# -*- coding: utf-8 -*-
# Environment PyCharm
# File_name NirCmd-Gui-Chinese |User Pfolg
# 2024/10/11 11:42
# 基于nircmd v2.87
import json
import os
import tkinter as tk
from tkinter import ttk, messagebox, filedialog, scrolledtext
import threading
import subprocess
url = "http://nircmd.nirsoft.net/"
defaultFont = ("Segoe UI", 10)
"请将这个程序与nircmd.exe放到同一父目录下,或者将nircmd.exe添加到环境变量"
# path = r"D:\PycharmProjects\pythonProject\Practice_project\NirCmd-Chinese\nircmd.exe"
def setConfig():
if not os.path.exists(".\\Config.json"):
with open(".\\Config.json", "w", encoding="utf-8") as setfile:
json.dump({"path": None}, setfile, ensure_ascii=False, indent=4)
return False
else:
with open(".\\Config.json", "r", encoding="utf-8") as setFile:
setDict = json.load(setFile)
p = setDict.get("path")
return p
path = setConfig()
def MakeSetting(key, p):
with open("Config.json", "r", encoding="utf-8") as setfile:
setDict = json.load(setfile)
setDict[key] = p
try:
with open("Config.json", "w", encoding="utf-8") as setFile:
json.dump(setDict, setFile, ensure_ascii=False, indent=4)
except BaseException:
messagebox.showerror("写入失败", "写入失败!") # 完美的代码是不会执行这一步的
def find_files(x):
win = tk.Tk()
win.withdraw()
file_path = filedialog.askopenfilename()
x.set(file_path)
win.destroy()
def find_directory(x):
win = tk.Tk()
win.withdraw()
file_path = filedialog.askdirectory()
x.set(file_path)
win.destroy()
# 复用率最高的函数!!!
def SecondRun(args=None, program=path, timeout=None):
if not args:
args = []
if program:
threading.Thread(target=lambda: subprocess.run([program] + args, timeout=timeout)).start()
else:
messagebox.showerror("路径错误!", "请先指定NirCmd.exe的路径!")
# 默认
funcTab1_1 = {
"蜂鸣声": lambda: SecondRun(args=["beep", "500", "2000"]),
"标准蜂鸣声": lambda: SecondRun(["stdbeep"]),
"终止关机": lambda: SecondRun(args=["abortshutdown"]),
"清空回收站": lambda: SecondRun(args=["emptybin"]),
"重启": lambda: SecondRun(args=["exitwin", "reboot"]),
"注销": lambda: SecondRun(["exitwin", "logoff"]),
"PowerOff": lambda: SecondRun(["exitwin", "poweroff"]),
"简单关机": lambda: SecondRun(["exitwin", "shutdown"]),
"在60秒内关闭系统": lambda: SecondRun(
["initshutdown", "Shut down the system within 60 seconds", "60", "reboot"]),
"锁定": lambda: SecondRun(["lockws"]),
"重启资源管理器1": lambda: os.system("taskkill /f /im explorer.exe && start explorer.exe"),
"重启资源管理器2": lambda: SecondRun(["restartexplorer"]),
"屏幕保护程序": lambda: SecondRun(["screensaver"]),
}
# 声音
funcTab1_2 = {
# changeappvolume iexplore.exe -0.2
# changeappvolume wmplayer.exe 0.55 Speakers
# changeappvolume /1275 -0.25 1
# changeappvolume Firefox.exe 0.5
"音量+2": lambda: SecondRun(["changesysvolume", "2000"]),
"音量-2": lambda: SecondRun(["changesysvolume", "-2000"]),
# Similar to changesysvolume, but instead of changing the whole sound volume, changesysvolume2 changes the left
# channel and the right channel separately. Examples: changesysvolume2 1000 -1000 changesysvolume2 -3000 0
# changesysvolume2 0 -5000 waveout changesysvolume2 -3000 0 master 1
"左音+2,右音-2": lambda: SecondRun(["changesysvolume2", "2000", "-2000"]),
"左音-2,右音+2": lambda: SecondRun(["changesysvolume2", "-2000", "2000"]),
"重置左右声道": lambda: SecondRun(["setvolume", "0", "0", "0"]), # http://nircmd.nirsoft.net/setvolume.html
# https://zh.101-help.com/7263afc0c3-windows-10zhong-left-and-right-channel-adjust-audio-balance/
}
# 显示
funcTab1_3 = {
"增加亮度": lambda: SecondRun(["changebrightness", "10"]),
"减小亮度": lambda: SecondRun(["changebrightness", "-10"]),
"关闭显示器": lambda: SecondRun(["monitor", "off"]),
"关闭显示器2": lambda: SecondRun(["monitor", "async_off"]),
"60秒后关闭显示器": lambda: SecondRun(["cmdwait", "60000", "monitor", "off"]),
"显示器低功耗": lambda: SecondRun(["monitor", "low"]),
}
# 粘贴板
funcTab1_4 = {
"清除粘贴板": lambda: SecondRun(["clipboard", "clear"]),
"打开Windows粘贴板": lambda: messagebox.showinfo("clipboard", "按win键+V键,即可呼出Windows粘贴板"),
"其他": lambda: messagebox.showinfo(
"clipboard", (
"set - 将指定文本设置到剪贴板。\n"
"readfile - 将指定文本文件的内容设置到剪贴板。\n"
"clear - 清除剪贴板。\n"
"writefile - 将剪贴板的内容写入到文件。(仅文本)\n"
"writeufile - 将剪贴板的内容写入到Unicode文件。(仅文本)\n"
"addfile - 将剪贴板的内容添加到文件。(仅文本)\n"
"addufile - 将剪贴板的内容添加到Unicode文件。(仅文本)\n"
"saveimage - 将剪贴板中的当前图像保存到文件。\n"
"copyimage - 将指定图像文件的内容复制到剪贴板。\n"
"saveclp - 将当前剪贴板数据保存到Windows .clp文件。\n"
"loadclp - 将Windows .clp文件加载到剪贴板。")
),
# set - 将指定文本设置到剪贴板。
# readfile - 将指定文本文件的内容设置到剪贴板。
# clear - 清除剪贴板。
# writefile - 将剪贴板的内容写入到文件。(仅文本)
# writeufile - 将剪贴板的内容写入到Unicode文件。(仅文本)
# addfile - 将剪贴板的内容添加到文件。(仅文本)
# addufile - 将剪贴板的内容添加到Unicode文件。(仅文本)
# saveimage - 将剪贴板中的当前图像保存到文件。
# copyimage - 将指定图像文件的内容复制到剪贴板。
# saveclp - 将当前剪贴板数据保存到Windows .clp文件。
# loadclp - 将Windows .clp文件加载到剪贴板。
}
# VIP程序专属!复制一个文件的创建/修改/访问时间到一个或多个文件。
def funcTab1_5(tab1_5_func):
# 标签
ttk.Label(tab1_5_func, text=(
"复制一个文件的创建/修改/访问时间到一个或多个文件。创建/修改/访问时间是从 [源文件名] 参数指定的文件中获取,并设置到 [通配符] 参数指定的文件或通配符匹配的文件中。\n"
"示例:\n"
"clonefiletime \"c:\\temp\\file1.txt\" \"c:\\files\\*.txt\"\n"
"clonefiletime \"c:\\temp\\file1.txt\" \"c:\\temp\\file2.txt\"\n\n"
"Clone the Created / Modified / Accessed time of one file into one or more files.\n"
"The Created / Modified / Accessed time is taken from the file specified in[source filename] parameter\n"
"and set into the file or wildcard specified in[Wildcard] parameter.\n"
"Examples:\n"
"clonefiletime \"c:\\temp\\file1.txt\" \"c:\\files\\*.txt\"\n"
"clonefiletime \"c:\\temp\\file1.txt\" \"c:\\temp\\file2.txt\"\n"
)).place(relx=.02, rely=.02)
# 控件
originFileT5F, targetFileT5F = tk.StringVar(), tk.StringVar()
ttk.Label(tab1_5_func, text="源文件路径").place(relx=.1, rely=.5)
tk.Entry(tab1_5_func, textvariable=originFileT5F).place(relx=.1, rely=.6)
ttk.Button(tab1_5_func, text="浏览", width=8, command=lambda: find_files(originFileT5F)).place(relx=.3, rely=.6)
ttk.Label(tab1_5_func, text="目标文件路径").place(relx=.5, rely=.5)
tk.Entry(tab1_5_func, textvariable=targetFileT5F).place(relx=
没有合适的资源?快使用搜索试试~ 我知道了~
NirCmd-Chinese.zip
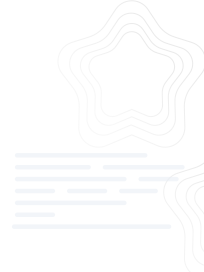
共3个文件
py:1个
md:1个
exe:1个

需积分: 0 0 下载量 174 浏览量
2024-10-19
00:51:24
上传
评论
收藏 10.18MB ZIP 举报
温馨提示
此程序是我的一个练习作品,单纯是为了提升编程水平,次要是为了做一个NirCmd的Gui,其实主要成分还是Gui,核心代码就两三行。 主要是Gui,功能基于 [nircmd v2.87] 实现,程序本身不提供一些重要的功能。 集成(于2024/11发布):https://github.com/Pfolg/PGBox 单发布:https://github.com/Pfolg/Pfolg_Source/releases/tag/v.max_NirCmd-Gui-Chinese
资源推荐
资源详情
资源评论
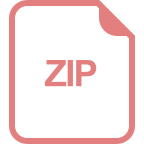
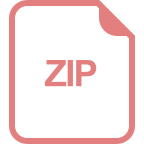
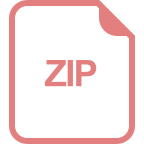
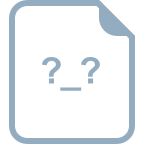
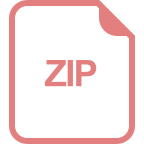
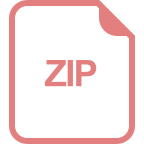
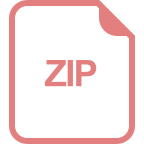
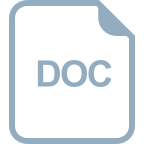
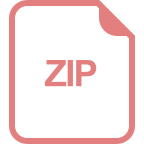
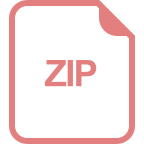
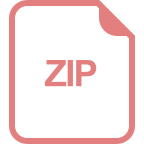
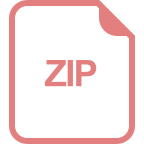
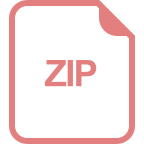
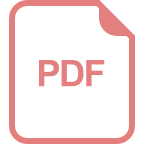
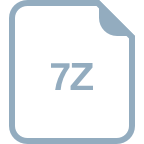
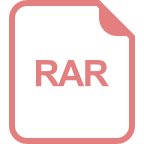
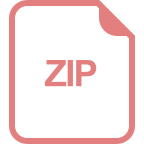
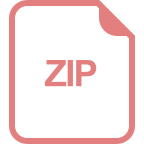
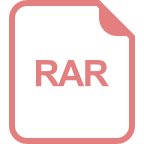
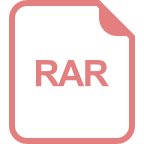
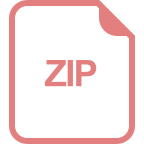
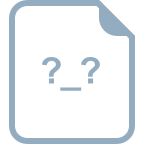
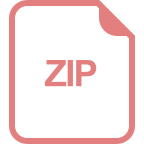
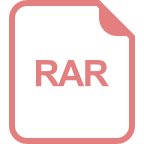
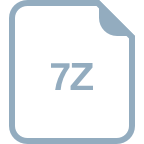
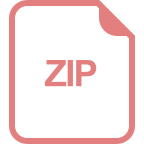
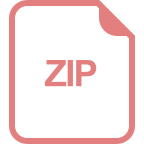
收起资源包目录

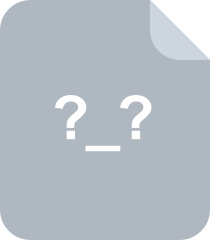
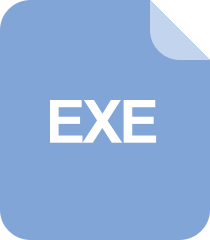
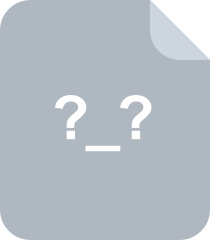
共 3 条
- 1
资源评论
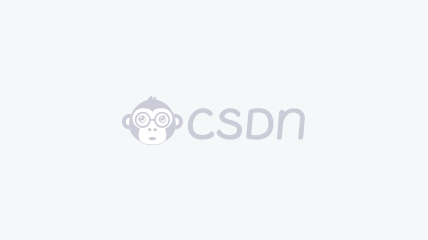

Pfolg
- 粉丝: 363
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

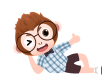
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


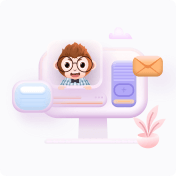
安全验证
文档复制为VIP权益,开通VIP直接复制
