[TOC]
# tvm.autotvm.MYGA
包含改进遗传算法的各类参数的枚举类及对应工具类,包括selection,crossover,mutation,aga,sa
包含配置类,用以设置遗传算法的各类参数
包含ga,用以进行遗传算法的迭代
## tvm.autotvm.MYGA.selection
包含枚举类EnumSelection和工具类GASelection
### EnumSelection
继承Enum,包含已配置选择算子种类,并可返回一随机选择算子
```python
class EnumSelection(Enum):
dup = 0
ecs = 1
etour = 2
otos = 3
rcs = 4
rps = 5
rws = 6
sus = 7
tour = 8
urs = 9
crws = 10
@staticmethod
def randomSelection():
l = len(EnumSelection)
idx = np.random.randint(l)
selection = EnumSelection(idx)
return selection
```
### GASeletion
提供具体选择算子函数,包括dup,ecs等11种选择算子函数
如
```python
@staticmethod
def dup(genes, scores, num=2):
tmp_scores = np.copy(scores)[:, np.newaxis]
p1 ,p2 = geatpy.dup(tmp_scores, num)
p1 %= len(genes)
p2 %= len(genes)
return p1, p2
```
## tvm.autotvm.MYGA.crossover
包含枚举类EnumCrossover和工具类GACrossover
### EnumCrossover
继承Enum,包含已配置交叉算子种类,并可返回一随机交叉算子
```python
class EnumCrossover(Enum):
spc = 0 # single point cross
tpc = 1 # two point cross
mpc = 2 # multipoint cross
ec = 3 # even cross
etpc = 4 # Even two-point cross
dc = 5 # Discrete cross
ac = 6 # Arithmetic cross
hc = 7 # Heuristic cross
@staticmethod
def randomCrossover():
l = len(EnumCrossover)
idx = np.random.randint(l)
crossover = EnumCrossover(idx)
return crossover
```
### GACrossover
提供具体交叉算子函数,包括spc,tpc等8种交叉算子函数
如
```python
@staticmethod
def spc(g1, g2, size):
if size >= 1:
point = np.random.randint(size)
tmp_gene = g1[:point] + g2[point:]
return tmp_gene
return g1
```
## tvm.autotvm.MYGA.mutation
包含枚举类EnumMutation和工具类GAMutation
### EnumMutation
继承Enum,包含已配置变异算子种类,并可返回一随机变异算子
```python
class EnumMutation(Enum):
rm = 0 # random mutation
cm = 1 # crossover mutation
sm = 2 # swap mutation
im = 3 # inversion mutation
Rm = 4
@staticmethod
def randomMutation():
l = len(EnumMutation)
idx = np.random.randint(l)
mutation = EnumMutation(idx)
return mutation
```
### GAMutation
提供具体变异算子函数,包括rm,cm等5种变异算子函数
如
```python
@staticmethod
def rm(gene, dims, rate):
for j, dim in enumerate(dims):
if np.random.random() < rate:
gene[j] = np.random.randint(dim)
return gene
```
## tvm.autotvm.MYGA.aga
增加自适应遗传算法,根据适应度动态调整个体交叉率和变异率,包含枚举类EnumAGA和工具类AGA
### EnumAGA
继承Enum,包含已配置AGA算子种类,并可返回一随机AGA算子
```python
class EnumAGA(Enum):
GA = 0 # normal Genetic Algorithm
AGA = 1 # Adaptive Genetic Algorithm
LAGA = 2 # Linear Adaptive Genetic Algorithm
CAGA = 3 # Cosine Adaptive Genetic Algorithm
IAGA = 4 # Improved Adaptive Genetic Algorithm
@staticmethod
def randomAGA():
l = len(EnumAGA)
idx = np.random.randint(l)
aga = EnumAGA(idx)
return aga
```
### AGA
提供具体AGA算子函数,包括GA,AGA等5种AGA算子函数
如
```python
@staticmethod
def AGA(k1, k2, f_max, f_avg, f, target):
if f < f_avg:
return k2
else:
return k1 * (f_max - f) / (f_max - f_avg)
```
## tvm.autotvm.MYGA.sa
增加模拟退火算法(SA),搭配GA使用,包含枚举类EnumAGA,具体算法见[tvm.autotvm.MYGA.ga.](#tvm.autotvm.MYGA.ga)
### EnumSA
继承Enum,包含已配置SA算子种类,并可返回一随机SA算子
```python
class EnumSA(Enum):
GA = 0
GA_SA1 = 1
GA_SA2 = 2
@staticmethod
def randomSA():
l = len(EnumSA)
idx = np.random.randint(l)
sa = EnumSA(idx)
return sa
```
## tvm.autotvm.MYGA.config
包含GA各参数对应配置类,包括SelectionConfig,CrossoverConfig,MutationConfig,ExtraConfig,PopulationConfig,MultipopulationConfig
### SelectionConfig
设置选择算子,其类型必须是EnumSelection或其name对应的str
### CrossoverConfig
设置交叉算子,其类型必须是EnumCrossover或其name对应的str
设置交叉率及最大最小值
### MutationConfig
设置变异算子,其类型必须是EnumMutation或其name对应的str
设置变异率及最大最小值
### ExtraConfig
设置AGA,其类型必须是EnumAGA或其name对应的str
设置SA,其类型必须是EnumSA或其name对应的str
### PopulationConfig
设置种群参数,包括种群规模,精英数量,SelectionConfig,CrossoverConfig,MutationConfig,ExtraConfig
输入为dict类型,例
```python
config = {
"pop_size": 200,
"elite_num": 3,
"selection": {
"op": "dup" # "dup" also can be EnumSelection.dup
},
"crossover": {
"op": "tpc", # "tpc" also can be EnumCrossover.tpc
"rate": 0.6
},
"mutation": {
"op": "im", # "im" also can be EnumMutation.im
"rate": 0.001
}
}
```
### MultipopulationConfig
设置多种群参数,输入为dict类型,包含各种群对应参数,例
```python
config = {
"config1" : {
"pop_size": 100,
"elite_num": 3,
"selection": {
"op": "dup"
},
"crossover": {
"op": "tpc",
"rate": 0.6
},
"mutation": {
"op": "im2",
"rate": 0.02
}
},
"config2": {
"pop_size": 200,
"elite_num": 4,
"selection": {
"op": "rws"
},
"crossover": {
"op": "spc",
"rate": 0.6
},
"mutation": {
"op": "rm",
"rate": 0.001
}
},
}
```
## tvm.autotvm.MYGA.ga
用以进行遗传算法的具体计算,包括类Solution,Population,Multipopulation
### Solution
种群个体,包括基因及适应度
```python
class Solution:
def __init__(self, gene, score=0.0):
self.gene = gene
self.score = score
```
### Population
核心类,单种群GA,下面具体介绍其函数
#### Population.\_\_init\_\_
```python
def __init__(self, tunerConfig, popConfig : PopulationConfig):
self.tunerConfig = tunerConfig # tuner config,don't need to care
self.popConfig = popConfig # pop config,set selection op and other op
self.space = len(self.tunerConfig.space) # get search space size
self.popConfig.setSize(min(self.popConfig.getSize(), self.space)) # pop size can't exceed the search space size
self.solutions = [] # pop individuals
self.elites = [] # pop elites
self.genes = [] # individual's gene
self.scores = [] # individual's score
self.tmp_solution = []
self.next_solution = []
self.next_batch = []
self.next_id = -1 # index of next individual
```
其中tunerConfig为搜索空间参数,tuner自动生成,无需关注
popConfig为种群配置类,包括该种群的各具体参数
#### Popolation.init
产生足够的随机
```python
def init(self):
# random initialization
for _ in range(self.popConfig.getSize()):
tmp_gene = point2knob(np.random.randint(self.space), self.tunerConfig.dims)
tmp_solution = Solution(tmp_gene)
self.solutions.append(tmp_solution)
```
#### Population.update
```python
def update(self):
# check whether to
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
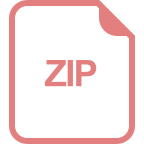
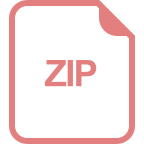
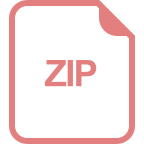
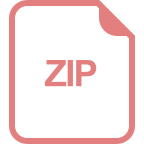
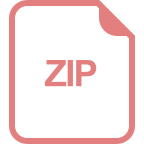
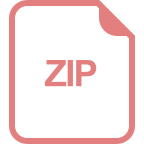
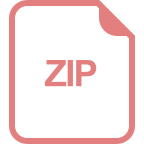
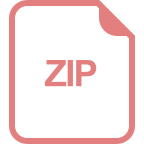
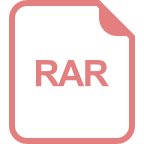
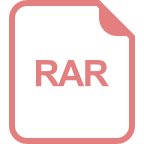
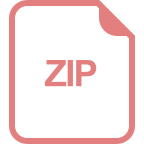
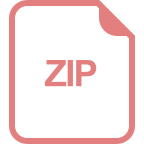
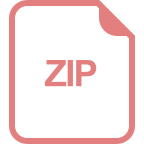
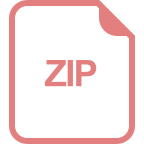
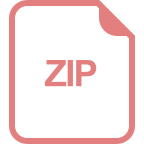
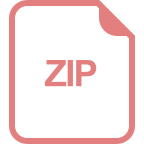
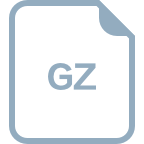
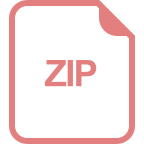
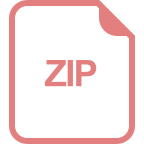
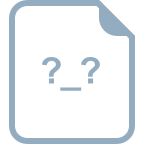
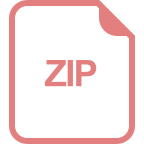
收起资源包目录

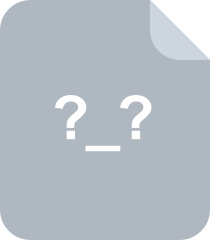
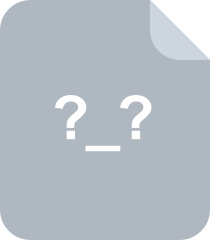
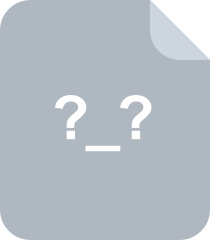
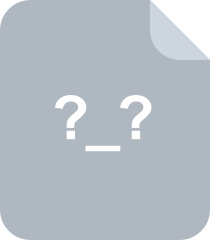
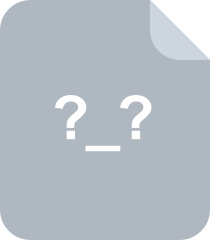
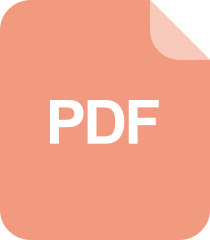
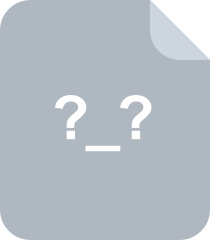
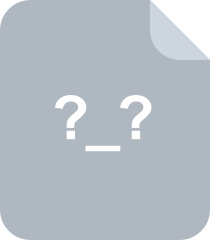
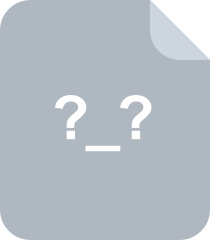
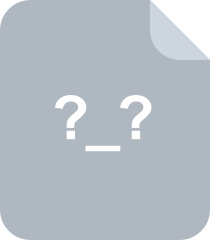
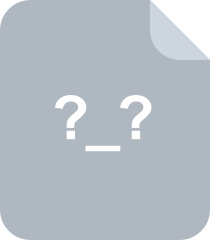
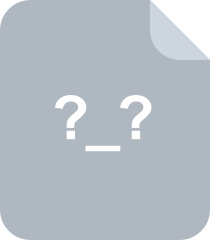
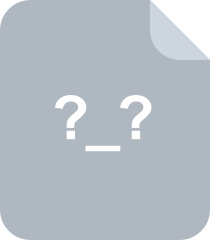
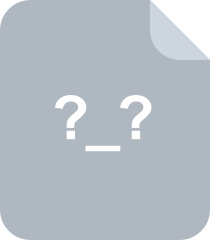
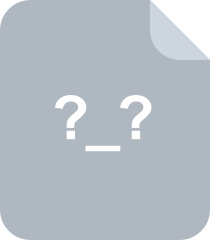
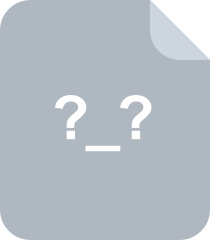
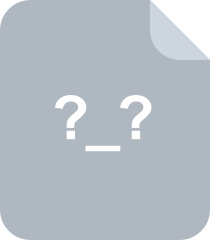
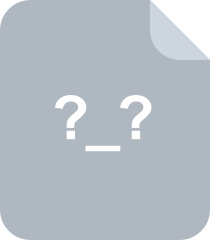
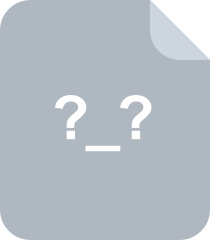
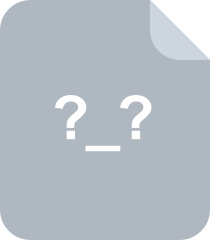
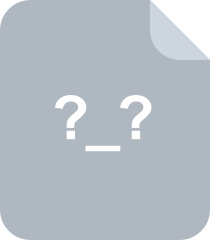
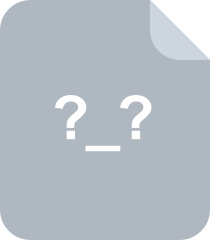
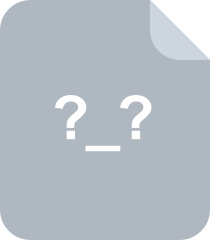
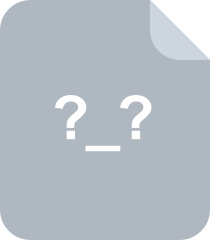
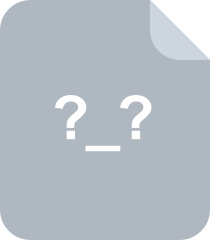
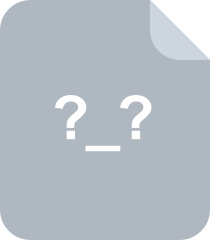
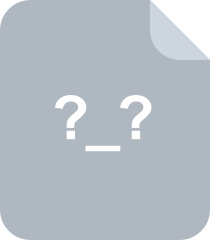
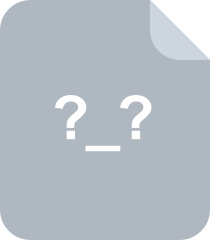
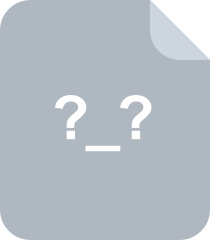
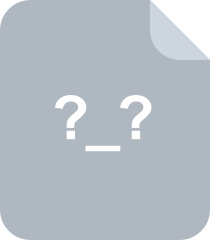
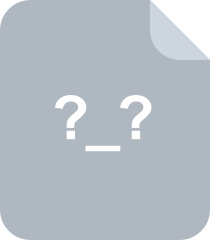
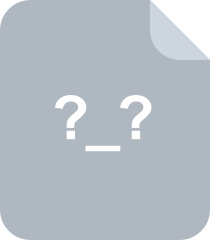
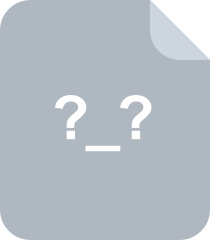
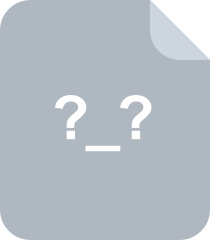
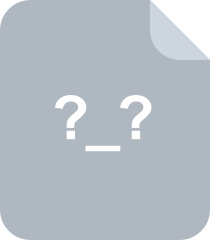
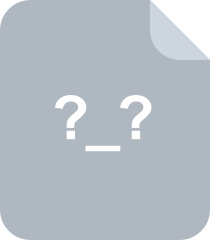
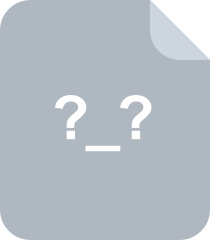
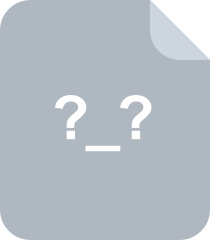
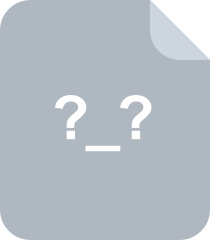
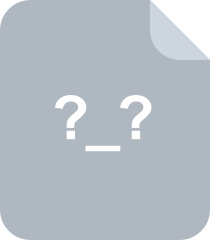
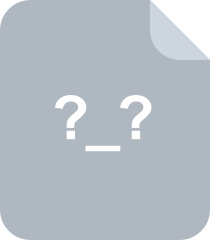
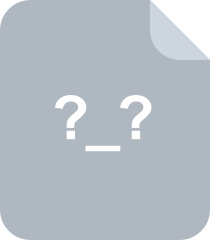
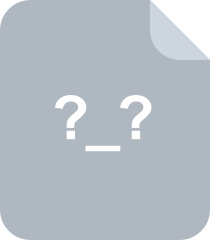
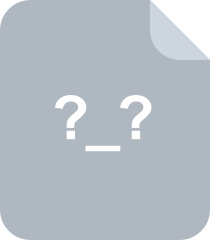
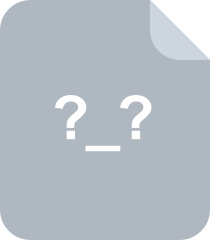
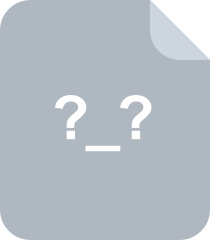
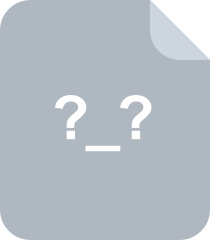
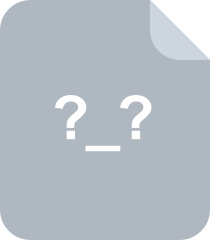
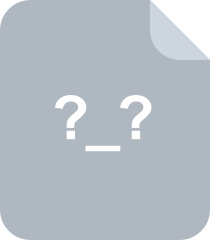
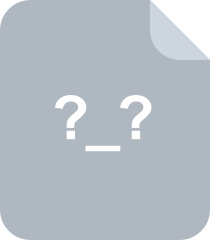
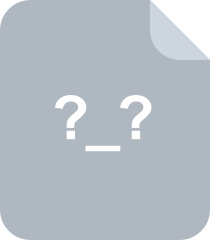
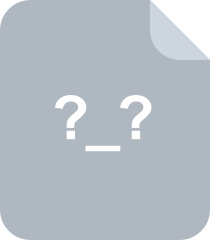
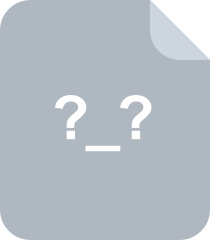
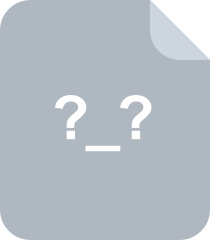
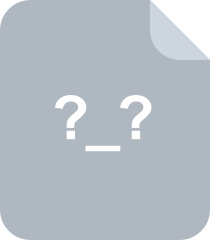
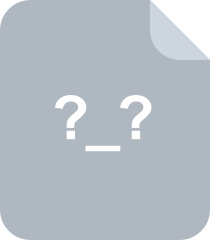
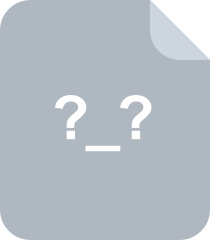
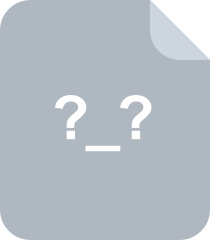
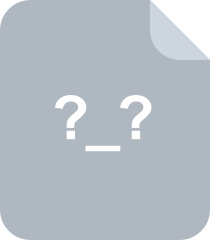
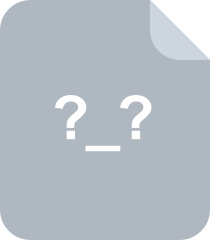
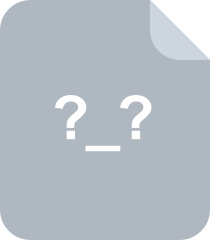
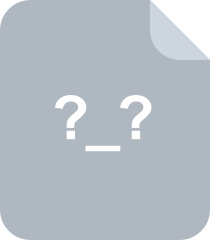
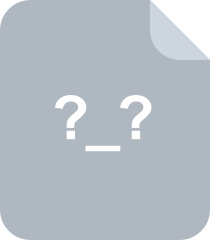
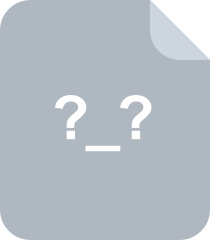
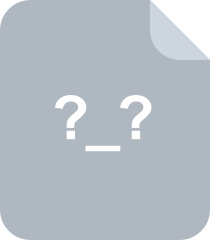
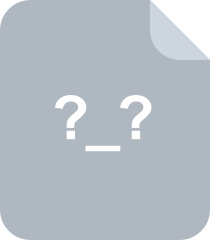
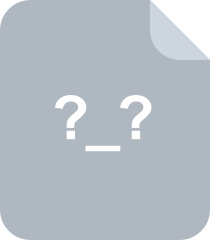
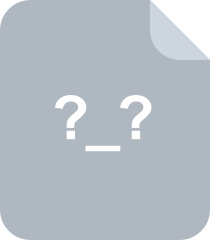
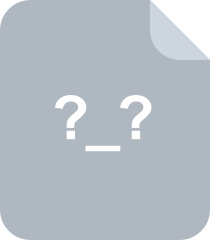
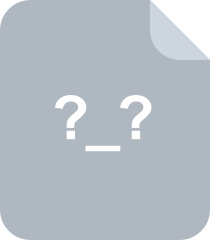
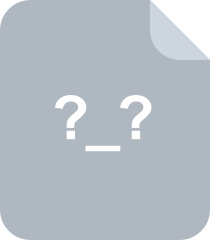
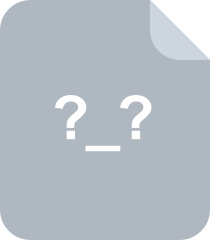
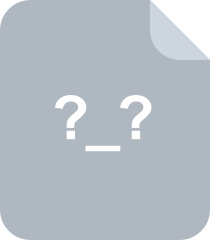
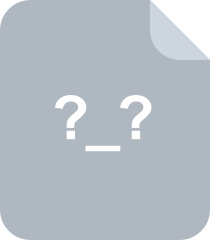
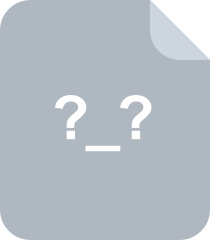
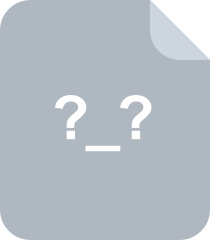
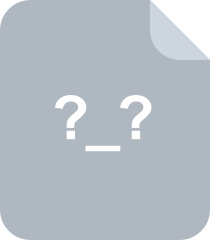
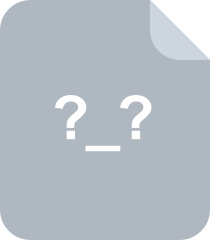
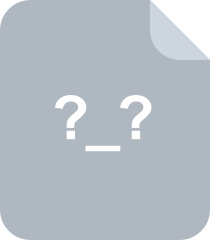
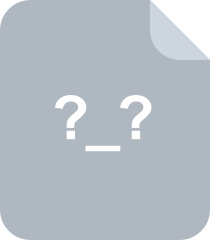
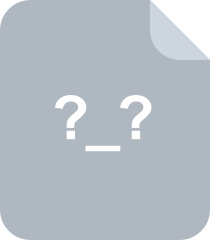
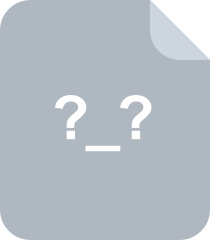
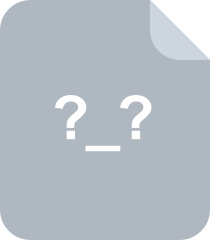
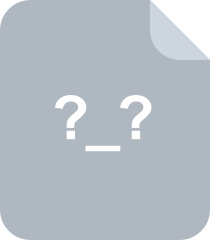
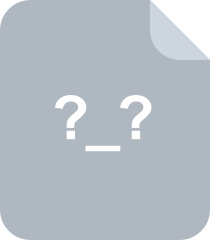
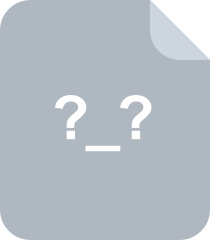
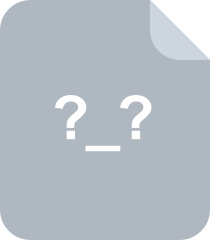
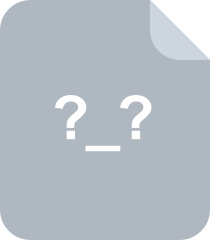
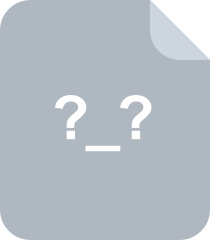
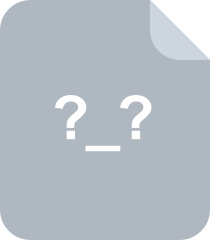
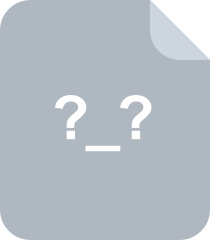
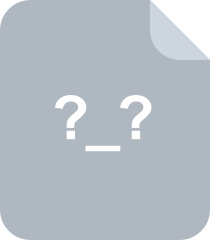
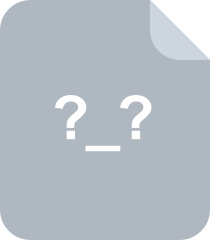
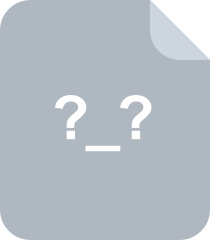
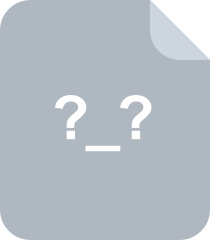
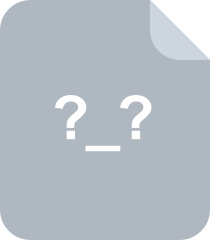
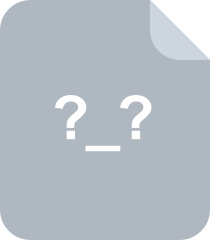
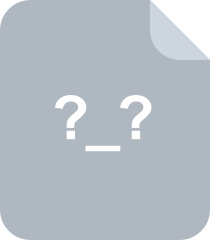
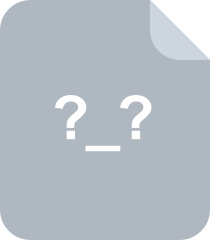
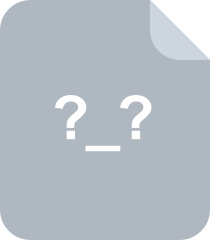
共 1921 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
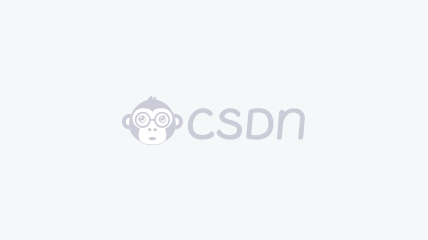

好家伙VCC
- 粉丝: 2151
- 资源: 9145

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

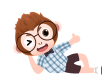
最新资源
- bdwptqmxgj11.zip
- onnxruntime-win-x86
- onnxruntime-win-x64-gpu-1.20.1.zip
- vs2019 c++20 语法规范 头文件 <ratio> 的源码阅读与注释,处理分数的存储,加减乘除,以及大小比较等运算
- 首次尝试使用 Win,DirectX C++ 中的形状渲染套件.zip
- 预乘混合模式是一种用途广泛的三合一混合模式 它已经存在很长时间了,但似乎每隔几年就会被重新发现 该项目包括使用预乘 alpha 的描述,示例和工具 .zip
- 项目描述 DirectX 引擎支持版本 9、10、11 库 Microsoft SDK 功能相机视图、照明、加载网格、动画、蒙皮、层次结构界面、动画控制器、网格容器、碰撞系统 .zip
- 项目 wiki 文档中使用的代码教程的源代码库.zip
- 面向对象的通用GUI框架.zip
- 基于Java语言的PlayerBase游戏角色设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


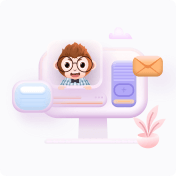
安全验证
文档复制为VIP权益,开通VIP直接复制
