import javax.swing.JFrame;
import javax.swing.JPanel;
import java.awt.BorderLayout;
import java.awt.Dimension;
import javax.swing.JTabbedPane;
import java.awt.Rectangle;
import java.awt.GridBagLayout;
import java.awt.GridBagConstraints;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import java.util.Calendar;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JButton;
import javax.swing.JLabel;
import javax.swing.JTextField;
import javax.swing.JComboBox;
import javax.swing.JPasswordField;
import javax.swing.JTextArea;
public class Adminfrm extends JFrame implements ActionListener {
//
private JFrame jFrame = null; // @jve:decl-index=0:visual-constraint="53,6"
private JPanel jContentPane = null;
private JTabbedPane jTabbedPane = null;
private JPanel jPanel = null;
private JPanel jPanel1 = null;
private JPanel jPanel2 = null;
private JPanel jPanel3 = null;
private JPanel jPanel4 = null;
private JTabbedPane jTabbedPane1 = null;
private JPanel jPanel6 = null;
private JPanel jPanel7 = null;
private JScrollPane jScrollPane = null;
private JTable jTable = null;
private JButton jButton = null;
private JLabel jLabel = null;
private JLabel jLabel1 = null;
private JLabel jLabel2 = null;
private JLabel jLabel3 = null;
private JLabel jLabel4 = null;
private JLabel jLabel5 = null;
public static Object rowdata[][]; // @jve:decl-index=0:
public static JTextField jTextField = null;
public static JTextField jTextField1 = null;
public static JTextField jTextField2 = null;
public static JTextField jTextField3 = null;
public static JTextField jTextField4 = null;
private JComboBox jComboBox = null;
private JButton jButton1 = null;
private JButton jButton2 = null;
private JTabbedPane jTabbedPane2 = null;
private JPanel jPanel8 = null;
private JPanel jPanel9 = null;
private JScrollPane jScrollPane1 = null;
private JTable jTable1 = null;
private JButton jButton3 = null;
private JLabel jLabel6 = null;
private JLabel jLabel7 = null;
private JLabel jLabel8 = null;
private JLabel jLabel9 = null;
private JComboBox jComboBox1 = null;
private JLabel jLabel10 = null;
public static JTextField jTextField5 = null;
public static JTextField jTextField7 = null;
public static JTextField jTextField8 = null;
private JButton jButton4 = null;
private JButton jButton5 = null;
private JButton jButton6 = null;
public static Object[] columnNames = {"�鼮���", "����", "����",
"������", "����", "����ʱ��","��������","�ڹ���Ŀ","�ع�"};
public static Object rowdata1[][];
public static Object[] columnNames1 = {"����","Ȩ��", "����", "�Ա�",
"������¼", "����"};
public static Object rowdata2[][];
public static Object[] columnNames2 = {"������","ѧ�����", "��������",
"��������", "�Ƿ���Ч"};
public static Object rowdata3[][];
public static Object[] columnNames3 = {"Υ�±��","������", "Υ������"};
public static JPasswordField jPasswordField = null;
private JLabel jLabel11 = null;
public static JPasswordField jPasswordField1 = null;
private JScrollPane jScrollPane2 = null;
private JTable jTable2 = null;
private JButton jButton7 = null;
private JTabbedPane jTabbedPane3 = null;
private JPanel jPanel10 = null;
private JPanel jPanel11 = null;
private JScrollPane jScrollPane3 = null;
private JTable jTable3 = null;
private JButton jButton8 = null;
private JLabel jLabel12 = null;
private JLabel jLabel13 = null;
public static JTextField jTextField6 = null;
public static JTextField jTextField9 = null;
private JButton jButton9 = null;
private JLabel jLabel14 = null;
private JLabel jLabel15 = null;
public static JTextField jTextField10 = null;
public static JTextField jTextField11 = null;
private JLabel jLabel16 = null;
public static JTextArea jTextArea = null;
private JButton jButton10 = null;
/**
* This is the default constructor
*/
public Adminfrm() {
super();
initialize();
}
/**
* This method initializes this
*
* @return void
*/
private void initialize() {
this.setSize(new Dimension(593, 424));
this.setContentPane(getJContentPane());
this.setTitle("����Ա����");
//this.setLocation(250,80);
this.addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent e) {
System.exit(0);
}
});
}
/**
* This method initializes jFrame
*
* @return javax.swing.JFrame
*/
private JFrame getJFrame() {
if (jFrame == null) {
jFrame = new JFrame();
jFrame.setSize(new Dimension(606, 425));
jFrame.setTitle("����Ա����");
jFrame.setContentPane(getJContentPane());
}
return jFrame;
}
/**
* This method initializes jContentPane
*
* @return javax.swing.JPanel
*/
private JPanel getJContentPane() {
if (jContentPane == null) {
GridBagConstraints gridBagConstraints = new GridBagConstraints();
gridBagConstraints.fill = GridBagConstraints.BOTH;
gridBagConstraints.gridy = 0;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.weighty = 1.0;
gridBagConstraints.gridx = 0;
jContentPane = new JPanel();
jContentPane.setLayout(new GridBagLayout());
jContentPane.add(getJTabbedPane(), gridBagConstraints);
}
return jContentPane;
}
/**
* This method initializes jTabbedPane
*
* @return javax.swing.JTabbedPane
*/
private JTabbedPane getJTabbedPane() {
if (jTabbedPane == null) {
jTabbedPane = new JTabbedPane();
jTabbedPane.setBounds(new Rectangle(1, 0, 734, 461));
jTabbedPane.addTab("�鿯����", null, getJPanel(), null);
jTabbedPane.addTab("�û�����", null, getJPanel1(), null);
jTabbedPane.addTab("������", null, getJPanel2(), null);
jTabbedPane.addTab("ԤԼ�б�", null, getJPanel3(), null);
jTabbedPane.addTab("Υ���б�", null, getJPanel4(), null);
}
return jTabbedPane;
}
/**
* This method initializes jPanel
*
* @return javax.swing.JPanel
*/
private JPanel getJPanel() {
if (jPanel == null) {
GridBagConstraints gridBagConstraints1 = new GridBagConstraints();
gridBagConstraints1.fill = GridBagConstraints.BOTH;
gridBagConstraints1.gridy = 0;
gridBagConstraints1.weightx = 1.0;
gridBagConstraints1.weighty = 1.0;
gridBagConstraints1.gridx = 0;
jPanel = new JPanel();
jPanel.setLayout(new GridBagLayout());
jPanel.add(getJTabbedPane1(), gridBagConstraints1);
}
return jPanel;
}
/**
* This method initializes jPanel1
*
* @return javax.swing.JPanel
*/
private JPanel getJPanel1() {
if (jPanel1 == null) {
GridBagConstraints gridBagConstraints2 = new GridBagConstraints();
gridBagConstraints2.fill = GridBagConstraints.BOTH;
gridBagConstraints2.gridy = 0;
gridBagConstraints2.weightx = 1.0;
gridBagConstraints2.weighty = 1.0;
gridBagConstraints2.gridx = 0;
jPanel1 = new JPanel();
jPanel1.setLayout(new GridBagLayout());
jPanel1.add(getJTabbedPane2(), gridBagConstraints2);
}
return jPanel1;
}
/**
* This method initializes jPanel2
*
* @return javax.swing.JPanel
*/
private JPanel getJPanel2() {
if (jPanel2 == null) {
GridBagConstraints gridBagConstraints3 = new GridBagConstraints();
gridBagConstraints3.fill = GridBagConstraints.BOTH;
gridBagConstraints3.gridy = 0;
gridBagConstraints3.weightx = 1.0;
gridBagConstraints3.weighty = 1.0;
gridBagConstraints3.gridx = 0;
jPanel2 = new JPanel
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
功能 图书管理:包括图书分类、入库、借阅、归还等功能; 读者管理:对读者信息进行管理,包括读者登记、读者信息修改、读者注销等; 借阅管理:对读者借阅记录进行管理,包括借书、还书等功能; 罚款管理:对逾期未还书籍的读者进行罚款管理; 统计分析:对各类信息进行统计分析。 实现方式 使用Java语言编写程序; 使用JavaSwing图形界面开发前端界面; 设计数据库表结构,使用SQLserver存储数据; 使用Java代码实现各模块的功能,包括增删改查等; 界面友好美观:采用JavaSwing图形界面设计前端页面,具有良好的视觉效果,易于操作。 功能全面:系统具有图书管理、读者管理、借阅管理、罚款管理等功能,能够方便地管理图书馆日常运营。 权限管理完善:系统支持管理员和读者两种权限,只有管理员才能进行数据修改和管理操作。 数据备份及恢复:系统具备数据备份和恢复功能,确保数据的安全性和可靠性。 扩展功能:可以按照自己的程度进行扩展。比如(1)简单的权限处理 (2)报表打印功能(3)甚至根据自己情况,可以加上学生信息,并扩充为图书借阅系统。(4)模糊查询 (5)综合查询 (6)统计功能 比如统计
资源推荐
资源详情
资源评论
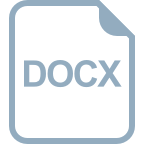
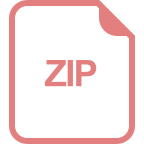
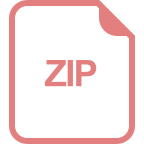
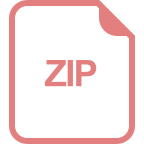
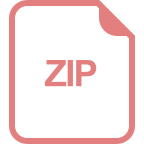
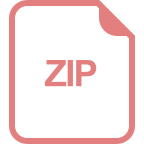
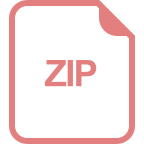
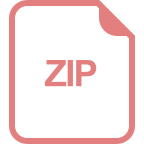
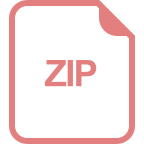
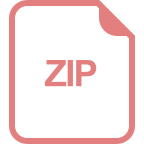
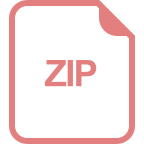
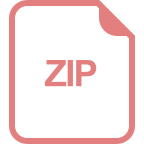
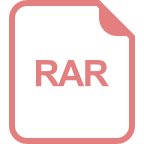
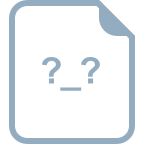
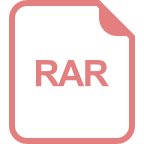
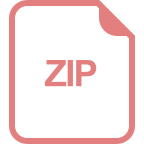
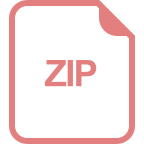
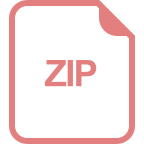
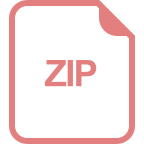
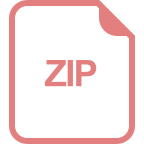
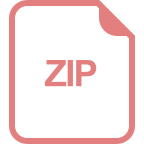
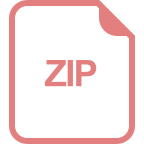
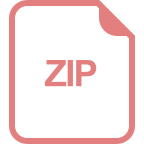
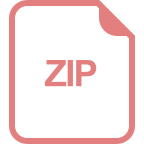
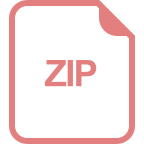
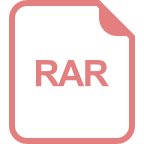
收起资源包目录


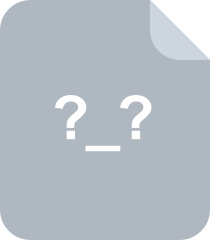

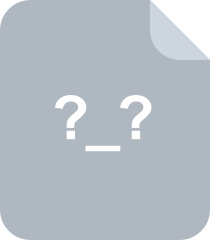
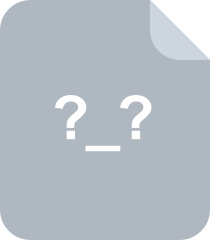
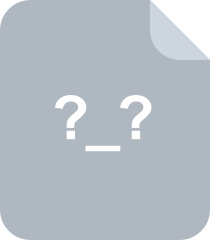
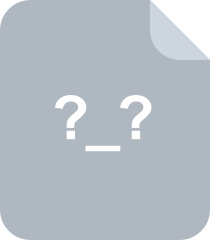
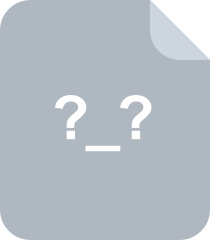
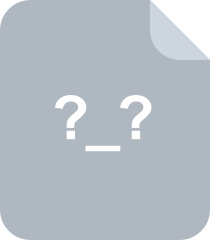

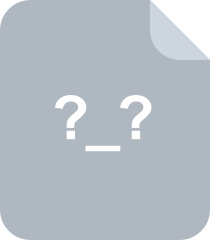
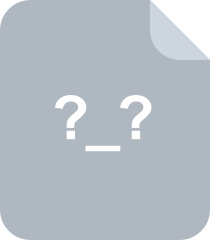
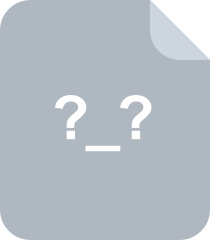
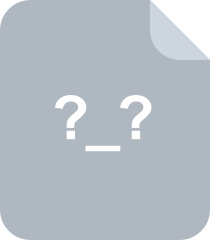
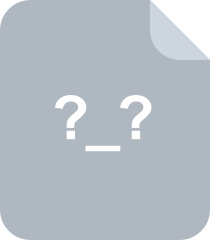
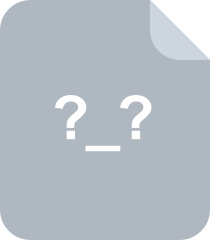

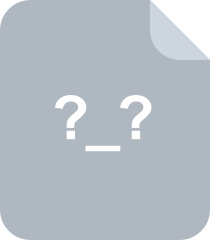
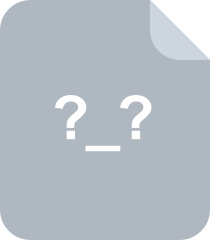
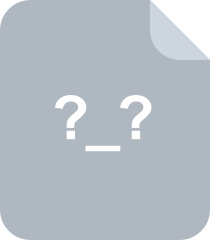
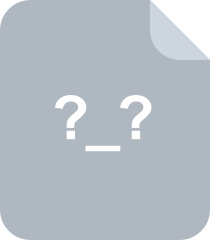
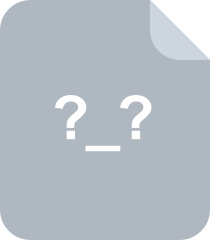
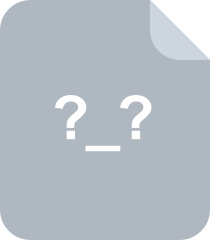
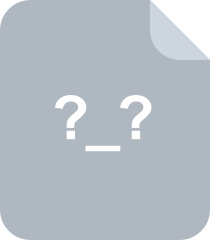
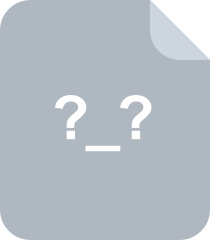
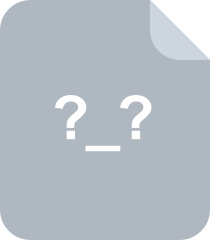
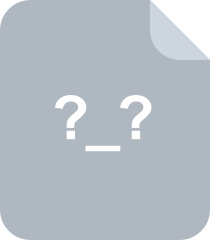
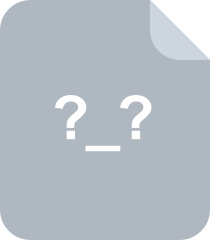
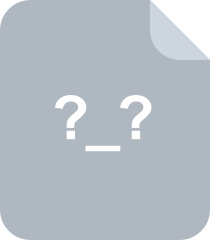
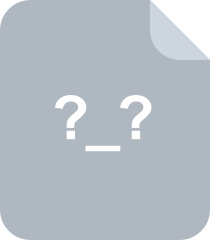
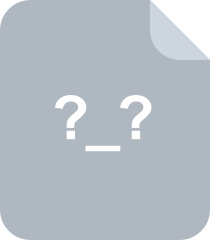
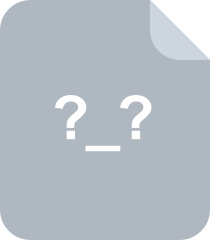
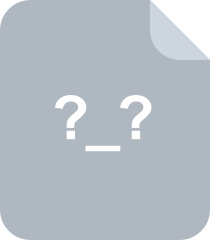
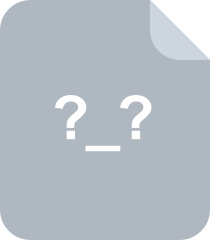
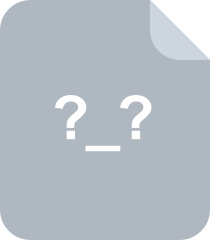
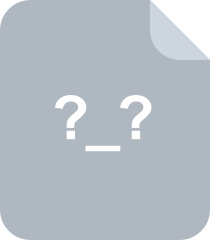
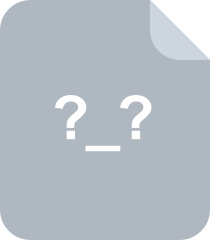
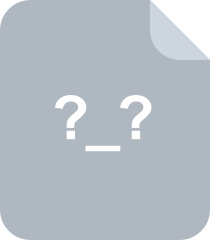
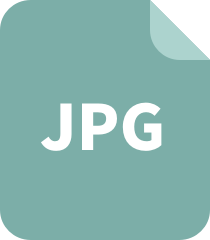
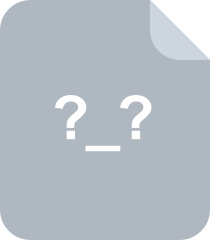
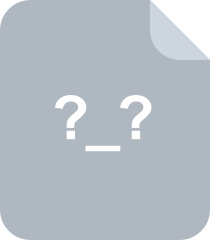
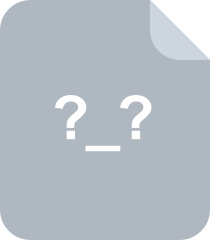
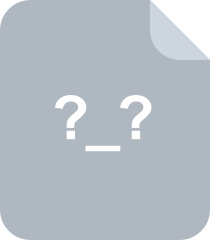
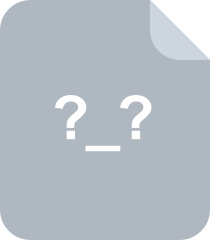
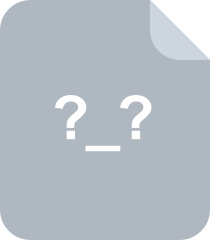
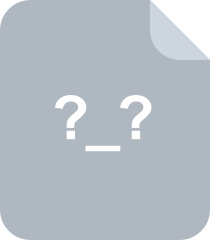
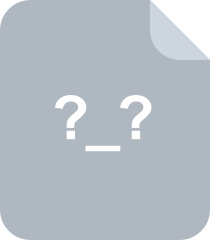
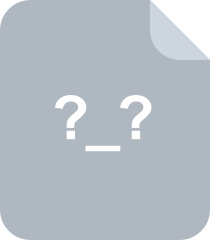
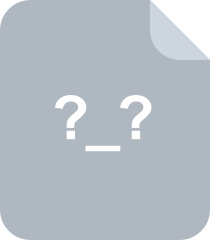
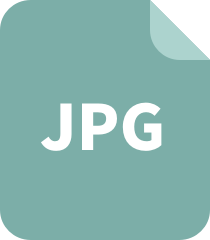
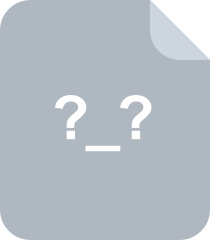
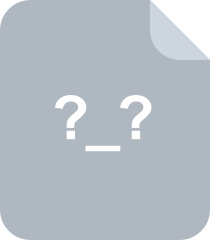
共 48 条
- 1


荒野大飞
- 粉丝: 1w+
- 资源: 2685

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

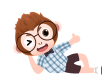
最新资源
- 5A90铝锂合金电子束焊接接头显微分析 - .pdf
- 5E83铝合金TIG焊接头残余应力分布研究 - .pdf
- 5万m-3LNG储罐9 Ni钢内罐焊接技术.pdf
- 6mm不锈钢对接焊接接头超声检测探讨.pdf
- 07MnNiVDR钢焊接热影响区再热脆化研究 - .pdf
- 07钢管相贯节点焊接缺陷类型及对极限承载力的影响.pdf
- 7A05铝合金激光-MIG复合焊接头组织分析 - .pdf
- 08Cr2AIMoSi板材焊接冷裂纹敏感性及焊后热处理试验.pdf
- 7N01-T4铝合金搅拌摩擦焊接头的组织和耐应力腐蚀性能 - .pdf
- 7N01铝合金焊接接头力学性能及静/动态失效分析 - .pdf
- 08采用冷轧带肋钢筋焊接网的抗震墙性能研究.pdf
- 8轴激光焊接实验平台的有限元分析及优化设计.pdf
- 09CrCuSb钢焊接材料选定及其焊接工艺 - .pdf
- 09MnNiDR低温钢的焊接工艺评定.pdf
- 09MnNiDR低温容器的焊接及热处理研究 - .pdf
- 09MnNiDR低温钢焊接性分析 - .pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


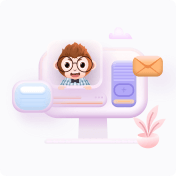
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页