package com.controller;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import com.utils.ValidatorUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.annotation.IgnoreAuth;
import com.entity.YuangongEntity;
import com.entity.view.YuangongView;
import com.service.YuangongService;
import com.service.TokenService;
import com.utils.PageUtils;
import com.utils.R;
import com.utils.MD5Util;
import com.utils.MPUtil;
import com.utils.CommonUtil;
/**
* 员工
* 后端接口
* @author
* @email
* @date 2021-04-29 16:24:23
*/
@RestController
@RequestMapping("/yuangong")
public class YuangongController {
@Autowired
private YuangongService yuangongService;
@Autowired
private TokenService tokenService;
/**
* 登录
*/
@IgnoreAuth
@RequestMapping(value = "/login")
public R login(String username, String password, String captcha, HttpServletRequest request) {
YuangongEntity user = yuangongService.selectOne(new EntityWrapper<YuangongEntity>().eq("gonghao", username));
if(user==null || !user.getMima().equals(password)) {
return R.error("账号或密码不正确");
}
String token = tokenService.generateToken(user.getId(), username,"yuangong", "员工" );
return R.ok().put("token", token);
}
/**
* 注册
*/
@IgnoreAuth
@RequestMapping("/register")
public R register(@RequestBody YuangongEntity yuangong){
//ValidatorUtils.validateEntity(yuangong);
YuangongEntity user = yuangongService.selectOne(new EntityWrapper<YuangongEntity>().eq("gonghao", yuangong.getGonghao()));
if(user!=null) {
return R.error("注册用户已存在");
}
Long uId = new Date().getTime();
yuangong.setId(uId);
yuangongService.insert(yuangong);
return R.ok();
}
/**
* 退出
*/
@RequestMapping("/logout")
public R logout(HttpServletRequest request) {
request.getSession().invalidate();
return R.ok("退出成功");
}
/**
* 获取用户的session用户信息
*/
@RequestMapping("/session")
public R getCurrUser(HttpServletRequest request){
Long id = (Long)request.getSession().getAttribute("userId");
YuangongEntity user = yuangongService.selectById(id);
return R.ok().put("data", user);
}
/**
* 密码重置
*/
@IgnoreAuth
@RequestMapping(value = "/resetPass")
public R resetPass(String username, HttpServletRequest request){
YuangongEntity user = yuangongService.selectOne(new EntityWrapper<YuangongEntity>().eq("gonghao", username));
if(user==null) {
return R.error("账号不存在");
}
user.setMima("123456");
yuangongService.updateById(user);
return R.ok("密码已重置为:123456");
}
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,YuangongEntity yuangong,
HttpServletRequest request){
EntityWrapper<YuangongEntity> ew = new EntityWrapper<YuangongEntity>();
PageUtils page = yuangongService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, yuangong), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,YuangongEntity yuangong,
HttpServletRequest request){
EntityWrapper<YuangongEntity> ew = new EntityWrapper<YuangongEntity>();
PageUtils page = yuangongService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, yuangong), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( YuangongEntity yuangong){
EntityWrapper<YuangongEntity> ew = new EntityWrapper<YuangongEntity>();
ew.allEq(MPUtil.allEQMapPre( yuangong, "yuangong"));
return R.ok().put("data", yuangongService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(YuangongEntity yuangong){
EntityWrapper< YuangongEntity> ew = new EntityWrapper< YuangongEntity>();
ew.allEq(MPUtil.allEQMapPre( yuangong, "yuangong"));
YuangongView yuangongView = yuangongService.selectView(ew);
return R.ok("查询员工成功").put("data", yuangongView);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
YuangongEntity yuangong = yuangongService.selectById(id);
return R.ok().put("data", yuangong);
}
/**
* 前端详情
*/
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") Long id){
YuangongEntity yuangong = yuangongService.selectById(id);
return R.ok().put("data", yuangong);
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody YuangongEntity yuangong, HttpServletRequest request){
yuangong.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(yuangong);
YuangongEntity user = yuangongService.selectOne(new EntityWrapper<YuangongEntity>().eq("gonghao", yuangong.getGonghao()));
if(user!=null) {
return R.error("用户已存在");
}
yuangong.setId(new Date().getTime());
yuangongService.insert(yuangong);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody YuangongEntity yuangong, HttpServletRequest request){
yuangong.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(yuangong);
YuangongEntity user = yuangongService.selectOne(new EntityWrapper<YuangongEntity>().eq("gonghao", yuangong.getGonghao()));
if(user!=null) {
return R.error("用户已存在");
}
yuangong.setId(new Date().getTime());
yuangongService.insert(yuangong);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody YuangongEntity yuangong, HttpServletRequest request){
//ValidatorUtils.validateEntity(yuangong);
yuangongService.updateById(yuangong);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
yuangongService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
* 提醒接口
*/
@RequestMapping("/remind/{columnName}/{type}")
public R remindCount(@PathVariable("columnName") String columnName, HttpServletRequest request,
@PathVariable("type") String type,@RequestParam Map<String, Object> map) {
map.put("column", columnName);
map.put("type", type);
if(type.equals("2")) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar c = Calendar.getInstance();
Date remindStartDate = null;
Date re
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
随着信息技术在管理上越来越深入而广泛的应用,管理信息系统的实施在技术上已逐步成熟。本文介绍了中小企业设备管理系统的开发全过程。通过分析中小企业设备管理系统管理的不足,创建了一个计算机管理中小企业设备管理系统的方案。文章介绍了中小企业设备管理系统的系统分析部分,包括可行性分析等,系统设计部分主要介绍了系统功能设计和数据库设计。 本中小企业设备管理系统管理员有个人中心,用户管理,员工管理,设备信息管理,配件信息管理,设备购买管理,配件购买管理,设备点检管理,设备润滑管理,设备改造管理,事务报警管理,设备类型管理。员工有个人中心,设备信息管理,设备购买管理,设备报修管理,售后检修管理,售后保养管理,设备安装管理,设备点检管理,设备润滑管理,设备改造管理,事务报警管理。因而具有一定的实用性。 本站是一个B/S模式系统,采用Spring Boot框架,MYSQL数据库设计开发,充分保证系统的稳定性。系统具有界面清晰、操作简单,功能齐全的特点,使得中小企业设备管理系统管理工作系统化、规范化。本系统的使用使管理人员从繁重的工作中解脱出来,实现无纸化办公,能够有效的提高中小企业设备管理系统管理效率。
资源推荐
资源详情
资源评论
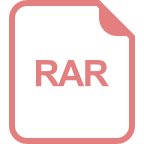
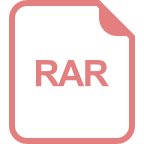
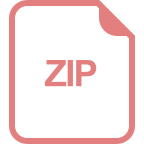
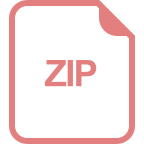
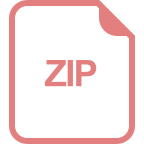
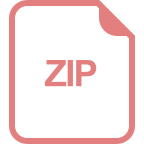
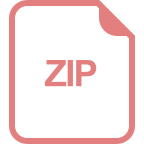
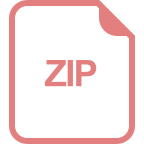
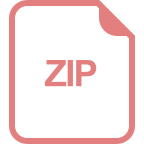
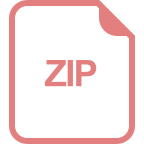
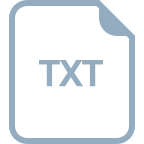
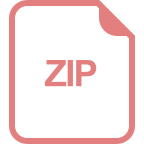
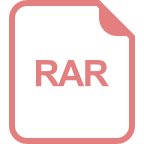
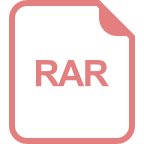
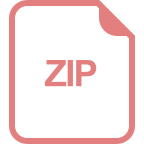
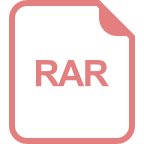
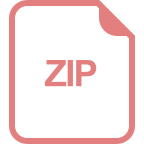
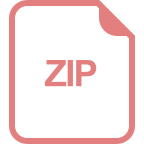
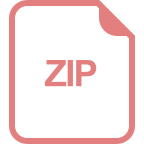
收起资源包目录

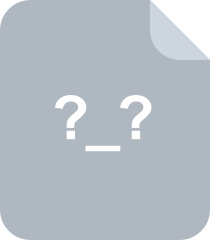
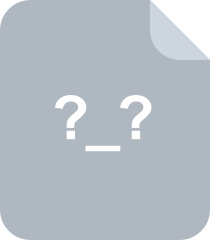
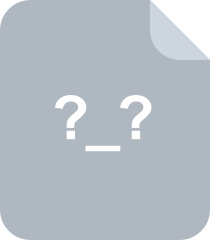
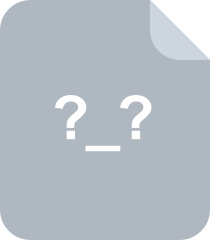
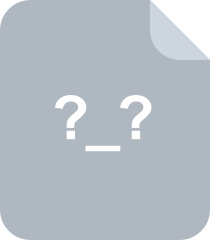
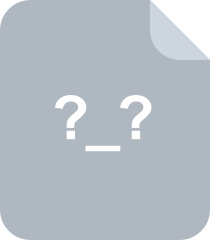
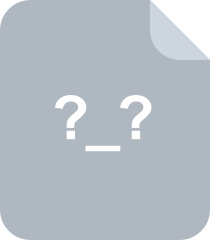
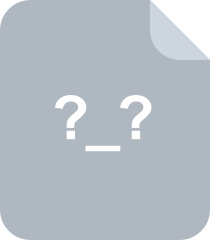
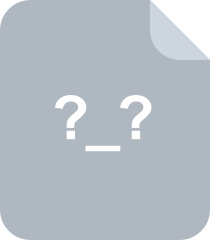
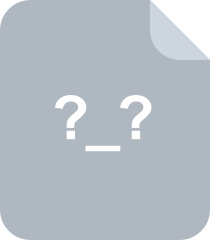
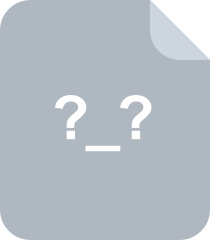
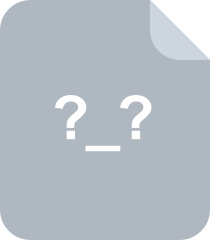
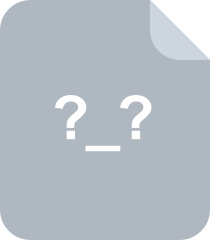
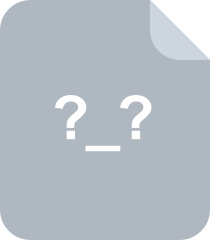
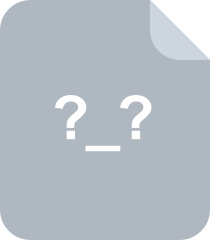
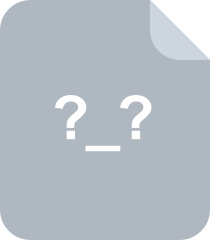
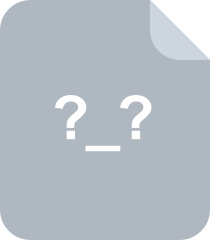
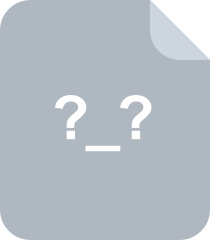
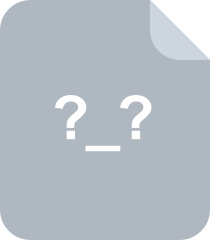
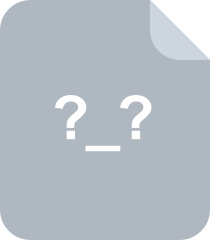
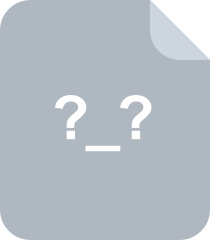
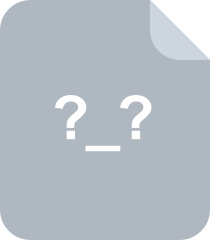
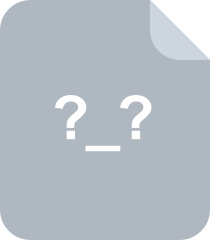
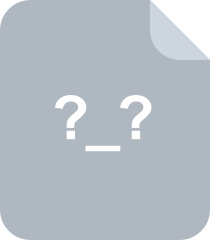
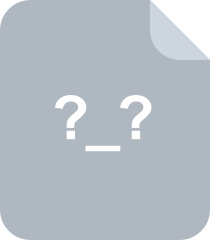
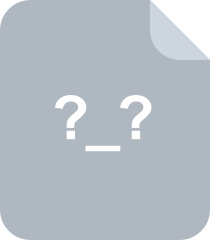
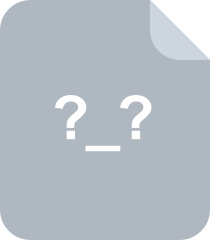
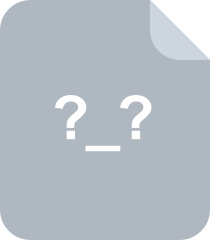
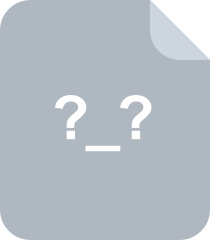
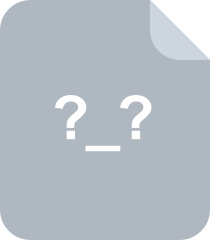
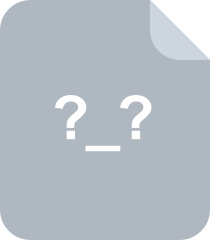
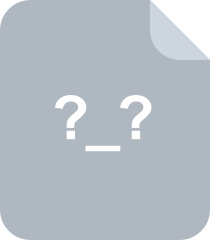
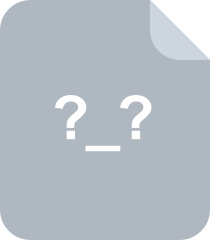
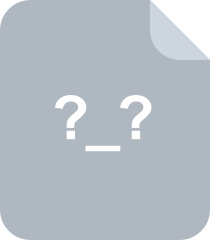
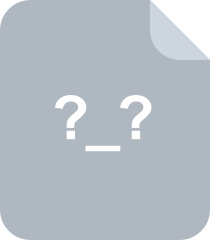
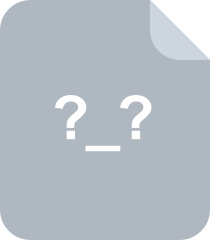
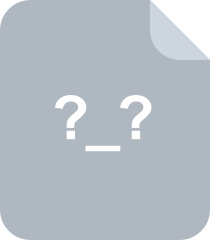
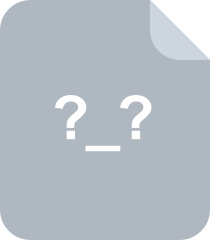
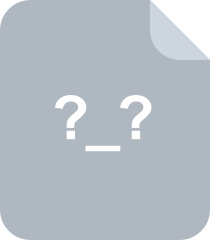
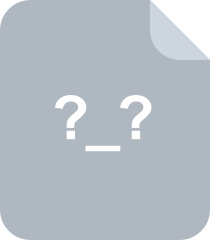
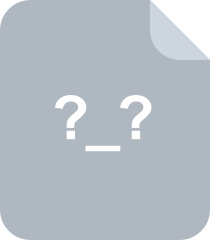
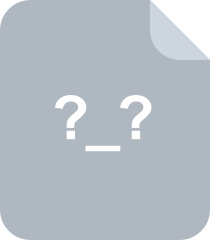
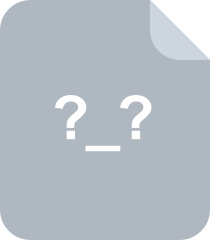
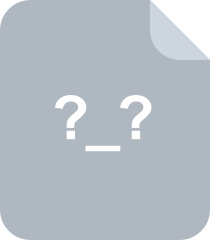
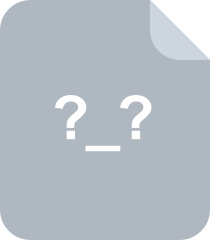
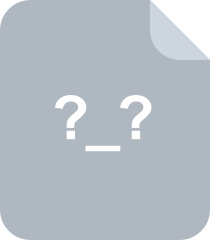
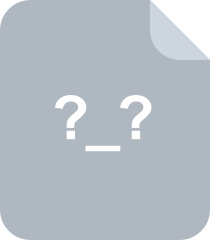
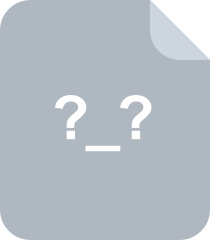
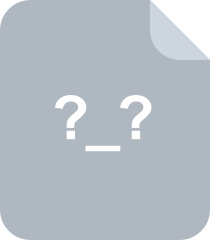
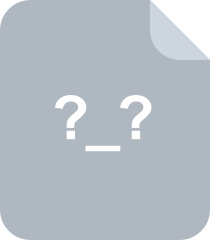
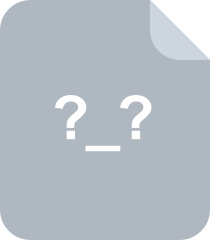
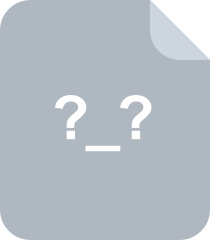
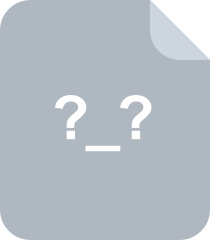
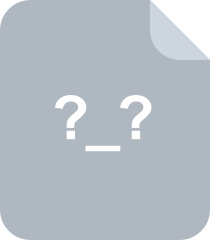
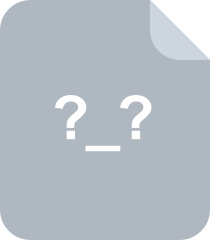
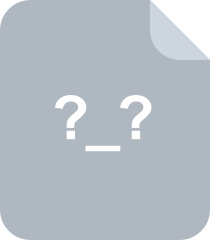
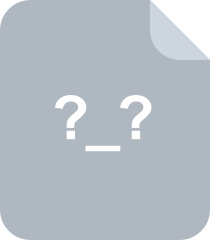
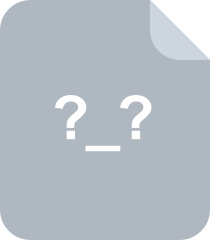
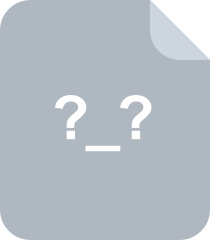
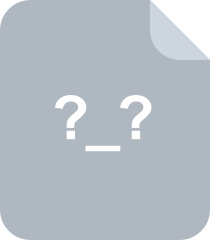
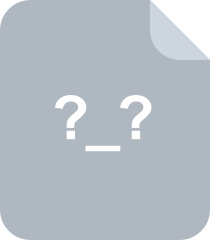
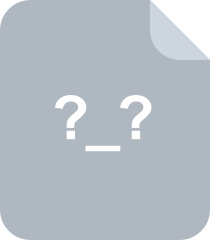
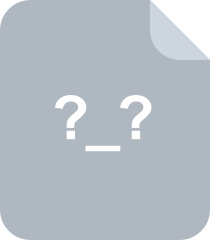
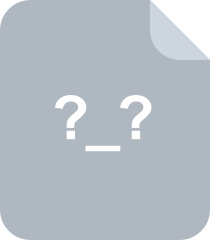
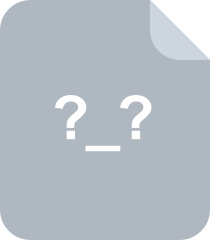
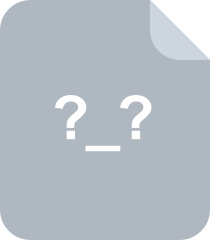
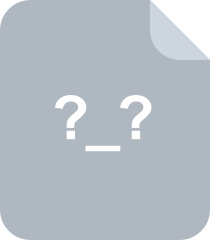
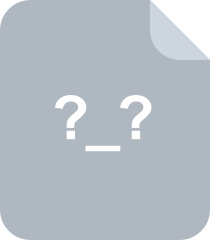
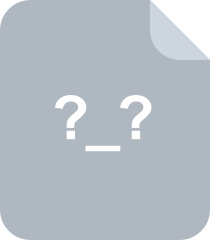
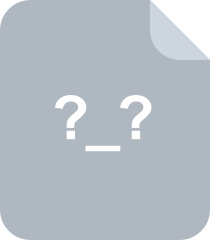
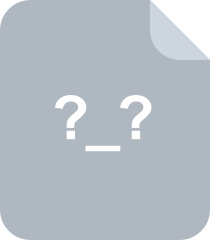
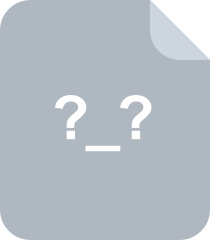
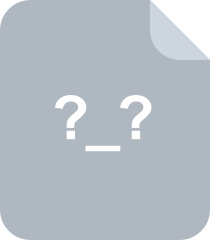
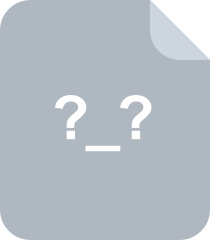
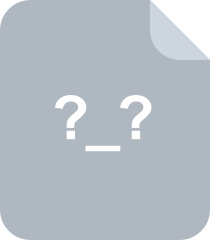
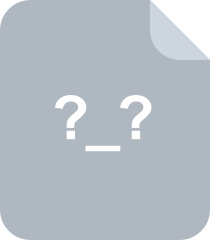
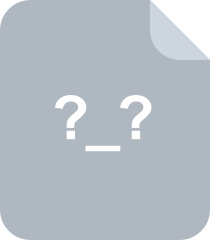
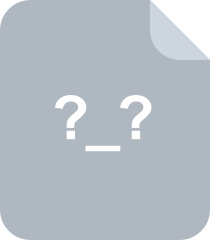
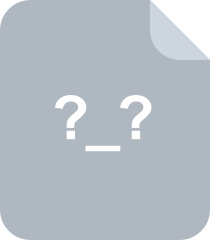
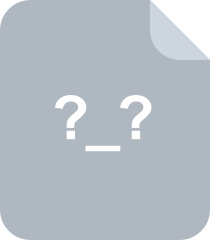
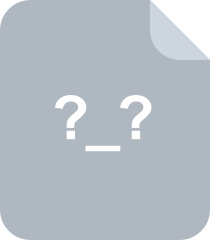
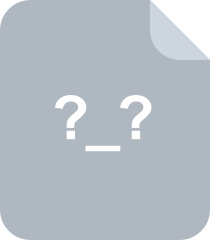
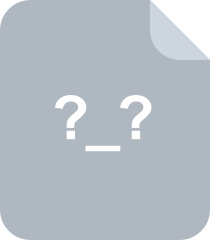
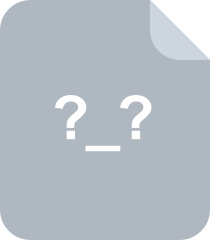
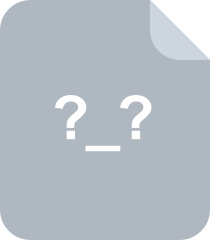
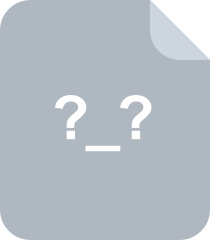
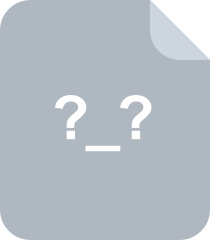
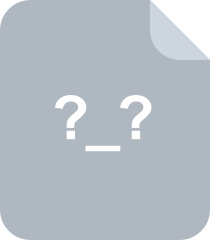
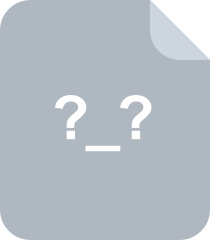
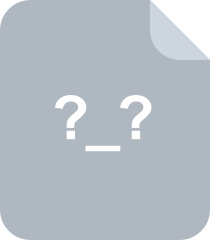
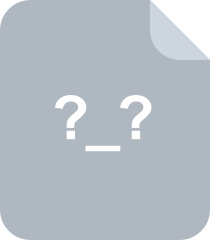
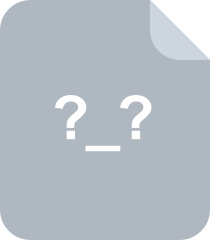
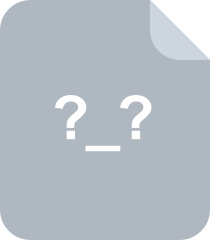
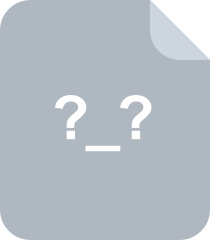
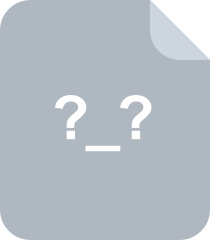
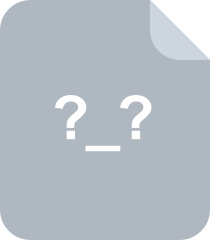
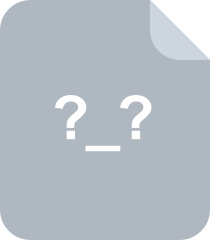
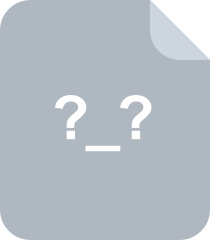
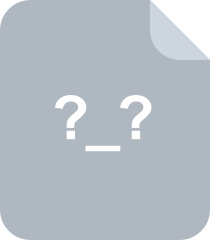
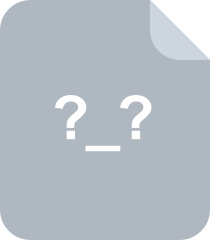
共 1027 条
- 1
- 2
- 3
- 4
- 5
- 6
- 11
资源评论
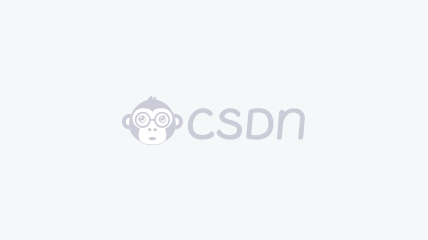

starry-star
- 粉丝: 1
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

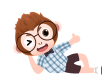
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


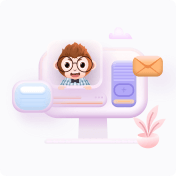
安全验证
文档复制为VIP权益,开通VIP直接复制
