using System;
using System.Linq;
using System.Windows.Forms;
using System.IO.Ports;
using System.Reflection;
using System.Threading;
using System.Runtime.InteropServices;
using Ginkgo;
using System.Collections.Generic;
using System.Drawing;
using Excel = Microsoft.Office.Interop.Excel;
namespace createxcel_2
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
[DllImport("kernel32.dll")]
extern static short QueryPerformanceCounter(ref long x);
[DllImport("kernel32.dll")]
extern static short QueryPerformanceFrequency(ref long x);
List<byte> rd_dat = new List<byte>();
List<byte> rd55_dat = new List<byte>();
List<byte> rdAA_dat = new List<byte>();
List<string> rw_flag = new List<string>();
List<byte> w_index = new List<byte>();
Byte[] w_repeat_index = new byte[4];
String[] w_repeat_judge = new string[4];
List<string> rd_repeat_1 = new List<string>();
List<string> rd_repeat_2 = new List<string>();
enum bus_mode {I2C_NOFT,I2C_OFT,SM_NCMD,SM_CMD_NCT,SM_CMD_CT,WRONG_MODE }
List<byte> pointer_autoinc = new List<byte>();
List<byte> pointer_cycle = new List<byte>();
// speed 用来存放 I2C 的选择speed
Dictionary<int,uint> bus_speed=new Dictionary<int, uint> { { 1, 10000 },{ 2, 100000 },{ 4, 400000 },{ 8, 1000000 } };
struct ini_i2c
{
byte wr_adr;
uint wr_offset;
byte wr_countbyte;
ushort wr_num;
ushort rd_num;
string err_ini;
//byte[] wr_dat ;
// byte[] rd_temp;
public void input_val(byte w1, uint w2,byte w3 ,ushort wr1, ushort rd1, string err1)
{
wr_adr = w1;
wr_offset = w2;
wr_countbyte = w3;
wr_num = wr1;
rd_num = rd1;
err_ini = err1;
}
public void output_val(out byte w1, out uint w2,out byte w3, out ushort wr1, out ushort rd1,out string err1)
{
w1 = wr_adr;
w2 = wr_offset;
w3 = wr_countbyte;
wr1 = wr_num;
rd1 = rd_num;
err1 = err_ini;
}
}
private void Form1_Load(object sender, EventArgs e) //窗体加载
{
check_i2coft.Checked = false;
text_i2coft.Enabled = false;
group_i2c.Enabled = false;
group_sm.Enabled = false;
group_showdat.Enabled = false;
//group_but.Enabled = false;
group_speed.Enabled = false;
}
private bus_mode Check_mode()
{
if (check_i2c.Checked)
{
if (check_i2coft.Checked) return bus_mode.I2C_OFT;
else return bus_mode.I2C_NOFT;
}
else if (check_sm.Checked)
{
if (check_smcmd.Checked && check_smcount.Checked) return bus_mode.SM_CMD_CT;
else if (check_smcmd.Checked) return bus_mode.SM_CMD_NCT;
else return bus_mode.SM_NCMD;
}
else return bus_mode.WRONG_MODE;
}
private ini_i2c initial_autotest()
{
//initial
int ret;
ini_i2c auto_temp = new ini_i2c();
byte wr_adr = 0x00;
uint wr_offset = 0x00;
ushort wr_num = 1;
ushort rd_num = 1;
byte wr_countbyte = 1;
string err_code_temp = "";
int bus_speed_temp;
int aa, bb, cc, dd;
int mode_temp;
// byte[] wr_dat = new byte[1];
//byte[] rd_temp = new byte[1];
//
ret = ControlI2C.VII_ScanDevice(1);
if (ret <= 0)
{
//MessageBox.Show("No device connect!");
err_code_temp = "No device connect";
return auto_temp;
}
ret = ControlI2C.VII_OpenDevice(ControlI2C.INIT_CONFIG.VII_USBI2C, 0, 0);
if (ret != ControlI2C.ERROR.SUCCESS)
{
//MessageBox.Show("Open device error!");
err_code_temp = "Open device error!";
return auto_temp;
}
// CONFIG i2c
ControlI2C.I2C_Config.AddrType = ControlI2C.INIT_CONFIG.VII_ADDR_7BIT;
aa = Convert.ToInt32(radio_10k.Checked);
bb= Convert.ToInt32(radio_100k.Checked);
cc= Convert.ToInt32(radio_400k.Checked);
dd= Convert.ToInt32(radio_1m.Checked);
bus_speed_temp = aa + bb * 2 + cc * 4 + dd * 8;
ControlI2C.I2C_Config.ClockSpeed = bus_speed[bus_speed_temp]; // 速度
//ControlI2C.I2C_Config.ClockSpeed = 400000;
label32.Text = bus_speed[bus_speed_temp].ToString();
ControlI2C.I2C_Config.ControlMode = ControlI2C.INIT_CONFIG.VII_HCTL_MODE; //硬件I2C
ControlI2C.I2C_Config.MasterMode = ControlI2C.INIT_CONFIG.VII_MASTER; // master 模式
mode_temp = Convert.ToInt32(Check_mode());
switch (mode_temp)
{
case (int)bus_mode.I2C_NOFT:
ControlI2C.I2C_Config.SubAddrWidth = ControlI2C.INIT_CONFIG.VII_SUB_ADDR_NONE;
err_code_temp = "Success_I2C_NOFT";
break;
case (int)bus_mode.I2C_OFT:
ControlI2C.I2C_Config.SubAddrWidth = ControlI2C.INIT_CONFIG.VII_SUB_ADDR_1BYTE;
if (text_i2coft.Text == "") wr_offset = 0x00;
else wr_offset = Convert.ToByte(text_i2coft.Text, 16);
err_code_temp = "Success_I2C_OFT";
break;
case (int)bus_mode.SM_NCMD:
ControlI2C.I2C_Config.SubAddrWidth = ControlI2C.INIT_CONFIG.VII_SUB_ADDR_NONE;
err_code_temp = "Success_SM_NCMD";
break;
case (int)bus_mode.SM_CMD_NCT:
ControlI2C.I2C_Config.SubAddrWidth = ControlI2C.INIT_CONFIG.VII_SUB_ADDR_1BYTE;
if (text_smcmd.Text == "") wr_offset = 0x00;
else wr_offset = Convert.ToByte(text_smcmd.Text, 16);
err_code_temp = "Success_SM_CMD_NCT";
break;
case (int)bus_mode.SM_CMD_CT:
ControlI2C.I2C_Config.SubAddrWidth = ControlI2C.INIT_CONFIG.VII_SUB_ADDR_1BYTE;
if (text_smcmd.Text == "") wr_offset = 0x00;
else wr_offset = Convert.ToByte(text_smcmd.Text, 16);
if (text_smcount.Text == "") wr_countbyte = 0x00;
else wr_countbyte = Convert.ToByte(text_smcount.Text, 16);
err_code_temp = "Success_SM_CMD_CT";
break;
case (int)bus_mode.WRONG_MODE:
// MessageBox.Show("Wrong Mode ");
err_code_temp = "Wrong Mode";
auto_temp.input_val(wr_adr, wr_offset, wr_countbyte, wr_num, rd_num, err_code_temp);
return auto_temp;
}
if(check_i2c.Checked)
{
if (text_i2caddr.Text.Length == 2)
{
wr_adr = (byte)(Convert.ToInt32(text_i2caddr.Text, 16) * 2 + 1);
}
else
{
// MessageBox.Show("Slave Address is wrong", "Error");
err_code_temp = "Wrong Slave Address";
auto_temp.input_val(wr_adr, wr_offset, wr_countbyte, wr_num, rd_nu
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
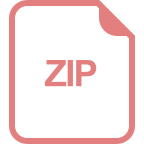
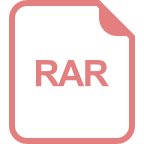
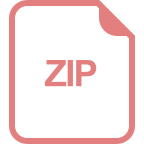
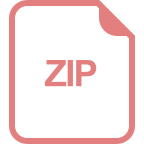
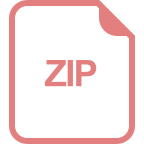
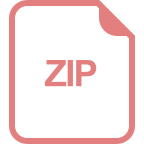
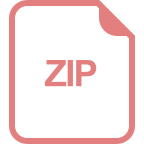
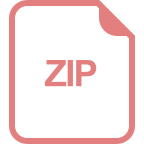
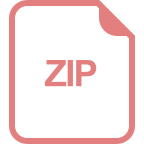
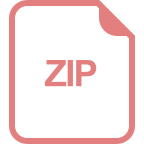
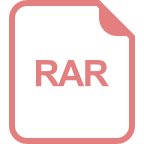
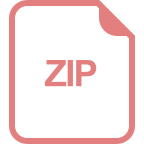
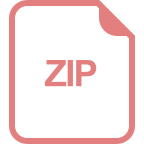
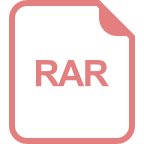
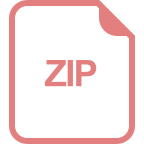
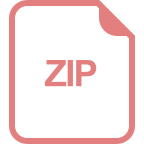
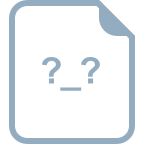
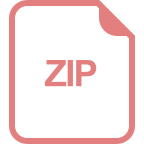
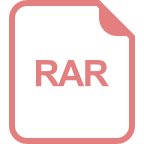
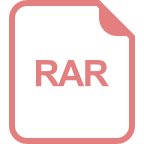
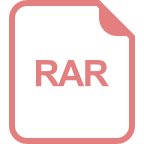
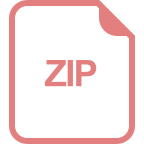
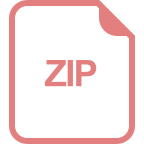
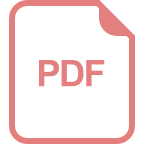
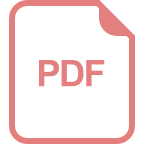
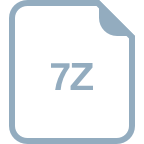
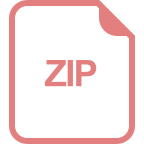
收起资源包目录

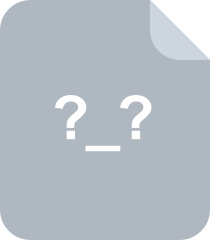
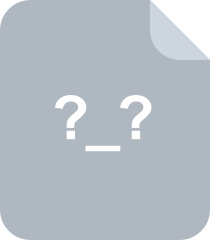
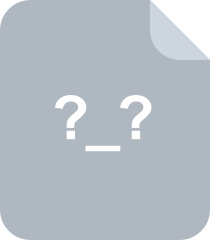
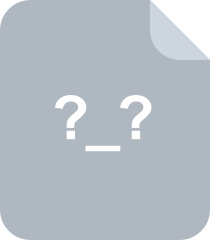
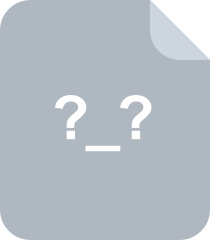
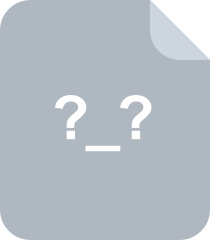
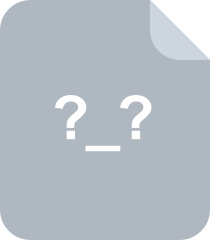
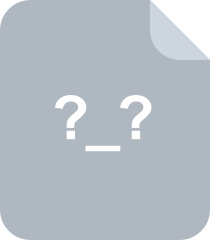
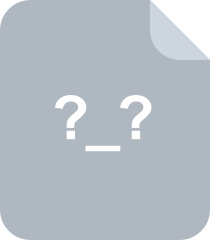
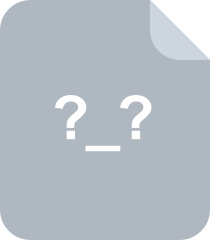
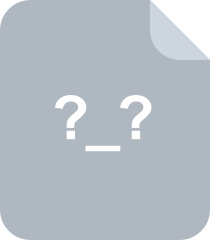
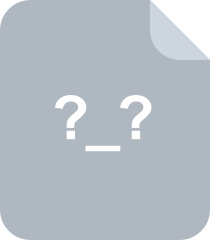
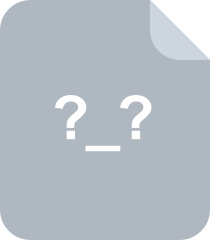
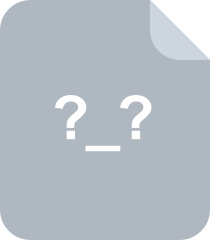
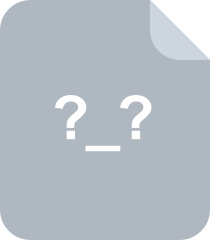
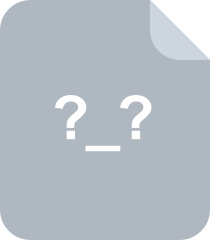
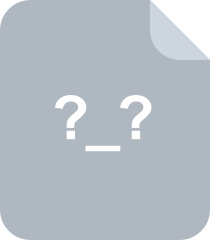
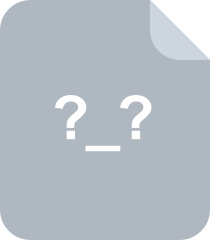
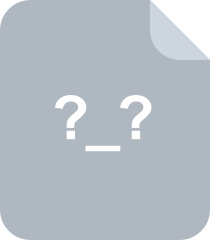
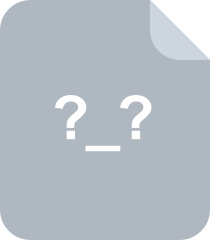
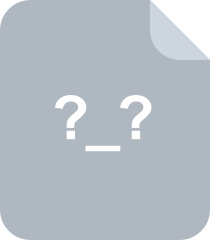
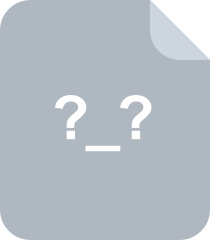
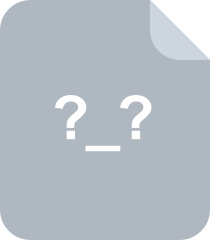
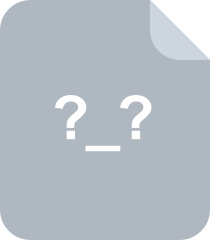
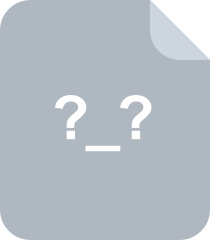
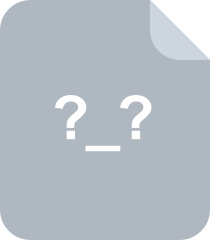
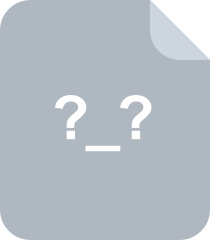
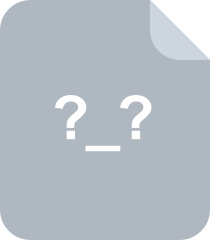
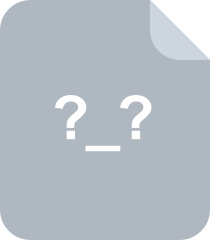
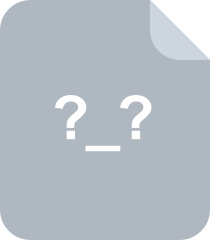
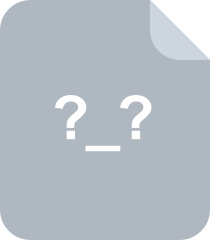
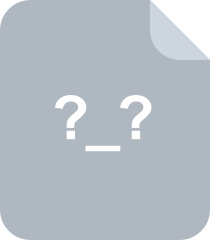
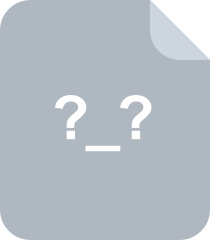
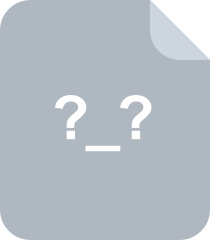
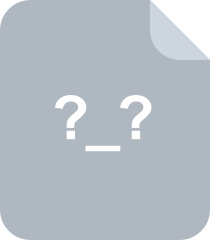
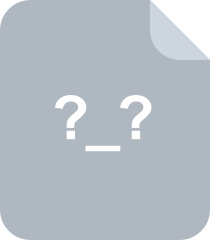
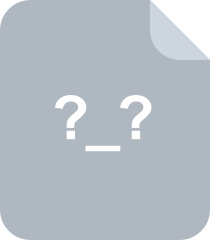
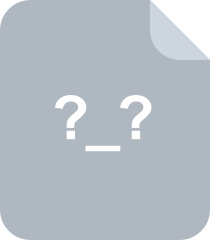
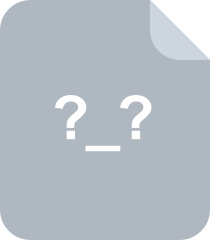
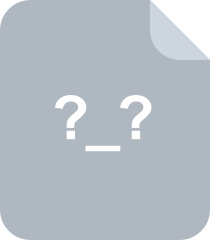
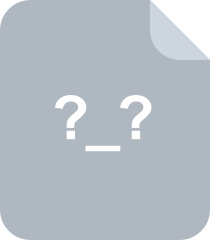
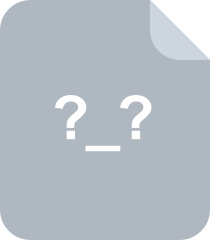
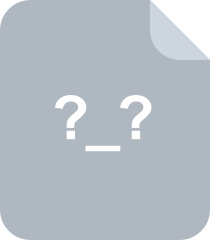
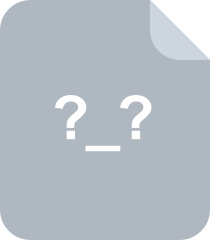
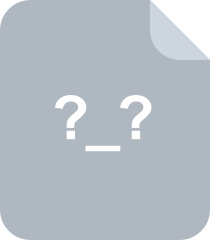
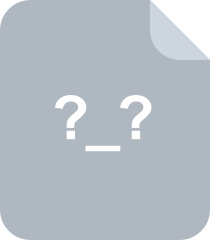
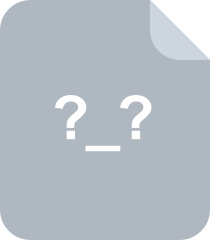
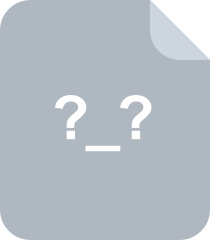
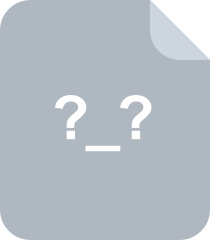
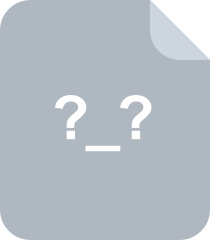
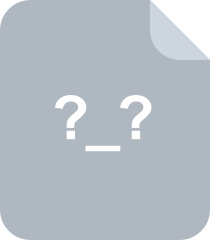
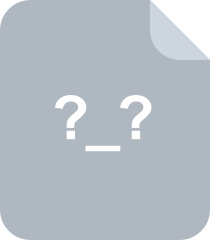
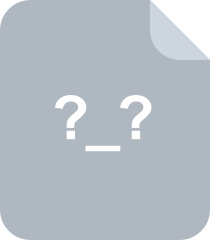
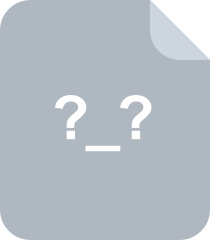
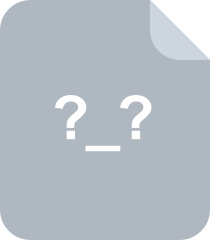
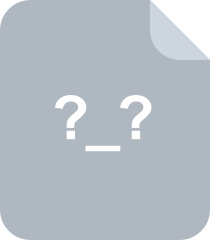
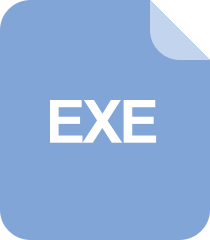
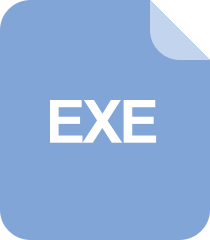
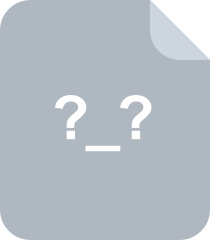
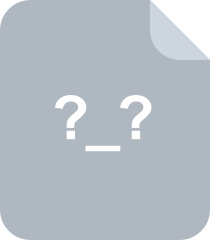
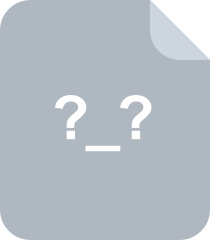
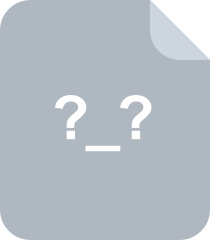
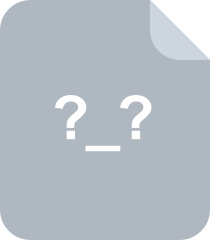
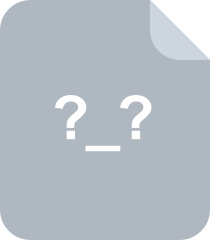
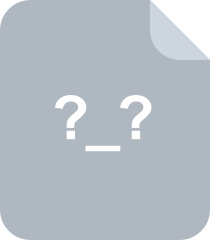
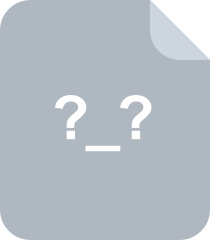
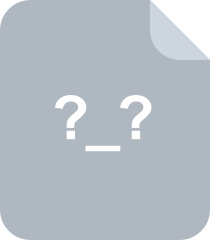
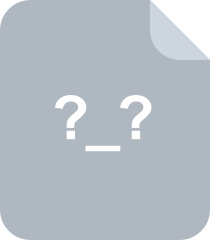
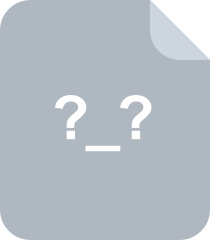
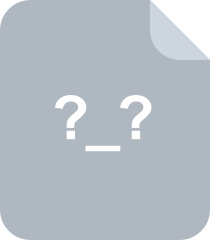
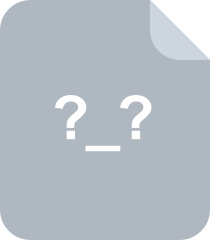
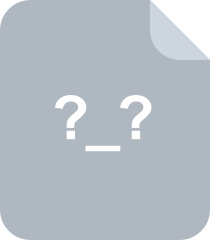
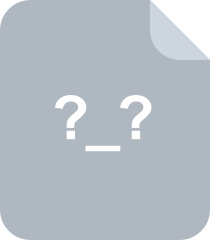
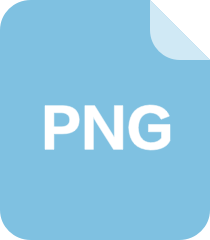
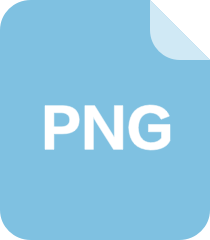
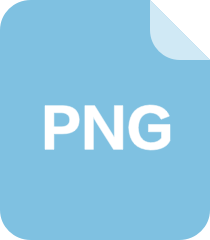
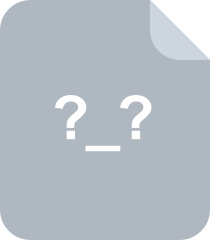
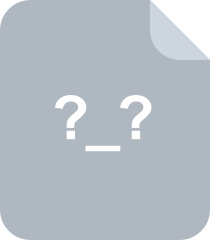
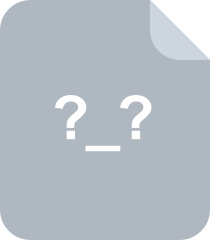
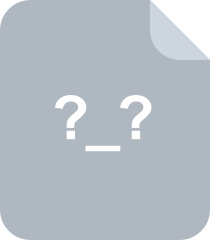
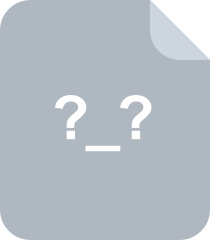
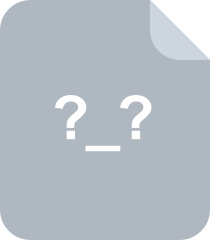
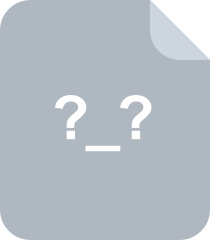
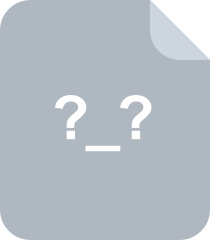
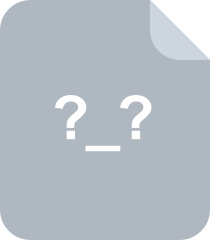
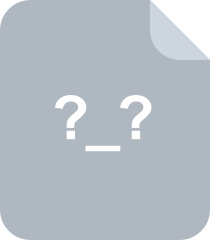
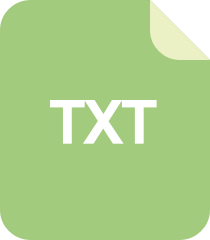
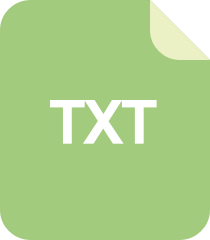
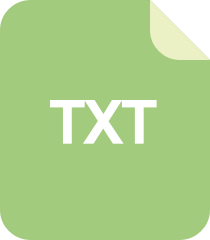
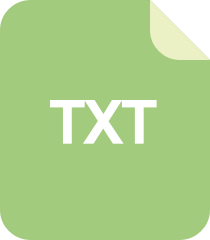
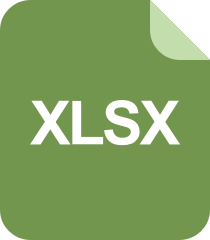
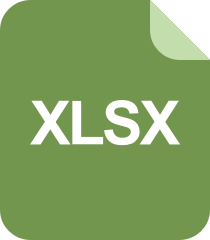
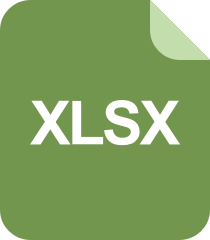
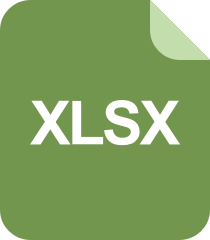
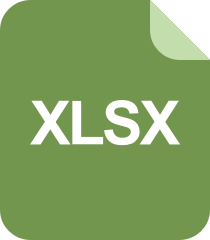
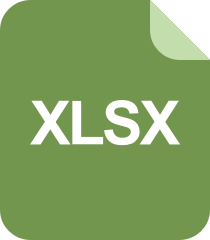
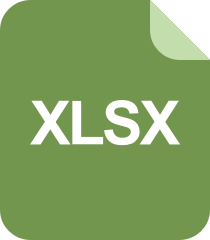
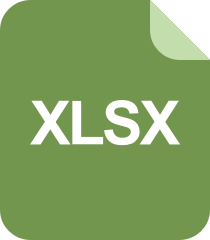
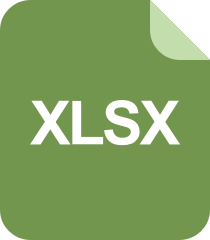
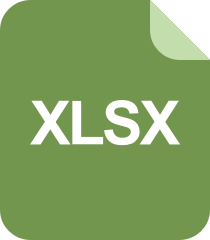
共 114 条
- 1
- 2
资源评论
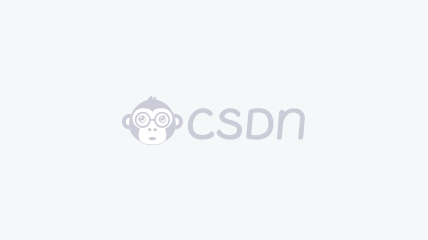

等天晴i
- 粉丝: 5923
- 资源: 10万+

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

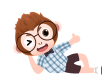
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


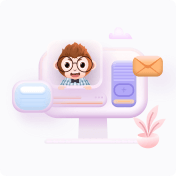
安全验证
文档复制为VIP权益,开通VIP直接复制
