/*
FxSound
Copyright (C) 2023 FxSound LLC
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <http://www.gnu.org/licenses/>.
*/
/*
* FILE: hrdwr\comSftwr\comSftwr.c
* DATE: 9/7/97
* AUTHOR: Paul Titchener
* DESCRIPTION:
*
* These functions handle the direct reads and writes to the DSP card.
* This is the version for use with the software dsp dll.
*/
/* Standard includes */
#ifdef WIN32
#include <windows.h>
#include <winuser.h>
#endif
#include <time.h>
#include <stdlib.h>
#include <malloc.h>
#include <stdio.h>
#include <string.h>
#include "codedefs.h"
#include "pt_defs.h"
#include "platform.h"
#include "boardrv1.h"
/* For setting demo bypass mode */
//#include "product_type.h"
/*
#include "hrdwr.h"
#include "hutil.h"
*/
#include "u_comSftwr.h"
#include "comSftwr.h"
#include "c_dsps.h"
#include "demodef.h"
/* Make sure PT_DSP_BUILD is set */
#if !defined(PT_DSP_BUILD)
#error PT_DSP_BUILD Not Defined.
#endif
/* For writing parameters to DSP.
* Note that the hrdwrWriteParameterIfNotBusy uses a hard wait loop to
* complete the second write operation (the actual value).
*/
int COMSFTWR_DECL comSftwrWriteParam(PT_HANDLE *hp_comSftwr, long l_offset, long l_val)
{
float *flt_ptr;
struct comSftwrHdlType *cast_handle;
cast_handle = (struct comSftwrHdlType *)hp_comSftwr;
if (cast_handle == NULL)
return(NOT_OKAY);
flt_ptr = (float *)&l_val;
#ifdef COMSFTWR_MESSAGE_BOXES
if((l_offset < 0) || (l_offset > 256))
MessageBoxA(NULL, "Invalid l_offset in comSftwrWriteParam", NULL,
(MB_OK | MB_ICONERROR | MB_TASKMODAL ) );
if( dsp_params == NULL )
MessageBoxA(NULL, "dsp_params is NULL in comSftwrWriteParam", NULL,
(MB_OK | MB_ICONERROR | MB_TASKMODAL ) );
#endif
cast_handle->dsp_params[l_offset] = *flt_ptr;
/* Set the recue pending flag */
cast_handle->comSftwrReCuePending = 1;
return(OKAY);
}
/*
* FUNCTION: comSftwrProcessWaveBuffer()
* DESCRIPTION:
*
* Process the passed wave buffer, and send it back (no A/D or D/A).
* Currently only processes stereo to stereo or mono to mono signals.
*
*/
int COMSFTWR_DECL comSftwrProcessWaveBuffer(PT_HANDLE *hp_comSftwr, long *lp_data, long l_length,
int i_stereo_in_mode, int i_stereo_out_mode,
int i_buffer_type)
{
float *param_addr;
int index;
struct comSftwrHdlType *cast_handle;
cast_handle = (struct comSftwrHdlType *)hp_comSftwr;
if (cast_handle == NULL)
return(NOT_OKAY);
/* l_length comes in with the buffer size in samples */
/* Don't process the buffer if DSP is not available */
#ifdef COMSFTWR_CHECK_OUT_DSP
if( hutsyncCheckOutDsp( (unsigned short)i_processor_index) == 0 )
return(OKAY);
#endif
/* Set up pointer to correct parameter space */
param_addr = &(cast_handle->dsp_params[0]);
index = cast_handle->dsp_function_index;
#ifdef COMSFTWR_MESSAGE_BOXES
if( (index < 0) || (index > 62) )
MessageBoxA(NULL, "Invalid function index in comSftwrProcessWaveBuffer", NULL,
(MB_OK | MB_ICONERROR | MB_TASKMODAL ) );
if(lp_data == NULL)
MessageBoxA(NULL, "lpdata NULL in comSftwrProcessWaveBuffer", NULL,
(MB_OK | MB_ICONERROR | MB_TASKMODAL ) );
if(param_addr == NULL)
MessageBoxA(NULL, "param_addr NULL in comSftwrProcessWaveBuffer", NULL,
(MB_OK | MB_ICONERROR | MB_TASKMODAL ) );
if( (cast_handle->comSftDspProcessPtr[index]) == NULL)
MessageBoxA(NULL, "NULL function ptr in comSftwrProcessWaveBuffer", NULL,
(MB_OK | MB_ICONERROR | MB_TASKMODAL ) );
if( (l_length < 0) || (l_length > 256000) )
MessageBoxA(NULL, "Invalid function index in comSftwrProcessWaveBuffer", NULL,
(MB_OK | MB_ICONERROR | MB_TASKMODAL ) );
#endif
/* Only do DSPFX style muting if this is the DSPFX product */
#if (PT_DSP_BUILD == PT_DSP_DSPFX)
/* Note zeroing operation is dependent on input/output modes */
if( comSftwrDemoFlag[i_processor_index] &&
(sample_count[i_processor_index] > COMSFTWR_DEMO_SAMPLES_ALLOWED) )
{
dspsZeroOutput(lp_data, l_length,
param_addr,
dsp_memory[i_processor_index],
dsp_state[i_processor_index],
&(ComSftwrMeterData[i_processor_index]), i_buffer_type);
silence_count[i_processor_index] += l_length;
if( silence_count[i_processor_index] > COMSFTWR_DEMO_SILENCE_COUNT )
{
silence_count[i_processor_index] = 0;
sample_count[i_processor_index] = 0;
}
}
else
#endif
{
/*
#define COMSFTWR_AUTO_DEMO
#define COMSFTWR_AUTO_DEMO_SECS 7.5
*/
#ifdef COMSFTWR_AUTO_DEMO
{
/* On off toggling for demo */
static int count = 0;
static int on = 0;
long int tmp_buf[16384];
count += l_length;
if( count > 44100 * COMSFTWR_AUTO_DEMO_SECS )
{
count = 0;
if( on )
on = 0;
else
on = 1;
}
if( on )
{
(*cast_handle->comSftDspProcessPtr[index])(lp_data, l_length,
param_addr,
cast_handle->dsp_memory,
cast_handle->dsp_state,
&(cast_handle->ComSftwrMeterData), i_buffer_type);
}
else
{
int k;
int len;
len = l_length;
if(i_stereo_in_mode)
len *= 2;
for(k=0; k<=len; k++)
tmp_buf[k] = lp_data[k];
(*cast_handle->comSftDspProcessPtr[index])(tmp_buf, l_length,
param_addr,
cast_handle->dsp_memory,
cast_handle->dsp_state,
&(cast_handle->ComSftwrMeterData), i_buffer_type);
}
}
#else
(*cast_handle->comSftDspProcessPtr[index])(lp_data, l_length,
param_addr,
cast_handle->dsp_memory,
cast_handle->dsp_state,
&(cast_handle->ComSftwrMeterData), i_buffer_type);
#endif
}
/* Only do DSPFX style metering correction if this is the DSPFX product */
#if (PT_DSP_BUILD == PT_DSP_DSPFX)
/* Correct meter values for averaging. Values are coming back as floats,
* we need to convert them.
*/
{
float factor = (float)PC_24BIT_FLOAT_PLUS_CLIP/(float)l_length;
cast_handle->ComSftwrMeterData.left_in =
(long)( *(float *)&(ComSftwrMeterData[i_processor_index].left_in) * factor);
cast_handle->ComSftwrMeterData.right_in =
(long)( *(float *)&(ComSftwrMeterData.right_in) * factor);
cast_handle->ComSftwrMeterData[i_processor_index].left_out =
(long)( *(float *)&(ComSftwrMeterData.left_out) * factor);
cast_handle->ComSftwrMeterData.right_out =
(long)( *(float *)&(ComSftwrMeterData.right_out) * factor);
}
#endif
/* Check DSP back in */
#ifdef COMSFTWR_CHECK_OUT_DSP
hutsyncCheckInDsp( (unsigned short)i_processor_index );
#endif
cast_handle->sample_count += l_length;
return(OKAY);
}
/*
* FUNCTION: comSftwrProcessActiveBuffer()
* DESCRIPTION:
*
* Process the passed Active Movie buffer, and send it back (no A/D or D/A).
*
*/
int COMSFTWR_DECL comSftwrProcessActiveBuffer(PT_HANDLE *hp_comSftwr, short *sp_data, long l_length,
int i_stereo_in_mode, int i_stereo_out_mode,
int i_buffer_type)
{
struct comSftwrHdlType *cast_handle;
cast_handle = (struct comSftwrHdlType *)hp_comSftwr;
if (cast_handle == NULL)
return(NOT_OKAY);
/* l_length comes in with the buffer size in samples */
/* For now, call original saw style processing */
if( comSftwrProcessWaveBuffer(hp_comSftwr, (long *)sp_data, l_length,
i_stereo_in_mode, i_stereo_out_mode, i_buffer_type) != OKAY)
return(NOT_OKAY);
return(OKAY);
}
/* F

好家伙VCC
- 粉丝: 2471
- 资源: 9138
最新资源
- 绩效考核管理制度.doc
- 企业绩效考核制度及方案(实例).doc
- 长虹集团绩效管理手册.doc
- 绩效考核制度.doc
- 美的干部绩效考核办法.doc
- 生产型企业绩效考核方案.doc
- 中国联通:绩效管理操作手册.doc
- 公司绩效考核全套流程表格.docx
- 中通关键岗位薪酬设计方案.doc
- “探讨功率因素调节中MPPT和SVPWM技术在三相光伏并网逆变器仿真模型中的应用:精确谐波畸变控制与性能优化”,500kW三相光伏并网逆变器的仿真模型: 1.光伏PV, DC DC采用MPPT最大功率
- buildnumber-maven-plugin-javadoc-1.2-7.el7.x64-86.rpm.tar.gz
- DSP2837系列串口升级方案:基于VS2013的双核与单核升级解决方案及源代码分享,DSP28377D串口升级方案 串口双核升级,上位机采用vs2013开发 稍微修改可支持2837x系列的单、双核
- bwidget-1.9.0-6.el7.x64-86.rpm.tar.gz
- 蓄电池与超级电容混合储能系统的功率分配及SOC管理策略-基于Matlab Simulink仿真模型探究,蓄电池与超级电容混合储能并网matlab simulink仿真模型 (1)混合储能采用低通滤
- byacc-1.9.20130304-3.el7.x64-86.rpm.tar.gz
- 1737485585760.png
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


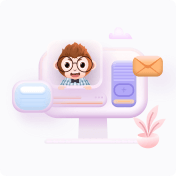