/*
* Copyright (c) 2015, 2020, Oracle and/or its affiliates.
*
* This program is free software; you can redistribute it and/or modify it under
* the terms of the GNU General Public License, version 2.0, as published by the
* Free Software Foundation.
*
* This program is also distributed with certain software (including but not
* limited to OpenSSL) that is licensed under separate terms, as designated in a
* particular file or component or in included license documentation. The
* authors of MySQL hereby grant you an additional permission to link the
* program and your derivative works with the separately licensed software that
* they have included with MySQL.
*
* Without limiting anything contained in the foregoing, this file, which is
* part of MySQL Connector/J, is also subject to the Universal FOSS Exception,
* version 1.0, a copy of which can be found at
* http://oss.oracle.com/licenses/universal-foss-exception.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU General Public License, version 2.0,
* for more details.
*
* You should have received a copy of the GNU General Public License along with
* this program; if not, write to the Free Software Foundation, Inc.,
* 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
*/
package com.mysql.cj.x.protobuf;
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: mysqlx_crud.proto
@SuppressWarnings({ "deprecation" })
public final class MysqlxCrud {
private MysqlxCrud() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* <pre>
* DataModel to use for filters, names, ...
* </pre>
*
* Protobuf enum {@code Mysqlx.Crud.DataModel}
*/
public enum DataModel
implements com.google.protobuf.ProtocolMessageEnum {
/**
* <code>DOCUMENT = 1;</code>
*/
DOCUMENT(1),
/**
* <code>TABLE = 2;</code>
*/
TABLE(2),
;
/**
* <code>DOCUMENT = 1;</code>
*/
public static final int DOCUMENT_VALUE = 1;
/**
* <code>TABLE = 2;</code>
*/
public static final int TABLE_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DataModel valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static DataModel forNumber(int value) {
switch (value) {
case 1: return DOCUMENT;
case 2: return TABLE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap<DataModel>
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
DataModel> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap<DataModel>() {
public DataModel findValueByNumber(int number) {
return DataModel.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.mysql.cj.x.protobuf.MysqlxCrud.getDescriptor().getEnumTypes().get(0);
}
private static final DataModel[] VALUES = values();
public static DataModel valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private DataModel(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:Mysqlx.Crud.DataModel)
}
/**
* <pre>
* ViewAlgorithm defines how MySQL Server processes the view
* </pre>
*
* Protobuf enum {@code Mysqlx.Crud.ViewAlgorithm}
*/
public enum ViewAlgorithm
implements com.google.protobuf.ProtocolMessageEnum {
/**
* <pre>
* MySQL chooses which algorithm to use
* </pre>
*
* <code>UNDEFINED = 1;</code>
*/
UNDEFINED(1),
/**
* <pre>
* the text of a statement that refers to the view and the view definition are merged
* </pre>
*
* <code>MERGE = 2;</code>
*/
MERGE(2),
/**
* <pre>
* the view are retrieved into a temporary table
* </pre>
*
* <code>TEMPTABLE = 3;</code>
*/
TEMPTABLE(3),
;
/**
* <pre>
* MySQL chooses which algorithm to use
* </pre>
*
* <code>UNDEFINED = 1;</code>
*/
public static final int UNDEFINED_VALUE = 1;
/**
* <pre>
* the text of a statement that refers to the view and the view definition are merged
* </pre>
*
* <code>MERGE = 2;</code>
*/
public static final int MERGE_VALUE = 2;
/**
* <pre>
* the view are retrieved into a temporary table
* </pre>
*
* <code>TEMPTABLE = 3;</code>
*/
public static final int TEMPTABLE_VALUE = 3;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ViewAlgorithm valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ViewAlgorithm forNumber(int value) {
switch (value) {
case 1: return UNDEFINED;
case 2: return MERGE;
case 3: return TEMPTABLE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap<ViewAlgorithm>
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ViewAlgorithm> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap<ViewAlgorithm>() {
public ViewAlgorithm findValueByNumber(int number) {
return ViewAlgorithm.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.mysql.cj.x.protobuf.MysqlxCrud.getDescriptor().getEnumTypes().get(1);
}
private static final ViewAlgorithm[] VALUES = values();
public static ViewAlgorithm valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw
没有合适的资源?快使用搜索试试~ 我知道了~
java程序设计—Java游戏设计打飞机程序(源代码+论文)69.rar
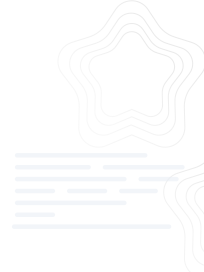
共692个文件
java:643个
pem:13个
properties:10个

需积分: 0 0 下载量 33 浏览量
更新于2024-07-24
收藏 5.13MB RAR 举报
java程序设计,可供正在学习的同学研究参考。
收起资源包目录

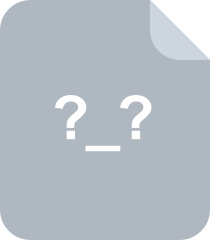
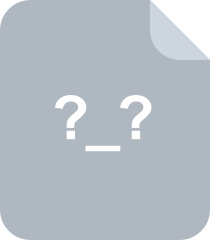
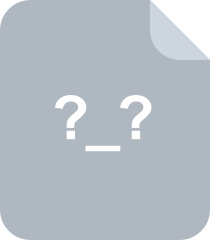
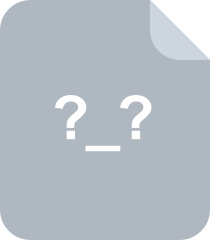
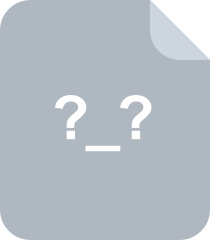
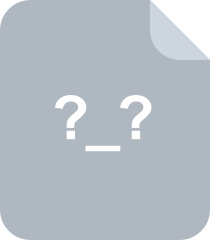
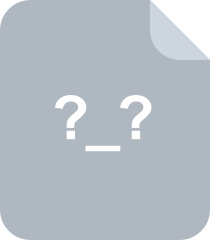
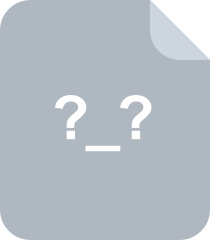
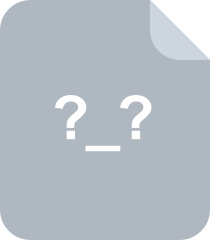
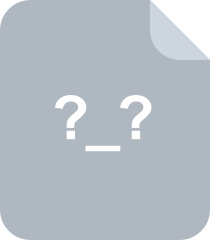
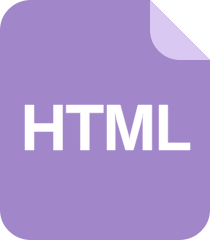
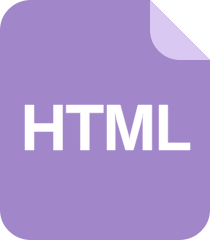
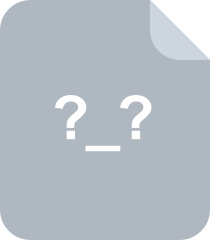
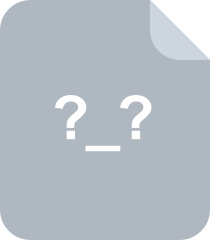
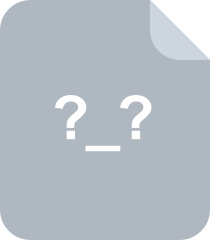
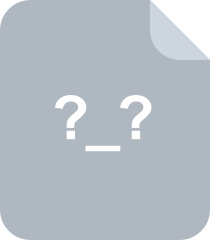
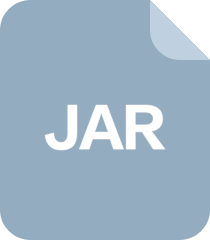
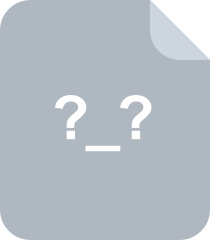
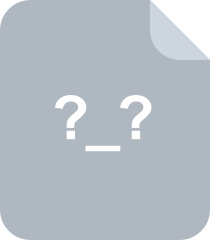
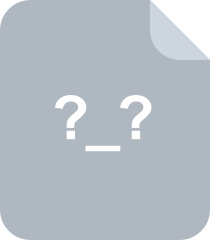
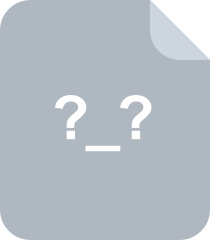
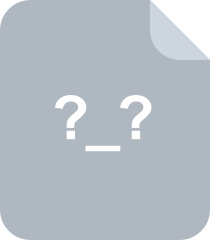
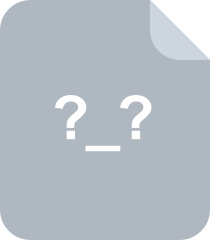
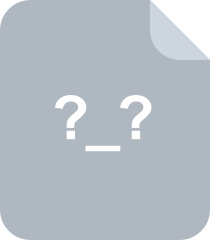
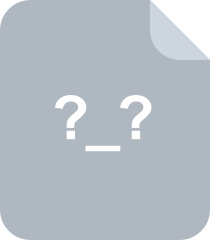
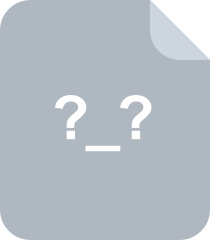
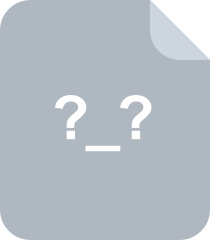
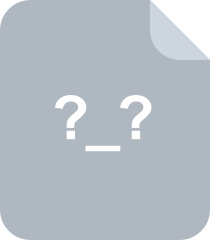
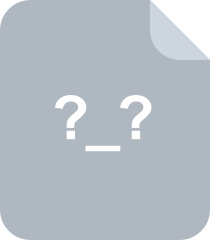
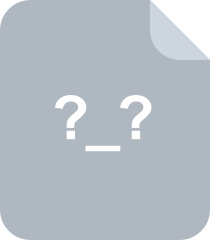
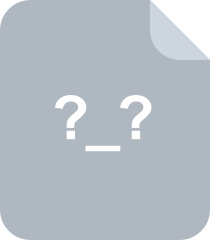
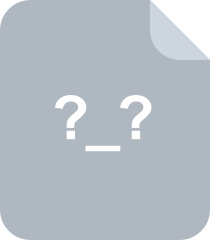
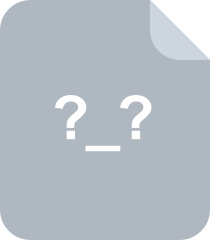
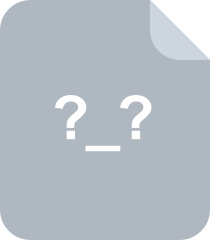
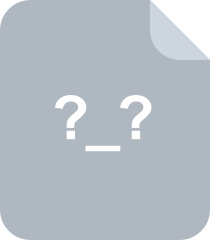
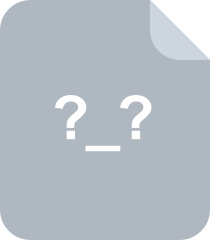
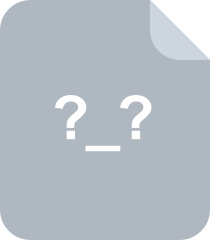
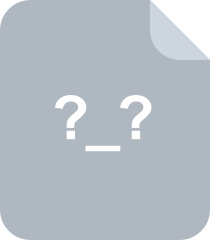
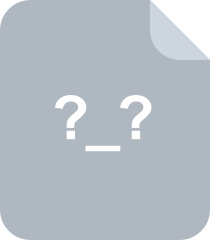
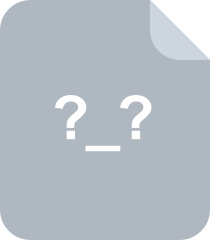
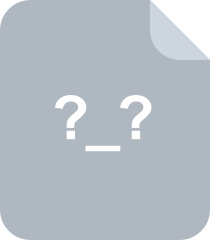
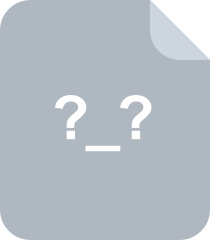
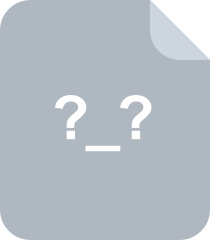
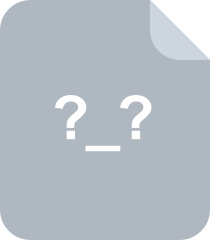
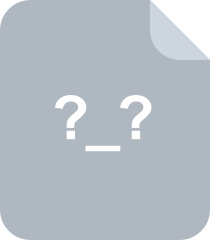
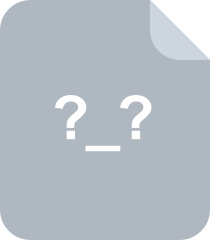
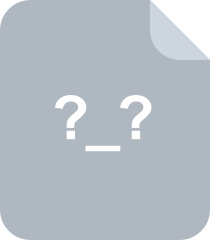
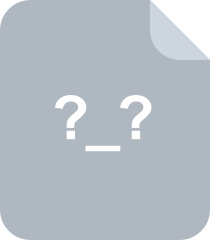
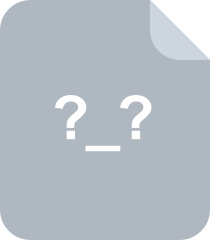
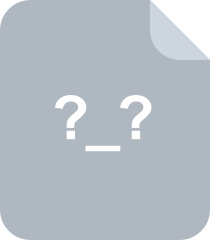
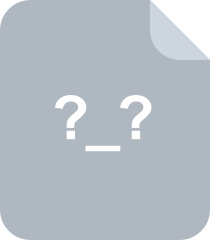
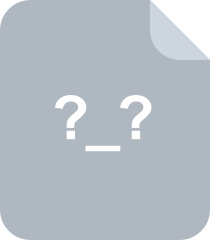
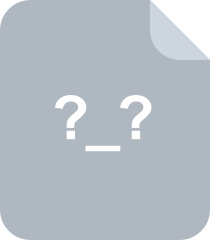
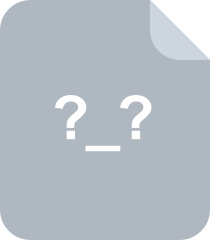
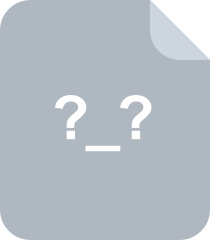
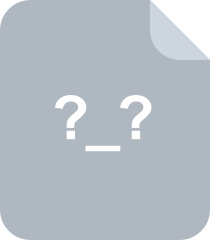
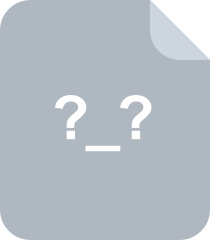
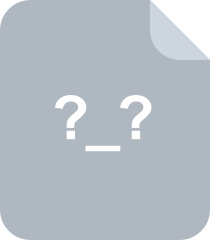
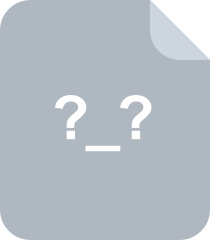
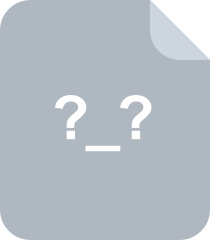
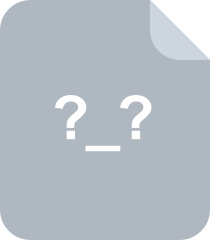
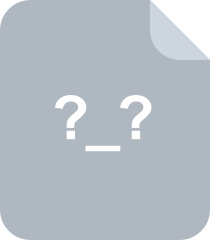
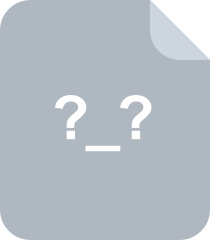
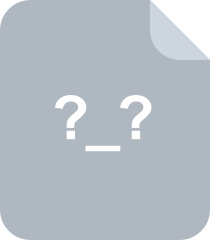
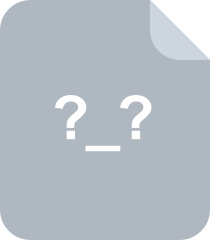
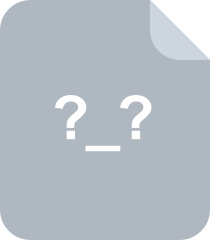
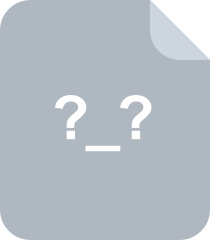
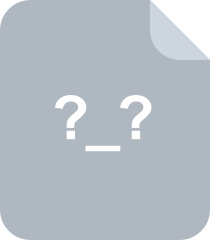
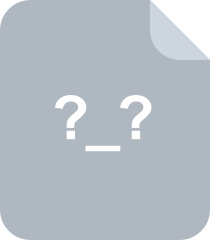
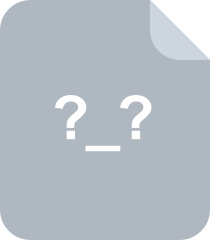
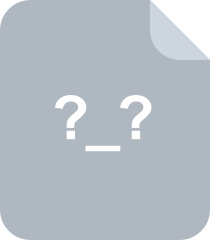
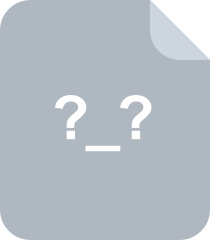
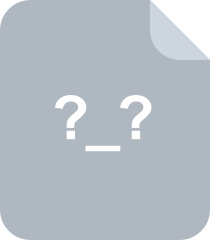
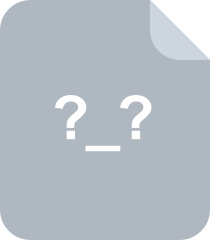
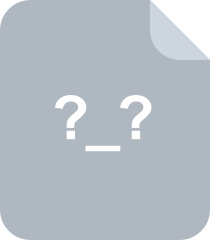
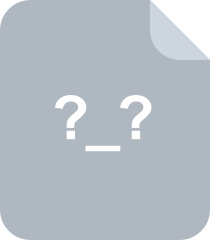
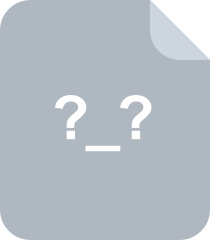
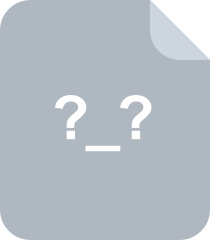
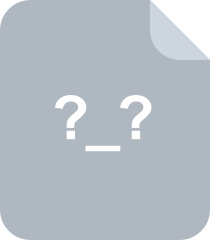
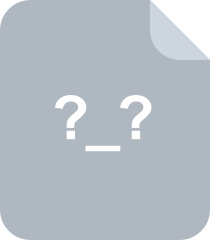
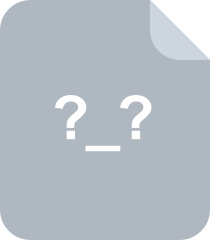
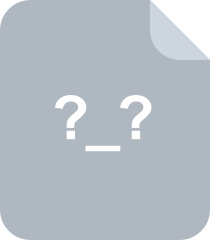
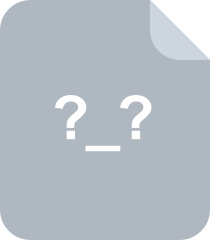
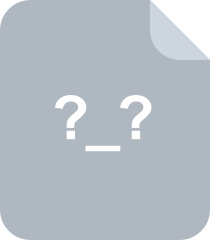
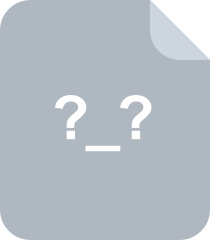
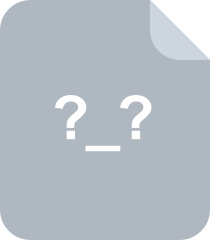
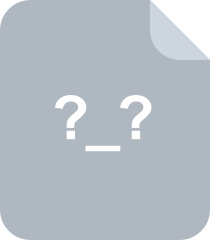
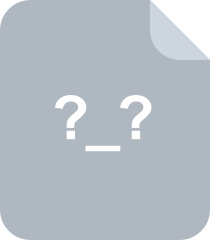
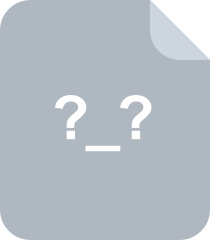
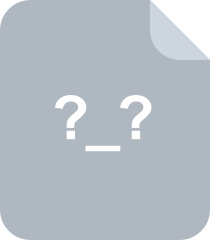
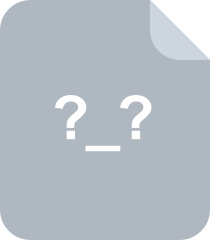
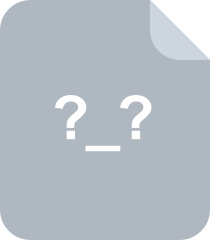
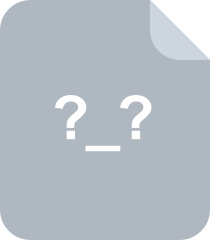
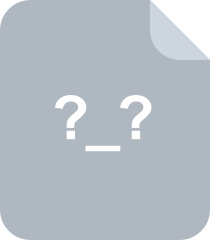
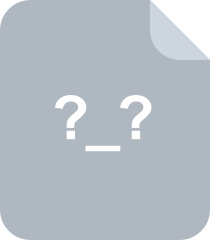
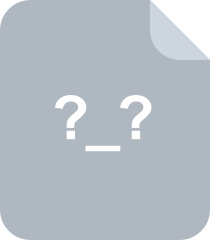
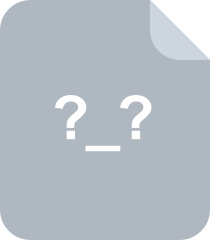
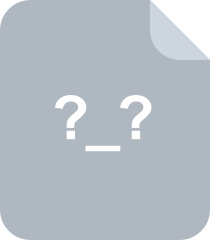
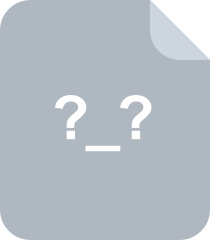
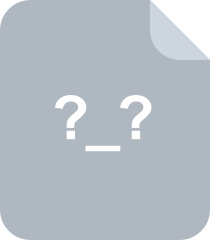
共 692 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源推荐
资源预览
资源评论
2024-01-05 上传
190 浏览量

119 浏览量
2024-03-12 上传
2024-01-05 上传
120 浏览量


2024-06-22 上传
114 浏览量
184 浏览量
174 浏览量
133 浏览量

173 浏览量
102 浏览量
2023-12-27 上传



138 浏览量
197 浏览量
2024-12-18 上传
112 浏览量
资源评论
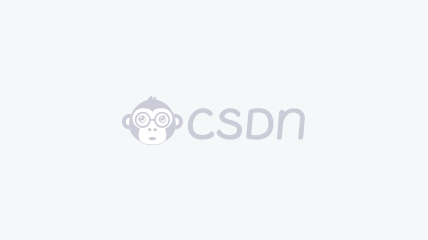

零度°
- 粉丝: 1929
- 资源: 1943
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

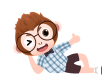
最新资源
- (176566234)数据库课程设计springboot209基于web的大学生一体化服务平台的设计与实现.sql
- 火与烟雾检测22-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord数据集合集.rar
- (176936394)Axure元件库(内含pc端,ios,安卓,微信小程序)
- DownKyi二维码补丁
- 火与烟雾检测23-YOLO(v5至v9)数据集合集.rar
- (177725628)自做JAVA课设项目-ATM柜员机模拟系统(内置GUI页面与Mysql数据库引用)
- (177898816)电力系统无功补偿Matlab仿真模型,simulink仿真,毕业设计,课程设计均已调试好
- (178961650)python3.12对应的dlib-19.24.99-cp312-cp312-win-amd64
- (19055830)java实现ATM柜员机模拟程序
- 文章分词并对词频用不同排序方法排序的系统项目全套技术资料.zip
- 火与烟雾检测24-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord数据集合集.rar
- 百度贴吧深度挖掘:精准抓取楼主发言Python爬虫
- Hibernate整合Spring增删改查示例源码.zip
- python教学示例-anagram-db
- 火灾检测16-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord数据集合集.rar
- IMG_20241223_160717.jpg
安全验证
文档复制为VIP权益,开通VIP直接复制
