package com.ahpu.util;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import java.sql.Timestamp;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.ParsePosition;
import java.text.SimpleDateFormat;
import java.util.*;
/**
* 时间类的工具类
*
*
*/
public class DateUtil {
private static final TimeZone timeZone = TimeZone.getTimeZone("GMT+08:00");
private static Log log = LogFactory.getLog(DateUtil.class);
/**
* 获取现在的年月
*
*/
public static String getTimeNY() {
SimpleDateFormat sf = new SimpleDateFormat("yyyy-MM");
return sf.format(new Date());
}
public static String getTimeSSS() {
SimpleDateFormat sdf = new SimpleDateFormat("SSS");
String format = sdf.format(new Date());
return format;
}
public static String dateToString(@NotNull Date date) {
return new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(date);
}
public static String dateToString(@NotNull String template, @NotNull Date date) {
return new SimpleDateFormat(template).format(date);
}
/**
* 获取两个时间中的所有时间
*
*/
public static List<String> getBetweenDates(String dateStart, String dataEnd) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Date begin = null;
Date end = null;
try {
begin = sdf.parse(dateStart);
end = sdf.parse(dataEnd);
} catch (ParseException e) {
log.error(e.toString());
}
List<String> result = new ArrayList<String>();
Calendar tempStart = Calendar.getInstance();
tempStart.setTime(begin);
while (begin.getTime() <= end.getTime()) {
result.add(sdf.format(tempStart.getTime()));
tempStart.add(Calendar.DAY_OF_YEAR, 1);
begin = tempStart.getTime();
}
return result;
}
public static String thisTime() {
SimpleDateFormat fs = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
return fs.format(new Date());
}
/**
* 将时间格式化为指定格式
*
*/
public static String formatDefinition(String time, String format) {
SimpleDateFormat fs = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.S");
SimpleDateFormat f = new SimpleDateFormat(format);
Date date;
try {
date = new Date(fs.parse(time).getTime());
} catch (ParseException e) {
try {
f.parse(time);
return time;
} catch (ParseException e1) {
return "FormatError";
}
}
return f.format(date);
}
/**
* 将时间格式化为指定格式
*
*/
public static String formatDefinition(String time, String format, String zdyFormat) {
SimpleDateFormat fs = new SimpleDateFormat(zdyFormat);
SimpleDateFormat f = new SimpleDateFormat(format);
Date date;
try {
date = new Date(fs.parse(time).getTime());
} catch (ParseException e) {
try {
f.parse(time);
return time;
} catch (ParseException e1) {
return "FormatError";
}
}
return f.format(date);
}
/**
* 把yyyy-MM-dd HH:mm:ss.S格式的时候格式化为yyyy-MM-dd HH:mm:ss格式
*
*/
public static String formatDeleteSecond(String time) {
SimpleDateFormat f = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
SimpleDateFormat fs = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.S");
Date date;
try {
date = new Date(fs.parse(time).getTime());
} catch (ParseException e) {
try {
f.parse(time);
return time;
} catch (ParseException e1) {
return "Error";
}
}
return f.format(date);
}
/**
* @param day 推移的天数
* @return date时间加天数的日期
*/
public static String addDate(String date, int day) {
GregorianCalendar calendar = new GregorianCalendar();
calendar.setTime(getDate(date, "yyyy-MM-dd"));
calendar.add(GregorianCalendar.DAY_OF_MONTH, day);
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd");
return format.format(calendar.getTime());
}
/**
* @param date 当前日期值
* @param year 推移的年数
* @param formatStr 返回的格式
* @return date时间加天数的日期
* @author Mengfw
*/
public static String addYear(String date, int year, String formatStr) {
GregorianCalendar calendar = new GregorianCalendar();
calendar.setTime(getDate(date, "yyyy-MM-dd"));
calendar.add(GregorianCalendar.YEAR, year);
SimpleDateFormat format = new SimpleDateFormat(formatStr);
return format.format(calendar.getTime());
}
public static String addDate2(String date, int day) {
GregorianCalendar calendar = new GregorianCalendar();
calendar.setTime(getDate(date, "yyyyMMdd"));
calendar.add(GregorianCalendar.DAY_OF_MONTH, day);
SimpleDateFormat format = new SimpleDateFormat("yyyyMMdd");
return format.format(calendar.getTime());
}
public static String addWeek(String date, int week) {
GregorianCalendar calendar = new GregorianCalendar();
calendar.setTime(getDate(date, "yyyy-MM-dd"));
calendar.add(GregorianCalendar.WEEK_OF_YEAR, week);
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd");
return format.format(calendar.getTime());
}
public static String addMonth(String date, int month) {
GregorianCalendar calendar = new GregorianCalendar();
calendar.setTime(getDate(date, "yyyy-MM-dd"));
calendar.add(GregorianCalendar.MONTH, month);
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd");
return format.format(calendar.getTime());
}
/**
* 日期转换成字符串 把长日期 yyyy-MM-dd HH:mm 转换成短日期格式 yyyy-MM-dd
*/
public static String dateToString(String StrDate) {
SimpleDateFormat d = new SimpleDateFormat("yyyy-MM-dd");
String dd = d.format(isToDate(StrDate));
return dd;
}
/**
*
* 日期转换成字符串 把长日期 yyyy-MM-dd HH:mm 转换成短日期格式 yyyy-MM-dd HH:mm:ss
*/
public static String dateToStringSecond(String strDate) {
SimpleDateFormat d = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date date;
String dd;
try {
date = d.parse(strDate);
dd = d.format(date);
return dd;
} catch (Exception e) {
log.error(e.toString());
return "";
}
}
public static synchronized String formatDate(int dateNum) {
String result = "";
SimpleDateFormat formatter = new SimpleDateFormat("yyyyMMdd");
try {
Date date = formatter.parse(String.valueOf(dateNum));
SimpleDateFormat formatter1 = new SimpleDateFormat("yyyy-MM-dd");
result = formatter1.format(date);
} catch (Exception e) {
}
return result;
}
public static synchronized int formatDate(Date date) {
String result = "0";
try {
SimpleDateFormat formatter1 = new SimpleDateFormat("yyyyMMdd");
result = formatter1.format(date);
} catch (Exception e) {
}
return Integer.parseInt(result);
}
/**
* @param msel 毫秒时间
* @return 毫秒时间 所对应的"yyyy-MM-dd HH:mm"格式的日期
*/
public static String formatTime(long msel) {
Date date = new Date(msel);
SimpleDateFormat formatter = new SimpleDateFor
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
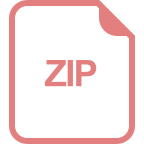
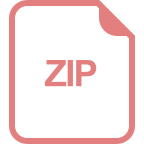
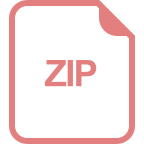
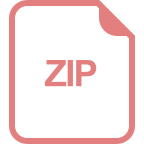
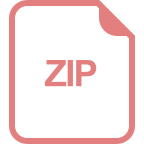
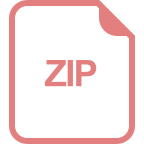
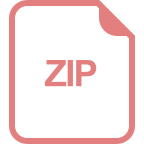
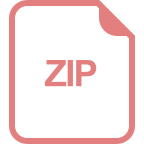
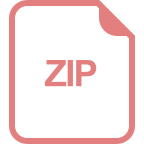
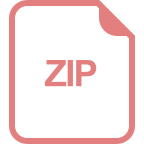
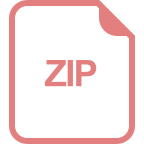
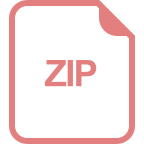
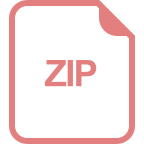
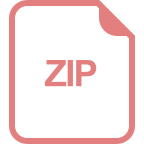
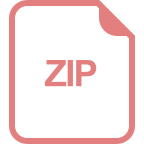
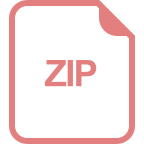
收起资源包目录

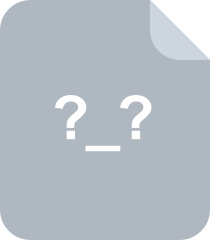
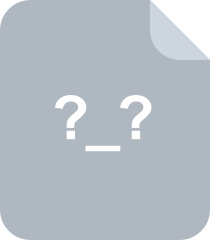
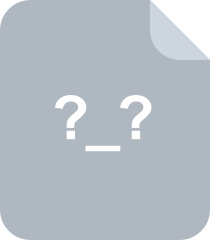
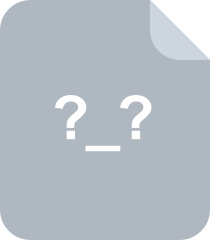
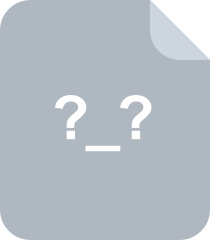
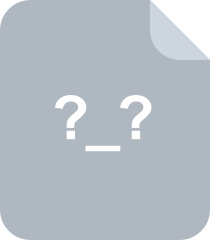
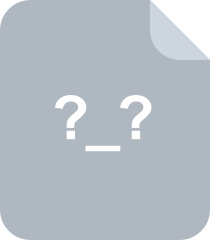
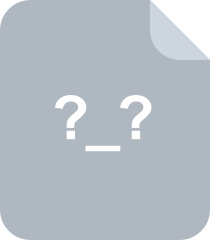
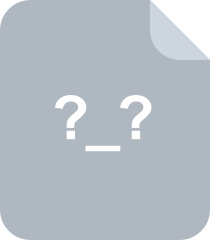
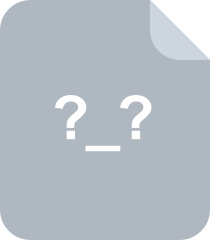
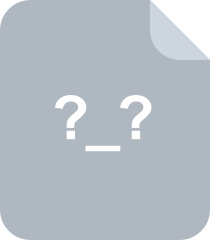
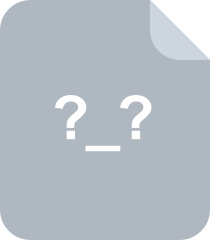
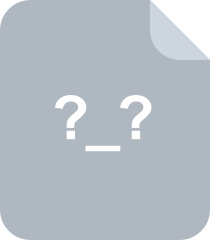
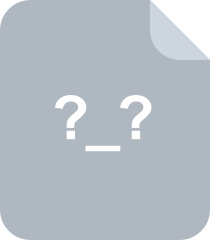
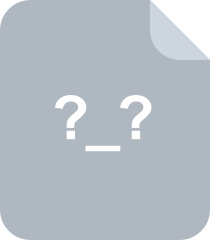
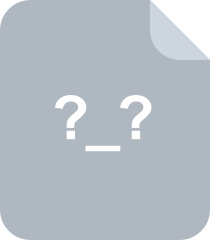
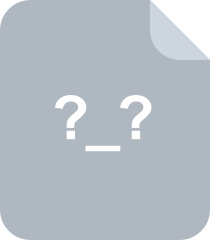
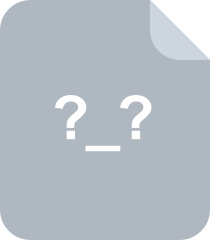
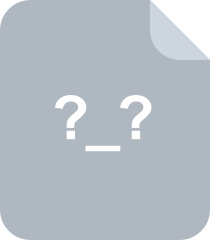
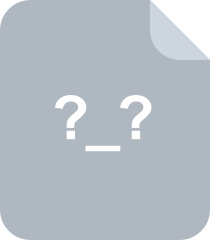
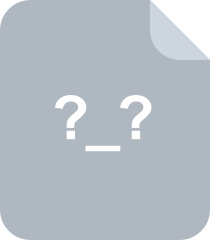
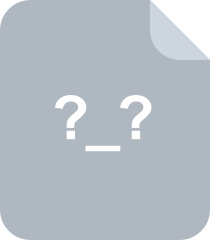
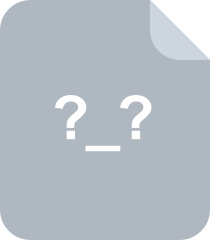
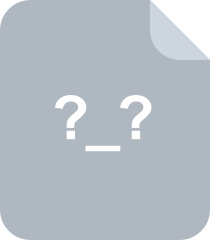
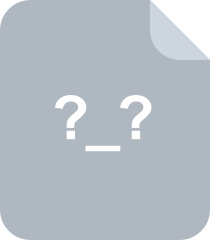
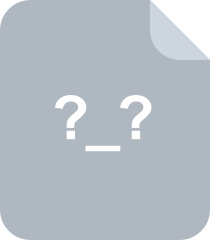
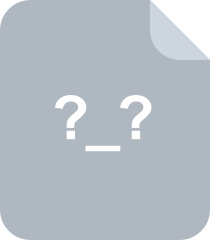
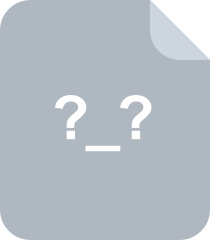
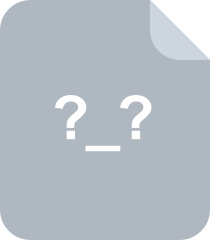
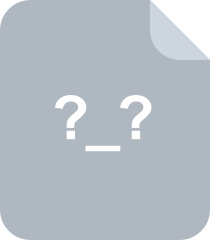
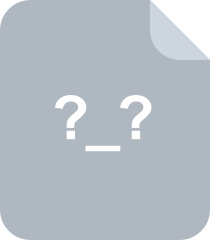
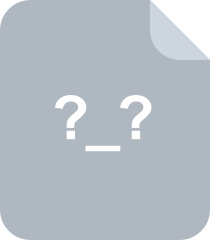
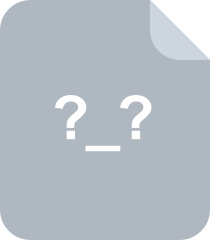
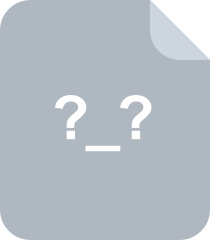
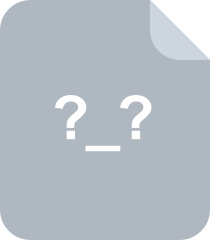
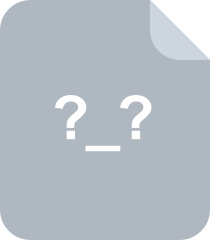
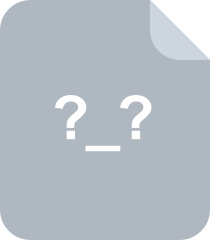
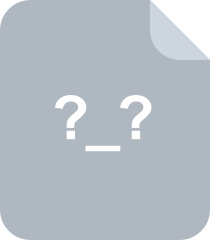
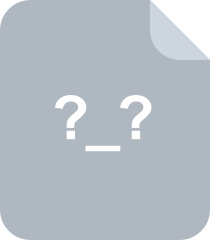
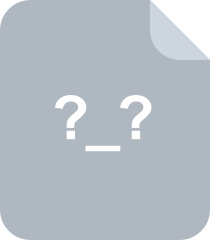
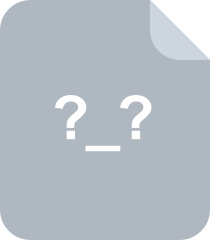
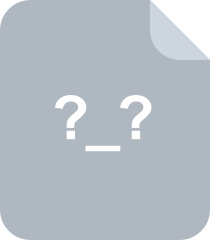
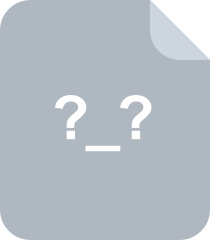
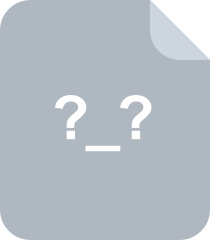
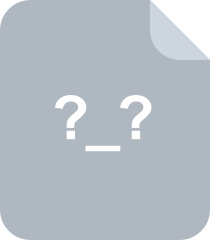
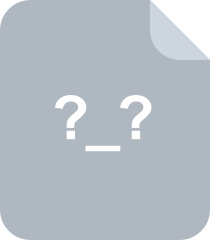
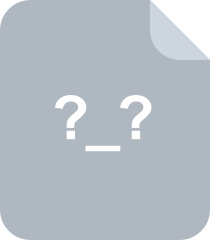
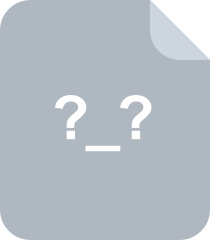
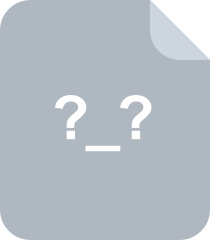
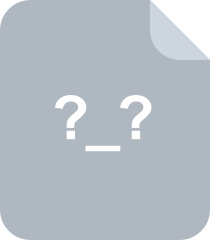
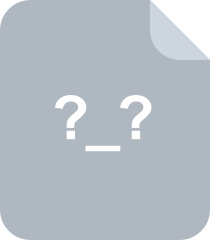
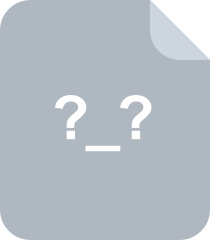
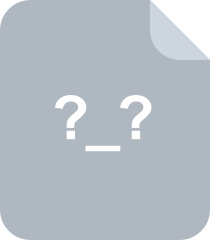
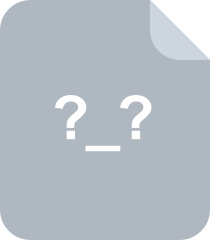
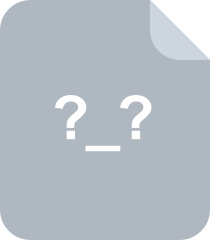
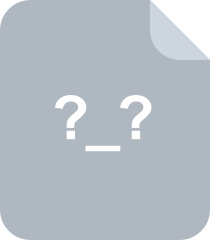
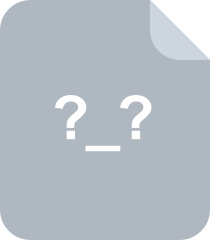
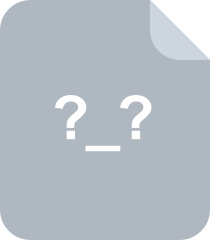
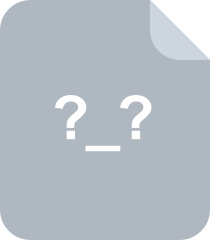
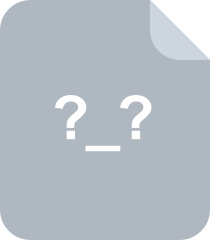
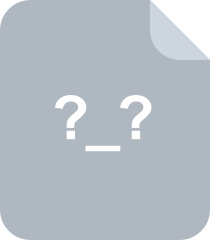
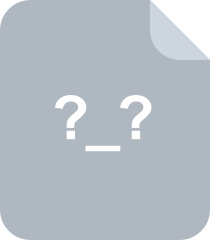
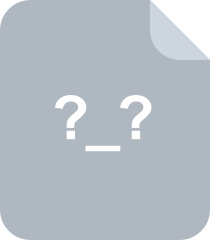
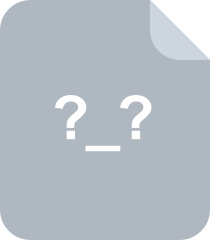
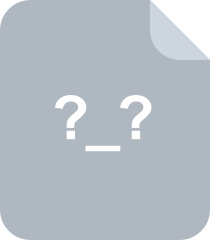
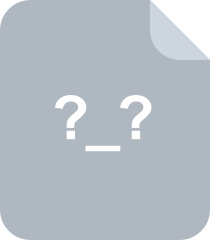
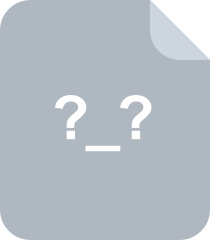
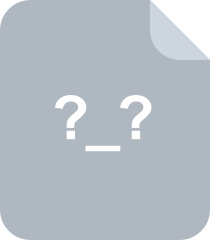
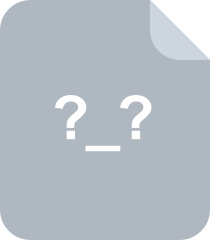
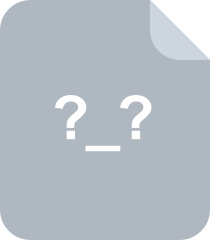
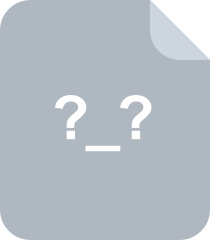
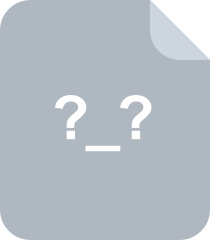
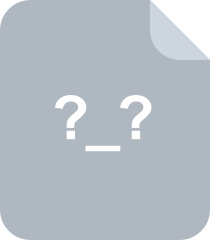
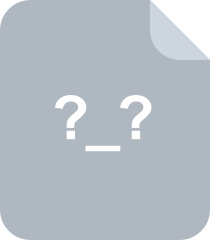
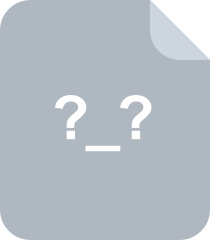
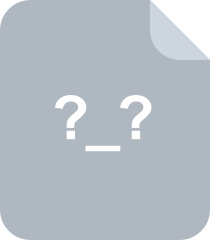
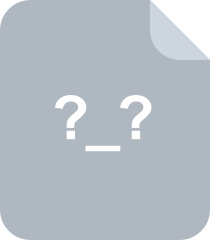
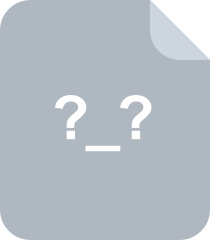
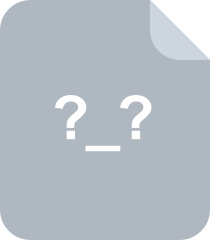
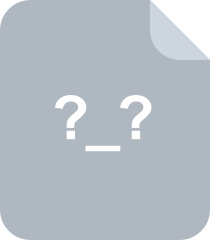
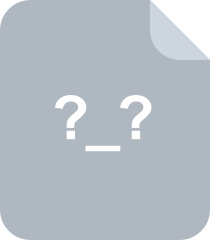
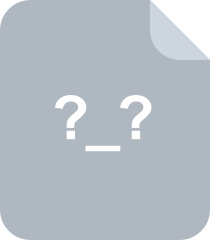
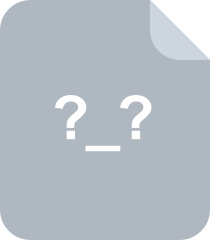
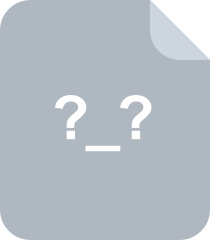
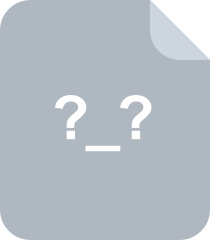
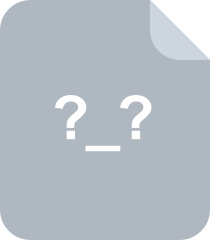
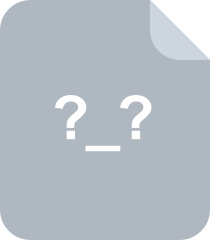
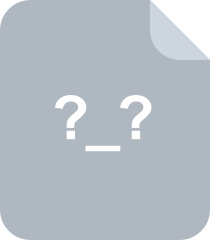
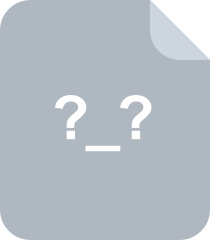
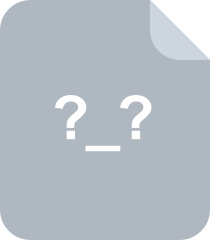
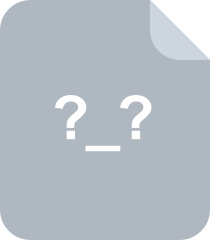
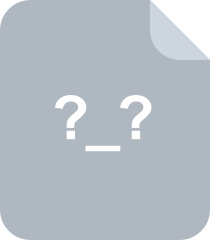
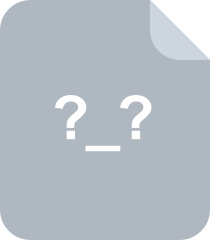
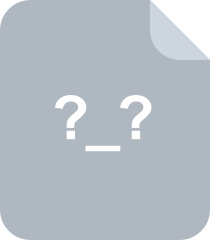
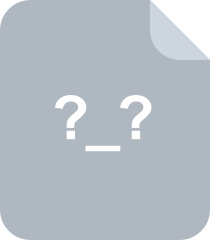
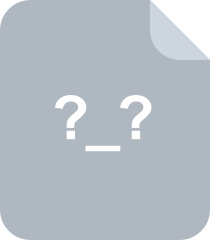
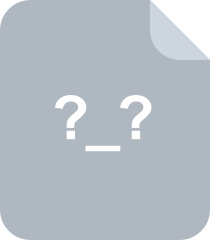
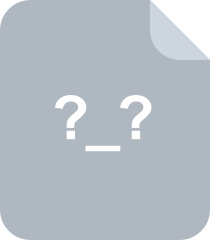
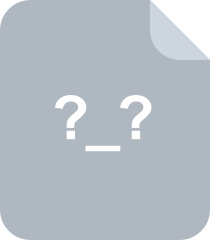
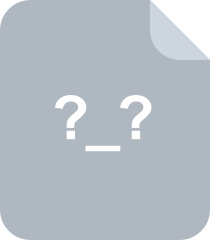
共 744 条
- 1
- 2
- 3
- 4
- 5
- 6
- 8
资源评论
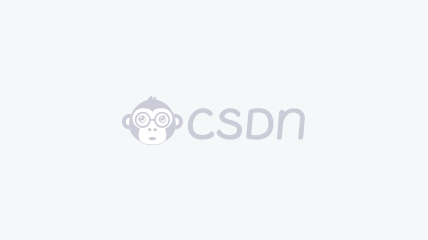

好家伙VCC
- 粉丝: 2303
- 资源: 9142
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

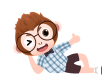
最新资源
- 资料阅读器(先下载解压) 5.0.zip
- 人、垃圾、非垃圾检测18-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 440379878861684smart-parking.zip
- 金智维RPA server安装包
- 二维码图形检测6-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord数据集合集.rar
- Matlab绘制绚丽烟花动画迎新年
- 厚壁圆筒弹性应力计算,过盈干涉量计算
- 实验八:实验程序202210409116武若豪.zip
- 网络实践11111111111111
- GO编写图片上传代码.txt
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


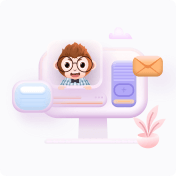
安全验证
文档复制为VIP权益,开通VIP直接复制
