# Face Recognition
_You can also read a translated version of this file [in Chinese 简体中文版](https://github.com/ageitgey/face_recognition/blob/master/README_Simplified_Chinese.md) or [in Korean 한국어](https://github.com/ageitgey/face_recognition/blob/master/README_Korean.md) or [in Japanese 日本語](https://github.com/m-i-k-i/face_recognition/blob/master/README_Japanese.md)._
Recognize and manipulate faces from Python or from the command line with
the world's simplest face recognition library.
Built using [dlib](http://dlib.net/)'s state-of-the-art face recognition
built with deep learning. The model has an accuracy of 99.38% on the
[Labeled Faces in the Wild](http://vis-www.cs.umass.edu/lfw/) benchmark.
This also provides a simple `face_recognition` command line tool that lets
you do face recognition on a folder of images from the command line!
[](https://pypi.python.org/pypi/face_recognition)
[](https://github.com/ageitgey/face_recognition/actions?query=workflow%3ACI)
[](http://face-recognition.readthedocs.io/en/latest/?badge=latest)
## Features
#### Find faces in pictures
Find all the faces that appear in a picture:

```python
import face_recognition
image = face_recognition.load_image_file("your_file.jpg")
face_locations = face_recognition.face_locations(image)
```
#### Find and manipulate facial features in pictures
Get the locations and outlines of each person's eyes, nose, mouth and chin.

```python
import face_recognition
image = face_recognition.load_image_file("your_file.jpg")
face_landmarks_list = face_recognition.face_landmarks(image)
```
Finding facial features is super useful for lots of important stuff. But you can also use it for really stupid stuff
like applying [digital make-up](https://github.com/ageitgey/face_recognition/blob/master/examples/digital_makeup.py) (think 'Meitu'):

#### Identify faces in pictures
Recognize who appears in each photo.

```python
import face_recognition
known_image = face_recognition.load_image_file("biden.jpg")
unknown_image = face_recognition.load_image_file("unknown.jpg")
biden_encoding = face_recognition.face_encodings(known_image)[0]
unknown_encoding = face_recognition.face_encodings(unknown_image)[0]
results = face_recognition.compare_faces([biden_encoding], unknown_encoding)
```
You can even use this library with other Python libraries to do real-time face recognition:

See [this example](https://github.com/ageitgey/face_recognition/blob/master/examples/facerec_from_webcam_faster.py) for the code.
## Online Demos
User-contributed shared Jupyter notebook demo (not officially supported): [](https://beta.deepnote.org/launch?template=face_recognition)
## Installation
### Requirements
* Python 3.3+ or Python 2.7
* macOS or Linux (Windows not officially supported, but might work)
### Installation Options:
#### Installing on Mac or Linux
First, make sure you have dlib already installed with Python bindings:
* [How to install dlib from source on macOS or Ubuntu](https://gist.github.com/ageitgey/629d75c1baac34dfa5ca2a1928a7aeaf)
Then, make sure you have cmake installed:
```brew install cmake```
Finally, install this module from pypi using `pip3` (or `pip2` for Python 2):
```bash
pip3 install face_recognition
```
Alternatively, you can try this library with [Docker](https://www.docker.com/), see [this section](#deployment).
If you are having trouble with installation, you can also try out a
[pre-configured VM](https://medium.com/@ageitgey/try-deep-learning-in-python-now-with-a-fully-pre-configured-vm-1d97d4c3e9b).
#### Installing on an Nvidia Jetson Nano board
* [Jetson Nano installation instructions](https://medium.com/@ageitgey/build-a-hardware-based-face-recognition-system-for-150-with-the-nvidia-jetson-nano-and-python-a25cb8c891fd)
* Please follow the instructions in the article carefully. There is current a bug in the CUDA libraries on the Jetson Nano that will cause this library to fail silently if you don't follow the instructions in the article to comment out a line in dlib and recompile it.
#### Installing on Raspberry Pi 2+
* [Raspberry Pi 2+ installation instructions](https://gist.github.com/ageitgey/1ac8dbe8572f3f533df6269dab35df65)
#### Installing on FreeBSD
```bash
pkg install graphics/py-face_recognition
```
#### Installing on Windows
While Windows isn't officially supported, helpful users have posted instructions on how to install this library:
* [@masoudr's Windows 10 installation guide (dlib + face_recognition)](https://github.com/ageitgey/face_recognition/issues/175#issue-257710508)
#### Installing a pre-configured Virtual Machine image
* [Download the pre-configured VM image](https://medium.com/@ageitgey/try-deep-learning-in-python-now-with-a-fully-pre-configured-vm-1d97d4c3e9b) (for VMware Player or VirtualBox).
## Usage
### Command-Line Interface
When you install `face_recognition`, you get two simple command-line
programs:
* `face_recognition` - Recognize faces in a photograph or folder full for
photographs.
* `face_detection` - Find faces in a photograph or folder full for photographs.
#### `face_recognition` command line tool
The `face_recognition` command lets you recognize faces in a photograph or
folder full for photographs.
First, you need to provide a folder with one picture of each person you
already know. There should be one image file for each person with the
files named according to who is in the picture:

Next, you need a second folder with the files you want to identify:

Then in you simply run the command `face_recognition`, passing in
the folder of known people and the folder (or single image) with unknown
people and it tells you who is in each image:
```bash
$ face_recognition ./pictures_of_people_i_know/ ./unknown_pictures/
/unknown_pictures/unknown.jpg,Barack Obama
/face_recognition_test/unknown_pictures/unknown.jpg,unknown_person
```
There's one line in the output for each face. The data is comma-separated
with the filename and the name of the person found.
An `unknown_person` is a face in the image that didn't match anyone in
your folder of known people.
#### `face_detection` command line tool
The `face_detection` command lets you find the location (pixel coordinatates)
of any faces in an image.
Just run the command `face_detection`, passing in a folder of images
to check (or a single image):
```bash
$ face_detection ./folder_with_pictures/
examples/image1.jpg,65,215,169,112
examples/image2.jpg,62,394,211,244
examples/image2.jpg,95,941,244,792
```
It prints one line for each face that was detected. The coordinates
reported are the top, right, bottom and left coordinates of the face (in pixels).
##### Adjusting Tolerance / Sensitivity
If you are getting multiple matches for the same person, it might be that
the people in your photos look very similar and a lower tolerance value
is needed

好家伙VCC
- 粉丝: 2305
- 资源: 9142
最新资源
- 基于 AT89C51 的电梯控制系统嵌入式系统实验详细文档+全部资料+高分项目+源码.zip
- 本科毕设-基于嵌入式arm的人脸识别智能门禁,包括代码、电路图,具体流程详细文档+全部资料+高分项目+源码.zip
- 毕设-基于WIFI车间设备监测与控制系统的研究)的主程序,采用Qt框架编写,以嵌入式ARM Linux系统作为软件运行平台详细文档+全部资料+高分项目+源码.zip
- 基于 react + koa, 开箱即用的 Material Design 风格博客系统..详细文档+全部资料+高分项目+源码.zip
- Project2.zip
- 用c++语言实现的各种算法源代码.zip
- 基于 u8g2 的单色 OLED 菜单 UI 框架。MiaoUI使用 C 语言实现,,适用于具有小型OLED屏幕的嵌入式设备。详细文档+全部资料+高分项目+源码
- 基于ARM的嵌入式小系统,在系统挂掉之后,提取现场的dump信息详细文档+全部资料+高分项目+源码.zip
- 基于Android基于WebView的嵌入式Youtube视频播放器,可识别多种Youtube分享视频的url详细文档+全部资料+高分项目+源码.zip
- 基于ARM-Linux的嵌入式视觉移动追踪系统,并通过Android APP进行无线控制详细文档+全部资料+高分项目+源码.zip
- 基于C++11,协作式调度物联网嵌入式操作系统详细文档+全部资料+高分项目+源码.zip
- 基于ARM架构Cortex-A8的IC卡嵌入式刷卡考勤系统详细文档+全部资料+高分项目+源码.zip
- 基于cc2530的嵌入式详细文档+全部资料+高分项目+源码.zip
- 基于ChatGPT的智能音箱嵌入式课程设计详细文档+全部资料+高分项目+源码.zip
- 基于Cortex-M内核的嵌入式操作系统,针对新手设计,简单易懂详细文档+全部资料+高分项目+源码.zip
- 基于contiki与ucGUI的嵌入式微操作系统例程详细文档+全部资料+高分项目+源码.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


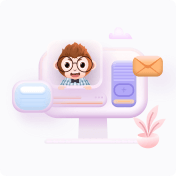