/**
*
*/
package edu.cst.aoot.project.maze;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Random;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Paint;
import edu.cst.aoot.project.common.Point;
/**
* @author ZuoJingchao
*
*/
public class MazeMap {
private int mCellCount;
private Random mRandom = null;
private List<Cell> visitedCells = null;
public Cell map[][] = null;
// width and height in mm
private float cellWidth;
private float cellHeight;
private int rowCount;
private int columnCount;
private Point firstRowStartPoint;
public MazeMap(int rowCount, int columnCount, float cellWidth,
float cellHeight) {
// TODO Auto-generated constructor stub
this.rowCount = rowCount;
this.columnCount = columnCount;
this.cellWidth = cellWidth;
this.cellHeight = cellHeight;
mCellCount = rowCount * columnCount;
mRandom = new Random(System.currentTimeMillis());
visitedCells = new ArrayList<Cell>();
map = new Cell[rowCount][columnCount];
firstRowStartPoint = new Point(1.0f, 1.0f);
}
public void prepareGame(){
initMap();
randomMazeMap();
map[0][0].setHasWall(Direction.TOP, false);
map[rowCount-1][columnCount -1].setHasWall(Direction.BOTTOM, false);
}
/**
* Initialise the maze map
*/
private void initMap() {
// 4 corner points of the first cell in the first row
Point leftUpPoint = firstRowStartPoint;
Point leftBottomPoint = new Point(leftUpPoint.getmPosX(), leftUpPoint
.getmPosY()
+ cellHeight);
Point rightUpPoint = new Point(leftUpPoint.getmPosX() + cellWidth,
leftUpPoint.getmPosY());
Point rightBottomPoint = new Point(leftBottomPoint.getmPosX()
+ cellWidth, leftBottomPoint.getmPosY());
for (int row = 0; row < rowCount; row++) {
// 2 corner points of the first cell in next row
Point nextRowLeftUpPoint = leftBottomPoint;
Point nextRowRightUpPoint = rightBottomPoint;
for (int column = 0; column < columnCount; column++) {
Cell cell = new Cell(row, column, leftUpPoint, rightUpPoint,
rightBottomPoint, leftBottomPoint, true);
// 4 corner points of next cell in the same row
leftUpPoint = rightUpPoint;
leftBottomPoint = rightBottomPoint;
rightUpPoint = new Point(leftUpPoint.getmPosX() + cellWidth,
leftUpPoint.getmPosY());
rightBottomPoint = new Point(leftBottomPoint.getmPosX()
+ cellWidth, leftBottomPoint.getmPosY());
map[row][column] = cell;
}
// 4 corner points of the first cell in next row
leftUpPoint = nextRowLeftUpPoint;
rightUpPoint = nextRowRightUpPoint;
leftBottomPoint = new Point(leftUpPoint.getmPosX(), leftUpPoint
.getmPosY()
+ cellHeight);
rightBottomPoint = new Point(
leftBottomPoint.getmPosX() + cellWidth, leftBottomPoint
.getmPosY());
}
}
/**
* generate a random Maze Map
*/
private void randomMazeMap() {
// if all the cell have been visited, then return
Cell theCell = map[mRandom.nextInt(rowCount)][mRandom
.nextInt(columnCount)];
while (visitedCells.size() != mCellCount) {
if (!theCell.isVisited()) {
theCell.setVisited(true);
visitedCells.add(theCell);
}
// get not visited neighbours
int neibCount = 0;
Cell[] neighbours = new Cell[4];
// left
Cell nextCell;
if (theCell.getCol() - 1 >= 0
&& !(nextCell = map[theCell.getRow()][theCell.getCol() - 1])
.isVisited()) {
neighbours[neibCount++] = nextCell;
}
// top
if (theCell.getRow() - 1 >= 0
&& !(nextCell = map[theCell.getRow() - 1][theCell.getCol()])
.isVisited()) {
neighbours[neibCount++] = nextCell;
}
// right
if (theCell.getCol() + 1 < columnCount
&& !(nextCell = map[theCell.getRow()][theCell.getCol() + 1])
.isVisited()) {
neighbours[neibCount++] = nextCell;
}
// bottom
if (theCell.getRow() + 1 < rowCount
&& !(nextCell = map[theCell.getRow() + 1][theCell.getCol()])
.isVisited()) {
neighbours[neibCount++] = nextCell;
}
// if all the neibours have been visited
if (neibCount == 0) {
theCell = visitedCells
.get(mRandom.nextInt(visitedCells.size()));
continue;
}
// random access next cell
nextCell = neighbours[mRandom.nextInt(neibCount)];
// delete the wall between two cells
// neighbour left
if (nextCell.getCol() < theCell.getCol()) {
nextCell.setHasWall(Direction.RIGHT, false);
theCell.setHasWall(Direction.LEFT, false);
}
// neighbour top
else if (nextCell.getRow() < theCell.getRow()) {
nextCell.setHasWall(Direction.BOTTOM, false);
theCell.setHasWall(Direction.TOP, false);
}
// neighbour right
else if (nextCell.getCol() > theCell.getCol()) {
nextCell.setHasWall(Direction.LEFT, false);
theCell.setHasWall(Direction.RIGHT, false);
}
// neighbour bottom
else if (nextCell.getRow() > theCell.getRow()) {
nextCell.setHasWall(Direction.TOP, false);
theCell.setHasWall(Direction.BOTTOM, false);
}
// prepare the next loop inviable
theCell = nextCell;
}
}
/**
*
* @param canvas
* @param paint
*/
public void paintMaze(Canvas canvas, Paint paint) {
for (int row = 0; row < rowCount; row++)
for (int colomn = 0; colomn < columnCount; colomn++)
map[row][colomn].drawCell(canvas, paint);
}
private List<Cell> findPath() {
Cell cell;
for (int row = 0; row < rowCount; row++)
for (int column = 0; column < columnCount; column++) {
cell = map[row][column];
cell.setVisited(false);
}
List<Cell> list = new ArrayList<Cell>();
list.add(map[0][0]);
Cell topCell = list.get(list.size() - 1);
while (topCell != map[rowCount - 1][columnCount - 1]) {
int neibCount = 0;
Cell[] neighbours = new Cell[4];
// if the 2 adjacent cells have no walls
// left
Cell nextCell;
if (topCell.getCol() - 1 >= 0
&& !(nextCell = map[topCell.getRow()][topCell.getCol() - 1])
.isVisited() && !topCell.hasWall(Direction.LEFT)) {
neighbours[neibCount++] = nextCell;
}
// top
if (topCell.getRow() - 1 >= 0
&& !(nextCell = map[topCell.getRow() - 1][topCell.getCol()])
.isVisited() && !topCell.hasWall(Direction.TOP)) {
neighbours[neibCount++] = nextCell;
}
// right
if (topCell.getCol() + 1 < columnCount
&& !(nextCell = map[topCell.getRow()][topCell.getCol() + 1])
.isVisited() && !topCell.hasWall(Direction.RIGHT)) {
neighbours[neibCount++] = nextCell;
}
// bottom
if (topCell.getRow() + 1 < rowCount
&& !(nextCell = map[topCell.getRow() + 1][topCell.getCol()])
.isVisited() && !topCell.hasWall(Direction.BOTTOM)) {
neighbours[neibCount++] = nextCell;
}
// if all the neighbours have been visited
if (neibCount == 0) {
list.remove(list.size() - 1);
// loop invariable
topCell = list.get(list.size() - 1);
continue;
}
// random access next cell
neighbours[0].setVisited(true);
list.add(neighbours[0]);
// loop invariable
topCell = list.get(list.size() - 1);
}
return list;
}
/**
* paint the path.
*
* @param canvas
* @param paint
*/
public void paintPath(Canvas canvas, Paint paint) {
paint.setColor(Color.RED);
List<Cell> list = findPath();
Cell cell1 = null, cell2 = null;
Iterator<Cell> i = list.iterator();
if (i.hasNext())
cell1 = i.next();
while (i.hasNext()) {
cell2 = i.next();
canvas.drawLine(cell1.getmCenterPoint().getmPosX(), cell1
.getmCenterPoint().getmPosY(), cell2.getmCenterPoint()
.getmPosX(), cell2.getmCenterPoint().getmPosY(), paint);
cell1 = cell2;
}
}
/**
* @param firstRowStartPoint
* the startPoint to set
*/
pu
没有合适的资源?快使用搜索试试~ 我知道了~
android 迷宫游戏设计.7z
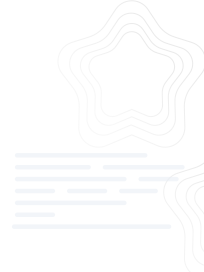
共129个文件
html:67个
class:24个
java:14个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 139 浏览量
2022-03-31
20:29:54
上传
评论
收藏 4.83MB 7Z 举报
温馨提示
Android手机正在迅速的普及,并且大部分都已配备重力感应系统。传统的迷宫游戏靠按键来控制角色的移动,虽然这些迷宫游戏无论是地图还是操作都已比较成熟,但运行在Android平台上无法展示出Android重力感应的优势。另一方面,运行在Android上的迷宫还很少见。
资源推荐
资源详情
资源评论
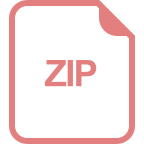
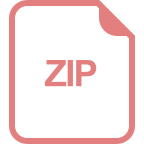
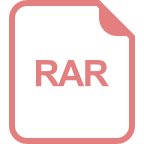
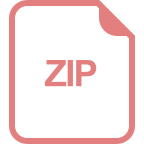
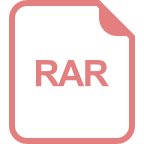
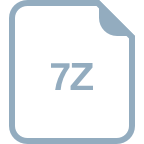
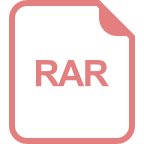
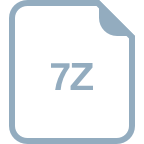
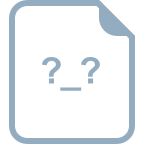
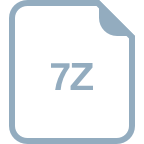
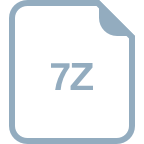
收起资源包目录

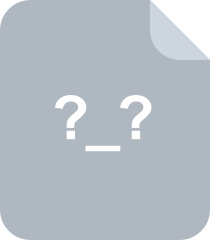
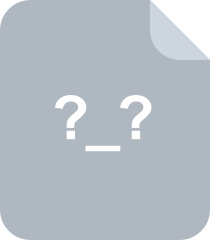
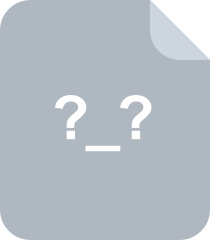
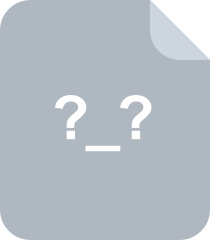
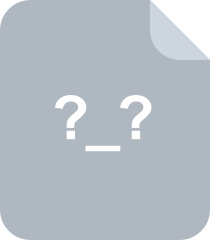
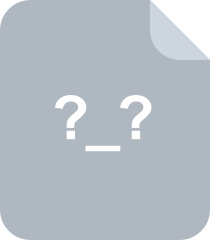
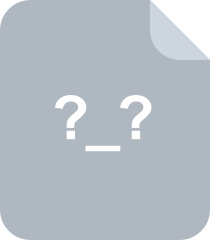
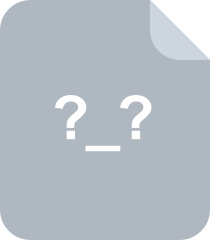
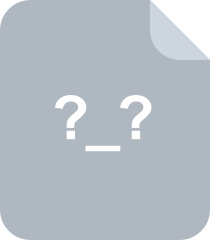
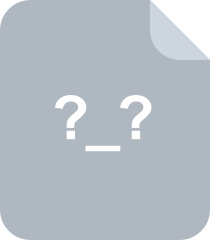
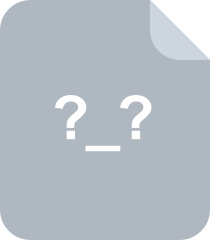
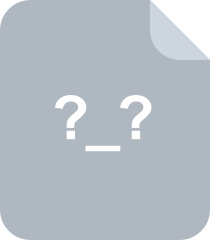
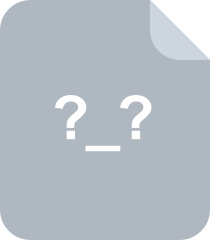
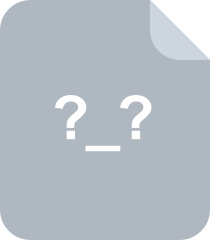
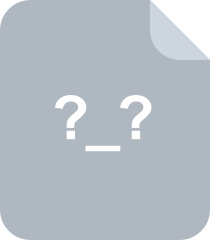
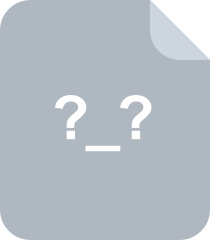
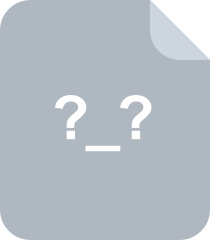
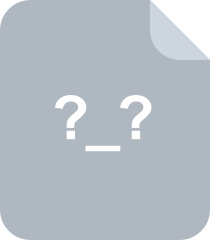
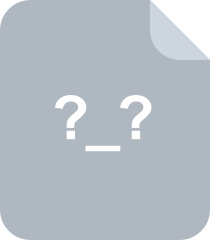
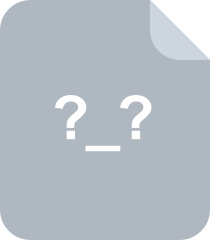
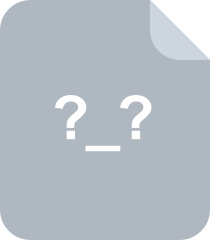
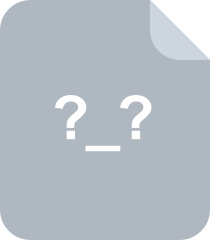
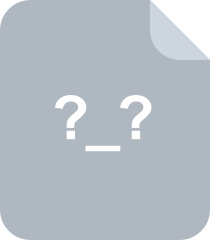
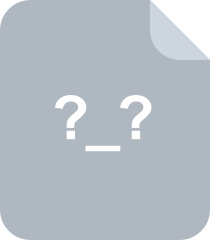
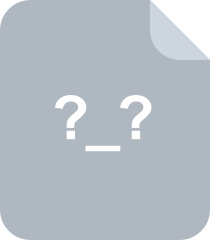
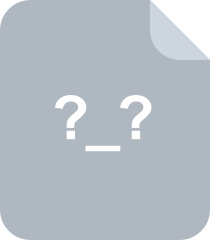
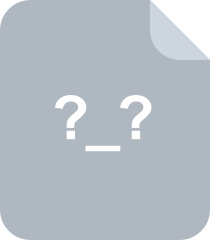
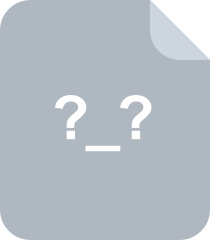
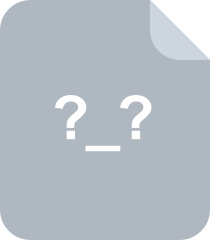
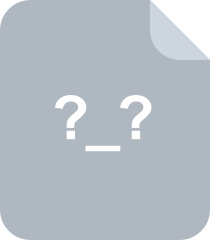
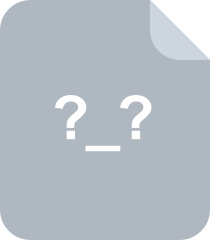
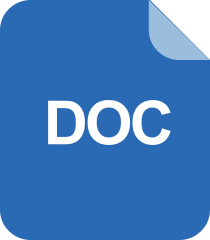
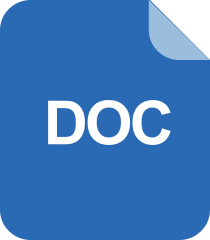
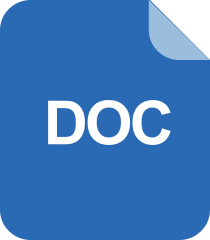
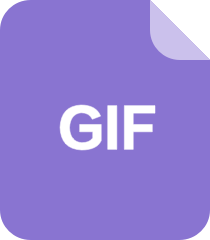
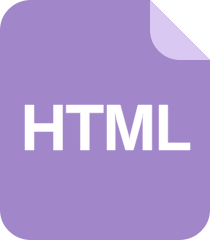
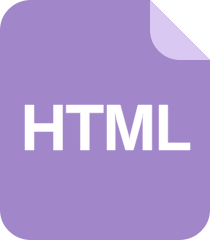
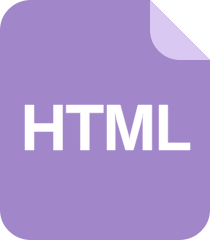
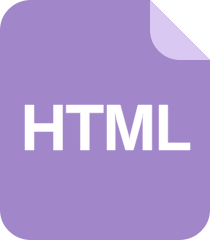
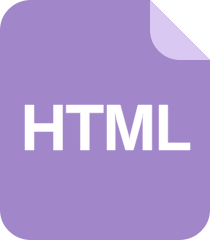
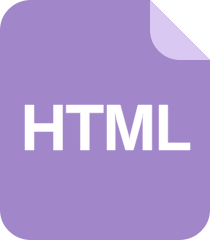
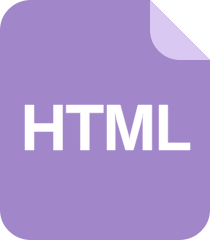
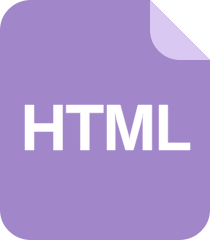
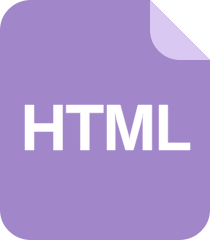
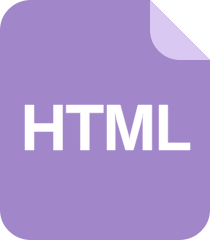
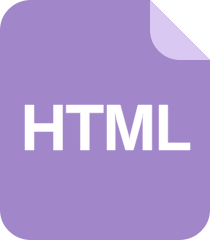
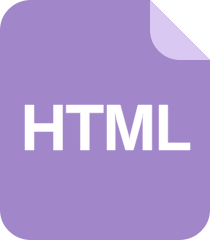
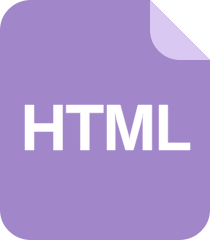
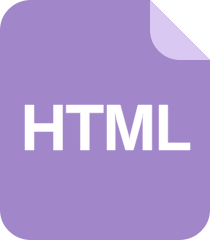
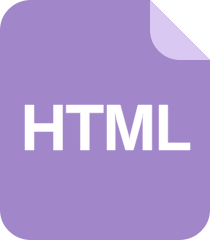
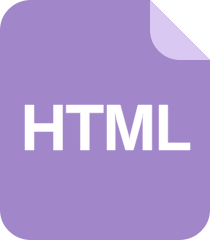
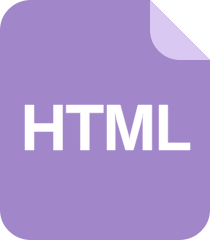
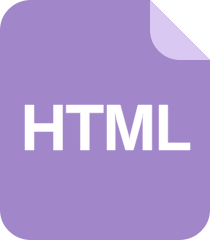
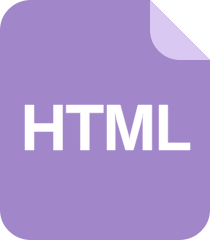
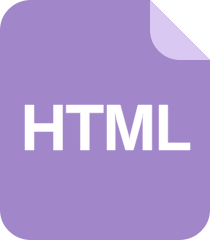
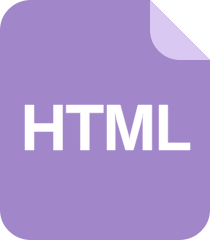
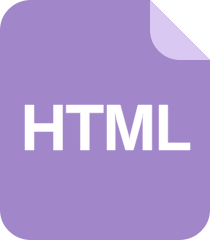
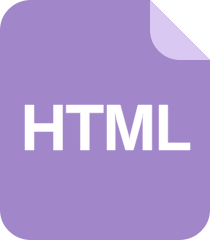
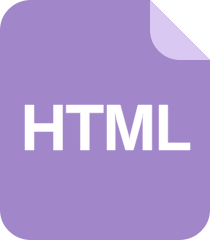
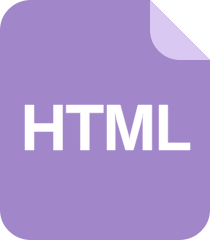
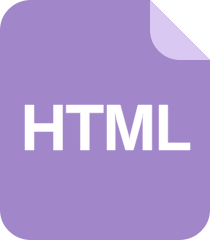
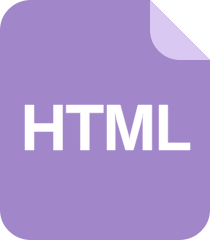
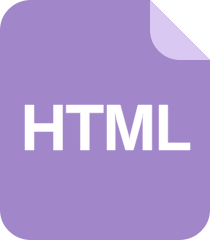
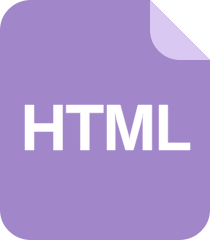
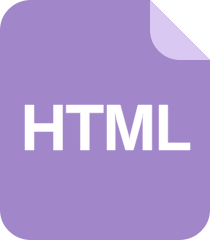
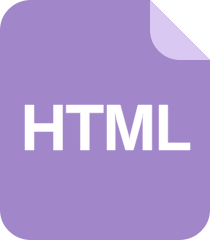
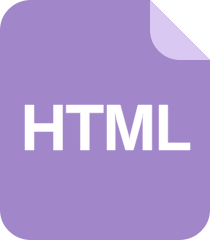
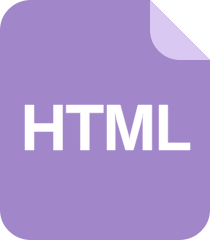
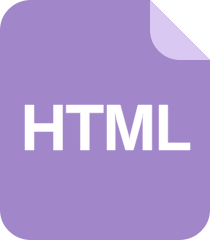
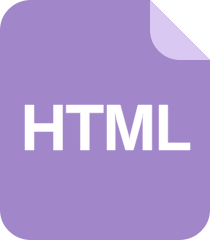
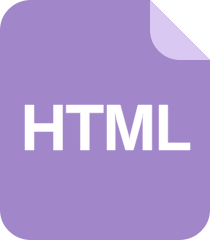
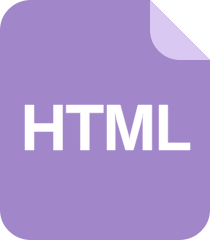
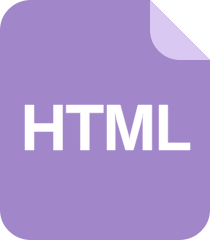
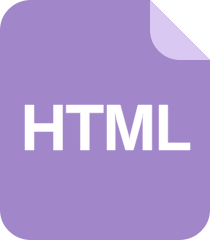
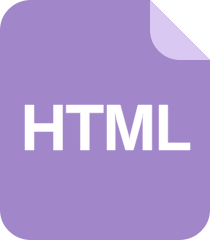
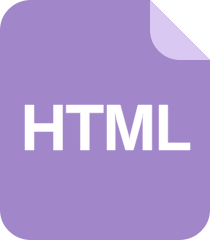
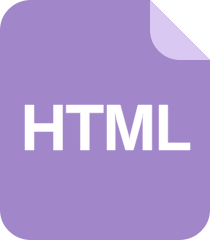
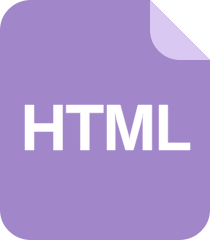
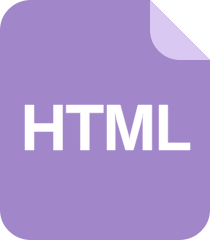
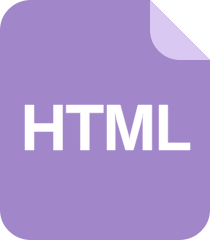
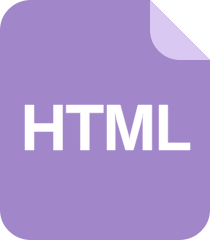
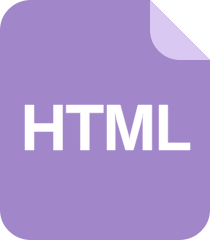
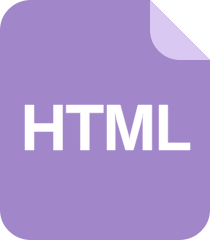
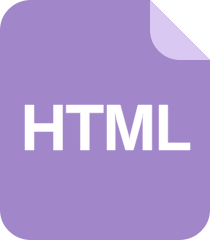
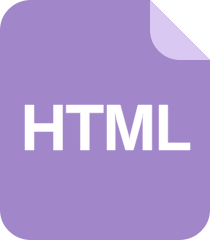
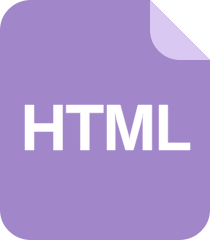
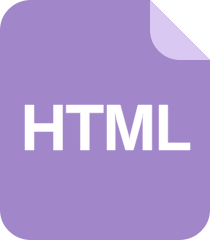
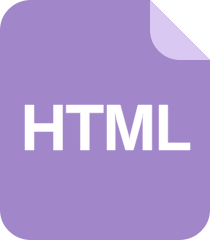
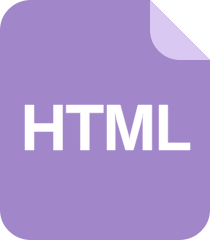
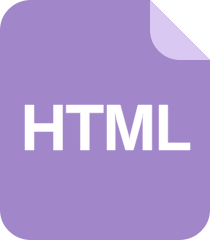
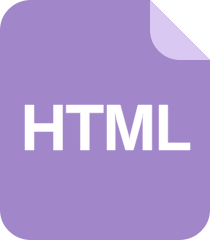
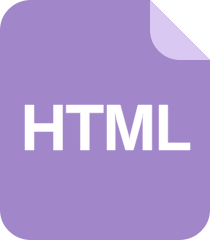
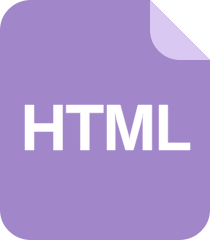
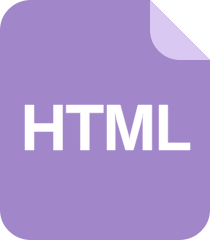
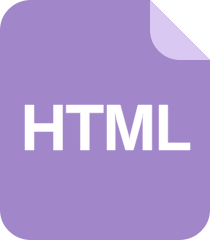
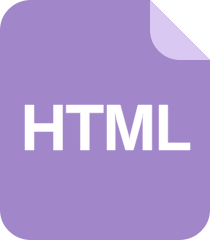
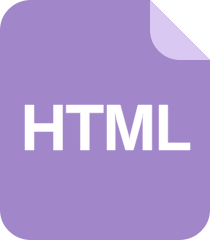
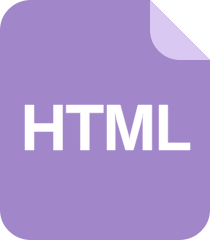
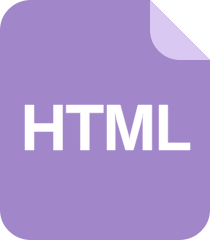
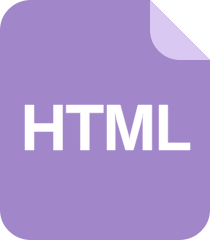
共 129 条
- 1
- 2
资源评论
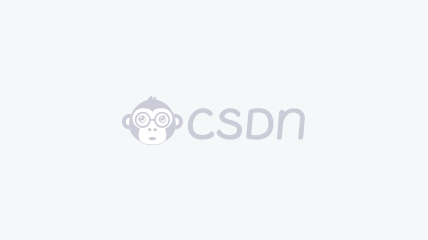

等天晴i
- 粉丝: 5848
- 资源: 10万+

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

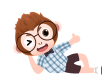
最新资源
- 统一平台 mes 管理系统 vue
- 开心消消乐【python实战小游戏】学习开发路上的最好实战教程.zip
- 利用Gurobi求解工厂生产规划问题代码
- 华为HCIE考试文档.zip
- 2010-2023英语二小作文真题范文.pdf
- bpm 流程管理系统 vue2
- C#ASP.NET视频会议OA源码+手机版OA源码带二次开发文档数据库 SQL2008源码类型 WebForm
- django旅游服务系统程序源码88939
- 【安卓毕业设计】图书管理系统安卓修改源码(完整前后端+mysql+说明文档).zip
- 【安卓毕业设计】基于安卓平台学生课堂质量采集分析查询系统源码(完整前后端+mysql+说明文档).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


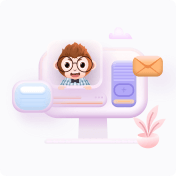
安全验证
文档复制为VIP权益,开通VIP直接复制
