#include <SPI.h>
#include <WiFi.h>
#include <WebServer.h>
#include <ESPmDNS.h>
#include <ArduinoJson.h>
#define ADS1299_PIN_RESET 25
#define ADS1299_PIN_DRDY 27 //data-ready output
#define ADS1299_PIN_SCK 14
#define ADS1299_PIN_MISO 12
#define ADS1299_PIN_MOSI 13
#define ADS1299_PIN_SS 15 //17
#define OPENBCI_DATA_BUFFER_SIZE 50
#define OPENBCI_NAME "OpenBCI-FFFF"
#define OPENBCI_VERSION "v2.0.5"
#define SOFT_AP_SSID "OpenBCI WiFi AP"
#define SOFT_AP_PASSWORD "12345678"
#define JSON_BUFFER_SIZE 1024
#define ADS_ID 0x3E // product ID for ADS1299
#define ID_REG 0x00 // this register contains ADS_ID
#define BOARD_ADS 15 // ADS chip select
#define _SDATAC 0x11 // Stop Read Data Continuous modeff
#define _RDATA 0x12 // Read data by command supports multiple read back
#define _RESET 0x06 // Reset the device registers to default
// Register Addresses
#define ID 0x00
#define CONFIG1 0x01
#define CONFIG2 0x02
#define CONFIG3 0x03
#define LOFF 0x04
#define CH1SET 0x05
#define CH2SET 0x06
#define CH3SET 0x07
#define CH4SET 0x08
#define CH5SET 0x09
#define CH6SET 0x0A
#define CH7SET 0x0B
#define CH8SET 0x0C
#define BIAS_SENSP 0x0D
#define BIAS_SENSN 0x0E
#define LOFF_SENSP 0x0F
#define LOFF_SENSN 0x10
#define LOFF_FLIP 0x11
#define LOFF_STATP 0x12
#define LOFF_STATN 0x13
#define GPIO 0x14
#define MISC1 0x15
#define MISC2 0x16
#define CONFIG4 0x17
// Test Signal Choices - p41
#define ADS1299_TEST_INT 0x10 //(0b00010000)
#define ADS1299_TESTSIGNAL_AMP_1X 0x00 //(0b00000000)
#define ADS1299_TESTSIGNAL_AMP_2X 0x40 //(0b00000100)
#define ADS1299_TESTSIGNAL_PULSE_SLOW 0x00 //(0b00000000)
#define ADS1299_TESTSIGNAL_PULSE_FAST 0x01 //(0b00000001)
#define ADS1299_TESTSIGNAL_DCSIG 0x03 //(0b00000011)
#define ADS1299_TESTSIGNAL_NOCHANGE 0xff //(0b11111111)
// SPI Command Definitions (Datasheet, 35)
#define _WAKEUP 0x02 // Wake-up from standby mode
#define _STANDBY 0x04 // Enter Standby mode
#define _RESET 0x06 // Reset the device registers to default
#define _START 0x08 // Start and restart (synchronize) conversions
#define _STOP 0x0A // Stop conversion
#define _RDATAC 0x10 // Enable Read Data Continuous mode (default mode at power-up)
#define _SDATAC 0x11 // Stop Read Data Continuous mode
#define _RDATA 0x12 // Read data by command; supports multiple read back
#define _RREG 0x20 // Read Register
#define _WREG 0x40 // Write to Register
// Gains
#define ADS1299_PGA_GAIN01 0x00 //(0b00000000)
#define ADS1299_PGA_GAIN02 0x10 //(0b00010000)
#define ADS1299_PGA_GAIN04 0x20 //(0b00100000)
#define ADS1299_PGA_GAIN06 0x30 //(0b00110000)
#define ADS1299_PGA_GAIN08 0x40 //(0b01000000)
#define ADS1299_PGA_GAIN12 0x50 //(0b01010000)
#define ADS1299_PGA_GAIN24 0x60 //(0b01100000)
// Input Modes - Channels
#define ADS1299_INPUT_PWR_DOWN 0x80 //(0b10000000)
#define ADS1299_INPUT_PWR_UP 0x00 //(0b00000000)
#define ADS1299_INPUT_NORMAL 0x00 //(0b00000000)
#define ADS1299_INPUT_SHORTED 0x01 //(0b00000001)
#define ADS1299_INPUT_MEAS_BIAS 0x02 //(0b00000010)
#define ADS1299_INPUT_SUPPLY 0x03 //(0b00000011)
#define ADS1299_INPUT_TEMP 0x04 //(0b00000100)
#define ADS1299_INPUT_TESTSIGNAL 0x05 //(0b00000101)
#define ADS1299_INPUT_SET_BIASP 0x06 //(0b00000110)
#define ADS1299_INPUT_SET_BIASN 0x07 //(0b00000111)
//Lead-off Signal Choices
#define LOFF_MAG_6NA 0x00 //(0b00000000)
#define LOFF_MAG_24NA 0x04 //(0b00000100)
#define LOFF_MAG_6UA 0x08 //(0b00001000)
#define LOFF_MAG_24UA 0x0c //(0b00001100)
#define LOFF_FREQ_DC 0x00 //(0b00000000)
#define LOFF_FREQ_7p8HZ 0x01 //(0b00000001)
#define LOFF_FREQ_31p2HZ 0x02 //(0b00000010)
#define LOFF_FREQ_FS_4 0x03 //(0b00000011)
#define PCHAN (1)
#define NCHAN (2)
#define BOTHCHAN (3)
byte regData[24] = {0}; // array is used to mirror register data
volatile int boardStat = 0; //
/*
enum ads1299_command : uint8_t 表示定义的枚举类型 ads1299_command 的底层存储类型为 uint8_t,
即无符号 8 位整数类型。这意味着枚举常量的值将被存储为 8 位无符号整数,通常取值范围是 0 到 255。
*/
enum ads1299_command : uint8_t
{
//datasheet, p.40
ads1299_command_start = 0x08,
ads1299_command_stop = 0x0A,
ads1299_command_rdatac = 0x10,
ads1299_command_sdatac = 0x11,
ads1299_command_rreg = 0x20,
ads1299_command_wreg = 0x40
};
typedef struct ads1299_register_packet
{
uint8_t id;
uint8_t config1;
uint8_t config2;
uint8_t config3;
uint8_t loff;
uint8_t chnset[8];
uint8_t bias_sensp;
uint8_t bias_sensn;
uint8_t loff_sensp;
uint8_t loff_sensn;
uint8_t loff_flip;
uint8_t loff_statp;
uint8_t loff_statn;
uint8_t gpio;
uint8_t misc1;
uint8_t misc2;
uint8_t config4;
} __attribute__ ((packed)) ads1299_register_packet;
typedef struct ads1299_data_packet
{
uint32_t stat : 24; //24表示stat成员变量分配了24位的存储空间
uint8_t channel_data[24];
} __attribute__ ((packed)) ads1299_data_packet;
typedef struct openbci_data_packet
{
uint8_t header;
uint8_t sample_number;
uint8_t channel_data[24];
uint8_t auxiliary_data[6];
uint8_t footer;
} __attribute__ ((packed)) openbci_data_packet;
ads1299_register_packet ads1299_register_buffer = {};
ads1299_data_packet ads1299_data_buffer = {};
openbci_data_packet openbci_data_buffer[OPENBCI_DATA_BUFFER_SIZE] = {{}};//OPENBCI_DATA_BUFFER_SIZE equals 50
uint16_t openbci_data_buffer_head = 0;
uint16_t openbci_data_buffer_tail = 0;
uint8_t channel_setting_buffer[8] = {0};
uint8_t sample_counter = 0;
bool streaming_enabled = false;
uint8_t* tcp_transfer_buffer = NULL;
IPAddress local_ip(192, 168, 4, 1);
IPAddress network_gateway(192, 168, 4, 1);
IPAddress subnet_mask(255, 255, 255, 0);
WebServer web_server(80);
WiFiClient tcp_client;
size_t wifi_latency = 0;
//SPI communication method
byte xfer(byte _data)
{
byte inByte;
inByte = SPI.transfer(_data);
return inByte;
}
void csHigh(int SS)
{ // deselect SPI slave
switch (SS)
{
case BOARD_ADS:
digitalWrite(BOARD_ADS, HIGH);
break;
default:
break;
}
}
//SPI chip select method
void csLow(int SS)
{ // select an SPI slave to talk to
switch (SS)
{
case BOARD_ADS:
//SPI.setMode(DSPI_MODE1);
//SPI.setSpeed(4000000);
//SPI.beginTransaction(SPISettings(4000000, MSBFIRST, SPI_MODE1));
digitalWrite(BOARD_ADS, LOW);
break;
default:
break;
}
}
byte RREG(byte _address, int targetSS)
{ // reads ONE register at _address
byte opcode1 = _address + 0x20; // RREG expects 001rrrrr where rrrrr = _address
csLow(targetSS); // open SPI
xfer(opcode1); // opcode1
xfer(0x00); // opcode2
regData[_address] = xfer(0x00); // update mirror location with returned byte
csHigh(targetSS); // close SPI
return regData[_address]; // return requested register value
}
void WREG(byte _address, byte _value )
{
int target_SS=BOARD_ADS; // Write ONE register at _address
byte opcode1 = _address + 0x40; // WREG expects 010rrrrr where rrrrr = _address
csLow(target_SS); // open SPI
xfer(opcode1); // Send WREG command & address
xfer(0x00); // Send number of registers to read -1
xfer(_value); // Write the value to the register
csHigh(target_SS); // close SPI
regData[_address] = _value; // update the mirror array
}
void SDATAC(int targetSS)
{
csLow(targetSS);
xfer(_SDATAC);
csHigh(targetSS);
delayMicroseconds(10); //must wait at least 4 tCLK cycles after executing this command (Datasheet, pg. 37)
}
void RDATAC(int targetSS)
{
csLow(targetSS);
xfer(_RDATAC);
csHigh(targetSS);
delayMicroseconds(10); //must wait at least 4 tCLK cycle

好家伙VCC
- 粉丝: 2411
- 资源: 9138
最新资源
- 基于java的仓库管理系统设计与实现.docx
- 基于java的爱心公益网站设计与实现.docx
- 基于java的草莓基地管理系统设计与实现.docx
- 电子级聚苯乙烯外壳行业发展趋势:预计2031年年复合增长率(CAGR)为5.8%(2025-2031)
- 基于java的大学生选修选课系统设计与实现.docx
- 基于java的高校汉服租赁网站设计与实现.docx
- 基于java的电影订票网站设计与实现.docx
- 《imgplay》安卓版提供给大家进行动图的制作了,轻松的完成动图制作的app,能够进行gif图片转换,能够让照片动起来 使用方法简单,制作教程便捷,让每个用户都可以简单完成制作,有兴趣的来下载使用吧
- 基于java的高校科研管理系统设计与实现.docx
- 基于java的高校实习管理系统设计与实现.docx
- 基于java的高校竞赛管理系统设计与实现.docx
- 基于java的高校实习信息发布网站设计与实现.docx
- 基于java的高校物品捐赠系统设计与实现.docx
- 基于java的高校宣讲会管理系统设计与实现.docx
- 基于java的驾校预约学习系统设计与实现.docx
- 基于java的教师个人成果管理系统设计与实现.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


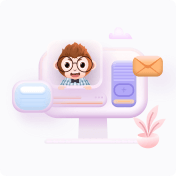