<!--
BEFORE EDITING THIS README
Our README.md is auto-generated by combining pages in website/docs and website/readme-sources
If you are sending a pull request to improve documentation, submit your changes
in the source markdown files and we will generate the README from there.
You can build the readme with this command:
cd website && yarn build-readme
-->
# [](https://typestrong.org/ts-node)
[](https://npmjs.org/package/ts-node)
[](https://npmjs.org/package/ts-node)
[](https://github.com/TypeStrong/ts-node/actions?query=workflow%3A%22Continuous+Integration%22)
[](https://codecov.io/gh/TypeStrong/ts-node)
> TypeScript execution and REPL for node.js, with source map and native ESM support.
The latest documentation can also be found on our website: <https://typestrong.org/ts-node>
# Table of Contents
* [Overview](#overview)
* [Features](#features)
* [Installation](#installation)
* [Usage](#usage)
* [Command Line](#command-line)
* [Shebang](#shebang)
* [node flags and other tools](#node-flags-and-other-tools)
* [Programmatic](#programmatic)
* [Configuration](#configuration)
* [CLI flags](#cli-flags)
* [Via tsconfig.json (recommended)](#via-tsconfigjson-recommended)
* [@tsconfig/bases](#tsconfigbases)
* [Default config](#default-config)
* [`node` flags](#node-flags)
* [Options](#options)
* [CLI Options](#cli-options)
* [help](#help)
* [version](#version)
* [eval](#eval)
* [print](#print)
* [interactive](#interactive)
* [esm](#esm)
* [TSConfig Options](#tsconfig-options)
* [project](#project)
* [skipProject](#skipproject)
* [cwdMode](#cwdmode)
* [compilerOptions](#compileroptions)
* [showConfig](#showconfig)
* [Typechecking](#typechecking)
* [transpileOnly](#transpileonly)
* [typeCheck](#typecheck)
* [compilerHost](#compilerhost)
* [files](#files)
* [ignoreDiagnostics](#ignorediagnostics)
* [Transpilation Options](#transpilation-options)
* [ignore](#ignore)
* [skipIgnore](#skipignore)
* [compiler](#compiler)
* [swc](#swc)
* [transpiler](#transpiler)
* [preferTsExts](#prefertsexts)
* [Diagnostic Options](#diagnostic-options)
* [logError](#logerror)
* [pretty](#pretty)
* [TS_NODE_DEBUG](#ts_node_debug)
* [Advanced Options](#advanced-options)
* [require](#require)
* [cwd](#cwd)
* [emit](#emit)
* [scope](#scope)
* [scopeDir](#scopedir)
* [moduleTypes](#moduletypes)
* [TS_NODE_HISTORY](#ts_node_history)
* [noExperimentalReplAwait](#noexperimentalreplawait)
* [experimentalResolver](#experimentalresolver)
* [experimentalSpecifierResolution](#experimentalspecifierresolution)
* [API Options](#api-options)
* [SWC](#swc-1)
* [CommonJS vs native ECMAScript modules](#commonjs-vs-native-ecmascript-modules)
* [CommonJS](#commonjs)
* [Native ECMAScript modules](#native-ecmascript-modules)
* [Troubleshooting](#troubleshooting)
* [Configuration](#configuration-1)
* [Common errors](#common-errors)
* [`TSError`](#tserror)
* [`SyntaxError`](#syntaxerror)
* [Unsupported JavaScript syntax](#unsupported-javascript-syntax)
* [`ERR_REQUIRE_ESM`](#err_require_esm)
* [`ERR_UNKNOWN_FILE_EXTENSION`](#err_unknown_file_extension)
* [Missing Types](#missing-types)
* [npx, yarn dlx, and node_modules](#npx-yarn-dlx-and-node_modules)
* [Performance](#performance)
* [Skip typechecking](#skip-typechecking)
* [With typechecking](#with-typechecking)
* [Advanced](#advanced)
* [How it works](#how-it-works)
* [Ignored files](#ignored-files)
* [File extensions](#file-extensions)
* [Skipping `node_modules`](#skipping-node_modules)
* [Skipping pre-compiled TypeScript](#skipping-pre-compiled-typescript)
* [Scope by directory](#scope-by-directory)
* [Ignore by regexp](#ignore-by-regexp)
* [paths and baseUrl
](#paths-and-baseurl)
* [Why is this not built-in to ts-node?](#why-is-this-not-built-in-to-ts-node)
* [Third-party compilers](#third-party-compilers)
* [Transpilers](#transpilers)
* [Third-party plugins](#third-party-plugins)
* [Write your own plugin](#write-your-own-plugin)
* [Module type overrides](#module-type-overrides)
* [Caveats](#caveats)
* [API](#api)
* [Recipes](#recipes)
* [Watching and restarting](#watching-and-restarting)
* [AVA](#ava)
* [CommonJS](#commonjs-1)
* [Native ECMAScript modules](#native-ecmascript-modules-1)
* [Gulp](#gulp)
* [IntelliJ and Webstorm](#intellij-and-webstorm)
* [Mocha](#mocha)
* [Mocha 7 and newer](#mocha-7-and-newer)
* [Mocha <=6](#mocha-6)
* [Tape](#tape)
* [Visual Studio Code](#visual-studio-code)
* [Other](#other)
* [License](#license)
# Overview
ts-node is a TypeScript execution engine and REPL for Node.js.
It JIT transforms TypeScript into JavaScript, enabling you to directly execute TypeScript on Node.js without precompiling.
This is accomplished by hooking node's module loading APIs, enabling it to be used seamlessly alongside other Node.js
tools and libraries.
## Features
* Automatic sourcemaps in stack traces
* Automatic `tsconfig.json` parsing
* Automatic defaults to match your node version
* Typechecking (optional)
* REPL
* Write standalone scripts
* Native ESM loader
* Use third-party transpilers
* Use custom transformers
* Integrate with test runners, debuggers, and CLI tools
* Compatible with pre-compilation for production

# Installation
```shell
# Locally in your project.
npm install -D typescript
npm install -D ts-node
# Or globally with TypeScript.
npm install -g typescript
npm install -g ts-node
# Depending on configuration, you may also need these
npm install -D tslib @types/node
```
**Tip:** Installing modules locally allows you to control and share the versions through `package.json`. ts-node will always resolve the compiler from `cwd` before checking relative to its own installation.
# Usage
## Command Line
```shell
# Execute a script as `node` + `tsc`.
ts-node script.ts
# Starts a TypeScript REPL.
ts-node
# Execute code with TypeScript.
ts-node -e 'console.log("Hello, world!")'
# Execute, and print, code with TypeScript.
ts-node -p -e '"Hello, world!"'
# Pipe scripts to execute with TypeScript.
echo 'console.log("Hello, world!")' | ts-node
# Equivalent to ts-node --transpileOnly
ts-node-transpile-only script.ts
# Equivalent to ts-node --cwdMode
ts-node-cwd script.ts
# Equivalent to ts-node --esm
ts-node-esm script.ts
```
## Shebang
To write scripts with maximum portability, [specify options in your `tsconfig.json`](#via-tsconfigjson-recommended) and omit them from the shebang.
```typescript twoslash
#!/usr/bin/env ts-node
// ts-node options are read from tsconfig.json
console.log("Hello, world!")
```
Including options within the shebang requires the [`env -S` flag](https://manpages.debian.org/bullseye/coreutils/env.1.en.html#S), which is available on recent versions of `env`. ([compatibility](https://github.com/TypeStrong/ts-node/pull/1448#issuecomment-91389576
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
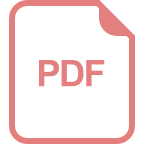
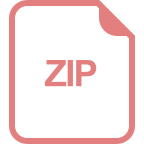
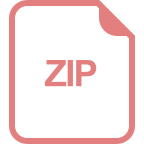
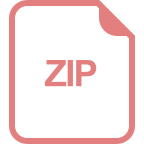
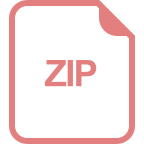
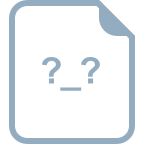
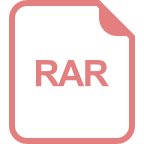
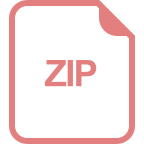
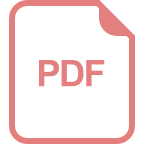
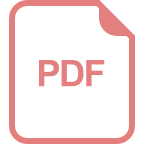
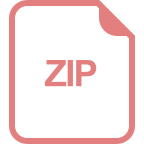
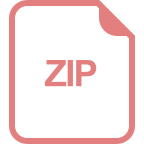
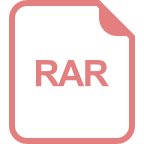
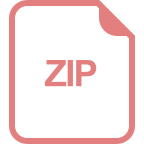
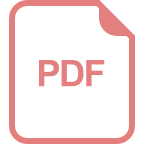
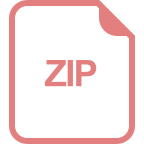
收起资源包目录

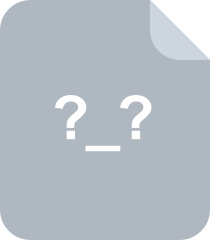
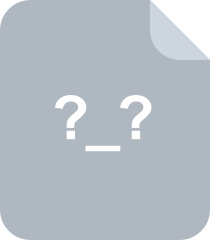
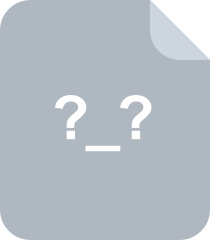
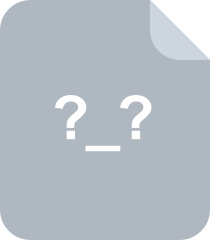
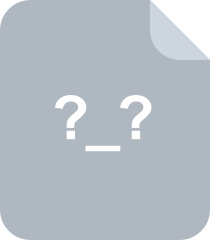
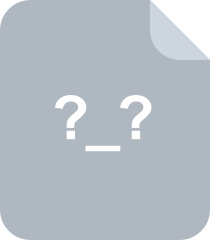
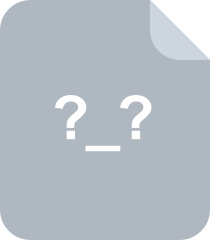
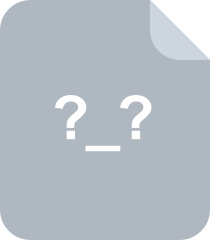
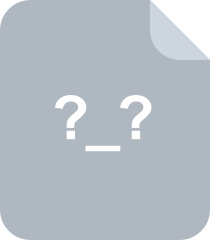
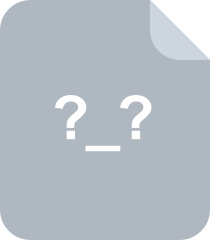
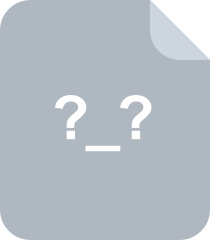
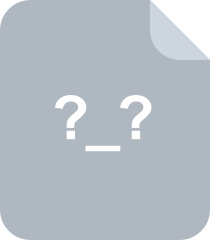
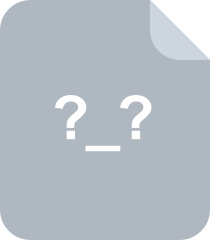
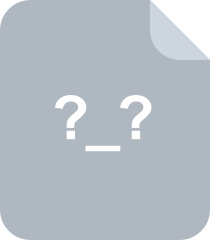
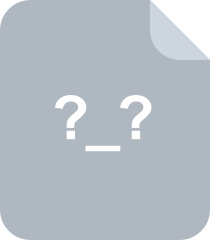
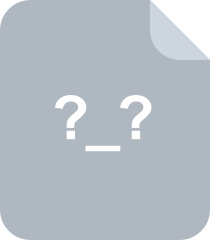
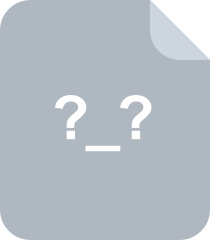
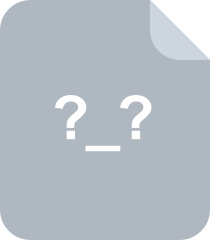
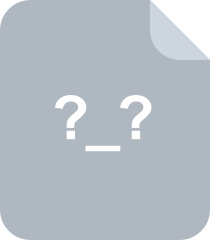
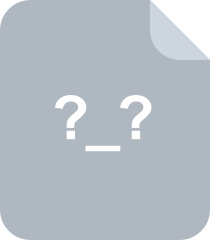
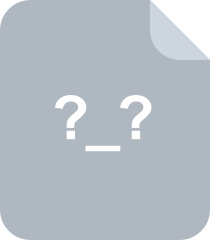
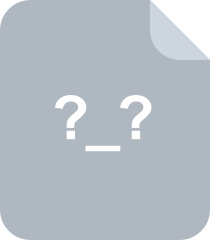
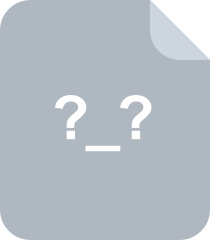
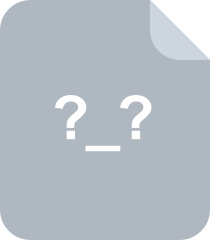
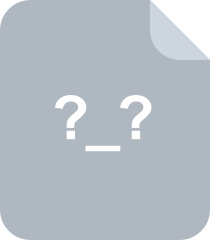
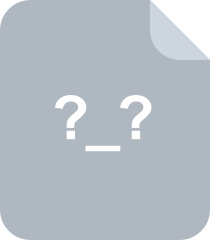
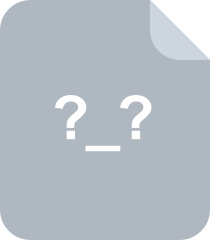
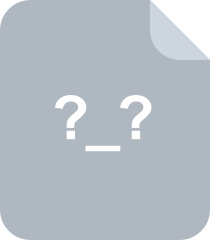
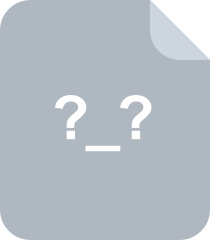
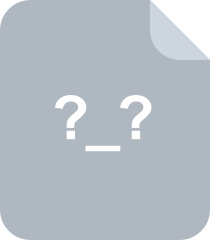
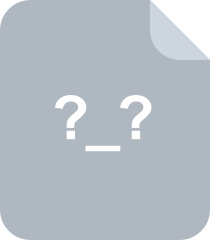
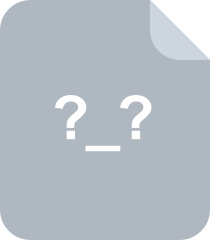
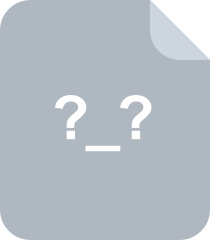
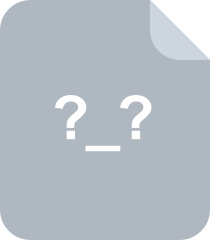
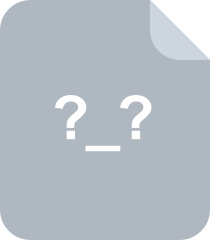
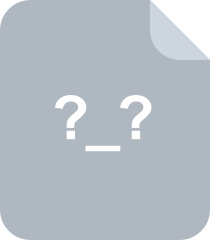
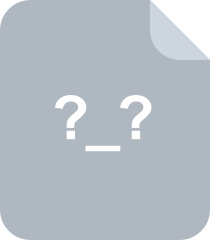
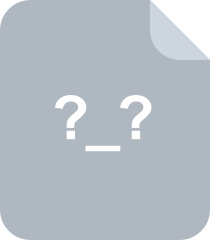
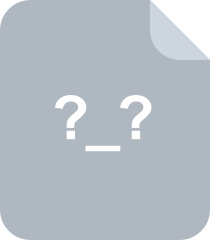
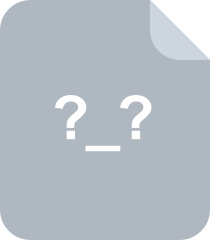
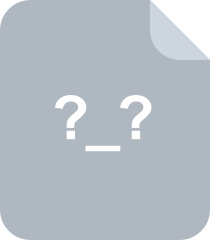
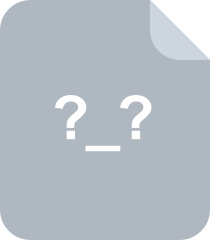
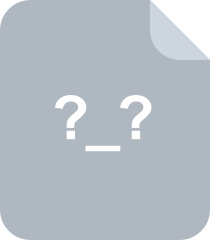
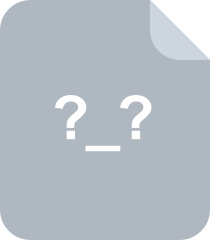
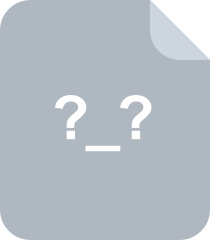
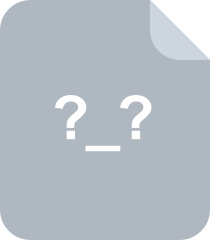
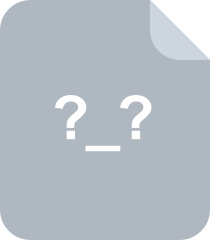
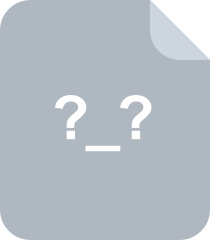
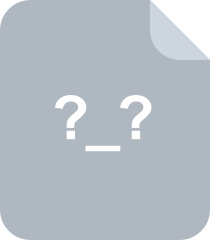
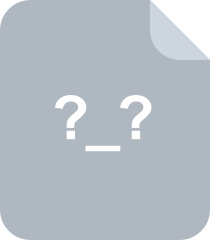
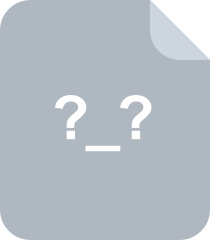
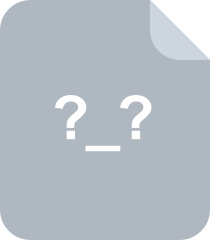
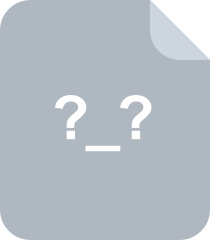
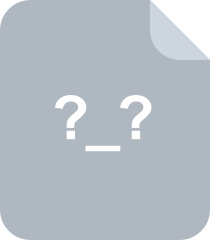
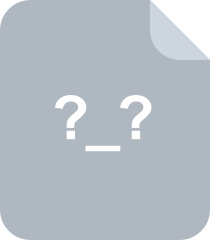
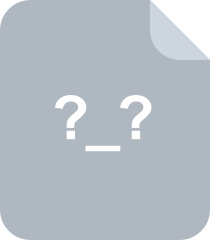
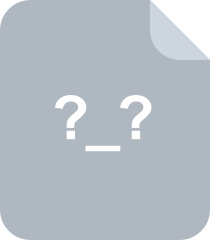
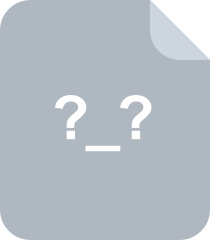
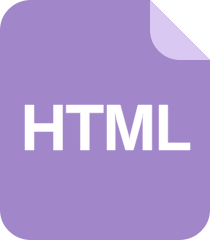
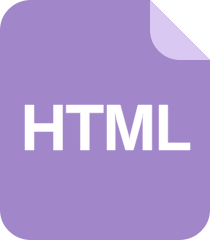
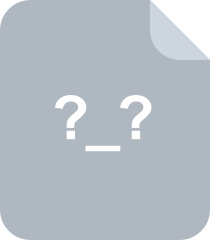
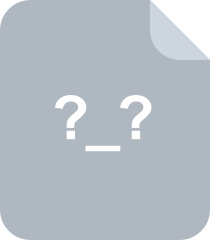
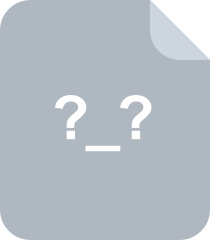
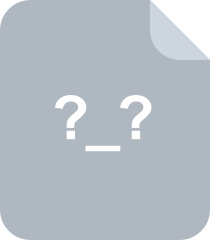
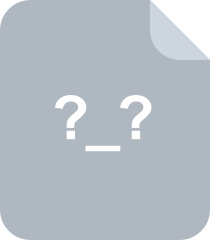
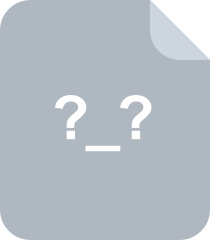
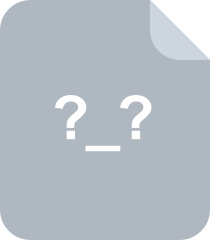
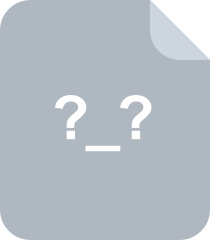
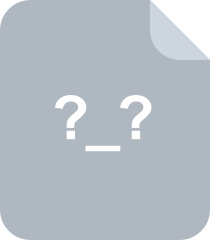
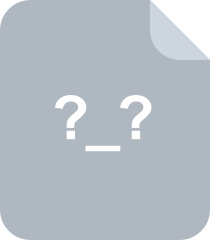
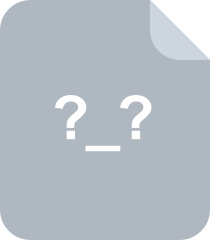
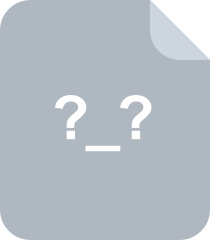
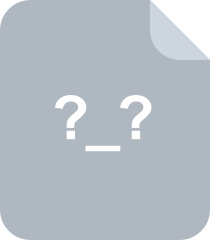
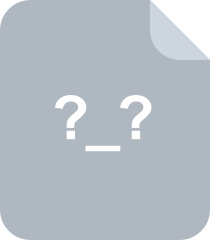
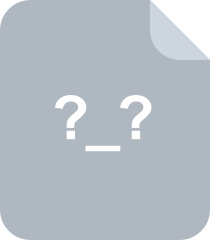
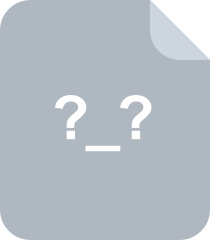
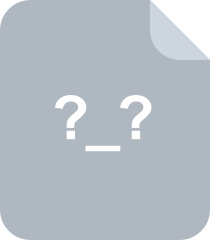
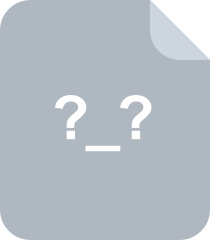
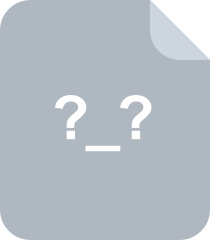
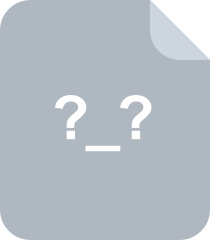
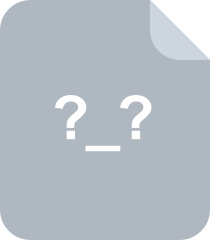
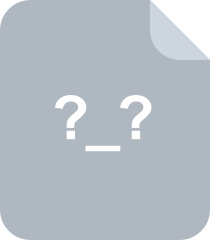
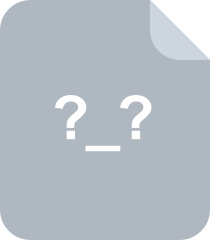
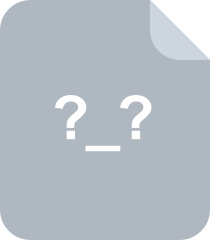
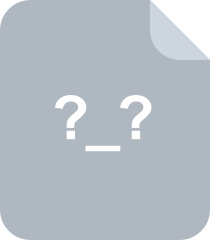
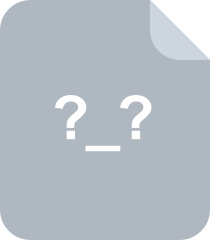
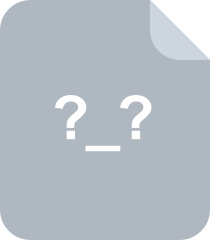
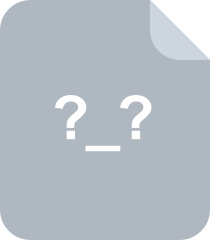
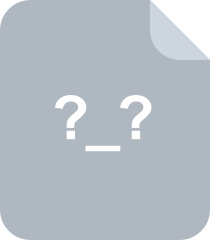
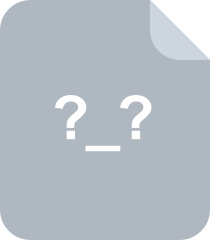
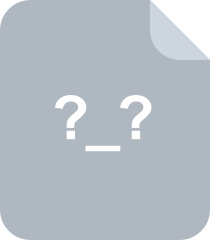
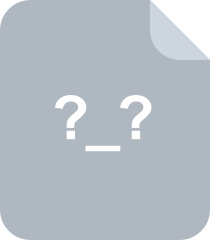
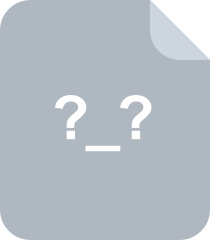
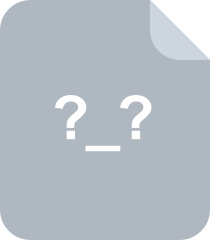
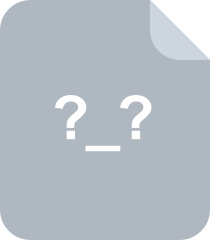
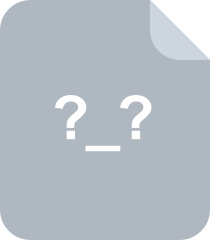
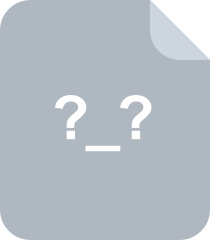
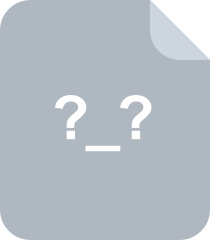
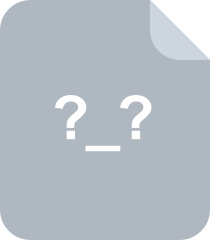
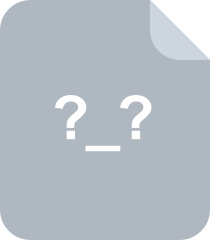
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
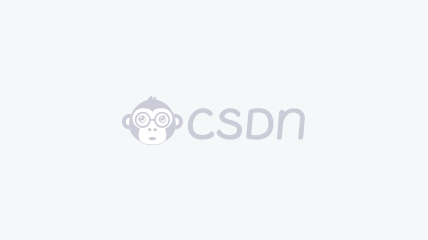
- 菜鸟北极雪2024-11-29资源很赞,希望多一些这类资源。
- 2301_774807192024-11-09超级好的资源,很值得参考学习,对我启发很大,支持!

我不是程序员~~~~
- 粉丝: 9w+
- 资源: 209
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

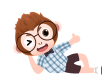
最新资源
- 交互设计中响应式页面的HTML、CSS和jQuery编码实践-可实现的-有问题请联系博主,博主会第一时间回复!!!
- pathlib-1.0.1-py3-none-any.whl
- 2drfgvdgvfhb
- 新英格兰电力系统风力发电集成研究及其对成本的影响分析-可实现的-有问题请联系博主,博主会第一时间回复!!!
- 电力电子工程中单相和三相AC/DC/AC电源转换系统的Simulink模型设计-可实现的-有问题请联系博主,博主会第一时间回复!!!
- colorit-0.1.0-py2.py3-none-any.whl
- 数据分析应用大作业模板.docx
- 电子电路计算机辅助设计(EIE440): OrCAD与PSpice的课程介绍及应用-可实现的-有问题请联系博主,博主会第一时间回复!!!
- 模拟天气数据.xlsx
- yarn-1.22.22.msi
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


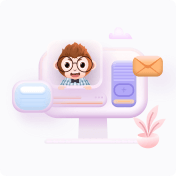
安全验证
文档复制为VIP权益,开通VIP直接复制
