package com.example.phonecontacts;
import android.app.Activity;
import android.content.Context;
import android.content.DialogInterface;
import android.content.Intent;
import android.net.Uri;
import android.telephony.SmsManager;
import android.view.LayoutInflater;
import android.view.MenuItem;
import android.view.View;
import android.view.ViewGroup;
import android.widget.EditText;
import android.widget.LinearLayout;
import android.widget.PopupMenu;
import android.widget.TextView;
import android.widget.Toast;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AlertDialog;
import androidx.recyclerview.widget.RecyclerView;
import com.example.phonecontacts.util.MyDatabaseHelper;
import java.util.ArrayList;
public class CustomAdapter extends RecyclerView.Adapter<CustomAdapter.MyViewHolder> {
private Context context;
Activity activity;
EditText pt_name1, pt_phone1, pt_address1, pt_unit1, pt_email1, pt_qq1;
private ArrayList phone_id, phone_name, phone_phone, phone_address,
phone_unit, phone_email, phone_qq;
CustomAdapter(Activity activity,
Context context,
ArrayList phone_id,
ArrayList phone_name,
ArrayList phone_phone,
ArrayList phone_address,
ArrayList phone_unit,
ArrayList phone_email,
ArrayList phone_qq) {
this.activity = activity;
this.context = context;
this.phone_id = phone_id;
this.phone_name = phone_name;
this.phone_phone = phone_phone;
this.phone_address = phone_address;
this.phone_unit = phone_unit;
this.phone_email = phone_email;
this.phone_qq = phone_qq;
}
@NonNull
@Override
public MyViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
LayoutInflater inflater = LayoutInflater.from(context);
View view = inflater.inflate(R.layout.my_row, parent, false);
return new MyViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull MyViewHolder holder, final int position) {
holder.phone_id_txt.setText(String.valueOf(phone_id.get(position)));
holder.phone_name_txt.setText(String.valueOf(phone_name.get(position)));
holder.phone_phone_txt.setText(String.valueOf(phone_phone.get(position)));
holder.phone_address_txt.setText(String.valueOf(phone_address.get(position)));
holder.mainLayout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(context, UpdataActivity.class);
intent.putExtra("id", String.valueOf(phone_id.get(position)));
intent.putExtra("name", String.valueOf(phone_name.get(position)));
intent.putExtra("phone", String.valueOf(phone_phone.get(position)));
intent.putExtra("address", String.valueOf(phone_address.get(position)));
intent.putExtra("unit", String.valueOf(phone_unit.get(position)));
intent.putExtra("email", String.valueOf(phone_email.get(position)));
intent.putExtra("qq", String.valueOf(phone_qq.get(position)));
activity.startActivityForResult(intent, 1);
}
});
//长按事件
holder.mainLayout.setOnLongClickListener(new View.OnLongClickListener() {
@Override
public boolean onLongClick(View view) {
myPopupMenu(view, position);
return false;
}
});
}
@Override
public int getItemCount() {
return phone_id.size();
}
public class MyViewHolder extends RecyclerView.ViewHolder {
TextView phone_id_txt, phone_name_txt, phone_phone_txt, phone_address_txt;
LinearLayout mainLayout;
public MyViewHolder(@NonNull View itemView) {
super(itemView);
phone_id_txt = itemView.findViewById(R.id.phone_id_txt);
phone_name_txt = itemView.findViewById(R.id.phone_name_txt);
phone_phone_txt = itemView.findViewById(R.id.phone_phone_txt);
phone_address_txt = itemView.findViewById(R.id.phone_address_txt);
pt_name1 = itemView.findViewById(R.id.pt_name1);
pt_phone1 = itemView.findViewById(R.id.pt_phone1);
pt_address1 = itemView.findViewById(R.id.pt_address1);
pt_unit1 = itemView.findViewById(R.id.pt_unit1);
pt_email1 = itemView.findViewById(R.id.pt_email1);
pt_qq1 = itemView.findViewById(R.id.pt_qq1);
mainLayout = itemView.findViewById(R.id.mainLayout);
}
}
private void myPopupMenu(View v, final int position) {
//创建一个对象
final PopupMenu popupMenu = new PopupMenu(context, v);
//设置布局
popupMenu.getMenuInflater().inflate(R.menu.my_menu1, popupMenu.getMenu());
//设置点击事件
popupMenu.setOnMenuItemClickListener(new PopupMenu.OnMenuItemClickListener() {
@Override
public boolean onMenuItemClick(MenuItem menuItem) {
Toast.makeText(context, "你点击了:" + menuItem.getTitle(), Toast.LENGTH_SHORT).show();
// 当你点击拨打电话,跳转拨打电话
if (menuItem.getTitle().equals("拨打电话")) {
Toast.makeText(context, "你点击了拨打电话:" + menuItem.getTitle(), Toast.LENGTH_SHORT).show();
Intent intent = new Intent(Intent.ACTION_DIAL);
intent.setData(Uri.parse("tel:" + String.valueOf(phone_phone.get(position))));
context.startActivity(intent);
} else if (menuItem.getTitle().equals("修改")) {
Toast.makeText(context, "你点击了修改:" + menuItem.getTitle(), Toast.LENGTH_SHORT).show();
Intent intent = new Intent(context, UpdataActivity.class);
//从这里获取数据,并设置意图在数据下方
intent.putExtra("id", String.valueOf(phone_id.get(position)));
intent.putExtra("name", String.valueOf(phone_name.get(position)));
intent.putExtra("phone", String.valueOf(phone_phone.get(position)));
intent.putExtra("address", String.valueOf(phone_address.get(position)));
intent.putExtra("unit", String.valueOf(phone_unit.get(position)));
intent.putExtra("email", String.valueOf(phone_email.get(position)));
intent.putExtra("qq", String.valueOf(phone_qq.get(position)));
activity.startActivityForResult(intent, 1);
} else if (menuItem.getTitle().equals("删除")) {
AlertDialog.Builder builder = new AlertDialog.Builder(context);
builder.setTitle("删除" + String.valueOf(phone_name.get(position)) + "信息?");
builder.setMessage("确认要" + String.valueOf(phone_name.get(position)) + "所有信息?");
builder.setPositiveButton("是", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
MyDatabaseHelper myDB = new MyDatabaseHelper(context);
myDB.deleteOneRow(String.valueOf(phone_id.get(position)));
Intent intent1 = new Intent(context, MainActivity.class);
context.startActivity(intent1);
activity.finish();
}
});
builder.setNegativeButton("否", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, i

xxin¥
- 粉丝: 473
- 资源: 1
最新资源
- 屏幕截图 2024-12-21 170328.png
- Python基于Opencv+keras的实时手势识别系统源码+文档
- 屏幕截图 2024-12-21 171010.png
- 屏幕截图 2024-12-21 170616.png
- 屏幕截图 2024-12-21 171921.png
- Python&Opencv手势识别系统(完整源码&自定义UI操作界面)
- 空中俯视物体检测22-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord数据集合集.rar
- Python毕业设计-基于Opencv手势识别系统源码+文档
- LabVIEW 通讯与罗克韦尔 AB Allen Bradley PLC通讯 EhernetIP 网口TCP标签通讯 SL500实测通过 常用功能一网打尽 1.命令帧读写 2.支持 I16 I3
- 结合多个机器学习模型进行分类,并使用交叉验证来评估模型性能,最后我们还会使用模型融合(Ensemble Learning)来尝试提高预测准确率
- python+windows+自动点击脚本
- 前后双电机扭矩分配,四驱扭矩分配,前后各一个电机,基于效率的扭矩分配 根据电机效率计算分配系数 系统效率最高 电动车四驱扭矩分配
- 基于YOLOV5的手势识别系统源码(毕设)+文档+数据集
- 基于多次多项式的机器学习脚本案例
- 外转子无刷直流电机温度场,瞬态热仿真
- 空中俯视物体检测23-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord数据集合集.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


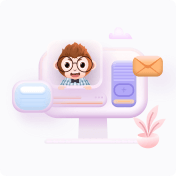