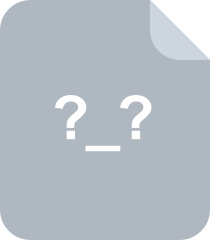
共 354 条
- 1
- 2
- 3
- 4
资源评论
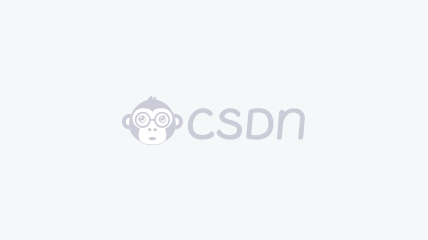

等天晴i
- 粉丝: 5961
- 资源: 10万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

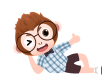
最新资源
- 三电平T型逆变器模型预测控制中点电压平衡控制,包括电流预测控制模型、功率预测控制模型,,Matlab simulink仿真(2018a及以上版本)
- directshow实现的VCAM虚拟摄像头
- AI生成测试用例的实现方案
- 多策略改进蜣螂优化MSADBO-CNN-BiGRU 多输入单输出预测 Python 代码 1.优化参数:filter, units1, units2, 学习率 2.此代码可用于数据回归预测和模型参数的
- Java程序设计实践教程(讲义)
- at32f403版本的汇川MD500E代码,完全版,有感无感都包含,除了数码管显示屏蔽了,别的都移植过去了 只适合学习用,不适用于量产
- 测试报告关键信息收集:涵盖文档准备、客户信息与系统配置
- 异步sar adc 10bit 250M 电路 刘纯成单调开关lunwen复现 带tsmc28nm工艺 电路,可仿真
- 图像修复- 基于mask操作和图像修复的去水印神经网络傻瓜式教学
- OfficeScrubber v13 卸载工具.rar
- 基于GLCIC-PyTorch人脸修复项目的全球人脸训练集img-align-celeba.zip-压缩包2
- HTML5实现好看的二手商品交易平台网站源码.zip
- HTML5实现好看的房地产建筑行业模板.zip
- HTML5实现好看的房产中介官网网站源码.zip
- HTML5实现好看的粉色个人博客主页网站源码.zip
- HTML5实现好看的粉色简洁蛋糕制作网站源码.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


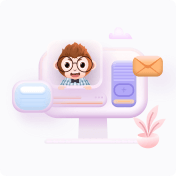
安全验证
文档复制为VIP权益,开通VIP直接复制
