/* Scan Example
This example code is in the Public Domain (or CC0 licensed, at your option.)
Unless required by applicable law or agreed to in writing, this
software is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
CONDITIONS OF ANY KIND, either express or implied.
*/
/*
This example shows how to use the All Channel Scan or Fast Scan to connect
to a Wi-Fi network.
In the Fast Scan mode, the scan will stop as soon as the first network matching
the SSID is found. In this mode, an application can set threshold for the
authentication mode and the Signal strength. Networks that do not meet the
threshold requirements will be ignored.
In the All Channel Scan mode, the scan will end only after all the channels
are scanned, and connection will start with the best network. The networks
can be sorted based on Authentication Mode or Signal Strength. The priority
for the Authentication mode is: WPA2 > WPA > WEP > Open
*/
#include <stdio.h>
#include <stdint.h>
#include <stddef.h>
#include <string.h>
#include "soc/rtc_cntl_reg.h"
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "freertos/semphr.h"
#include "freertos/queue.h"
#include "freertos/event_groups.h"
#include "esp_wifi.h"
#include "esp_log.h"
#include "esp_system.h"
#include "esp_event_loop.h"
#include "nvs_flash.h"
#include "tcpip_adapter.h"
#include "lwip/api.h"
#include "esp_err.h"
#if CONFIG_WIFI_ALL_CHANNEL_SCAN
#define DEFAULT_SCAN_METHOD WIFI_ALL_CHANNEL_SCAN
#elif CONFIG_WIFI_FAST_SCAN
#define DEFAULT_SCAN_METHOD WIFI_FAST_SCAN
#else
#define DEFAULT_SCAN_METHOD WIFI_FAST_SCAN
#endif /*CONFIG_SCAN_METHOD*/
#if CONFIG_WIFI_CONNECT_AP_BY_SIGNAL
#define DEFAULT_SORT_METHOD WIFI_CONNECT_AP_BY_SIGNAL
#elif CONFIG_WIFI_CONNECT_AP_BY_SECURITY
#define DEFAULT_SORT_METHOD WIFI_CONNECT_AP_BY_SECURITY
#else
#define DEFAULT_SORT_METHOD WIFI_CONNECT_AP_BY_SIGNAL
#endif /*CONFIG_SORT_METHOD*/
#if CONFIG_FAST_SCAN_THRESHOLD
#define DEFAULT_RSSI CONFIG_FAST_SCAN_MINIMUM_SIGNAL
#if CONFIG_EXAMPLE_OPEN
#define DEFAULT_AUTHMODE WIFI_AUTH_OPEN
#elif CONFIG_EXAMPLE_WEP
#define DEFAULT_AUTHMODE WIFI_AUTH_WEP
#elif CONFIG_EXAMPLE_WPA
#define DEFAULT_AUTHMODE WIFI_AUTH_WPA_PSK
#elif CONFIG_EXAMPLE_WPA2
#define DEFAULT_AUTHMODE WIFI_AUTH_WPA2_PSK
#else
#define DEFAULT_AUTHMODE WIFI_AUTH_OPEN
#endif
#else
#define DEFAULT_RSSI -127
#define DEFAULT_AUTHMODE WIFI_AUTH_OPEN
#endif /*CONFIG_FAST_SCAN_THRESHOLD*/
void wifi_sniffer_cb(void *recv_buf, wifi_promiscuous_pkt_type_t type);
void TCP_Client(void *pvParameter);
static esp_err_t event_handler(void *ctx, system_event_t *event);
static void wifi_scan(void);
void app_main();
void transform();
typedef struct {
uint8_t header[4];
uint8_t dest_mac[6];
uint8_t source_mac[6];
uint8_t bssid[6];
uint8_t payload[0];
} sniffer_payload_t;
typedef struct station_info {
uint8_t bssid[6];
int8_t rssi;
uint8_t channel;
uint32_t timestamp;
struct station_info *next;
} station_info_t;
#define TCP_Client_RX_BUFSIZE 199
static const uint8_t esp_module_mac[32][3] = {
{0x54, 0x5A, 0xA6}, {0x24, 0x0A, 0xC4}, {0xD8, 0xA0, 0x1D}, {0xEC, 0xFA, 0xBC},
{0xA0, 0x20, 0xA6}, {0x90, 0x97, 0xD5}, {0x18, 0xFE, 0x34}, {0x60, 0x01, 0x94},
{0x2C, 0x3A, 0xE8}, {0xA4, 0x7B, 0x9D}, {0xDC, 0x4F, 0x22}, {0x5C, 0xCF, 0x7F},
{0xAC, 0xD0, 0x74}, {0x30, 0xAE, 0xA4}, {0x24, 0xB2, 0xDE}, {0x68, 0xC6, 0x3A},
};
const static int CONNECTED_BIT = BIT0;
int s_device_info_num = 0;
station_info_t *station_info = NULL;
station_info_t *g_station_list = NULL;
//char tcp_client_sendbuf[500]="";
static EventGroupHandle_t s_wifi_event_group;
/* The callback function of sniffer */
void wifi_sniffer_cb(void *recv_buf, wifi_promiscuous_pkt_type_t type)
{
wifi_promiscuous_pkt_t *sniffer = (wifi_promiscuous_pkt_t *)recv_buf;
sniffer_payload_t *sniffer_payload = (sniffer_payload_t *)sniffer->payload;
/* Check if the packet is Probo Request */
if (sniffer_payload->header[0] != 0x40) {
return;
}
if (!g_station_list) {
g_station_list = malloc(sizeof(station_info_t));
g_station_list->next = NULL;
}
/* Check if there is enough memoory to use */
if (esp_get_free_heap_size() < 60 * 1024) {
s_device_info_num = 0;
for (station_info = g_station_list->next; station_info; station_info = g_station_list->next) {
g_station_list->next = station_info->next;
free(station_info);
}
}
/* Filter out some useless packet */
for (int i = 0; i < 32; ++i) {
if (!memcmp(sniffer_payload->source_mac, esp_module_mac[i], 3)) {
return;
}
}
/* Traversing the chain table to check the presence of the device */
for (station_info = g_station_list->next; station_info; station_info = station_info->next) {
if (!memcmp(station_info->bssid, sniffer_payload->source_mac, sizeof(station_info->bssid))) {
return;
}
}
/* Add the device information to chain table */
if (!station_info) {
station_info = malloc(sizeof(station_info_t));
station_info->next = g_station_list->next;
g_station_list->next = station_info;
}
station_info->rssi = sniffer->rx_ctrl.rssi;
station_info->channel = sniffer->rx_ctrl.channel;
memcpy(station_info->bssid, sniffer_payload->source_mac, sizeof(station_info->bssid));
s_device_info_num++;
printf("\nCurrent device num = %d\n", s_device_info_num);
printf("MAC: 0x%02X.0x%02X.0x%02X.0x%02X.0x%02X.0x%02X, The rssi = %d\n", station_info->bssid[0], station_info->bssid[1], station_info->bssid[2], station_info->bssid[3], station_info->bssid[4], station_info->bssid[5], station_info->rssi);
}
void TCP_Client(void *pvParameter)
{
uint32_t date_len=0;
esp_err_t err,recv_err;
static u16_t server_port,local_port;
static ip_addr_t server_ipaddr,loca_ipaddr;
struct pbuf *q;
struct netconn *tcp_clientconn;
//char send[200] = "test,by";
char *tcp_client_sendbuf;
//char tcp_client_recvbuf[20];
//xEventGroupWaitBits(s_wifi_event_group,CONNECTED_BIT,false,true,protMAX_DELAY);
LWIP_UNUSED_ARG(pvParameter);
server_port=12345;
IP4_ADDR(&(server_ipaddr.u_addr.ip4),67,216,211,146);
while(1)
{
tcp_clientconn = netconn_new(NETCONN_TCP);
err = netconn_connect(tcp_clientconn,&server_ipaddr,server_port);
if( err!=ERR_OK )
{
printf("Connect error");
netconn_delete(tcp_clientconn);
}
else if(err == ERR_OK)
{
tcp_clientconn->recv_timeout=10;
netconn_getaddr(tcp_clientconn,&loca_ipaddr,&local_port,1);
printf("Connect server\r\n");
while(1)
{
vTaskDelay(15000/portTICK_PERIOD_MS);
//memcpy(tcp_client_sendbuf,"", 0);
if(s_device_info_num > 0)
{
tcp_client_sendbuf = (char *) malloc(50*s_device_info_num);
//memset(tcp_client_sendbuf,'\0',sizeof(tcp_client_sendbuf));
strcpy(tcp_client_sendbuf,"");
for(station_info = g_station_list->next; station_info; station_info = station_info->next)
{
sprintf(tcp_client_sendbuf,"%sMAC:0x%02X.0x%02X.0x%02X.0x%02X.0x%02X.0x%02X,rssi=%d\n",
tcp_client_sendbuf,station_info->bssid[0], station_info->bssid[1], station_info->bssid[2], station_info->bssid[3], station_info->bssid[4], station_info->bssid[5], station_info->rssi);
}
}else{
tcp_client_sendbuf = (char *) malloc(50);
strcpy(tcp_client_sendbuf,"MAC:0xFF.0xFF.0xFF.0xFF.0xFF.0xFF,rssi=0\n");
}
struct netbuf *recvbuf;
err = netconn_write(tcp_clientconn,tcp_client_sendbuf,strlen((char *)tcp_client_sendbuf),NETCONN_COPY);
//err = netconn_write(tcp_clientconn,send,strlen((char *)send),NETCONN_NOCOPY);
printf("write over,err = %d,tcp_client_sendbuf = %s,length = %d\n", err, tcp_client_sendbuf, strlen((char *)tcp_client_sendbuf));
if(err!= ERR_OK)
{
printf("Send error,err = %d\r\n",err);
f

好家伙VCC
- 粉丝: 2407
- 资源: 9139
最新资源
- 洞见研报江阴振宏重型锻造(锻件及粉末冶金制品制造商,振宏重工(江苏)股份有限公司)创投信息
- 大学生在线租房平台--论文pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 垃圾分类网站-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 大学生就业服务平台--论文pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 基于java的美食信息推荐系统的设计与实现pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 洞见研报科沃斯(家庭服务机器人研发与生产商,科沃斯机器人股份有限公司)创投信息
- 大学生创新创业项目管理系统--论文pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 大学生平时成绩量化管理系统pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 工资信息管理系统--论文pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 当代中国获奖的知名作家信息管理系统的设计与实现pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 房屋租赁管理系统boot--论文pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 果蔬作物疾病防治系统pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 爱心商城系统pf-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 商务安全邮箱邮件收发-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 洞见研报卢米蓝(新型OLED材料研发生产商,宁波卢米蓝新材料有限公司)创投信息
- 基于python后端开发框架
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


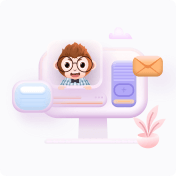