/**
* 该类是“World-of-Zuul”应用程序的主类。
* 《World of Zuul》是一款简单的文本冒险游戏。用户可以在一些房间组成的迷宫中探险。
* 你们可以通过扩展该游戏的功能使它更有趣!.
*
* 如果想开始执行这个游戏,用户需要创建Game类的一个实例并调用“play”方法。
*
* Game类的实例将创建并初始化所有其他类:它创建所有房间,并将它们连接成迷宫;它创建解析器
* 接收用户输入,并将用户输入转换成命令后开始运行游戏。
*
* @author Michael Kölling and David J. Barnes
* @version 1.0
*/
package cn.edu.whut.sept.zuul;
import java.util.*;
public class Game
{
private Parser parser;
Stack stack=new Stack(); //储存玩家的移动过程
Stack roomStack=new Stack(); //储存玩家被传送前的位置
private Room currentRoom; //玩家当前所处位置
ArrayList<Room> rooms = new ArrayList<>(); //存储所有房间对象
Room outside = new Room("outside the main entrance of the university");
/**
* 创建游戏并初始化内部数据和解析器.
*/
public Game()
{
createRooms();
parser = new Parser();
}
/**
* 创建所有房间对象并连接其出口用以构建迷宫.
*/
private void createRooms()
{
Room theater, pub, lab;
TransporterRoom office;
// create the rooms
theater = new Room("in a lecture theater");
pub = new Room("in the campus pub");
lab = new Room("in a computing lab");
office = new TransporterRoom("in the computing admin office");
// initialise room exits
outside.setExit("east", theater);
outside.setExit("south", lab);
outside.setExit("west", pub);
theater.setExit("west", outside);
pub.setExit("east", outside);
lab.setExit("north", outside);
lab.setExit("east", office);
office.setExit("west", lab);
currentRoom = outside; // 从outside开始游戏
rooms.add(outside);
rooms.add(theater);
rooms.add(pub);
rooms.add(lab);
}
/**
* 游戏主控循环,直到用户输入退出命令后结束整个程序.
*/
public void play()
{
printWelcome();
// Enter the main command loop. Here we repeatedly read commands and
// execute them until the game is over.
boolean finished = false;
while (! finished) {
Command command = parser.getCommand();
finished = processCommand(command);
}
System.out.println("Thank you for playing. Good bye.");
}
/**
* 向用户输出欢迎信息.
*/
private void printWelcome()
{
System.out.println();
System.out.println("Welcome to the World of Zuul!");
System.out.println("World of Zuul is a new, incredibly boring adventure game.");
System.out.println("Type 'help' if you need help.");
System.out.println();
System.out.println(currentRoom.getLongDescription());
}
/**
* 执行用户输入的游戏指令.
* @param command 待处理的游戏指令,由解析器从用户输入内容生成.
* @return 如果执行的是游戏结束指令,则返回true,否则返回false.
*/
private boolean processCommand(Command command)
{
boolean wantToQuit = false;
if(command.isUnknown()) {
System.out.println("I don't know what you mean...");
return false;
}
String commandWord = command.getCommandWord();
String words[] = parser.getCommandWords();
int index = 0;
for(; index<words.length; index++)
if (commandWord.equals(words[index]))
break;
switch(index) {
case 0: goRoom(command); break;
case 1: wantToQuit = quit(command); break;
case 2: printHelp(); break;
case 3: backRoom(); break;
}
/*
if (commandWord.equals("help")) {
printHelp();
}
else if (commandWord.equals("go")) {
goRoom(command);
}
else if (commandWord.equals("quit")) {
wantToQuit = quit(command);
}
*/
// else command not recognised.
return wantToQuit;
}
// implementations of user commands:
/**
* 执行help指令,在终端打印游戏帮助信息.
* 此处会输出游戏中用户可以输入的命令列表
*/
private void printHelp()
{
System.out.println("You are lost. You are alone. You wander");
System.out.println("around at the university.");
System.out.println();
System.out.println("Your command words are:");
parser.showCommands();
}
/**
*执行go指令,向房间的指定方向出口移动,如果该出口连接了另一个房间,则会进入该房间,
* 否则打印输出错误提示信息.
* 保存玩家的移动路径
* 若进入有传送门的房间则传送到任意房间
*/
private void goRoom(Command command)
{
if(!command.hasSecondWord()) {
// if there is no second word, we don't know where to go...
System.out.println("Go where?");
return;
}
String direction = command.getSecondWord();
stack.push(direction);
// Try to leave current room.
Room nextRoom = currentRoom.getExit(direction, rooms);
if (nextRoom == null) {
System.out.println("There is no door!");
}
else {
if(currentRoom.getExit(direction, rooms) instanceof TransporterRoom){
roomStack.push(currentRoom);
stack.push("tran");
nextRoom = currentRoom.getExit(direction, rooms);
currentRoom = nextRoom.getExit(direction, rooms);
System.out.println(currentRoom.getLongDescription());
}
else{
currentRoom = nextRoom;
System.out.println(currentRoom.getLongDescription());
}
}
}
/* 执行back指令,若栈为空则说明玩家已经回到起点
* 如果弹栈元素为方位指令,则玩家反向移动以退回上一个房间
* 如果弹栈元素为tran,则将房间栈弹出一个元素,即玩家被传送之前的位置
*/
private void backRoom()
{
if(stack.empty())
System.out.println("You have been in the staring point.\nExits: east south west");
else{
String direction = (String)stack.pop();
if(direction.equals("west"))
direction = "east";
if(direction.equals("east"))
direction = "west";
if(direction.equals("south"))
direction = "north";
if(direction.equals("north"))
direction = "south";
if(direction.equals("tran")) {
currentRoom = (Room)roomStack.pop();
stack.pop();
}
else {
Room nextRoom = currentRoom.getExit(direction, rooms);
currentRoom = nextRoom;
}
if (currentRoom == null)
currentRoom = outside;
System.out.println(currentRoom.getLongDescription());
}
}
/**
* 执行Quit指令,用户退出游戏。如果用户在命令中输入了其他参数,则进一步询问用户是否真的退出.
* @return 如果游戏需要退出则返回true,否则返回false.
*/
private boolean quit(Command command)
{
if(command.hasSe
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
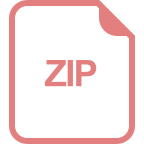
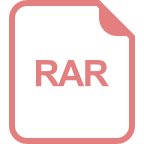
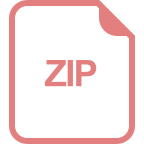
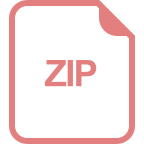
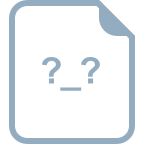
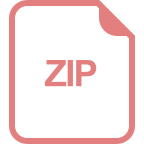
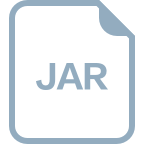
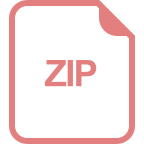
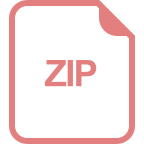
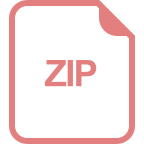
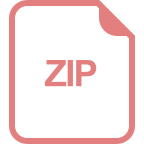
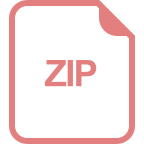
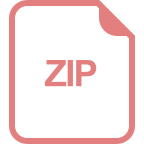
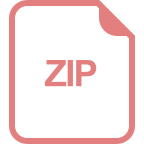
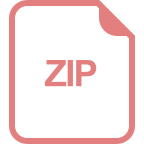
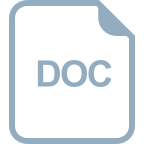
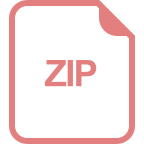
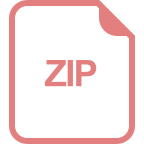
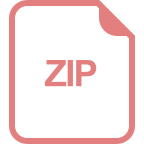
收起资源包目录

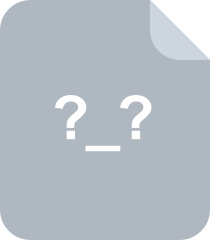
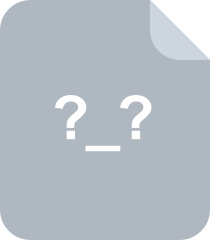
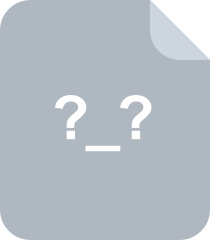
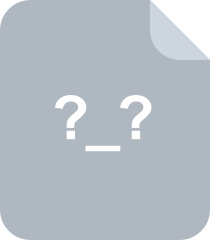
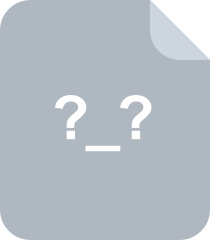
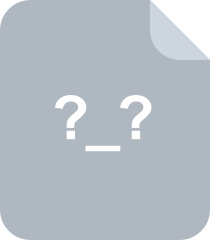
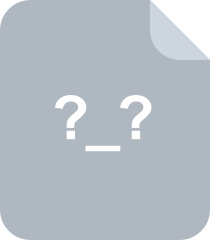
共 7 条
- 1
资源评论
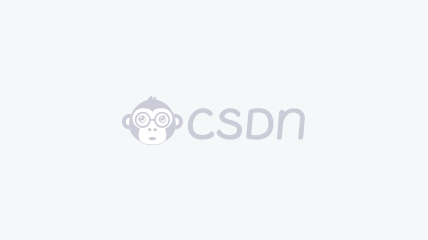

谛凌
- 粉丝: 3w+
- 资源: 75
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

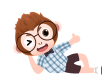
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


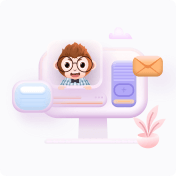
安全验证
文档复制为VIP权益,开通VIP直接复制
