# Fluent ffmpeg-API for node.js
[](https://coveralls.io/github/fluent-ffmpeg/node-fluent-ffmpeg?branch=master)
[](https://app.fossa.io/projects/git%2Bgithub.com%2Ffluent-ffmpeg%2Fnode-fluent-ffmpeg?ref=badge_shield)
> **Fluent-ffmpeg is looking for new maintainers**
> More details [on the wiki](https://github.com/fluent-ffmpeg/node-fluent-ffmpeg/wiki/Looking-for-a-new-maintainer)
This library abstracts the complex command-line usage of ffmpeg into a fluent, easy to use node.js module. In order to be able to use this module, make sure you have [ffmpeg](http://www.ffmpeg.org) installed on your system (including all necessary encoding libraries like libmp3lame or libx264).
> This is the documentation for fluent-ffmpeg 2.x.
> You can still access the code and documentation for fluent-ffmpeg 1.7 [here](https://github.com/fluent-ffmpeg/node-fluent-ffmpeg/tree/1.x).
## Installation
Via npm:
```sh
$ npm install fluent-ffmpeg
```
Or as a submodule:
```sh
$ git submodule add git://github.com/schaermu/node-fluent-ffmpeg.git vendor/fluent-ffmpeg
```
## Usage
You will find a lot of usage examples (including a real-time streaming example using [flowplayer](http://www.flowplayer.org) and [express](https://github.com/visionmedia/express)!) in the `examples` folder.
### Prerequisites
#### ffmpeg and ffprobe
fluent-ffmpeg requires ffmpeg >= 0.9 to work. It may work with previous versions but several features won't be available (and the library is not tested with lower versions anylonger).
If the `FFMPEG_PATH` environment variable is set, fluent-ffmpeg will use it as the full path to the `ffmpeg` executable. Otherwise, it will attempt to call `ffmpeg` directly (so it should be in your `PATH`). You must also have ffprobe installed (it comes with ffmpeg in most distributions). Similarly, fluent-ffmpeg will use the `FFPROBE_PATH` environment variable if it is set, otherwise it will attempt to call it in the `PATH`.
Most features should work when using avconv and avprobe instead of ffmpeg and ffprobe, but they are not officially supported at the moment.
**Windows users**: most probably ffmpeg and ffprobe will _not_ be in your `%PATH`, so you _must_ set `%FFMPEG_PATH` and `%FFPROBE_PATH`.
**Debian/Ubuntu users**: the official repositories have the ffmpeg/ffprobe executable in the `libav-tools` package, and they are actually rebranded avconv/avprobe executables (avconv is a fork of ffmpeg). They should be mostly compatible, but should you encounter any issue, you may want to use the real ffmpeg instead. You can either compile it from source or find a pre-built .deb package at https://ffmpeg.org/download.html (For Ubuntu, the `ppa:mc3man/trusty-media` PPA provides recent builds).
#### flvtool2 or flvmeta
If you intend to encode FLV videos, you must have either flvtool2 or flvmeta installed and in your `PATH` or fluent-ffmpeg won't be able to produce streamable output files. If you set either the `FLVTOOL2_PATH` or `FLVMETA_PATH`, fluent-ffmpeg will try to use it instead of searching in the `PATH`.
#### Setting binary paths manually
Alternatively, you may set the ffmpeg, ffprobe and flvtool2/flvmeta binary paths manually by using the following API commands:
* **Ffmpeg.setFfmpegPath(path)** Argument `path` is a string with the full path to the ffmpeg binary.
* **Ffmpeg.setFfprobePath(path)** Argument `path` is a string with the full path to the ffprobe binary.
* **Ffmpeg.setFlvtoolPath(path)** Argument `path` is a string with the full path to the flvtool2 or flvmeta binary.
### Creating an FFmpeg command
The fluent-ffmpeg module returns a constructor that you can use to instanciate FFmpeg commands.
```js
var FfmpegCommand = require('fluent-ffmpeg');
var command = new FfmpegCommand();
```
You can also use the constructor without the `new` operator.
```js
var ffmpeg = require('fluent-ffmpeg');
var command = ffmpeg();
```
You may pass an input file name or readable stream, a configuration object, or both to the constructor.
```js
var command = ffmpeg('/path/to/file.avi');
var command = ffmpeg(fs.createReadStream('/path/to/file.avi'));
var command = ffmpeg({ option: "value", ... });
var command = ffmpeg('/path/to/file.avi', { option: "value", ... });
```
The following options are available:
* `source`: input file name or readable stream (ignored if an input file is passed to the constructor)
* `timeout`: ffmpeg timeout in seconds (defaults to no timeout)
* `preset` or `presets`: directory to load module presets from (defaults to the `lib/presets` directory in fluent-ffmpeg tree)
* `niceness` or `priority`: ffmpeg niceness value, between -20 and 20; ignored on Windows platforms (defaults to 0)
* `logger`: logger object with `debug()`, `info()`, `warn()` and `error()` methods (defaults to no logging)
* `stdoutLines`: maximum number of lines from ffmpeg stdout/stderr to keep in memory (defaults to 100, use 0 for unlimited storage)
### Specifying inputs
You can add any number of inputs to an Ffmpeg command. An input can be:
* a file name (eg. `/path/to/file.avi`);
* an image pattern (eg. `/path/to/frame%03d.png`);
* a readable stream; only one input stream may be used for a command, but you can use both an input stream and one or several file names.
```js
// Note that all fluent-ffmpeg methods are chainable
ffmpeg('/path/to/input1.avi')
.input('/path/to/input2.avi')
.input(fs.createReadStream('/path/to/input3.avi'));
// Passing an input to the constructor is the same as calling .input()
ffmpeg()
.input('/path/to/input1.avi')
.input('/path/to/input2.avi');
// Most methods have several aliases, here you may use addInput or mergeAdd instead
ffmpeg()
.addInput('/path/to/frame%02d.png')
.addInput('/path/to/soundtrack.mp3');
ffmpeg()
.mergeAdd('/path/to/input1.avi')
.mergeAdd('/path/to/input2.avi');
```
### Input options
The following methods enable passing input-related options to ffmpeg. Each of these methods apply on the last input added (including the one passed to the constructor, if any). You must add an input before calling those, or an error will be thrown.
#### inputFormat(format): specify input format
**Aliases**: `fromFormat()`, `withInputFormat()`.
This is only useful for raw inputs, as ffmpeg can determine the input format automatically.
```js
ffmpeg()
.input('/dev/video0')
.inputFormat('mov')
.input('/path/to/file.avi')
.inputFormat('avi');
```
Fluent-ffmpeg checks for format availability before actually running the command, and throws an error when a specified input format is not available.
#### inputFPS(fps): specify input framerate
**Aliases**: `withInputFps()`, `withInputFPS()`, `withFpsInput()`, `withFPSInput()`, `inputFps()`, `fpsInput()`, `FPSInput()`.
This is only valid for raw inputs, as ffmpeg can determine the input framerate automatically.
```js
ffmpeg('/dev/video0').inputFPS(29.7);
```
#### native(): read input at native framerate
**Aliases**: `nativeFramerate()`, `withNativeFramerate()`.
```js
ffmpeg('/path/to/file.avi').native();
```
#### seekInput(time): set input start time
**Alias**: `setStartTime()`.
Seeks an input and only start decoding at given time offset. The `time` argument may be a number (in seconds) or a timestamp string (with format `[[hh:]mm:]ss[.xxx]`).
```js
ffmpeg('/path/to/file.avi').seekInput(134.5);
ffmpeg('/path/to/file.avi').seekInput('2:14.500');
```
#### loop([duration]): loop over input
```js
ffmpeg('/path/to/file.avi').loop();
ffmpeg('/path/to/file.avi').loop(134.5);
ffmpeg('/path/to/file.avi').loop('2:14.500');
```
#### inputOptions(option...): add custom input options
**Aliases**: `inputOption()`, `addInputOption()`, `addInputOptions()`, `withInputOption()`, `withInputOptions()`.
This method allows passing any
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
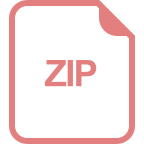
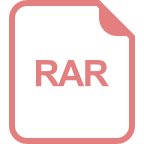
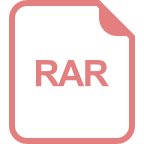
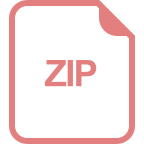
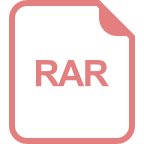
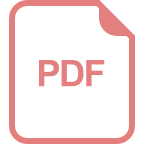
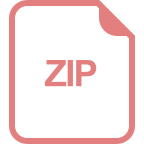
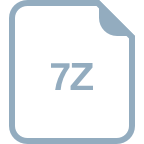
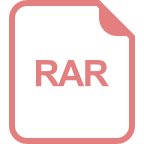
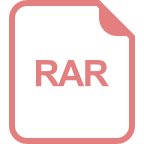
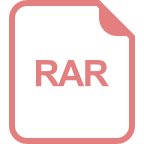
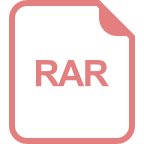
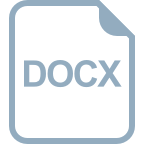
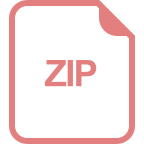
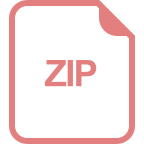
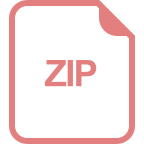
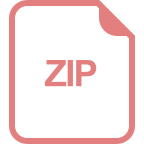
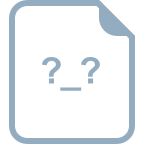
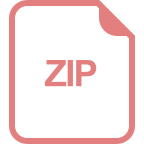
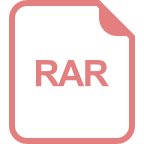
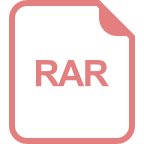
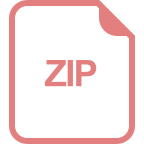
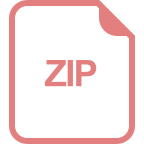
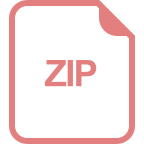
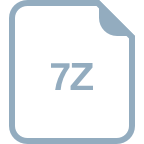
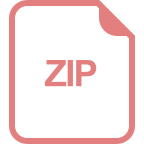
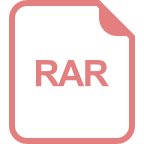
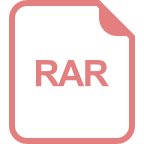
收起资源包目录

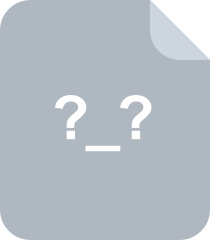
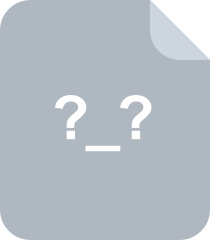
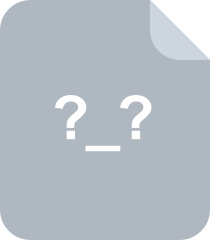
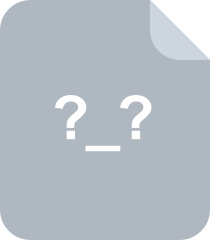
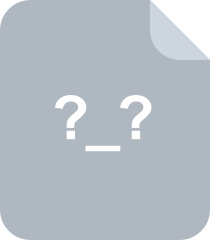
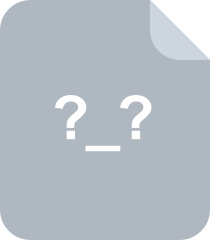
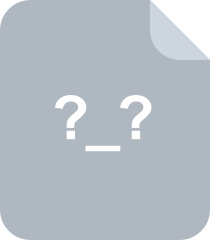
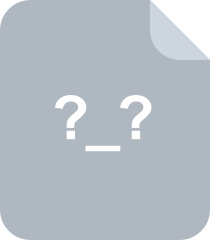
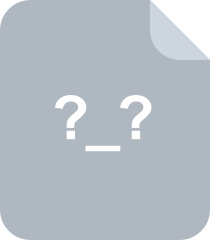
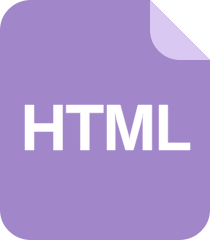
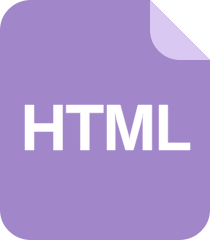
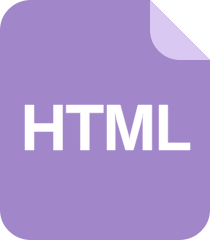
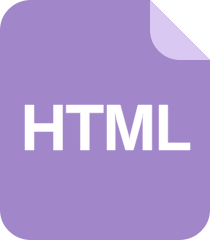
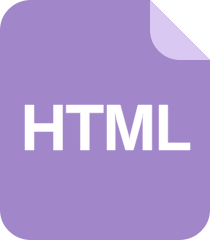
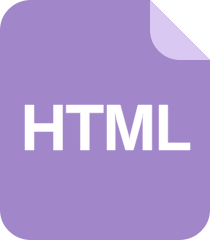
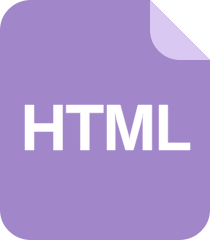
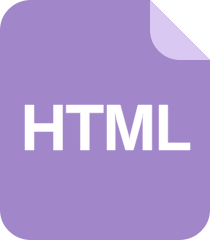
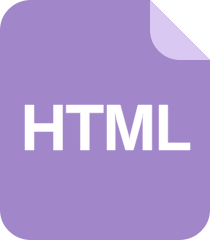
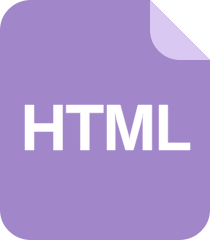
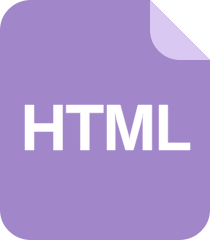
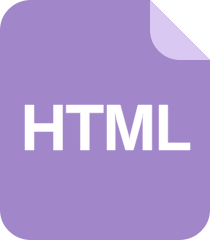
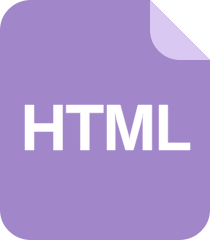
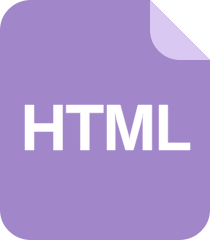
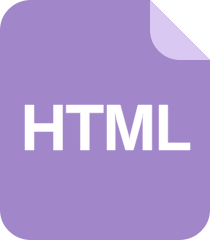
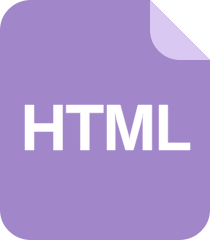
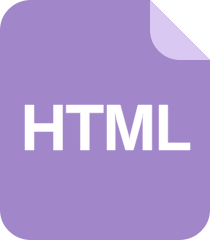
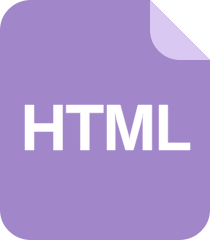
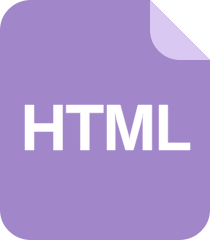
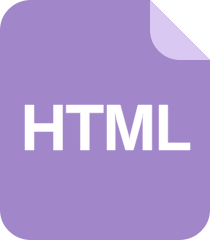
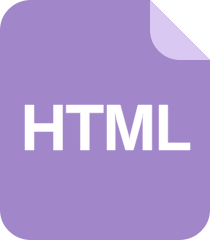
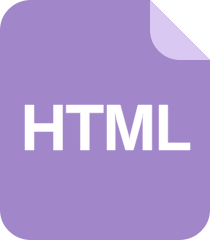
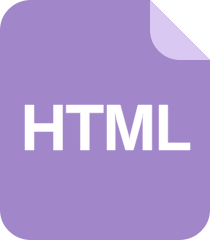
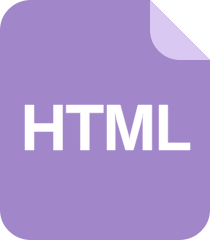
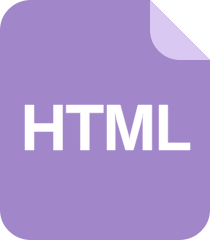
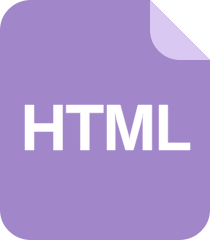
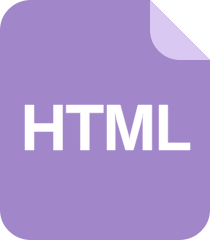
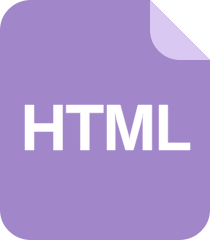
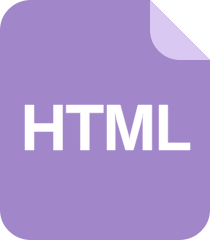
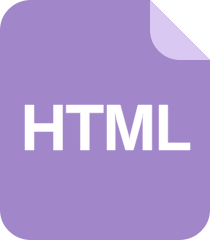
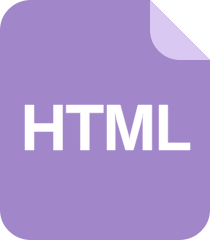
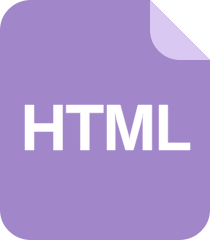
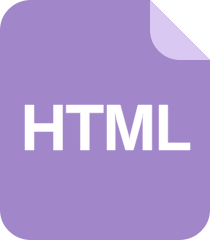
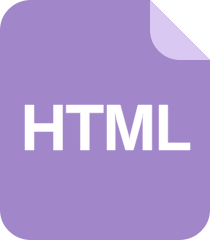
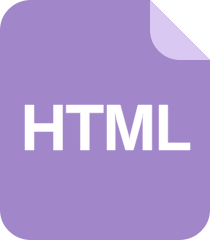
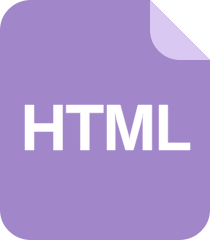
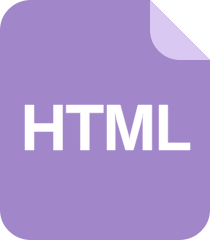
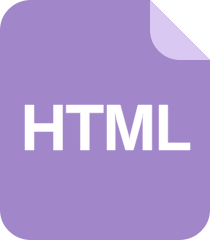
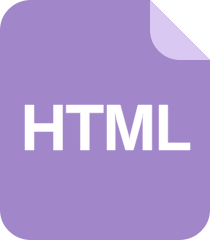
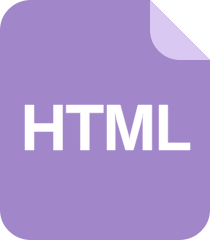
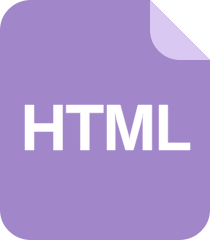
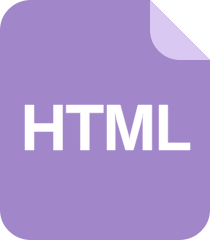
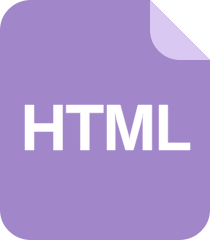
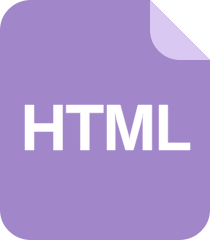
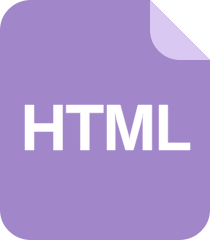
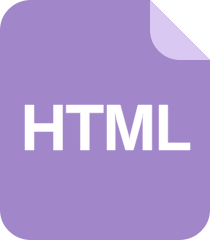
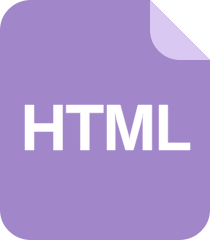
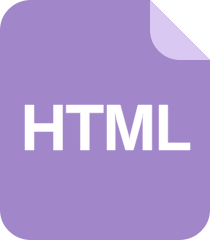
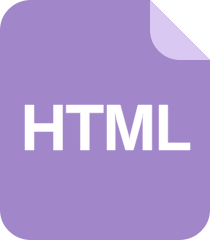
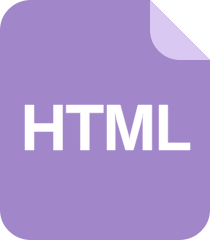
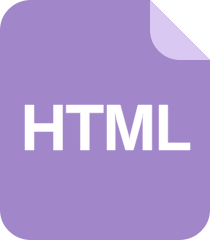
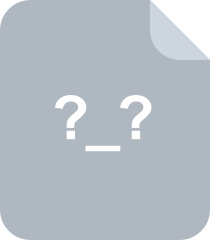
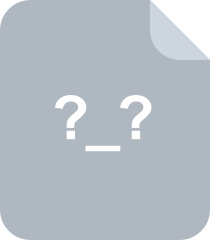
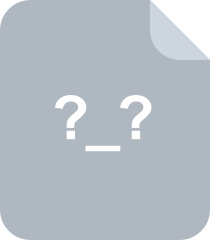
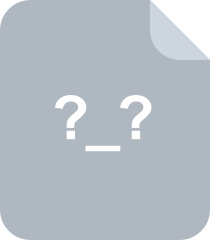
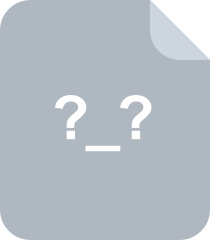
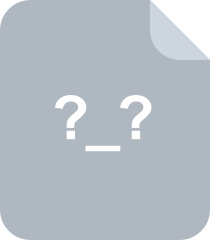
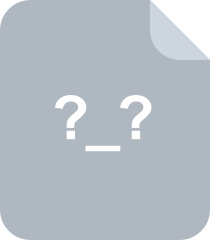
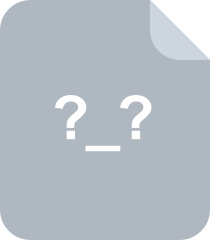
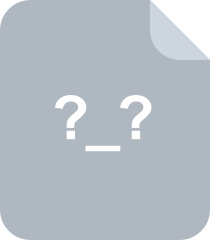
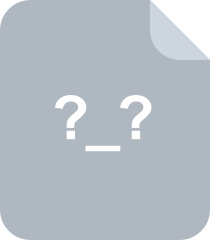
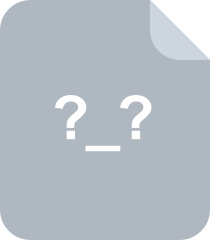
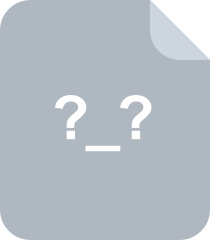
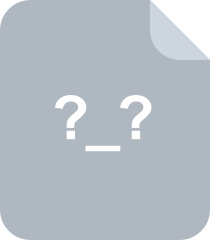
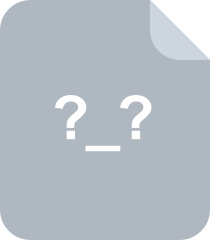
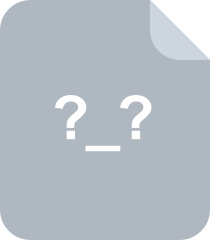
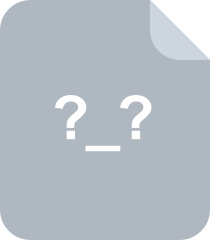
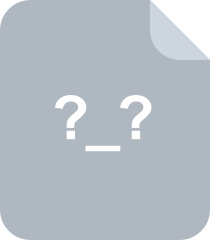
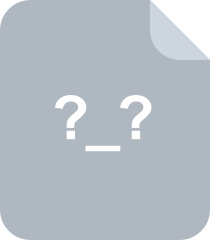
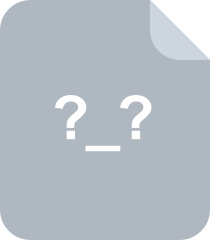
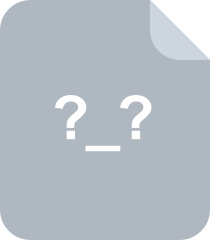
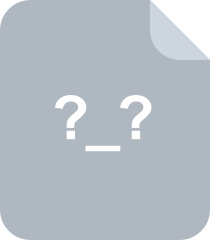
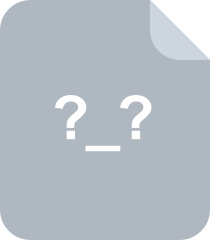
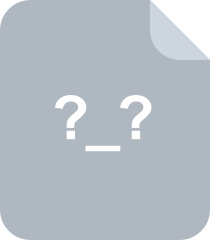
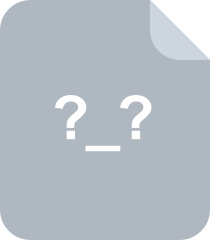
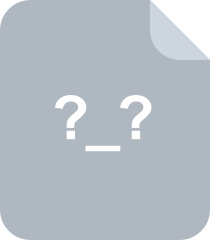
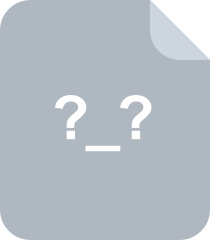
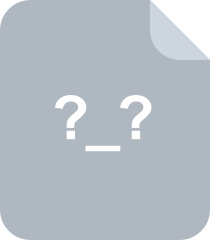
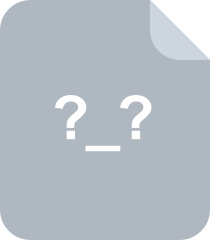
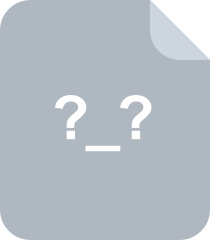
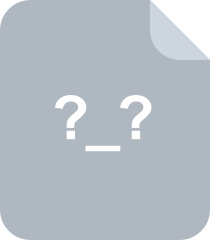
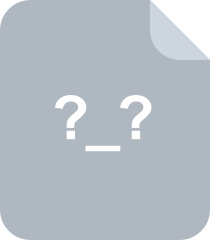
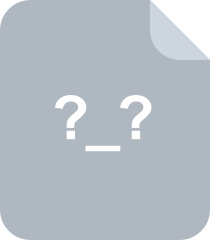
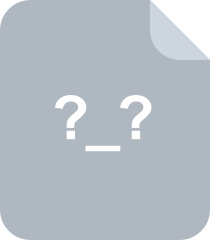
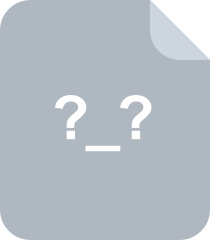
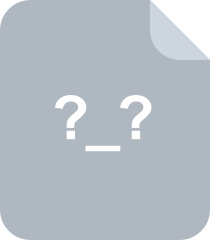
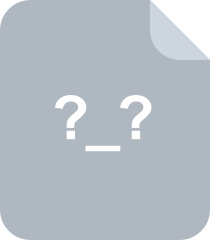
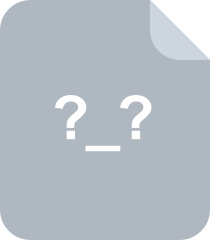
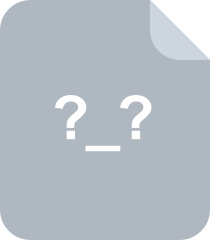
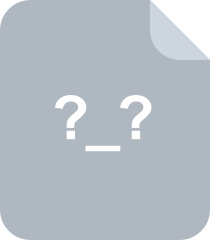
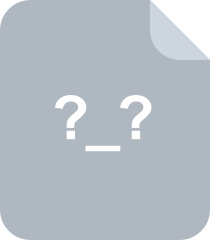
共 186 条
- 1
- 2
资源评论
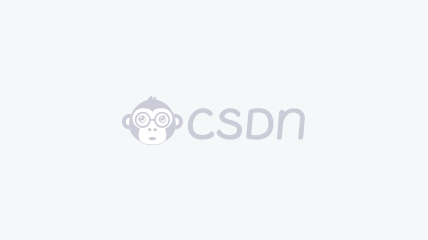

博客zhu虎康
- 粉丝: 3w+
- 资源: 37
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

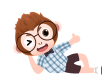
最新资源
- 塑料瓶水瓶检测33-YOLO(v5至v9)、CreateML、Darknet、TFRecord、VOC数据集合集.rar
- 全栈架构:从0开始,Vue的搭建与开发
- JavaScript基本手册.rar
- 塑料瓶水瓶子瓶罐子检测18-YOLO(v8至v9)数据集合集.rar
- php教程之【使用mysqli扩展库】
- Python编程题目60个
- ZABAPGIT-STANDALONE
- 塑料瓶水瓶子瓶罐子检测17-YOLO(v5至v9)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 抖音运营:抖音橱窗引流:新营销模式解析.pptx
- 《C语言指针经验总结》.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


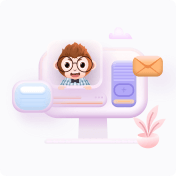
安全验证
文档复制为VIP权益,开通VIP直接复制
